STM32769I_EVAL BSP User Manual
|
stm32f769i_eval_sd.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32f769i_eval_sd.c 00004 * @author MCD Application Team 00005 * @version V2.0.1 00006 * @date 06-April-2017 00007 * @brief This file includes the uSD card driver mounted on STM32F769I-EVAL 00008 * evaluation boards. 00009 @verbatim 00010 How To use this driver: 00011 ----------------------- 00012 - This driver is used to drive the micro SD external cards mounted on STM32F769I-EVAL 00013 evaluation board. 00014 - This driver does not need a specific component driver for the micro SD device 00015 to be included with. 00016 00017 Driver description: 00018 ------------------ 00019 + Initialization steps: 00020 o Initialize the micro SD card using the BSP_SD_InitEx() function. This 00021 function includes the MSP layer hardware resources initialization and the 00022 SDIO interface configuration to interface with the external micro SD. It 00023 also includes the micro SD initialization sequence for SDCard1 or SDCard2. 00024 When BSP_SD_Init() is called, SDCard1 is by default initialized. 00025 o To check the SD card presence you can use the function BSP_SD_IsDetectedEx() which 00026 returns the detection status for SDCard1 or SDCard2. 00027 the function BSP_SD_IsDetected() returns the detection status for SDCard1. 00028 o If SD presence detection interrupt mode is desired, you must configure the 00029 SD detection interrupt mode by calling the functions BSP_SD_ITConfig() for 00030 SDCard1 or BSP_SD_ITConfigEx() for SDCard2 . The interrupt is generated as 00031 an external interrupt whenever the micro SD card is plugged/unplugged 00032 in/from the evaluation board. The SD detection is managed by MFX, so the 00033 SD detection interrupt has to be treated by MFX_IRQOUT gpio pin IRQ handler. 00034 o The function BSP_SD_GetCardInfo()/BSP_SD_GetCardInfoEx() are used to get 00035 the micro SD card information which is stored in the structure 00036 "HAL_SD_CardInfoTypedef". 00037 00038 + Micro SD card operations 00039 o The micro SD card can be accessed with read/write block(s) operations once 00040 it is ready for access. The access, by default to SDCard1, can be performed whether 00041 using the polling mode by calling the functions BSP_SD_ReadBlocks()/BSP_SD_WriteBlocks(), 00042 or by DMA transfer using the functions BSP_SD_ReadBlocks_DMA()/BSP_SD_WriteBlocks_DMA(). 00043 The access can be performed to SDCard1 or SDCard2 by calling BSP_SD_ReadBlocksEx(), 00044 BSP_SD_WriteBlocksEx() or by calling BSP_SD_ReadBlocks_DMAEx()/BSP_SD_WriteBlocks_DMAEx(). 00045 o The DMA transfer complete is used with interrupt mode. Once the SD transfer 00046 is complete, the SD interrupt is handled using the function BSP_SDMMC1_IRQHandler() 00047 when SDCard1 is used or BSP_SDMMC2_IRQHandler() when SDCard2 is used. 00048 The DMA Tx/Rx transfer complete are handled using the functions 00049 BSP_SDMMC1_DMA_Tx_IRQHandler(), BSP_SDMMC1_DMA_Rx_IRQHandler(), 00050 BSP_SDMMC2_DMA_Tx_IRQHandler(), BSP_SDMMC2_DMA_Rx_IRQHandler(). The corresponding 00051 user callbacks are implemented by the user at application level. 00052 o The SD erase block(s) is performed using the functions BSP_SD_Erase()/BSP_SD_EraseEx() 00053 with specifying the number of blocks to erase. 00054 o The SD runtime status is returned when calling the function BSP_SD_GetCardState() 00055 BSP_SD_GetCardStateEx(). 00056 00057 @endverbatim 00058 ****************************************************************************** 00059 * @attention 00060 * 00061 * <h2><center>© COPYRIGHT(c) 2017 STMicroelectronics</center></h2> 00062 * 00063 * Redistribution and use in source and binary forms, with or without modification, 00064 * are permitted provided that the following conditions are met: 00065 * 1. Redistributions of source code must retain the above copyright notice, 00066 * this list of conditions and the following disclaimer. 00067 * 2. Redistributions in binary form must reproduce the above copyright notice, 00068 * this list of conditions and the following disclaimer in the documentation 00069 * and/or other materials provided with the distribution. 00070 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00071 * may be used to endorse or promote products derived from this software 00072 * without specific prior written permission. 00073 * 00074 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00075 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00076 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00077 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00078 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00079 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00080 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00081 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00082 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00083 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00084 * 00085 ****************************************************************************** 00086 */ 00087 00088 /* Dependencies 00089 - stm32f769i_eval.c 00090 - stm32f7xx_hal_sd.c 00091 - stm32f7xx_ll_sdmmc.c 00092 - stm32f7xx_hal_dma.c 00093 - stm32f7xx_hal_gpio.c 00094 - stm32f7xx_hal_cortex.c 00095 - stm32f7xx_hal_rcc_ex.h 00096 EndDependencies */ 00097 00098 /* Includes ------------------------------------------------------------------*/ 00099 #include "stm32f769i_eval_sd.h" 00100 00101 /** @addtogroup BSP 00102 * @{ 00103 */ 00104 00105 /** @addtogroup STM32F769I_EVAL 00106 * @{ 00107 */ 00108 00109 /** @defgroup STM32F769I_EVAL_SD STM32F769I_EVAL SD 00110 * @{ 00111 */ 00112 00113 00114 /** @defgroup STM32F769I_EVAL_SD_Private_TypesDefinitions SD Private TypesDefinitions 00115 * @{ 00116 */ 00117 /** 00118 * @} 00119 */ 00120 00121 /** @defgroup STM32F769I_EVAL_SD_Private_Defines SD Private Defines 00122 * @{ 00123 */ 00124 /** 00125 * @} 00126 */ 00127 00128 /** @defgroup STM32F769I_EVAL_SD_Private_Macros SD Private Macros 00129 * @{ 00130 */ 00131 /** 00132 * @} 00133 */ 00134 00135 /** @defgroup STM32F769I_EVAL_SD_Private_Variables SD Private Variables 00136 * @{ 00137 */ 00138 SD_HandleTypeDef uSdHandle; 00139 SD_HandleTypeDef uSdHandle2; 00140 static uint8_t UseExtiModeDetection = 0; 00141 00142 /** 00143 * @} 00144 */ 00145 00146 /** @defgroup STM32F769I_EVAL_SD_Private_Functions_Prototypes SD Private Functions Prototypes 00147 * @{ 00148 */ 00149 /** 00150 * @} 00151 */ 00152 00153 /** @defgroup STM32F769I_EVAL_SD_Private_Functions SD Private Functions 00154 * @{ 00155 */ 00156 00157 /** 00158 * @brief Initializes the SD card device. 00159 * @retval SD status 00160 */ 00161 uint8_t BSP_SD_Init(void) 00162 { 00163 /* By default, initialize SDMMC1 */ 00164 return BSP_SD_InitEx(SD_CARD1); 00165 } 00166 00167 /** 00168 * @brief Initializes the SD card device. 00169 * @param SdCard: SD card to be used, that should be SD_CARD1 or SD_CARD2 00170 * @retval SD status 00171 */ 00172 uint8_t BSP_SD_InitEx(uint32_t SdCard) 00173 { 00174 uint8_t sd_state = MSD_OK; 00175 00176 /* uSD device interface configuration */ 00177 if(SdCard == SD_CARD1) 00178 { 00179 uSdHandle.Instance = SDMMC1; 00180 uSdHandle.Init.ClockEdge = SDMMC_CLOCK_EDGE_RISING; 00181 uSdHandle.Init.ClockBypass = SDMMC_CLOCK_BYPASS_DISABLE; 00182 uSdHandle.Init.ClockPowerSave = SDMMC_CLOCK_POWER_SAVE_DISABLE; 00183 uSdHandle.Init.BusWide = SDMMC_BUS_WIDE_1B; 00184 uSdHandle.Init.HardwareFlowControl = SDMMC_HARDWARE_FLOW_CONTROL_DISABLE; 00185 uSdHandle.Init.ClockDiv = SDMMC_TRANSFER_CLK_DIV; 00186 } 00187 else 00188 { 00189 uSdHandle2.Instance = SDMMC2; 00190 uSdHandle2.Init.ClockEdge = SDMMC_CLOCK_EDGE_RISING; 00191 uSdHandle2.Init.ClockBypass = SDMMC_CLOCK_BYPASS_DISABLE; 00192 uSdHandle2.Init.ClockPowerSave = SDMMC_CLOCK_POWER_SAVE_DISABLE; 00193 uSdHandle2.Init.BusWide = SDMMC_BUS_WIDE_1B; 00194 uSdHandle2.Init.HardwareFlowControl = SDMMC_HARDWARE_FLOW_CONTROL_DISABLE; 00195 uSdHandle2.Init.ClockDiv = SDMMC_TRANSFER_CLK_DIV; 00196 } 00197 /* Initialize IO functionalities (MFX) used by SD detect pin */ 00198 BSP_IO_Init(); 00199 00200 /* Check if the SD card is plugged in the slot */ 00201 if(SdCard == SD_CARD1) 00202 { 00203 BSP_IO_ConfigPin(SD1_DETECT_PIN, IO_MODE_INPUT_PU); 00204 00205 if(BSP_SD_IsDetected() != SD_PRESENT) 00206 { 00207 return MSD_ERROR_SD_NOT_PRESENT; 00208 } 00209 00210 /* Msp SD initialization */ 00211 BSP_SD_MspInit(&uSdHandle, NULL); 00212 00213 /* HAL SD initialization */ 00214 if(HAL_SD_Init(&uSdHandle) != HAL_OK) 00215 { 00216 sd_state = MSD_ERROR; 00217 } 00218 } 00219 else 00220 { 00221 BSP_IO_ConfigPin(SD2_DETECT_PIN, IO_MODE_INPUT_PU); 00222 00223 if(BSP_SD_IsDetectedEx(SD_CARD2) != SD_PRESENT) 00224 { 00225 return MSD_ERROR_SD_NOT_PRESENT; 00226 } 00227 /* Msp SD initialization */ 00228 BSP_SD_MspInit(&uSdHandle2, NULL); 00229 00230 /* HAL SD initialization */ 00231 if(HAL_SD_Init(&uSdHandle2) != HAL_OK) 00232 { 00233 sd_state = MSD_ERROR; 00234 } 00235 } 00236 00237 00238 /* Configure SD Bus width */ 00239 if(sd_state == MSD_OK) 00240 { 00241 if(SdCard == SD_CARD1) 00242 { 00243 /* Enable wide operation */ 00244 sd_state = HAL_SD_ConfigWideBusOperation(&uSdHandle, SDMMC_BUS_WIDE_4B); 00245 } 00246 else 00247 { 00248 /* Enable wide operation */ 00249 sd_state = HAL_SD_ConfigWideBusOperation(&uSdHandle2, SDMMC_BUS_WIDE_4B); 00250 } 00251 if(sd_state != HAL_OK) 00252 { 00253 sd_state = MSD_ERROR; 00254 } 00255 else 00256 { 00257 sd_state = MSD_OK; 00258 } 00259 } 00260 00261 return sd_state; 00262 } 00263 00264 00265 /** 00266 * @brief DeInitializes the SD card device. 00267 * @retval SD status 00268 */ 00269 uint8_t BSP_SD_DeInit(void) 00270 { 00271 /* By default, DeInitialize SDMMC1 */ 00272 return BSP_SD_DeInitEx(SD_CARD1); 00273 } 00274 00275 /** 00276 * @brief DeInitializes the SD card device. 00277 * @param SdCard: SD card to be used, that should be SD_CARD1 or SD_CARD2 00278 * @retval SD status 00279 */ 00280 uint8_t BSP_SD_DeInitEx(uint32_t SdCard) 00281 { 00282 uint8_t sd_state = MSD_OK; 00283 00284 /* Set back Mfx pin to INPUT mode in case it was in exti */ 00285 UseExtiModeDetection = 0; 00286 if(SdCard == SD_CARD1) 00287 { 00288 uSdHandle.Instance = SDMMC1; 00289 /* HAL SD deinitialization */ 00290 if(HAL_SD_DeInit(&uSdHandle) != HAL_OK) 00291 { 00292 sd_state = MSD_ERROR; 00293 } 00294 00295 /* Msp SD deinitialization */ 00296 BSP_SD_MspDeInit(&uSdHandle, NULL); 00297 BSP_IO_ConfigPin(SD1_DETECT_PIN, IO_MODE_INPUT_PU); 00298 } 00299 else 00300 { 00301 uSdHandle2.Instance = SDMMC2; 00302 BSP_IO_ConfigPin(SD2_DETECT_PIN, IO_MODE_INPUT_PU); 00303 00304 /* HAL SD deinitialization */ 00305 if(HAL_SD_DeInit(&uSdHandle2) != HAL_OK) 00306 { 00307 sd_state = MSD_ERROR; 00308 } 00309 00310 /* Msp SD deinitialization */ 00311 BSP_SD_MspDeInit(&uSdHandle2, NULL); 00312 } 00313 return sd_state; 00314 } 00315 00316 /** 00317 * @brief Configures Interrupt mode for SD1 detection pin. 00318 * @retval Returns 0 00319 */ 00320 uint8_t BSP_SD_ITConfig(void) 00321 { 00322 /* Configure Interrupt mode for SD1 detection pin */ 00323 /* Note: disabling exti mode can be done calling BSP_SD_DeInit() */ 00324 UseExtiModeDetection = 1; 00325 BSP_SD_IsDetected(); 00326 00327 return 0; 00328 } 00329 00330 /** 00331 * @brief Configures Interrupt mode for SD detection pin. 00332 * @param SdCard: SD card to be used, that should be SD_CARD1 or SD_CARD2 00333 * @retval Returns 0 00334 */ 00335 uint8_t BSP_SD_ITConfigEx(uint32_t SdCard) 00336 { 00337 /* Configure Interrupt mode for SD2 detection pin */ 00338 /* Note: disabling exti mode can be done calling BSP_SD_DeInitEx(SD_CARD2) */ 00339 UseExtiModeDetection = 1; 00340 BSP_SD_IsDetectedEx(SdCard); 00341 00342 return 0; 00343 } 00344 00345 /** 00346 * @brief Detects if SD card is correctly plugged in the memory slot or not. 00347 * @retval Returns if SD is detected or not 00348 */ 00349 uint8_t BSP_SD_IsDetected(void) 00350 { 00351 return BSP_SD_IsDetectedEx(SD_CARD1); 00352 } 00353 00354 /** 00355 * @brief Detects if SD card is correctly plugged in the memory slot or not. 00356 * @param SdCard: SD card to be used, that should be SD_CARD1 or SD_CARD2 00357 * @retval Returns if SD is detected or not 00358 */ 00359 uint8_t BSP_SD_IsDetectedEx(uint32_t SdCard) 00360 { 00361 __IO uint8_t status = SD_PRESENT; 00362 00363 if(SdCard == SD_CARD1) 00364 { 00365 /* Check SD card detect pin */ 00366 if((BSP_IO_ReadPin(SD1_DETECT_PIN)&SD1_DETECT_PIN) != SD1_DETECT_PIN) 00367 { 00368 if (UseExtiModeDetection) 00369 { 00370 BSP_IO_ConfigPin(SD1_DETECT_PIN, IO_MODE_IT_RISING_EDGE_PU); 00371 } 00372 } 00373 else 00374 { 00375 status = SD_NOT_PRESENT; 00376 if (UseExtiModeDetection) 00377 { 00378 BSP_IO_ConfigPin(SD1_DETECT_PIN, IO_MODE_IT_FALLING_EDGE_PU); 00379 } 00380 } 00381 } 00382 else 00383 { 00384 /* Check SD card detect pin */ 00385 if((BSP_IO_ReadPin(SD2_DETECT_PIN)&SD2_DETECT_PIN) != SD2_DETECT_PIN) 00386 { 00387 if (UseExtiModeDetection) 00388 { 00389 BSP_IO_ConfigPin(SD2_DETECT_PIN, IO_MODE_IT_RISING_EDGE_PU); 00390 } 00391 } 00392 else 00393 { 00394 status = SD_NOT_PRESENT; 00395 if (UseExtiModeDetection) 00396 { 00397 BSP_IO_ConfigPin(SD2_DETECT_PIN, IO_MODE_IT_FALLING_EDGE_PU); 00398 } 00399 } 00400 } 00401 return status; 00402 } 00403 00404 /** 00405 * @brief Reads block(s) from a specified address in an SD card, in polling mode. 00406 * @param pData: Pointer to the buffer that will contain the data to transmit 00407 * @param ReadAddr: Address from where data is to be read 00408 * @param NumOfBlocks: Number of SD blocks to read 00409 * @param Timeout: Timeout for read operation 00410 * @retval SD status 00411 */ 00412 uint8_t BSP_SD_ReadBlocks(uint32_t *pData, uint32_t ReadAddr, uint32_t NumOfBlocks, uint32_t Timeout) 00413 { 00414 return BSP_SD_ReadBlocksEx(SD_CARD1, pData, ReadAddr, NumOfBlocks, Timeout); 00415 } 00416 00417 /** 00418 * @brief Reads block(s) from a specified address in an SD card, in polling mode. 00419 * @param SdCard: SD card to be used, that should be SD_CARD1 or SD_CARD2 00420 * @param pData: Pointer to the buffer that will contain the data to transmit 00421 * @param ReadAddr: Address from where data is to be read 00422 * @param NumOfBlocks: Number of SD blocks to read 00423 * @param Timeout: Timeout for read operation 00424 * @retval SD status 00425 */ 00426 uint8_t BSP_SD_ReadBlocksEx(uint32_t SdCard, uint32_t *pData, uint32_t ReadAddr, uint32_t NumOfBlocks, uint32_t Timeout) 00427 { 00428 HAL_StatusTypeDef sd_state = HAL_OK; 00429 00430 if(SdCard == SD_CARD1) 00431 { 00432 sd_state = HAL_SD_ReadBlocks(&uSdHandle, (uint8_t *)pData, ReadAddr, NumOfBlocks, Timeout); 00433 } 00434 else 00435 { 00436 sd_state = HAL_SD_ReadBlocks(&uSdHandle2, (uint8_t *)pData, ReadAddr, NumOfBlocks, Timeout); 00437 } 00438 00439 if( sd_state == HAL_OK) 00440 { 00441 return MSD_OK; 00442 } 00443 else 00444 { 00445 return MSD_ERROR; 00446 } 00447 } 00448 00449 /** 00450 * @brief Writes block(s) to a specified address in an SD card, in polling mode. 00451 * @param pData: Pointer to the buffer that will contain the data to transmit 00452 * @param WriteAddr: Address from where data is to be written 00453 * @param NumOfBlocks: Number of SD blocks to write 00454 * @param Timeout: Timeout for write operation 00455 * @retval SD status 00456 */ 00457 uint8_t BSP_SD_WriteBlocks(uint32_t *pData, uint32_t WriteAddr, uint32_t NumOfBlocks, uint32_t Timeout) 00458 { 00459 return BSP_SD_WriteBlocksEx(SD_CARD1, pData, WriteAddr, NumOfBlocks, Timeout); 00460 } 00461 00462 /** 00463 * @brief Writes block(s) to a specified address in an SD card, in polling mode. 00464 * @param SdCard: SD card to be used, that should be SD_CARD1 or SD_CARD2 00465 * @param pData: Pointer to the buffer that will contain the data to transmit 00466 * @param WriteAddr: Address from where data is to be written 00467 * @param NumOfBlocks: Number of SD blocks to write 00468 * @param Timeout: Timeout for write operation 00469 * @retval SD status 00470 */ 00471 uint8_t BSP_SD_WriteBlocksEx(uint32_t SdCard, uint32_t *pData, uint32_t WriteAddr, uint32_t NumOfBlocks, uint32_t Timeout) 00472 { 00473 HAL_StatusTypeDef sd_state = HAL_OK; 00474 00475 if(SdCard == SD_CARD1) 00476 { 00477 sd_state = HAL_SD_WriteBlocks(&uSdHandle, (uint8_t *)pData, WriteAddr, NumOfBlocks, Timeout); 00478 } 00479 else 00480 { 00481 sd_state = HAL_SD_WriteBlocks(&uSdHandle2, (uint8_t *)pData, WriteAddr, NumOfBlocks, Timeout); 00482 } 00483 00484 if( sd_state == HAL_OK) 00485 { 00486 return MSD_OK; 00487 } 00488 else 00489 { 00490 return MSD_ERROR; 00491 } 00492 } 00493 00494 /** 00495 * @brief Reads block(s) from a specified address in an SD card, in DMA mode. 00496 * @param pData: Pointer to the buffer that will contain the data to transmit 00497 * @param ReadAddr: Address from where data is to be read 00498 * @param NumOfBlocks: Number of SD blocks to read 00499 * @retval SD status 00500 */ 00501 uint8_t BSP_SD_ReadBlocks_DMA(uint32_t *pData, uint32_t ReadAddr, uint32_t NumOfBlocks) 00502 { 00503 return BSP_SD_ReadBlocks_DMAEx(SD_CARD1, pData, ReadAddr, NumOfBlocks); 00504 } 00505 00506 /** 00507 * @brief Reads block(s) from a specified address in an SD card, in DMA mode. 00508 * @param SdCard: SD card to be used, that should be SD_CARD1 or SD_CARD2 00509 * @param pData: Pointer to the buffer that will contain the data to transmit 00510 * @param ReadAddr: Address from where data is to be read 00511 * @param NumOfBlocks: Number of SD blocks to read 00512 * @retval SD status 00513 */ 00514 uint8_t BSP_SD_ReadBlocks_DMAEx(uint32_t SdCard, uint32_t *pData, uint32_t ReadAddr, uint32_t NumOfBlocks) 00515 { 00516 HAL_StatusTypeDef sd_state = HAL_OK; 00517 00518 if(SdCard == SD_CARD1) 00519 { 00520 /* Read block(s) in DMA transfer mode */ 00521 sd_state = HAL_SD_ReadBlocks_DMA(&uSdHandle, (uint8_t *)pData, ReadAddr, NumOfBlocks); 00522 } 00523 else 00524 { 00525 /* Read block(s) in DMA transfer mode */ 00526 sd_state = HAL_SD_ReadBlocks_DMA(&uSdHandle2, (uint8_t *)pData, ReadAddr, NumOfBlocks); 00527 } 00528 00529 if( sd_state == HAL_OK) 00530 { 00531 return MSD_OK; 00532 } 00533 else 00534 { 00535 return MSD_ERROR; 00536 } 00537 } 00538 00539 /** 00540 * @brief Writes block(s) to a specified address in an SD card, in DMA mode. 00541 * @param pData: Pointer to the buffer that will contain the data to transmit 00542 * @param WriteAddr: Address from where data is to be written 00543 * @param NumOfBlocks: Number of SD blocks to write 00544 * @retval SD status 00545 */ 00546 uint8_t BSP_SD_WriteBlocks_DMA(uint32_t *pData, uint32_t WriteAddr, uint32_t NumOfBlocks) 00547 { 00548 return BSP_SD_WriteBlocks_DMAEx(SD_CARD1, pData, WriteAddr, NumOfBlocks); 00549 } 00550 00551 /** 00552 * @brief Writes block(s) to a specified address in an SD card, in DMA mode. 00553 * @param SdCard: SD card to be used, that should be SD_CARD1 or SD_CARD2 00554 * @param pData: Pointer to the buffer that will contain the data to transmit 00555 * @param WriteAddr: Address from where data is to be written 00556 * @param NumOfBlocks: Number of SD blocks to write 00557 * @retval SD status 00558 */ 00559 uint8_t BSP_SD_WriteBlocks_DMAEx(uint32_t SdCard, uint32_t *pData, uint32_t WriteAddr, uint32_t NumOfBlocks) 00560 { 00561 HAL_StatusTypeDef sd_state = HAL_OK; 00562 00563 if(SdCard == SD_CARD1) 00564 { 00565 /* Write block(s) in DMA transfer mode */ 00566 sd_state = HAL_SD_WriteBlocks_DMA(&uSdHandle, (uint8_t *)pData, WriteAddr, NumOfBlocks); 00567 } 00568 else 00569 { 00570 /* Write block(s) in DMA transfer mode */ 00571 sd_state = HAL_SD_WriteBlocks_DMA(&uSdHandle2, (uint8_t *)pData, WriteAddr, NumOfBlocks); 00572 } 00573 00574 if( sd_state == HAL_OK) 00575 { 00576 return MSD_OK; 00577 } 00578 else 00579 { 00580 return MSD_ERROR; 00581 } 00582 } 00583 00584 /** 00585 * @brief Erases the specified memory area of the given SD card. 00586 * @param StartAddr: Start byte address 00587 * @param EndAddr: End byte address 00588 * @retval SD status 00589 */ 00590 uint8_t BSP_SD_Erase(uint32_t StartAddr, uint32_t EndAddr) 00591 { 00592 return BSP_SD_EraseEx(SD_CARD1, StartAddr, EndAddr); 00593 } 00594 00595 /** 00596 * @brief Erases the specified memory area of the given SD card. 00597 * @param SdCard: SD card to be used, that should be SD_CARD1 or SD_CARD2 00598 * @param StartAddr: Start byte address 00599 * @param EndAddr: End byte address 00600 * @retval SD status 00601 */ 00602 uint8_t BSP_SD_EraseEx(uint32_t SdCard, uint32_t StartAddr, uint32_t EndAddr) 00603 { 00604 HAL_StatusTypeDef sd_state = HAL_OK; 00605 00606 if(SdCard == SD_CARD1) 00607 { 00608 sd_state = HAL_SD_Erase(&uSdHandle, StartAddr, EndAddr); 00609 } 00610 else 00611 { 00612 sd_state = HAL_SD_Erase(&uSdHandle2, StartAddr, EndAddr); 00613 } 00614 00615 if( sd_state == HAL_OK) 00616 { 00617 return MSD_OK; 00618 } 00619 else 00620 { 00621 return MSD_ERROR; 00622 } 00623 } 00624 00625 /** 00626 * @brief Initializes the SD MSP. 00627 * @param hsd: SD handle 00628 * @param Params 00629 * @retval None 00630 */ 00631 __weak void BSP_SD_MspInit(SD_HandleTypeDef *hsd, void *Params) 00632 { 00633 static DMA_HandleTypeDef dma_rx_handle; 00634 static DMA_HandleTypeDef dma_tx_handle; 00635 static DMA_HandleTypeDef dma_rx_handle2; 00636 static DMA_HandleTypeDef dma_tx_handle2; 00637 GPIO_InitTypeDef gpio_init_structure; 00638 00639 /* Camera has to be powered down as some signals use same GPIOs between 00640 * SD card and camera bus. Camera drives its signals to low impedance 00641 * when powered ON. So the camera is powered off to let its signals 00642 * in high impedance */ 00643 00644 /* Camera power down sequence */ 00645 BSP_IO_ConfigPin(RSTI_PIN, IO_MODE_OUTPUT); 00646 BSP_IO_ConfigPin(XSDN_PIN, IO_MODE_OUTPUT); 00647 /* De-assert the camera STANDBY pin (active high) */ 00648 BSP_IO_WritePin(XSDN_PIN, BSP_IO_PIN_RESET); 00649 /* Assert the camera RSTI pin (active low) */ 00650 BSP_IO_WritePin(RSTI_PIN, BSP_IO_PIN_RESET); 00651 if(hsd->Instance == SDMMC1) 00652 { 00653 /* Enable SDIO clock */ 00654 __HAL_RCC_SDMMC1_CLK_ENABLE(); 00655 00656 /* Enable DMA2 clocks */ 00657 __DMAx_TxRx_CLK_ENABLE(); 00658 00659 /* Enable GPIOs clock */ 00660 __HAL_RCC_GPIOC_CLK_ENABLE(); 00661 __HAL_RCC_GPIOD_CLK_ENABLE(); 00662 00663 /* Common GPIO configuration */ 00664 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00665 gpio_init_structure.Pull = GPIO_PULLUP; 00666 gpio_init_structure.Speed = GPIO_SPEED_HIGH; 00667 gpio_init_structure.Alternate = GPIO_AF12_SDMMC1; 00668 00669 /* GPIOC configuration: SD1_D0, SD1_D1, SD1_D2, SD1_D3 and SD1_CLK pins */ 00670 gpio_init_structure.Pin = GPIO_PIN_8 | GPIO_PIN_9 | GPIO_PIN_10 | GPIO_PIN_11 | GPIO_PIN_12; 00671 00672 HAL_GPIO_Init(GPIOC, &gpio_init_structure); 00673 00674 /* GPIOD configuration: SD1_CMD pin */ 00675 gpio_init_structure.Pin = GPIO_PIN_2; 00676 HAL_GPIO_Init(GPIOD, &gpio_init_structure); 00677 00678 /* NVIC configuration for SDIO interrupts */ 00679 HAL_NVIC_SetPriority(SDMMC1_IRQn, 0x0E, 0); 00680 HAL_NVIC_EnableIRQ(SDMMC1_IRQn); 00681 00682 dma_rx_handle.Init.Channel = SD1_DMAx_Rx_CHANNEL; 00683 dma_rx_handle.Init.Direction = DMA_PERIPH_TO_MEMORY; 00684 dma_rx_handle.Init.PeriphInc = DMA_PINC_DISABLE; 00685 dma_rx_handle.Init.MemInc = DMA_MINC_ENABLE; 00686 dma_rx_handle.Init.PeriphDataAlignment = DMA_PDATAALIGN_WORD; 00687 dma_rx_handle.Init.MemDataAlignment = DMA_MDATAALIGN_WORD; 00688 dma_rx_handle.Init.Mode = DMA_PFCTRL; 00689 dma_rx_handle.Init.Priority = DMA_PRIORITY_VERY_HIGH; 00690 dma_rx_handle.Init.FIFOMode = DMA_FIFOMODE_ENABLE; 00691 dma_rx_handle.Init.FIFOThreshold = DMA_FIFO_THRESHOLD_FULL; 00692 dma_rx_handle.Init.MemBurst = DMA_MBURST_INC4; 00693 dma_rx_handle.Init.PeriphBurst = DMA_PBURST_INC4; 00694 dma_rx_handle.Instance = SD1_DMAx_Rx_STREAM; 00695 00696 /* Associate the DMA handle */ 00697 __HAL_LINKDMA(hsd, hdmarx, dma_rx_handle); 00698 00699 /* Deinitialize the stream for new transfer */ 00700 HAL_DMA_DeInit(&dma_rx_handle); 00701 00702 /* Configure the DMA stream */ 00703 HAL_DMA_Init(&dma_rx_handle); 00704 00705 dma_tx_handle.Init.Channel = SD1_DMAx_Tx_CHANNEL; 00706 dma_tx_handle.Init.Direction = DMA_MEMORY_TO_PERIPH; 00707 dma_tx_handle.Init.PeriphInc = DMA_PINC_DISABLE; 00708 dma_tx_handle.Init.MemInc = DMA_MINC_ENABLE; 00709 dma_tx_handle.Init.PeriphDataAlignment = DMA_PDATAALIGN_WORD; 00710 dma_tx_handle.Init.MemDataAlignment = DMA_MDATAALIGN_WORD; 00711 dma_tx_handle.Init.Mode = DMA_PFCTRL; 00712 dma_tx_handle.Init.Priority = DMA_PRIORITY_VERY_HIGH; 00713 dma_tx_handle.Init.FIFOMode = DMA_FIFOMODE_ENABLE; 00714 dma_tx_handle.Init.FIFOThreshold = DMA_FIFO_THRESHOLD_FULL; 00715 dma_tx_handle.Init.MemBurst = DMA_MBURST_INC4; 00716 dma_tx_handle.Init.PeriphBurst = DMA_PBURST_INC4; 00717 dma_tx_handle.Instance = SD1_DMAx_Tx_STREAM; 00718 00719 /* Associate the DMA handle */ 00720 __HAL_LINKDMA(hsd, hdmatx, dma_tx_handle); 00721 00722 /* Deinitialize the stream for new transfer */ 00723 HAL_DMA_DeInit(&dma_tx_handle); 00724 00725 /* Configure the DMA stream */ 00726 HAL_DMA_Init(&dma_tx_handle); 00727 00728 /* NVIC configuration for DMA transfer complete interrupt */ 00729 HAL_NVIC_SetPriority(SD1_DMAx_Rx_IRQn, 0x0F, 0); 00730 HAL_NVIC_EnableIRQ(SD1_DMAx_Rx_IRQn); 00731 00732 /* NVIC configuration for DMA transfer complete interrupt */ 00733 HAL_NVIC_SetPriority(SD1_DMAx_Tx_IRQn, 0x0F, 0); 00734 HAL_NVIC_EnableIRQ(SD1_DMAx_Tx_IRQn); 00735 } 00736 else 00737 { 00738 /* Enable SDIO clock */ 00739 __HAL_RCC_SDMMC2_CLK_ENABLE(); 00740 00741 /* Enable DMA2 clocks */ 00742 __DMAx_TxRx_CLK_ENABLE(); 00743 00744 /* Enable GPIOs clock */ 00745 __HAL_RCC_GPIOB_CLK_ENABLE(); 00746 __HAL_RCC_GPIOD_CLK_ENABLE(); 00747 __HAL_RCC_GPIOG_CLK_ENABLE(); 00748 00749 /* Common GPIO configuration */ 00750 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00751 gpio_init_structure.Pull = GPIO_PULLUP; 00752 gpio_init_structure.Speed = GPIO_SPEED_HIGH; 00753 gpio_init_structure.Alternate = GPIO_AF10_SDMMC2; 00754 00755 /* GPIOB configuration: SD2_D2 and SD2_D3 pins */ 00756 gpio_init_structure.Pin = GPIO_PIN_3 | GPIO_PIN_4; 00757 00758 HAL_GPIO_Init(GPIOB, &gpio_init_structure); 00759 00760 /* GPIOD configuration: SD2_CLK and SD2_CMD pins */ 00761 gpio_init_structure.Pin = GPIO_PIN_6 | GPIO_PIN_7; 00762 gpio_init_structure.Alternate = GPIO_AF11_SDMMC2; 00763 HAL_GPIO_Init(GPIOD, &gpio_init_structure); 00764 00765 /* GPIOG configuration: SD2_D0 and SD2_D1 pins */ 00766 gpio_init_structure.Pin = GPIO_PIN_9 | GPIO_PIN_10; 00767 00768 HAL_GPIO_Init(GPIOG, &gpio_init_structure); 00769 00770 /* NVIC configuration for SDIO interrupts */ 00771 HAL_NVIC_SetPriority(SDMMC2_IRQn, 0x0E, 0); 00772 HAL_NVIC_EnableIRQ(SDMMC2_IRQn); 00773 00774 00775 dma_rx_handle2.Init.Channel = SD2_DMAx_Rx_CHANNEL; 00776 dma_rx_handle2.Init.Direction = DMA_PERIPH_TO_MEMORY; 00777 dma_rx_handle2.Init.PeriphInc = DMA_PINC_DISABLE; 00778 dma_rx_handle2.Init.MemInc = DMA_MINC_ENABLE; 00779 dma_rx_handle2.Init.PeriphDataAlignment = DMA_PDATAALIGN_WORD; 00780 dma_rx_handle2.Init.MemDataAlignment = DMA_MDATAALIGN_BYTE; 00781 dma_rx_handle2.Init.Mode = DMA_PFCTRL; 00782 dma_rx_handle2.Init.Priority = DMA_PRIORITY_VERY_HIGH; 00783 dma_rx_handle2.Init.FIFOMode = DMA_FIFOMODE_ENABLE; 00784 dma_rx_handle2.Init.FIFOThreshold = DMA_FIFO_THRESHOLD_FULL; 00785 dma_rx_handle2.Init.MemBurst = DMA_MBURST_INC16; 00786 dma_rx_handle2.Init.PeriphBurst = DMA_PBURST_INC4; 00787 dma_rx_handle2.Instance = SD2_DMAx_Rx_STREAM; 00788 00789 /* Associate the DMA handle */ 00790 __HAL_LINKDMA(hsd, hdmarx, dma_rx_handle2); 00791 00792 /* Deinitialize the stream for new transfer */ 00793 HAL_DMA_DeInit(&dma_rx_handle2); 00794 00795 /* Configure the DMA stream */ 00796 HAL_DMA_Init(&dma_rx_handle2); 00797 00798 dma_tx_handle2.Init.Channel = SD2_DMAx_Tx_CHANNEL; 00799 dma_tx_handle2.Init.Direction = DMA_MEMORY_TO_PERIPH; 00800 dma_tx_handle2.Init.PeriphInc = DMA_PINC_DISABLE; 00801 dma_tx_handle2.Init.MemInc = DMA_MINC_ENABLE; 00802 dma_tx_handle2.Init.PeriphDataAlignment = DMA_PDATAALIGN_WORD; 00803 dma_tx_handle2.Init.MemDataAlignment = DMA_MDATAALIGN_BYTE; 00804 dma_tx_handle2.Init.Mode = DMA_PFCTRL; 00805 dma_tx_handle2.Init.Priority = DMA_PRIORITY_VERY_HIGH; 00806 dma_tx_handle2.Init.FIFOMode = DMA_FIFOMODE_ENABLE; 00807 dma_tx_handle2.Init.FIFOThreshold = DMA_FIFO_THRESHOLD_FULL; 00808 dma_tx_handle2.Init.MemBurst = DMA_MBURST_INC16; 00809 dma_tx_handle2.Init.PeriphBurst = DMA_PBURST_INC4; 00810 dma_tx_handle2.Instance = SD2_DMAx_Tx_STREAM; 00811 00812 /* Associate the DMA handle */ 00813 __HAL_LINKDMA(hsd, hdmatx, dma_tx_handle2); 00814 00815 /* Deinitialize the stream for new transfer */ 00816 HAL_DMA_DeInit(&dma_tx_handle2); 00817 00818 /* Configure the DMA stream */ 00819 HAL_DMA_Init(&dma_tx_handle2); 00820 00821 /* NVIC configuration for DMA transfer complete interrupt */ 00822 HAL_NVIC_SetPriority(SD2_DMAx_Rx_IRQn, 0x0F, 0); 00823 HAL_NVIC_EnableIRQ(SD2_DMAx_Rx_IRQn); 00824 00825 /* NVIC configuration for DMA transfer complete interrupt */ 00826 HAL_NVIC_SetPriority(SD2_DMAx_Tx_IRQn, 0x0F, 0); 00827 HAL_NVIC_EnableIRQ(SD2_DMAx_Tx_IRQn); 00828 } 00829 } 00830 00831 /** 00832 * @brief DeInitializes the SD MSP. 00833 * @param hsd: SD handle 00834 * @param Params 00835 * @retval None 00836 */ 00837 __weak void BSP_SD_MspDeInit(SD_HandleTypeDef *hsd, void *Params) 00838 { 00839 static DMA_HandleTypeDef dma_rx_handle; 00840 static DMA_HandleTypeDef dma_tx_handle; 00841 static DMA_HandleTypeDef dma_rx_handle2; 00842 static DMA_HandleTypeDef dma_tx_handle2; 00843 00844 if(hsd->Instance == SDMMC1) 00845 { 00846 /* Disable NVIC for DMA transfer complete interrupts */ 00847 HAL_NVIC_DisableIRQ(SD1_DMAx_Rx_IRQn); 00848 HAL_NVIC_DisableIRQ(SD1_DMAx_Tx_IRQn); 00849 00850 /* Deinitialize the stream for new transfer */ 00851 dma_rx_handle.Instance = SD1_DMAx_Rx_STREAM; 00852 HAL_DMA_DeInit(&dma_rx_handle); 00853 00854 /* Deinitialize the stream for new transfer */ 00855 dma_tx_handle.Instance = SD1_DMAx_Tx_STREAM; 00856 HAL_DMA_DeInit(&dma_tx_handle); 00857 00858 /* Disable NVIC for SDIO interrupts */ 00859 HAL_NVIC_DisableIRQ(SDMMC1_IRQn); 00860 00861 /* DeInit GPIO pins can be done in the application 00862 (by surcharging this __weak function) */ 00863 00864 /* Disable SDMMC1 clock */ 00865 __HAL_RCC_SDMMC1_CLK_DISABLE(); 00866 } 00867 else 00868 { /* Disable NVIC for DMA transfer complete interrupts */ 00869 HAL_NVIC_DisableIRQ(SD2_DMAx_Rx_IRQn); 00870 HAL_NVIC_DisableIRQ(SD2_DMAx_Tx_IRQn); 00871 00872 /* Deinitialize the stream for new transfer */ 00873 dma_rx_handle2.Instance = SD2_DMAx_Rx_STREAM; 00874 HAL_DMA_DeInit(&dma_rx_handle2); 00875 00876 /* Deinitialize the stream for new transfer */ 00877 dma_tx_handle2.Instance = SD2_DMAx_Tx_STREAM; 00878 HAL_DMA_DeInit(&dma_tx_handle2); 00879 00880 /* Disable NVIC for SDIO interrupts */ 00881 HAL_NVIC_DisableIRQ(SDMMC2_IRQn); 00882 00883 /* DeInit GPIO pins can be done in the application 00884 (by surcharging this __weak function) */ 00885 00886 /* Disable SDMMC2 clock */ 00887 __HAL_RCC_SDMMC2_CLK_DISABLE(); 00888 } 00889 /* GPIO pins clock and DMA clocks can be shut down in the application 00890 by surcharging this __weak function */ 00891 } 00892 00893 /** 00894 * @brief Gets the current SD card data status. 00895 * @retval Data transfer state. 00896 * This value can be one of the following values: 00897 * @arg SD_TRANSFER_OK: No data transfer is acting 00898 * @arg SD_TRANSFER_BUSY: Data transfer is acting 00899 */ 00900 uint8_t BSP_SD_GetCardState(void) 00901 { 00902 return BSP_SD_GetCardStateEx(SD_CARD1); 00903 } 00904 00905 /** 00906 * @brief Gets the current SD card data status. 00907 * @param SdCard: SD_CARD1 or SD_CARD2 00908 * @retval Data transfer state. 00909 * This value can be one of the following values: 00910 * @arg SD_TRANSFER_OK: No data transfer is acting 00911 * @arg SD_TRANSFER_BUSY: Data transfer is acting 00912 */ 00913 uint8_t BSP_SD_GetCardStateEx(uint32_t SdCard) 00914 { 00915 if(SdCard == SD_CARD1) 00916 { 00917 return((HAL_SD_GetCardState(&uSdHandle) == HAL_SD_CARD_TRANSFER ) ? SD_TRANSFER_OK : SD_TRANSFER_BUSY); 00918 } 00919 else 00920 { 00921 return((HAL_SD_GetCardState(&uSdHandle2) == HAL_SD_CARD_TRANSFER ) ? SD_TRANSFER_OK : SD_TRANSFER_BUSY); 00922 } 00923 } 00924 00925 /** 00926 * @brief Get SD information about specific SD card. 00927 * @param CardInfo: Pointer to HAL_SD_CardInfoTypedef structure 00928 * @retval None 00929 */ 00930 void BSP_SD_GetCardInfo(BSP_SD_CardInfo *CardInfo) 00931 { 00932 BSP_SD_GetCardInfoEx(SD_CARD1, CardInfo); 00933 } 00934 00935 /** 00936 * @brief Get SD information about specific SD card. 00937 * @param SdCard: SD card to be used, that should be SD_CARD1 or SD_CARD2 00938 * @param CardInfo: Pointer to HAL_SD_CardInfoTypedef structure 00939 * @retval None 00940 */ 00941 void BSP_SD_GetCardInfoEx(uint32_t SdCard, BSP_SD_CardInfo *CardInfo) 00942 { 00943 /* Get SD card Information */ 00944 if(SdCard == SD_CARD1) 00945 { 00946 HAL_SD_GetCardInfo(&uSdHandle, CardInfo); 00947 } 00948 else 00949 { 00950 HAL_SD_GetCardInfo(&uSdHandle2, CardInfo); 00951 } 00952 } 00953 00954 /** 00955 * @brief SD Abort callbacks 00956 * @param hsd: SD handle 00957 * @retval None 00958 */ 00959 void HAL_SD_AbortCallback(SD_HandleTypeDef *hsd) 00960 { 00961 BSP_SD_AbortCallback((hsd == &uSdHandle) ? SD_CARD1 : SD_CARD2); 00962 } 00963 00964 /** 00965 * @brief Tx Transfer completed callbacks 00966 * @param hsd: SD handle 00967 * @retval None 00968 */ 00969 void HAL_SD_TxCpltCallback(SD_HandleTypeDef *hsd) 00970 { 00971 BSP_SD_WriteCpltCallback((hsd == &uSdHandle) ? SD_CARD1 : SD_CARD2); 00972 } 00973 00974 /** 00975 * @brief Rx Transfer completed callbacks 00976 * @param hsd: SD handle 00977 * @retval None 00978 */ 00979 void HAL_SD_RxCpltCallback(SD_HandleTypeDef *hsd) 00980 { 00981 BSP_SD_ReadCpltCallback((hsd == &uSdHandle) ? SD_CARD1 : SD_CARD2); 00982 } 00983 00984 /** 00985 * @brief BSP SD Abort callbacks 00986 * @param SdCard: SD_CARD1 or SD_CARD2 00987 * @retval None 00988 */ 00989 __weak void BSP_SD_AbortCallback(uint32_t SdCard) 00990 { 00991 00992 } 00993 00994 /** 00995 * @brief BSP Tx Transfer completed callbacks 00996 * @param SdCard: SD_CARD1 or SD_CARD2 00997 * @retval None 00998 */ 00999 __weak void BSP_SD_WriteCpltCallback(uint32_t SdCard) 01000 { 01001 01002 } 01003 01004 /** 01005 * @brief BSP Rx Transfer completed callbacks 01006 * @param SdCard: SD_CARD1 or SD_CARD2 01007 * @retval None 01008 */ 01009 __weak void BSP_SD_ReadCpltCallback(uint32_t SdCard) 01010 { 01011 01012 } 01013 /** 01014 * @} 01015 */ 01016 01017 /** 01018 * @} 01019 */ 01020 01021 /** 01022 * @} 01023 */ 01024 01025 /** 01026 * @} 01027 */ 01028 01029 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Thu May 25 2017 11:03:11 for STM32769I_EVAL BSP User Manual by
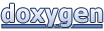