STM32769I_EVAL BSP User Manual
|
stm32f769i_eval_sram.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32f769i_eval_sram.c 00004 * @author MCD Application Team 00005 * @version V2.0.1 00006 * @date 06-April-2017 00007 * @brief This file includes the SRAM driver for the IS61WV102416BLL-10M memory 00008 * device mounted on STM32F769I-EVAL evaluation boards. 00009 @verbatim 00010 How To use this driver: 00011 ----------------------- 00012 - This driver is used to drive the IS61WV102416BLL-10M SRAM external memory mounted 00013 on STM32F769I-EVAL evaluation board. 00014 - This driver does not need a specific component driver for the SRAM device 00015 to be included with. 00016 00017 Driver description: 00018 ------------------ 00019 + Initialization steps: 00020 o Initialize the SRAM external memory using the BSP_SRAM_Init() function. This 00021 function includes the MSP layer hardware resources initialization and the 00022 FMC controller configuration to interface with the external SRAM memory. 00023 00024 + SRAM read/write operations 00025 o SRAM external memory can be accessed with read/write operations once it is 00026 initialized. 00027 Read/write operation can be performed with AHB access using the functions 00028 BSP_SRAM_ReadData()/BSP_SRAM_WriteData(), or by DMA transfer using the functions 00029 BSP_SRAM_ReadData_DMA()/BSP_SRAM_WriteData_DMA(). 00030 o The AHB access is performed with 16-bit width transaction, the DMA transfer 00031 configuration is fixed at single (no burst) halfword transfer 00032 (see the SRAM_MspInit() static function). 00033 o User can implement his own functions for read/write access with his desired 00034 configurations. 00035 o If interrupt mode is used for DMA transfer, the function BSP_SRAM_DMA_IRQHandler() 00036 is called in IRQ handler file, to serve the generated interrupt once the DMA 00037 transfer is complete. 00038 @endverbatim 00039 ****************************************************************************** 00040 * @attention 00041 * 00042 * <h2><center>© COPYRIGHT(c) 2017 STMicroelectronics</center></h2> 00043 * 00044 * Redistribution and use in source and binary forms, with or without modification, 00045 * are permitted provided that the following conditions are met: 00046 * 1. Redistributions of source code must retain the above copyright notice, 00047 * this list of conditions and the following disclaimer. 00048 * 2. Redistributions in binary form must reproduce the above copyright notice, 00049 * this list of conditions and the following disclaimer in the documentation 00050 * and/or other materials provided with the distribution. 00051 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00052 * may be used to endorse or promote products derived from this software 00053 * without specific prior written permission. 00054 * 00055 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00056 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00057 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00058 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00059 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00060 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00061 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00062 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00063 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00064 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00065 * 00066 ****************************************************************************** 00067 */ 00068 00069 /* Dependencies 00070 - stm32f7xx_hal_sram.c 00071 - stm32f7xx_ll_fmc.c 00072 - stm32f7xx_hal_dma.c 00073 - stm32f7xx_hal_gpio.c 00074 - stm32f7xx_hal_cortex.c 00075 - stm32f7xx_hal_rcc_ex.h 00076 EndDependencies */ 00077 00078 /* Includes ------------------------------------------------------------------*/ 00079 #include "stm32f769i_eval_sram.h" 00080 00081 /** @addtogroup BSP 00082 * @{ 00083 */ 00084 00085 /** @addtogroup STM32F769I_EVAL 00086 * @{ 00087 */ 00088 00089 /** @defgroup STM32F769I_EVAL_SRAM STM32F769I_EVAL SRAM 00090 * @{ 00091 */ 00092 00093 /** @defgroup STM32F769I_EVAL_SRAM_Private_Types_Definitions SRAM Private Types Definitions 00094 * @{ 00095 */ 00096 /** 00097 * @} 00098 */ 00099 00100 /** @defgroup STM32F769I_EVAL_SRAM_Private_Defines EVAL SRAM Private Defines 00101 * @{ 00102 */ 00103 /** 00104 * @} 00105 */ 00106 00107 /** @defgroup STM32F769I_EVAL_SRAM_Private_Macros SRAM Private Macros 00108 * @{ 00109 */ 00110 /** 00111 * @} 00112 */ 00113 00114 /** @defgroup STM32F769I_EVAL_SRAM_Private_Variables SRAM Private Variables 00115 * @{ 00116 */ 00117 SRAM_HandleTypeDef sramHandle; 00118 static FMC_NORSRAM_TimingTypeDef Timing; 00119 /** 00120 * @} 00121 */ 00122 00123 /** @defgroup STM32F769I_EVAL_SRAM_Private_Functions_Prototypes SRAM Private Functions Prototypes 00124 * @{ 00125 */ 00126 /** 00127 * @} 00128 */ 00129 00130 /** @defgroup STM32F769I_EVAL_SRAM_Private_Functions SRAM Private Functions 00131 * @{ 00132 */ 00133 00134 /** 00135 * @brief Initializes the SRAM device. 00136 * @retval SRAM status 00137 */ 00138 uint8_t BSP_SRAM_Init(void) 00139 { 00140 static uint8_t sram_status = SRAM_ERROR; 00141 /* SRAM device configuration */ 00142 sramHandle.Instance = FMC_NORSRAM_DEVICE; 00143 sramHandle.Extended = FMC_NORSRAM_EXTENDED_DEVICE; 00144 00145 /* SRAM device configuration */ 00146 /* Timing configuration derived from system clock (up to 216Mhz) 00147 for 108Mhz as SRAM clock frequency */ 00148 Timing.AddressSetupTime = 2; 00149 Timing.AddressHoldTime = 1; 00150 Timing.DataSetupTime = 2; 00151 Timing.BusTurnAroundDuration = 1; 00152 Timing.CLKDivision = 2; 00153 Timing.DataLatency = 2; 00154 Timing.AccessMode = FMC_ACCESS_MODE_A; 00155 00156 sramHandle.Init.NSBank = FMC_NORSRAM_BANK3; 00157 sramHandle.Init.DataAddressMux = FMC_DATA_ADDRESS_MUX_DISABLE; 00158 sramHandle.Init.MemoryType = FMC_MEMORY_TYPE_SRAM; 00159 sramHandle.Init.MemoryDataWidth = SRAM_MEMORY_WIDTH; 00160 sramHandle.Init.BurstAccessMode = SRAM_BURSTACCESS; 00161 sramHandle.Init.WaitSignalPolarity = FMC_WAIT_SIGNAL_POLARITY_LOW; 00162 sramHandle.Init.WaitSignalActive = FMC_WAIT_TIMING_BEFORE_WS; 00163 sramHandle.Init.WriteOperation = FMC_WRITE_OPERATION_ENABLE; 00164 sramHandle.Init.WaitSignal = FMC_WAIT_SIGNAL_DISABLE; 00165 sramHandle.Init.ExtendedMode = FMC_EXTENDED_MODE_DISABLE; 00166 sramHandle.Init.AsynchronousWait = FMC_ASYNCHRONOUS_WAIT_DISABLE; 00167 sramHandle.Init.WriteBurst = SRAM_WRITEBURST; 00168 sramHandle.Init.ContinuousClock = CONTINUOUSCLOCK_FEATURE; 00169 00170 /* SRAM controller initialization */ 00171 BSP_SRAM_MspInit(&sramHandle, NULL); /* __weak function can be rewritten by the application */ 00172 if(HAL_SRAM_Init(&sramHandle, &Timing, &Timing) != HAL_OK) 00173 { 00174 sram_status = SRAM_ERROR; 00175 } 00176 else 00177 { 00178 sram_status = SRAM_OK; 00179 } 00180 return sram_status; 00181 } 00182 00183 /** 00184 * @brief DeInitializes the SRAM device. 00185 * @retval SRAM status 00186 */ 00187 uint8_t BSP_SRAM_DeInit(void) 00188 { 00189 static uint8_t sram_status = SRAM_ERROR; 00190 /* SRAM device de-initialization */ 00191 sramHandle.Instance = FMC_NORSRAM_DEVICE; 00192 sramHandle.Extended = FMC_NORSRAM_EXTENDED_DEVICE; 00193 00194 if(HAL_SRAM_DeInit(&sramHandle) != HAL_OK) 00195 { 00196 sram_status = SRAM_ERROR; 00197 } 00198 else 00199 { 00200 sram_status = SRAM_OK; 00201 } 00202 00203 /* SRAM controller de-initialization */ 00204 BSP_SRAM_MspDeInit(&sramHandle, NULL); 00205 00206 return sram_status; 00207 } 00208 00209 /** 00210 * @brief Reads an amount of data from the SRAM device in polling mode. 00211 * @param uwStartAddress: Read start address 00212 * @param pData: Pointer to data to be read 00213 * @param uwDataSize: Size of read data from the memory 00214 * @retval SRAM status 00215 */ 00216 uint8_t BSP_SRAM_ReadData(uint32_t uwStartAddress, uint16_t *pData, uint32_t uwDataSize) 00217 { 00218 if(HAL_SRAM_Read_16b(&sramHandle, (uint32_t *)uwStartAddress, pData, uwDataSize) != HAL_OK) 00219 { 00220 return SRAM_ERROR; 00221 } 00222 else 00223 { 00224 return SRAM_OK; 00225 } 00226 } 00227 00228 /** 00229 * @brief Reads an amount of data from the SRAM device in DMA mode. 00230 * @param uwStartAddress: Read start address 00231 * @param pData: Pointer to data to be read 00232 * @param uwDataSize: Size of read data from the memory 00233 * @retval SRAM status 00234 */ 00235 uint8_t BSP_SRAM_ReadData_DMA(uint32_t uwStartAddress, uint16_t *pData, uint32_t uwDataSize) 00236 { 00237 if(HAL_SRAM_Read_DMA(&sramHandle, (uint32_t *)uwStartAddress, (uint32_t *)pData, uwDataSize) != HAL_OK) 00238 { 00239 return SRAM_ERROR; 00240 } 00241 else 00242 { 00243 return SRAM_OK; 00244 } 00245 } 00246 00247 /** 00248 * @brief Writes an amount of data from the SRAM device in polling mode. 00249 * @param uwStartAddress: Write start address 00250 * @param pData: Pointer to data to be written 00251 * @param uwDataSize: Size of written data from the memory 00252 * @retval SRAM status 00253 */ 00254 uint8_t BSP_SRAM_WriteData(uint32_t uwStartAddress, uint16_t *pData, uint32_t uwDataSize) 00255 { 00256 if(HAL_SRAM_Write_16b(&sramHandle, (uint32_t *)uwStartAddress, pData, uwDataSize) != HAL_OK) 00257 { 00258 return SRAM_ERROR; 00259 } 00260 else 00261 { 00262 return SRAM_OK; 00263 } 00264 } 00265 00266 /** 00267 * @brief Writes an amount of data from the SRAM device in DMA mode. 00268 * @param uwStartAddress: Write start address 00269 * @param pData: Pointer to data to be written 00270 * @param uwDataSize: Size of written data from the memory 00271 * @retval SRAM status 00272 */ 00273 uint8_t BSP_SRAM_WriteData_DMA(uint32_t uwStartAddress, uint16_t *pData, uint32_t uwDataSize) 00274 { 00275 if(HAL_SRAM_Write_DMA(&sramHandle, (uint32_t *)uwStartAddress, (uint32_t *)pData, uwDataSize) != HAL_OK) 00276 { 00277 return SRAM_ERROR; 00278 } 00279 else 00280 { 00281 return SRAM_OK; 00282 } 00283 } 00284 00285 /** 00286 * @brief Initializes SRAM MSP. 00287 * @param hsram: SRAM handle 00288 * @param Params 00289 * @retval None 00290 */ 00291 __weak void BSP_SRAM_MspInit(SRAM_HandleTypeDef *hsram, void *Params) 00292 { 00293 static DMA_HandleTypeDef dma_handle; 00294 GPIO_InitTypeDef gpio_init_structure; 00295 00296 /* Enable FMC clock */ 00297 __HAL_RCC_FMC_CLK_ENABLE(); 00298 00299 /* Enable chosen DMAx clock */ 00300 __SRAM_DMAx_CLK_ENABLE(); 00301 00302 /* Enable GPIOs clock */ 00303 __HAL_RCC_GPIOD_CLK_ENABLE(); 00304 __HAL_RCC_GPIOE_CLK_ENABLE(); 00305 __HAL_RCC_GPIOF_CLK_ENABLE(); 00306 __HAL_RCC_GPIOG_CLK_ENABLE(); 00307 00308 /* Common GPIO configuration */ 00309 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00310 gpio_init_structure.Pull = GPIO_PULLUP; 00311 gpio_init_structure.Speed = GPIO_SPEED_FREQ_VERY_HIGH; 00312 gpio_init_structure.Alternate = GPIO_AF12_FMC; 00313 00314 /* GPIOD configuration */ 00315 gpio_init_structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_4 | GPIO_PIN_5 | GPIO_PIN_8 |\ 00316 GPIO_PIN_9 | GPIO_PIN_10 | GPIO_PIN_11 | GPIO_PIN_12 | GPIO_PIN_13 |\ 00317 GPIO_PIN_14 | GPIO_PIN_15; 00318 HAL_GPIO_Init(GPIOD, &gpio_init_structure); 00319 00320 /* GPIOE configuration */ 00321 gpio_init_structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_3| GPIO_PIN_4 | GPIO_PIN_7 |\ 00322 GPIO_PIN_8 | GPIO_PIN_9 | GPIO_PIN_10 | GPIO_PIN_11 | GPIO_PIN_12 |\ 00323 GPIO_PIN_13 | GPIO_PIN_14 | GPIO_PIN_15; 00324 HAL_GPIO_Init(GPIOE, &gpio_init_structure); 00325 00326 /* GPIOF configuration */ 00327 gpio_init_structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_2| GPIO_PIN_3 | GPIO_PIN_4 |\ 00328 GPIO_PIN_5 | GPIO_PIN_12 | GPIO_PIN_13 | GPIO_PIN_14 | GPIO_PIN_15; 00329 HAL_GPIO_Init(GPIOF, &gpio_init_structure); 00330 00331 /* GPIOG configuration */ 00332 gpio_init_structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_2| GPIO_PIN_3 | GPIO_PIN_4 |\ 00333 GPIO_PIN_5 | GPIO_PIN_6; 00334 HAL_GPIO_Init(GPIOG, &gpio_init_structure); 00335 00336 /* Configure common DMA parameters */ 00337 dma_handle.Init.Channel = SRAM_DMAx_CHANNEL; 00338 dma_handle.Init.Direction = DMA_MEMORY_TO_MEMORY; 00339 dma_handle.Init.PeriphInc = DMA_PINC_ENABLE; 00340 dma_handle.Init.MemInc = DMA_MINC_ENABLE; 00341 dma_handle.Init.PeriphDataAlignment = DMA_PDATAALIGN_HALFWORD; 00342 dma_handle.Init.MemDataAlignment = DMA_MDATAALIGN_HALFWORD; 00343 dma_handle.Init.Mode = DMA_NORMAL; 00344 dma_handle.Init.Priority = DMA_PRIORITY_HIGH; 00345 dma_handle.Init.FIFOMode = DMA_FIFOMODE_DISABLE; 00346 dma_handle.Init.FIFOThreshold = DMA_FIFO_THRESHOLD_FULL; 00347 dma_handle.Init.MemBurst = DMA_MBURST_SINGLE; 00348 dma_handle.Init.PeriphBurst = DMA_PBURST_SINGLE; 00349 00350 dma_handle.Instance = SRAM_DMAx_STREAM; 00351 00352 /* Associate the DMA handle */ 00353 __HAL_LINKDMA(hsram, hdma, dma_handle); 00354 00355 /* Deinitialize the Stream for new transfer */ 00356 HAL_DMA_DeInit(&dma_handle); 00357 00358 /* Configure the DMA Stream */ 00359 HAL_DMA_Init(&dma_handle); 00360 00361 /* NVIC configuration for DMA transfer complete interrupt */ 00362 HAL_NVIC_SetPriority(SRAM_DMAx_IRQn, 0x0F, 0); 00363 HAL_NVIC_EnableIRQ(SRAM_DMAx_IRQn); 00364 } 00365 00366 00367 /** 00368 * @brief DeInitializes SRAM MSP. 00369 * @param hsram: SRAM handle 00370 * @param Params 00371 * @retval None 00372 */ 00373 __weak void BSP_SRAM_MspDeInit(SRAM_HandleTypeDef *hsram, void *Params) 00374 { 00375 static DMA_HandleTypeDef dma_handle; 00376 00377 /* Disable NVIC configuration for DMA interrupt */ 00378 HAL_NVIC_DisableIRQ(SRAM_DMAx_IRQn); 00379 00380 /* Deinitialize the stream for new transfer */ 00381 dma_handle.Instance = SRAM_DMAx_STREAM; 00382 HAL_DMA_DeInit(&dma_handle); 00383 00384 /* GPIO pins clock, FMC clock and DMA clock can be shut down in the applications 00385 by surcharging this __weak function */ 00386 } 00387 00388 /** 00389 * @} 00390 */ 00391 00392 /** 00393 * @} 00394 */ 00395 00396 /** 00397 * @} 00398 */ 00399 00400 /** 00401 * @} 00402 */ 00403 00404 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Thu May 25 2017 11:03:11 for STM32769I_EVAL BSP User Manual by
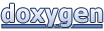