STM32769I_EVAL BSP User Manual
|
stm32f769i_eval_sram.h
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32f769i_eval_sram.h 00004 * @author MCD Application Team 00005 * @version V2.0.1 00006 * @date 06-April-2017 00007 * @brief This file contains the common defines and functions prototypes for 00008 * the stm32f769i_eval_sram.c driver. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2017 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* Define to prevent recursive inclusion -------------------------------------*/ 00040 #ifndef __STM32F769I_EVAL_SRAM_H 00041 #define __STM32F769I_EVAL_SRAM_H 00042 00043 #ifdef __cplusplus 00044 extern "C" { 00045 #endif 00046 00047 /* Includes ------------------------------------------------------------------*/ 00048 #include "stm32f7xx_hal.h" 00049 00050 /** @addtogroup BSP 00051 * @{ 00052 */ 00053 00054 /** @addtogroup STM32F769I_EVAL 00055 * @{ 00056 */ 00057 00058 /** @addtogroup STM32F769I_EVAL_SRAM 00059 * @{ 00060 */ 00061 00062 /** @defgroup STM32F769I_EVAL_SRAM_Exported_Types SRAM Exported Types 00063 * @{ 00064 */ 00065 /** 00066 * @} 00067 */ 00068 00069 /** @defgroup STM32F769I_EVAL_SRAM_Exported_Constants SRAM Exported Constants 00070 * @{ 00071 */ 00072 00073 /** 00074 * @brief SRAM status structure definition 00075 */ 00076 #define SRAM_OK ((uint8_t)0x00) 00077 #define SRAM_ERROR ((uint8_t)0x01) 00078 00079 #define SRAM_DEVICE_ADDR ((uint32_t)0x68000000) 00080 #define SRAM_DEVICE_SIZE ((uint32_t)0x200000) /* SRAM device size in MBytes */ 00081 00082 /* #define SRAM_MEMORY_WIDTH FMC_NORSRAM_MEM_BUS_WIDTH_8*/ 00083 #define SRAM_MEMORY_WIDTH FMC_NORSRAM_MEM_BUS_WIDTH_16 00084 00085 #define SRAM_BURSTACCESS FMC_BURST_ACCESS_MODE_DISABLE 00086 /* #define SRAM_BURSTACCESS FMC_BURST_ACCESS_MODE_ENABLE*/ 00087 00088 #define SRAM_WRITEBURST FMC_WRITE_BURST_DISABLE 00089 /* #define SRAM_WRITEBURST FMC_WRITE_BURST_ENABLE */ 00090 00091 #define CONTINUOUSCLOCK_FEATURE FMC_CONTINUOUS_CLOCK_SYNC_ONLY 00092 /* #define CONTINUOUSCLOCK_FEATURE FMC_CONTINUOUS_CLOCK_SYNC_ASYNC */ 00093 00094 /* DMA definitions for SRAM DMA transfer */ 00095 #define __SRAM_DMAx_CLK_ENABLE __HAL_RCC_DMA2_CLK_ENABLE 00096 #define __SRAM_DMAx_CLK_DISABLE __HAL_RCC_DMA2_CLK_DISABLE 00097 #define SRAM_DMAx_CHANNEL DMA_CHANNEL_0 00098 #define SRAM_DMAx_STREAM DMA2_Stream4 00099 #define SRAM_DMAx_IRQn DMA2_Stream4_IRQn 00100 #define BSP_SRAM_DMA_IRQHandler DMA2_Stream4_IRQHandler 00101 /** 00102 * @} 00103 */ 00104 00105 /** @defgroup STM32F769I_EVAL_SRAM_Exported_Macro SRAM Exported Macro 00106 * @{ 00107 */ 00108 /** 00109 * @} 00110 */ 00111 00112 /** @defgroup STM32F769I_EVAL_SRAM_Exported_Functions SRAM Exported Functions 00113 * @{ 00114 */ 00115 uint8_t BSP_SRAM_Init(void); 00116 uint8_t BSP_SRAM_DeInit(void); 00117 uint8_t BSP_SRAM_ReadData(uint32_t uwStartAddress, uint16_t *pData, uint32_t uwDataSize); 00118 uint8_t BSP_SRAM_ReadData_DMA(uint32_t uwStartAddress, uint16_t *pData, uint32_t uwDataSize); 00119 uint8_t BSP_SRAM_WriteData(uint32_t uwStartAddress, uint16_t *pData, uint32_t uwDataSize); 00120 uint8_t BSP_SRAM_WriteData_DMA(uint32_t uwStartAddress, uint16_t *pData, uint32_t uwDataSize); 00121 00122 /* These functions can be modified in case the current settings (e.g. DMA stream) 00123 need to be changed for specific application needs */ 00124 void BSP_SRAM_MspInit(SRAM_HandleTypeDef *hsram, void *Params); 00125 void BSP_SRAM_MspDeInit(SRAM_HandleTypeDef *hsram, void *Params); 00126 00127 /** 00128 * @} 00129 */ 00130 00131 /** 00132 * @} 00133 */ 00134 00135 /** 00136 * @} 00137 */ 00138 00139 /** 00140 * @} 00141 */ 00142 00143 00144 #ifdef __cplusplus 00145 } 00146 #endif 00147 00148 #endif /* __STM32F769I_EVAL_SRAM_H */ 00149 00150 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Thu May 25 2017 11:03:11 for STM32769I_EVAL BSP User Manual by
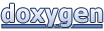