STM324x9I_EVAL BSP User Manual
|
stm324x9i_eval_lcd.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm324x9i_eval_lcd.c 00004 * @author MCD Application Team 00005 * @version V2.2.2 00006 * @date 13-January-2016 00007 * @brief This file includes the driver for Liquid Crystal Display (LCD) module 00008 * mounted on STM324x9I-EVAL evaluation board. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* File Info: ------------------------------------------------------------------ 00040 User NOTES 00041 1. How To use this driver: 00042 -------------------------- 00043 - This driver is used to drive directly an LCD TFT using the LTDC controller. 00044 - This driver selects dynamically the mounted LCD, AMPIRE 640x480 LCD mounted 00045 on MB1063 or AMPIRE 480x272 LCD mounted on MB1046 daughter board, 00046 and uses the adequate timing and setting for the specified LCD using 00047 device ID of the STMPE811 mounted on MB1046 daughter board. 00048 00049 2. Driver description: 00050 --------------------- 00051 + Initialization steps: 00052 o Initialize the LCD using the BSP_LCD_Init() function. 00053 o Apply the Layer configuration using the BSP_LCD_LayerDefaultInit() function. 00054 o Select the LCD layer to be used using the BSP_LCD_SelectLayer() function. 00055 o Enable the LCD display using the BSP_LCD_DisplayOn() function. 00056 00057 + Options 00058 o Configure and enable the color keying functionality using the 00059 BSP_LCD_SetColorKeying() function. 00060 o Modify in the fly the transparency and/or the frame buffer address 00061 using the following functions: 00062 - BSP_LCD_SetTransparency() 00063 - BSP_LCD_SetLayerAddress() 00064 00065 + Display on LCD 00066 o Clear the hole LCD using BSP_LCD_Clear() function or only one specified string 00067 line using the BSP_LCD_ClearStringLine() function. 00068 o Display a character on the specified line and column using the BSP_LCD_DisplayChar() 00069 function or a complete string line using the BSP_LCD_DisplayStringAtLine() function. 00070 o Display a string line on the specified position (x,y in pixel) and align mode 00071 using the BSP_LCD_DisplayStringAtLine() function. 00072 o Draw and fill a basic shapes (dot, line, rectangle, circle, ellipse, .. bitmap) 00073 on LCD using the available set of functions. 00074 00075 ------------------------------------------------------------------------------*/ 00076 00077 /* Includes ------------------------------------------------------------------*/ 00078 #include "stm324x9i_eval_lcd.h" 00079 #include "../../../Utilities/Fonts/fonts.h" 00080 #include "../../../Utilities/Fonts/font24.c" 00081 #include "../../../Utilities/Fonts/font20.c" 00082 #include "../../../Utilities/Fonts/font16.c" 00083 #include "../../../Utilities/Fonts/font12.c" 00084 #include "../../../Utilities/Fonts/font8.c" 00085 00086 /** @addtogroup BSP 00087 * @{ 00088 */ 00089 00090 /** @addtogroup STM324x9I_EVAL 00091 * @{ 00092 */ 00093 00094 /** @defgroup STM324x9I_EVAL_LCD STM324x9I EVAL LCD 00095 * @{ 00096 */ 00097 00098 /** @defgroup STM324x9I_EVAL_LCD_Private_TypesDefinitions STM324x9I EVAL LCD Private TypesDefinitions 00099 * @{ 00100 */ 00101 /** 00102 * @} 00103 */ 00104 00105 /** @defgroup STM324x9I_EVAL_LCD_Private_Defines STM324x9I EVAL LCD Private Defines 00106 * @{ 00107 */ 00108 #define POLY_X(Z) ((int32_t)((Points + Z)->X)) 00109 #define POLY_Y(Z) ((int32_t)((Points + Z)->Y)) 00110 /** 00111 * @} 00112 */ 00113 00114 /** @defgroup STM324x9I_EVAL_LCD_Private_Macros STM324x9I EVAL LCD Private Macros 00115 * @{ 00116 */ 00117 #define ABS(X) ((X) > 0 ? (X) : -(X)) 00118 /** 00119 * @} 00120 */ 00121 00122 /** @defgroup STM324x9I_EVAL_LCD_Private_Variables STM324x9I EVAL LCD Private Variables 00123 * @{ 00124 */ 00125 static LTDC_HandleTypeDef hltdc_eval; 00126 static DMA2D_HandleTypeDef hdma2d_eval; 00127 static uint32_t PCLK_profile = LCD_MAX_PCLK; 00128 00129 /* Default LCD configuration with LCD Layer 1 */ 00130 static uint32_t ActiveLayer = 0; 00131 static LCD_DrawPropTypeDef DrawProp[MAX_LAYER_NUMBER]; 00132 /** 00133 * @} 00134 */ 00135 00136 /** @defgroup STM324x9I_EVAL_LCD_Private_FunctionPrototypes STM324x9I EVAL LCD Private FunctionPrototypes 00137 * @{ 00138 */ 00139 static void MspInit(void); 00140 static void DrawChar(uint16_t Xpos, uint16_t Ypos, const uint8_t *c); 00141 static void FillTriangle(uint16_t x1, uint16_t x2, uint16_t x3, uint16_t y1, uint16_t y2, uint16_t y3); 00142 static void LL_FillBuffer(uint32_t LayerIndex, void *pDst, uint32_t xSize, uint32_t ySize, uint32_t OffLine, uint32_t ColorIndex); 00143 static void LL_ConvertLineToARGB8888(void * pSrc, void *pDst, uint32_t xSize, uint32_t ColorMode); 00144 /** 00145 * @} 00146 */ 00147 00148 /** @defgroup STM324x9I_EVAL_LCD_Private_Functions STM324x9I EVAL LCD Private Functions 00149 * @{ 00150 */ 00151 /** 00152 * @brief Initializes the LCD. 00153 * @retval LCD state 00154 */ 00155 uint8_t BSP_LCD_Init(void) 00156 { 00157 return (BSP_LCD_InitEx(LCD_MAX_PCLK)); 00158 } 00159 00160 /** 00161 * @brief Initializes the LCD. 00162 * @param PclkConfig : pixel clock profile 00163 * @retval LCD state 00164 */ 00165 uint8_t BSP_LCD_InitEx(uint32_t PclkConfig) 00166 { 00167 PCLK_profile = PclkConfig; 00168 00169 /* Select the used LCD */ 00170 /* The AMPIRE 480x272 does not contain an ID register then we check the availability 00171 of AMPIRE 480x640 LCD using device ID of the STMPE811 mounted on MB1046 daughter board */ 00172 if(stmpe811_ts_drv.ReadID(TS_I2C_ADDRESS) == STMPE811_ID) 00173 { 00174 /* The AMPIRE LCD 480x272 is selected */ 00175 /* Timing Configuration */ 00176 hltdc_eval.Init.HorizontalSync = (AMPIRE480272_HSYNC - 1); 00177 hltdc_eval.Init.VerticalSync = (AMPIRE480272_VSYNC - 1); 00178 hltdc_eval.Init.AccumulatedHBP = (AMPIRE480272_HSYNC + AMPIRE480272_HBP - 1); 00179 hltdc_eval.Init.AccumulatedVBP = (AMPIRE480272_VSYNC + AMPIRE480272_VBP - 1); 00180 hltdc_eval.Init.AccumulatedActiveH = (AMPIRE480272_HEIGHT + AMPIRE480272_VSYNC + AMPIRE480272_VBP - 1); 00181 hltdc_eval.Init.AccumulatedActiveW = (AMPIRE480272_WIDTH + AMPIRE480272_HSYNC + AMPIRE480272_HBP - 1); 00182 hltdc_eval.Init.TotalHeigh = (AMPIRE480272_HEIGHT + AMPIRE480272_VSYNC + AMPIRE480272_VBP + AMPIRE480272_VFP - 1); 00183 hltdc_eval.Init.TotalWidth = (AMPIRE480272_WIDTH + AMPIRE480272_HSYNC + AMPIRE480272_HBP + AMPIRE480272_HFP - 1); 00184 00185 /* Initialize the LCD pixel width and pixel height */ 00186 hltdc_eval.LayerCfg->ImageWidth = AMPIRE480272_WIDTH; 00187 hltdc_eval.LayerCfg->ImageHeight = AMPIRE480272_HEIGHT; 00188 } 00189 else 00190 { 00191 /* The LCD AMPIRE 640x480 is selected */ 00192 /* Timing configuration */ 00193 hltdc_eval.Init.HorizontalSync = (AMPIRE640480_HSYNC - 1); 00194 hltdc_eval.Init.VerticalSync = (AMPIRE640480_VSYNC - 1); 00195 hltdc_eval.Init.AccumulatedHBP = (AMPIRE640480_HSYNC + AMPIRE640480_HBP - 1); 00196 hltdc_eval.Init.AccumulatedVBP = (AMPIRE640480_VSYNC + AMPIRE640480_VBP - 1); 00197 hltdc_eval.Init.AccumulatedActiveH = (AMPIRE640480_HEIGHT + AMPIRE640480_VSYNC + AMPIRE640480_VBP - 1); 00198 hltdc_eval.Init.AccumulatedActiveW = (AMPIRE640480_WIDTH + AMPIRE640480_HSYNC + AMPIRE640480_HBP - 1); 00199 hltdc_eval.Init.TotalHeigh = (AMPIRE640480_HEIGHT + AMPIRE640480_VSYNC + AMPIRE640480_VBP + AMPIRE640480_VFP - 1); 00200 hltdc_eval.Init.TotalWidth = (AMPIRE640480_WIDTH + AMPIRE640480_HSYNC + AMPIRE640480_HBP + AMPIRE640480_HFP - 1); 00201 00202 /* Initialize the LCD pixel width and pixel height */ 00203 hltdc_eval.LayerCfg->ImageWidth = AMPIRE640480_WIDTH; 00204 hltdc_eval.LayerCfg->ImageHeight = AMPIRE640480_HEIGHT; 00205 } 00206 00207 /* Background value */ 00208 hltdc_eval.Init.Backcolor.Blue = 0; 00209 hltdc_eval.Init.Backcolor.Green = 0; 00210 hltdc_eval.Init.Backcolor.Red = 0; 00211 00212 /* Polarity */ 00213 hltdc_eval.Init.HSPolarity = LTDC_HSPOLARITY_AL; 00214 hltdc_eval.Init.VSPolarity = LTDC_VSPOLARITY_AL; 00215 hltdc_eval.Init.DEPolarity = LTDC_DEPOLARITY_AL; 00216 hltdc_eval.Init.PCPolarity = LTDC_PCPOLARITY_IPC; 00217 hltdc_eval.Instance = LTDC; 00218 00219 /* LCD clock configuration */ 00220 BSP_LCD_ClockConfig(&hltdc_eval, &PCLK_profile); 00221 00222 MspInit(); 00223 HAL_LTDC_Init(&hltdc_eval); 00224 00225 #if !defined(DATA_IN_ExtSDRAM) 00226 /* Initialize the SDRAM */ 00227 BSP_SDRAM_Init(); 00228 #endif /* DATA_IN_ExtSDRAM */ 00229 00230 /* Initialize the font */ 00231 BSP_LCD_SetFont(&LCD_DEFAULT_FONT); 00232 00233 return LCD_OK; 00234 } 00235 00236 /** 00237 * @brief Gets the LCD X size. 00238 * @retval Used LCD X size 00239 */ 00240 uint32_t BSP_LCD_GetXSize(void) 00241 { 00242 return hltdc_eval.LayerCfg[ActiveLayer].ImageWidth; 00243 } 00244 00245 /** 00246 * @brief Gets the LCD Y size. 00247 * @retval Used LCD Y size 00248 */ 00249 uint32_t BSP_LCD_GetYSize(void) 00250 { 00251 return hltdc_eval.LayerCfg[ActiveLayer].ImageHeight; 00252 } 00253 00254 /** 00255 * @brief Initializes the LCD layers. 00256 * @param LayerIndex: Layer foreground or background 00257 * @param FB_Address: Layer frame buffer 00258 */ 00259 void BSP_LCD_LayerDefaultInit(uint16_t LayerIndex, uint32_t FB_Address) 00260 { 00261 LCD_LayerCfgTypeDef Layercfg; 00262 00263 /* Layer Init */ 00264 Layercfg.WindowX0 = 0; 00265 Layercfg.WindowX1 = BSP_LCD_GetXSize(); 00266 Layercfg.WindowY0 = 0; 00267 Layercfg.WindowY1 = BSP_LCD_GetYSize(); 00268 Layercfg.PixelFormat = LTDC_PIXEL_FORMAT_ARGB8888; 00269 Layercfg.FBStartAdress = FB_Address; 00270 Layercfg.Alpha = 255; 00271 Layercfg.Alpha0 = 0; 00272 Layercfg.Backcolor.Blue = 0; 00273 Layercfg.Backcolor.Green = 0; 00274 Layercfg.Backcolor.Red = 0; 00275 Layercfg.BlendingFactor1 = LTDC_BLENDING_FACTOR1_PAxCA; 00276 Layercfg.BlendingFactor2 = LTDC_BLENDING_FACTOR2_PAxCA; 00277 Layercfg.ImageWidth = BSP_LCD_GetXSize(); 00278 Layercfg.ImageHeight = BSP_LCD_GetYSize(); 00279 00280 HAL_LTDC_ConfigLayer(&hltdc_eval, &Layercfg, LayerIndex); 00281 00282 DrawProp[LayerIndex].BackColor = LCD_COLOR_WHITE; 00283 DrawProp[LayerIndex].pFont = &Font24; 00284 DrawProp[LayerIndex].TextColor = LCD_COLOR_BLACK; 00285 } 00286 00287 /** 00288 * @brief Selects the LCD Layer. 00289 * @param LayerIndex: Layer foreground or background 00290 */ 00291 void BSP_LCD_SelectLayer(uint32_t LayerIndex) 00292 { 00293 ActiveLayer = LayerIndex; 00294 } 00295 00296 /** 00297 * @brief Sets an LCD Layer visible 00298 * @param LayerIndex: Visible Layer 00299 * @param State: New state of the specified layer 00300 * This parameter can be one of the following values: 00301 * @arg ENABLE 00302 * @arg DISABLE 00303 */ 00304 void BSP_LCD_SetLayerVisible(uint32_t LayerIndex, FunctionalState State) 00305 { 00306 if(State == ENABLE) 00307 { 00308 __HAL_LTDC_LAYER_ENABLE(&hltdc_eval, LayerIndex); 00309 } 00310 else 00311 { 00312 __HAL_LTDC_LAYER_DISABLE(&hltdc_eval, LayerIndex); 00313 } 00314 __HAL_LTDC_RELOAD_CONFIG(&hltdc_eval); 00315 } 00316 00317 /** 00318 * @brief Configures the transparency. 00319 * @param LayerIndex: Layer foreground or background. 00320 * @param Transparency: Transparency 00321 * This parameter must be a number between Min_Data = 0x00 and Max_Data = 0xFF 00322 */ 00323 void BSP_LCD_SetTransparency(uint32_t LayerIndex, uint8_t Transparency) 00324 { 00325 HAL_LTDC_SetAlpha(&hltdc_eval, Transparency, LayerIndex); 00326 } 00327 00328 /** 00329 * @brief Sets an LCD layer frame buffer address. 00330 * @param LayerIndex: Layer foreground or background 00331 * @param Address: New LCD frame buffer value 00332 */ 00333 void BSP_LCD_SetLayerAddress(uint32_t LayerIndex, uint32_t Address) 00334 { 00335 HAL_LTDC_SetAddress(&hltdc_eval, Address, LayerIndex); 00336 } 00337 00338 /** 00339 * @brief Sets display window. 00340 * @param LayerIndex: Layer index 00341 * @param Xpos: LCD X position 00342 * @param Ypos: LCD Y position 00343 * @param Width: LCD window width 00344 * @param Height: LCD window height 00345 */ 00346 void BSP_LCD_SetLayerWindow(uint16_t LayerIndex, uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) 00347 { 00348 /* Reconfigure the layer size */ 00349 HAL_LTDC_SetWindowSize(&hltdc_eval, Width, Height, LayerIndex); 00350 00351 /* Reconfigure the layer position */ 00352 HAL_LTDC_SetWindowPosition(&hltdc_eval, Xpos, Ypos, LayerIndex); 00353 } 00354 00355 /** 00356 * @brief Configures and sets the color keying. 00357 * @param LayerIndex: Layer foreground or background 00358 * @param RGBValue: Color reference 00359 */ 00360 void BSP_LCD_SetColorKeying(uint32_t LayerIndex, uint32_t RGBValue) 00361 { 00362 /* Configure and Enable the color Keying for LCD Layer */ 00363 HAL_LTDC_ConfigColorKeying(&hltdc_eval, RGBValue, LayerIndex); 00364 HAL_LTDC_EnableColorKeying(&hltdc_eval, LayerIndex); 00365 } 00366 00367 /** 00368 * @brief Disables the color keying. 00369 * @param LayerIndex: Layer foreground or background 00370 */ 00371 void BSP_LCD_ResetColorKeying(uint32_t LayerIndex) 00372 { 00373 /* Disable the color Keying for LCD Layer */ 00374 HAL_LTDC_DisableColorKeying(&hltdc_eval, LayerIndex); 00375 } 00376 00377 /** 00378 * @brief Sets the LCD text color. 00379 * @param Color: Text color code ARGB(8-8-8-8) 00380 */ 00381 void BSP_LCD_SetTextColor(uint32_t Color) 00382 { 00383 DrawProp[ActiveLayer].TextColor = Color; 00384 } 00385 00386 /** 00387 * @brief Gets the LCD text color. 00388 * @retval Used text color. 00389 */ 00390 uint32_t BSP_LCD_GetTextColor(void) 00391 { 00392 return DrawProp[ActiveLayer].TextColor; 00393 } 00394 00395 /** 00396 * @brief Sets the LCD background color. 00397 * @param Color: Layer background color code ARGB(8-8-8-8) 00398 */ 00399 void BSP_LCD_SetBackColor(uint32_t Color) 00400 { 00401 DrawProp[ActiveLayer].BackColor = Color; 00402 } 00403 00404 /** 00405 * @brief Gets the LCD background color. 00406 * @retval Used background color 00407 */ 00408 uint32_t BSP_LCD_GetBackColor(void) 00409 { 00410 return DrawProp[ActiveLayer].BackColor; 00411 } 00412 00413 /** 00414 * @brief Sets the LCD text font. 00415 * @param fonts: Layer font to be used 00416 */ 00417 void BSP_LCD_SetFont(sFONT *fonts) 00418 { 00419 DrawProp[ActiveLayer].pFont = fonts; 00420 } 00421 00422 /** 00423 * @brief Gets the LCD text font. 00424 * @retval Used layer font 00425 */ 00426 sFONT *BSP_LCD_GetFont(void) 00427 { 00428 return DrawProp[ActiveLayer].pFont; 00429 } 00430 00431 /** 00432 * @brief Reads an LCD pixel. 00433 * @param Xpos: X position 00434 * @param Ypos: Y position 00435 * @retval RGB pixel color 00436 */ 00437 uint32_t BSP_LCD_ReadPixel(uint16_t Xpos, uint16_t Ypos) 00438 { 00439 uint32_t ret = 0; 00440 00441 if(hltdc_eval.LayerCfg[ActiveLayer].PixelFormat == LTDC_PIXEL_FORMAT_ARGB8888) 00442 { 00443 /* Read data value from SDRAM memory */ 00444 ret = *(__IO uint32_t*) (hltdc_eval.LayerCfg[ActiveLayer].FBStartAdress + (2*(Ypos*BSP_LCD_GetXSize() + Xpos))); 00445 } 00446 else if(hltdc_eval.LayerCfg[ActiveLayer].PixelFormat == LTDC_PIXEL_FORMAT_RGB888) 00447 { 00448 /* Read data value from SDRAM memory */ 00449 ret = (*(__IO uint32_t*) (hltdc_eval.LayerCfg[ActiveLayer].FBStartAdress + (2*(Ypos*BSP_LCD_GetXSize() + Xpos))) & 0x00FFFFFF); 00450 } 00451 else if((hltdc_eval.LayerCfg[ActiveLayer].PixelFormat == LTDC_PIXEL_FORMAT_RGB565) || \ 00452 (hltdc_eval.LayerCfg[ActiveLayer].PixelFormat == LTDC_PIXEL_FORMAT_ARGB4444) || \ 00453 (hltdc_eval.LayerCfg[ActiveLayer].PixelFormat == LTDC_PIXEL_FORMAT_AL88)) 00454 { 00455 /* Read data value from SDRAM memory */ 00456 ret = *(__IO uint16_t*) (hltdc_eval.LayerCfg[ActiveLayer].FBStartAdress + (2*(Ypos*BSP_LCD_GetXSize() + Xpos))); 00457 } 00458 else 00459 { 00460 /* Read data value from SDRAM memory */ 00461 ret = *(__IO uint8_t*) (hltdc_eval.LayerCfg[ActiveLayer].FBStartAdress + (2*(Ypos*BSP_LCD_GetXSize() + Xpos))); 00462 } 00463 00464 return ret; 00465 } 00466 00467 /** 00468 * @brief Clears the hole LCD. 00469 * @param Color: Color of the background 00470 */ 00471 void BSP_LCD_Clear(uint32_t Color) 00472 { 00473 /* Clear the LCD */ 00474 LL_FillBuffer(ActiveLayer, (uint32_t *)(hltdc_eval.LayerCfg[ActiveLayer].FBStartAdress), BSP_LCD_GetXSize(), BSP_LCD_GetYSize(), 0, Color); 00475 } 00476 00477 /** 00478 * @brief Clears the selected line. 00479 * @param Line: Line to be cleared 00480 */ 00481 void BSP_LCD_ClearStringLine(uint32_t Line) 00482 { 00483 uint32_t color_backup = DrawProp[ActiveLayer].TextColor; 00484 DrawProp[ActiveLayer].TextColor = DrawProp[ActiveLayer].BackColor; 00485 00486 /* Draw rectangle with background color */ 00487 BSP_LCD_FillRect(0, (Line * DrawProp[ActiveLayer].pFont->Height), BSP_LCD_GetXSize(), DrawProp[ActiveLayer].pFont->Height); 00488 00489 DrawProp[ActiveLayer].TextColor = color_backup; 00490 BSP_LCD_SetTextColor(DrawProp[ActiveLayer].TextColor); 00491 } 00492 00493 /** 00494 * @brief Displays one character. 00495 * @param Xpos: Start column address 00496 * @param Ypos: Line where to display the character shape. 00497 * @param Ascii: Character ascii code 00498 * This parameter must be a number between Min_Data = 0x20 and Max_Data = 0x7E 00499 */ 00500 void BSP_LCD_DisplayChar(uint16_t Xpos, uint16_t Ypos, uint8_t Ascii) 00501 { 00502 DrawChar(Xpos, Ypos, &DrawProp[ActiveLayer].pFont->table[(Ascii-' ') *\ 00503 DrawProp[ActiveLayer].pFont->Height * ((DrawProp[ActiveLayer].pFont->Width + 7) / 8)]); 00504 } 00505 00506 /** 00507 * @brief Displays characters on the LCD. 00508 * @param Xpos: X position (in pixel) 00509 * @param Ypos: Y position (in pixel) 00510 * @param Text: Pointer to string to display on LCD 00511 * @param Mode: Display mode 00512 * This parameter can be one of the following values: 00513 * @arg CENTER_MODE 00514 * @arg RIGHT_MODE 00515 * @arg LEFT_MODE 00516 */ 00517 void BSP_LCD_DisplayStringAt(uint16_t Xpos, uint16_t Ypos, uint8_t *Text, Text_AlignModeTypdef Mode) 00518 { 00519 uint16_t refcolumn = 1, i = 0; 00520 uint32_t size = 0, xsize = 0; 00521 uint8_t *ptr = Text; 00522 00523 /* Get the text size */ 00524 while (*ptr++) size ++ ; 00525 00526 /* Characters number per line */ 00527 xsize = (BSP_LCD_GetXSize()/DrawProp[ActiveLayer].pFont->Width); 00528 00529 switch (Mode) 00530 { 00531 case CENTER_MODE: 00532 { 00533 refcolumn = Xpos + ((xsize - size)* DrawProp[ActiveLayer].pFont->Width) / 2; 00534 break; 00535 } 00536 case LEFT_MODE: 00537 { 00538 refcolumn = Xpos; 00539 break; 00540 } 00541 case RIGHT_MODE: 00542 { 00543 refcolumn = - Xpos + ((xsize - size)*DrawProp[ActiveLayer].pFont->Width); 00544 break; 00545 } 00546 default: 00547 { 00548 refcolumn = Xpos; 00549 break; 00550 } 00551 } 00552 00553 /* Send the string character by character on LCD */ 00554 while ((*Text != 0) & (((BSP_LCD_GetXSize() - (i*DrawProp[ActiveLayer].pFont->Width)) & 0xFFFF) >= DrawProp[ActiveLayer].pFont->Width)) 00555 { 00556 /* Display one character on LCD */ 00557 BSP_LCD_DisplayChar(refcolumn, Ypos, *Text); 00558 /* Decrement the column position by 16 */ 00559 refcolumn += DrawProp[ActiveLayer].pFont->Width; 00560 /* Point on the next character */ 00561 Text++; 00562 i++; 00563 } 00564 } 00565 00566 /** 00567 * @brief Displays a maximum of 60 characters on the LCD. 00568 * @param Line: Line where to display the character shape 00569 * @param ptr: Pointer to string to display on LCD 00570 */ 00571 void BSP_LCD_DisplayStringAtLine(uint16_t Line, uint8_t *ptr) 00572 { 00573 BSP_LCD_DisplayStringAt(0, LINE(Line), ptr, LEFT_MODE); 00574 } 00575 00576 /** 00577 * @brief Draws an horizontal line. 00578 * @param Xpos: X position 00579 * @param Ypos: Y position 00580 * @param Length: Line length 00581 */ 00582 void BSP_LCD_DrawHLine(uint16_t Xpos, uint16_t Ypos, uint16_t Length) 00583 { 00584 uint32_t Xaddress = 0; 00585 00586 /* Get the line address */ 00587 Xaddress = (hltdc_eval.LayerCfg[ActiveLayer].FBStartAdress) + 4*(BSP_LCD_GetXSize()*Ypos + Xpos); 00588 00589 /* Write line */ 00590 LL_FillBuffer(ActiveLayer, (uint32_t *)Xaddress, Length, 1, 0, DrawProp[ActiveLayer].TextColor); 00591 } 00592 00593 /** 00594 * @brief Draws a vertical line. 00595 * @param Xpos: X position 00596 * @param Ypos: Y position 00597 * @param Length: Line length 00598 */ 00599 void BSP_LCD_DrawVLine(uint16_t Xpos, uint16_t Ypos, uint16_t Length) 00600 { 00601 uint32_t Xaddress = 0; 00602 00603 /* Get the line address */ 00604 Xaddress = (hltdc_eval.LayerCfg[ActiveLayer].FBStartAdress) + 4*(BSP_LCD_GetXSize()*Ypos + Xpos); 00605 00606 /* Write line */ 00607 LL_FillBuffer(ActiveLayer, (uint32_t *)Xaddress, 1, Length, (BSP_LCD_GetXSize() - 1), DrawProp[ActiveLayer].TextColor); 00608 } 00609 00610 /** 00611 * @brief Draws an uni-line (between two points). 00612 * @param x1: Point 1 X position 00613 * @param y1: Point 1 Y position 00614 * @param x2: Point 2 X position 00615 * @param y2: Point 2 Y position 00616 */ 00617 void BSP_LCD_DrawLine(uint16_t x1, uint16_t y1, uint16_t x2, uint16_t y2) 00618 { 00619 int16_t deltax = 0, deltay = 0, x = 0, y = 0, xinc1 = 0, xinc2 = 0, 00620 yinc1 = 0, yinc2 = 0, den = 0, num = 0, numadd = 0, numpixels = 0, 00621 curpixel = 0; 00622 00623 deltax = ABS(x2 - x1); /* The difference between the x's */ 00624 deltay = ABS(y2 - y1); /* The difference between the y's */ 00625 x = x1; /* Start x off at the first pixel */ 00626 y = y1; /* Start y off at the first pixel */ 00627 00628 if (x2 >= x1) /* The x-values are increasing */ 00629 { 00630 xinc1 = 1; 00631 xinc2 = 1; 00632 } 00633 else /* The x-values are decreasing */ 00634 { 00635 xinc1 = -1; 00636 xinc2 = -1; 00637 } 00638 00639 if (y2 >= y1) /* The y-values are increasing */ 00640 { 00641 yinc1 = 1; 00642 yinc2 = 1; 00643 } 00644 else /* The y-values are decreasing */ 00645 { 00646 yinc1 = -1; 00647 yinc2 = -1; 00648 } 00649 00650 if (deltax >= deltay) /* There is at least one x-value for every y-value */ 00651 { 00652 xinc1 = 0; /* Don't change the x when numerator >= denominator */ 00653 yinc2 = 0; /* Don't change the y for every iteration */ 00654 den = deltax; 00655 num = deltax / 2; 00656 numadd = deltay; 00657 numpixels = deltax; /* There are more x-values than y-values */ 00658 } 00659 else /* There is at least one y-value for every x-value */ 00660 { 00661 xinc2 = 0; /* Don't change the x for every iteration */ 00662 yinc1 = 0; /* Don't change the y when numerator >= denominator */ 00663 den = deltay; 00664 num = deltay / 2; 00665 numadd = deltax; 00666 numpixels = deltay; /* There are more y-values than x-values */ 00667 } 00668 00669 for (curpixel = 0; curpixel <= numpixels; curpixel++) 00670 { 00671 BSP_LCD_DrawPixel(x, y, DrawProp[ActiveLayer].TextColor); /* Draw the current pixel */ 00672 num += numadd; /* Increase the numerator by the top of the fraction */ 00673 if (num >= den) /* Check if numerator >= denominator */ 00674 { 00675 num -= den; /* Calculate the new numerator value */ 00676 x += xinc1; /* Change the x as appropriate */ 00677 y += yinc1; /* Change the y as appropriate */ 00678 } 00679 x += xinc2; /* Change the x as appropriate */ 00680 y += yinc2; /* Change the y as appropriate */ 00681 } 00682 } 00683 00684 /** 00685 * @brief Draws a rectangle. 00686 * @param Xpos: X position 00687 * @param Ypos: Y position 00688 * @param Width: Rectangle width 00689 * @param Height: Rectangle height 00690 */ 00691 void BSP_LCD_DrawRect(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) 00692 { 00693 /* Draw horizontal lines */ 00694 BSP_LCD_DrawHLine(Xpos, Ypos, Width); 00695 BSP_LCD_DrawHLine(Xpos, (Ypos+ Height), Width); 00696 00697 /* Draw vertical lines */ 00698 BSP_LCD_DrawVLine(Xpos, Ypos, Height); 00699 BSP_LCD_DrawVLine((Xpos + Width), Ypos, Height); 00700 } 00701 00702 /** 00703 * @brief Draws a circle. 00704 * @param Xpos: X position 00705 * @param Ypos: Y position 00706 * @param Radius: Circle radius 00707 */ 00708 void BSP_LCD_DrawCircle(uint16_t Xpos, uint16_t Ypos, uint16_t Radius) 00709 { 00710 int32_t D; /* Decision Variable */ 00711 uint32_t CurX; /* Current X Value */ 00712 uint32_t CurY; /* Current Y Value */ 00713 00714 D = 3 - (Radius << 1); 00715 CurX = 0; 00716 CurY = Radius; 00717 00718 while (CurX <= CurY) 00719 { 00720 BSP_LCD_DrawPixel((Xpos + CurX), (Ypos - CurY), DrawProp[ActiveLayer].TextColor); 00721 00722 BSP_LCD_DrawPixel((Xpos - CurX), (Ypos - CurY), DrawProp[ActiveLayer].TextColor); 00723 00724 BSP_LCD_DrawPixel((Xpos + CurY), (Ypos - CurX), DrawProp[ActiveLayer].TextColor); 00725 00726 BSP_LCD_DrawPixel((Xpos - CurY), (Ypos - CurX), DrawProp[ActiveLayer].TextColor); 00727 00728 BSP_LCD_DrawPixel((Xpos + CurX), (Ypos + CurY), DrawProp[ActiveLayer].TextColor); 00729 00730 BSP_LCD_DrawPixel((Xpos - CurX), (Ypos + CurY), DrawProp[ActiveLayer].TextColor); 00731 00732 BSP_LCD_DrawPixel((Xpos + CurY), (Ypos + CurX), DrawProp[ActiveLayer].TextColor); 00733 00734 BSP_LCD_DrawPixel((Xpos - CurY), (Ypos + CurX), DrawProp[ActiveLayer].TextColor); 00735 00736 if (D < 0) 00737 { 00738 D += (CurX << 2) + 6; 00739 } 00740 else 00741 { 00742 D += ((CurX - CurY) << 2) + 10; 00743 CurY--; 00744 } 00745 CurX++; 00746 } 00747 } 00748 00749 /** 00750 * @brief Draws an poly-line (between many points). 00751 * @param Points: Pointer to the points array 00752 * @param PointCount: Number of points 00753 */ 00754 void BSP_LCD_DrawPolygon(pPoint Points, uint16_t PointCount) 00755 { 00756 int16_t X = 0, Y = 0; 00757 00758 if(PointCount < 2) 00759 { 00760 return; 00761 } 00762 00763 BSP_LCD_DrawLine(Points->X, Points->Y, (Points+PointCount-1)->X, (Points+PointCount-1)->Y); 00764 00765 while(--PointCount) 00766 { 00767 X = Points->X; 00768 Y = Points->Y; 00769 Points++; 00770 BSP_LCD_DrawLine(X, Y, Points->X, Points->Y); 00771 } 00772 } 00773 00774 /** 00775 * @brief Draws an ellipse on LCD. 00776 * @param Xpos: X position 00777 * @param Ypos: Y position 00778 * @param XRadius: Ellipse X radius 00779 * @param YRadius: Ellipse Y radius 00780 */ 00781 void BSP_LCD_DrawEllipse(int Xpos, int Ypos, int XRadius, int YRadius) 00782 { 00783 int x = 0, y = -YRadius, err = 2-2*XRadius, e2; 00784 float K = 0, rad1 = 0, rad2 = 0; 00785 00786 rad1 = XRadius; 00787 rad2 = YRadius; 00788 00789 K = (float)(rad2/rad1); 00790 00791 do { 00792 BSP_LCD_DrawPixel((Xpos-(uint16_t)(x/K)), (Ypos+y), DrawProp[ActiveLayer].TextColor); 00793 BSP_LCD_DrawPixel((Xpos+(uint16_t)(x/K)), (Ypos+y), DrawProp[ActiveLayer].TextColor); 00794 BSP_LCD_DrawPixel((Xpos+(uint16_t)(x/K)), (Ypos-y), DrawProp[ActiveLayer].TextColor); 00795 BSP_LCD_DrawPixel((Xpos-(uint16_t)(x/K)), (Ypos-y), DrawProp[ActiveLayer].TextColor); 00796 00797 e2 = err; 00798 if (e2 <= x) { 00799 err += ++x*2+1; 00800 if (-y == x && e2 <= y) e2 = 0; 00801 } 00802 if (e2 > y) err += ++y*2+1; 00803 } 00804 while (y <= 0); 00805 } 00806 00807 /** 00808 * @brief Draws a bitmap picture loaded in the internal Flash (32 bpp). 00809 * @param Xpos: Bmp X position in the LCD 00810 * @param Ypos: Bmp Y position in the LCD 00811 * @param pbmp: Pointer to Bmp picture address in the internal Flash 00812 */ 00813 void BSP_LCD_DrawBitmap(uint32_t Xpos, uint32_t Ypos, uint8_t *pbmp) 00814 { 00815 uint32_t index = 0, width = 0, height = 0, bit_pixel = 0; 00816 uint32_t Address; 00817 uint32_t InputColorMode = 0; 00818 00819 /* Get bitmap data address offset */ 00820 index = *(__IO uint16_t *) (pbmp + 10); 00821 index |= (*(__IO uint16_t *) (pbmp + 12)) << 16; 00822 00823 /* Read bitmap width */ 00824 width = *(uint16_t *) (pbmp + 18); 00825 width |= (*(uint16_t *) (pbmp + 20)) << 16; 00826 00827 /* Read bitmap height */ 00828 height = *(uint16_t *) (pbmp + 22); 00829 height |= (*(uint16_t *) (pbmp + 24)) << 16; 00830 00831 /* Read bit/pixel */ 00832 bit_pixel = *(uint16_t *) (pbmp + 28); 00833 00834 /* Set the address */ 00835 Address = hltdc_eval.LayerCfg[ActiveLayer].FBStartAdress + (((BSP_LCD_GetXSize()*Ypos) + Xpos)*(4)); 00836 00837 /* Get the layer pixel format */ 00838 if ((bit_pixel/8) == 4) 00839 { 00840 InputColorMode = CM_ARGB8888; 00841 } 00842 else if ((bit_pixel/8) == 2) 00843 { 00844 InputColorMode = CM_RGB565; 00845 } 00846 else 00847 { 00848 InputColorMode = CM_RGB888; 00849 } 00850 00851 /* Bypass the bitmap header */ 00852 pbmp += (index + (width * (height - 1) * (bit_pixel/8))); 00853 00854 /* Convert picture to ARGB8888 pixel format */ 00855 for(index=0; index < height; index++) 00856 { 00857 /* Pixel format conversion */ 00858 LL_ConvertLineToARGB8888((uint32_t *)pbmp, (uint32_t *)Address, width, InputColorMode); 00859 00860 /* Increment the source and destination buffers */ 00861 Address+= (BSP_LCD_GetXSize()*4); 00862 pbmp -= width*(bit_pixel/8); 00863 } 00864 } 00865 00866 /** 00867 * @brief Draws a full rectangle. 00868 * @param Xpos: X position 00869 * @param Ypos: Y position 00870 * @param Width: Rectangle width 00871 * @param Height: Rectangle height 00872 */ 00873 void BSP_LCD_FillRect(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) 00874 { 00875 uint32_t Xaddress = 0; 00876 00877 /* Set the text color */ 00878 BSP_LCD_SetTextColor(DrawProp[ActiveLayer].TextColor); 00879 00880 /* Get the rectangle start address */ 00881 Xaddress = (hltdc_eval.LayerCfg[ActiveLayer].FBStartAdress) + 4*(BSP_LCD_GetXSize()*Ypos + Xpos); 00882 00883 /* Fill the rectangle */ 00884 LL_FillBuffer(ActiveLayer, (uint32_t *)Xaddress, Width, Height, (BSP_LCD_GetXSize() - Width), DrawProp[ActiveLayer].TextColor); 00885 } 00886 00887 /** 00888 * @brief Draws a full circle. 00889 * @param Xpos: X position 00890 * @param Ypos: Y position 00891 * @param Radius: Circle radius 00892 */ 00893 void BSP_LCD_FillCircle(uint16_t Xpos, uint16_t Ypos, uint16_t Radius) 00894 { 00895 int32_t D; /* Decision Variable */ 00896 uint32_t CurX; /* Current X Value */ 00897 uint32_t CurY; /* Current Y Value */ 00898 00899 D = 3 - (Radius << 1); 00900 00901 CurX = 0; 00902 CurY = Radius; 00903 00904 BSP_LCD_SetTextColor(DrawProp[ActiveLayer].TextColor); 00905 00906 while (CurX <= CurY) 00907 { 00908 if(CurY > 0) 00909 { 00910 BSP_LCD_DrawHLine(Xpos - CurY, Ypos + CurX, 2*CurY); 00911 BSP_LCD_DrawHLine(Xpos - CurY, Ypos - CurX, 2*CurY); 00912 } 00913 00914 if(CurX > 0) 00915 { 00916 BSP_LCD_DrawHLine(Xpos - CurX, Ypos - CurY, 2*CurX); 00917 BSP_LCD_DrawHLine(Xpos - CurX, Ypos + CurY, 2*CurX); 00918 } 00919 if (D < 0) 00920 { 00921 D += (CurX << 2) + 6; 00922 } 00923 else 00924 { 00925 D += ((CurX - CurY) << 2) + 10; 00926 CurY--; 00927 } 00928 CurX++; 00929 } 00930 00931 BSP_LCD_SetTextColor(DrawProp[ActiveLayer].TextColor); 00932 BSP_LCD_DrawCircle(Xpos, Ypos, Radius); 00933 } 00934 00935 /** 00936 * @brief Draws a full poly-line (between many points). 00937 * @param Points: Pointer to the points array 00938 * @param PointCount: Number of points 00939 */ 00940 void BSP_LCD_FillPolygon(pPoint Points, uint16_t PointCount) 00941 { 00942 int16_t X = 0, Y = 0, X2 = 0, Y2 = 0, X_center = 0, Y_center = 0, X_first = 0, Y_first = 0, pixelX = 0, pixelY = 0, counter = 0; 00943 uint16_t IMAGE_LEFT = 0, IMAGE_RIGHT = 0, IMAGE_TOP = 0, IMAGE_BOTTOM = 0; 00944 00945 IMAGE_LEFT = IMAGE_RIGHT = Points->X; 00946 IMAGE_TOP= IMAGE_BOTTOM = Points->Y; 00947 00948 for(counter = 1; counter < PointCount; counter++) 00949 { 00950 pixelX = POLY_X(counter); 00951 if(pixelX < IMAGE_LEFT) 00952 { 00953 IMAGE_LEFT = pixelX; 00954 } 00955 if(pixelX > IMAGE_RIGHT) 00956 { 00957 IMAGE_RIGHT = pixelX; 00958 } 00959 00960 pixelY = POLY_Y(counter); 00961 if(pixelY < IMAGE_TOP) 00962 { 00963 IMAGE_TOP = pixelY; 00964 } 00965 if(pixelY > IMAGE_BOTTOM) 00966 { 00967 IMAGE_BOTTOM = pixelY; 00968 } 00969 } 00970 00971 if(PointCount < 2) 00972 { 00973 return; 00974 } 00975 00976 X_center = (IMAGE_LEFT + IMAGE_RIGHT)/2; 00977 Y_center = (IMAGE_BOTTOM + IMAGE_TOP)/2; 00978 00979 X_first = Points->X; 00980 Y_first = Points->Y; 00981 00982 while(--PointCount) 00983 { 00984 X = Points->X; 00985 Y = Points->Y; 00986 Points++; 00987 X2 = Points->X; 00988 Y2 = Points->Y; 00989 00990 FillTriangle(X, X2, X_center, Y, Y2, Y_center); 00991 FillTriangle(X, X_center, X2, Y, Y_center, Y2); 00992 FillTriangle(X_center, X2, X, Y_center, Y2, Y); 00993 } 00994 00995 FillTriangle(X_first, X2, X_center, Y_first, Y2, Y_center); 00996 FillTriangle(X_first, X_center, X2, Y_first, Y_center, Y2); 00997 FillTriangle(X_center, X2, X_first, Y_center, Y2, Y_first); 00998 } 00999 01000 /** 01001 * @brief Draws a full ellipse. 01002 * @param Xpos: X position 01003 * @param Ypos: Y position 01004 * @param XRadius: Ellipse X radius 01005 * @param YRadius: Ellipse Y radius 01006 */ 01007 void BSP_LCD_FillEllipse(int Xpos, int Ypos, int XRadius, int YRadius) 01008 { 01009 int x = 0, y = -YRadius, err = 2-2*XRadius, e2; 01010 float K = 0, rad1 = 0, rad2 = 0; 01011 01012 rad1 = XRadius; 01013 rad2 = YRadius; 01014 01015 K = (float)(rad2/rad1); 01016 01017 do 01018 { 01019 BSP_LCD_DrawHLine((Xpos-(uint16_t)(x/K)), (Ypos+y), (2*(uint16_t)(x/K) + 1)); 01020 BSP_LCD_DrawHLine((Xpos-(uint16_t)(x/K)), (Ypos-y), (2*(uint16_t)(x/K) + 1)); 01021 01022 e2 = err; 01023 if (e2 <= x) 01024 { 01025 err += ++x*2+1; 01026 if (-y == x && e2 <= y) e2 = 0; 01027 } 01028 if (e2 > y) err += ++y*2+1; 01029 } 01030 while (y <= 0); 01031 } 01032 01033 /** 01034 * @brief Enables the display. 01035 */ 01036 void BSP_LCD_DisplayOn(void) 01037 { 01038 /* Display On */ 01039 __HAL_LTDC_ENABLE(&hltdc_eval); 01040 } 01041 01042 /** 01043 * @brief Disables the display. 01044 */ 01045 void BSP_LCD_DisplayOff(void) 01046 { 01047 /* Display Off */ 01048 __HAL_LTDC_DISABLE(&hltdc_eval); 01049 } 01050 01051 /******************************************************************************* 01052 LTDC and DMA2D BSP Routines 01053 *******************************************************************************/ 01054 01055 /** 01056 * @brief Initializes the LTDC MSP. 01057 */ 01058 static void MspInit(void) 01059 { 01060 GPIO_InitTypeDef GPIO_Init_Structure; 01061 01062 /* Enable the LTDC and DMA2D clocks */ 01063 __LTDC_CLK_ENABLE(); 01064 __DMA2D_CLK_ENABLE(); 01065 01066 /* Enable GPIOs clock */ 01067 __GPIOI_CLK_ENABLE(); 01068 __GPIOJ_CLK_ENABLE(); 01069 __GPIOK_CLK_ENABLE(); 01070 01071 /*** LTDC Pins configuration ***/ 01072 /* GPIOI configuration */ 01073 GPIO_Init_Structure.Pin = GPIO_PIN_12 | GPIO_PIN_13 | GPIO_PIN_14 | GPIO_PIN_15; 01074 GPIO_Init_Structure.Mode = GPIO_MODE_AF_PP; 01075 GPIO_Init_Structure.Pull = GPIO_NOPULL; 01076 GPIO_Init_Structure.Speed = GPIO_SPEED_FAST; 01077 GPIO_Init_Structure.Alternate = GPIO_AF14_LTDC; 01078 HAL_GPIO_Init(GPIOI, &GPIO_Init_Structure); 01079 01080 /* GPIOJ configuration */ 01081 GPIO_Init_Structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_2 | GPIO_PIN_3 | \ 01082 GPIO_PIN_4 | GPIO_PIN_5 | GPIO_PIN_6 | GPIO_PIN_7 | \ 01083 GPIO_PIN_8 | GPIO_PIN_9 | GPIO_PIN_10 | GPIO_PIN_11 | \ 01084 GPIO_PIN_12 | GPIO_PIN_13 | GPIO_PIN_14 | GPIO_PIN_15; 01085 GPIO_Init_Structure.Mode = GPIO_MODE_AF_PP; 01086 GPIO_Init_Structure.Pull = GPIO_NOPULL; 01087 GPIO_Init_Structure.Speed = GPIO_SPEED_FAST; 01088 GPIO_Init_Structure.Alternate = GPIO_AF14_LTDC; 01089 HAL_GPIO_Init(GPIOJ, &GPIO_Init_Structure); 01090 01091 /* GPIOK configuration */ 01092 GPIO_Init_Structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_2 | GPIO_PIN_3 | \ 01093 GPIO_PIN_4 | GPIO_PIN_5 | GPIO_PIN_6 | GPIO_PIN_7; 01094 GPIO_Init_Structure.Mode = GPIO_MODE_AF_PP; 01095 GPIO_Init_Structure.Pull = GPIO_NOPULL; 01096 GPIO_Init_Structure.Speed = GPIO_SPEED_FAST; 01097 GPIO_Init_Structure.Alternate = GPIO_AF14_LTDC; 01098 HAL_GPIO_Init(GPIOK, &GPIO_Init_Structure); 01099 } 01100 01101 /** 01102 * @brief Clock Config. 01103 * @param hltdc: LTDC handle 01104 * @param Params: LTDC pixel clock 01105 * @note This API is called by BSP_LCD_Init() 01106 * Being __weak it can be overwritten by the application 01107 */ 01108 __weak void BSP_LCD_ClockConfig(LTDC_HandleTypeDef *hltdc, void *Params) 01109 { 01110 static RCC_PeriphCLKInitTypeDef periph_clk_init_struct; 01111 01112 if(stmpe811_ts_drv.ReadID(TS_I2C_ADDRESS) == STMPE811_ID) 01113 { 01114 /* AMPIRE480272 LCD clock configuration */ 01115 /* PLLSAI_VCO Input = HSE_VALUE/PLL_M = 1 Mhz */ 01116 /* PLLSAI_VCO Output = PLLSAI_VCO Input * PLLSAIN = 192 Mhz */ 01117 /* PLLLCDCLK = PLLSAI_VCO Output/PLLSAIR = 192/5 = 38.4 Mhz */ 01118 /* LTDC clock frequency = PLLLCDCLK / LTDC_PLLSAI_DIVR_4 = 38.4/4 = 9.6Mhz */ 01119 periph_clk_init_struct.PeriphClockSelection = RCC_PERIPHCLK_LTDC; 01120 periph_clk_init_struct.PLLSAI.PLLSAIN = 192; 01121 periph_clk_init_struct.PLLSAI.PLLSAIR = AMPIRE480272_FREQUENCY_DIVIDER; 01122 periph_clk_init_struct.PLLSAIDivR = RCC_PLLSAIDIVR_4; 01123 HAL_RCCEx_PeriphCLKConfig(&periph_clk_init_struct); 01124 } 01125 else 01126 { 01127 /* The programmed LTDC pixel clock depends on the vertical refresh rate of the panel 60Hz => 25.16MHz and 01128 the LCD/SDRAM bandwidth affected by the several access on the bus and the number of used layers. 01129 */ 01130 if(*(uint32_t *)Params == LCD_MAX_PCLK) 01131 { 01132 /* In case of single layer the bandwidth is arround 160MBytesPerSec ==> theorical PCLK of 40MHz */ 01133 /* AMPIRE640480 typical PCLK is 25.16 MHz so the PLLSAI is configured to provide this clock */ 01134 /* AMPIRE640480 LCD clock configuration */ 01135 /* PLLSAI_VCO Input = HSE_VALUE/PLL_M = 1 Mhz */ 01136 /* PLLSAI_VCO Output = PLLSAI_VCO Input * PLLSAIN = 151 Mhz */ 01137 /* PLLLCDCLK = PLLSAI_VCO Output/PLLSAIR = 151/3 = 50.3 Mhz */ 01138 /* LTDC clock frequency = PLLLCDCLK / LTDC_PLLSAI_DIVR_2 = 50.3/2 = 25.16 Mhz */ 01139 periph_clk_init_struct.PLLSAI.PLLSAIN = 151; 01140 } 01141 else 01142 { 01143 /* In case of double layers the bandwidth is arround 72MBytesPerSec => 18MHz (<25,16MHz) */ 01144 /* so the PLLSAI is configured to provide this clock */ 01145 /* AMPIRE640480 LCD clock configuration */ 01146 /* PLLSAI_VCO Input = HSE_VALUE/PLL_M = 1 Mhz */ 01147 /* PLLSAI_VCO Output = PLLSAI_VCO Input * PLLSAIN = 108 Mhz */ 01148 /* PLLLCDCLK = PLLSAI_VCO Output/PLLSAIR = 108/3 = 36 Mhz */ 01149 /* LTDC clock frequency = PLLLCDCLK / LTDC_PLLSAI_DIVR_2 = 36/2 = 18 Mhz */ 01150 periph_clk_init_struct.PLLSAI.PLLSAIN = 108; 01151 } 01152 periph_clk_init_struct.PeriphClockSelection = RCC_PERIPHCLK_LTDC; 01153 periph_clk_init_struct.PLLSAI.PLLSAIR = 3; 01154 periph_clk_init_struct.PLLSAIDivR = RCC_PLLSAIDIVR_2; 01155 HAL_RCCEx_PeriphCLKConfig(&periph_clk_init_struct); 01156 } 01157 } 01158 /******************************************************************************* 01159 Static Functions 01160 *******************************************************************************/ 01161 01162 /** 01163 * @brief Draws a pixel on LCD. 01164 * @param Xpos: X position 01165 * @param Ypos: Y position 01166 * @param RGB_Code: Pixel color in ARGB mode (8-8-8-8) 01167 */ 01168 void BSP_LCD_DrawPixel(uint16_t Xpos, uint16_t Ypos, uint32_t RGB_Code) 01169 { 01170 /* Write data value to all SDRAM memory */ 01171 *(__IO uint32_t*) (hltdc_eval.LayerCfg[ActiveLayer].FBStartAdress + (4*(Ypos*BSP_LCD_GetXSize() + Xpos))) = RGB_Code; 01172 } 01173 01174 /** 01175 * @brief Draws a character on LCD. 01176 * @param Xpos: Line where to display the character shape 01177 * @param Ypos: Start column address 01178 * @param c: Pointer to the character data 01179 */ 01180 static void DrawChar(uint16_t Xpos, uint16_t Ypos, const uint8_t *c) 01181 { 01182 uint32_t i = 0, j = 0; 01183 uint16_t height, width; 01184 uint8_t offset; 01185 uint8_t *pchar; 01186 uint32_t line; 01187 01188 height = DrawProp[ActiveLayer].pFont->Height; 01189 width = DrawProp[ActiveLayer].pFont->Width; 01190 01191 offset = 8 *((width + 7)/8) - width ; 01192 01193 for(i = 0; i < height; i++) 01194 { 01195 pchar = ((uint8_t *)c + (width + 7)/8 * i); 01196 01197 switch(((width + 7)/8)) 01198 { 01199 01200 case 1: 01201 line = pchar[0]; 01202 break; 01203 01204 case 2: 01205 line = (pchar[0]<< 8) | pchar[1]; 01206 break; 01207 01208 case 3: 01209 default: 01210 line = (pchar[0]<< 16) | (pchar[1]<< 8) | pchar[2]; 01211 break; 01212 } 01213 01214 for (j = 0; j < width; j++) 01215 { 01216 if(line & (1 << (width- j + offset- 1))) 01217 { 01218 BSP_LCD_DrawPixel((Xpos + j), Ypos, DrawProp[ActiveLayer].TextColor); 01219 } 01220 else 01221 { 01222 BSP_LCD_DrawPixel((Xpos + j), Ypos, DrawProp[ActiveLayer].BackColor); 01223 } 01224 } 01225 Ypos++; 01226 } 01227 } 01228 01229 /** 01230 * @brief Fills a triangle (between 3 points). 01231 * @param x1: Point 1 X position 01232 * @param y1: Point 1 Y position 01233 * @param x2: Point 2 X position 01234 * @param y2: Point 2 Y position 01235 * @param x3: Point 3 X position 01236 * @param y3: Point 3 Y position 01237 */ 01238 static void FillTriangle(uint16_t x1, uint16_t x2, uint16_t x3, uint16_t y1, uint16_t y2, uint16_t y3) 01239 { 01240 int16_t deltax = 0, deltay = 0, x = 0, y = 0, xinc1 = 0, xinc2 = 0, 01241 yinc1 = 0, yinc2 = 0, den = 0, num = 0, numadd = 0, numpixels = 0, 01242 curpixel = 0; 01243 01244 deltax = ABS(x2 - x1); /* The difference between the x's */ 01245 deltay = ABS(y2 - y1); /* The difference between the y's */ 01246 x = x1; /* Start x off at the first pixel */ 01247 y = y1; /* Start y off at the first pixel */ 01248 01249 if (x2 >= x1) /* The x-values are increasing */ 01250 { 01251 xinc1 = 1; 01252 xinc2 = 1; 01253 } 01254 else /* The x-values are decreasing */ 01255 { 01256 xinc1 = -1; 01257 xinc2 = -1; 01258 } 01259 01260 if (y2 >= y1) /* The y-values are increasing */ 01261 { 01262 yinc1 = 1; 01263 yinc2 = 1; 01264 } 01265 else /* The y-values are decreasing */ 01266 { 01267 yinc1 = -1; 01268 yinc2 = -1; 01269 } 01270 01271 if (deltax >= deltay) /* There is at least one x-value for every y-value */ 01272 { 01273 xinc1 = 0; /* Don't change the x when numerator >= denominator */ 01274 yinc2 = 0; /* Don't change the y for every iteration */ 01275 den = deltax; 01276 num = deltax / 2; 01277 numadd = deltay; 01278 numpixels = deltax; /* There are more x-values than y-values */ 01279 } 01280 else /* There is at least one y-value for every x-value */ 01281 { 01282 xinc2 = 0; /* Don't change the x for every iteration */ 01283 yinc1 = 0; /* Don't change the y when numerator >= denominator */ 01284 den = deltay; 01285 num = deltay / 2; 01286 numadd = deltax; 01287 numpixels = deltay; /* There are more y-values than x-values */ 01288 } 01289 01290 for (curpixel = 0; curpixel <= numpixels; curpixel++) 01291 { 01292 BSP_LCD_DrawLine(x, y, x3, y3); 01293 01294 num += numadd; /* Increase the numerator by the top of the fraction */ 01295 if (num >= den) /* Check if numerator >= denominator */ 01296 { 01297 num -= den; /* Calculate the new numerator value */ 01298 x += xinc1; /* Change the x as appropriate */ 01299 y += yinc1; /* Change the y as appropriate */ 01300 } 01301 x += xinc2; /* Change the x as appropriate */ 01302 y += yinc2; /* Change the y as appropriate */ 01303 } 01304 } 01305 01306 /** 01307 * @brief Fills a buffer. 01308 * @param LayerIndex: Layer index 01309 * @param pDst: Pointer to destination buffer 01310 * @param xSize: Buffer width 01311 * @param ySize: Buffer height 01312 * @param OffLine: Offset 01313 * @param ColorIndex: Color index 01314 */ 01315 static void LL_FillBuffer(uint32_t LayerIndex, void *pDst, uint32_t xSize, uint32_t ySize, uint32_t OffLine, uint32_t ColorIndex) 01316 { 01317 /* Register to memory mode with ARGB8888 as color Mode */ 01318 hdma2d_eval.Init.Mode = DMA2D_R2M; 01319 hdma2d_eval.Init.ColorMode = DMA2D_ARGB8888; 01320 hdma2d_eval.Init.OutputOffset = OffLine; 01321 01322 hdma2d_eval.Instance = DMA2D; 01323 01324 /* DMA2D Initialization */ 01325 if(HAL_DMA2D_Init(&hdma2d_eval) == HAL_OK) 01326 { 01327 if(HAL_DMA2D_ConfigLayer(&hdma2d_eval, LayerIndex) == HAL_OK) 01328 { 01329 if (HAL_DMA2D_Start(&hdma2d_eval, ColorIndex, (uint32_t)pDst, xSize, ySize) == HAL_OK) 01330 { 01331 /* Polling For DMA transfer */ 01332 HAL_DMA2D_PollForTransfer(&hdma2d_eval, 10); 01333 } 01334 } 01335 } 01336 } 01337 01338 /** 01339 * @brief Converts a line to an ARGB8888 pixel format. 01340 * @param pSrc: Pointer to source buffer 01341 * @param pDst: Output color 01342 * @param xSize: Buffer width 01343 * @param ColorMode: Input color mode 01344 */ 01345 static void LL_ConvertLineToARGB8888(void *pSrc, void *pDst, uint32_t xSize, uint32_t ColorMode) 01346 { 01347 /* Configure the DMA2D Mode, Color Mode and output offset */ 01348 hdma2d_eval.Init.Mode = DMA2D_M2M_PFC; 01349 hdma2d_eval.Init.ColorMode = DMA2D_ARGB8888; 01350 hdma2d_eval.Init.OutputOffset = 0; 01351 01352 /* Foreground Configuration */ 01353 hdma2d_eval.LayerCfg[1].AlphaMode = DMA2D_NO_MODIF_ALPHA; 01354 hdma2d_eval.LayerCfg[1].InputAlpha = 0xFF; 01355 hdma2d_eval.LayerCfg[1].InputColorMode = ColorMode; 01356 hdma2d_eval.LayerCfg[1].InputOffset = 0; 01357 01358 hdma2d_eval.Instance = DMA2D; 01359 01360 /* DMA2D Initialization */ 01361 if(HAL_DMA2D_Init(&hdma2d_eval) == HAL_OK) 01362 { 01363 if(HAL_DMA2D_ConfigLayer(&hdma2d_eval, 1) == HAL_OK) 01364 { 01365 if (HAL_DMA2D_Start(&hdma2d_eval, (uint32_t)pSrc, (uint32_t)pDst, xSize, 1) == HAL_OK) 01366 { 01367 /* Polling For DMA transfer */ 01368 HAL_DMA2D_PollForTransfer(&hdma2d_eval, 10); 01369 } 01370 } 01371 } 01372 } 01373 01374 /** 01375 * @} 01376 */ 01377 01378 /** 01379 * @} 01380 */ 01381 01382 /** 01383 * @} 01384 */ 01385 01386 /** 01387 * @} 01388 */ 01389 01390 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Wed Jan 13 2016 15:52:54 for STM324x9I_EVAL BSP User Manual by
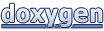