STM32L476G-Discovery BSP User Manual
|
stm32l476g_discovery_compass.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l476g_discovery_compass.c 00004 * @author MCD Application Team 00005 * @version $VERSION$ 00006 * @date $DATE$ 00007 * @brief This file provides a set of functions needed to manage the E-Compass 00008 * (ACCELEROMETER + MAGNETOMETER) MEMS LSM303C available on STM32L476G-Discovery 00009 * board. 00010 ****************************************************************************** 00011 * @attention 00012 * 00013 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00014 * 00015 * Redistribution and use in source and binary forms, with or without modification, 00016 * are permitted provided that the following conditions are met: 00017 * 1. Redistributions of source code must retain the above copyright notice, 00018 * this list of conditions and the following disclaimer. 00019 * 2. Redistributions in binary form must reproduce the above copyright notice, 00020 * this list of conditions and the following disclaimer in the documentation 00021 * and/or other materials provided with the distribution. 00022 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00023 * may be used to endorse or promote products derived from this software 00024 * without specific prior written permission. 00025 * 00026 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00027 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00028 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00029 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00030 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00031 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00032 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00033 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00034 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00035 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00036 * 00037 ****************************************************************************** 00038 */ 00039 00040 /* Includes ------------------------------------------------------------------*/ 00041 #include "stm32l476g_discovery.h" 00042 #include "stm32l476g_discovery_compass.h" 00043 #include "../Components/lsm303c/lsm303c.h" 00044 #include <math.h> 00045 00046 /** @addtogroup BSP 00047 * @{ 00048 */ 00049 00050 /** @addtogroup STM32L476G_DISCOVERY 00051 * @{ 00052 */ 00053 00054 /** @defgroup STM32L476G_DISCOVERY_COMPASS STM32L476G-DISCOVERY COMPASS 00055 * @{ 00056 */ 00057 00058 /* Private typedef -----------------------------------------------------------*/ 00059 /** @defgroup STM32L476G_DISCOVERY_COMPASS_Private_Types Private Types 00060 * @{ 00061 */ 00062 /** 00063 * @} 00064 */ 00065 00066 /* Private defines ------------------------------------------------------------*/ 00067 /** @defgroup STM32L476G_DISCOVERY_COMPASS_Private_Constants Private Constants 00068 * @{ 00069 */ 00070 /** 00071 * @} 00072 */ 00073 00074 /* Private macros ------------------------------------------------------------*/ 00075 /** @defgroup STM32L476G_DISCOVERY_COMPASS_Private_Macros Private Macros 00076 * @{ 00077 */ 00078 /** 00079 * @} 00080 */ 00081 00082 /* Private variables ---------------------------------------------------------*/ 00083 /** @defgroup STM32L476G_DISCOVERY_COMPASS_Private_Variables Private Variables 00084 * @{ 00085 */ 00086 static ACCELERO_DrvTypeDef *AccelerometerDrv; 00087 static MAGNETO_DrvTypeDef *MagnetoDrv; 00088 00089 /** 00090 * @} 00091 */ 00092 00093 /* Private function prototypes -----------------------------------------------*/ 00094 /** @addtogroup STM32L476G_DISCOVERY_COMPASS_Private_FunctionPrototypes Private Functions 00095 * @{ 00096 */ 00097 /** 00098 * @} 00099 */ 00100 00101 /* Exported functions ---------------------------------------------------------*/ 00102 /** @addtogroup STM32L476G_DISCOVERY_COMPASS_Exported_Functions 00103 * @{ 00104 */ 00105 extern void ACCELERO_IO_DeInit(void); 00106 extern void MAGNETO_IO_DeInit(void); 00107 00108 /** 00109 * @brief Initialize the COMPASS. 00110 * @retval COMPASS_OK or COMPASS_ERROR 00111 */ 00112 COMPASS_StatusTypeDef BSP_COMPASS_Init(void) 00113 { 00114 COMPASS_StatusTypeDef ret = COMPASS_OK; 00115 uint16_t ctrl = 0x0000; 00116 ACCELERO_InitTypeDef LSM303C_InitStructure; 00117 ACCELERO_FilterConfigTypeDef LSM303C_FilterStructure; 00118 MAGNETO_InitTypeDef LSM303C_InitStructureMag; 00119 00120 if(Lsm303cDrv_accelero.ReadID() != LMS303C_ACC_ID) 00121 { 00122 ret = COMPASS_ERROR; 00123 } 00124 else 00125 { 00126 /* Initialize the COMPASS accelerometer driver structure */ 00127 AccelerometerDrv = &Lsm303cDrv_accelero; 00128 00129 /* MEMS configuration ------------------------------------------------------*/ 00130 /* Fill the COMPASS accelerometer structure */ 00131 LSM303C_InitStructure.AccOutput_DataRate = LSM303C_ACC_ODR_50_HZ; 00132 LSM303C_InitStructure.Axes_Enable= LSM303C_ACC_AXES_ENABLE; 00133 LSM303C_InitStructure.AccFull_Scale = LSM303C_ACC_FULLSCALE_2G; 00134 LSM303C_InitStructure.BlockData_Update = LSM303C_ACC_BDU_CONTINUOUS; 00135 LSM303C_InitStructure.High_Resolution = LSM303C_ACC_HR_DISABLE; 00136 LSM303C_InitStructure.Communication_Mode = LSM303C_ACC_SPI_MODE; 00137 00138 /* Configure MEMS: data rate, power mode, full scale and axes */ 00139 ctrl = (LSM303C_InitStructure.High_Resolution | LSM303C_InitStructure.AccOutput_DataRate | \ 00140 LSM303C_InitStructure.Axes_Enable | LSM303C_InitStructure.BlockData_Update); 00141 00142 ctrl |= (LSM303C_InitStructure.AccFull_Scale | LSM303C_InitStructure.Communication_Mode) << 8; 00143 00144 /* Configure the COMPASS accelerometer main parameters */ 00145 AccelerometerDrv->Init(ctrl); 00146 00147 /* Fill the COMPASS accelerometer HPF structure */ 00148 LSM303C_FilterStructure.HighPassFilter_Mode_Selection = LSM303C_ACC_HPM_NORMAL_MODE; 00149 LSM303C_FilterStructure.HighPassFilter_CutOff_Frequency = LSM303C_ACC_DFC1_ODRDIV50; 00150 LSM303C_FilterStructure.HighPassFilter_Stat = LSM303C_ACC_HPI2S_INT1_DISABLE | LSM303C_ACC_HPI2S_INT2_DISABLE; 00151 00152 /* Configure MEMS: mode, cutoff frequency, Filter status, Click, AOI1 and AOI2 */ 00153 ctrl = (uint8_t) (LSM303C_FilterStructure.HighPassFilter_Mode_Selection |\ 00154 LSM303C_FilterStructure.HighPassFilter_CutOff_Frequency|\ 00155 LSM303C_FilterStructure.HighPassFilter_Stat); 00156 00157 /* Configure the COMPASS accelerometer LPF main parameters */ 00158 AccelerometerDrv->FilterConfig(ctrl); 00159 } 00160 00161 if(Lsm303cDrv_magneto.ReadID() != LMS303C_MAG_ID) 00162 { 00163 ret = COMPASS_ERROR; 00164 } 00165 else 00166 { 00167 /* Initialize the COMPASS magnetometer driver structure */ 00168 MagnetoDrv = &Lsm303cDrv_magneto; 00169 00170 /* MEMS configuration ------------------------------------------------------*/ 00171 /* Fill the COMPASS magnetometer structure */ 00172 LSM303C_InitStructureMag.Register1 = LSM303C_MAG_TEMPSENSOR_DISABLE | LSM303C_MAG_OM_XY_ULTRAHIGH | LSM303C_MAG_ODR_40_HZ; 00173 LSM303C_InitStructureMag.Register2 = LSM303C_MAG_FS_16_GA | LSM303C_MAG_REBOOT_DEFAULT | LSM303C_MAG_SOFT_RESET_DEFAULT; 00174 LSM303C_InitStructureMag.Register3 = LSM303C_MAG_SPI_MODE | LSM303C_MAG_CONFIG_NORMAL_MODE | LSM303C_MAG_CONTINUOUS_MODE; 00175 LSM303C_InitStructureMag.Register4 = LSM303C_MAG_OM_Z_ULTRAHIGH | LSM303C_MAG_BLE_LSB; 00176 LSM303C_InitStructureMag.Register5 = LSM303C_MAG_BDU_CONTINUOUS; 00177 /* Configure the COMPASS magnetometer main parameters */ 00178 MagnetoDrv->Init(LSM303C_InitStructureMag); 00179 } 00180 00181 return ret; 00182 } 00183 00184 /** 00185 * @brief DeInitialize the COMPASS. 00186 * @retval None. 00187 */ 00188 void BSP_COMPASS_DeInit(void) 00189 { 00190 /* DeInitialize the COMPASS accelerometer & magnetometer IO interfaces */ 00191 ACCELERO_IO_DeInit(); 00192 MAGNETO_IO_DeInit(); 00193 } 00194 00195 /** 00196 * @brief Put the COMPASS in low power mode. 00197 * @retval None 00198 */ 00199 void BSP_COMPASS_LowPower(void) 00200 { 00201 /* Put the COMPASS accelerometer in low power mode */ 00202 if(AccelerometerDrv->LowPower != NULL) 00203 { 00204 AccelerometerDrv->LowPower(); 00205 } 00206 /* Put the COMPASS magnetometer in low power mode */ 00207 if(MagnetoDrv->LowPower != NULL) 00208 { 00209 MagnetoDrv->LowPower(); 00210 } 00211 } 00212 00213 /** 00214 * @brief Get XYZ acceleration values. 00215 * @param pDataXYZ Pointer on 3 angular accelerations table with 00216 * pDataXYZ[0] = X axis, pDataXYZ[1] = Y axis, pDataXYZ[2] = Z axis 00217 * @retval None 00218 */ 00219 void BSP_COMPASS_AccGetXYZ(int16_t *pDataXYZ) 00220 { 00221 if(AccelerometerDrv->GetXYZ!= NULL) 00222 { 00223 AccelerometerDrv->GetXYZ(pDataXYZ); 00224 } 00225 } 00226 00227 /** 00228 * @brief Get XYZ magnetometer values. 00229 * @param pDataXYZ Pointer on 3 magnetometer values table with 00230 * pDataXYZ[0] = X axis, pDataXYZ[1] = Y axis, pDataXYZ[2] = Z axis 00231 * @retval None 00232 */ 00233 void BSP_COMPASS_MagGetXYZ(int16_t *pDataXYZ) 00234 { 00235 if(MagnetoDrv->GetXYZ!= NULL) 00236 { 00237 MagnetoDrv->GetXYZ(pDataXYZ); 00238 } 00239 } 00240 00241 /** 00242 * @} 00243 */ 00244 00245 /** 00246 * @} 00247 */ 00248 00249 /** 00250 * @} 00251 */ 00252 00253 /** 00254 * @} 00255 */ 00256 00257 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Tue Jun 23 2015 17:15:45 for STM32L476G-Discovery BSP User Manual by
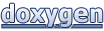