STM324x9I_EVAL BSP User Manual
|
stm324x9i_eval.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm324x9i_eval.c 00004 * @author MCD Application Team 00005 * @version V2.2.2 00006 * @date 13-January-2016 00007 * @brief This file provides a set of firmware functions to manage LEDs, 00008 * push-buttons and COM ports available on STM324x9I-EVAL evaluation 00009 * board(MB1045) RevB from STMicroelectronics. 00010 ****************************************************************************** 00011 * @attention 00012 * 00013 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00014 * 00015 * Redistribution and use in source and binary forms, with or without modification, 00016 * are permitted provided that the following conditions are met: 00017 * 1. Redistributions of source code must retain the above copyright notice, 00018 * this list of conditions and the following disclaimer. 00019 * 2. Redistributions in binary form must reproduce the above copyright notice, 00020 * this list of conditions and the following disclaimer in the documentation 00021 * and/or other materials provided with the distribution. 00022 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00023 * may be used to endorse or promote products derived from this software 00024 * without specific prior written permission. 00025 * 00026 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00027 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00028 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00029 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00030 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00031 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00032 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00033 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00034 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00035 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00036 * 00037 ****************************************************************************** 00038 */ 00039 00040 /* File Info: ------------------------------------------------------------------ 00041 User NOTE 00042 00043 This driver requires the stm324x9i_eval_io to manage the joystick 00044 00045 ------------------------------------------------------------------------------*/ 00046 00047 /* Includes ------------------------------------------------------------------*/ 00048 #include "stm324x9i_eval.h" 00049 #include "stm324x9i_eval_io.h" 00050 00051 /** @defgroup BSP BSP 00052 * @{ 00053 */ 00054 00055 /** @defgroup STM324x9I_EVAL STM324x9I EVAL 00056 * @{ 00057 */ 00058 00059 /** @defgroup STM324x9I_EVAL_LOW_LEVEL STM324x9I EVAL LOW LEVEL 00060 * @{ 00061 */ 00062 00063 /** @defgroup STM324x9I_EVAL_LOW_LEVEL_Private_TypesDefinitions STM324x9I EVAL LOW LEVEL Private TypesDefinitions 00064 * @{ 00065 */ 00066 /** 00067 * @} 00068 */ 00069 00070 /** @defgroup STM324x9I_EVAL_LOW_LEVEL_Private_Defines STM324x9I EVAL LOW LEVEL Private Defines 00071 * @{ 00072 */ 00073 /** 00074 * @brief STM324x9I EVAL BSP Driver version number V2.2.2 00075 */ 00076 #define __STM324x9I_EVAL_BSP_VERSION_MAIN (0x02) /*!< [31:24] main version */ 00077 #define __STM324x9I_EVAL_BSP_VERSION_SUB1 (0x02) /*!< [23:16] sub1 version */ 00078 #define __STM324x9I_EVAL_BSP_VERSION_SUB2 (0x02) /*!< [15:8] sub2 version */ 00079 #define __STM324x9I_EVAL_BSP_VERSION_RC (0x00) /*!< [7:0] release candidate */ 00080 #define __STM324x9I_EVAL_BSP_VERSION ((__STM324x9I_EVAL_BSP_VERSION_MAIN << 24)\ 00081 |(__STM324x9I_EVAL_BSP_VERSION_SUB1 << 16)\ 00082 |(__STM324x9I_EVAL_BSP_VERSION_SUB2 << 8 )\ 00083 |(__STM324x9I_EVAL_BSP_VERSION_RC)) 00084 /** 00085 * @} 00086 */ 00087 00088 /** @defgroup STM324x9I_EVAL_LOW_LEVEL_Private_Macros STM324x9I EVAL LOW_LEVEL_Private_Macros 00089 * @{ 00090 */ 00091 /** 00092 * @} 00093 */ 00094 00095 /** @defgroup STM324x9I_EVAL_LOW_LEVEL_Private_Variables STM324x9I EVAL LOW LEVEL Private Variables 00096 * @{ 00097 */ 00098 GPIO_TypeDef* GPIO_PORT[LEDn] = {LED1_GPIO_PORT, 00099 LED2_GPIO_PORT, 00100 LED3_GPIO_PORT, 00101 LED4_GPIO_PORT}; 00102 00103 const uint16_t GPIO_PIN[LEDn] = {LED1_PIN, 00104 LED2_PIN, 00105 LED3_PIN, 00106 LED4_PIN}; 00107 00108 GPIO_TypeDef* BUTTON_PORT[BUTTONn] = {WAKEUP_BUTTON_GPIO_PORT, 00109 TAMPER_BUTTON_GPIO_PORT, 00110 KEY_BUTTON_GPIO_PORT}; 00111 00112 const uint16_t BUTTON_PIN[BUTTONn] = {WAKEUP_BUTTON_PIN, 00113 TAMPER_BUTTON_PIN, 00114 KEY_BUTTON_PIN}; 00115 00116 const uint16_t BUTTON_IRQn[BUTTONn] = {WAKEUP_BUTTON_EXTI_IRQn, 00117 TAMPER_BUTTON_EXTI_IRQn, 00118 KEY_BUTTON_EXTI_IRQn}; 00119 00120 USART_TypeDef* COM_USART[COMn] = {EVAL_COM1}; 00121 00122 GPIO_TypeDef* COM_TX_PORT[COMn] = {EVAL_COM1_TX_GPIO_PORT}; 00123 00124 GPIO_TypeDef* COM_RX_PORT[COMn] = {EVAL_COM1_RX_GPIO_PORT}; 00125 00126 const uint16_t COM_TX_PIN[COMn] = {EVAL_COM1_TX_PIN}; 00127 00128 const uint16_t COM_RX_PIN[COMn] = {EVAL_COM1_RX_PIN}; 00129 00130 const uint16_t COM_TX_AF[COMn] = {EVAL_COM1_TX_AF}; 00131 00132 const uint16_t COM_RX_AF[COMn] = {EVAL_COM1_RX_AF}; 00133 00134 static I2C_HandleTypeDef heval_I2c; 00135 00136 /** 00137 * @} 00138 */ 00139 00140 /** @defgroup STM324x9I_EVAL_LOW_LEVEL_Private_FunctionPrototypes STM324x9I EVAL LOW LEVEL Private FunctionPrototypes 00141 * @{ 00142 */ 00143 static void I2Cx_MspInit(void); 00144 static void I2Cx_Init(void); 00145 static void I2Cx_ITConfig(void); 00146 static void I2Cx_Write(uint8_t Addr, uint8_t Reg, uint8_t Value); 00147 static uint8_t I2Cx_Read(uint8_t Addr, uint8_t Reg); 00148 static HAL_StatusTypeDef I2Cx_ReadMultiple(uint8_t Addr, uint16_t Reg, uint16_t MemAddSize, uint8_t *Buffer, uint16_t Length); 00149 static HAL_StatusTypeDef I2Cx_WriteMultiple(uint8_t Addr, uint16_t Reg, uint16_t MemAddSize, uint8_t *Buffer, uint16_t Length); 00150 static HAL_StatusTypeDef I2Cx_IsDeviceReady(uint16_t DevAddress, uint32_t Trials); 00151 static void I2Cx_Error(uint8_t Addr); 00152 00153 /* IOExpander IO functions */ 00154 void IOE_Init(void); 00155 void IOE_ITConfig(void); 00156 void IOE_Delay(uint32_t Delay); 00157 void IOE_Write(uint8_t Addr, uint8_t Reg, uint8_t Value); 00158 uint8_t IOE_Read(uint8_t Addr, uint8_t Reg); 00159 uint16_t IOE_ReadMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length); 00160 void IOE_WriteMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length); 00161 00162 /* AUDIO IO functions */ 00163 void AUDIO_IO_Init(void); 00164 void AUDIO_IO_DeInit(void); 00165 void AUDIO_IO_Write(uint8_t Addr, uint16_t Reg, uint16_t Value); 00166 uint16_t AUDIO_IO_Read(uint8_t Addr, uint16_t Reg); 00167 void AUDIO_IO_Delay(uint32_t Delay); 00168 00169 /* CAMERA IO functions */ 00170 void CAMERA_IO_Init(void); 00171 void CAMERA_Delay(uint32_t Delay); 00172 void CAMERA_IO_Write(uint8_t Addr, uint8_t Reg, uint8_t Value); 00173 uint8_t CAMERA_IO_Read(uint8_t Addr, uint8_t Reg); 00174 00175 /* I2C EEPROM IO function */ 00176 void EEPROM_IO_Init(void); 00177 HAL_StatusTypeDef EEPROM_IO_WriteData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize); 00178 HAL_StatusTypeDef EEPROM_IO_ReadData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize); 00179 HAL_StatusTypeDef EEPROM_IO_IsDeviceReady(uint16_t DevAddress, uint32_t Trials); 00180 /** 00181 * @} 00182 */ 00183 00184 /** @defgroup STM324x9I_EVAL_LOW_LEVEL_Private_Functions STM324x9I EVAL LOW LEVEL Private Functions 00185 * @{ 00186 */ 00187 00188 /** 00189 * @brief This method returns the STM324x9I EVAL BSP Driver revision 00190 * @retval version: 0xXYZR (8bits for each decimal, R for RC) 00191 */ 00192 uint32_t BSP_GetVersion(void) 00193 { 00194 return __STM324x9I_EVAL_BSP_VERSION; 00195 } 00196 00197 /** 00198 * @brief Configures LED GPIO. 00199 * @param Led: LED to be configured. 00200 * This parameter can be one of the following values: 00201 * @arg LED1 00202 * @arg LED2 00203 * @arg LED3 00204 * @arg LED4 00205 */ 00206 void BSP_LED_Init(Led_TypeDef Led) 00207 { 00208 GPIO_InitTypeDef GPIO_InitStruct; 00209 00210 /* Enable the GPIO_LED clock */ 00211 LEDx_GPIO_CLK_ENABLE(Led); 00212 00213 /* Configure the GPIO_LED pin */ 00214 GPIO_InitStruct.Pin = GPIO_PIN[Led]; 00215 GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP; 00216 GPIO_InitStruct.Pull = GPIO_PULLUP; 00217 GPIO_InitStruct.Speed = GPIO_SPEED_FAST; 00218 00219 HAL_GPIO_Init(GPIO_PORT[Led], &GPIO_InitStruct); 00220 00221 HAL_GPIO_WritePin(GPIO_PORT[Led], GPIO_PIN[Led], GPIO_PIN_SET); 00222 } 00223 00224 /** 00225 * @brief Turns selected LED On. 00226 * @param Led: LED to be set on 00227 * This parameter can be one of the following values: 00228 * @arg LED1 00229 * @arg LED2 00230 * @arg LED3 00231 * @arg LED4 00232 */ 00233 void BSP_LED_On(Led_TypeDef Led) 00234 { 00235 HAL_GPIO_WritePin(GPIO_PORT[Led], GPIO_PIN[Led], GPIO_PIN_RESET); 00236 } 00237 00238 /** 00239 * @brief Turns selected LED Off. 00240 * @param Led: LED to be set off 00241 * This parameter can be one of the following values: 00242 * @arg LED1 00243 * @arg LED2 00244 * @arg LED3 00245 * @arg LED4 00246 */ 00247 void BSP_LED_Off(Led_TypeDef Led) 00248 { 00249 HAL_GPIO_WritePin(GPIO_PORT[Led], GPIO_PIN[Led], GPIO_PIN_SET); 00250 } 00251 00252 /** 00253 * @brief Toggles the selected LED. 00254 * @param Led: LED to be toggled 00255 * This parameter can be one of the following values: 00256 * @arg LED1 00257 * @arg LED2 00258 * @arg LED3 00259 * @arg LED4 00260 */ 00261 void BSP_LED_Toggle(Led_TypeDef Led) 00262 { 00263 HAL_GPIO_TogglePin(GPIO_PORT[Led], GPIO_PIN[Led]); 00264 } 00265 00266 /** 00267 * @brief Configures button GPIO and EXTI Line. 00268 * @param Button: Button to be configured 00269 * This parameter can be one of the following values: 00270 * @arg BUTTON_WAKEUP: Wakeup Push Button 00271 * @arg BUTTON_TAMPER: Tamper Push Button 00272 * @param Button_Mode: Button mode 00273 * This parameter can be one of the following values: 00274 * @arg BUTTON_MODE_GPIO: Button will be used as simple IO 00275 * @arg BUTTON_MODE_EXTI: Button will be connected to EXTI line 00276 * with interrupt generation capability 00277 */ 00278 void BSP_PB_Init(Button_TypeDef Button, ButtonMode_TypeDef Button_Mode) 00279 { 00280 GPIO_InitTypeDef GPIO_InitStruct; 00281 00282 /* Enable the BUTTON clock */ 00283 BUTTONx_GPIO_CLK_ENABLE(Button); 00284 00285 if(Button_Mode == BUTTON_MODE_GPIO) 00286 { 00287 /* Configure Button pin as input */ 00288 GPIO_InitStruct.Pin = BUTTON_PIN[Button]; 00289 GPIO_InitStruct.Mode = GPIO_MODE_INPUT; 00290 GPIO_InitStruct.Pull = GPIO_NOPULL; 00291 GPIO_InitStruct.Speed = GPIO_SPEED_FAST; 00292 HAL_GPIO_Init(BUTTON_PORT[Button], &GPIO_InitStruct); 00293 } 00294 00295 if(Button_Mode == BUTTON_MODE_EXTI) 00296 { 00297 /* Configure Button pin as input with External interrupt */ 00298 GPIO_InitStruct.Pin = BUTTON_PIN[Button]; 00299 GPIO_InitStruct.Pull = GPIO_NOPULL; 00300 GPIO_InitStruct.Speed = GPIO_SPEED_FAST; 00301 00302 if(Button != BUTTON_WAKEUP) 00303 { 00304 GPIO_InitStruct.Mode = GPIO_MODE_IT_FALLING; 00305 } 00306 else 00307 { 00308 GPIO_InitStruct.Mode = GPIO_MODE_IT_RISING; 00309 } 00310 00311 HAL_GPIO_Init(BUTTON_PORT[Button], &GPIO_InitStruct); 00312 00313 /* Enable and set Button EXTI Interrupt to the lowest priority */ 00314 HAL_NVIC_SetPriority((IRQn_Type)(BUTTON_IRQn[Button]), 0x0F, 0x00); 00315 HAL_NVIC_EnableIRQ((IRQn_Type)(BUTTON_IRQn[Button])); 00316 } 00317 } 00318 00319 /** 00320 * @brief Returns the selected button state. 00321 * @param Button: Button to be checked 00322 * This parameter can be one of the following values: 00323 * @arg BUTTON_WAKEUP: Wakeup Push Button 00324 * @arg BUTTON_TAMPER: Tamper Push Button 00325 * @arg BUTTON_KEY: Key Push Button 00326 * @retval The Button GPIO pin value 00327 */ 00328 uint32_t BSP_PB_GetState(Button_TypeDef Button) 00329 { 00330 return HAL_GPIO_ReadPin(BUTTON_PORT[Button], BUTTON_PIN[Button]); 00331 } 00332 00333 /** 00334 * @brief Configures COM port. 00335 * @param COM: COM port to be configured. 00336 * This parameter can be one of the following values: 00337 * @arg COM1 00338 * @arg COM2 00339 * @param huart: Pointer to a UART_HandleTypeDef structure that contains the 00340 * configuration information for the specified USART peripheral. 00341 */ 00342 void BSP_COM_Init(COM_TypeDef COM, UART_HandleTypeDef *huart) 00343 { 00344 GPIO_InitTypeDef GPIO_InitStruct; 00345 00346 /* Enable GPIO clock */ 00347 EVAL_COMx_TX_GPIO_CLK_ENABLE(COM); 00348 EVAL_COMx_RX_GPIO_CLK_ENABLE(COM); 00349 00350 /* Enable USART clock */ 00351 EVAL_COMx_CLK_ENABLE(COM); 00352 00353 /* Configure USART Tx as alternate function */ 00354 GPIO_InitStruct.Pin = COM_TX_PIN[COM]; 00355 GPIO_InitStruct.Mode = GPIO_MODE_AF_PP; 00356 GPIO_InitStruct.Speed = GPIO_SPEED_FAST; 00357 GPIO_InitStruct.Pull = GPIO_PULLUP; 00358 GPIO_InitStruct.Alternate = COM_TX_AF[COM]; 00359 HAL_GPIO_Init(COM_TX_PORT[COM], &GPIO_InitStruct); 00360 00361 /* Configure USART Rx as alternate function */ 00362 GPIO_InitStruct.Pin = COM_RX_PIN[COM]; 00363 GPIO_InitStruct.Mode = GPIO_MODE_AF_PP; 00364 GPIO_InitStruct.Alternate = COM_RX_AF[COM]; 00365 HAL_GPIO_Init(COM_RX_PORT[COM], &GPIO_InitStruct); 00366 00367 /* USART configuration */ 00368 huart->Instance = COM_USART[COM]; 00369 HAL_UART_Init(huart); 00370 } 00371 00372 /** 00373 * @brief Configures joystick GPIO and EXTI modes. 00374 * @param Joy_Mode: Button mode. 00375 * This parameter can be one of the following values: 00376 * @arg JOY_MODE_GPIO: Joystick pins will be used as simple IOs 00377 * @arg JOY_MODE_EXTI: Joystick pins will be connected to EXTI line 00378 * with interrupt generation capability 00379 * @retval IO_OK: if all initializations are OK. Other value if error. 00380 */ 00381 uint8_t BSP_JOY_Init(JOYMode_TypeDef Joy_Mode) 00382 { 00383 uint8_t ret = 0; 00384 00385 /* Initialize the IO functionalities */ 00386 ret = BSP_IO_Init(); 00387 00388 /* Configure joystick pins in IT mode */ 00389 if(Joy_Mode == JOY_MODE_EXTI) 00390 { 00391 /* Configure IO interrupt acquisition mode */ 00392 BSP_IO_ConfigPin(JOY_ALL_PINS, IO_MODE_IT_FALLING_EDGE); 00393 } 00394 00395 return ret; 00396 } 00397 00398 /** 00399 * @brief Returns the current joystick status. 00400 * @retval Code of the joystick key pressed 00401 * This code can be one of the following values: 00402 * @arg JOY_NONE 00403 * @arg JOY_SEL 00404 * @arg JOY_DOWN 00405 * @arg JOY_LEFT 00406 * @arg JOY_RIGHT 00407 * @arg JOY_UP 00408 */ 00409 JOYState_TypeDef BSP_JOY_GetState(void) 00410 { 00411 uint16_t tmp = 0; 00412 00413 /* Read the status joystick pins */ 00414 tmp = BSP_IO_ReadPin(JOY_ALL_PINS); 00415 00416 /* Check the pressed keys */ 00417 if((tmp & JOY_NONE_PIN) == JOY_NONE) 00418 { 00419 return(JOYState_TypeDef) JOY_NONE; 00420 } 00421 else if(!(tmp & JOY_SEL_PIN)) 00422 { 00423 return(JOYState_TypeDef) JOY_SEL; 00424 } 00425 else if(!(tmp & JOY_DOWN_PIN)) 00426 { 00427 return(JOYState_TypeDef) JOY_DOWN; 00428 } 00429 else if(!(tmp & JOY_LEFT_PIN)) 00430 { 00431 return(JOYState_TypeDef) JOY_LEFT; 00432 } 00433 else if(!(tmp & JOY_RIGHT_PIN)) 00434 { 00435 return(JOYState_TypeDef) JOY_RIGHT; 00436 } 00437 else if(!(tmp & JOY_UP_PIN)) 00438 { 00439 return(JOYState_TypeDef) JOY_UP; 00440 } 00441 else 00442 { 00443 return(JOYState_TypeDef) JOY_NONE; 00444 } 00445 } 00446 00447 /** 00448 * @brief Check TS3510 touch screen presence 00449 * @retval Return 0 if TS3510 is detected, return 1 if not detected 00450 */ 00451 uint8_t BSP_TS3510_IsDetected(void) 00452 { 00453 HAL_StatusTypeDef status = HAL_OK; 00454 uint32_t error = 0; 00455 uint8_t a_buffer; 00456 00457 uint8_t tmp_buffer[2] = {0x81, 0x08}; 00458 00459 /* Prepare for LCD read data */ 00460 IOE_WriteMultiple(TS3510_I2C_ADDRESS, 0x8A, tmp_buffer, 2); 00461 00462 status = HAL_I2C_Mem_Read(&heval_I2c, TS3510_I2C_ADDRESS, 0x8A, I2C_MEMADD_SIZE_8BIT, &a_buffer, 1, 1000); 00463 00464 /* Check the communication status */ 00465 if(status != HAL_OK) 00466 { 00467 error = (uint32_t)HAL_I2C_GetError(&heval_I2c); 00468 00469 /* I2C error occured */ 00470 I2Cx_Error(TS3510_I2C_ADDRESS); 00471 00472 if(error == HAL_I2C_ERROR_AF) 00473 { 00474 return 1; 00475 } 00476 } 00477 return 0; 00478 } 00479 /******************************************************************************* 00480 BUS OPERATIONS 00481 *******************************************************************************/ 00482 00483 /******************************* I2C Routines *********************************/ 00484 /** 00485 * @brief Initializes I2C MSP. 00486 */ 00487 static void I2Cx_MspInit(void) 00488 { 00489 GPIO_InitTypeDef GPIO_InitStruct; 00490 00491 /*** Configure the GPIOs ***/ 00492 /* Enable GPIO clock */ 00493 EVAL_I2Cx_SCL_SDA_GPIO_CLK_ENABLE(); 00494 00495 /* Configure I2C Tx as alternate function */ 00496 GPIO_InitStruct.Pin = EVAL_I2Cx_SCL_PIN; 00497 GPIO_InitStruct.Mode = GPIO_MODE_AF_OD; 00498 GPIO_InitStruct.Pull = GPIO_NOPULL; 00499 GPIO_InitStruct.Speed = GPIO_SPEED_FAST; 00500 GPIO_InitStruct.Alternate = EVAL_I2Cx_SCL_SDA_AF; 00501 HAL_GPIO_Init(EVAL_I2Cx_SCL_SDA_GPIO_PORT, &GPIO_InitStruct); 00502 00503 /* Configure I2C Rx as alternate function */ 00504 GPIO_InitStruct.Pin = EVAL_I2Cx_SDA_PIN; 00505 HAL_GPIO_Init(EVAL_I2Cx_SCL_SDA_GPIO_PORT, &GPIO_InitStruct); 00506 00507 /*** Configure the I2C peripheral ***/ 00508 /* Enable I2C clock */ 00509 EVAL_I2Cx_CLK_ENABLE(); 00510 00511 /* Force the I2C peripheral clock reset */ 00512 EVAL_I2Cx_FORCE_RESET(); 00513 00514 /* Release the I2C peripheral clock reset */ 00515 EVAL_I2Cx_RELEASE_RESET(); 00516 00517 /* Enable and set I2Cx Interrupt to a lower priority */ 00518 HAL_NVIC_SetPriority(EVAL_I2Cx_EV_IRQn, 0x05, 0); 00519 HAL_NVIC_EnableIRQ(EVAL_I2Cx_EV_IRQn); 00520 00521 /* Enable and set I2Cx Interrupt to a lower priority */ 00522 HAL_NVIC_SetPriority(EVAL_I2Cx_ER_IRQn, 0x05, 0); 00523 HAL_NVIC_EnableIRQ(EVAL_I2Cx_ER_IRQn); 00524 } 00525 00526 /** 00527 * @brief Initializes I2C HAL. 00528 */ 00529 static void I2Cx_Init(void) 00530 { 00531 if(HAL_I2C_GetState(&heval_I2c) == HAL_I2C_STATE_RESET) 00532 { 00533 heval_I2c.Instance = I2C1; 00534 heval_I2c.Init.ClockSpeed = BSP_I2C_SPEED; 00535 heval_I2c.Init.DutyCycle = I2C_DUTYCYCLE_2; 00536 heval_I2c.Init.OwnAddress1 = 0; 00537 heval_I2c.Init.AddressingMode = I2C_ADDRESSINGMODE_7BIT; 00538 heval_I2c.Init.DualAddressMode = I2C_DUALADDRESS_DISABLED; 00539 heval_I2c.Init.OwnAddress2 = 0; 00540 heval_I2c.Init.GeneralCallMode = I2C_GENERALCALL_DISABLED; 00541 heval_I2c.Init.NoStretchMode = I2C_NOSTRETCH_DISABLED; 00542 00543 /* Init the I2C */ 00544 I2Cx_MspInit(); 00545 HAL_I2C_Init(&heval_I2c); 00546 } 00547 } 00548 00549 /** 00550 * @brief Configures I2C Interrupt. 00551 */ 00552 static void I2Cx_ITConfig(void) 00553 { 00554 static uint8_t I2C_IT_Enabled = 0; 00555 GPIO_InitTypeDef GPIO_InitStruct; 00556 00557 if(I2C_IT_Enabled == 0) 00558 { 00559 I2C_IT_Enabled = 1; 00560 /* Enable the GPIO EXTI clock */ 00561 __GPIOI_CLK_ENABLE(); 00562 __SYSCFG_CLK_ENABLE(); 00563 00564 GPIO_InitStruct.Pin = GPIO_PIN_8; 00565 GPIO_InitStruct.Pull = GPIO_NOPULL; 00566 GPIO_InitStruct.Speed = GPIO_SPEED_LOW; 00567 GPIO_InitStruct.Mode = GPIO_MODE_IT_FALLING; 00568 HAL_GPIO_Init(GPIOI, &GPIO_InitStruct); 00569 00570 /* Enable and set GPIO EXTI Interrupt to the lowest priority */ 00571 HAL_NVIC_SetPriority((IRQn_Type)(EXTI9_5_IRQn), 0x0F, 0x0F); 00572 HAL_NVIC_EnableIRQ((IRQn_Type)(EXTI9_5_IRQn)); 00573 } 00574 } 00575 00576 /** 00577 * @brief Writes a single data. 00578 * @param Addr: I2C address 00579 * @param Reg: Register address 00580 * @param Value: Data to be written 00581 */ 00582 static void I2Cx_Write(uint8_t Addr, uint8_t Reg, uint8_t Value) 00583 { 00584 HAL_StatusTypeDef status = HAL_OK; 00585 00586 status = HAL_I2C_Mem_Write(&heval_I2c, Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT, &Value, 1, 100); 00587 00588 /* Check the communication status */ 00589 if(status != HAL_OK) 00590 { 00591 /* Execute user timeout callback */ 00592 I2Cx_Error(Addr); 00593 } 00594 } 00595 00596 /** 00597 * @brief Reads a single data. 00598 * @param Addr: I2C address 00599 * @param Reg: Register address 00600 * @retval Read data 00601 */ 00602 static uint8_t I2Cx_Read(uint8_t Addr, uint8_t Reg) 00603 { 00604 HAL_StatusTypeDef status = HAL_OK; 00605 uint8_t Value = 0; 00606 00607 status = HAL_I2C_Mem_Read(&heval_I2c, Addr, Reg, I2C_MEMADD_SIZE_8BIT, &Value, 1, 1000); 00608 00609 /* Check the communication status */ 00610 if(status != HAL_OK) 00611 { 00612 /* Execute user timeout callback */ 00613 I2Cx_Error(Addr); 00614 } 00615 return Value; 00616 } 00617 00618 /** 00619 * @brief Reads multiple data. 00620 * @param Addr: I2C address 00621 * @param Reg: Reg address 00622 * @param MemAddress: Internal memory address 00623 * @param Buffer: Pointer to data buffer 00624 * @param Length: Length of the data 00625 * @retval Number of read data 00626 */ 00627 static HAL_StatusTypeDef I2Cx_ReadMultiple(uint8_t Addr, uint16_t Reg, uint16_t MemAddress, uint8_t *Buffer, uint16_t Length) 00628 { 00629 HAL_StatusTypeDef status = HAL_OK; 00630 00631 if(Addr == EXC7200_I2C_ADDRESS) 00632 { 00633 status = HAL_I2C_Master_Receive(&heval_I2c, Addr, Buffer, Length, 1000); 00634 } 00635 else 00636 { 00637 status = HAL_I2C_Mem_Read(&heval_I2c, Addr, (uint16_t)Reg, MemAddress, Buffer, Length, 1000); 00638 } 00639 00640 /* Check the communication status */ 00641 if(status != HAL_OK) 00642 { 00643 /* I2C error occured */ 00644 I2Cx_Error(Addr); 00645 } 00646 return status; 00647 } 00648 00649 /** 00650 * @brief Writes a value in a register of the device through BUS in using DMA mode. 00651 * @param Addr: Device address on BUS Bus. 00652 * @param Reg: The target register address to write 00653 * @param MemAddress: Internal memory address 00654 * @param Buffer: The target register value to be written 00655 * @param Length: buffer size to be written 00656 * @retval HAL status 00657 */ 00658 static HAL_StatusTypeDef I2Cx_WriteMultiple(uint8_t Addr, uint16_t Reg, uint16_t MemAddress, uint8_t *Buffer, uint16_t Length) 00659 { 00660 HAL_StatusTypeDef status = HAL_OK; 00661 00662 status = HAL_I2C_Mem_Write(&heval_I2c, Addr, (uint16_t)Reg, MemAddress, Buffer, Length, 1000); 00663 00664 /* Check the communication status */ 00665 if(status != HAL_OK) 00666 { 00667 /* Re-Initialize the I2C Bus */ 00668 I2Cx_Error(Addr); 00669 } 00670 return status; 00671 } 00672 00673 /** 00674 * @brief Checks if target device is ready for communication. 00675 * @note This function is used with Memory devices 00676 * @param DevAddress: Target device address 00677 * @param Trials: Number of trials 00678 * @retval HAL status 00679 */ 00680 static HAL_StatusTypeDef I2Cx_IsDeviceReady(uint16_t DevAddress, uint32_t Trials) 00681 { 00682 return (HAL_I2C_IsDeviceReady(&heval_I2c, DevAddress, Trials, 1000)); 00683 } 00684 00685 /** 00686 * @brief Manages error callback by re-initializing I2C. 00687 * @param Addr: I2C Address 00688 */ 00689 static void I2Cx_Error(uint8_t Addr) 00690 { 00691 /* De-initialize the I2C communication bus */ 00692 HAL_I2C_DeInit(&heval_I2c); 00693 00694 /* Re-Initialize the I2C communication bus */ 00695 I2Cx_Init(); 00696 } 00697 00698 /******************************************************************************* 00699 LINK OPERATIONS 00700 *******************************************************************************/ 00701 00702 /********************************* LINK IOE ***********************************/ 00703 00704 /** 00705 * @brief Initializes IOE low level. 00706 */ 00707 void IOE_Init(void) 00708 { 00709 I2Cx_Init(); 00710 } 00711 00712 /** 00713 * @brief Configures IOE low level interrupt. 00714 */ 00715 void IOE_ITConfig(void) 00716 { 00717 I2Cx_ITConfig(); 00718 } 00719 00720 /** 00721 * @brief IOE writes single data. 00722 * @param Addr: I2C address 00723 * @param Reg: Register address 00724 * @param Value: Data to be written 00725 */ 00726 void IOE_Write(uint8_t Addr, uint8_t Reg, uint8_t Value) 00727 { 00728 I2Cx_Write(Addr, Reg, Value); 00729 } 00730 00731 /** 00732 * @brief IOE reads single data. 00733 * @param Addr: I2C address 00734 * @param Reg: Register address 00735 * @retval Read data 00736 */ 00737 uint8_t IOE_Read(uint8_t Addr, uint8_t Reg) 00738 { 00739 return I2Cx_Read(Addr, Reg); 00740 } 00741 00742 /** 00743 * @brief IOE reads multiple data. 00744 * @param Addr: I2C address 00745 * @param Reg: Register address 00746 * @param Buffer: Pointer to data buffer 00747 * @param Length: Length of the data 00748 * @retval Number of read data 00749 */ 00750 uint16_t IOE_ReadMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length) 00751 { 00752 return I2Cx_ReadMultiple(Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT, Buffer, Length); 00753 } 00754 00755 /** 00756 * @brief IOE writes multiple data. 00757 * @param Addr: I2C address 00758 * @param Reg: Register address 00759 * @param Buffer: Pointer to data buffer 00760 * @param Length: Length of the data 00761 */ 00762 void IOE_WriteMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length) 00763 { 00764 I2Cx_WriteMultiple(Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT, Buffer, Length); 00765 } 00766 00767 /** 00768 * @brief IOE delay 00769 * @param Delay: Delay in ms 00770 */ 00771 void IOE_Delay(uint32_t Delay) 00772 { 00773 HAL_Delay(Delay); 00774 } 00775 00776 /********************************* LINK AUDIO *********************************/ 00777 00778 /** 00779 * @brief Initializes Audio low level. 00780 */ 00781 void AUDIO_IO_Init(void) 00782 { 00783 I2Cx_Init(); 00784 } 00785 00786 /** 00787 * @brief DeInitializes Audio low level. 00788 */ 00789 void AUDIO_IO_DeInit(void) 00790 { 00791 00792 } 00793 00794 /** 00795 * @brief Writes a single data. 00796 * @param Addr: I2C address 00797 * @param Reg: Reg address 00798 * @param Value: Data to be written 00799 */ 00800 void AUDIO_IO_Write(uint8_t Addr, uint16_t Reg, uint16_t Value) 00801 { 00802 uint16_t tmp = Value; 00803 00804 Value = ((uint16_t)(tmp >> 8) & 0x00FF); 00805 00806 Value |= ((uint16_t)(tmp << 8)& 0xFF00); 00807 00808 I2Cx_WriteMultiple(Addr, Reg, I2C_MEMADD_SIZE_16BIT,(uint8_t*)&Value, 2); 00809 } 00810 00811 /** 00812 * @brief Reads a single data. 00813 * @param Addr: I2C address 00814 * @param Reg: Reg address 00815 * @retval Data to be read 00816 */ 00817 uint16_t AUDIO_IO_Read(uint8_t Addr, uint16_t Reg) 00818 { 00819 uint16_t Read_Value = 0, tmp = 0; 00820 00821 I2Cx_ReadMultiple(Addr, Reg, I2C_MEMADD_SIZE_16BIT, (uint8_t*)&Read_Value, 2); 00822 00823 tmp = ((uint16_t)(Read_Value >> 8) & 0x00FF); 00824 00825 tmp |= ((uint16_t)(Read_Value << 8)& 0xFF00); 00826 00827 Read_Value = tmp; 00828 00829 return Read_Value; 00830 } 00831 00832 /** 00833 * @brief AUDIO Codec delay 00834 * @param Delay: Delay in ms 00835 */ 00836 void AUDIO_IO_Delay(uint32_t Delay) 00837 { 00838 HAL_Delay(Delay); 00839 } 00840 00841 /********************************* LINK CAMERA ********************************/ 00842 00843 /** 00844 * @brief Initializes Camera low level. 00845 */ 00846 void CAMERA_IO_Init(void) 00847 { 00848 I2Cx_Init(); 00849 } 00850 00851 /** 00852 * @brief Camera writes single data. 00853 * @param Addr: I2C address 00854 * @param Reg: Register address 00855 * @param Value: Data to be written 00856 */ 00857 void CAMERA_IO_Write(uint8_t Addr, uint8_t Reg, uint8_t Value) 00858 { 00859 I2Cx_Write(Addr, Reg, Value); 00860 } 00861 00862 /** 00863 * @brief Camera reads single data. 00864 * @param Addr: I2C address 00865 * @param Reg: Register address 00866 * @retval Read data 00867 */ 00868 uint8_t CAMERA_IO_Read(uint8_t Addr, uint8_t Reg) 00869 { 00870 return I2Cx_Read(Addr, Reg); 00871 } 00872 00873 /** 00874 * @brief Camera delay 00875 * @param Delay: Delay in ms 00876 */ 00877 void CAMERA_Delay(uint32_t Delay) 00878 { 00879 HAL_Delay(Delay); 00880 } 00881 00882 /******************************** LINK I2C EEPROM *****************************/ 00883 00884 /** 00885 * @brief Initializes peripherals used by the I2C EEPROM driver. 00886 */ 00887 void EEPROM_IO_Init(void) 00888 { 00889 I2Cx_Init(); 00890 } 00891 00892 /** 00893 * @brief Write data to I2C EEPROM driver in using DMA channel. 00894 * @param DevAddress: Target device address 00895 * @param MemAddress: Internal memory address 00896 * @param pBuffer: Pointer to data buffer 00897 * @param BufferSize: Amount of data to be sent 00898 * @retval HAL status 00899 */ 00900 HAL_StatusTypeDef EEPROM_IO_WriteData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize) 00901 { 00902 return (I2Cx_WriteMultiple(DevAddress, MemAddress, I2C_MEMADD_SIZE_16BIT, pBuffer, BufferSize)); 00903 } 00904 00905 /** 00906 * @brief Read data from I2C EEPROM driver in using DMA channel. 00907 * @param DevAddress: Target device address 00908 * @param MemAddress: Internal memory address 00909 * @param pBuffer: Pointer to data buffer 00910 * @param BufferSize: Amount of data to be read 00911 * @retval HAL status 00912 */ 00913 HAL_StatusTypeDef EEPROM_IO_ReadData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize) 00914 { 00915 return (I2Cx_ReadMultiple(DevAddress, MemAddress, I2C_MEMADD_SIZE_16BIT, pBuffer, BufferSize)); 00916 } 00917 00918 /** 00919 * @brief Checks if target device is ready for communication. 00920 * @note This function is used with Memory devices 00921 * @param DevAddress: Target device address 00922 * @param Trials: Number of trials 00923 * @retval HAL status 00924 */ 00925 HAL_StatusTypeDef EEPROM_IO_IsDeviceReady(uint16_t DevAddress, uint32_t Trials) 00926 { 00927 return (I2Cx_IsDeviceReady(DevAddress, Trials)); 00928 } 00929 00930 /** 00931 * @} 00932 */ 00933 00934 /** 00935 * @} 00936 */ 00937 00938 /** 00939 * @} 00940 */ 00941 00942 /** 00943 * @} 00944 */ 00945 00946 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Wed Jan 13 2016 15:52:54 for STM324x9I_EVAL BSP User Manual by
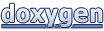