STM32769I_EVAL BSP User Manual
|
Functions | |
uint8_t | BSP_LCD_Init (void) |
Initializes the DSI LCD. | |
uint8_t | BSP_LCD_InitEx (LCD_OrientationTypeDef orientation) |
Initializes the DSI LCD. | |
void | BSP_LCD_Reset (void) |
BSP LCD Reset Hw reset the LCD DSI activating its XRES signal (active low for some time) and desactivating it later. | |
uint32_t | BSP_LCD_GetXSize (void) |
Gets the LCD X size. | |
uint32_t | BSP_LCD_GetYSize (void) |
Gets the LCD Y size. | |
void | BSP_LCD_SetXSize (uint32_t imageWidthPixels) |
Set the LCD X size. | |
void | BSP_LCD_SetYSize (uint32_t imageHeightPixels) |
Set the LCD Y size. | |
void | BSP_LCD_LayerDefaultInit (uint16_t LayerIndex, uint32_t FB_Address) |
Initializes the LCD layers. | |
void | BSP_LCD_SelectLayer (uint32_t LayerIndex) |
Selects the LCD Layer. | |
void | BSP_LCD_SetLayerVisible (uint32_t LayerIndex, FunctionalState State) |
Sets an LCD Layer visible. | |
void | BSP_LCD_SetTransparency (uint32_t LayerIndex, uint8_t Transparency) |
Configures the transparency. | |
void | BSP_LCD_SetLayerAddress (uint32_t LayerIndex, uint32_t Address) |
Sets an LCD layer frame buffer address. | |
void | BSP_LCD_SetLayerWindow (uint16_t LayerIndex, uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) |
Sets display window. | |
void | BSP_LCD_SetColorKeying (uint32_t LayerIndex, uint32_t RGBValue) |
Configures and sets the color keying. | |
void | BSP_LCD_ResetColorKeying (uint32_t LayerIndex) |
Disables the color keying. | |
void | BSP_LCD_SetTextColor (uint32_t Color) |
Sets the LCD text color. | |
uint32_t | BSP_LCD_GetTextColor (void) |
Gets the LCD text color. | |
void | BSP_LCD_SetBackColor (uint32_t Color) |
Sets the LCD background color. | |
uint32_t | BSP_LCD_GetBackColor (void) |
Gets the LCD background color. | |
void | BSP_LCD_SetFont (sFONT *fonts) |
Sets the LCD text font. | |
sFONT * | BSP_LCD_GetFont (void) |
Gets the LCD text font. | |
uint32_t | BSP_LCD_ReadPixel (uint16_t Xpos, uint16_t Ypos) |
Reads an LCD pixel. | |
void | BSP_LCD_Clear (uint32_t Color) |
Clears the whole currently active layer of LTDC. | |
void | BSP_LCD_ClearStringLine (uint32_t Line) |
Clears the selected line in currently active layer. | |
void | BSP_LCD_DisplayChar (uint16_t Xpos, uint16_t Ypos, uint8_t Ascii) |
Displays one character in currently active layer. | |
void | BSP_LCD_DisplayStringAt (uint16_t Xpos, uint16_t Ypos, uint8_t *Text, Text_AlignModeTypdef Mode) |
Displays characters in currently active layer. | |
void | BSP_LCD_DisplayStringAtLine (uint16_t Line, uint8_t *ptr) |
Displays a maximum of 60 characters on the LCD. | |
void | BSP_LCD_DrawHLine (uint16_t Xpos, uint16_t Ypos, uint16_t Length) |
Draws an horizontal line in currently active layer. | |
void | BSP_LCD_DrawVLine (uint16_t Xpos, uint16_t Ypos, uint16_t Length) |
Draws a vertical line in currently active layer. | |
void | BSP_LCD_DrawLine (uint16_t x1, uint16_t y1, uint16_t x2, uint16_t y2) |
Draws an uni-line (between two points) in currently active layer. | |
void | BSP_LCD_DrawRect (uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) |
Draws a rectangle in currently active layer. | |
void | BSP_LCD_DrawCircle (uint16_t Xpos, uint16_t Ypos, uint16_t Radius) |
Draws a circle in currently active layer. | |
void | BSP_LCD_DrawPolygon (pPoint Points, uint16_t PointCount) |
Draws an poly-line (between many points) in currently active layer. | |
void | BSP_LCD_DrawEllipse (int Xpos, int Ypos, int XRadius, int YRadius) |
Draws an ellipse on LCD in currently active layer. | |
void | BSP_LCD_DrawBitmap (uint32_t Xpos, uint32_t Ypos, uint8_t *pbmp) |
Draws a bitmap picture loaded in the internal Flash (32 bpp) in currently active layer. | |
void | BSP_LCD_FillRect (uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) |
Draws a full rectangle in currently active layer. | |
void | BSP_LCD_FillCircle (uint16_t Xpos, uint16_t Ypos, uint16_t Radius) |
Draws a full circle in currently active layer. | |
void | BSP_LCD_FillPolygon (pPoint Points, uint16_t PointCount) |
Draws a full poly-line (between many points) in currently active layer. | |
void | BSP_LCD_FillEllipse (int Xpos, int Ypos, int XRadius, int YRadius) |
Draws a full ellipse in currently active layer. | |
void | BSP_LCD_DisplayOn (void) |
Switch back on the display if was switched off by previous call of BSP_LCD_DisplayOff(). | |
void | BSP_LCD_DisplayOff (void) |
Switch Off the display. | |
void | BSP_LCD_SetBrightness (uint8_t BrightnessValue) |
Set the brightness value. | |
void | DSI_IO_WriteCmd (uint32_t NbrParams, uint8_t *pParams) |
DCS or Generic short/long write command. | |
__weak void | BSP_LCD_MspDeInit (void) |
De-Initializes the BSP LCD Msp Application can surcharge if needed this function implementation. | |
__weak void | BSP_LCD_MspInit (void) |
Initialize the BSP LCD Msp. | |
void | BSP_LCD_DrawPixel (uint16_t Xpos, uint16_t Ypos, uint32_t RGB_Code) |
Draws a pixel on LCD. | |
static uint16_t | LCD_IO_GetID (void) |
Returns the ID of connected screen by checking the HDMI (adv7533 component) ID or LCD DSI (via TS ID) ID. | |
static void | DrawChar (uint16_t Xpos, uint16_t Ypos, const uint8_t *c) |
Draws a character on LCD. | |
static void | FillTriangle (uint16_t x1, uint16_t x2, uint16_t x3, uint16_t y1, uint16_t y2, uint16_t y3) |
Fills a triangle (between 3 points). | |
static void | LL_FillBuffer (uint32_t LayerIndex, void *pDst, uint32_t xSize, uint32_t ySize, uint32_t OffLine, uint32_t ColorIndex) |
Fills a buffer. | |
static void | LL_ConvertLineToARGB8888 (void *pSrc, void *pDst, uint32_t xSize, uint32_t ColorMode) |
Converts a line to an ARGB8888 pixel format. |
Function Documentation
void BSP_LCD_Clear | ( | uint32_t | Color | ) |
Clears the whole currently active layer of LTDC.
- Parameters:
-
Color,: Color of the background
Definition at line 977 of file stm32f769i_eval_lcd.c.
References ActiveLayer, BSP_LCD_GetXSize(), BSP_LCD_GetYSize(), hltdc_eval, and LL_FillBuffer().
void BSP_LCD_ClearStringLine | ( | uint32_t | Line | ) |
Clears the selected line in currently active layer.
- Parameters:
-
Line,: Line to be cleared
Definition at line 987 of file stm32f769i_eval_lcd.c.
References ActiveLayer, LCD_DrawPropTypeDef::BackColor, BSP_LCD_FillRect(), BSP_LCD_GetXSize(), BSP_LCD_SetTextColor(), and LCD_DrawPropTypeDef::TextColor.
void BSP_LCD_DisplayChar | ( | uint16_t | Xpos, |
uint16_t | Ypos, | ||
uint8_t | Ascii | ||
) |
Displays one character in currently active layer.
- Parameters:
-
Xpos,: Start column address Ypos,: Line where to display the character shape. Ascii,: Character ascii code This parameter must be a number between Min_Data = 0x20 and Max_Data = 0x7E
Definition at line 1006 of file stm32f769i_eval_lcd.c.
References ActiveLayer, DrawChar(), and LCD_DrawPropTypeDef::pFont.
Referenced by BSP_LCD_DisplayStringAt().
void BSP_LCD_DisplayOff | ( | void | ) |
Switch Off the display.
Enter DSI ULPM mode if was allowed and configured in Dsi Configuration.
Definition at line 1576 of file stm32f769i_eval_lcd.c.
References hdsi_eval, and hdsivideo_handle.
void BSP_LCD_DisplayOn | ( | void | ) |
Switch back on the display if was switched off by previous call of BSP_LCD_DisplayOff().
Exit DSI ULPM mode if was allowed and configured in Dsi Configuration.
Definition at line 1551 of file stm32f769i_eval_lcd.c.
References hdsi_eval, and hdsivideo_handle.
void BSP_LCD_DisplayStringAt | ( | uint16_t | Xpos, |
uint16_t | Ypos, | ||
uint8_t * | Text, | ||
Text_AlignModeTypdef | Mode | ||
) |
Displays characters in currently active layer.
- Parameters:
-
Xpos,: X position (in pixel) Ypos,: Y position (in pixel) Text,: Pointer to string to display on LCD Mode,: Display mode This parameter can be one of the following values: - CENTER_MODE
- RIGHT_MODE
- LEFT_MODE
Definition at line 1023 of file stm32f769i_eval_lcd.c.
References ActiveLayer, BSP_LCD_DisplayChar(), BSP_LCD_GetXSize(), CENTER_MODE, LEFT_MODE, LCD_DrawPropTypeDef::pFont, and RIGHT_MODE.
Referenced by BSP_LCD_DisplayStringAtLine().
void BSP_LCD_DisplayStringAtLine | ( | uint16_t | Line, |
uint8_t * | ptr | ||
) |
Displays a maximum of 60 characters on the LCD.
- Parameters:
-
Line,: Line where to display the character shape ptr,: Pointer to string to display on LCD
Definition at line 1085 of file stm32f769i_eval_lcd.c.
References BSP_LCD_DisplayStringAt(), and LEFT_MODE.
void BSP_LCD_DrawBitmap | ( | uint32_t | Xpos, |
uint32_t | Ypos, | ||
uint8_t * | pbmp | ||
) |
Draws a bitmap picture loaded in the internal Flash (32 bpp) in currently active layer.
- Parameters:
-
Xpos,: Bmp X position in the LCD Ypos,: Bmp Y position in the LCD pbmp,: Pointer to Bmp picture address in the internal Flash
Definition at line 1327 of file stm32f769i_eval_lcd.c.
References ActiveLayer, BSP_LCD_GetXSize(), hltdc_eval, and LL_ConvertLineToARGB8888().
void BSP_LCD_DrawCircle | ( | uint16_t | Xpos, |
uint16_t | Ypos, | ||
uint16_t | Radius | ||
) |
Draws a circle in currently active layer.
- Parameters:
-
Xpos,: X position Ypos,: Y position Radius,: Circle radius
Definition at line 1222 of file stm32f769i_eval_lcd.c.
References ActiveLayer, and BSP_LCD_DrawPixel().
Referenced by BSP_LCD_FillCircle().
void BSP_LCD_DrawEllipse | ( | int | Xpos, |
int | Ypos, | ||
int | XRadius, | ||
int | YRadius | ||
) |
Draws an ellipse on LCD in currently active layer.
- Parameters:
-
Xpos,: X position Ypos,: Y position XRadius,: Ellipse X radius YRadius,: Ellipse Y radius
Definition at line 1295 of file stm32f769i_eval_lcd.c.
References ActiveLayer, and BSP_LCD_DrawPixel().
void BSP_LCD_DrawHLine | ( | uint16_t | Xpos, |
uint16_t | Ypos, | ||
uint16_t | Length | ||
) |
Draws an horizontal line in currently active layer.
- Parameters:
-
Xpos,: X position Ypos,: Y position Length,: Line length
Definition at line 1096 of file stm32f769i_eval_lcd.c.
References ActiveLayer, BSP_LCD_GetXSize(), hltdc_eval, and LL_FillBuffer().
Referenced by BSP_LCD_DrawRect(), BSP_LCD_FillCircle(), and BSP_LCD_FillEllipse().
void BSP_LCD_DrawLine | ( | uint16_t | x1, |
uint16_t | y1, | ||
uint16_t | x2, | ||
uint16_t | y2 | ||
) |
Draws an uni-line (between two points) in currently active layer.
- Parameters:
-
x1,: Point 1 X position y1,: Point 1 Y position x2,: Point 2 X position y2,: Point 2 Y position
Definition at line 1131 of file stm32f769i_eval_lcd.c.
References ABS, ActiveLayer, and BSP_LCD_DrawPixel().
Referenced by BSP_LCD_DrawPolygon(), and FillTriangle().
void BSP_LCD_DrawPixel | ( | uint16_t | Xpos, |
uint16_t | Ypos, | ||
uint32_t | RGB_Code | ||
) |
Draws a pixel on LCD.
- Parameters:
-
Xpos,: X position Ypos,: Y position RGB_Code,: Pixel color in ARGB mode (8-8-8-8)
Definition at line 1743 of file stm32f769i_eval_lcd.c.
References ActiveLayer, BSP_LCD_GetXSize(), and hltdc_eval.
Referenced by BSP_LCD_DrawCircle(), BSP_LCD_DrawEllipse(), BSP_LCD_DrawLine(), and DrawChar().
void BSP_LCD_DrawPolygon | ( | pPoint | Points, |
uint16_t | PointCount | ||
) |
Draws an poly-line (between many points) in currently active layer.
- Parameters:
-
Points,: Pointer to the points array PointCount,: Number of points
Definition at line 1268 of file stm32f769i_eval_lcd.c.
References BSP_LCD_DrawLine(), Point::X, and Point::Y.
void BSP_LCD_DrawRect | ( | uint16_t | Xpos, |
uint16_t | Ypos, | ||
uint16_t | Width, | ||
uint16_t | Height | ||
) |
Draws a rectangle in currently active layer.
- Parameters:
-
Xpos,: X position Ypos,: Y position Width,: Rectangle width Height,: Rectangle height
Definition at line 1205 of file stm32f769i_eval_lcd.c.
References BSP_LCD_DrawHLine(), and BSP_LCD_DrawVLine().
void BSP_LCD_DrawVLine | ( | uint16_t | Xpos, |
uint16_t | Ypos, | ||
uint16_t | Length | ||
) |
Draws a vertical line in currently active layer.
- Parameters:
-
Xpos,: X position Ypos,: Y position Length,: Line length
Definition at line 1113 of file stm32f769i_eval_lcd.c.
References ActiveLayer, BSP_LCD_GetXSize(), hltdc_eval, and LL_FillBuffer().
Referenced by BSP_LCD_DrawRect().
void BSP_LCD_FillCircle | ( | uint16_t | Xpos, |
uint16_t | Ypos, | ||
uint16_t | Radius | ||
) |
Draws a full circle in currently active layer.
- Parameters:
-
Xpos,: X position Ypos,: Y position Radius,: Circle radius
Definition at line 1407 of file stm32f769i_eval_lcd.c.
References ActiveLayer, BSP_LCD_DrawCircle(), BSP_LCD_DrawHLine(), and BSP_LCD_SetTextColor().
void BSP_LCD_FillEllipse | ( | int | Xpos, |
int | Ypos, | ||
int | XRadius, | ||
int | YRadius | ||
) |
Draws a full ellipse in currently active layer.
- Parameters:
-
Xpos,: X position Ypos,: Y position XRadius,: Ellipse X radius YRadius,: Ellipse Y radius
Definition at line 1521 of file stm32f769i_eval_lcd.c.
References BSP_LCD_DrawHLine().
void BSP_LCD_FillPolygon | ( | pPoint | Points, |
uint16_t | PointCount | ||
) |
Draws a full poly-line (between many points) in currently active layer.
- Parameters:
-
Points,: Pointer to the points array PointCount,: Number of points
Definition at line 1454 of file stm32f769i_eval_lcd.c.
References FillTriangle(), POLY_X, POLY_Y, Point::X, and Point::Y.
void BSP_LCD_FillRect | ( | uint16_t | Xpos, |
uint16_t | Ypos, | ||
uint16_t | Width, | ||
uint16_t | Height | ||
) |
Draws a full rectangle in currently active layer.
- Parameters:
-
Xpos,: X position Ypos,: Y position Width,: Rectangle width Height,: Rectangle height
Definition at line 1387 of file stm32f769i_eval_lcd.c.
References ActiveLayer, BSP_LCD_GetXSize(), BSP_LCD_SetTextColor(), hltdc_eval, and LL_FillBuffer().
Referenced by BSP_LCD_ClearStringLine().
uint32_t BSP_LCD_GetBackColor | ( | void | ) |
Gets the LCD background color.
- Return values:
-
Used background color
Definition at line 914 of file stm32f769i_eval_lcd.c.
References ActiveLayer, and LCD_DrawPropTypeDef::BackColor.
sFONT * BSP_LCD_GetFont | ( | void | ) |
Gets the LCD text font.
- Return values:
-
Used layer font
Definition at line 932 of file stm32f769i_eval_lcd.c.
References ActiveLayer, and LCD_DrawPropTypeDef::pFont.
uint32_t BSP_LCD_GetTextColor | ( | void | ) |
Gets the LCD text color.
- Return values:
-
Used text color.
Definition at line 896 of file stm32f769i_eval_lcd.c.
References ActiveLayer, and LCD_DrawPropTypeDef::TextColor.
uint32_t BSP_LCD_GetXSize | ( | void | ) |
Gets the LCD X size.
- Return values:
-
Used LCD X size
Definition at line 718 of file stm32f769i_eval_lcd.c.
References lcd_x_size.
Referenced by BSP_LCD_Clear(), BSP_LCD_ClearStringLine(), BSP_LCD_DisplayStringAt(), BSP_LCD_DrawBitmap(), BSP_LCD_DrawHLine(), BSP_LCD_DrawPixel(), BSP_LCD_DrawVLine(), BSP_LCD_FillRect(), BSP_LCD_LayerDefaultInit(), and BSP_LCD_ReadPixel().
uint32_t BSP_LCD_GetYSize | ( | void | ) |
Gets the LCD Y size.
- Return values:
-
Used LCD Y size
Definition at line 727 of file stm32f769i_eval_lcd.c.
References lcd_y_size.
Referenced by BSP_LCD_Clear(), and BSP_LCD_LayerDefaultInit().
uint8_t BSP_LCD_Init | ( | void | ) |
Initializes the DSI LCD.
- Return values:
-
LCD state
Definition at line 279 of file stm32f769i_eval_lcd.c.
References BSP_LCD_InitEx(), and LCD_ORIENTATION_LANDSCAPE.
uint8_t BSP_LCD_InitEx | ( | LCD_OrientationTypeDef | orientation | ) |
Initializes the DSI LCD.
The ititialization is done as below:
- DSI PLL ititialization
- DSI ititialization
- LTDC ititialization
- OTM8009A LCD Display IC Driver ititialization
- Parameters:
-
orientation,: LCD_ORIENTATION_PORTRAIT or LCD_ORIENTATION_LANDSCAPE
- Return values:
-
LCD state
< LcdClk = 27429 kHz
< Vertical start active time in units of lines
< Vertical Back Porch time in units of lines
< Vertical Front Porch time in units of lines
< Vertical Active time in units of lines = imageSize Y in pixels to display
< Horizontal start active time in units of lcdClk
< Horizontal Back Porch time in units of lcdClk
< Horizontal Front Porch time in units of lcdClk
< Horizontal Active time in units of lcdClk = imageSize X in pixels to display
LCD clock configuration Note: The following values should not be changed as the PLLSAI is also used to clock the USB FS PLLSAI_VCO Input = HSE_VALUE/PLL_M = 1 Mhz PLLSAI_VCO Output = PLLSAI_VCO Input * PLLSAIN = 384 Mhz PLLLCDCLK = PLLSAI_VCO Output/PLLSAIR = 384 MHz / 7 = 54.85 MHz LTDC clock frequency = PLLLCDCLK / LTDC_PLLSAI_DIVR_2 = 54.85 MHz / 2 = 27.429 MHz
Definition at line 294 of file stm32f769i_eval_lcd.c.
References BSP_LCD_MspInit(), BSP_LCD_Reset(), BSP_LCD_SetFont(), BSP_SDRAM_Init(), hdsi_eval, hdsivideo_handle, hltdc_eval, LCD_DEFAULT_FONT, LCD_DSI_ID, LCD_DSI_PIXEL_DATA_FMT_RBG888, LCD_ERROR, LCD_IO_GetID(), LCD_OK, LCD_ORIENTATION_PORTRAIT, LCD_OTM8009A_ID, lcd_x_size, and lcd_y_size.
Referenced by BSP_LCD_Init().
void BSP_LCD_LayerDefaultInit | ( | uint16_t | LayerIndex, |
uint32_t | FB_Address | ||
) |
Initializes the LCD layers.
- Parameters:
-
LayerIndex,: Layer foreground or background FB_Address,: Layer frame buffer
- Return values:
-
None
Definition at line 758 of file stm32f769i_eval_lcd.c.
References LCD_DrawPropTypeDef::BackColor, BSP_LCD_GetXSize(), BSP_LCD_GetYSize(), hltdc_eval, LCD_COLOR_BLACK, LCD_COLOR_WHITE, LCD_LayerCfgTypeDef, LCD_DrawPropTypeDef::pFont, and LCD_DrawPropTypeDef::TextColor.
void BSP_LCD_MspDeInit | ( | void | ) |
De-Initializes the BSP LCD Msp Application can surcharge if needed this function implementation.
Disable IRQ of LTDC IP
Disable IRQ of DMA2D IP
Disable IRQ of DSI IP
Force and let in reset state LTDC, DMA2D and DSI Host + Wrapper IPs
Disable the LTDC, DMA2D and DSI Host and Wrapper clocks
Definition at line 1675 of file stm32f769i_eval_lcd.c.
void BSP_LCD_MspInit | ( | void | ) |
Initialize the BSP LCD Msp.
Application can surcharge if needed this function implementation
Enable the LTDC clock
Toggle Sw reset of LTDC IP
Enable the DMA2D clock
Toggle Sw reset of DMA2D IP
Enable DSI Host and wrapper clocks
Soft Reset the DSI Host and wrapper
NVIC configuration for LTDC interrupt that is now enabled
NVIC configuration for DMA2D interrupt that is now enabled
NVIC configuration for DSI interrupt that is now enabled
Definition at line 1701 of file stm32f769i_eval_lcd.c.
Referenced by BSP_LCD_InitEx().
uint32_t BSP_LCD_ReadPixel | ( | uint16_t | Xpos, |
uint16_t | Ypos | ||
) |
Reads an LCD pixel.
- Parameters:
-
Xpos,: X position Ypos,: Y position
- Return values:
-
RGB pixel color
Definition at line 943 of file stm32f769i_eval_lcd.c.
References ActiveLayer, BSP_LCD_GetXSize(), and hltdc_eval.
void BSP_LCD_Reset | ( | void | ) |
BSP LCD Reset Hw reset the LCD DSI activating its XRES signal (active low for some time) and desactivating it later.
Definition at line 688 of file stm32f769i_eval_lcd.c.
Referenced by BSP_LCD_InitEx().
void BSP_LCD_ResetColorKeying | ( | uint32_t | LayerIndex | ) |
Disables the color keying.
- Parameters:
-
LayerIndex,: Layer foreground or background
Definition at line 877 of file stm32f769i_eval_lcd.c.
References hltdc_eval.
void BSP_LCD_SelectLayer | ( | uint32_t | LayerIndex | ) |
Selects the LCD Layer.
- Parameters:
-
LayerIndex,: Layer foreground or background
Definition at line 791 of file stm32f769i_eval_lcd.c.
References ActiveLayer.
void BSP_LCD_SetBackColor | ( | uint32_t | Color | ) |
Sets the LCD background color.
- Parameters:
-
Color,: Layer background color code ARGB(8-8-8-8)
Definition at line 905 of file stm32f769i_eval_lcd.c.
References ActiveLayer, and LCD_DrawPropTypeDef::BackColor.
void BSP_LCD_SetBrightness | ( | uint8_t | BrightnessValue | ) |
Set the brightness value.
- Parameters:
-
BrightnessValue,: [00: Min (black), 100 Max]
Definition at line 1600 of file stm32f769i_eval_lcd.c.
References hdsi_eval, and LCD_OTM8009A_ID.
void BSP_LCD_SetColorKeying | ( | uint32_t | LayerIndex, |
uint32_t | RGBValue | ||
) |
Configures and sets the color keying.
- Parameters:
-
LayerIndex,: Layer foreground or background RGBValue,: Color reference
Definition at line 866 of file stm32f769i_eval_lcd.c.
References hltdc_eval.
void BSP_LCD_SetFont | ( | sFONT * | fonts | ) |
Sets the LCD text font.
- Parameters:
-
fonts,: Layer font to be used
Definition at line 923 of file stm32f769i_eval_lcd.c.
References ActiveLayer, and LCD_DrawPropTypeDef::pFont.
Referenced by BSP_LCD_InitEx().
void BSP_LCD_SetLayerAddress | ( | uint32_t | LayerIndex, |
uint32_t | Address | ||
) |
Sets an LCD layer frame buffer address.
- Parameters:
-
LayerIndex,: Layer foreground or background Address,: New LCD frame buffer value
Definition at line 836 of file stm32f769i_eval_lcd.c.
References hltdc_eval.
void BSP_LCD_SetLayerVisible | ( | uint32_t | LayerIndex, |
FunctionalState | State | ||
) |
Sets an LCD Layer visible.
- Parameters:
-
LayerIndex,: Visible Layer State,: New state of the specified layer This parameter can be one of the following values: - ENABLE
- DISABLE
Definition at line 804 of file stm32f769i_eval_lcd.c.
References hltdc_eval.
void BSP_LCD_SetLayerWindow | ( | uint16_t | LayerIndex, |
uint16_t | Xpos, | ||
uint16_t | Ypos, | ||
uint16_t | Width, | ||
uint16_t | Height | ||
) |
Sets display window.
- Parameters:
-
LayerIndex,: Layer index Xpos,: LCD X position Ypos,: LCD Y position Width,: LCD window width Height,: LCD window height
Definition at line 851 of file stm32f769i_eval_lcd.c.
References hltdc_eval.
void BSP_LCD_SetTextColor | ( | uint32_t | Color | ) |
Sets the LCD text color.
- Parameters:
-
Color,: Text color code ARGB(8-8-8-8)
Definition at line 887 of file stm32f769i_eval_lcd.c.
References ActiveLayer, and LCD_DrawPropTypeDef::TextColor.
Referenced by BSP_LCD_ClearStringLine(), BSP_LCD_FillCircle(), and BSP_LCD_FillRect().
void BSP_LCD_SetTransparency | ( | uint32_t | LayerIndex, |
uint8_t | Transparency | ||
) |
Configures the transparency.
- Parameters:
-
LayerIndex,: Layer foreground or background. Transparency,: Transparency This parameter must be a number between Min_Data = 0x00 and Max_Data = 0xFF
Definition at line 824 of file stm32f769i_eval_lcd.c.
References hltdc_eval.
void BSP_LCD_SetXSize | ( | uint32_t | imageWidthPixels | ) |
Set the LCD X size.
- Parameters:
-
imageWidthPixels : uint32_t image width in pixels unit
- Return values:
-
None
Definition at line 737 of file stm32f769i_eval_lcd.c.
References ActiveLayer, and hltdc_eval.
void BSP_LCD_SetYSize | ( | uint32_t | imageHeightPixels | ) |
Set the LCD Y size.
- Parameters:
-
imageHeightPixels : uint32_t image height in lines unit
Definition at line 746 of file stm32f769i_eval_lcd.c.
References ActiveLayer, and hltdc_eval.
static void DrawChar | ( | uint16_t | Xpos, |
uint16_t | Ypos, | ||
const uint8_t * | c | ||
) | [static] |
Draws a character on LCD.
- Parameters:
-
Xpos,: Line where to display the character shape Ypos,: Start column address c,: Pointer to the character data
Definition at line 1756 of file stm32f769i_eval_lcd.c.
References ActiveLayer, BSP_LCD_DrawPixel(), and LCD_DrawPropTypeDef::pFont.
Referenced by BSP_LCD_DisplayChar().
void DSI_IO_WriteCmd | ( | uint32_t | NbrParams, |
uint8_t * | pParams | ||
) |
DCS or Generic short/long write command.
- Parameters:
-
NbrParams,: Number of parameters. It indicates the write command mode: If inferior to 2, a long write command is performed else short. pParams,: Pointer to parameter values table.
- Return values:
-
HAL status
Definition at line 1626 of file stm32f769i_eval_lcd.c.
References hdsi_eval, and LCD_OTM8009A_ID.
static void FillTriangle | ( | uint16_t | x1, |
uint16_t | x2, | ||
uint16_t | x3, | ||
uint16_t | y1, | ||
uint16_t | y2, | ||
uint16_t | y3 | ||
) | [static] |
Fills a triangle (between 3 points).
- Parameters:
-
x1,: Point 1 X position y1,: Point 1 Y position x2,: Point 2 X position y2,: Point 2 Y position x3,: Point 3 X position y3,: Point 3 Y position
Definition at line 1814 of file stm32f769i_eval_lcd.c.
References ABS, and BSP_LCD_DrawLine().
Referenced by BSP_LCD_FillPolygon().
static uint16_t LCD_IO_GetID | ( | void | ) | [static] |
Returns the ID of connected screen by checking the HDMI (adv7533 component) ID or LCD DSI (via TS ID) ID.
- Return values:
-
LCD ID
Definition at line 1643 of file stm32f769i_eval_lcd.c.
References LCD_DSI_ADDRESS, LCD_DSI_ID, and LCD_DSI_ID_REG.
Referenced by BSP_LCD_InitEx().
static void LL_ConvertLineToARGB8888 | ( | void * | pSrc, |
void * | pDst, | ||
uint32_t | xSize, | ||
uint32_t | ColorMode | ||
) | [static] |
Converts a line to an ARGB8888 pixel format.
- Parameters:
-
pSrc,: Pointer to source buffer pDst,: Output color xSize,: Buffer width ColorMode,: Input color mode
Definition at line 1921 of file stm32f769i_eval_lcd.c.
References hdma2d_eval.
Referenced by BSP_LCD_DrawBitmap().
static void LL_FillBuffer | ( | uint32_t | LayerIndex, |
void * | pDst, | ||
uint32_t | xSize, | ||
uint32_t | ySize, | ||
uint32_t | OffLine, | ||
uint32_t | ColorIndex | ||
) | [static] |
Fills a buffer.
- Parameters:
-
LayerIndex,: Layer index pDst,: Pointer to destination buffer xSize,: Buffer width ySize,: Buffer height OffLine,: Offset ColorIndex,: Color index
Definition at line 1891 of file stm32f769i_eval_lcd.c.
References hdma2d_eval.
Referenced by BSP_LCD_Clear(), BSP_LCD_DrawHLine(), BSP_LCD_DrawVLine(), and BSP_LCD_FillRect().
Generated on Thu May 25 2017 11:03:12 for STM32769I_EVAL BSP User Manual by
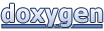