STM324x9I_EVAL BSP User Manual
|
stm324x9i_eval.h
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm324x9i_eval.h 00004 * @author MCD Application Team 00005 * @version V2.2.2 00006 * @date 13-January-2016 00007 * @brief This file contains definitions for STM324x9I_EVAL's LEDs, 00008 * push-buttons and COM ports hardware resources. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* Define to prevent recursive inclusion -------------------------------------*/ 00040 #ifndef __STM324X9I_EVAL_H 00041 #define __STM324X9I_EVAL_H 00042 00043 #ifdef __cplusplus 00044 extern "C" { 00045 #endif 00046 00047 /* Includes ------------------------------------------------------------------*/ 00048 #include "stm32f4xx_hal.h" 00049 00050 /** @addtogroup BSP 00051 * @{ 00052 */ 00053 00054 /** @addtogroup STM324x9I_EVAL 00055 * @{ 00056 */ 00057 00058 /** @addtogroup STM324x9I_EVAL_LOW_LEVEL 00059 * @{ 00060 */ 00061 00062 /** @defgroup STM324x9I_EVAL_LOW_LEVEL_Exported_Types STM324x9I EVAL LOW LEVEL Exported Types 00063 * @{ 00064 */ 00065 typedef enum 00066 { 00067 LED1 = 0, 00068 LED2 = 1, 00069 LED3 = 2, 00070 LED4 = 3 00071 }Led_TypeDef; 00072 00073 typedef enum 00074 { 00075 BUTTON_WAKEUP = 0, 00076 BUTTON_TAMPER = 1, 00077 BUTTON_KEY = 2 00078 }Button_TypeDef; 00079 00080 typedef enum 00081 { 00082 BUTTON_MODE_GPIO = 0, 00083 BUTTON_MODE_EXTI = 1 00084 }ButtonMode_TypeDef; 00085 00086 typedef enum 00087 { 00088 JOY_MODE_GPIO = 0, 00089 JOY_MODE_EXTI = 1 00090 }JOYMode_TypeDef; 00091 00092 typedef enum 00093 { 00094 JOY_NONE = 0, 00095 JOY_SEL = 1, 00096 JOY_DOWN = 2, 00097 JOY_LEFT = 3, 00098 JOY_RIGHT = 4, 00099 JOY_UP = 5 00100 }JOYState_TypeDef; 00101 00102 typedef enum 00103 { 00104 COM1 = 0, 00105 COM2 = 1 00106 }COM_TypeDef; 00107 /** 00108 * @} 00109 */ 00110 00111 /** @defgroup STM324x9I_EVAL_LOW_LEVEL_Exported_Constants STM324x9I EVAL LOW LEVEL Exported Constants 00112 * @{ 00113 */ 00114 00115 /** 00116 * @brief Define for STM324x9I_EVAL board 00117 */ 00118 #if !defined (USE_STM324x9I_EVAL) 00119 #define USE_STM324x9I_EVAL 00120 #endif 00121 00122 /** @defgroup STM324x9I_EVAL_LOW_LEVEL_LED STM324x9I EVAL LOW LEVEL LED 00123 * @{ 00124 */ 00125 #define LEDn 4 00126 00127 #define LED1_PIN GPIO_PIN_6 00128 #define LED1_GPIO_PORT GPIOG 00129 #define LED1_GPIO_CLK_ENABLE() __GPIOG_CLK_ENABLE() 00130 #define LED1_GPIO_CLK_DISABLE() __GPIOG_CLK_DISABLE() 00131 00132 00133 #define LED2_PIN GPIO_PIN_7 00134 #define LED2_GPIO_PORT GPIOG 00135 #define LED2_GPIO_CLK_ENABLE() __GPIOG_CLK_ENABLE() 00136 #define LED2_GPIO_CLK_DISABLE() __GPIOG_CLK_DISABLE() 00137 00138 #define LED3_PIN GPIO_PIN_10 00139 #define LED3_GPIO_PORT GPIOG 00140 #define LED3_GPIO_CLK_ENABLE() __GPIOG_CLK_ENABLE() 00141 #define LED3_GPIO_CLK_DISABLE() __GPIOG_CLK_DISABLE() 00142 00143 #define LED4_PIN GPIO_PIN_12 00144 #define LED4_GPIO_PORT GPIOG 00145 #define LED4_GPIO_CLK_ENABLE() __GPIOG_CLK_ENABLE() 00146 #define LED4_GPIO_CLK_DISABLE() __GPIOG_CLK_DISABLE() 00147 00148 #define LEDx_GPIO_CLK_ENABLE(__INDEX__) do{if((__INDEX__) == 0) LED1_GPIO_CLK_ENABLE(); else \ 00149 if((__INDEX__) == 1) LED2_GPIO_CLK_ENABLE(); else \ 00150 if((__INDEX__) == 2) LED3_GPIO_CLK_ENABLE(); else \ 00151 if((__INDEX__) == 3) LED4_GPIO_CLK_ENABLE(); \ 00152 }while(0) 00153 00154 #define LEDx_GPIO_CLK_DISABLE(__INDEX__) do{if((__INDEX__) == 0) LED1_GPIO_CLK_DISABLE(); else \ 00155 if((__INDEX__) == 1) LED2_GPIO_CLK_DISABLE(); else \ 00156 if((__INDEX__) == 2) LED3_GPIO_CLK_DISABLE(); else \ 00157 if((__INDEX__) == 3) LED4_GPIO_CLK_DISABLE(); \ 00158 }while(0) 00159 00160 /** 00161 * @} 00162 */ 00163 00164 /** @defgroup STM324x9I_EVAL_LOW_LEVEL_BUTTON STM324x9I EVAL LOW LEVEL BUTTON 00165 * @{ 00166 */ 00167 /* Joystick pins are connected to IO Expander (accessible through I2C1 interface) */ 00168 #define BUTTONn 3 00169 00170 /** 00171 * @brief Wakeup push-button 00172 */ 00173 #define WAKEUP_BUTTON_PIN GPIO_PIN_0 00174 #define WAKEUP_BUTTON_GPIO_PORT GPIOA 00175 #define WAKEUP_BUTTON_GPIO_CLK_ENABLE() __GPIOA_CLK_ENABLE() 00176 #define WAKEUP_BUTTON_GPIO_CLK_DISABLE() __GPIOA_CLK_DISABLE() 00177 #define WAKEUP_BUTTON_EXTI_IRQn EXTI0_IRQn 00178 00179 /** 00180 * @brief Tamper push-button 00181 */ 00182 #define TAMPER_BUTTON_PIN GPIO_PIN_13 00183 #define TAMPER_BUTTON_GPIO_PORT GPIOC 00184 #define TAMPER_BUTTON_GPIO_CLK_ENABLE() __GPIOC_CLK_ENABLE() 00185 #define TAMPER_BUTTON_GPIO_CLK_DISABLE() __GPIOC_CLK_DISABLE() 00186 #define TAMPER_BUTTON_EXTI_IRQn EXTI15_10_IRQn 00187 00188 /** 00189 * @brief Key push-button 00190 */ 00191 #define KEY_BUTTON_PIN GPIO_PIN_13 00192 #define KEY_BUTTON_GPIO_PORT GPIOC 00193 #define KEY_BUTTON_GPIO_CLK_ENABLE() __GPIOC_CLK_ENABLE() 00194 #define KEY_BUTTON_GPIO_CLK_DISABLE() __GPIOC_CLK_DISABLE() 00195 #define KEY_BUTTON_EXTI_IRQn EXTI15_10_IRQn 00196 00197 #define BUTTONx_GPIO_CLK_ENABLE(__INDEX__) do{if((__INDEX__) == 0) WAKEUP_BUTTON_GPIO_CLK_ENABLE(); else \ 00198 if((__INDEX__) == 1) TAMPER_BUTTON_GPIO_CLK_ENABLE(); else \ 00199 if ((__INDEX__) == 2) KEY_BUTTON_GPIO_CLK_ENABLE(); \ 00200 }while(0) 00201 #define BUTTONx_GPIO_CLK_DISABLE(__INDEX__) do{if((__INDEX__) == 0) WAKEUP_BUTTON_GPIO_CLK_DISABLE(); else \ 00202 if((__INDEX__) == 1) TAMPER_BUTTON_GPIO_CLK_DISABLE(); else \ 00203 if ((__INDEX__) == 2) KEY_BUTTON_GPIO_CLK_DISABLE(); \ 00204 }while(0) 00205 /** 00206 * @} 00207 */ 00208 00209 /** @defgroup STM324x9I_EVAL_LOW_LEVEL_COM STM324x9I EVAL LOW LEVEL COM 00210 * @{ 00211 */ 00212 #define COMn 1 00213 00214 /** 00215 * @brief Definition for COM port1, connected to USART1 00216 */ 00217 #define EVAL_COM1 USART1 00218 #define EVAL_COM1_CLK_ENABLE() __USART1_CLK_ENABLE() 00219 #define EVAL_COM1_CLK_DISABLE() __USART1_CLK_DISABLE() 00220 00221 #define EVAL_COM1_TX_PIN GPIO_PIN_9 00222 #define EVAL_COM1_TX_GPIO_PORT GPIOA 00223 #define EVAL_COM1_TX_GPIO_CLK_ENABLE() __GPIOA_CLK_ENABLE() 00224 #define EVAL_COM1_TX_GPIO_CLK_DISABLE() __GPIOA_CLK_DISABLE() 00225 #define EVAL_COM1_TX_AF GPIO_AF7_USART1 00226 00227 #define EVAL_COM1_RX_PIN GPIO_PIN_10 00228 #define EVAL_COM1_RX_GPIO_PORT GPIOA 00229 #define EVAL_COM1_RX_GPIO_CLK_ENABLE() __GPIOA_CLK_ENABLE() 00230 #define EVAL_COM1_RX_GPIO_CLK_DISABLE() __GPIOA_CLK_DISABLE() 00231 #define EVAL_COM1_RX_AF GPIO_AF7_USART1 00232 00233 #define EVAL_COM1_IRQn USART1_IRQn 00234 00235 #define EVAL_COMx_CLK_ENABLE(__INDEX__) do{if((__INDEX__) == 0) EVAL_COM1_CLK_ENABLE(); \ 00236 }while(0) 00237 #define EVAL_COMx_CLK_DISABLE(__INDEX__) do{if((__INDEX__) == 0) EVAL_COM1_CLK_DISABLE(); \ 00238 }while(0) 00239 00240 #define EVAL_COMx_TX_GPIO_CLK_ENABLE(__INDEX__) do{if((__INDEX__) == 0) EVAL_COM1_TX_GPIO_CLK_ENABLE(); \ 00241 }while(0) 00242 #define EVAL_COMx_TX_GPIO_CLK_DISABLE(__INDEX__) do{if((__INDEX__) == 0) EVAL_COM1_TX_GPIO_CLK_DISABLE(); \ 00243 }while(0) 00244 00245 #define EVAL_COMx_RX_GPIO_CLK_ENABLE(__INDEX__) do{if((__INDEX__) == 0) EVAL_COM1_RX_GPIO_CLK_ENABLE(); \ 00246 }while(0) 00247 #define EVAL_COMx_RX_GPIO_CLK_DISABLE(__INDEX__) do{if((__INDEX__) == 0) EVAL_COM1_RX_GPIO_CLK_DISABLE(); \ 00248 }while(0) 00249 00250 /** 00251 * @brief Joystick Pins definition 00252 */ 00253 #define JOY_SEL_PIN IO_PIN_14 00254 #define JOY_DOWN_PIN IO_PIN_13 00255 #define JOY_LEFT_PIN IO_PIN_12 00256 #define JOY_RIGHT_PIN IO_PIN_11 00257 #define JOY_UP_PIN IO_PIN_10 00258 #define JOY_NONE_PIN JOY_ALL_PINS 00259 #define JOY_ALL_PINS (IO_PIN_10 | IO_PIN_11 | IO_PIN_12 | IO_PIN_13 | IO_PIN_14) 00260 00261 /** 00262 * @brief Eval Pins definition 00263 */ 00264 #define XSDN_PIN IO_PIN_0 00265 #define MII_INT_PIN IO_PIN_1 00266 #define RSTI_PIN IO_PIN_2 00267 #define CAM_PLUG_PIN IO_PIN_3 00268 #define LCD_INT_PIN IO_PIN_4 00269 #define AUDIO_INT_PIN IO_PIN_5 00270 #define OTG_FS1_OVER_CURRENT_PIN IO_PIN_6 00271 #define OTG_FS1_POWER_SWITCH_PIN IO_PIN_7 00272 #define OTG_FS2_OVER_CURRENT_PIN IO_PIN_8 00273 #define OTG_FS2_POWER_SWITCH_PIN IO_PIN_9 00274 #define SD_DETECT_PIN IO_PIN_15 00275 00276 /* Exported constant IO ------------------------------------------------------*/ 00277 #define IO_I2C_ADDRESS 0x84 00278 #define TS_I2C_ADDRESS 0x82 00279 #define TS3510_I2C_ADDRESS 0x80 00280 #define EXC7200_I2C_ADDRESS 0x08 00281 #define CAMERA_I2C_ADDRESS 0x60 00282 #define AUDIO_I2C_ADDRESS 0x34 00283 #define EEPROM_I2C_ADDRESS_A01 0xA0 00284 #define EEPROM_I2C_ADDRESS_A02 0xA6 00285 /* I2C clock speed configuration (in Hz) 00286 WARNING: 00287 Make sure that this define is not already declared in other files (ie. 00288 stm324x9I_eval.h file). It can be used in parallel by other modules. */ 00289 #ifndef BSP_I2C_SPEED 00290 #define BSP_I2C_SPEED 100000 00291 #endif /* BSP_I2C_SPEED */ 00292 00293 /* User can use this section to tailor I2Cx/I2Cx instance used and associated 00294 resources */ 00295 /* Definition for I2Cx clock resources */ 00296 #define EVAL_I2Cx I2C1 00297 #define EVAL_I2Cx_CLK_ENABLE() __I2C1_CLK_ENABLE() 00298 #define EVAL_DMAx_CLK_ENABLE() __DMA1_CLK_ENABLE() 00299 #define EVAL_I2Cx_SCL_SDA_GPIO_CLK_ENABLE() __GPIOB_CLK_ENABLE() 00300 00301 #define EVAL_I2Cx_FORCE_RESET() __I2C1_FORCE_RESET() 00302 #define EVAL_I2Cx_RELEASE_RESET() __I2C1_RELEASE_RESET() 00303 00304 /* Definition for I2Cx Pins */ 00305 #define EVAL_I2Cx_SCL_PIN GPIO_PIN_6 00306 #define EVAL_I2Cx_SCL_SDA_GPIO_PORT GPIOB 00307 #define EVAL_I2Cx_SCL_SDA_AF GPIO_AF4_I2C1 00308 #define EVAL_I2Cx_SDA_PIN GPIO_PIN_9 00309 00310 /* I2C interrupt requests */ 00311 #define EVAL_I2Cx_EV_IRQn I2C1_EV_IRQn 00312 #define EVAL_I2Cx_ER_IRQn I2C1_ER_IRQn 00313 00314 /** 00315 * @} 00316 */ 00317 00318 /** 00319 * @} 00320 */ 00321 00322 /** @defgroup STM324x9I_EVAL_LOW_LEVEL_Exported_Macros STM324x9I EVAL LOW LEVEL Exported Macros 00323 * @{ 00324 */ 00325 /** 00326 * @} 00327 */ 00328 00329 /** @defgroup STM324x9I_EVAL_LOW_LEVEL_Exported_Functions STM324x9I EVAL LOW LEVEL Exported Functions 00330 * @{ 00331 */ 00332 uint32_t BSP_GetVersion(void); 00333 void BSP_LED_Init(Led_TypeDef Led); 00334 void BSP_LED_On(Led_TypeDef Led); 00335 void BSP_LED_Off(Led_TypeDef Led); 00336 void BSP_LED_Toggle(Led_TypeDef Led); 00337 void BSP_PB_Init(Button_TypeDef Button, ButtonMode_TypeDef Button_Mode); 00338 uint32_t BSP_PB_GetState(Button_TypeDef Button); 00339 void BSP_COM_Init(COM_TypeDef COM, UART_HandleTypeDef *husart); 00340 uint8_t BSP_JOY_Init(JOYMode_TypeDef Joy_Mode); 00341 JOYState_TypeDef BSP_JOY_GetState(void); 00342 uint8_t BSP_TS3510_IsDetected(void); 00343 00344 /** 00345 * @} 00346 */ 00347 00348 /** 00349 * @} 00350 */ 00351 00352 /** 00353 * @} 00354 */ 00355 00356 /** 00357 * @} 00358 */ 00359 00360 #ifdef __cplusplus 00361 } 00362 #endif 00363 00364 #endif /* __STM324X9I_EVAL_H */ 00365 00366 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Wed Jan 13 2016 15:52:54 for STM324x9I_EVAL BSP User Manual by
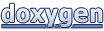