STM324x9I_EVAL BSP User Manual
|
stm324x9i_eval_sdram.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm324x9i_eval_sdram.c 00004 * @author MCD Application Team 00005 * @version V2.2.2 00006 * @date 13-January-2016 00007 * @brief This file includes the SDRAM driver for the MT48LC4M32B2B5-7 memory 00008 * device mounted on STM324x9I-EVAL evaluation board. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* File Info : ----------------------------------------------------------------- 00040 User NOTES 00041 1. How To use this driver: 00042 -------------------------- 00043 - This driver is used to drive the MT48LC4M32B2B5-7 SDRAM external memory mounted 00044 on STM324x9I-EVAL evaluation board. 00045 - This driver does not need a specific component driver for the SDRAM device 00046 to be included with. 00047 00048 2. Driver description: 00049 --------------------- 00050 + Initialization steps: 00051 o Initialize the SDRAM external memory using the BSP_SDRAM_Init() function. This 00052 function includes the MSP layer hardware resources initialization and the 00053 FMC controller configuration to interface with the external SDRAM memory. 00054 o It contains the SDRAM initialization sequence to program the SDRAM external 00055 device using the function BSP_SDRAM_Initialization_sequence(). Note that this 00056 sequence is standard for all SDRAM devices, but can include some differences 00057 from a device to another. If it is the case, the right sequence should be 00058 implemented separately. 00059 00060 + SDRAM read/write operations 00061 o SDRAM external memory can be accessed with read/write operations once it is 00062 initialized. 00063 Read/write operation can be performed with AHB access using the functions 00064 BSP_SDRAM_ReadData()/BSP_SDRAM_WriteData(), or by DMA transfer using the functions 00065 BSP_SDRAM_ReadData_DMA()/BSP_SDRAM_WriteData_DMA(). 00066 o The AHB access is performed with 32-bit width transaction, the DMA transfer 00067 configuration is fixed at single (no burst) word transfer (see the 00068 SDRAM_MspInit() static function). 00069 o User can implement his own functions for read/write access with his desired 00070 configurations. 00071 o If interrupt mode is used for DMA transfer, the function BSP_SDRAM_DMA_IRQHandler() 00072 is called in IRQ handler file, to serve the generated interrupt once the DMA 00073 transfer is complete. 00074 o You can send a command to the SDRAM device in runtime using the function 00075 BSP_SDRAM_Sendcmd(), and giving the desired command as parameter chosen between 00076 the predefined commands of the "FMC_SDRAM_CommandTypeDef" structure. 00077 00078 ------------------------------------------------------------------------------*/ 00079 00080 /* Includes ------------------------------------------------------------------*/ 00081 #include "stm324x9i_eval_sdram.h" 00082 00083 /** @addtogroup BSP 00084 * @{ 00085 */ 00086 00087 /** @addtogroup STM324x9I_EVAL 00088 * @{ 00089 */ 00090 00091 /** @defgroup STM324x9I_EVAL_SDRAM STM324x9I EVAL SDRAM 00092 * @{ 00093 */ 00094 00095 /** @defgroup STM324x9I_EVAL_SDRAM_Private_Types_Definitions STM324x9I EVAL SDRAM Private Types Definitions 00096 * @{ 00097 */ 00098 /** 00099 * @} 00100 */ 00101 00102 /** @defgroup STM324x9I_EVAL_SDRAM_Private_Defines STM324x9I EVAL SDRAM Private Defines 00103 * @{ 00104 */ 00105 /** 00106 * @} 00107 */ 00108 00109 /** @defgroup STM324x9I_EVAL_SDRAM_Private_Macros STM324x9I EVAL SDRAM Private Macros 00110 * @{ 00111 */ 00112 /** 00113 * @} 00114 */ 00115 00116 /** @defgroup STM324x9I_EVAL_SDRAM_Private_Variables STM324x9I EVAL SDRAM Private Variables 00117 * @{ 00118 */ 00119 static SDRAM_HandleTypeDef sdramHandle; 00120 static FMC_SDRAM_TimingTypeDef Timing; 00121 static FMC_SDRAM_CommandTypeDef Command; 00122 /** 00123 * @} 00124 */ 00125 00126 /** @defgroup STM324x9I_EVAL_SDRAM_Private_Function_Prototypes STM324x9I EVAL SDRAM Private Function Prototypes 00127 * @{ 00128 */ 00129 static void SDRAM_MspInit(void); 00130 /** 00131 * @} 00132 */ 00133 00134 /** @defgroup STM324x9I_EVAL_SDRAM_Private_Functions STM324x9I EVAL SDRAM Private Functions 00135 * @{ 00136 */ 00137 00138 /** 00139 * @brief Initializes the SDRAM device. 00140 * @retval SDRAM status 00141 */ 00142 uint8_t BSP_SDRAM_Init(void) 00143 { 00144 static uint8_t sdramstatus = SDRAM_ERROR; 00145 /* SDRAM device configuration */ 00146 sdramHandle.Instance = FMC_SDRAM_DEVICE; 00147 00148 /* Timing configuration for 90Mhz as SD clock frequency (System clock is up to 180Mhz */ 00149 Timing.LoadToActiveDelay = 2; 00150 Timing.ExitSelfRefreshDelay = 7; 00151 Timing.SelfRefreshTime = 4; 00152 Timing.RowCycleDelay = 7; 00153 Timing.WriteRecoveryTime = 2; 00154 Timing.RPDelay = 2; 00155 Timing.RCDDelay = 2; 00156 00157 sdramHandle.Init.SDBank = FMC_SDRAM_BANK1; 00158 sdramHandle.Init.ColumnBitsNumber = FMC_SDRAM_COLUMN_BITS_NUM_9; 00159 sdramHandle.Init.RowBitsNumber = FMC_SDRAM_ROW_BITS_NUM_12; 00160 sdramHandle.Init.MemoryDataWidth = SDRAM_MEMORY_WIDTH; 00161 sdramHandle.Init.InternalBankNumber = FMC_SDRAM_INTERN_BANKS_NUM_4; 00162 sdramHandle.Init.CASLatency = FMC_SDRAM_CAS_LATENCY_3; 00163 sdramHandle.Init.WriteProtection = FMC_SDRAM_WRITE_PROTECTION_DISABLE; 00164 sdramHandle.Init.SDClockPeriod = SDCLOCK_PERIOD; 00165 sdramHandle.Init.ReadBurst = FMC_SDRAM_RBURST_ENABLE; 00166 sdramHandle.Init.ReadPipeDelay = FMC_SDRAM_RPIPE_DELAY_0; 00167 00168 /* SDRAM controller initialization */ 00169 SDRAM_MspInit(); 00170 if(HAL_SDRAM_Init(&sdramHandle, &Timing) != HAL_OK) 00171 { 00172 sdramstatus = SDRAM_ERROR; 00173 } 00174 else 00175 { 00176 sdramstatus = SDRAM_OK; 00177 } 00178 00179 /* SDRAM initialization sequence */ 00180 BSP_SDRAM_Initialization_sequence(REFRESH_COUNT); 00181 00182 return sdramstatus; 00183 } 00184 00185 /** 00186 * @brief Programs the SDRAM device. 00187 * @param RefreshCount: SDRAM refresh counter value 00188 */ 00189 void BSP_SDRAM_Initialization_sequence(uint32_t RefreshCount) 00190 { 00191 __IO uint32_t tmpmrd = 0; 00192 00193 /* Step 1: Configure a clock configuration enable command */ 00194 Command.CommandMode = FMC_SDRAM_CMD_CLK_ENABLE; 00195 Command.CommandTarget = FMC_SDRAM_CMD_TARGET_BANK1; 00196 Command.AutoRefreshNumber = 1; 00197 Command.ModeRegisterDefinition = 0; 00198 00199 /* Send the command */ 00200 HAL_SDRAM_SendCommand(&sdramHandle, &Command, SDRAM_TIMEOUT); 00201 00202 /* Step 2: Insert 100 us minimum delay */ 00203 /* Inserted delay is equal to 1 ms due to systick time base unit (ms) */ 00204 HAL_Delay(1); 00205 00206 /* Step 3: Configure a PALL (precharge all) command */ 00207 Command.CommandMode = FMC_SDRAM_CMD_PALL; 00208 Command.CommandTarget = FMC_SDRAM_CMD_TARGET_BANK1; 00209 Command.AutoRefreshNumber = 1; 00210 Command.ModeRegisterDefinition = 0; 00211 00212 /* Send the command */ 00213 HAL_SDRAM_SendCommand(&sdramHandle, &Command, SDRAM_TIMEOUT); 00214 00215 /* Step 4: Configure an Auto Refresh command */ 00216 Command.CommandMode = FMC_SDRAM_CMD_AUTOREFRESH_MODE; 00217 Command.CommandTarget = FMC_SDRAM_CMD_TARGET_BANK1; 00218 Command.AutoRefreshNumber = 8; 00219 Command.ModeRegisterDefinition = 0; 00220 00221 /* Send the command */ 00222 HAL_SDRAM_SendCommand(&sdramHandle, &Command, SDRAM_TIMEOUT); 00223 00224 /* Step 5: Program the external memory mode register */ 00225 tmpmrd = (uint32_t)SDRAM_MODEREG_BURST_LENGTH_1 |\ 00226 SDRAM_MODEREG_BURST_TYPE_SEQUENTIAL |\ 00227 SDRAM_MODEREG_CAS_LATENCY_3 |\ 00228 SDRAM_MODEREG_OPERATING_MODE_STANDARD |\ 00229 SDRAM_MODEREG_WRITEBURST_MODE_SINGLE; 00230 00231 Command.CommandMode = FMC_SDRAM_CMD_LOAD_MODE; 00232 Command.CommandTarget = FMC_SDRAM_CMD_TARGET_BANK1; 00233 Command.AutoRefreshNumber = 1; 00234 Command.ModeRegisterDefinition = tmpmrd; 00235 00236 /* Send the command */ 00237 HAL_SDRAM_SendCommand(&sdramHandle, &Command, SDRAM_TIMEOUT); 00238 00239 /* Step 6: Set the refresh rate counter */ 00240 /* Set the device refresh rate */ 00241 HAL_SDRAM_ProgramRefreshRate(&sdramHandle, RefreshCount); 00242 } 00243 00244 /** 00245 * @brief Reads an mount of data from the SDRAM memory in polling mode. 00246 * @param uwStartAddress: Read start address 00247 * @param pData: Pointer to data to be read 00248 * @param uwDataSize: Size of read data from the memory 00249 * @retval SDRAM status 00250 */ 00251 uint8_t BSP_SDRAM_ReadData(uint32_t uwStartAddress, uint32_t *pData, uint32_t uwDataSize) 00252 { 00253 if(HAL_SDRAM_Read_32b(&sdramHandle, (uint32_t *)uwStartAddress, pData, uwDataSize) != HAL_OK) 00254 { 00255 return SDRAM_ERROR; 00256 } 00257 else 00258 { 00259 return SDRAM_OK; 00260 } 00261 } 00262 00263 /** 00264 * @brief Reads an mount of data from the SDRAM memory in DMA mode. 00265 * @param uwStartAddress: Read start address 00266 * @param pData: Pointer to data to be read 00267 * @param uwDataSize: Size of read data from the memory 00268 * @retval SDRAM status 00269 */ 00270 uint8_t BSP_SDRAM_ReadData_DMA(uint32_t uwStartAddress, uint32_t *pData, uint32_t uwDataSize) 00271 { 00272 if(HAL_SDRAM_Read_DMA(&sdramHandle, (uint32_t *)uwStartAddress, pData, uwDataSize) != HAL_OK) 00273 { 00274 return SDRAM_ERROR; 00275 } 00276 else 00277 { 00278 return SDRAM_OK; 00279 } 00280 } 00281 00282 /** 00283 * @brief Writes an mount of data to the SDRAM memory in polling mode. 00284 * @param uwStartAddress: Write start address 00285 * @param pData: Pointer to data to be written 00286 * @param uwDataSize: Size of written data from the memory 00287 * @retval SDRAM status 00288 */ 00289 uint8_t BSP_SDRAM_WriteData(uint32_t uwStartAddress, uint32_t *pData, uint32_t uwDataSize) 00290 { 00291 if(HAL_SDRAM_Write_32b(&sdramHandle, (uint32_t *)uwStartAddress, pData, uwDataSize) != HAL_OK) 00292 { 00293 return SDRAM_ERROR; 00294 } 00295 else 00296 { 00297 return SDRAM_OK; 00298 } 00299 } 00300 00301 /** 00302 * @brief Writes an mount of data to the SDRAM memory in DMA mode. 00303 * @param uwStartAddress: Write start address 00304 * @param pData: Pointer to data to be written 00305 * @param uwDataSize: Size of written data from the memory 00306 * @retval SDRAM status 00307 */ 00308 uint8_t BSP_SDRAM_WriteData_DMA(uint32_t uwStartAddress, uint32_t *pData, uint32_t uwDataSize) 00309 { 00310 if(HAL_SDRAM_Write_DMA(&sdramHandle, (uint32_t *)uwStartAddress, pData, uwDataSize) != HAL_OK) 00311 { 00312 return SDRAM_ERROR; 00313 } 00314 else 00315 { 00316 return SDRAM_OK; 00317 } 00318 } 00319 00320 /** 00321 * @brief Sends command to the SDRAM bank. 00322 * @param SdramCmd: Pointer to SDRAM command structure 00323 * @retval HAL status 00324 */ 00325 uint8_t BSP_SDRAM_Sendcmd(FMC_SDRAM_CommandTypeDef *SdramCmd) 00326 { 00327 if(HAL_SDRAM_SendCommand(&sdramHandle, SdramCmd, SDRAM_TIMEOUT) != HAL_OK) 00328 { 00329 return SDRAM_ERROR; 00330 } 00331 else 00332 { 00333 return SDRAM_OK; 00334 } 00335 } 00336 00337 /** 00338 * @brief Handles SDRAM DMA transfer interrupt request. 00339 */ 00340 void BSP_SDRAM_DMA_IRQHandler(void) 00341 { 00342 HAL_DMA_IRQHandler(sdramHandle.hdma); 00343 } 00344 00345 /** 00346 * @brief Initializes SDRAM MSP. 00347 */ 00348 static void SDRAM_MspInit(void) 00349 { 00350 static DMA_HandleTypeDef dmaHandle; 00351 GPIO_InitTypeDef GPIO_Init_Structure; 00352 SDRAM_HandleTypeDef *hsdram = &sdramHandle; 00353 00354 /* Enable FMC clock */ 00355 __FMC_CLK_ENABLE(); 00356 00357 /* Enable chosen DMAx clock */ 00358 __DMAx_CLK_ENABLE(); 00359 00360 /* Enable GPIOs clock */ 00361 __GPIOD_CLK_ENABLE(); 00362 __GPIOE_CLK_ENABLE(); 00363 __GPIOF_CLK_ENABLE(); 00364 __GPIOG_CLK_ENABLE(); 00365 __GPIOH_CLK_ENABLE(); 00366 __GPIOI_CLK_ENABLE(); 00367 00368 /* Common GPIO configuration */ 00369 GPIO_Init_Structure.Mode = GPIO_MODE_AF_PP; 00370 GPIO_Init_Structure.Pull = GPIO_PULLUP; 00371 GPIO_Init_Structure.Speed = GPIO_SPEED_FAST; 00372 GPIO_Init_Structure.Alternate = GPIO_AF12_FMC; 00373 00374 /* GPIOD configuration */ 00375 GPIO_Init_Structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_8| GPIO_PIN_9 | GPIO_PIN_10 |\ 00376 GPIO_PIN_14 | GPIO_PIN_15; 00377 00378 00379 HAL_GPIO_Init(GPIOD, &GPIO_Init_Structure); 00380 00381 /* GPIOE configuration */ 00382 GPIO_Init_Structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_7| GPIO_PIN_8 | GPIO_PIN_9 |\ 00383 GPIO_PIN_10 | GPIO_PIN_11 | GPIO_PIN_12 | GPIO_PIN_13 | GPIO_PIN_14 |\ 00384 GPIO_PIN_15; 00385 00386 HAL_GPIO_Init(GPIOE, &GPIO_Init_Structure); 00387 00388 /* GPIOF configuration */ 00389 GPIO_Init_Structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_2| GPIO_PIN_3 | GPIO_PIN_4 |\ 00390 GPIO_PIN_5 | GPIO_PIN_11 | GPIO_PIN_12 | GPIO_PIN_13 | GPIO_PIN_14 |\ 00391 GPIO_PIN_15; 00392 00393 HAL_GPIO_Init(GPIOF, &GPIO_Init_Structure); 00394 00395 /* GPIOG configuration */ 00396 GPIO_Init_Structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_4| GPIO_PIN_5 | GPIO_PIN_8 |\ 00397 GPIO_PIN_15; 00398 HAL_GPIO_Init(GPIOG, &GPIO_Init_Structure); 00399 00400 /* GPIOH configuration */ 00401 GPIO_Init_Structure.Pin = GPIO_PIN_2 | GPIO_PIN_3 | GPIO_PIN_5 | GPIO_PIN_8 | GPIO_PIN_9 |\ 00402 GPIO_PIN_10 | GPIO_PIN_11 | GPIO_PIN_12 | GPIO_PIN_13 | GPIO_PIN_14 |\ 00403 GPIO_PIN_15; 00404 HAL_GPIO_Init(GPIOH, &GPIO_Init_Structure); 00405 00406 /* GPIOI configuration */ 00407 GPIO_Init_Structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_2 | GPIO_PIN_3 | GPIO_PIN_4 |\ 00408 GPIO_PIN_5 | GPIO_PIN_6 | GPIO_PIN_7 | GPIO_PIN_9 | GPIO_PIN_10; 00409 HAL_GPIO_Init(GPIOI, &GPIO_Init_Structure); 00410 00411 /* Configure common DMA parameters */ 00412 dmaHandle.Init.Channel = SDRAM_DMAx_CHANNEL; 00413 dmaHandle.Init.Direction = DMA_MEMORY_TO_MEMORY; 00414 dmaHandle.Init.PeriphInc = DMA_PINC_ENABLE; 00415 dmaHandle.Init.MemInc = DMA_MINC_ENABLE; 00416 dmaHandle.Init.PeriphDataAlignment = DMA_PDATAALIGN_WORD; 00417 dmaHandle.Init.MemDataAlignment = DMA_MDATAALIGN_WORD; 00418 dmaHandle.Init.Mode = DMA_NORMAL; 00419 dmaHandle.Init.Priority = DMA_PRIORITY_HIGH; 00420 dmaHandle.Init.FIFOMode = DMA_FIFOMODE_DISABLE; 00421 dmaHandle.Init.FIFOThreshold = DMA_FIFO_THRESHOLD_FULL; 00422 dmaHandle.Init.MemBurst = DMA_MBURST_SINGLE; 00423 dmaHandle.Init.PeriphBurst = DMA_PBURST_SINGLE; 00424 00425 dmaHandle.Instance = SDRAM_DMAx_STREAM; 00426 00427 /* Associate the DMA handle */ 00428 __HAL_LINKDMA(hsdram, hdma, dmaHandle); 00429 00430 /* Deinitialize the stream for new transfer */ 00431 HAL_DMA_DeInit(&dmaHandle); 00432 00433 /* Configure the DMA stream */ 00434 HAL_DMA_Init(&dmaHandle); 00435 00436 /* NVIC configuration for DMA transfer complete interrupt */ 00437 HAL_NVIC_SetPriority(SDRAM_DMAx_IRQn, 5, 0); 00438 HAL_NVIC_EnableIRQ(SDRAM_DMAx_IRQn); 00439 } 00440 00441 /** 00442 * @} 00443 */ 00444 00445 /** 00446 * @} 00447 */ 00448 00449 /** 00450 * @} 00451 */ 00452 00453 /** 00454 * @} 00455 */ 00456 00457 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Wed Jan 13 2016 15:52:54 for STM324x9I_EVAL BSP User Manual by
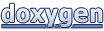