STM324x9I_EVAL BSP User Manual
|
Functions | |
uint32_t | BSP_GetVersion (void) |
This method returns the STM324x9I EVAL BSP Driver revision. | |
void | BSP_LED_Init (Led_TypeDef Led) |
Configures LED GPIO. | |
void | BSP_LED_On (Led_TypeDef Led) |
Turns selected LED On. | |
void | BSP_LED_Off (Led_TypeDef Led) |
Turns selected LED Off. | |
void | BSP_LED_Toggle (Led_TypeDef Led) |
Toggles the selected LED. | |
void | BSP_PB_Init (Button_TypeDef Button, ButtonMode_TypeDef Button_Mode) |
Configures button GPIO and EXTI Line. | |
uint32_t | BSP_PB_GetState (Button_TypeDef Button) |
Returns the selected button state. | |
void | BSP_COM_Init (COM_TypeDef COM, UART_HandleTypeDef *huart) |
Configures COM port. | |
uint8_t | BSP_JOY_Init (JOYMode_TypeDef Joy_Mode) |
Configures joystick GPIO and EXTI modes. | |
JOYState_TypeDef | BSP_JOY_GetState (void) |
Returns the current joystick status. | |
uint8_t | BSP_TS3510_IsDetected (void) |
Check TS3510 touch screen presence. | |
static void | I2Cx_Write (uint8_t Addr, uint8_t Reg, uint8_t Value) |
Writes a single data. | |
static uint8_t | I2Cx_Read (uint8_t Addr, uint8_t Reg) |
Reads a single data. | |
static HAL_StatusTypeDef | I2Cx_ReadMultiple (uint8_t Addr, uint16_t Reg, uint16_t MemAddress, uint8_t *Buffer, uint16_t Length) |
Reads multiple data. | |
static HAL_StatusTypeDef | I2Cx_WriteMultiple (uint8_t Addr, uint16_t Reg, uint16_t MemAddress, uint8_t *Buffer, uint16_t Length) |
Writes a value in a register of the device through BUS in using DMA mode. | |
static HAL_StatusTypeDef | I2Cx_IsDeviceReady (uint16_t DevAddress, uint32_t Trials) |
Checks if target device is ready for communication. | |
static void | I2Cx_Error (uint8_t Addr) |
Manages error callback by re-initializing I2C. | |
void | IOE_Write (uint8_t Addr, uint8_t Reg, uint8_t Value) |
IOE writes single data. | |
uint8_t | IOE_Read (uint8_t Addr, uint8_t Reg) |
IOE reads single data. | |
uint16_t | IOE_ReadMultiple (uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length) |
IOE reads multiple data. | |
void | IOE_WriteMultiple (uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length) |
IOE writes multiple data. | |
void | IOE_Delay (uint32_t Delay) |
IOE delay. | |
void | AUDIO_IO_Write (uint8_t Addr, uint16_t Reg, uint16_t Value) |
Writes a single data. | |
uint16_t | AUDIO_IO_Read (uint8_t Addr, uint16_t Reg) |
Reads a single data. | |
void | AUDIO_IO_Delay (uint32_t Delay) |
AUDIO Codec delay. | |
void | CAMERA_IO_Write (uint8_t Addr, uint8_t Reg, uint8_t Value) |
Camera writes single data. | |
uint8_t | CAMERA_IO_Read (uint8_t Addr, uint8_t Reg) |
Camera reads single data. | |
void | CAMERA_Delay (uint32_t Delay) |
Camera delay. | |
HAL_StatusTypeDef | EEPROM_IO_WriteData (uint16_t DevAddress, uint16_t MemAddress, uint8_t *pBuffer, uint32_t BufferSize) |
Write data to I2C EEPROM driver in using DMA channel. | |
HAL_StatusTypeDef | EEPROM_IO_ReadData (uint16_t DevAddress, uint16_t MemAddress, uint8_t *pBuffer, uint32_t BufferSize) |
Read data from I2C EEPROM driver in using DMA channel. | |
HAL_StatusTypeDef | EEPROM_IO_IsDeviceReady (uint16_t DevAddress, uint32_t Trials) |
Checks if target device is ready for communication. |
Function Documentation
void AUDIO_IO_Delay | ( | uint32_t | Delay | ) |
uint16_t AUDIO_IO_Read | ( | uint8_t | Addr, |
uint16_t | Reg | ||
) |
Reads a single data.
- Parameters:
-
Addr,: I2C address Reg,: Reg address
- Return values:
-
Data to be read
Definition at line 817 of file stm324x9i_eval.c.
References I2Cx_ReadMultiple().
void AUDIO_IO_Write | ( | uint8_t | Addr, |
uint16_t | Reg, | ||
uint16_t | Value | ||
) |
Writes a single data.
- Parameters:
-
Addr,: I2C address Reg,: Reg address Value,: Data to be written
Definition at line 800 of file stm324x9i_eval.c.
References I2Cx_WriteMultiple().
void BSP_COM_Init | ( | COM_TypeDef | COM, |
UART_HandleTypeDef * | huart | ||
) |
Configures COM port.
- Parameters:
-
COM,: COM port to be configured. This parameter can be one of the following values: - COM1
- COM2
huart,: Pointer to a UART_HandleTypeDef structure that contains the configuration information for the specified USART peripheral.
Definition at line 342 of file stm324x9i_eval.c.
References COM_RX_AF, COM_RX_PIN, COM_RX_PORT, COM_TX_AF, COM_TX_PIN, COM_TX_PORT, COM_USART, EVAL_COMx_CLK_ENABLE, EVAL_COMx_RX_GPIO_CLK_ENABLE, and EVAL_COMx_TX_GPIO_CLK_ENABLE.
uint32_t BSP_GetVersion | ( | void | ) |
This method returns the STM324x9I EVAL BSP Driver revision.
- Return values:
-
version,: 0xXYZR (8bits for each decimal, R for RC)
Definition at line 192 of file stm324x9i_eval.c.
References __STM324x9I_EVAL_BSP_VERSION.
JOYState_TypeDef BSP_JOY_GetState | ( | void | ) |
Returns the current joystick status.
- Return values:
-
Code of the joystick key pressed This code can be one of the following values: - JOY_NONE
- JOY_SEL
- JOY_DOWN
- JOY_LEFT
- JOY_RIGHT
- JOY_UP
Definition at line 409 of file stm324x9i_eval.c.
References BSP_IO_ReadPin(), JOY_ALL_PINS, JOY_DOWN, JOY_DOWN_PIN, JOY_LEFT, JOY_LEFT_PIN, JOY_NONE, JOY_NONE_PIN, JOY_RIGHT, JOY_RIGHT_PIN, JOY_SEL, JOY_SEL_PIN, JOY_UP, and JOY_UP_PIN.
uint8_t BSP_JOY_Init | ( | JOYMode_TypeDef | Joy_Mode | ) |
Configures joystick GPIO and EXTI modes.
- Parameters:
-
Joy_Mode,: Button mode. This parameter can be one of the following values: - JOY_MODE_GPIO: Joystick pins will be used as simple IOs
- JOY_MODE_EXTI: Joystick pins will be connected to EXTI line with interrupt generation capability
- Return values:
-
IO_OK,: if all initializations are OK. Other value if error.
Definition at line 381 of file stm324x9i_eval.c.
References BSP_IO_ConfigPin(), BSP_IO_Init(), JOY_ALL_PINS, and JOY_MODE_EXTI.
void BSP_LED_Init | ( | Led_TypeDef | Led | ) |
Configures LED GPIO.
- Parameters:
-
Led,: LED to be configured. This parameter can be one of the following values: - LED1
- LED2
- LED3
- LED4
Definition at line 206 of file stm324x9i_eval.c.
References GPIO_PIN, GPIO_PORT, and LEDx_GPIO_CLK_ENABLE.
void BSP_LED_Off | ( | Led_TypeDef | Led | ) |
Turns selected LED Off.
- Parameters:
-
Led,: LED to be set off This parameter can be one of the following values: - LED1
- LED2
- LED3
- LED4
Definition at line 247 of file stm324x9i_eval.c.
void BSP_LED_On | ( | Led_TypeDef | Led | ) |
Turns selected LED On.
- Parameters:
-
Led,: LED to be set on This parameter can be one of the following values: - LED1
- LED2
- LED3
- LED4
Definition at line 233 of file stm324x9i_eval.c.
void BSP_LED_Toggle | ( | Led_TypeDef | Led | ) |
Toggles the selected LED.
- Parameters:
-
Led,: LED to be toggled This parameter can be one of the following values: - LED1
- LED2
- LED3
- LED4
Definition at line 261 of file stm324x9i_eval.c.
uint32_t BSP_PB_GetState | ( | Button_TypeDef | Button | ) |
Returns the selected button state.
- Parameters:
-
Button,: Button to be checked This parameter can be one of the following values: - BUTTON_WAKEUP: Wakeup Push Button
- BUTTON_TAMPER: Tamper Push Button
- BUTTON_KEY: Key Push Button
- Return values:
-
The Button GPIO pin value
Definition at line 328 of file stm324x9i_eval.c.
References BUTTON_PIN, and BUTTON_PORT.
void BSP_PB_Init | ( | Button_TypeDef | Button, |
ButtonMode_TypeDef | Button_Mode | ||
) |
Configures button GPIO and EXTI Line.
- Parameters:
-
Button,: Button to be configured This parameter can be one of the following values: - BUTTON_WAKEUP: Wakeup Push Button
- BUTTON_TAMPER: Tamper Push Button
Button_Mode,: Button mode This parameter can be one of the following values: - BUTTON_MODE_GPIO: Button will be used as simple IO
- BUTTON_MODE_EXTI: Button will be connected to EXTI line with interrupt generation capability
Definition at line 278 of file stm324x9i_eval.c.
References BUTTON_IRQn, BUTTON_MODE_EXTI, BUTTON_MODE_GPIO, BUTTON_PIN, BUTTON_PORT, BUTTON_WAKEUP, and BUTTONx_GPIO_CLK_ENABLE.
uint8_t BSP_TS3510_IsDetected | ( | void | ) |
Check TS3510 touch screen presence.
- Return values:
-
Return 0 if TS3510 is detected, return 1 if not detected
Definition at line 451 of file stm324x9i_eval.c.
References heval_I2c, I2Cx_Error(), IOE_WriteMultiple(), and TS3510_I2C_ADDRESS.
Referenced by BSP_TS_Init().
void CAMERA_Delay | ( | uint32_t | Delay | ) |
uint8_t CAMERA_IO_Read | ( | uint8_t | Addr, |
uint8_t | Reg | ||
) |
Camera reads single data.
- Parameters:
-
Addr,: I2C address Reg,: Register address
- Return values:
-
Read data
Definition at line 868 of file stm324x9i_eval.c.
References I2Cx_Read().
void CAMERA_IO_Write | ( | uint8_t | Addr, |
uint8_t | Reg, | ||
uint8_t | Value | ||
) |
Camera writes single data.
- Parameters:
-
Addr,: I2C address Reg,: Register address Value,: Data to be written
Definition at line 857 of file stm324x9i_eval.c.
References I2Cx_Write().
HAL_StatusTypeDef EEPROM_IO_IsDeviceReady | ( | uint16_t | DevAddress, |
uint32_t | Trials | ||
) |
Checks if target device is ready for communication.
- Note:
- This function is used with Memory devices
- Parameters:
-
DevAddress,: Target device address Trials,: Number of trials
- Return values:
-
HAL status
Definition at line 925 of file stm324x9i_eval.c.
References I2Cx_IsDeviceReady().
Referenced by BSP_EEPROM_Init(), and BSP_EEPROM_WaitEepromStandbyState().
HAL_StatusTypeDef EEPROM_IO_ReadData | ( | uint16_t | DevAddress, |
uint16_t | MemAddress, | ||
uint8_t * | pBuffer, | ||
uint32_t | BufferSize | ||
) |
Read data from I2C EEPROM driver in using DMA channel.
- Parameters:
-
DevAddress,: Target device address MemAddress,: Internal memory address pBuffer,: Pointer to data buffer BufferSize,: Amount of data to be read
- Return values:
-
HAL status
Definition at line 913 of file stm324x9i_eval.c.
References I2Cx_ReadMultiple().
Referenced by BSP_EEPROM_ReadBuffer().
HAL_StatusTypeDef EEPROM_IO_WriteData | ( | uint16_t | DevAddress, |
uint16_t | MemAddress, | ||
uint8_t * | pBuffer, | ||
uint32_t | BufferSize | ||
) |
Write data to I2C EEPROM driver in using DMA channel.
- Parameters:
-
DevAddress,: Target device address MemAddress,: Internal memory address pBuffer,: Pointer to data buffer BufferSize,: Amount of data to be sent
- Return values:
-
HAL status
Definition at line 900 of file stm324x9i_eval.c.
References I2Cx_WriteMultiple().
Referenced by BSP_EEPROM_WritePage().
static void I2Cx_Error | ( | uint8_t | Addr | ) | [static] |
Manages error callback by re-initializing I2C.
- Parameters:
-
Addr,: I2C Address
Definition at line 689 of file stm324x9i_eval.c.
References heval_I2c, and I2Cx_Init().
Referenced by BSP_TS3510_IsDetected(), I2Cx_Read(), I2Cx_ReadMultiple(), I2Cx_Write(), and I2Cx_WriteMultiple().
static HAL_StatusTypeDef I2Cx_IsDeviceReady | ( | uint16_t | DevAddress, |
uint32_t | Trials | ||
) | [static] |
Checks if target device is ready for communication.
- Note:
- This function is used with Memory devices
- Parameters:
-
DevAddress,: Target device address Trials,: Number of trials
- Return values:
-
HAL status
Definition at line 680 of file stm324x9i_eval.c.
References heval_I2c.
Referenced by EEPROM_IO_IsDeviceReady().
static uint8_t I2Cx_Read | ( | uint8_t | Addr, |
uint8_t | Reg | ||
) | [static] |
Reads a single data.
- Parameters:
-
Addr,: I2C address Reg,: Register address
- Return values:
-
Read data
Definition at line 602 of file stm324x9i_eval.c.
References heval_I2c, and I2Cx_Error().
Referenced by CAMERA_IO_Read(), and IOE_Read().
static HAL_StatusTypeDef I2Cx_ReadMultiple | ( | uint8_t | Addr, |
uint16_t | Reg, | ||
uint16_t | MemAddress, | ||
uint8_t * | Buffer, | ||
uint16_t | Length | ||
) | [static] |
Reads multiple data.
- Parameters:
-
Addr,: I2C address Reg,: Reg address MemAddress,: Internal memory address Buffer,: Pointer to data buffer Length,: Length of the data
- Return values:
-
Number of read data
Definition at line 627 of file stm324x9i_eval.c.
References EXC7200_I2C_ADDRESS, heval_I2c, and I2Cx_Error().
Referenced by AUDIO_IO_Read(), EEPROM_IO_ReadData(), and IOE_ReadMultiple().
static void I2Cx_Write | ( | uint8_t | Addr, |
uint8_t | Reg, | ||
uint8_t | Value | ||
) | [static] |
Writes a single data.
- Parameters:
-
Addr,: I2C address Reg,: Register address Value,: Data to be written
Definition at line 582 of file stm324x9i_eval.c.
References heval_I2c, and I2Cx_Error().
Referenced by CAMERA_IO_Write(), and IOE_Write().
static HAL_StatusTypeDef I2Cx_WriteMultiple | ( | uint8_t | Addr, |
uint16_t | Reg, | ||
uint16_t | MemAddress, | ||
uint8_t * | Buffer, | ||
uint16_t | Length | ||
) | [static] |
Writes a value in a register of the device through BUS in using DMA mode.
- Parameters:
-
Addr,: Device address on BUS Bus. Reg,: The target register address to write MemAddress,: Internal memory address Buffer,: The target register value to be written Length,: buffer size to be written
- Return values:
-
HAL status
Definition at line 658 of file stm324x9i_eval.c.
References heval_I2c, and I2Cx_Error().
Referenced by AUDIO_IO_Write(), EEPROM_IO_WriteData(), and IOE_WriteMultiple().
void IOE_Delay | ( | uint32_t | Delay | ) |
uint8_t IOE_Read | ( | uint8_t | Addr, |
uint8_t | Reg | ||
) |
IOE reads single data.
- Parameters:
-
Addr,: I2C address Reg,: Register address
- Return values:
-
Read data
Definition at line 737 of file stm324x9i_eval.c.
References I2Cx_Read().
uint16_t IOE_ReadMultiple | ( | uint8_t | Addr, |
uint8_t | Reg, | ||
uint8_t * | Buffer, | ||
uint16_t | Length | ||
) |
IOE reads multiple data.
- Parameters:
-
Addr,: I2C address Reg,: Register address Buffer,: Pointer to data buffer Length,: Length of the data
- Return values:
-
Number of read data
Definition at line 750 of file stm324x9i_eval.c.
References I2Cx_ReadMultiple().
void IOE_Write | ( | uint8_t | Addr, |
uint8_t | Reg, | ||
uint8_t | Value | ||
) |
IOE writes single data.
- Parameters:
-
Addr,: I2C address Reg,: Register address Value,: Data to be written
Definition at line 726 of file stm324x9i_eval.c.
References I2Cx_Write().
void IOE_WriteMultiple | ( | uint8_t | Addr, |
uint8_t | Reg, | ||
uint8_t * | Buffer, | ||
uint16_t | Length | ||
) |
IOE writes multiple data.
- Parameters:
-
Addr,: I2C address Reg,: Register address Buffer,: Pointer to data buffer Length,: Length of the data
Definition at line 762 of file stm324x9i_eval.c.
References I2Cx_WriteMultiple().
Referenced by BSP_TS3510_IsDetected().
Generated on Wed Jan 13 2016 15:52:54 for STM324x9I_EVAL BSP User Manual by
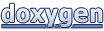