STM324x9I_EVAL BSP User Manual
|
Functions | |
uint8_t | BSP_LCD_Init (void) |
Initializes the LCD. | |
uint8_t | BSP_LCD_InitEx (uint32_t PclkConfig) |
Initializes the LCD. | |
uint32_t | BSP_LCD_GetXSize (void) |
Gets the LCD X size. | |
uint32_t | BSP_LCD_GetYSize (void) |
Gets the LCD Y size. | |
void | BSP_LCD_LayerDefaultInit (uint16_t LayerIndex, uint32_t FrameBuffer) |
Initializes the LCD layers. | |
void | BSP_LCD_SetTransparency (uint32_t LayerIndex, uint8_t Transparency) |
Configures the transparency. | |
void | BSP_LCD_SetLayerAddress (uint32_t LayerIndex, uint32_t Address) |
Sets an LCD layer frame buffer address. | |
void | BSP_LCD_SetColorKeying (uint32_t LayerIndex, uint32_t RGBValue) |
Configures and sets the color keying. | |
void | BSP_LCD_ResetColorKeying (uint32_t LayerIndex) |
Disables the color keying. | |
void | BSP_LCD_SetLayerWindow (uint16_t LayerIndex, uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) |
Sets display window. | |
void | BSP_LCD_SelectLayer (uint32_t LayerIndex) |
Selects the LCD Layer. | |
void | BSP_LCD_SetLayerVisible (uint32_t LayerIndex, FunctionalState State) |
Sets an LCD Layer visible. | |
void | BSP_LCD_SetTextColor (uint32_t Color) |
Sets the LCD text color. | |
uint32_t | BSP_LCD_GetTextColor (void) |
Gets the LCD text color. | |
void | BSP_LCD_SetBackColor (uint32_t Color) |
Sets the LCD background color. | |
uint32_t | BSP_LCD_GetBackColor (void) |
Gets the LCD background color. | |
void | BSP_LCD_SetFont (sFONT *fonts) |
Sets the LCD text font. | |
sFONT * | BSP_LCD_GetFont (void) |
Gets the LCD text font. | |
uint32_t | BSP_LCD_ReadPixel (uint16_t Xpos, uint16_t Ypos) |
Reads an LCD pixel. | |
void | BSP_LCD_DrawPixel (uint16_t Xpos, uint16_t Ypos, uint32_t pixel) |
Draws a pixel on LCD. | |
void | BSP_LCD_Clear (uint32_t Color) |
Clears the hole LCD. | |
void | BSP_LCD_ClearStringLine (uint32_t Line) |
Clears the selected line. | |
void | BSP_LCD_DisplayStringAtLine (uint16_t Line, uint8_t *ptr) |
Displays a maximum of 60 characters on the LCD. | |
void | BSP_LCD_DisplayStringAt (uint16_t Xpos, uint16_t Ypos, uint8_t *Text, Text_AlignModeTypdef Mode) |
Displays characters on the LCD. | |
void | BSP_LCD_DisplayChar (uint16_t Xpos, uint16_t Ypos, uint8_t Ascii) |
Displays one character. | |
void | BSP_LCD_DrawHLine (uint16_t Xpos, uint16_t Ypos, uint16_t Length) |
Draws an horizontal line. | |
void | BSP_LCD_DrawVLine (uint16_t Xpos, uint16_t Ypos, uint16_t Length) |
Draws a vertical line. | |
void | BSP_LCD_DrawLine (uint16_t x1, uint16_t y1, uint16_t x2, uint16_t y2) |
Draws an uni-line (between two points). | |
void | BSP_LCD_DrawRect (uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) |
Draws a rectangle. | |
void | BSP_LCD_DrawCircle (uint16_t Xpos, uint16_t Ypos, uint16_t Radius) |
Draws a circle. | |
void | BSP_LCD_DrawPolygon (pPoint Points, uint16_t PointCount) |
Draws an poly-line (between many points). | |
void | BSP_LCD_DrawEllipse (int Xpos, int Ypos, int XRadius, int YRadius) |
Draws an ellipse on LCD. | |
void | BSP_LCD_DrawBitmap (uint32_t Xpos, uint32_t Ypos, uint8_t *pbmp) |
Draws a bitmap picture loaded in the internal Flash (32 bpp). | |
void | BSP_LCD_FillRect (uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) |
Draws a full rectangle. | |
void | BSP_LCD_FillCircle (uint16_t Xpos, uint16_t Ypos, uint16_t Radius) |
Draws a full circle. | |
void | BSP_LCD_FillPolygon (pPoint Points, uint16_t PointCount) |
Draws a full poly-line (between many points). | |
void | BSP_LCD_FillEllipse (int Xpos, int Ypos, int XRadius, int YRadius) |
Draws a full ellipse. | |
void | BSP_LCD_DisplayOff (void) |
Disables the display. | |
void | BSP_LCD_DisplayOn (void) |
Enables the display. | |
void | BSP_LCD_ClockConfig (LTDC_HandleTypeDef *hltdc, void *Params) |
Clock Config. |
Function Documentation
void BSP_LCD_Clear | ( | uint32_t | Color | ) |
Clears the hole LCD.
- Parameters:
-
Color,: Color of the background
Definition at line 471 of file stm324x9i_eval_lcd.c.
References ActiveLayer, BSP_LCD_GetXSize(), BSP_LCD_GetYSize(), hltdc_eval, and LL_FillBuffer().
void BSP_LCD_ClearStringLine | ( | uint32_t | Line | ) |
Clears the selected line.
- Parameters:
-
Line,: Line to be cleared
Definition at line 481 of file stm324x9i_eval_lcd.c.
References ActiveLayer, LCD_DrawPropTypeDef::BackColor, BSP_LCD_FillRect(), BSP_LCD_GetXSize(), BSP_LCD_SetTextColor(), and LCD_DrawPropTypeDef::TextColor.
void BSP_LCD_ClockConfig | ( | LTDC_HandleTypeDef * | hltdc, |
void * | Params | ||
) |
Clock Config.
- Parameters:
-
hltdc,: LTDC handle Params,: LTDC pixel clock
- Note:
- This API is called by BSP_LCD_Init() Being __weak it can be overwritten by the application
Definition at line 1108 of file stm324x9i_eval_lcd.c.
References LCD_MAX_PCLK, and TS_I2C_ADDRESS.
Referenced by BSP_LCD_InitEx().
void BSP_LCD_DisplayChar | ( | uint16_t | Xpos, |
uint16_t | Ypos, | ||
uint8_t | Ascii | ||
) |
Displays one character.
- Parameters:
-
Xpos,: Start column address Ypos,: Line where to display the character shape. Ascii,: Character ascii code This parameter must be a number between Min_Data = 0x20 and Max_Data = 0x7E
Definition at line 500 of file stm324x9i_eval_lcd.c.
References ActiveLayer, DrawChar(), and LCD_DrawPropTypeDef::pFont.
Referenced by BSP_LCD_DisplayStringAt().
void BSP_LCD_DisplayOff | ( | void | ) |
void BSP_LCD_DisplayOn | ( | void | ) |
void BSP_LCD_DisplayStringAt | ( | uint16_t | Xpos, |
uint16_t | Ypos, | ||
uint8_t * | Text, | ||
Text_AlignModeTypdef | Mode | ||
) |
Displays characters on the LCD.
- Parameters:
-
Xpos,: X position (in pixel) Ypos,: Y position (in pixel) Text,: Pointer to string to display on LCD Mode,: Display mode This parameter can be one of the following values: - CENTER_MODE
- RIGHT_MODE
- LEFT_MODE
Definition at line 517 of file stm324x9i_eval_lcd.c.
References ActiveLayer, BSP_LCD_DisplayChar(), BSP_LCD_GetXSize(), CENTER_MODE, LEFT_MODE, LCD_DrawPropTypeDef::pFont, and RIGHT_MODE.
Referenced by BSP_LCD_DisplayStringAtLine().
void BSP_LCD_DisplayStringAtLine | ( | uint16_t | Line, |
uint8_t * | ptr | ||
) |
Displays a maximum of 60 characters on the LCD.
- Parameters:
-
Line,: Line where to display the character shape ptr,: Pointer to string to display on LCD
Definition at line 571 of file stm324x9i_eval_lcd.c.
References BSP_LCD_DisplayStringAt(), and LEFT_MODE.
void BSP_LCD_DrawBitmap | ( | uint32_t | Xpos, |
uint32_t | Ypos, | ||
uint8_t * | pbmp | ||
) |
Draws a bitmap picture loaded in the internal Flash (32 bpp).
- Parameters:
-
Xpos,: Bmp X position in the LCD Ypos,: Bmp Y position in the LCD pbmp,: Pointer to Bmp picture address in the internal Flash
Definition at line 813 of file stm324x9i_eval_lcd.c.
References ActiveLayer, BSP_LCD_GetXSize(), hltdc_eval, and LL_ConvertLineToARGB8888().
void BSP_LCD_DrawCircle | ( | uint16_t | Xpos, |
uint16_t | Ypos, | ||
uint16_t | Radius | ||
) |
Draws a circle.
- Parameters:
-
Xpos,: X position Ypos,: Y position Radius,: Circle radius
Definition at line 708 of file stm324x9i_eval_lcd.c.
References ActiveLayer, and BSP_LCD_DrawPixel().
Referenced by BSP_LCD_FillCircle().
void BSP_LCD_DrawEllipse | ( | int | Xpos, |
int | Ypos, | ||
int | XRadius, | ||
int | YRadius | ||
) |
Draws an ellipse on LCD.
- Parameters:
-
Xpos,: X position Ypos,: Y position XRadius,: Ellipse X radius YRadius,: Ellipse Y radius
Definition at line 781 of file stm324x9i_eval_lcd.c.
References ActiveLayer, and BSP_LCD_DrawPixel().
void BSP_LCD_DrawHLine | ( | uint16_t | Xpos, |
uint16_t | Ypos, | ||
uint16_t | Length | ||
) |
Draws an horizontal line.
- Parameters:
-
Xpos,: X position Ypos,: Y position Length,: Line length
Definition at line 582 of file stm324x9i_eval_lcd.c.
References ActiveLayer, BSP_LCD_GetXSize(), hltdc_eval, and LL_FillBuffer().
Referenced by BSP_LCD_DrawRect(), BSP_LCD_FillCircle(), and BSP_LCD_FillEllipse().
void BSP_LCD_DrawLine | ( | uint16_t | x1, |
uint16_t | y1, | ||
uint16_t | x2, | ||
uint16_t | y2 | ||
) |
Draws an uni-line (between two points).
- Parameters:
-
x1,: Point 1 X position y1,: Point 1 Y position x2,: Point 2 X position y2,: Point 2 Y position
Definition at line 617 of file stm324x9i_eval_lcd.c.
References ABS, ActiveLayer, and BSP_LCD_DrawPixel().
Referenced by BSP_LCD_DrawPolygon(), and FillTriangle().
void BSP_LCD_DrawPixel | ( | uint16_t | Xpos, |
uint16_t | Ypos, | ||
uint32_t | RGB_Code | ||
) |
Draws a pixel on LCD.
- Parameters:
-
Xpos,: X position Ypos,: Y position RGB_Code,: Pixel color in ARGB mode (8-8-8-8)
Definition at line 1168 of file stm324x9i_eval_lcd.c.
References ActiveLayer, BSP_LCD_GetXSize(), and hltdc_eval.
Referenced by BSP_LCD_DrawCircle(), BSP_LCD_DrawEllipse(), BSP_LCD_DrawLine(), and DrawChar().
void BSP_LCD_DrawPolygon | ( | pPoint | Points, |
uint16_t | PointCount | ||
) |
Draws an poly-line (between many points).
- Parameters:
-
Points,: Pointer to the points array PointCount,: Number of points
Definition at line 754 of file stm324x9i_eval_lcd.c.
References BSP_LCD_DrawLine(), Point::X, and Point::Y.
void BSP_LCD_DrawRect | ( | uint16_t | Xpos, |
uint16_t | Ypos, | ||
uint16_t | Width, | ||
uint16_t | Height | ||
) |
Draws a rectangle.
- Parameters:
-
Xpos,: X position Ypos,: Y position Width,: Rectangle width Height,: Rectangle height
Definition at line 691 of file stm324x9i_eval_lcd.c.
References BSP_LCD_DrawHLine(), and BSP_LCD_DrawVLine().
void BSP_LCD_DrawVLine | ( | uint16_t | Xpos, |
uint16_t | Ypos, | ||
uint16_t | Length | ||
) |
Draws a vertical line.
- Parameters:
-
Xpos,: X position Ypos,: Y position Length,: Line length
Definition at line 599 of file stm324x9i_eval_lcd.c.
References ActiveLayer, BSP_LCD_GetXSize(), hltdc_eval, and LL_FillBuffer().
Referenced by BSP_LCD_DrawRect().
void BSP_LCD_FillCircle | ( | uint16_t | Xpos, |
uint16_t | Ypos, | ||
uint16_t | Radius | ||
) |
Draws a full circle.
- Parameters:
-
Xpos,: X position Ypos,: Y position Radius,: Circle radius
Definition at line 893 of file stm324x9i_eval_lcd.c.
References ActiveLayer, BSP_LCD_DrawCircle(), BSP_LCD_DrawHLine(), and BSP_LCD_SetTextColor().
void BSP_LCD_FillEllipse | ( | int | Xpos, |
int | Ypos, | ||
int | XRadius, | ||
int | YRadius | ||
) |
Draws a full ellipse.
- Parameters:
-
Xpos,: X position Ypos,: Y position XRadius,: Ellipse X radius YRadius,: Ellipse Y radius
Definition at line 1007 of file stm324x9i_eval_lcd.c.
References BSP_LCD_DrawHLine().
void BSP_LCD_FillPolygon | ( | pPoint | Points, |
uint16_t | PointCount | ||
) |
Draws a full poly-line (between many points).
- Parameters:
-
Points,: Pointer to the points array PointCount,: Number of points
Definition at line 940 of file stm324x9i_eval_lcd.c.
References FillTriangle(), POLY_X, POLY_Y, Point::X, and Point::Y.
void BSP_LCD_FillRect | ( | uint16_t | Xpos, |
uint16_t | Ypos, | ||
uint16_t | Width, | ||
uint16_t | Height | ||
) |
Draws a full rectangle.
- Parameters:
-
Xpos,: X position Ypos,: Y position Width,: Rectangle width Height,: Rectangle height
Definition at line 873 of file stm324x9i_eval_lcd.c.
References ActiveLayer, BSP_LCD_GetXSize(), BSP_LCD_SetTextColor(), hltdc_eval, and LL_FillBuffer().
Referenced by BSP_LCD_ClearStringLine().
uint32_t BSP_LCD_GetBackColor | ( | void | ) |
Gets the LCD background color.
- Return values:
-
Used background color
Definition at line 408 of file stm324x9i_eval_lcd.c.
References ActiveLayer, and LCD_DrawPropTypeDef::BackColor.
sFONT* BSP_LCD_GetFont | ( | void | ) |
Gets the LCD text font.
- Return values:
-
Used layer font
Definition at line 426 of file stm324x9i_eval_lcd.c.
References ActiveLayer, and LCD_DrawPropTypeDef::pFont.
uint32_t BSP_LCD_GetTextColor | ( | void | ) |
Gets the LCD text color.
- Return values:
-
Used text color.
Definition at line 390 of file stm324x9i_eval_lcd.c.
References ActiveLayer, and LCD_DrawPropTypeDef::TextColor.
uint32_t BSP_LCD_GetXSize | ( | void | ) |
Gets the LCD X size.
- Return values:
-
Used LCD X size
Definition at line 240 of file stm324x9i_eval_lcd.c.
References ActiveLayer, and hltdc_eval.
Referenced by BSP_LCD_Clear(), BSP_LCD_ClearStringLine(), BSP_LCD_DisplayStringAt(), BSP_LCD_DrawBitmap(), BSP_LCD_DrawHLine(), BSP_LCD_DrawPixel(), BSP_LCD_DrawVLine(), BSP_LCD_FillRect(), BSP_LCD_LayerDefaultInit(), and BSP_LCD_ReadPixel().
uint32_t BSP_LCD_GetYSize | ( | void | ) |
Gets the LCD Y size.
- Return values:
-
Used LCD Y size
Definition at line 249 of file stm324x9i_eval_lcd.c.
References ActiveLayer, and hltdc_eval.
Referenced by BSP_LCD_Clear(), and BSP_LCD_LayerDefaultInit().
uint8_t BSP_LCD_Init | ( | void | ) |
Initializes the LCD.
- Return values:
-
LCD state
Definition at line 155 of file stm324x9i_eval_lcd.c.
References BSP_LCD_InitEx(), and LCD_MAX_PCLK.
uint8_t BSP_LCD_InitEx | ( | uint32_t | PclkConfig | ) |
Initializes the LCD.
- Parameters:
-
PclkConfig : pixel clock profile
- Return values:
-
LCD state
Definition at line 165 of file stm324x9i_eval_lcd.c.
References BSP_LCD_ClockConfig(), BSP_LCD_SetFont(), BSP_SDRAM_Init(), hltdc_eval, LCD_DEFAULT_FONT, LCD_OK, MspInit(), PCLK_profile, and TS_I2C_ADDRESS.
Referenced by BSP_LCD_Init().
void BSP_LCD_LayerDefaultInit | ( | uint16_t | LayerIndex, |
uint32_t | FB_Address | ||
) |
Initializes the LCD layers.
- Parameters:
-
LayerIndex,: Layer foreground or background FB_Address,: Layer frame buffer
Definition at line 259 of file stm324x9i_eval_lcd.c.
References LCD_DrawPropTypeDef::BackColor, BSP_LCD_GetXSize(), BSP_LCD_GetYSize(), hltdc_eval, LCD_COLOR_BLACK, LCD_COLOR_WHITE, LCD_LayerCfgTypeDef, LCD_DrawPropTypeDef::pFont, and LCD_DrawPropTypeDef::TextColor.
uint32_t BSP_LCD_ReadPixel | ( | uint16_t | Xpos, |
uint16_t | Ypos | ||
) |
Reads an LCD pixel.
- Parameters:
-
Xpos,: X position Ypos,: Y position
- Return values:
-
RGB pixel color
Definition at line 437 of file stm324x9i_eval_lcd.c.
References ActiveLayer, BSP_LCD_GetXSize(), and hltdc_eval.
void BSP_LCD_ResetColorKeying | ( | uint32_t | LayerIndex | ) |
Disables the color keying.
- Parameters:
-
LayerIndex,: Layer foreground or background
Definition at line 371 of file stm324x9i_eval_lcd.c.
References hltdc_eval.
void BSP_LCD_SelectLayer | ( | uint32_t | LayerIndex | ) |
Selects the LCD Layer.
- Parameters:
-
LayerIndex,: Layer foreground or background
Definition at line 291 of file stm324x9i_eval_lcd.c.
References ActiveLayer.
void BSP_LCD_SetBackColor | ( | uint32_t | Color | ) |
Sets the LCD background color.
- Parameters:
-
Color,: Layer background color code ARGB(8-8-8-8)
Definition at line 399 of file stm324x9i_eval_lcd.c.
References ActiveLayer, and LCD_DrawPropTypeDef::BackColor.
void BSP_LCD_SetColorKeying | ( | uint32_t | LayerIndex, |
uint32_t | RGBValue | ||
) |
Configures and sets the color keying.
- Parameters:
-
LayerIndex,: Layer foreground or background RGBValue,: Color reference
Definition at line 360 of file stm324x9i_eval_lcd.c.
References hltdc_eval.
void BSP_LCD_SetFont | ( | sFONT * | fonts | ) |
Sets the LCD text font.
- Parameters:
-
fonts,: Layer font to be used
Definition at line 417 of file stm324x9i_eval_lcd.c.
References ActiveLayer, and LCD_DrawPropTypeDef::pFont.
Referenced by BSP_LCD_InitEx().
void BSP_LCD_SetLayerAddress | ( | uint32_t | LayerIndex, |
uint32_t | Address | ||
) |
Sets an LCD layer frame buffer address.
- Parameters:
-
LayerIndex,: Layer foreground or background Address,: New LCD frame buffer value
Definition at line 333 of file stm324x9i_eval_lcd.c.
References hltdc_eval.
void BSP_LCD_SetLayerVisible | ( | uint32_t | LayerIndex, |
FunctionalState | State | ||
) |
Sets an LCD Layer visible.
- Parameters:
-
LayerIndex,: Visible Layer State,: New state of the specified layer This parameter can be one of the following values: - ENABLE
- DISABLE
Definition at line 304 of file stm324x9i_eval_lcd.c.
References hltdc_eval.
void BSP_LCD_SetLayerWindow | ( | uint16_t | LayerIndex, |
uint16_t | Xpos, | ||
uint16_t | Ypos, | ||
uint16_t | Width, | ||
uint16_t | Height | ||
) |
Sets display window.
- Parameters:
-
LayerIndex,: Layer index Xpos,: LCD X position Ypos,: LCD Y position Width,: LCD window width Height,: LCD window height
Definition at line 346 of file stm324x9i_eval_lcd.c.
References hltdc_eval.
void BSP_LCD_SetTextColor | ( | uint32_t | Color | ) |
Sets the LCD text color.
- Parameters:
-
Color,: Text color code ARGB(8-8-8-8)
Definition at line 381 of file stm324x9i_eval_lcd.c.
References ActiveLayer, and LCD_DrawPropTypeDef::TextColor.
Referenced by BSP_LCD_ClearStringLine(), BSP_LCD_FillCircle(), and BSP_LCD_FillRect().
void BSP_LCD_SetTransparency | ( | uint32_t | LayerIndex, |
uint8_t | Transparency | ||
) |
Configures the transparency.
- Parameters:
-
LayerIndex,: Layer foreground or background. Transparency,: Transparency This parameter must be a number between Min_Data = 0x00 and Max_Data = 0xFF
Definition at line 323 of file stm324x9i_eval_lcd.c.
References hltdc_eval.
Generated on Wed Jan 13 2016 15:52:54 for STM324x9I_EVAL BSP User Manual by
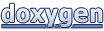