STM32L4R9I_EVAL BSP User Manual
|
stm32l4r9i_eval.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l4r9i_eval.c 00004 * @author MCD Application Team 00005 * @brief This file provides a set of firmware functions to manage Leds, 00006 * push-button and COM ports for STM32L4R9I_EVAL 00007 ****************************************************************************** 00008 * @attention 00009 * 00010 * <h2><center>© COPYRIGHT(c) 2017 STMicroelectronics</center></h2> 00011 * 00012 * Redistribution and use in source and binary forms, with or without modification, 00013 * are permitted provided that the following conditions are met: 00014 * 1. Redistributions of source code must retain the above copyright notice, 00015 * this list of conditions and the following disclaimer. 00016 * 2. Redistributions in binary form must reproduce the above copyright notice, 00017 * this list of conditions and the following disclaimer in the documentation 00018 * and/or other materials provided with the distribution. 00019 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00020 * may be used to endorse or promote products derived from this software 00021 * without specific prior written permission. 00022 * 00023 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00024 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00025 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00026 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00027 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00028 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00029 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00030 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00031 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00032 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00033 * 00034 ****************************************************************************** 00035 */ 00036 00037 /* Includes ------------------------------------------------------------------*/ 00038 #include "stm32l4r9i_eval.h" 00039 #include "stm32l4r9i_eval_io.h" 00040 00041 /** @addtogroup BSP 00042 * @{ 00043 */ 00044 00045 /** @defgroup STM32L4R9I_EVAL STM32L4R9I_EVAL 00046 * @{ 00047 */ 00048 00049 /** @defgroup STM32L4R9I_EVAL_Common STM32L4R9I_EVAL Common 00050 * @{ 00051 */ 00052 00053 /** @defgroup STM32L4R9I_EVAL_Private_TypesDefinitions Private Types Definitions 00054 * @brief This file provides firmware functions to manage Leds, push-buttons, 00055 * Joystick, and COM ports available on STM32L4R9I-EVAL evaluation board 00056 * from STMicroelectronics. 00057 * @{ 00058 */ 00059 00060 /** 00061 * @} 00062 */ 00063 00064 /** @defgroup STM32L4R9I_EVAL_Exported_Defines Exported Defines 00065 * @{ 00066 */ 00067 00068 /** 00069 * @brief STM32L4R9I EVAL BSP Driver version number 00070 */ 00071 #define __STM32L4R9I_EVAL_BSP_VERSION_MAIN (0x01) /*!< [31:24] main version */ 00072 #define __STM32L4R9I_EVAL_BSP_VERSION_SUB1 (0x00) /*!< [23:16] sub1 version */ 00073 #define __STM32L4R9I_EVAL_BSP_VERSION_SUB2 (0x00) /*!< [15:8] sub2 version */ 00074 #define __STM32L4R9I_EVAL_BSP_VERSION_RC (0x00) /*!< [7:0] release candidate */ 00075 #define __STM32L4R9I_EVAL_BSP_VERSION ((__STM32L4R9I_EVAL_BSP_VERSION_MAIN << 24)\ 00076 |(__STM32L4R9I_EVAL_BSP_VERSION_SUB1 << 16)\ 00077 |(__STM32L4R9I_EVAL_BSP_VERSION_SUB2 << 8 )\ 00078 |(__STM32L4R9I_EVAL_BSP_VERSION_RC)) 00079 00080 #ifdef HAL_I2C_MODULE_ENABLED 00081 /** 00082 * @brief BSP I2C users 00083 */ 00084 #define BSP_I2C_NO_USER 0x00000000U 00085 #define BSP_I2C_AUDIO_USER 0x00000001U 00086 #define BSP_I2C_TS_USER 0x00000002U 00087 #define BSP_I2C_MFX_USER 0x00000004U 00088 #define BSP_I2C_EEPROM_USER 0x00000008U 00089 #define BSP_I2C_ALL_USERS 0x0000000FU 00090 #endif 00091 00092 /** 00093 * @} 00094 */ 00095 00096 00097 /** @defgroup STM32L4R9I_EVAL_Exported_Variables Exported Variables 00098 * @{ 00099 */ 00100 00101 /** 00102 * @brief LED variables 00103 */ 00104 GPIO_TypeDef* LED_PORT[LEDn] = {LED1_GPIO_PORT, 00105 LED2_GPIO_PORT, 00106 LED3_GPIO_PORT, 00107 LED4_GPIO_PORT}; 00108 00109 const uint16_t LED_PIN[LEDn] = {LED1_PIN, 00110 LED2_PIN, 00111 LED3_PIN, 00112 LED4_PIN}; 00113 00114 /** 00115 * @brief BUTTON variables 00116 */ 00117 GPIO_TypeDef* BUTTON_PORT[BUTTONn] = {WAKEUP_BUTTON_GPIO_PORT, 00118 (GPIO_TypeDef *)0xFFFF, 00119 (GPIO_TypeDef *)0xFFFF, 00120 (GPIO_TypeDef *)0xFFFF, 00121 (GPIO_TypeDef *)0xFFFF, 00122 (GPIO_TypeDef *)0xFFFF}; 00123 /* 0xFFFF to mark as invalid port (Joystick buttons accessed thru IOExpander) */ 00124 00125 const uint16_t BUTTON_PIN[BUTTONn] = {WAKEUP_BUTTON_PIN, 00126 SEL_JOY_PIN, 00127 LEFT_JOY_PIN, 00128 RIGHT_JOY_PIN, 00129 DOWN_JOY_PIN, 00130 UP_JOY_PIN}; 00131 00132 const uint8_t BUTTON_IRQn[BUTTONn] = {WAKEUP_BUTTON_EXTI_IRQn, 00133 SEL_JOY_EXTI_IRQn, 00134 LEFT_JOY_EXTI_IRQn, 00135 RIGHT_JOY_EXTI_IRQn, 00136 DOWN_JOY_EXTI_IRQn, 00137 UP_JOY_EXTI_IRQn}; 00138 00139 /** 00140 * @brief JOYSTICK variables 00141 */ 00142 00143 const uint16_t JOY_PIN[JOYn] = {SEL_JOY_PIN, 00144 LEFT_JOY_PIN, 00145 RIGHT_JOY_PIN, 00146 DOWN_JOY_PIN, 00147 UP_JOY_PIN}; 00148 00149 const uint8_t JOY_IRQn[JOYn] = {SEL_JOY_EXTI_IRQn, 00150 LEFT_JOY_EXTI_IRQn, 00151 RIGHT_JOY_EXTI_IRQn, 00152 DOWN_JOY_EXTI_IRQn, 00153 UP_JOY_EXTI_IRQn}; 00154 00155 /** 00156 * @brief COM variables 00157 */ 00158 USART_TypeDef* COM_USART[COMn] = {EVAL_COM1}; 00159 00160 GPIO_TypeDef* COM_TX_PORT[COMn] = {EVAL_COM1_TX_GPIO_PORT}; 00161 00162 GPIO_TypeDef* COM_RX_PORT[COMn] = {EVAL_COM1_RX_GPIO_PORT}; 00163 00164 const uint16_t COM_TX_PIN[COMn] = {EVAL_COM1_TX_PIN}; 00165 00166 const uint16_t COM_RX_PIN[COMn] = {EVAL_COM1_RX_PIN}; 00167 00168 const uint16_t COM_TX_AF[COMn] = {EVAL_COM1_TX_AF}; 00169 00170 const uint16_t COM_RX_AF[COMn] = {EVAL_COM1_RX_AF}; 00171 00172 /** 00173 * @brief BUS variables 00174 */ 00175 00176 #ifdef HAL_I2C_MODULE_ENABLED 00177 uint32_t hi2c_evalTimeout = EVAL_I2Cx_TIMEOUT_MAX; /*<! Value of Timeout when I2C communication fails */ 00178 I2C_HandleTypeDef hi2c_eval; 00179 static uint32_t v_bspI2cUsers = BSP_I2C_NO_USER; 00180 #if defined(BSP_USE_CMSIS_OS) 00181 static osSemaphoreId BspI2cSemaphore; 00182 #endif 00183 #endif /* HAL_I2C_MODULE_ENABLED */ 00184 00185 #ifdef HAL_ADC_MODULE_ENABLED 00186 static ADC_HandleTypeDef hEvalADC = {0}; 00187 #endif 00188 00189 /** 00190 * @} 00191 */ 00192 00193 /** @defgroup STM32L4R9I_EVAL_Private_FunctionPrototypes Private Functions 00194 * @{ 00195 */ 00196 /* I2Cx bus function */ 00197 #ifdef HAL_I2C_MODULE_ENABLED 00198 /* Link function I2C for EEPROM, Audio Codec peripheral and IO expanders*/ 00199 static void I2Cx_Init(uint32_t user); 00200 static void I2Cx_MspInit(I2C_HandleTypeDef *hi2c); 00201 static void I2Cx_DeInit(uint32_t user); 00202 static void I2Cx_MspDeInit(I2C_HandleTypeDef *hi2c); 00203 static HAL_StatusTypeDef I2Cx_WriteBuffer(uint16_t Addr, uint16_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length); 00204 static HAL_StatusTypeDef I2Cx_ReadBuffer(uint16_t Addr, uint16_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length); 00205 static HAL_StatusTypeDef I2Cx_IsDeviceReady(uint16_t DevAddress, uint32_t Trials); 00206 static void I2Cx_Error (void); 00207 00208 /* Link function I2C for IO expanders */ 00209 static void I2Cx_WriteData(uint16_t Addr, uint16_t Reg, uint16_t RegSize, uint8_t Value); 00210 static void I2Cx_WriteMultiple(uint16_t Addr, uint16_t Reg, uint16_t MemAddress, uint8_t *Buffer, uint16_t Length); 00211 static uint8_t I2Cx_ReadData(uint16_t Addr, uint16_t Reg, uint16_t RegSize); 00212 static uint8_t I2Cx_ReadMultiple(uint16_t Addr, uint16_t Reg, uint16_t MemAddress, uint8_t *Buffer, uint16_t Length); 00213 00214 /* Link function for IOExpander IO functions */ 00215 void MFX_IO_Init(void); 00216 void MFX_IO_DeInit(void); 00217 void MFX_IO_Wakeup(void); 00218 void MFX_IO_EnableWakeupPin(void); 00219 void MFX_IO_ITConfig(void); 00220 void MFX_IO_Delay(uint32_t Delay); 00221 void MFX_IO_Write(uint16_t Addr, uint8_t Reg, uint8_t Value); 00222 uint8_t MFX_IO_Read(uint16_t Addr, uint8_t Reg); 00223 uint16_t MFX_IO_ReadMultiple(uint16_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length); 00224 00225 /* Link function for TOUCHSCREEN IO functions */ 00226 void TS_IO_Init(void); 00227 void TS_IO_Write(uint8_t Addr, uint8_t Reg, uint8_t Value); 00228 uint8_t TS_IO_Read(uint8_t Addr, uint8_t Reg); 00229 uint16_t TS_IO_ReadMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length); 00230 void TS_IO_Delay(uint32_t Delay); 00231 00232 /* Link function for EEPROM peripheral over I2C */ 00233 void EEPROM_IO_Init(void); 00234 HAL_StatusTypeDef EEPROM_IO_WriteData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize); 00235 HAL_StatusTypeDef EEPROM_IO_ReadData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize); 00236 HAL_StatusTypeDef EEPROM_IO_IsDeviceReady(uint16_t DevAddress, uint32_t Trials); 00237 00238 /* Link function for Audio Codec peripheral */ 00239 void AUDIO_IO_Init(void); 00240 void AUDIO_IO_DeInit(void); 00241 void AUDIO_IO_Write(uint8_t Addr, uint16_t Reg, uint16_t Value); 00242 uint16_t AUDIO_IO_Read(uint8_t Addr, uint16_t Reg); 00243 void AUDIO_IO_Delay(uint32_t delay); 00244 00245 #endif/* HAL_I2C_MODULE_ENABLED */ 00246 00247 /** 00248 * @} 00249 */ 00250 00251 /** @defgroup STM32L4R9I_EVAL_Exported_Functions Exported Functions 00252 * @{ 00253 */ 00254 00255 /** 00256 * @brief Error Handler 00257 * @note Defined as a weak function to be overwritten by the application. 00258 * @retval None 00259 */ 00260 __weak void BSP_ErrorHandler(void) 00261 { 00262 while(1); 00263 } 00264 00265 /** 00266 * @brief This method returns the STM32L4R9I EVAL BSP Driver revision 00267 * @retval version : 0xXYZR (8bits for each decimal, R for RC) 00268 */ 00269 uint32_t BSP_GetVersion(void) 00270 { 00271 return __STM32L4R9I_EVAL_BSP_VERSION; 00272 } 00273 00274 /** 00275 * @brief Initialize LED GPIO. 00276 * @param Led: Specifies the Led to be configured. 00277 * This parameter can be one of following parameters: 00278 * @arg LED1 00279 * @arg LED2 00280 * @arg LED3 00281 * @arg LED4 00282 * @retval None 00283 */ 00284 void BSP_LED_Init(Led_TypeDef Led) 00285 { 00286 GPIO_InitTypeDef gpioinitstruct = {0}; 00287 00288 /* Enable the GPIO_LED clock */ 00289 LEDx_GPIO_CLK_ENABLE(Led); 00290 00291 /* Configure the GPIO_LED pin */ 00292 gpioinitstruct.Pin = LED_PIN[Led]; 00293 gpioinitstruct.Mode = GPIO_MODE_OUTPUT_PP; 00294 gpioinitstruct.Pull = GPIO_PULLUP; 00295 gpioinitstruct.Speed = GPIO_SPEED_FREQ_VERY_HIGH; 00296 00297 HAL_GPIO_Init(LED_PORT[Led], &gpioinitstruct); 00298 00299 /* By default, turn off LED */ 00300 HAL_GPIO_WritePin(LED_PORT[Led], LED_PIN[Led], GPIO_PIN_SET); 00301 } 00302 00303 /** 00304 * @brief DeInitialize LED GPIO. 00305 * @param Led: Specifies the Led to be configured. 00306 * This parameter can be one of following parameters: 00307 * @arg LED1 00308 * @arg LED2 00309 * @arg LED3 00310 * @arg LED4 00311 * @retval None 00312 */ 00313 void BSP_LED_DeInit(Led_TypeDef Led) 00314 { 00315 /* Enable the GPIO_LED clock */ 00316 LEDx_GPIO_CLK_ENABLE(Led); 00317 00318 HAL_GPIO_DeInit(LED_PORT[Led], LED_PIN[Led]); 00319 } 00320 00321 /** 00322 * @brief Turn selected LED On. 00323 * @param Led: Specifies the Led to be set on. 00324 * This parameter can be one of following parameters: 00325 * @arg LED1 00326 * @arg LED2 00327 * @arg LED3 00328 * @arg LED4 00329 * @retval None 00330 */ 00331 void BSP_LED_On(Led_TypeDef Led) 00332 { 00333 HAL_GPIO_WritePin(LED_PORT[Led], LED_PIN[Led], GPIO_PIN_RESET); 00334 } 00335 00336 /** 00337 * @brief Turn selected LED Off. 00338 * @param Led: Specifies the Led to be set off. 00339 * This parameter can be one of following parameters: 00340 * @arg LED1 00341 * @arg LED2 00342 * @arg LED3 00343 * @arg LED4 00344 * @retval None 00345 */ 00346 void BSP_LED_Off(Led_TypeDef Led) 00347 { 00348 HAL_GPIO_WritePin(LED_PORT[Led], LED_PIN[Led], GPIO_PIN_SET); 00349 } 00350 00351 /** 00352 * @brief Toggle the selected LED. 00353 * @param Led: Specifies the Led to be toggled. 00354 * This parameter can be one of following parameters: 00355 * @arg LED1 00356 * @arg LED2 00357 * @arg LED3 00358 * @arg LED4 00359 * @retval None 00360 */ 00361 void BSP_LED_Toggle(Led_TypeDef Led) 00362 { 00363 HAL_GPIO_TogglePin(LED_PORT[Led], LED_PIN[Led]); 00364 } 00365 00366 /** 00367 * @brief Initialize push button GPIO and EXTI Line. 00368 * @param Button: Button to be configured. 00369 * This parameter can be one of the following values: 00370 * @arg BUTTON_WAKEUP: Wakeup/Tamper Push Button 00371 * @param ButtonMode: Button mode requested. 00372 * This parameter can be one of the following values: 00373 * @arg BUTTON_MODE_GPIO: Button will be used as simple IO 00374 * @arg BUTTON_MODE_EXTI: Button will be connected to EXTI line 00375 * with interrupt generation capability 00376 * @retval None 00377 */ 00378 void BSP_PB_Init(Button_TypeDef Button, ButtonMode_TypeDef ButtonMode) 00379 { 00380 GPIO_InitTypeDef gpioinitstruct = {0}; 00381 00382 if (Button == BUTTON_WAKEUP) 00383 { 00384 /* Enable the corresponding Push Button clock */ 00385 BUTTONx_GPIO_CLK_ENABLE(Button); 00386 00387 /* Configure Push Button pin as input */ 00388 gpioinitstruct.Pin = BUTTON_PIN[Button]; 00389 gpioinitstruct.Pull = GPIO_PULLDOWN; 00390 gpioinitstruct.Speed = GPIO_SPEED_FREQ_VERY_HIGH; 00391 00392 if (ButtonMode == BUTTON_MODE_GPIO) 00393 { 00394 /* Configure Button pin as input */ 00395 gpioinitstruct.Mode = GPIO_MODE_INPUT; 00396 HAL_GPIO_Init(BUTTON_PORT[Button], &gpioinitstruct); 00397 } 00398 else 00399 { 00400 /* Configure Tamper/Wake-up Push Button pin as input with External interrupt, rising edge */ 00401 gpioinitstruct.Mode = GPIO_MODE_IT_RISING; 00402 00403 HAL_GPIO_Init(BUTTON_PORT[Button], &gpioinitstruct); 00404 00405 /* Enable and set Button EXTI Interrupt to the lowest priority */ 00406 HAL_NVIC_SetPriority((IRQn_Type)(BUTTON_IRQn[Button]), 0x0F, 0); 00407 HAL_NVIC_EnableIRQ((IRQn_Type)(BUTTON_IRQn[Button])); 00408 } 00409 } 00410 } 00411 00412 /** 00413 * @brief DeInitialize push button. 00414 * @param Button: Button to be deinitialized. 00415 * This parameter can be one of the following values: 00416 * @arg BUTTON_WAKEUP: Wakeup/Tamper Push Button 00417 * @retval None 00418 */ 00419 void BSP_PB_DeInit(Button_TypeDef Button) 00420 { 00421 if (Button == BUTTON_WAKEUP) 00422 { 00423 /* Enable the corresponding Push Button clock */ 00424 BUTTONx_GPIO_CLK_ENABLE(Button); 00425 00426 HAL_GPIO_DeInit(BUTTON_PORT[Button], BUTTON_PIN[Button]); 00427 00428 /* Make sure Button EXTI Interrupt is disabled */ 00429 HAL_NVIC_DisableIRQ((IRQn_Type)(BUTTON_IRQn[Button])); 00430 } 00431 } 00432 00433 /** 00434 * @brief Return the selected button state. 00435 * @param Button: Button to be checked. 00436 * This parameter can be one of the following values: 00437 * @arg BUTTON_WAKEUP: Wakeup/Tamper Push Button 00438 * @retval Button state 00439 */ 00440 uint32_t BSP_PB_GetState(Button_TypeDef Button) 00441 { 00442 return HAL_GPIO_ReadPin(BUTTON_PORT[Button], BUTTON_PIN[Button]); 00443 } 00444 00445 /** 00446 * @brief Initialize all buttons of the joystick in GPIO or EXTI modes. 00447 * @param JoyMode: Joystick mode. 00448 * This parameter can be one of the following values: 00449 * @arg JOY_MODE_GPIO: Joystick pins will be used as simple IOs 00450 * @arg JOY_MODE_EXTI: Joystick pins will be connected to EXTI line 00451 * with interrupt generation capability 00452 * @retval HAL_OK: if all initializations are OK. Other value if error. 00453 */ 00454 uint8_t BSP_JOY_Init(JOYMode_TypeDef JoyMode) 00455 { 00456 if (BSP_IO_Init() == IO_ERROR) 00457 { 00458 BSP_ErrorHandler(); 00459 } 00460 00461 /* Configure joystick pins in IT mode */ 00462 if(JoyMode == JOY_MODE_EXTI) 00463 { 00464 BSP_IO_ConfigPin(JOY_ALL_PINS, IO_MODE_IT_LOW_LEVEL_PU); 00465 00466 /* Configure IO Expander interrupt */ 00467 MFX_IO_ITConfig(); 00468 } 00469 else 00470 { 00471 /* GPIO mode configuration */ 00472 BSP_IO_ConfigPin(JOY_ALL_PINS,IO_MODE_INPUT_PU); 00473 } 00474 00475 return HAL_OK; 00476 } 00477 00478 /** 00479 * @brief DeInitialize all buttons of the joystick. 00480 * @retval None 00481 */ 00482 void BSP_JOY_DeInit(void) 00483 { 00484 BSP_IO_ConfigPin(JOY_ALL_PINS, IO_MODE_OFF); 00485 } 00486 00487 /** 00488 * @brief Return the current joystick status. 00489 * @retval Code of the joystick key pressed 00490 * This code can be one of the following values: 00491 * @arg JOY_NONE 00492 * @arg JOY_SEL 00493 * @arg JOY_DOWN 00494 * @arg JOY_LEFT 00495 * @arg JOY_RIGHT 00496 * @arg JOY_UP 00497 */ 00498 JOYState_TypeDef BSP_JOY_GetState(void) 00499 { 00500 uint32_t tmp = 0; 00501 00502 /* Read the status of all the joystick pins */ 00503 tmp = BSP_IO_ReadPin(JOY_ALL_PINS); 00504 00505 /* Check the pressed joystick button */ 00506 if(!(tmp & SEL_JOY_PIN)) 00507 { 00508 return(JOYState_TypeDef) JOY_SEL; 00509 } 00510 else if(!(tmp & DOWN_JOY_PIN)) 00511 { 00512 return(JOYState_TypeDef) JOY_DOWN; 00513 } 00514 else if(!(tmp & LEFT_JOY_PIN)) 00515 { 00516 return(JOYState_TypeDef) JOY_LEFT; 00517 } 00518 else if(!(tmp & RIGHT_JOY_PIN)) 00519 { 00520 return(JOYState_TypeDef) JOY_RIGHT; 00521 } 00522 else if(!(tmp & UP_JOY_PIN)) 00523 { 00524 return(JOYState_TypeDef) JOY_UP; 00525 } 00526 else 00527 { 00528 return(JOYState_TypeDef) JOY_NONE; 00529 } 00530 } 00531 00532 #ifdef HAL_UART_MODULE_ENABLED 00533 /** 00534 * @brief Initialize COM port. 00535 * @param COM: Specifies the COM port to be configured. 00536 * This parameter can be one of following parameters: 00537 * @arg COM1 00538 * @param huart: pointer to a UART_HandleTypeDef structure that 00539 * contains the configuration information for the specified UART peripheral. 00540 * @retval None 00541 */ 00542 void BSP_COM_Init(COM_TypeDef COM, UART_HandleTypeDef* huart) 00543 { 00544 GPIO_InitTypeDef gpioinitstruct = {0}; 00545 00546 /* Enable GPIO clock */ 00547 COMx_TX_GPIO_CLK_ENABLE(COM); 00548 COMx_RX_GPIO_CLK_ENABLE(COM); 00549 00550 /* Enable USART clock */ 00551 COMx_CLK_ENABLE(COM); 00552 00553 /* IOSV bit MUST be set to access GPIO port G[2:15] */ 00554 __HAL_RCC_PWR_CLK_ENABLE(); 00555 HAL_PWREx_EnableVddIO2(); 00556 00557 /* Configure USART Tx as alternate function push-pull */ 00558 gpioinitstruct.Pin = COM_TX_PIN[COM]; 00559 gpioinitstruct.Mode = GPIO_MODE_AF_PP; 00560 gpioinitstruct.Speed = GPIO_SPEED_FREQ_VERY_HIGH; 00561 gpioinitstruct.Pull = GPIO_PULLUP; 00562 gpioinitstruct.Alternate = COM_TX_AF[COM]; 00563 HAL_GPIO_Init(COM_TX_PORT[COM], &gpioinitstruct); 00564 00565 /* Configure USART Rx as alternate function push-pull */ 00566 gpioinitstruct.Pin = COM_RX_PIN[COM]; 00567 gpioinitstruct.Alternate = COM_RX_AF[COM]; 00568 HAL_GPIO_Init(COM_RX_PORT[COM], &gpioinitstruct); 00569 00570 /* USART configuration */ 00571 huart->Instance = COM_USART[COM]; 00572 HAL_UART_Init(huart); 00573 } 00574 00575 /** 00576 * @brief DeInitialize COM port. 00577 * @param COM: Specifies the COM port to be deinitialized. 00578 * This parameter can be one of following parameters: 00579 * @arg COM1 00580 * @param huart: pointer to a UART_HandleTypeDef structure that 00581 * contains the configuration information for the specified UART peripheral. 00582 * @retval None 00583 */ 00584 void BSP_COM_DeInit(COM_TypeDef COM, UART_HandleTypeDef* huart) 00585 { 00586 /* USART configuration */ 00587 huart->Instance = COM_USART[COM]; 00588 HAL_UART_DeInit(huart); 00589 } 00590 #endif /* HAL_UART_MODULE_ENABLED */ 00591 00592 00593 #ifdef HAL_ADC_MODULE_ENABLED 00594 /** 00595 * @brief Init the potentioneter RV2 measurement thanks to ADC. 00596 * Limitations : the potentiometer are exclusive with MFX, Audio codex, 00597 * OctoSPIP1, Debugging connector and MC operation. And they are mutually exclusive. 00598 * Please deinit all these components before usage. 00599 * @retval BSP_POTENTIOMETER_OK if no error 00600 */ 00601 uint32_t BSP_POTENTIOMETER_Init(void) 00602 { 00603 GPIO_InitTypeDef GPIO_InitStruct; 00604 ADC_ChannelConfTypeDef sConfig; 00605 00606 /* ADC clock enable */ 00607 ADCx_CLK_ENABLE(); 00608 /* ADC an GPIO Periph clock enable */ 00609 ADCx_CHANNEL_GPIO_CLK_ENABLE(); 00610 /* ADC Periph interface clock configuration */ 00611 __HAL_RCC_ADC_CONFIG(RCC_ADCCLKSOURCE_SYSCLK); 00612 00613 /* ADC Channel GPIO pin configuration */ 00614 GPIO_InitStruct.Pin = ADCx_CHANNEL_PIN; 00615 GPIO_InitStruct.Mode = GPIO_MODE_ANALOG; 00616 GPIO_InitStruct.Pull = GPIO_NOPULL; 00617 00618 HAL_GPIO_Init(ADCx_CHANNEL_GPIO_PORT, &GPIO_InitStruct); 00619 00620 /*##-1- Configure the ADC peripheral #######################################*/ 00621 hEvalADC.Instance = ADCx; 00622 00623 if (HAL_ADC_DeInit(&hEvalADC)) return BSP_POTENTIOMETER_ERROR; 00624 00625 hEvalADC.Init.ClockPrescaler = ADC_CLOCK_SYNC_PCLK_DIV2; /* Synchronous clock mode, input ADC clock not divided */ 00626 hEvalADC.Init.Resolution = ADC_RESOLUTION_12B; /* 12-bit resolution for converted data */ 00627 hEvalADC.Init.DataAlign = ADC_DATAALIGN_RIGHT; /* Right-alignment for converted data */ 00628 hEvalADC.Init.ScanConvMode = DISABLE; /* Sequencer disabled (ADC conversion on only 1 channel: channel set on rank 1) */ 00629 hEvalADC.Init.EOCSelection = ADC_EOC_SINGLE_CONV; /* EOC flag picked-up to indicate conversion end */ 00630 hEvalADC.Init.LowPowerAutoWait = DISABLE; /* Auto-delayed conversion feature disabled */ 00631 hEvalADC.Init.ContinuousConvMode = DISABLE; /* Continuous mode disabled to have only 1 conversion at each conversion trig */ 00632 hEvalADC.Init.NbrOfConversion = 1; /* Parameter discarded because sequencer is disabled */ 00633 hEvalADC.Init.DiscontinuousConvMode = DISABLE; /* Parameter discarded because sequencer is disabled */ 00634 hEvalADC.Init.NbrOfDiscConversion = 1; /* Parameter discarded because sequencer is disabled */ 00635 hEvalADC.Init.ExternalTrigConv = ADC_SOFTWARE_START; /* Software start to trig the 1st conversion manually, without external event */ 00636 hEvalADC.Init.ExternalTrigConvEdge = ADC_EXTERNALTRIGCONVEDGE_NONE; /* Parameter discarded because software trigger chosen */ 00637 hEvalADC.Init.DMAContinuousRequests = DISABLE; /* DMA one-shot mode selected (not applied to this example) */ 00638 hEvalADC.Init.Overrun = ADC_OVR_DATA_OVERWRITTEN; /* DR register is overwritten with the last conversion result in case of overrun */ 00639 hEvalADC.Init.OversamplingMode = DISABLE; /* No oversampling */ 00640 00641 if (HAL_ADC_Init(&hEvalADC) != HAL_OK) return BSP_POTENTIOMETER_ERROR; 00642 00643 /*##-2- Configure ADC regular channel ######################################*/ 00644 sConfig.Channel = ADCx_CHANNEL; /* Sampled channel number */ 00645 sConfig.Rank = ADC_REGULAR_RANK_1; /* Rank of sampled channel number ADCx_CHANNEL */ 00646 sConfig.SamplingTime = ADC_SAMPLETIME_6CYCLES_5; /* Sampling time (number of clock cycles unit) */ 00647 sConfig.SingleDiff = ADC_SINGLE_ENDED; /* Single-ended input channel */ 00648 sConfig.OffsetNumber = ADC_OFFSET_NONE; /* No offset subtraction */ 00649 sConfig.Offset = 0; /* Parameter discarded because offset correction is disabled */ 00650 00651 00652 if (HAL_ADC_ConfigChannel(&hEvalADC, &sConfig) != HAL_OK) return BSP_POTENTIOMETER_ERROR; 00653 00654 /* Run the ADC calibration in single-ended mode */ 00655 if (HAL_ADCEx_Calibration_Start(&hEvalADC, ADC_SINGLE_ENDED)) return BSP_POTENTIOMETER_ERROR; 00656 00657 00658 return BSP_POTENTIOMETER_OK; 00659 } 00660 00661 /** 00662 * @brief DeInitialize the potentioneter RV2 measurement thanks to ADC. 00663 * Limitations : the potentiometer are exclusive with MFX, Audio codex, 00664 * OctoSPIP1, Debugging connector and MC operation. And they are mutually exclusive. 00665 * Please deinit all these components before usage. 00666 * @retval BSP_POTENTIOMETER_OK if no error 00667 */ 00668 uint32_t BSP_POTENTIOMETER_DeInit(void) 00669 { 00670 if (HAL_ADC_DeInit(&hEvalADC) != HAL_OK) return BSP_POTENTIOMETER_ERROR; 00671 00672 return BSP_POTENTIOMETER_OK; 00673 } 00674 00675 /** 00676 * @brief Get the potentioneter RV2 measurement thanks to ADC. 00677 * Limitations : the potentiometer are exclusive with MFX, Audio codex, 00678 * OctoSPIP1, Debugging connector and MC operation. And they are mutually exclusive. 00679 * Please deinit all these components before usage. 00680 * @retval value if no error else 0xFFFFFFFF 00681 */ 00682 uint32_t BSP_POTENTIOMETER_GetLevel(void) 00683 { 00684 /*##-3- Start the conversion process #######################################*/ 00685 if (HAL_ADC_Start(&hEvalADC) != HAL_OK) return BSP_POTENTIOMETER_ERROR; 00686 00687 if(HAL_ADC_PollForConversion(&hEvalADC, ADCx_POLL_TIMEOUT)== HAL_OK) 00688 { 00689 return (HAL_ADC_GetValue(&hEvalADC)); 00690 } 00691 else 00692 { 00693 return 0xFFFFFFFF; 00694 } 00695 } 00696 #endif /* HAL_ADC_MODULE_ENABLED */ 00697 00698 /** 00699 * @} 00700 */ 00701 00702 /** @defgroup STM32L4R9I_EVAL_BusOperations_Functions Bus Operations Functions 00703 * @{ 00704 */ 00705 00706 /******************************************************************************* 00707 BUS OPERATIONS 00708 *******************************************************************************/ 00709 #ifdef HAL_I2C_MODULE_ENABLED 00710 /******************************* I2C Routines**********************************/ 00711 00712 /** 00713 * @brief Eval I2Cx MSP Initialization 00714 * @param hi2c: I2C handle 00715 * @retval None 00716 */ 00717 static void I2Cx_MspInit(I2C_HandleTypeDef *hi2c) 00718 { 00719 GPIO_InitTypeDef gpioinitstruct = {0}; 00720 RCC_PeriphCLKInitTypeDef RCC_PeriphCLKInitStruct; 00721 00722 /* IOSV bit MUST be set to access GPIO port G[2:15] */ 00723 __HAL_RCC_PWR_CLK_ENABLE(); 00724 HAL_PWREx_EnableVddIO2(); 00725 00726 if (hi2c->Instance == EVAL_I2Cx) 00727 { 00728 /*##-1- Configure the Eval I2C clock source. The clock is derived from the SYSCLK #*/ 00729 if(EVAL_RCC_PERIPHCLK_I2C == RCC_PERIPHCLK_I2C2) 00730 { 00731 RCC_PeriphCLKInitStruct.PeriphClockSelection = RCC_PERIPHCLK_I2C2; 00732 RCC_PeriphCLKInitStruct.I2c2ClockSelection = EVAL_RCC_CLKSOURCE_I2C; 00733 } 00734 else 00735 { 00736 RCC_PeriphCLKInitStruct.PeriphClockSelection = RCC_PERIPHCLK_I2C1; 00737 RCC_PeriphCLKInitStruct.I2c1ClockSelection = EVAL_RCC_CLKSOURCE_I2C; 00738 } 00739 HAL_RCCEx_PeriphCLKConfig(&RCC_PeriphCLKInitStruct); 00740 00741 /*##-2- Configure the GPIOs ################################################*/ 00742 00743 /* Enable GPIO clock */ 00744 EVAL_I2Cx_SDA_GPIO_CLK_ENABLE(); 00745 EVAL_I2Cx_SCL_GPIO_CLK_ENABLE(); 00746 00747 /* Configure I2C Rx/Tx as alternate function */ 00748 gpioinitstruct.Pin = EVAL_I2Cx_SCL_PIN | EVAL_I2Cx_SDA_PIN; 00749 gpioinitstruct.Mode = GPIO_MODE_AF_OD; 00750 gpioinitstruct.Pull = GPIO_PULLUP; 00751 gpioinitstruct.Speed = GPIO_SPEED_FREQ_MEDIUM; // GPIO_SPEED_FREQ_VERY_HIGH; 00752 gpioinitstruct.Alternate = EVAL_I2Cx_SCL_SDA_AF; 00753 HAL_GPIO_Init(EVAL_I2Cx_SCL_GPIO_PORT, &gpioinitstruct); 00754 00755 /*##-3- Configure the Eval I2Cx peripheral #######################################*/ 00756 /* Enable Eval_I2Cx clock */ 00757 EVAL_I2Cx_CLK_ENABLE(); 00758 00759 /* Force the I2C Peripheral Clock Reset */ 00760 EVAL_I2Cx_FORCE_RESET(); 00761 00762 /* Release the I2C Peripheral Clock Reset */ 00763 EVAL_I2Cx_RELEASE_RESET(); 00764 00765 /* Enable and set Eval I2Cx Interrupt to the highest priority */ 00766 HAL_NVIC_SetPriority(EVAL_I2Cx_EV_IRQn, 0x00, 0); 00767 HAL_NVIC_EnableIRQ(EVAL_I2Cx_EV_IRQn); 00768 00769 /* Enable and set Eval I2Cx Interrupt to the highest priority */ 00770 HAL_NVIC_SetPriority(EVAL_I2Cx_ER_IRQn, 0x00, 0); 00771 HAL_NVIC_EnableIRQ(EVAL_I2Cx_ER_IRQn); 00772 } 00773 } 00774 00775 /** 00776 * @brief Eval I2Cx Bus initialization 00777 * @retval None 00778 */ 00779 static void I2Cx_Init(uint32_t user) 00780 { 00781 if(HAL_I2C_GetState(&hi2c_eval) == HAL_I2C_STATE_RESET) 00782 { 00783 #if defined(BSP_USE_CMSIS_OS) 00784 /* Create semaphore to prevent multiple I2C access */ 00785 osSemaphoreDef(BSP_I2C_SEM); 00786 BspI2cSemaphore = osSemaphoreCreate(osSemaphore(BSP_I2C_SEM), 1); 00787 #endif 00788 00789 hi2c_eval.Instance = EVAL_I2Cx; 00790 hi2c_eval.Init.Timing = EVAL_I2Cx_TIMING; 00791 hi2c_eval.Init.OwnAddress1 = 0; 00792 hi2c_eval.Init.AddressingMode = I2C_ADDRESSINGMODE_7BIT; 00793 hi2c_eval.Init.DualAddressMode = I2C_DUALADDRESS_DISABLE; 00794 hi2c_eval.Init.OwnAddress2 = 0; 00795 hi2c_eval.Init.GeneralCallMode = I2C_GENERALCALL_DISABLE; 00796 hi2c_eval.Init.NoStretchMode = I2C_NOSTRETCH_DISABLE; 00797 00798 HAL_I2C_DeInit(&hi2c_eval); 00799 /* Init the I2C */ 00800 I2Cx_MspInit(&hi2c_eval); 00801 HAL_I2C_Init(&hi2c_eval); 00802 } 00803 /* Update BSP I2C users list */ 00804 v_bspI2cUsers |= user; 00805 } 00806 00807 /** 00808 * @brief Eval I2Cx MSP Deinitialization 00809 * @param hi2c: I2C handle 00810 * @retval None 00811 */ 00812 static void I2Cx_MspDeInit(I2C_HandleTypeDef *hi2c) 00813 { 00814 } 00815 00816 /** 00817 * @brief Eval I2Cx Bus deinitialization 00818 * @retval None 00819 */ 00820 static void I2Cx_DeInit(uint32_t user) 00821 { 00822 /* Update BSP I2C users list */ 00823 v_bspI2cUsers &= ~(user); 00824 00825 if((HAL_I2C_GetState(&hi2c_eval) != HAL_I2C_STATE_RESET) && (v_bspI2cUsers == BSP_I2C_NO_USER)) 00826 { 00827 /* Deinit the I2C */ 00828 HAL_I2C_DeInit(&hi2c_eval); 00829 I2Cx_MspDeInit(&hi2c_eval); 00830 00831 #if defined(BSP_USE_CMSIS_OS) 00832 /* Delete semaphore to prevent multiple I2C access */ 00833 osSemaphoreDelete(BspI2cSemaphore); 00834 #endif 00835 } 00836 } 00837 00838 /** 00839 * @brief Write a value in a register of the device through BUS. 00840 * @param Addr: Device address on BUS Bus. 00841 * @param Reg: The target register address to write 00842 * @param RegSize: The target register size (can be 8BIT or 16BIT) 00843 * @param pBuffer: The target register value to be written 00844 * @param Length: buffer size to be written 00845 * @retval None 00846 */ 00847 static HAL_StatusTypeDef I2Cx_WriteBuffer(uint16_t Addr, uint16_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length) 00848 { 00849 HAL_StatusTypeDef status = HAL_OK; 00850 00851 #if defined(BSP_USE_CMSIS_OS) 00852 /* Get semaphore to prevent multiple I2C access */ 00853 osSemaphoreWait(BspI2cSemaphore, osWaitForever); 00854 #endif 00855 00856 status = HAL_I2C_Mem_Write(&hi2c_eval, Addr, (uint16_t)Reg, RegSize, pBuffer, Length, hi2c_evalTimeout); 00857 00858 #if defined(BSP_USE_CMSIS_OS) 00859 /* Release semaphore to prevent multiple I2C access */ 00860 osSemaphoreRelease(BspI2cSemaphore); 00861 #endif 00862 00863 /* Check the communication status */ 00864 if(status != HAL_OK) 00865 { 00866 /* Re-Initiaize the BUS */ 00867 I2Cx_Error(); 00868 } 00869 return status; 00870 } 00871 00872 /** 00873 * @brief Write a value in a register of the device through BUS. 00874 * @param Addr: Device address on BUS Bus. 00875 * @param Reg: The target register address to write 00876 * @param RegSize: The target register size (can be 8BIT or 16BIT) 00877 * @param Value: The target register value to be written 00878 * @retval None 00879 */ 00880 static void I2Cx_WriteData(uint16_t Addr, uint16_t Reg, uint16_t RegSize, uint8_t Value) 00881 { 00882 HAL_StatusTypeDef status = HAL_OK; 00883 00884 #if defined(BSP_USE_CMSIS_OS) 00885 /* Get semaphore to prevent multiple I2C access */ 00886 osSemaphoreWait(BspI2cSemaphore, osWaitForever); 00887 #endif 00888 00889 status = HAL_I2C_Mem_Write(&hi2c_eval, Addr, (uint16_t)Reg, RegSize, &Value, 1, hi2c_evalTimeout); 00890 00891 #if defined(BSP_USE_CMSIS_OS) 00892 /* Release semaphore to prevent multiple I2C access */ 00893 osSemaphoreRelease(BspI2cSemaphore); 00894 #endif 00895 00896 /* Check the communication status */ 00897 if(status != HAL_OK) 00898 { 00899 /* Re-Initiaize the BUS */ 00900 I2Cx_Error(); 00901 } 00902 } 00903 00904 /** 00905 * @brief Write multiple data value in a register of the device through BUS. 00906 * @param Addr: Device address on BUS Bus. 00907 * @param Reg: The target register address to write 00908 * @param MemAddress: Memory address 00909 * @param Buffer: The target register value to be written 00910 * @param Length: buffer size to be written 00911 * @retval None 00912 */ 00913 static void I2Cx_WriteMultiple(uint16_t Addr, uint16_t Reg, uint16_t MemAddress, uint8_t *Buffer, uint16_t Length) 00914 { 00915 HAL_StatusTypeDef status = HAL_OK; 00916 00917 #if defined(BSP_USE_CMSIS_OS) 00918 /* Get semaphore to prevent multiple I2C access */ 00919 osSemaphoreWait(BspI2cSemaphore, osWaitForever); 00920 #endif 00921 00922 status = HAL_I2C_Mem_Write(&hi2c_eval, Addr, (uint16_t)Reg, MemAddress, Buffer, Length, hi2c_evalTimeout); 00923 00924 #if defined(BSP_USE_CMSIS_OS) 00925 /* Release semaphore to prevent multiple I2C access */ 00926 osSemaphoreRelease(BspI2cSemaphore); 00927 #endif 00928 00929 /* Check the communication status */ 00930 if(status != HAL_OK) 00931 { 00932 /* Re-Initiaize the BUS */ 00933 I2Cx_Error(); 00934 } 00935 } 00936 00937 /** 00938 * @brief Read a register of the device through BUS 00939 * @param Addr: Device address on BUS 00940 * @param Reg: The target register address to read 00941 * @param RegSize: The target register size (can be 8BIT or 16BIT) 00942 * @retval read register value 00943 */ 00944 static uint8_t I2Cx_ReadData(uint16_t Addr, uint16_t Reg, uint16_t RegSize) 00945 { 00946 HAL_StatusTypeDef status = HAL_OK; 00947 uint8_t value = 0x0; 00948 00949 #if defined(BSP_USE_CMSIS_OS) 00950 /* Get semaphore to prevent multiple I2C access */ 00951 osSemaphoreWait(BspI2cSemaphore, osWaitForever); 00952 #endif 00953 00954 status = HAL_I2C_Mem_Read(&hi2c_eval, Addr, Reg, RegSize, &value, 1, hi2c_evalTimeout); 00955 00956 #if defined(BSP_USE_CMSIS_OS) 00957 /* Release semaphore to prevent multiple I2C access */ 00958 osSemaphoreRelease(BspI2cSemaphore); 00959 #endif 00960 00961 /* Check the communication status */ 00962 if(status != HAL_OK) 00963 { 00964 /* Execute user timeout callback */ 00965 I2Cx_Error(); 00966 00967 } 00968 return value; 00969 } 00970 00971 /** 00972 * @brief Read multiple data from a register of the device through BUS 00973 * @param Addr: Device address on BUS 00974 * @param Reg: The target register address to read 00975 * @param MemAddress: Memory address 00976 * @param Buffer: Pointer to data buffer 00977 * @param Length: Length of the data 00978 * @retval Number of read data 00979 */ 00980 static uint8_t I2Cx_ReadMultiple(uint16_t Addr, uint16_t Reg, uint16_t MemAddress, uint8_t *Buffer, uint16_t Length) 00981 { 00982 HAL_StatusTypeDef status = HAL_OK; 00983 uint8_t value = 0x0; 00984 00985 #if defined(BSP_USE_CMSIS_OS) 00986 /* Get semaphore to prevent multiple I2C access */ 00987 osSemaphoreWait(BspI2cSemaphore, osWaitForever); 00988 #endif 00989 00990 status = HAL_I2C_Mem_Read(&hi2c_eval, Addr, Reg, MemAddress, Buffer, Length, hi2c_evalTimeout); 00991 00992 #if defined(BSP_USE_CMSIS_OS) 00993 /* Release semaphore to prevent multiple I2C access */ 00994 osSemaphoreRelease(BspI2cSemaphore); 00995 #endif 00996 00997 /* Check the communication status */ 00998 if(status != HAL_OK) 00999 { 01000 /* Execute user timeout callback */ 01001 I2Cx_Error(); 01002 01003 } 01004 return value; 01005 } 01006 01007 /** 01008 * @brief Read multiple data on the BUS. 01009 * @param Addr: I2C Address 01010 * @param Reg: Reg Address 01011 * @param RegSize : The target register size (can be 8BIT or 16BIT) 01012 * @param pBuffer: pointer to read data buffer 01013 * @param Length: length of the data 01014 * @retval 0 if no problems to read multiple data 01015 */ 01016 static HAL_StatusTypeDef I2Cx_ReadBuffer(uint16_t Addr, uint16_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length) 01017 { 01018 HAL_StatusTypeDef status = HAL_OK; 01019 01020 #if defined(BSP_USE_CMSIS_OS) 01021 /* Get semaphore to prevent multiple I2C access */ 01022 osSemaphoreWait(BspI2cSemaphore, osWaitForever); 01023 #endif 01024 01025 status = HAL_I2C_Mem_Read(&hi2c_eval, Addr, (uint16_t)Reg, RegSize, pBuffer, Length, hi2c_evalTimeout); 01026 01027 #if defined(BSP_USE_CMSIS_OS) 01028 /* Release semaphore to prevent multiple I2C access */ 01029 osSemaphoreRelease(BspI2cSemaphore); 01030 #endif 01031 01032 /* Check the communication status */ 01033 if(status != HAL_OK) 01034 { 01035 /* Re-Initiaize the BUS */ 01036 I2Cx_Error(); 01037 } 01038 return status; 01039 } 01040 01041 /** 01042 * @brief Checks if target device is ready for communication. 01043 * @note This function is used with Memory devices 01044 * @param DevAddress: Target device address 01045 * @param Trials: Number of trials 01046 * @retval HAL status 01047 */ 01048 static HAL_StatusTypeDef I2Cx_IsDeviceReady(uint16_t DevAddress, uint32_t Trials) 01049 { 01050 HAL_StatusTypeDef status = HAL_OK; 01051 01052 #if defined(BSP_USE_CMSIS_OS) 01053 /* Get semaphore to prevent multiple I2C access */ 01054 osSemaphoreWait(BspI2cSemaphore, osWaitForever); 01055 #endif 01056 01057 status = HAL_I2C_IsDeviceReady(&hi2c_eval, DevAddress, Trials, hi2c_evalTimeout); 01058 01059 #if defined(BSP_USE_CMSIS_OS) 01060 /* Release semaphore to prevent multiple I2C access */ 01061 osSemaphoreRelease(BspI2cSemaphore); 01062 #endif 01063 01064 return status; 01065 } 01066 01067 01068 /** 01069 * @brief Eval I2Cx error treatment function 01070 * @retval None 01071 */ 01072 static void I2Cx_Error (void) 01073 { 01074 uint32_t tmpI2cUsers; 01075 01076 BSP_ErrorHandler(); 01077 01078 /* De-initialize the I2C communication BUS */ 01079 tmpI2cUsers = v_bspI2cUsers; 01080 I2Cx_DeInit(BSP_I2C_ALL_USERS); 01081 01082 /* Re- Initiaize the I2C communication BUS */ 01083 I2Cx_Init(tmpI2cUsers); 01084 } 01085 01086 /******************************************************************************* 01087 LINK OPERATIONS 01088 *******************************************************************************/ 01089 01090 /***************************** LINK MFX_IO ************************************/ 01091 01092 /** 01093 * @brief Initialize MFX_IO low level. 01094 * @retval None 01095 */ 01096 void MFX_IO_Init(void) 01097 { 01098 I2Cx_Init(BSP_I2C_MFX_USER); 01099 } 01100 01101 /** 01102 * @brief Configure MFX_IO low level Interrupt. 01103 * @retval None 01104 */ 01105 void MFX_IO_ITConfig(void) 01106 { 01107 GPIO_InitTypeDef gpioinitstruct = {0}; 01108 01109 /* Enable the GPIO EXTI clock */ 01110 MFX_INT_GPIO_CLK_ENABLE(); 01111 01112 /* Configure MFX Interrupt GPIO */ 01113 gpioinitstruct.Pin = MFX_INT_PIN; 01114 gpioinitstruct.Pull = GPIO_NOPULL; 01115 gpioinitstruct.Speed = GPIO_SPEED_FREQ_LOW; 01116 gpioinitstruct.Mode = GPIO_MODE_IT_RISING; 01117 HAL_GPIO_Init(MFX_INT_GPIO_PORT, &gpioinitstruct); 01118 01119 /* Enable and set GPIO EXTI Interrupt to the lowest priority */ 01120 HAL_NVIC_SetPriority((IRQn_Type)(MFX_INT_EXTI_IRQn), 0x0F, 0x0F); 01121 HAL_NVIC_EnableIRQ(MFX_INT_EXTI_IRQn); 01122 } 01123 01124 /** 01125 * @brief MFX_IO writes single data. 01126 * @param Addr: I2C address 01127 * @param Reg: Reg address 01128 * @param Value: Data to be written 01129 * @retval None 01130 */ 01131 void MFX_IO_Write(uint16_t Addr, uint8_t Reg, uint8_t Value) 01132 { 01133 I2Cx_WriteData(Addr, Reg, I2C_MEMADD_SIZE_8BIT, Value); 01134 } 01135 01136 /** 01137 * @brief MFX_IO reads single data. 01138 * @param Addr: I2C address 01139 * @param Reg: Reg address 01140 * @retval Read data 01141 */ 01142 uint8_t MFX_IO_Read(uint16_t Addr, uint8_t Reg) 01143 { 01144 return I2Cx_ReadData(Addr, Reg, I2C_MEMADD_SIZE_8BIT); 01145 } 01146 01147 /** 01148 * @brief MFX_IO reads multiple data. 01149 * @param Addr: I2C address 01150 * @param Reg: Reg address 01151 * @param Buffer: Pointer to data buffer 01152 * @param Length: Length of the data 01153 */ 01154 uint16_t MFX_IO_ReadMultiple(uint16_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length) 01155 { 01156 return I2Cx_ReadBuffer(Addr, Reg, I2C_MEMADD_SIZE_8BIT, Buffer, Length); 01157 } 01158 01159 /** 01160 * @brief MFX_IO delay. 01161 * @param Delay: Delay in ms 01162 * @retval None 01163 */ 01164 void MFX_IO_Delay(uint32_t Delay) 01165 { 01166 HAL_Delay(Delay); 01167 } 01168 01169 /** 01170 * @brief MFX_IO wakeup. 01171 * @retval None 01172 */ 01173 void MFX_IO_Wakeup(void) 01174 { 01175 /* Set Wakeup pin to high to wakeup MFX component from standby mode */ 01176 HAL_GPIO_WritePin(MFX_WAKEUP_GPIO_PORT, MFX_WAKEUP_PIN, GPIO_PIN_SET); 01177 01178 /* Wait */ 01179 HAL_Delay(1); 01180 01181 /* Set gpio pin basck to low */ 01182 HAL_GPIO_WritePin(MFX_WAKEUP_GPIO_PORT, MFX_WAKEUP_PIN, GPIO_PIN_RESET); 01183 } 01184 01185 /** 01186 * @brief MFX_IO enable wakeup pin. 01187 * @retval None 01188 */ 01189 void MFX_IO_EnableWakeupPin(void) 01190 { 01191 GPIO_InitTypeDef GPIO_InitStruct; 01192 01193 /* Enable wakeup gpio clock */ 01194 MFX_WAKEUP_GPIO_CLK_ENABLE(); 01195 01196 /* MFX wakeup pin configuration */ 01197 GPIO_InitStruct.Pin = MFX_WAKEUP_PIN; 01198 GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP; 01199 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_HIGH; 01200 GPIO_InitStruct.Pull = GPIO_NOPULL; 01201 HAL_GPIO_Init(MFX_WAKEUP_GPIO_PORT, &GPIO_InitStruct); 01202 } 01203 01204 /** 01205 * @brief DeInitializes MFX_IO low level. 01206 * @retval None 01207 */ 01208 void MFX_IO_DeInit(void) 01209 { 01210 GPIO_InitTypeDef GPIO_InitStruct; 01211 01212 /* Enable wakeup gpio clock */ 01213 MFX_WAKEUP_GPIO_CLK_ENABLE(); 01214 01215 /* MFX wakeup pin configuration */ 01216 GPIO_InitStruct.Pin = MFX_WAKEUP_PIN; 01217 GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP; 01218 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_LOW; 01219 GPIO_InitStruct.Pull = GPIO_PULLDOWN; 01220 HAL_GPIO_Init(MFX_WAKEUP_GPIO_PORT, &GPIO_InitStruct); 01221 01222 /* DeInit interrupt pin : disable IRQ before to avoid spurious interrupt */ 01223 HAL_NVIC_DisableIRQ((IRQn_Type)(MFX_INT_EXTI_IRQn)); 01224 MFX_INT_GPIO_CLK_ENABLE(); 01225 HAL_GPIO_DeInit(MFX_INT_GPIO_PORT, MFX_INT_PIN); 01226 01227 I2Cx_DeInit(BSP_I2C_MFX_USER); 01228 } 01229 01230 /********************************* LINK TOUCHSCREEN *********************************/ 01231 01232 /** 01233 * @brief Initializes Touchscreen low level. 01234 * @retval None 01235 */ 01236 void TS_IO_Init(void) 01237 { 01238 I2Cx_Init(BSP_I2C_TS_USER); 01239 } 01240 01241 /** 01242 * @brief Writes a single data. 01243 * @param Addr: I2C address 01244 * @param Reg: Reg address 01245 * @param Value: Data to be written 01246 * @retval None 01247 */ 01248 void TS_IO_Write(uint8_t Addr, uint8_t Reg, uint8_t Value) 01249 { 01250 I2Cx_WriteMultiple(Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT,(uint8_t*)&Value, 1); 01251 } 01252 01253 /** 01254 * @brief Reads a single data. 01255 * @param Addr: I2C address 01256 * @param Reg: Reg address 01257 * @retval Data to be read 01258 */ 01259 uint8_t TS_IO_Read(uint8_t Addr, uint8_t Reg) 01260 { 01261 uint8_t read_value = 0; 01262 01263 I2Cx_ReadMultiple(Addr, Reg, I2C_MEMADD_SIZE_8BIT, (uint8_t*)&read_value, 1); 01264 01265 return read_value; 01266 } 01267 01268 /** 01269 * @brief Reads multiple data with I2C communication 01270 * channel from TouchScreen. 01271 * @param Addr: I2C address 01272 * @param Reg: Register address 01273 * @param Buffer: Pointer to data buffer 01274 * @param Length: Length of the data 01275 * @retval Number of read data 01276 */ 01277 uint16_t TS_IO_ReadMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length) 01278 { 01279 return I2Cx_ReadMultiple(Addr, Reg, I2C_MEMADD_SIZE_8BIT, Buffer, Length); 01280 } 01281 01282 /** 01283 * @brief TS delay 01284 * @param Delay: Delay in ms 01285 * @retval None 01286 */ 01287 void TS_IO_Delay(uint32_t Delay) 01288 { 01289 HAL_Delay(Delay); 01290 } 01291 01292 01293 /********************************* LINK AUDIO *********************************/ 01294 01295 /** 01296 * @brief Initialize Audio low level. 01297 * @retval None 01298 */ 01299 void AUDIO_IO_Init(void) 01300 { 01301 I2Cx_Init(BSP_I2C_AUDIO_USER); 01302 } 01303 01304 /** 01305 * @brief Deinitialize Audio low level. 01306 * @retval None 01307 */ 01308 void AUDIO_IO_DeInit(void) 01309 { 01310 I2Cx_DeInit(BSP_I2C_AUDIO_USER); 01311 } 01312 01313 /** 01314 * @brief Writes a single data. 01315 * @param Addr: I2C address 01316 * @param Reg: Reg address 01317 * @param Value: Data to be written 01318 * @retval None 01319 */ 01320 void AUDIO_IO_Write(uint8_t Addr, uint16_t Reg, uint16_t Value) 01321 { 01322 uint16_t tmp = Value; 01323 01324 Value = ((uint16_t)(tmp >> 8) & 0x00FF); 01325 Value |= ((uint16_t)(tmp << 8)& 0xFF00); 01326 01327 I2Cx_WriteBuffer(Addr, Reg, I2C_MEMADD_SIZE_16BIT,(uint8_t*)&Value, 2); 01328 } 01329 01330 /** 01331 * @brief Reads a single data. 01332 * @param Addr: I2C address 01333 * @param Reg: Reg address 01334 * @retval Data to be read 01335 */ 01336 uint16_t AUDIO_IO_Read(uint8_t Addr, uint16_t Reg) 01337 { 01338 uint16_t Read_Value = 0, tmp = 0; 01339 01340 I2Cx_ReadBuffer(Addr, Reg, I2C_MEMADD_SIZE_16BIT, (uint8_t*)&Read_Value, 2); 01341 01342 tmp = ((uint16_t)(Read_Value >> 8) & 0x00FF); 01343 tmp |= ((uint16_t)(Read_Value << 8)& 0xFF00); 01344 01345 Read_Value = tmp; 01346 01347 return Read_Value; 01348 } 01349 01350 /** 01351 * @brief AUDIO Codec delay 01352 * @param Delay: Delay in ms 01353 * @retval None 01354 */ 01355 void AUDIO_IO_Delay(uint32_t Delay) 01356 { 01357 HAL_Delay(Delay); 01358 } 01359 01360 /********************************* LINK I2C EEPROM *****************************/ 01361 /** 01362 * @brief Initialize peripherals used by the I2C EEPROM driver. 01363 * @retval None 01364 */ 01365 void EEPROM_IO_Init(void) 01366 { 01367 I2Cx_Init(BSP_I2C_EEPROM_USER); 01368 } 01369 01370 /** 01371 * @brief Write data to I2C EEPROM driver 01372 * @param DevAddress: Target device address 01373 * @param MemAddress: Internal memory address 01374 * @param pBuffer: Pointer to data buffer 01375 * @param BufferSize: Amount of data to be sent 01376 * @retval HAL status 01377 */ 01378 HAL_StatusTypeDef EEPROM_IO_WriteData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize) 01379 { 01380 return (I2Cx_WriteBuffer(DevAddress, MemAddress, I2C_MEMADD_SIZE_16BIT, pBuffer, BufferSize)); 01381 } 01382 01383 /** 01384 * @brief Read data from I2C EEPROM driver 01385 * @param DevAddress: Target device address 01386 * @param MemAddress: Internal memory address 01387 * @param pBuffer: Pointer to data buffer 01388 * @param BufferSize: Amount of data to be read 01389 * @retval HAL status 01390 */ 01391 HAL_StatusTypeDef EEPROM_IO_ReadData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize) 01392 { 01393 return (I2Cx_ReadBuffer(DevAddress, MemAddress, I2C_MEMADD_SIZE_16BIT, pBuffer, BufferSize)); 01394 } 01395 01396 /** 01397 * @brief Checks if target device is ready for communication. 01398 * @note This function is used with Memory devices 01399 * @param DevAddress: Target device address 01400 * @param Trials: Number of trials 01401 * @retval HAL status 01402 */ 01403 HAL_StatusTypeDef EEPROM_IO_IsDeviceReady(uint16_t DevAddress, uint32_t Trials) 01404 { 01405 return (I2Cx_IsDeviceReady(DevAddress, Trials)); 01406 } 01407 01408 #endif /*HAL_I2C_MODULE_ENABLED*/ 01409 01410 01411 /** 01412 * @} 01413 */ 01414 01415 /** 01416 * @} 01417 */ 01418 01419 /** 01420 * @} 01421 */ 01422 01423 /** 01424 * @} 01425 */ 01426 01427 01428 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Thu Oct 12 2017 10:53:59 for STM32L4R9I_EVAL BSP User Manual by
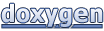