STM32769I_EVAL BSP User Manual
|
This file includes the uSD card driver mounted on STM32F769I-EVAL evaluation boards. More...
#include "stm32f769i_eval_sd.h"
Go to the source code of this file.
Functions | |
uint8_t | BSP_SD_Init (void) |
Initializes the SD card device. | |
uint8_t | BSP_SD_InitEx (uint32_t SdCard) |
Initializes the SD card device. | |
uint8_t | BSP_SD_DeInit (void) |
DeInitializes the SD card device. | |
uint8_t | BSP_SD_DeInitEx (uint32_t SdCard) |
DeInitializes the SD card device. | |
uint8_t | BSP_SD_ITConfig (void) |
Configures Interrupt mode for SD1 detection pin. | |
uint8_t | BSP_SD_ITConfigEx (uint32_t SdCard) |
Configures Interrupt mode for SD detection pin. | |
uint8_t | BSP_SD_IsDetected (void) |
Detects if SD card is correctly plugged in the memory slot or not. | |
uint8_t | BSP_SD_IsDetectedEx (uint32_t SdCard) |
Detects if SD card is correctly plugged in the memory slot or not. | |
uint8_t | BSP_SD_ReadBlocks (uint32_t *pData, uint32_t ReadAddr, uint32_t NumOfBlocks, uint32_t Timeout) |
Reads block(s) from a specified address in an SD card, in polling mode. | |
uint8_t | BSP_SD_ReadBlocksEx (uint32_t SdCard, uint32_t *pData, uint32_t ReadAddr, uint32_t NumOfBlocks, uint32_t Timeout) |
Reads block(s) from a specified address in an SD card, in polling mode. | |
uint8_t | BSP_SD_WriteBlocks (uint32_t *pData, uint32_t WriteAddr, uint32_t NumOfBlocks, uint32_t Timeout) |
Writes block(s) to a specified address in an SD card, in polling mode. | |
uint8_t | BSP_SD_WriteBlocksEx (uint32_t SdCard, uint32_t *pData, uint32_t WriteAddr, uint32_t NumOfBlocks, uint32_t Timeout) |
Writes block(s) to a specified address in an SD card, in polling mode. | |
uint8_t | BSP_SD_ReadBlocks_DMA (uint32_t *pData, uint32_t ReadAddr, uint32_t NumOfBlocks) |
Reads block(s) from a specified address in an SD card, in DMA mode. | |
uint8_t | BSP_SD_ReadBlocks_DMAEx (uint32_t SdCard, uint32_t *pData, uint32_t ReadAddr, uint32_t NumOfBlocks) |
Reads block(s) from a specified address in an SD card, in DMA mode. | |
uint8_t | BSP_SD_WriteBlocks_DMA (uint32_t *pData, uint32_t WriteAddr, uint32_t NumOfBlocks) |
Writes block(s) to a specified address in an SD card, in DMA mode. | |
uint8_t | BSP_SD_WriteBlocks_DMAEx (uint32_t SdCard, uint32_t *pData, uint32_t WriteAddr, uint32_t NumOfBlocks) |
Writes block(s) to a specified address in an SD card, in DMA mode. | |
uint8_t | BSP_SD_Erase (uint32_t StartAddr, uint32_t EndAddr) |
Erases the specified memory area of the given SD card. | |
uint8_t | BSP_SD_EraseEx (uint32_t SdCard, uint32_t StartAddr, uint32_t EndAddr) |
Erases the specified memory area of the given SD card. | |
__weak void | BSP_SD_MspInit (SD_HandleTypeDef *hsd, void *Params) |
Initializes the SD MSP. | |
__weak void | BSP_SD_MspDeInit (SD_HandleTypeDef *hsd, void *Params) |
DeInitializes the SD MSP. | |
uint8_t | BSP_SD_GetCardState (void) |
Gets the current SD card data status. | |
uint8_t | BSP_SD_GetCardStateEx (uint32_t SdCard) |
Gets the current SD card data status. | |
void | BSP_SD_GetCardInfo (BSP_SD_CardInfo *CardInfo) |
Get SD information about specific SD card. | |
void | BSP_SD_GetCardInfoEx (uint32_t SdCard, BSP_SD_CardInfo *CardInfo) |
Get SD information about specific SD card. | |
void | HAL_SD_AbortCallback (SD_HandleTypeDef *hsd) |
SD Abort callbacks. | |
void | HAL_SD_TxCpltCallback (SD_HandleTypeDef *hsd) |
Tx Transfer completed callbacks. | |
void | HAL_SD_RxCpltCallback (SD_HandleTypeDef *hsd) |
Rx Transfer completed callbacks. | |
__weak void | BSP_SD_AbortCallback (uint32_t SdCard) |
BSP SD Abort callbacks. | |
__weak void | BSP_SD_WriteCpltCallback (uint32_t SdCard) |
BSP Tx Transfer completed callbacks. | |
__weak void | BSP_SD_ReadCpltCallback (uint32_t SdCard) |
BSP Rx Transfer completed callbacks. | |
Variables | |
SD_HandleTypeDef | uSdHandle |
SD_HandleTypeDef | uSdHandle2 |
static uint8_t | UseExtiModeDetection = 0 |
Detailed Description
This file includes the uSD card driver mounted on STM32F769I-EVAL evaluation boards.
- Attention:
© COPYRIGHT(c) 2017 STMicroelectronics
Redistribution and use in source and binary forms, with or without modification, are permitted provided that the following conditions are met: 1. Redistributions of source code must retain the above copyright notice, this list of conditions and the following disclaimer. 2. Redistributions in binary form must reproduce the above copyright notice, this list of conditions and the following disclaimer in the documentation and/or other materials provided with the distribution. 3. Neither the name of STMicroelectronics nor the names of its contributors may be used to endorse or promote products derived from this software without specific prior written permission.
THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
Definition in file stm32f769i_eval_sd.c.
Generated on Thu May 25 2017 11:03:12 for STM32769I_EVAL BSP User Manual by
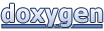