STM32L4R9I_EVAL BSP User Manual
|
stm32l4r9i_eval_dsi_lcd.h
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l4r9i_eval_dsi_lcd.h 00004 * @author MCD Application Team 00005 * @brief This file contains the common defines and functions prototypes for 00006 * the stm32l4r9i_eval_dsi_lcd.c driver. 00007 ****************************************************************************** 00008 * @attention 00009 * 00010 * <h2><center>© COPYRIGHT(c) 2017 STMicroelectronics</center></h2> 00011 * 00012 * Redistribution and use in source and binary forms, with or without modification, 00013 * are permitted provided that the following conditions are met: 00014 * 1. Redistributions of source code must retain the above copyright notice, 00015 * this list of conditions and the following disclaimer. 00016 * 2. Redistributions in binary form must reproduce the above copyright notice, 00017 * this list of conditions and the following disclaimer in the documentation 00018 * and/or other materials provided with the distribution. 00019 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00020 * may be used to endorse or promote products derived from this software 00021 * without specific prior written permission. 00022 * 00023 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00024 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00025 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00026 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00027 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00028 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00029 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00030 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00031 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00032 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00033 * 00034 ****************************************************************************** 00035 */ 00036 00037 /* Define to prevent recursive inclusion -------------------------------------*/ 00038 #ifndef __STM32L4R9I_EVAL_DSI_LCD_H 00039 #define __STM32L4R9I_EVAL_DSI_LCD_H 00040 00041 #ifdef __cplusplus 00042 extern "C" { 00043 #endif 00044 00045 /* Includes ------------------------------------------------------------------*/ 00046 /* Include LCD component Driver */ 00047 00048 #include "stm32l4r9i_eval.h" 00049 00050 /** @addtogroup BSP 00051 * @{ 00052 */ 00053 00054 /** @addtogroup STM32L4R9I_EVAL 00055 * @{ 00056 */ 00057 00058 /** @defgroup STM32L4R9I_EVAL_LCD STM32L4R9I_EVAL LCD 00059 * @{ 00060 */ 00061 00062 /** @defgroup STM32L4R9I_EVAL_DSI_LCD STM32L4R9I_EVAL DSI LCD 00063 * @{ 00064 */ 00065 00066 /** @addtogroup STM32L4R9I_EVAL_DSI_LCD_Exported_Variables 00067 * @{ 00068 */ 00069 00070 /* DMA2D handle variable */ 00071 extern DMA2D_HandleTypeDef hdma2d_eval; 00072 00073 /** 00074 * @} 00075 */ 00076 00077 /** @addtogroup STM32L4R9I_EVAL_DSI_LCD_Exported_Functions 00078 * @{ 00079 */ 00080 00081 uint8_t BSP_DSI_LCD_Init(void); 00082 uint8_t BSP_DSI_LCD_DeInit(void); 00083 00084 uint32_t BSP_DSI_LCD_GetXSize(void); 00085 uint32_t BSP_DSI_LCD_GetYSize(void); 00086 00087 uint8_t BSP_DSI_LCD_SetTransparency(uint32_t LayerIndex, uint8_t Transparency); 00088 uint8_t BSP_DSI_LCD_SetColorKeying(uint32_t LayerIndex, uint32_t RGBValue); 00089 uint8_t BSP_DSI_LCD_ResetColorKeying(uint32_t LayerIndex); 00090 00091 uint8_t BSP_DSI_LCD_SelectLayer(uint32_t LayerIndex); 00092 uint8_t BSP_DSI_LCD_SetLayerVisible(uint32_t LayerIndex, FunctionalState State); 00093 00094 void BSP_DSI_LCD_SetTextColor(uint32_t Color); 00095 uint32_t BSP_DSI_LCD_GetTextColor(void); 00096 void BSP_DSI_LCD_SetBackColor(uint32_t Color); 00097 uint32_t BSP_DSI_LCD_GetBackColor(void); 00098 void BSP_DSI_LCD_SetFont(sFONT *fonts); 00099 sFONT *BSP_DSI_LCD_GetFont(void); 00100 00101 uint32_t BSP_DSI_LCD_ReadPixel(uint16_t Xpos, uint16_t Ypos); 00102 void BSP_DSI_LCD_DrawPixel(uint16_t Xpos, uint16_t Ypos, uint32_t pixel); 00103 void BSP_DSI_LCD_Clear(uint32_t Color); 00104 void BSP_DSI_LCD_ClearStringLine(uint32_t Line); 00105 void BSP_DSI_LCD_DisplayStringAtLine(uint16_t Line, uint8_t *ptr); 00106 void BSP_DSI_LCD_DisplayStringAt(uint16_t Xpos, uint16_t Ypos, uint8_t *Text, Text_AlignModeTypdef Mode); 00107 void BSP_DSI_LCD_DisplayChar(uint16_t Xpos, uint16_t Ypos, uint8_t Ascii); 00108 00109 void BSP_DSI_LCD_DrawHLine(uint16_t Xpos, uint16_t Ypos, uint16_t Length); 00110 void BSP_DSI_LCD_DrawVLine(uint16_t Xpos, uint16_t Ypos, uint16_t Length); 00111 void BSP_DSI_LCD_DrawLine(uint16_t x1, uint16_t y1, uint16_t x2, uint16_t y2); 00112 void BSP_DSI_LCD_DrawRect(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height); 00113 void BSP_DSI_LCD_DrawCircle(uint16_t Xpos, uint16_t Ypos, uint16_t Radius); 00114 void BSP_DSI_LCD_DrawPolygon(pPoint Points, uint16_t PointCount); 00115 void BSP_DSI_LCD_DrawEllipse(int Xpos, int Ypos, int XRadius, int YRadius); 00116 void BSP_DSI_LCD_DrawBitmap(uint32_t Xpos, uint32_t Ypos, uint8_t *pbmp); 00117 00118 void BSP_DSI_LCD_FillRect(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height); 00119 void BSP_DSI_LCD_FillCircle(uint16_t Xpos, uint16_t Ypos, uint16_t Radius); 00120 void BSP_DSI_LCD_FillPolygon(pPoint Points, uint16_t PointCount); 00121 void BSP_DSI_LCD_FillEllipse(int Xpos, int Ypos, int XRadius, int YRadius); 00122 00123 void BSP_DSI_LCD_DisplayOff(void); 00124 void BSP_DSI_LCD_DisplayOn(void); 00125 00126 void BSP_DSI_LCD_Refresh(void); 00127 uint8_t BSP_DSI_LCD_IsFrameBufferAvailable(void); 00128 00129 void BSP_DSI_LCD_SetBrightness(uint8_t BrightnessValue); 00130 00131 /* These __weak functions can be surcharged by application code for specific application needs */ 00132 void BSP_DSI_LCD_MspInit(void); 00133 void BSP_DSI_LCD_MspDeInit(void); 00134 void BSP_DSI_LCD_DMA2D_IRQHandler(void); 00135 void BSP_DSI_LCD_DSI_IRQHandler(void); 00136 void BSP_DSI_LCD_LTDC_IRQHandler(void); 00137 void BSP_DSI_LCD_LTDC_ER_IRQHandler(void); 00138 00139 /** 00140 * @} 00141 */ 00142 00143 /** 00144 * @} 00145 */ 00146 00147 /** 00148 * @} 00149 */ 00150 00151 /** 00152 * @} 00153 */ 00154 00155 /** 00156 * @} 00157 */ 00158 00159 #ifdef __cplusplus 00160 } 00161 #endif 00162 00163 #endif /* __STM32L4R9I_EVAL_DSI_LCD_H */ 00164 00165 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Thu Oct 12 2017 10:53:59 for STM32L4R9I_EVAL BSP User Manual by
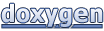