STM324x9I_EVAL BSP User Manual
|
stm324x9i_eval_ts.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm324x9i_eval_ts.c 00004 * @author MCD Application Team 00005 * @version V2.2.2 00006 * @date 13-January-2016 00007 * @brief This file provides a set of functions needed to manage the Touch 00008 * Screen on STM324x9I-EVAL evaluation board. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* File Info : ----------------------------------------------------------------- 00040 User NOTES 00041 1. How To use this driver: 00042 -------------------------- 00043 - This driver is used to drive the touch screen module of the STM324x9I-EVAL 00044 evaluation board on the AMPIRE 640x480 LCD mounted on MB1063 or AMPIRE 00045 480x272 LCD mounted on MB1046 daughter board. 00046 - If the AMPIRE 640x480 LCD is used, the TS3510 or EXC7200 component driver 00047 must be included according to the touch screen driver present on this board. 00048 - If the AMPIRE 480x272 LCD is used, the STMPE811 IO expander device component 00049 driver must be included in order to run the TS module commanded by the IO 00050 expander device, the STMPE1600 IO expander device component driver must be 00051 also included in case of interrupt mode use of the TS. 00052 00053 2. Driver description: 00054 --------------------- 00055 + Initialization steps: 00056 o Initialize the TS module using the BSP_TS_Init() function. This 00057 function includes the MSP layer hardware resources initialization and the 00058 communication layer configuration to start the TS use. The LCD size properties 00059 (x and y) are passed as parameters. 00060 o If TS interrupt mode is desired, you must configure the TS interrupt mode 00061 by calling the function BSP_TS_ITConfig(). The TS interrupt mode is generated 00062 as an external interrupt whenever a touch is detected. 00063 The interrupt mode internally uses the IO functionalities driver driven by 00064 the IO expander, to configure the IT line. 00065 00066 + Touch screen use 00067 o The touch screen state is captured whenever the function BSP_TS_GetState() is 00068 used. This function returns information about the last LCD touch occurred 00069 in the TS_StateTypeDef structure. 00070 o If TS interrupt mode is used, the function BSP_TS_ITGetStatus() is needed to get 00071 the interrupt status. To clear the IT pending bits, you should call the 00072 function BSP_TS_ITClear(). 00073 o The IT is handled using the corresponding external interrupt IRQ handler, 00074 the user IT callback treatment is implemented on the same external interrupt 00075 callback. 00076 00077 ------------------------------------------------------------------------------*/ 00078 00079 /* Includes ------------------------------------------------------------------*/ 00080 #include "stm324x9i_eval_ts.h" 00081 #include "stm324x9i_eval_io.h" 00082 00083 /** @addtogroup BSP 00084 * @{ 00085 */ 00086 00087 /** @addtogroup STM324x9I_EVAL 00088 * @{ 00089 */ 00090 00091 /** @defgroup STM324x9I_EVAL_TS STM324x9I EVAL TS 00092 * @{ 00093 */ 00094 00095 /** @defgroup STM324x9I_EVAL_TS_Private_Types_Definitions STM324x9I EVAL TS Private Types Definitions 00096 * @{ 00097 */ 00098 /** 00099 * @} 00100 */ 00101 00102 /** @defgroup STM324x9I_EVAL_TS_Private_Defines STM324x9I EVAL TS Private Defines 00103 * @{ 00104 */ 00105 /** 00106 * @} 00107 */ 00108 00109 /** @defgroup STM324x9I_EVAL_TS_Private_Macros STM324x9I EVAL TS Private Macros 00110 * @{ 00111 */ 00112 /** 00113 * @} 00114 */ 00115 00116 /** @defgroup STM324x9I_EVAL_TS_Private_Variables STM324x9I EVAL TS Private Variables 00117 * @{ 00118 */ 00119 static TS_DrvTypeDef *ts_driver; 00120 static uint16_t ts_x_boundary, ts_y_boundary; 00121 static uint8_t ts_orientation; 00122 static uint8_t I2C_Address; 00123 /** 00124 * @} 00125 */ 00126 00127 /** @defgroup STM324x9I_EVAL_TS_Private_Function_Prototypes STM324x9I EVAL TS Private Function Prototypes 00128 * @{ 00129 */ 00130 /** 00131 * @} 00132 */ 00133 00134 /** @defgroup STM324x9I_EVAL_TS_Private_Functions STM324x9I EVAL TS Private Functions 00135 * @{ 00136 */ 00137 00138 /** 00139 * @brief Initializes and configures the touch screen functionalities and 00140 * configures all necessary hardware resources (GPIOs, clocks..). 00141 * @param xSize: Maximum X size of the TS area on LCD 00142 * @param ySize: Maximum Y size of the TS area on LCD 00143 * @retval TS_OK if all initializations are OK. Other value if error. 00144 */ 00145 uint8_t BSP_TS_Init(uint16_t xSize, uint16_t ySize) 00146 { 00147 uint8_t status = TS_OK; 00148 ts_x_boundary = xSize; 00149 ts_y_boundary = ySize; 00150 00151 /* Read ID and verify if the IO expander is ready */ 00152 if(stmpe811_ts_drv.ReadID(TS_I2C_ADDRESS) == STMPE811_ID) 00153 { 00154 /* Initialize the TS driver structure */ 00155 ts_driver = &stmpe811_ts_drv; 00156 I2C_Address = TS_I2C_ADDRESS; 00157 ts_orientation = TS_SWAP_Y; 00158 } 00159 else 00160 { 00161 IOE_Init(); 00162 00163 /* Check TS3510 touch screen driver presence to determine if TS3510 or 00164 * EXC7200 driver will be used */ 00165 if(BSP_TS3510_IsDetected() == 0) 00166 { 00167 /* Initialize the TS driver structure */ 00168 ts_driver = &ts3510_ts_drv; 00169 I2C_Address = TS3510_I2C_ADDRESS; 00170 } 00171 else 00172 { 00173 /* Initialize the TS driver structure */ 00174 ts_driver = &exc7200_ts_drv; 00175 I2C_Address = EXC7200_I2C_ADDRESS; 00176 } 00177 ts_orientation = TS_SWAP_NONE; 00178 } 00179 00180 /* Initialize the TS driver */ 00181 ts_driver->Init(I2C_Address); 00182 ts_driver->Start(I2C_Address); 00183 00184 return status; 00185 } 00186 00187 /** 00188 * @brief DeInitializes the TouchScreen. 00189 * @retval TS state 00190 */ 00191 uint8_t BSP_TS_DeInit(void) 00192 { 00193 /* Actually ts_driver does not provide a DeInit function */ 00194 return TS_OK; 00195 } 00196 00197 /** 00198 * @brief Configures and enables the touch screen interrupts. 00199 * @retval TS_OK if all initializations are OK. Other value if error. 00200 */ 00201 uint8_t BSP_TS_ITConfig(void) 00202 { 00203 /* Initialize the IO */ 00204 BSP_IO_Init(); 00205 00206 /* Configure TS IT line IO */ 00207 BSP_IO_ConfigPin(TS_INT_PIN, IO_MODE_IT_FALLING_EDGE); 00208 00209 /* Enable the TS ITs */ 00210 ts_driver->EnableIT(I2C_Address); 00211 00212 return TS_OK; 00213 } 00214 00215 /** 00216 * @brief Gets the touch screen interrupt status. 00217 * @retval TS_OK if all initializations are OK. Other value if error. 00218 */ 00219 uint8_t BSP_TS_ITGetStatus(void) 00220 { 00221 /* Return the TS IT status */ 00222 return (ts_driver->GetITStatus(I2C_Address)); 00223 } 00224 00225 /** 00226 * @brief Returns status and positions of the touch screen. 00227 * @param TS_State: Pointer to touch screen current state structure 00228 * @retval TS_OK if all initializations are OK. Other value if error. 00229 */ 00230 uint8_t BSP_TS_GetState(TS_StateTypeDef *TS_State) 00231 { 00232 static uint32_t _x = 0, _y = 0; 00233 uint16_t xDiff, yDiff , x , y; 00234 uint16_t swap; 00235 00236 TS_State->TouchDetected = ts_driver->DetectTouch(I2C_Address); 00237 00238 if(TS_State->TouchDetected) 00239 { 00240 ts_driver->GetXY(I2C_Address, &x, &y); 00241 00242 if(ts_orientation & TS_SWAP_X) 00243 { 00244 x = 4096 - x; 00245 } 00246 00247 if(ts_orientation & TS_SWAP_Y) 00248 { 00249 y = 4096 - y; 00250 } 00251 00252 if(ts_orientation & TS_SWAP_XY) 00253 { 00254 swap = y; 00255 y = x; 00256 x = swap; 00257 } 00258 00259 xDiff = x > _x? (x - _x): (_x - x); 00260 yDiff = y > _y? (y - _y): (_y - y); 00261 00262 if (xDiff + yDiff > 5) 00263 { 00264 _x = x; 00265 _y = y; 00266 } 00267 00268 TS_State->x = (ts_x_boundary * _x) >> 12; 00269 TS_State->y = (ts_y_boundary * _y) >> 12; 00270 } 00271 return TS_OK; 00272 } 00273 00274 /** 00275 * @brief Clears all touch screen interrupts. 00276 */ 00277 void BSP_TS_ITClear(void) 00278 { 00279 /* Clear all IO IT pin */ 00280 BSP_IO_ITClear(); 00281 00282 /* Clear TS IT pending bits */ 00283 ts_driver->ClearIT(I2C_Address); 00284 } 00285 00286 /** 00287 * @} 00288 */ 00289 00290 /** 00291 * @} 00292 */ 00293 00294 /** 00295 * @} 00296 */ 00297 00298 /** 00299 * @} 00300 */ 00301 00302 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Wed Jan 13 2016 15:52:54 for STM324x9I_EVAL BSP User Manual by
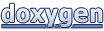