STM32446E_EVAL BSP User Manual
|
stm32446e_eval.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32446e_eval.c 00004 * @author MCD Application Team 00005 * @version V1.1.1 00006 * @date 13-January-2016 00007 * @brief This file provides a set of firmware functions to manage LEDs, 00008 * push-buttons and COM ports available on STM32446E-EVAL evaluation 00009 * board(MB1045) RevB from STMicroelectronics. 00010 ****************************************************************************** 00011 * @attention 00012 * 00013 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00014 * 00015 * Redistribution and use in source and binary forms, with or without modification, 00016 * are permitted provided that the following conditions are met: 00017 * 1. Redistributions of source code must retain the above copyright notice, 00018 * this list of conditions and the following disclaimer. 00019 * 2. Redistributions in binary form must reproduce the above copyright notice, 00020 * this list of conditions and the following disclaimer in the documentation 00021 * and/or other materials provided with the distribution. 00022 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00023 * may be used to endorse or promote products derived from this software 00024 * without specific prior written permission. 00025 * 00026 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00027 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00028 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00029 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00030 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00031 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00032 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00033 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00034 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00035 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00036 * 00037 ****************************************************************************** 00038 */ 00039 00040 /* File Info: ------------------------------------------------------------------ 00041 User NOTE 00042 00043 This driver requires the stm32446e_eval_io to manage the joystick 00044 00045 ------------------------------------------------------------------------------*/ 00046 00047 /* Includes ------------------------------------------------------------------*/ 00048 #include "stm32446e_eval.h" 00049 #if defined(USE_IOEXPANDER) 00050 #include "stm32446e_eval_io.h" 00051 #endif /* USE_IOEXPANDER */ 00052 00053 00054 /** @defgroup BSP BSP 00055 * @{ 00056 */ 00057 00058 /** @defgroup STM32446E_EVAL STM32446E EVAL 00059 * @{ 00060 */ 00061 00062 /** @defgroup STM32446E_EVAL_LOW_LEVEL STM32446E EVAL LOW LEVEL 00063 * @{ 00064 */ 00065 00066 /** @defgroup STM32446E_EVAL_LOW_LEVEL_Private_TypesDefinitions STM32446E EVAL LOW LEVEL Private TypesDefinitions 00067 * @{ 00068 */ 00069 typedef struct 00070 { 00071 __IO uint16_t REG; 00072 __IO uint16_t RAM; 00073 }LCD_CONTROLLER_TypeDef; 00074 /** 00075 * @} 00076 */ 00077 00078 /** @defgroup STM32446E_EVAL_LOW_LEVEL_Private_Defines STM32446E EVAL LOW LEVEL Private Defines 00079 * @{ 00080 */ 00081 /** 00082 * @brief STM32446E EVAL BSP Driver version number V1.1.1 00083 */ 00084 #define __STM32446E_EVAL_BSP_VERSION_MAIN (0x01) /*!< [31:24] main version */ 00085 #define __STM32446E_EVAL_BSP_VERSION_SUB1 (0x01) /*!< [23:16] sub1 version */ 00086 #define __STM32446E_EVAL_BSP_VERSION_SUB2 (0x01) /*!< [15:8] sub2 version */ 00087 #define __STM32446E_EVAL_BSP_VERSION_RC (0x00) /*!< [7:0] release candidate */ 00088 #define __STM32446E_EVAL_BSP_VERSION ((__STM32446E_EVAL_BSP_VERSION_MAIN << 24)\ 00089 |(__STM32446E_EVAL_BSP_VERSION_SUB1 << 16)\ 00090 |(__STM32446E_EVAL_BSP_VERSION_SUB2 << 8 )\ 00091 |(__STM32446E_EVAL_BSP_VERSION_RC)) 00092 00093 /* compared to F4xG we use BANK1 rather then BANK3 since we use FMC_NE1 signal (not FMC_NE3) */ 00094 #define FMC_BANK1_BASE ((uint32_t)(0x60000000 | 0x00000000)) 00095 #define FMC_BANK3_BASE ((uint32_t)(0x60000000 | 0x08000000)) 00096 #define FMC_BANK1 ((LCD_CONTROLLER_TypeDef *) FMC_BANK1_BASE) 00097 00098 /** 00099 * @} 00100 */ 00101 00102 /** @defgroup STM32446E_EVAL_LOW_LEVEL_Private_Macros STM32446E EVAL LOW LEVEL Private Macros 00103 * @{ 00104 */ 00105 /** 00106 * @} 00107 */ 00108 00109 /** @defgroup STM32446E_EVAL_LOW_LEVEL_Private_Variables STM32446E EVAL LOW LEVEL Private Variables 00110 * @{ 00111 */ 00112 00113 #if defined(USE_IOEXPANDER) 00114 const uint32_t GPIO_PIN[LEDn] = {LED1_PIN, 00115 LED2_PIN, 00116 LED3_PIN, 00117 LED4_PIN}; 00118 #else 00119 const uint32_t GPIO_PIN[LEDn] = {LED1_PIN, 00120 LED3_PIN}; 00121 #endif /* USE_IOEXPANDER */ 00122 00123 00124 GPIO_TypeDef* BUTTON_PORT[BUTTONn] = {WAKEUP_BUTTON_GPIO_PORT, 00125 TAMPER_BUTTON_GPIO_PORT, 00126 KEY_BUTTON_GPIO_PORT}; 00127 00128 const uint16_t BUTTON_PIN[BUTTONn] = {WAKEUP_BUTTON_PIN, 00129 TAMPER_BUTTON_PIN, 00130 KEY_BUTTON_PIN}; 00131 00132 const uint16_t BUTTON_IRQn[BUTTONn] = {WAKEUP_BUTTON_EXTI_IRQn, 00133 TAMPER_BUTTON_EXTI_IRQn, 00134 KEY_BUTTON_EXTI_IRQn}; 00135 00136 USART_TypeDef* COM_USART[COMn] = {EVAL_COM1}; 00137 00138 GPIO_TypeDef* COM_TX_PORT[COMn] = {EVAL_COM1_TX_GPIO_PORT}; 00139 00140 GPIO_TypeDef* COM_RX_PORT[COMn] = {EVAL_COM1_RX_GPIO_PORT}; 00141 00142 const uint16_t COM_TX_PIN[COMn] = {EVAL_COM1_TX_PIN}; 00143 00144 const uint16_t COM_RX_PIN[COMn] = {EVAL_COM1_RX_PIN}; 00145 00146 const uint16_t COM_TX_AF[COMn] = {EVAL_COM1_TX_AF}; 00147 00148 const uint16_t COM_RX_AF[COMn] = {EVAL_COM1_RX_AF}; 00149 00150 static FMPI2C_HandleTypeDef hEvalI2c; 00151 00152 /** 00153 * @} 00154 */ 00155 00156 /** @defgroup STM32446E_EVAL_LOW_LEVEL_Private_FunctionPrototypes STM32446E EVAL LOW LEVEL Private FunctionPrototypes 00157 * @{ 00158 */ 00159 static void I2Cx_MspInit(void); 00160 static void I2Cx_Init(void); 00161 #if defined(USE_IOEXPANDER) 00162 static void I2Cx_Write(uint8_t Addr, uint8_t Reg, uint8_t Value); 00163 static uint8_t I2Cx_Read(uint8_t Addr, uint8_t Reg); 00164 #endif /* USE_IOEXPANDER */ 00165 00166 static HAL_StatusTypeDef I2Cx_ReadMultiple(uint8_t Addr, uint16_t Reg, uint16_t MemAddSize, uint8_t *Buffer, uint16_t Length); 00167 static HAL_StatusTypeDef I2Cx_WriteMultiple(uint8_t Addr, uint16_t Reg, uint16_t MemAddSize, uint8_t *Buffer, uint16_t Length); 00168 static HAL_StatusTypeDef I2Cx_IsDeviceReady(uint16_t DevAddress, uint32_t Trials); 00169 static void I2Cx_Error(uint8_t Addr); 00170 00171 static void FMC_BANK1_WriteData(uint16_t Data); 00172 static void FMC_BANK1_WriteReg(uint8_t Reg); 00173 static uint16_t FMC_BANK1_ReadData(void); 00174 static void FMC_BANK1_Init(void); 00175 static void FMC_BANK1_MspInit(void); 00176 00177 /* IOExpander IO functions */ 00178 #if defined(USE_IOEXPANDER) 00179 void MFX_IO_Init(void); 00180 void MFX_IO_DeInit(void); 00181 void MFX_IO_ITConfig(void); 00182 void MFX_IO_Delay(uint32_t Delay); 00183 void MFX_IO_Write(uint16_t Addr, uint8_t Reg, uint8_t Value); 00184 uint8_t MFX_IO_Read(uint16_t Addr, uint8_t Reg); 00185 uint16_t MFX_IO_ReadMultiple(uint16_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length); 00186 void MFX_IO_Wakeup(void); 00187 void MFX_IO_EnableWakeupPin(void); 00188 #endif /* USE_IOEXPANDER */ 00189 00190 /* LCD IO functions */ 00191 void LCD_IO_Init(void); 00192 void LCD_IO_WriteData(uint16_t RegValue); 00193 void LCD_IO_WriteReg(uint8_t Reg); 00194 uint16_t LCD_IO_ReadData(void); 00195 00196 /* AUDIO IO functions */ 00197 void AUDIO_IO_Init(void); 00198 void AUDIO_IO_DeInit(void); 00199 void AUDIO_IO_Write(uint8_t Addr, uint16_t Reg, uint16_t Value); 00200 uint16_t AUDIO_IO_Read(uint8_t Addr, uint16_t Reg); 00201 void AUDIO_IO_Delay(uint32_t Delay); 00202 00203 /* CAMERA IO functions */ 00204 void CAMERA_IO_Init(void); 00205 void CAMERA_Delay(uint32_t Delay); 00206 void CAMERA_IO_Write(uint8_t Addr, uint16_t Reg, uint16_t Value); 00207 uint16_t CAMERA_IO_Read(uint8_t Addr, uint16_t Reg); 00208 00209 /* I2C EEPROM IO function */ 00210 void EEPROM_IO_Init(void); 00211 HAL_StatusTypeDef EEPROM_IO_WriteData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize); 00212 HAL_StatusTypeDef EEPROM_IO_ReadData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize); 00213 HAL_StatusTypeDef EEPROM_IO_IsDeviceReady(uint16_t DevAddress, uint32_t Trials); 00214 00215 00216 /** 00217 * @} 00218 */ 00219 00220 00221 00222 /** @defgroup STM32446E_EVAL_LOW_LEVEL_Private_Functions STM32446E EVAL LOW LEVEL Private Functions 00223 * @{ 00224 */ 00225 00226 /** 00227 * @brief This method returns the STM32446E EVAL BSP Driver revision 00228 * @retval version: 0xXYZR (8bits for each decimal, R for RC) 00229 */ 00230 uint32_t BSP_GetVersion(void) 00231 { 00232 return __STM32446E_EVAL_BSP_VERSION; 00233 } 00234 00235 /** 00236 * @brief Configures LEDs. 00237 * @param Led: LED to be configured. 00238 * This parameter can be one of the following values: 00239 * @arg LED1 00240 * @arg LED2 00241 * @arg LED3 00242 * @arg LED4 00243 */ 00244 void BSP_LED_Init(Led_TypeDef Led) 00245 { 00246 #if !defined(USE_STM32446E_EVAL_REVA) 00247 GPIO_InitTypeDef gpio_init_structure; 00248 00249 /* On RevB led1 and Led3 are on GPIO while Led2 and Led4 on Mfx*/ 00250 if ((Led == LED1) || (Led == LED3)) 00251 { 00252 /* Enable the GPIO_LED clock */ 00253 LEDx_GPIO_CLK_ENABLE(); 00254 00255 /* Configure the GPIO_LED pin */ 00256 gpio_init_structure.Pin = GPIO_PIN[Led]; 00257 gpio_init_structure.Mode = GPIO_MODE_OUTPUT_PP; 00258 gpio_init_structure.Pull = GPIO_PULLUP; 00259 gpio_init_structure.Speed = GPIO_SPEED_HIGH; 00260 00261 HAL_GPIO_Init(LEDx_GPIO_PORT, &gpio_init_structure); 00262 00263 /* By default, turn off LED */ 00264 HAL_GPIO_WritePin(LEDx_GPIO_PORT, GPIO_PIN[Led], GPIO_PIN_SET); 00265 } 00266 else /* Led2 and Led4 */ 00267 { 00268 #endif /* !USE_STM32446E_EVAL_REVA */ 00269 00270 #if defined(USE_IOEXPANDER) /* (USE_IOEXPANDER always defined for RevA) */ 00271 /* Initialize the IO functionalities (Mfx) */ 00272 BSP_IO_Init(); 00273 /* GPIO_PIN[Led] depends on the board revision: */ 00274 /* - in case of RevA all leds are on IOEXPANDER (Mfx) */ 00275 /* - in case of RevB just led 2 and led4 on IOEXPANDER (Mfx) */ 00276 BSP_IO_ConfigPin(GPIO_PIN[Led], IO_MODE_OUTPUT_PP_PU); 00277 BSP_IO_WritePin(GPIO_PIN[Led], BSP_IO_PIN_SET); 00278 #endif /* USE_IOEXPANDER */ 00279 00280 #if !defined(USE_STM32446E_EVAL_REVA) 00281 } 00282 #endif /* !USE_STM32446E_EVAL_REVA */ 00283 } 00284 00285 00286 /** 00287 * @brief DeInit LEDs. 00288 * @param Led: LED to be configured. 00289 * This parameter can be one of the following values: 00290 * @arg LED1 00291 * @arg LED2 00292 * @arg LED3 00293 * @arg LED4 00294 * @note Led DeInit does not disable the GPIO clock nor disable the Mfx 00295 */ 00296 void BSP_LED_DeInit(Led_TypeDef Led) 00297 { 00298 #if !defined(USE_STM32446E_EVAL_REVA) 00299 GPIO_InitTypeDef gpio_init_structure; 00300 00301 /* On RevB led1 and Led3 are on GPIO while Led2 and Led4 on Mfx*/ 00302 if ((Led == LED1) || (Led == LED3)) 00303 { 00304 /* Turn off LED */ 00305 HAL_GPIO_WritePin(LEDx_GPIO_PORT, GPIO_PIN[Led], GPIO_PIN_RESET); 00306 /* DeInit the GPIO_LED pin */ 00307 gpio_init_structure.Pin = GPIO_PIN[Led]; 00308 HAL_GPIO_DeInit(LEDx_GPIO_PORT, gpio_init_structure.Pin); 00309 } 00310 else 00311 { 00312 #endif /* !USE_STM32446E_EVAL_REVA */ 00313 00314 #if defined(USE_IOEXPANDER) /* (USE_IOEXPANDER always defined for RevA) */ 00315 /* GPIO_PIN[Led] depends on the board revision: */ 00316 /* - in case of RevA all leds are on IOEXPANDER (Mfx) */ 00317 /* - in case of RevB just led 2 and led4 on IOEXPANDER (Mfx) */ 00318 BSP_IO_ConfigPin(GPIO_PIN[Led], IO_MODE_OFF); 00319 #endif /* USE_IOEXPANDER */ 00320 00321 #if !defined(USE_STM32446E_EVAL_REVA) 00322 } 00323 #endif /* !USE_STM32446E_EVAL_REVA */ 00324 } 00325 00326 /** 00327 * @brief Turns selected LED On. 00328 * @param Led: LED to be set on 00329 * This parameter can be one of the following values: 00330 * @arg LED1 00331 * @arg LED2 00332 * @arg LED3 00333 * @arg LED4 00334 */ 00335 void BSP_LED_On(Led_TypeDef Led) 00336 { 00337 00338 #if !defined(USE_STM32446E_EVAL_REVA) 00339 /* On RevB led1 and Led3 are on GPIO while Led2 and Led4 on Mfx*/ 00340 if ((Led == LED1) || (Led == LED3)) 00341 { 00342 HAL_GPIO_WritePin(LEDx_GPIO_PORT, GPIO_PIN[Led], GPIO_PIN_RESET); 00343 } 00344 else 00345 { 00346 #endif /* !USE_STM32446E_EVAL_REVA */ 00347 00348 #if defined(USE_IOEXPANDER) /* (USE_IOEXPANDER always defined for RevA) */ 00349 /* GPIO_PIN[Led] depends on the board revision: */ 00350 /* - in case of RevA all leds are on IOEXPANDER (Mfx) */ 00351 /* - in case of RevB just led 2 and led4 on IOEXPANDER (Mfx) */ 00352 BSP_IO_WritePin(GPIO_PIN[Led], BSP_IO_PIN_RESET); 00353 #endif /* USE_IOEXPANDER */ 00354 00355 #if !defined(USE_STM32446E_EVAL_REVA) 00356 } 00357 #endif /* !USE_STM32446E_EVAL_REVA */ 00358 } 00359 00360 /** 00361 * @brief Turns selected LED Off. 00362 * @param Led: LED to be set off 00363 * This parameter can be one of the following values: 00364 * @arg LED1 00365 * @arg LED2 00366 * @arg LED3 00367 * @arg LED4 00368 */ 00369 void BSP_LED_Off(Led_TypeDef Led) 00370 { 00371 00372 #if !defined(USE_STM32446E_EVAL_REVA) 00373 /* On RevB led1 and Led3 are on GPIO while Led2 and Led4 on Mfx*/ 00374 if ((Led == LED1) || (Led == LED3)) 00375 { 00376 HAL_GPIO_WritePin(LEDx_GPIO_PORT, GPIO_PIN[Led], GPIO_PIN_SET); 00377 } 00378 else 00379 { 00380 #endif /* !USE_STM32446E_EVAL_REVA */ 00381 00382 #if defined(USE_IOEXPANDER) /* (USE_IOEXPANDER always defined for RevA) */ 00383 /* GPIO_PIN[Led] depends on the board revision: */ 00384 /* - in case of RevA all leds are on IOEXPANDER (Mfx) */ 00385 /* - in case of RevB just led 2 and led4 on IOEXPANDER (Mfx) */ 00386 BSP_IO_WritePin(GPIO_PIN[Led], BSP_IO_PIN_SET); 00387 #endif /* USE_IOEXPANDER */ 00388 00389 #if !defined(USE_STM32446E_EVAL_REVA) 00390 } 00391 #endif /* !USE_STM32446E_EVAL_REVA */ 00392 00393 } 00394 00395 /** 00396 * @brief Toggles the selected LED. 00397 * @param Led: LED to be toggled 00398 * This parameter can be one of the following values: 00399 * @arg LED1 00400 * @arg LED2 00401 * @arg LED3 00402 * @arg LED4 00403 */ 00404 void BSP_LED_Toggle(Led_TypeDef Led) 00405 { 00406 00407 #if !defined(USE_STM32446E_EVAL_REVA) 00408 /* On RevB led1 and Led3 are on GPIO while Led2 and Led4 on Mfx*/ 00409 if ((Led == LED1) || (Led == LED3)) 00410 { 00411 HAL_GPIO_TogglePin(LEDx_GPIO_PORT, GPIO_PIN[Led]); 00412 } 00413 else 00414 { 00415 #endif /* !USE_STM32446E_EVAL_REVA */ 00416 00417 #if defined(USE_IOEXPANDER) /* (USE_IOEXPANDER always defined for RevA) */ 00418 /* GPIO_PIN[Led] depends on the board revision: */ 00419 /* - in case of RevA all leds are on IOEXPANDER (Mfx) */ 00420 /* - in case of RevB just led 2 and led4 on IOEXPANDER (Mfx) */ 00421 BSP_IO_TogglePin(GPIO_PIN[Led]); 00422 #endif /* USE_IOEXPANDER */ 00423 00424 #if !defined(USE_STM32446E_EVAL_REVA) 00425 } 00426 #endif /* !USE_STM32446E_EVAL_REVA */ 00427 } 00428 00429 /** 00430 * @brief Configures button GPIO and EXTI Line. 00431 * @param Button: Button to be configured 00432 * This parameter can be one of the following values: 00433 * @arg BUTTON_WAKEUP: Wakeup Push Button 00434 * @arg BUTTON_TAMPER: Tamper Push Button 00435 * @arg BUTTON_KEY: Key Push Button 00436 * @param ButtonMode: Button mode 00437 * This parameter can be one of the following values: 00438 * @arg BUTTON_MODE_GPIO: Button will be used as simple IO 00439 * @arg BUTTON_MODE_EXTI: Button will be connected to EXTI line 00440 * with interrupt generation capability 00441 * @note On STM32446E-EVAL evaluation board, the three buttons (Wakeup, Tamper 00442 * and key buttons) are mapped on the same push button named "Wakeup/Tamper" 00443 * on the board serigraphy. 00444 */ 00445 void BSP_PB_Init(Button_TypeDef Button, ButtonMode_TypeDef ButtonMode) 00446 { 00447 GPIO_InitTypeDef gpio_init_structure; 00448 00449 /* Enable the BUTTON clock */ 00450 BUTTONx_GPIO_CLK_ENABLE(Button); 00451 00452 if(ButtonMode == BUTTON_MODE_GPIO) 00453 { 00454 /* Configure Button pin as input */ 00455 gpio_init_structure.Pin = BUTTON_PIN[Button]; 00456 gpio_init_structure.Mode = GPIO_MODE_INPUT; 00457 gpio_init_structure.Pull = GPIO_NOPULL; 00458 gpio_init_structure.Speed = GPIO_SPEED_FAST; 00459 HAL_GPIO_Init(BUTTON_PORT[Button], &gpio_init_structure); 00460 } 00461 00462 if(ButtonMode == BUTTON_MODE_EXTI) 00463 { 00464 /* Configure Button pin as input with External interrupt */ 00465 gpio_init_structure.Pin = BUTTON_PIN[Button]; 00466 gpio_init_structure.Pull = GPIO_NOPULL; 00467 gpio_init_structure.Speed = GPIO_SPEED_FAST; 00468 00469 if(Button != BUTTON_WAKEUP) 00470 { 00471 gpio_init_structure.Mode = GPIO_MODE_IT_FALLING; 00472 } 00473 else 00474 { 00475 gpio_init_structure.Mode = GPIO_MODE_IT_RISING; 00476 } 00477 00478 HAL_GPIO_Init(BUTTON_PORT[Button], &gpio_init_structure); 00479 00480 /* Enable and set Button EXTI Interrupt to the lowest priority */ 00481 HAL_NVIC_SetPriority((IRQn_Type)(BUTTON_IRQn[Button]), 0x0F, 0x00); 00482 HAL_NVIC_EnableIRQ((IRQn_Type)(BUTTON_IRQn[Button])); 00483 } 00484 } 00485 00486 /** 00487 * @brief Push Button DeInit. 00488 * @param Button: Button to be configured 00489 * This parameter can be one of the following values: 00490 * @arg BUTTON_WAKEUP: Wakeup Push Button 00491 * @arg BUTTON_TAMPER: Tamper Push Button 00492 * @arg BUTTON_KEY: Key Push Button 00493 * @note On STM32446E-EVAL evaluation board, the three buttons (Wakeup, Tamper 00494 * and key buttons) are mapped on the same push button nammed "Wakeup/Tamper" 00495 * on the board serigraphy. 00496 * @note PB DeInit does not disable the GPIO clock 00497 */ 00498 void BSP_PB_DeInit(Button_TypeDef Button) 00499 { 00500 GPIO_InitTypeDef gpio_init_structure; 00501 00502 gpio_init_structure.Pin = BUTTON_PIN[Button]; 00503 HAL_NVIC_DisableIRQ((IRQn_Type)(BUTTON_IRQn[Button])); 00504 HAL_GPIO_DeInit(BUTTON_PORT[Button], gpio_init_structure.Pin); 00505 } 00506 00507 00508 /** 00509 * @brief Returns the selected button state. 00510 * @param Button: Button to be checked 00511 * This parameter can be one of the following values: 00512 * @arg BUTTON_WAKEUP: Wakeup Push Button 00513 * @arg BUTTON_TAMPER: Tamper Push Button 00514 * @arg BUTTON_KEY: Key Push Button 00515 * @note On STM32446E-EVAL evaluation board, the three buttons (Wakeup, Tamper 00516 * and key buttons) are mapped on the same push button nammed "Wakeup/Tamper" 00517 * on the board serigraphy. 00518 * @retval The Button GPIO pin value 00519 */ 00520 uint32_t BSP_PB_GetState(Button_TypeDef Button) 00521 { 00522 return HAL_GPIO_ReadPin(BUTTON_PORT[Button], BUTTON_PIN[Button]); 00523 } 00524 00525 /** 00526 * @brief Configures COM port. 00527 * @param COM: COM port to be configured. 00528 * This parameter can be one of the following values: 00529 * @arg COM1 00530 * @arg COM2 00531 * @param huart: Pointer to a UART_HandleTypeDef structure that contains the 00532 * configuration information for the specified USART peripheral. 00533 */ 00534 void BSP_COM_Init(COM_TypeDef COM, UART_HandleTypeDef *huart) 00535 { 00536 GPIO_InitTypeDef gpio_init_structure; 00537 00538 /* Enable GPIO clock */ 00539 EVAL_COMx_TX_GPIO_CLK_ENABLE(COM); 00540 EVAL_COMx_RX_GPIO_CLK_ENABLE(COM); 00541 00542 /* Enable USART clock */ 00543 EVAL_COMx_CLK_ENABLE(COM); 00544 00545 /* Configure USART Tx as alternate function */ 00546 gpio_init_structure.Pin = COM_TX_PIN[COM]; 00547 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00548 gpio_init_structure.Speed = GPIO_SPEED_FAST; 00549 gpio_init_structure.Pull = GPIO_PULLUP; 00550 gpio_init_structure.Alternate = COM_TX_AF[COM]; 00551 HAL_GPIO_Init(COM_TX_PORT[COM], &gpio_init_structure); 00552 00553 /* Configure USART Rx as alternate function */ 00554 gpio_init_structure.Pin = COM_RX_PIN[COM]; 00555 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00556 gpio_init_structure.Alternate = COM_RX_AF[COM]; 00557 HAL_GPIO_Init(COM_RX_PORT[COM], &gpio_init_structure); 00558 00559 /* USART configuration */ 00560 huart->Instance = COM_USART[COM]; 00561 HAL_UART_Init(huart); 00562 } 00563 00564 /** 00565 * @brief DeInit COM port. 00566 * @param COM: COM port to be configured. 00567 * This parameter can be one of the following values: 00568 * @arg COM1 00569 * @arg COM2 00570 * @param huart: Pointer to a UART_HandleTypeDef structure that contains the 00571 * configuration information for the specified USART peripheral. 00572 */ 00573 void BSP_COM_DeInit(COM_TypeDef COM, UART_HandleTypeDef *huart) 00574 { 00575 /* USART configuration */ 00576 huart->Instance = COM_USART[COM]; 00577 HAL_UART_DeInit(huart); 00578 00579 /* Enable USART clock */ 00580 EVAL_COMx_CLK_DISABLE(COM); 00581 00582 /* DeInit GPIO pins can be done in the application 00583 (by surcharging this __weak function) */ 00584 00585 /* GPIO pins clock, FMC clock and DMA clock can be shut down in the application 00586 by surcharging this __weak function */ 00587 } 00588 00589 #if defined(USE_IOEXPANDER) 00590 00591 /** 00592 * @brief Configures joystick GPIO and EXTI modes. 00593 * @param JoyMode: Button mode. 00594 * This parameter can be one of the following values: 00595 * @arg JOY_MODE_GPIO: Joystick pins will be used as simple IOs 00596 * @arg JOY_MODE_EXTI: Joystick pins will be connected to EXTI line 00597 * with interrupt generation capability 00598 * @retval IO_OK: if all initializations are OK. Other value if error. 00599 */ 00600 uint8_t BSP_JOY_Init(JOYMode_TypeDef JoyMode) 00601 { 00602 uint8_t ret = 0; 00603 00604 /* Initialize the IO functionalities */ 00605 ret = BSP_IO_Init(); 00606 00607 /* Configure joystick pins in IT mode */ 00608 if(JoyMode == JOY_MODE_EXTI) 00609 { 00610 /* Configure IO interrupt acquisition mode */ 00611 BSP_IO_ConfigPin(JOY_ALL_PINS, IO_MODE_IT_FALLING_EDGE_PU); 00612 } 00613 else 00614 { 00615 BSP_IO_ConfigPin(JOY_ALL_PINS, IO_MODE_INPUT_PU); 00616 } 00617 00618 return ret; 00619 } 00620 00621 00622 /** 00623 * @brief DeInit joystick GPIOs. 00624 * @note JOY DeInit does not disable the Mfx, just set the Mfx pins in Off mode 00625 */ 00626 void BSP_JOY_DeInit(void) 00627 { 00628 BSP_IO_ConfigPin(JOY_ALL_PINS, IO_MODE_OFF); 00629 } 00630 00631 /** 00632 * @brief Returns the current joystick status. 00633 * @retval Code of the joystick key pressed 00634 * This code can be one of the following values: 00635 * @arg JOY_NONE 00636 * @arg JOY_SEL 00637 * @arg JOY_DOWN 00638 * @arg JOY_LEFT 00639 * @arg JOY_RIGHT 00640 * @arg JOY_UP 00641 */ 00642 JOYState_TypeDef BSP_JOY_GetState(void) 00643 { 00644 uint16_t pin_status = 0; 00645 00646 /* Read the status joystick pins */ 00647 pin_status = BSP_IO_ReadPin(JOY_ALL_PINS); 00648 00649 /* Check the pressed keys */ 00650 if((pin_status & JOY_NONE_PIN) == JOY_NONE) 00651 { 00652 return(JOYState_TypeDef) JOY_NONE; 00653 } 00654 else if(!(pin_status & JOY_SEL_PIN)) 00655 { 00656 return(JOYState_TypeDef) JOY_SEL; 00657 } 00658 else if(!(pin_status & JOY_DOWN_PIN)) 00659 { 00660 return(JOYState_TypeDef) JOY_DOWN; 00661 } 00662 else if(!(pin_status & JOY_LEFT_PIN)) 00663 { 00664 return(JOYState_TypeDef) JOY_LEFT; 00665 } 00666 else if(!(pin_status & JOY_RIGHT_PIN)) 00667 { 00668 return(JOYState_TypeDef) JOY_RIGHT; 00669 } 00670 else if(!(pin_status & JOY_UP_PIN)) 00671 { 00672 return(JOYState_TypeDef) JOY_UP; 00673 } 00674 else 00675 { 00676 return(JOYState_TypeDef) JOY_NONE; 00677 } 00678 } 00679 00680 #endif /* USE_IOEXPANDER */ 00681 00682 00683 /******************************************************************************* 00684 BUS OPERATIONS 00685 *******************************************************************************/ 00686 00687 /******************************* I2C Routines *********************************/ 00688 /** 00689 * @brief Initializes I2C MSP. 00690 */ 00691 static void I2Cx_MspInit(void) 00692 { 00693 GPIO_InitTypeDef gpio_init_structure; 00694 00695 /*** Configure the GPIOs ***/ 00696 /* Enable GPIO clock */ 00697 EVAL_I2Cx_SCL_SDA_GPIO_CLK_ENABLE(); 00698 00699 /* Configure I2C Tx as alternate function */ 00700 gpio_init_structure.Pin = EVAL_I2Cx_SCL_PIN; 00701 gpio_init_structure.Mode = GPIO_MODE_AF_OD; 00702 gpio_init_structure.Pull = GPIO_NOPULL; 00703 gpio_init_structure.Speed = GPIO_SPEED_FAST; 00704 gpio_init_structure.Alternate = EVAL_I2Cx_SCL_SDA_AF; 00705 HAL_GPIO_Init(EVAL_I2Cx_SCL_SDA_GPIO_PORT, &gpio_init_structure); 00706 00707 /* Configure I2C Rx as alternate function */ 00708 gpio_init_structure.Pin = EVAL_I2Cx_SDA_PIN; 00709 HAL_GPIO_Init(EVAL_I2Cx_SCL_SDA_GPIO_PORT, &gpio_init_structure); 00710 00711 /*** Configure the I2C peripheral ***/ 00712 /* Enable I2C clock */ 00713 EVAL_I2Cx_CLK_ENABLE(); 00714 00715 /* Force the I2C peripheral clock reset */ 00716 EVAL_I2Cx_FORCE_RESET(); 00717 00718 /* Release the I2C peripheral clock reset */ 00719 EVAL_I2Cx_RELEASE_RESET(); 00720 00721 /* Enable and set I2Cx Interrupt to a lower priority */ 00722 HAL_NVIC_SetPriority(EVAL_I2Cx_EV_IRQn, 0x05, 0); 00723 HAL_NVIC_EnableIRQ(EVAL_I2Cx_EV_IRQn); 00724 00725 /* Enable and set I2Cx Interrupt to a lower priority */ 00726 HAL_NVIC_SetPriority(EVAL_I2Cx_ER_IRQn, 0x05, 0); 00727 HAL_NVIC_EnableIRQ(EVAL_I2Cx_ER_IRQn); 00728 } 00729 00730 /** 00731 * @brief Initializes I2C HAL. 00732 */ 00733 static void I2Cx_Init(void) 00734 { 00735 if(HAL_FMPI2C_GetState(&hEvalI2c) == HAL_FMPI2C_STATE_RESET) 00736 { 00737 hEvalI2c.Instance = EVAL_I2Cx; 00738 hEvalI2c.Init.Timing = EVAL_I2Cx_TIMING; 00739 hEvalI2c.Init.OwnAddress1 = 0; 00740 hEvalI2c.Init.AddressingMode = FMPI2C_ADDRESSINGMODE_7BIT; 00741 hEvalI2c.Init.DualAddressMode = FMPI2C_DUALADDRESS_DISABLE; 00742 hEvalI2c.Init.OwnAddress2 = 0; 00743 hEvalI2c.Init.GeneralCallMode = FMPI2C_GENERALCALL_DISABLE; 00744 hEvalI2c.Init.NoStretchMode = FMPI2C_NOSTRETCH_DISABLE; 00745 00746 /* Init the I2C */ 00747 I2Cx_MspInit(); 00748 HAL_FMPI2C_Init(&hEvalI2c); 00749 } 00750 } 00751 00752 #if defined(USE_IOEXPANDER) 00753 00754 00755 /** 00756 * @brief Writes a single data. 00757 * @param Addr: I2C address 00758 * @param Reg: Register address 00759 * @param Value: Data to be written 00760 */ 00761 static void I2Cx_Write(uint8_t Addr, uint8_t Reg, uint8_t Value) 00762 { 00763 HAL_StatusTypeDef status = HAL_OK; 00764 00765 status = HAL_FMPI2C_Mem_Write(&hEvalI2c, Addr, (uint16_t)Reg, FMPI2C_MEMADD_SIZE_8BIT, &Value, 1, 100); 00766 00767 /* Check the communication status */ 00768 if(status != HAL_OK) 00769 { 00770 /* Execute user timeout callback */ 00771 I2Cx_Error(Addr); 00772 } 00773 } 00774 00775 /** 00776 * @brief Reads a single data. 00777 * @param Addr: I2C address 00778 * @param Reg: Register address 00779 * @retval Read data 00780 */ 00781 static uint8_t I2Cx_Read(uint8_t Addr, uint8_t Reg) 00782 { 00783 HAL_StatusTypeDef status = HAL_OK; 00784 uint8_t Value = 0; 00785 00786 status = HAL_FMPI2C_Mem_Read(&hEvalI2c, Addr, Reg, FMPI2C_MEMADD_SIZE_8BIT, &Value, 1, 1000); 00787 00788 /* Check the communication status */ 00789 if(status != HAL_OK) 00790 { 00791 /* Execute user timeout callback */ 00792 I2Cx_Error(Addr); 00793 } 00794 return Value; 00795 } 00796 00797 #endif /* USE_IOEXPANDER */ 00798 00799 /** 00800 * @brief Reads multiple data. 00801 * @param Addr: I2C address 00802 * @param Reg: Reg address 00803 * @param MemAddSize: address size 00804 * @param Buffer: Pointer to data buffer 00805 * @param Length: Length of the data 00806 * @retval Number of read data 00807 */ 00808 static HAL_StatusTypeDef I2Cx_ReadMultiple(uint8_t Addr, uint16_t Reg, uint16_t MemAddSize, uint8_t *Buffer, uint16_t Length) 00809 { 00810 HAL_StatusTypeDef status = HAL_OK; 00811 00812 status = HAL_FMPI2C_Mem_Read(&hEvalI2c, Addr, (uint16_t)Reg, MemAddSize, Buffer, Length, 1000); 00813 00814 /* Check the communication status */ 00815 if(status != HAL_OK) 00816 { 00817 /* I2C error occurred */ 00818 I2Cx_Error(Addr); 00819 } 00820 return status; 00821 } 00822 00823 /** 00824 * @brief Writes a value in a register of the device through BUS in using DMA mode. 00825 * @param Addr: Device address on BUS Bus. 00826 * @param Reg: The target register address to write 00827 * @param MemAddSize: address size 00828 * @param Buffer: The target register value to be written 00829 * @param Length: buffer size to be written 00830 * @retval HAL status 00831 */ 00832 static HAL_StatusTypeDef I2Cx_WriteMultiple(uint8_t Addr, uint16_t Reg, uint16_t MemAddSize, uint8_t *Buffer, uint16_t Length) 00833 { 00834 HAL_StatusTypeDef status = HAL_OK; 00835 00836 status = HAL_FMPI2C_Mem_Write(&hEvalI2c, Addr, (uint16_t)Reg, MemAddSize, Buffer, Length, 1000); 00837 00838 /* Check the communication status */ 00839 if(status != HAL_OK) 00840 { 00841 /* Re-Initialize the I2C Bus */ 00842 I2Cx_Error(Addr); 00843 } 00844 return status; 00845 } 00846 00847 /** 00848 * @brief Checks if target device is ready for communication. 00849 * @note This function is used with Memory devices 00850 * @param DevAddress: Target device address 00851 * @param Trials: Number of trials 00852 * @retval HAL status 00853 */ 00854 static HAL_StatusTypeDef I2Cx_IsDeviceReady(uint16_t DevAddress, uint32_t Trials) 00855 { 00856 return (HAL_FMPI2C_IsDeviceReady(&hEvalI2c, DevAddress, Trials, 1000)); 00857 } 00858 00859 /** 00860 * @brief Manages error callback by re-initializing I2C. 00861 * @param Addr: I2C Address 00862 */ 00863 static void I2Cx_Error(uint8_t Addr) 00864 { 00865 /* De-initialize the I2C communication bus */ 00866 HAL_FMPI2C_DeInit(&hEvalI2c); 00867 00868 /* Re-Initialize the I2C communication bus */ 00869 I2Cx_Init(); 00870 } 00871 00872 /*************************** FMC Routines ************************************/ 00873 /** 00874 * @brief Initializes FMC_BANK1 MSP. 00875 */ 00876 static void FMC_BANK1_MspInit(void) 00877 { 00878 GPIO_InitTypeDef gpio_init_structure; 00879 00880 /* Enable FMC clock */ 00881 __HAL_RCC_FMC_CLK_ENABLE(); 00882 00883 /* Enable GPIOs clock */ 00884 __HAL_RCC_GPIOD_CLK_ENABLE(); 00885 __HAL_RCC_GPIOE_CLK_ENABLE(); 00886 __HAL_RCC_GPIOF_CLK_ENABLE(); 00887 00888 /* Common GPIO configuration */ 00889 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00890 gpio_init_structure.Pull = GPIO_PULLUP; 00891 gpio_init_structure.Speed = GPIO_SPEED_HIGH; 00892 gpio_init_structure.Alternate = GPIO_AF12_FMC; 00893 00894 /* GPIOD configuration */ /* GPIO_PIN_7 is FMC_NE1 */ 00895 gpio_init_structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_4 | GPIO_PIN_5 | GPIO_PIN_8 |\ 00896 GPIO_PIN_9 | GPIO_PIN_10 | GPIO_PIN_14 | GPIO_PIN_15 | GPIO_PIN_7; 00897 00898 HAL_GPIO_Init(GPIOD, &gpio_init_structure); 00899 00900 /* GPIOE configuration */ 00901 gpio_init_structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_3| GPIO_PIN_4 | GPIO_PIN_7 |\ 00902 GPIO_PIN_8 | GPIO_PIN_9 | GPIO_PIN_10 | GPIO_PIN_11 | GPIO_PIN_12 |\ 00903 GPIO_PIN_13 | GPIO_PIN_14 | GPIO_PIN_15; 00904 HAL_GPIO_Init(GPIOE, &gpio_init_structure); 00905 00906 /* GPIOF configuration */ 00907 gpio_init_structure.Pin = GPIO_PIN_0 ; 00908 HAL_GPIO_Init(GPIOF, &gpio_init_structure); 00909 } 00910 00911 00912 /** 00913 * @brief Initializes LCD IO. 00914 */ 00915 static void FMC_BANK1_Init(void) 00916 { 00917 SRAM_HandleTypeDef hsram; 00918 FMC_NORSRAM_TimingTypeDef sram_timing; 00919 00920 /*** Configure the SRAM Bank 1 ***/ 00921 /* Configure IPs */ 00922 hsram.Instance = FMC_NORSRAM_DEVICE; 00923 hsram.Extended = FMC_NORSRAM_EXTENDED_DEVICE; 00924 00925 sram_timing.AddressSetupTime = 5; 00926 sram_timing.AddressHoldTime = 1; 00927 sram_timing.DataSetupTime = 9; 00928 sram_timing.BusTurnAroundDuration = 0; 00929 sram_timing.CLKDivision = 2; 00930 sram_timing.DataLatency = 2; 00931 sram_timing.AccessMode = FMC_ACCESS_MODE_A; 00932 00933 hsram.Init.NSBank = FMC_NORSRAM_BANK1; 00934 hsram.Init.DataAddressMux = FMC_DATA_ADDRESS_MUX_DISABLE; 00935 hsram.Init.MemoryType = FMC_MEMORY_TYPE_SRAM; 00936 hsram.Init.MemoryDataWidth = FMC_NORSRAM_MEM_BUS_WIDTH_16; 00937 hsram.Init.BurstAccessMode = FMC_BURST_ACCESS_MODE_DISABLE; 00938 hsram.Init.WaitSignalPolarity = FMC_WAIT_SIGNAL_POLARITY_LOW; 00939 hsram.Init.WrapMode = FMC_WRAP_MODE_DISABLE; 00940 hsram.Init.WaitSignalActive = FMC_WAIT_TIMING_BEFORE_WS; 00941 hsram.Init.WriteOperation = FMC_WRITE_OPERATION_ENABLE; 00942 hsram.Init.WaitSignal = FMC_WAIT_SIGNAL_DISABLE; 00943 hsram.Init.ExtendedMode = FMC_EXTENDED_MODE_DISABLE; 00944 hsram.Init.AsynchronousWait = FMC_ASYNCHRONOUS_WAIT_DISABLE; 00945 hsram.Init.WriteBurst = FMC_WRITE_BURST_DISABLE; 00946 hsram.Init.ContinuousClock = FMC_CONTINUOUS_CLOCK_SYNC_ONLY; 00947 hsram.Init.WriteFifo = FMC_WRITE_FIFO_DISABLE; 00948 hsram.Init.PageSize = FMC_PAGE_SIZE_NONE; 00949 00950 /* Initialize the SRAM controller */ 00951 FMC_BANK1_MspInit(); 00952 HAL_SRAM_Init(&hsram, &sram_timing, &sram_timing); 00953 } 00954 00955 00956 /** 00957 * @brief Writes register value. 00958 * @param Data: Data to be written 00959 */ 00960 static void FMC_BANK1_WriteData(uint16_t Data) 00961 { 00962 /* Write 16-bit Reg */ 00963 FMC_BANK1->RAM = Data; 00964 } 00965 00966 /** 00967 * @brief Writes register address. 00968 * @param Reg: Register to be written 00969 */ 00970 static void FMC_BANK1_WriteReg(uint8_t Reg) 00971 { 00972 /* Write 16-bit Index, then write register */ 00973 FMC_BANK1->REG = Reg; 00974 } 00975 00976 /** 00977 * @brief Reads register value. 00978 * @retval Read value 00979 */ 00980 static uint16_t FMC_BANK1_ReadData(void) 00981 { 00982 return FMC_BANK1->RAM; 00983 } 00984 00985 /******************************************************************************* 00986 LINK OPERATIONS 00987 *******************************************************************************/ 00988 00989 #if defined(USE_IOEXPANDER) 00990 00991 /********************************* LINK MFX ***********************************/ 00992 00993 /** 00994 * @brief Initializes MFX low level. 00995 */ 00996 void MFX_IO_Init(void) 00997 { 00998 I2Cx_Init(); 00999 } 01000 01001 /** 01002 * @brief DeInitializes MFX low level. 01003 */ 01004 void MFX_IO_DeInit(void) 01005 { 01006 } 01007 01008 /** 01009 * @brief Configures MFX low level interrupt. 01010 */ 01011 void MFX_IO_ITConfig(void) 01012 { 01013 GPIO_InitTypeDef gpio_init_structure; 01014 01015 /* Enable the GPIO EXTI clock */ 01016 __HAL_RCC_GPIOA_CLK_ENABLE(); 01017 __HAL_RCC_SYSCFG_CLK_ENABLE(); 01018 01019 /* PA0 is the MFX_OUT_IRQ (often used for EXTI_WKUP) */ 01020 gpio_init_structure.Pin = GPIO_PIN_0; 01021 gpio_init_structure.Pull = GPIO_NOPULL; 01022 gpio_init_structure.Speed = GPIO_SPEED_LOW; 01023 gpio_init_structure.Mode = GPIO_MODE_IT_RISING; 01024 HAL_GPIO_Init(GPIOA, &gpio_init_structure); 01025 01026 /* Enable and set GPIO EXTI Interrupt to the lowest priority */ 01027 HAL_NVIC_SetPriority((IRQn_Type)(EXTI0_IRQn), 0x0F, 0x0F); 01028 HAL_NVIC_EnableIRQ((IRQn_Type)(EXTI0_IRQn)); 01029 } 01030 01031 /** 01032 * @brief MFX writes single data. 01033 * @param Addr: I2C address 01034 * @param Reg: Register address 01035 * @param Value: Data to be written 01036 */ 01037 void MFX_IO_Write(uint16_t Addr, uint8_t Reg, uint8_t Value) 01038 { 01039 I2Cx_Write((uint8_t) Addr, Reg, Value); 01040 } 01041 01042 /** 01043 * @brief MFX reads single data. 01044 * @param Addr: I2C address 01045 * @param Reg: Register address 01046 * @retval Read data 01047 */ 01048 uint8_t MFX_IO_Read(uint16_t Addr, uint8_t Reg) 01049 { 01050 return I2Cx_Read((uint8_t) Addr, Reg); 01051 } 01052 01053 /** 01054 * @brief MFX reads multiple data. 01055 * @param Addr: I2C address 01056 * @param Reg: Register address 01057 * @param Buffer: Pointer to data buffer 01058 * @param Length: Length of the data 01059 * @retval Number of read data 01060 */ 01061 uint16_t MFX_IO_ReadMultiple(uint16_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length) 01062 { 01063 return I2Cx_ReadMultiple((uint8_t) Addr, (uint16_t)Reg, FMPI2C_MEMADD_SIZE_8BIT, Buffer, Length); 01064 } 01065 01066 /** 01067 * @brief MFX delay 01068 * @param Delay: Delay in ms 01069 */ 01070 void MFX_IO_Delay(uint32_t Delay) 01071 { 01072 HAL_Delay(Delay); 01073 } 01074 01075 /** 01076 * @brief Used by Lx family but requested for MFX component compatibility. 01077 */ 01078 void MFX_IO_Wakeup(void) 01079 { 01080 } 01081 01082 /** 01083 * @brief Used by Lx family but requested for MXF component compatibility. 01084 */ 01085 void MFX_IO_EnableWakeupPin(void) 01086 { 01087 } 01088 01089 #endif /* USE_IOEXPANDER */ 01090 01091 /********************************* LINK LCD ***********************************/ 01092 01093 /** 01094 * @brief Initializes LCD low level. 01095 */ 01096 void LCD_IO_Init(void) 01097 { 01098 FMC_BANK1_Init(); 01099 } 01100 01101 /** 01102 * @brief Writes data on LCD data register. 01103 * @param RegValue: Data to be written 01104 */ 01105 void LCD_IO_WriteData(uint16_t RegValue) 01106 { 01107 /* Write 16-bit Reg */ 01108 FMC_BANK1_WriteData(RegValue); 01109 } 01110 01111 /** 01112 * @brief Writes register on LCD register. 01113 * @param Reg: Register to be written 01114 */ 01115 void LCD_IO_WriteReg(uint8_t Reg) 01116 { 01117 /* Write 16-bit Index, then Write Reg */ 01118 FMC_BANK1_WriteReg(Reg); 01119 } 01120 01121 /** 01122 * @brief Reads data from LCD data register. 01123 * @retval Read data. 01124 */ 01125 uint16_t LCD_IO_ReadData(void) 01126 { 01127 return FMC_BANK1_ReadData(); 01128 } 01129 01130 /********************************* LINK AUDIO *********************************/ 01131 01132 /** 01133 * @brief Initializes Audio low level. 01134 */ 01135 void AUDIO_IO_Init(void) 01136 { 01137 I2Cx_Init(); 01138 } 01139 01140 /** 01141 * @brief DeInitializes Audio low level. 01142 */ 01143 void AUDIO_IO_DeInit(void) 01144 { 01145 01146 } 01147 01148 /** 01149 * @brief Writes a single data. 01150 * @param Addr: I2C address 01151 * @param Reg: Reg address 01152 * @param Value: Data to be written 01153 */ 01154 void AUDIO_IO_Write(uint8_t Addr, uint16_t Reg, uint16_t Value) 01155 { 01156 uint16_t tmp = Value; 01157 01158 Value = ((uint16_t)(tmp >> 8) & 0x00FF); 01159 01160 Value |= ((uint16_t)(tmp << 8)& 0xFF00); 01161 01162 I2Cx_WriteMultiple(Addr, Reg, FMPI2C_MEMADD_SIZE_16BIT,(uint8_t*)&Value, 2); 01163 } 01164 01165 /** 01166 * @brief Reads a single data. 01167 * @param Addr: I2C address 01168 * @param Reg: Reg address 01169 * @retval Data to be read 01170 */ 01171 uint16_t AUDIO_IO_Read(uint8_t Addr, uint16_t Reg) 01172 { 01173 uint16_t read_value = 0, tmp = 0; 01174 01175 I2Cx_ReadMultiple(Addr, Reg, FMPI2C_MEMADD_SIZE_16BIT, (uint8_t*)&read_value, 2); 01176 01177 tmp = ((uint16_t)(read_value >> 8) & 0x00FF); 01178 01179 tmp |= ((uint16_t)(read_value << 8)& 0xFF00); 01180 01181 read_value = tmp; 01182 01183 return read_value; 01184 } 01185 01186 /** 01187 * @brief AUDIO Codec delay 01188 * @param Delay: Delay in ms 01189 */ 01190 void AUDIO_IO_Delay(uint32_t Delay) 01191 { 01192 HAL_Delay(Delay); 01193 } 01194 01195 /********************************* LINK CAMERA ********************************/ 01196 01197 /** 01198 * @brief Initializes Camera low level. 01199 */ 01200 void CAMERA_IO_Init(void) 01201 { 01202 I2Cx_Init(); 01203 } 01204 01205 /** 01206 * @brief Camera writes single data. 01207 * @param Addr: I2C address 01208 * @param Reg: Register address 01209 * @param Value: Data to be written 01210 */ 01211 void CAMERA_IO_Write(uint8_t Addr, uint16_t Reg, uint16_t Value) 01212 { 01213 uint16_t tmp = Value; 01214 /* For S5K5CAG sensor, 16 bits accesses are used */ 01215 Value = ((uint16_t)(tmp >> 8) & 0x00FF); 01216 Value |= ((uint16_t)(tmp << 8)& 0xFF00); 01217 I2Cx_WriteMultiple(Addr, Reg, FMPI2C_MEMADD_SIZE_16BIT,(uint8_t*)&Value, 2); 01218 } 01219 01220 /** 01221 * @brief Camera reads single data. 01222 * @param Addr: I2C address 01223 * @param Reg: Register address 01224 * @retval Read data 01225 */ 01226 uint16_t CAMERA_IO_Read(uint8_t Addr, uint16_t Reg) 01227 { 01228 uint16_t read_value = 0, tmp = 0; 01229 /* For S5K5CAG sensor, 16 bits accesses are used */ 01230 I2Cx_ReadMultiple(Addr, Reg, FMPI2C_MEMADD_SIZE_16BIT, (uint8_t*)&read_value, 2); 01231 tmp = ((uint16_t)(read_value >> 8) & 0x00FF); 01232 tmp |= ((uint16_t)(read_value << 8)& 0xFF00); 01233 read_value = tmp; 01234 return read_value; 01235 } 01236 01237 /** 01238 * @brief Camera delay 01239 * @param Delay: Delay in ms 01240 */ 01241 void CAMERA_Delay(uint32_t Delay) 01242 { 01243 HAL_Delay(Delay); 01244 } 01245 01246 /******************************** LINK I2C EEPROM *****************************/ 01247 01248 /** 01249 * @brief Initializes peripherals used by the I2C EEPROM driver. 01250 */ 01251 void EEPROM_IO_Init(void) 01252 { 01253 I2Cx_Init(); 01254 } 01255 01256 /** 01257 * @brief Write data to I2C EEPROM driver in using DMA channel. 01258 * @param DevAddress: Target device address 01259 * @param MemAddress: Internal memory address 01260 * @param pBuffer: Pointer to data buffer 01261 * @param BufferSize: Amount of data to be sent 01262 * @retval HAL status 01263 */ 01264 HAL_StatusTypeDef EEPROM_IO_WriteData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize) 01265 { 01266 return (I2Cx_WriteMultiple(DevAddress, MemAddress, FMPI2C_MEMADD_SIZE_16BIT, pBuffer, BufferSize)); 01267 } 01268 01269 /** 01270 * @brief Read data from I2C EEPROM driver in using DMA channel. 01271 * @param DevAddress: Target device address 01272 * @param MemAddress: Internal memory address 01273 * @param pBuffer: Pointer to data buffer 01274 * @param BufferSize: Amount of data to be read 01275 * @retval HAL status 01276 */ 01277 HAL_StatusTypeDef EEPROM_IO_ReadData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize) 01278 { 01279 return (I2Cx_ReadMultiple(DevAddress, MemAddress, FMPI2C_MEMADD_SIZE_16BIT, pBuffer, BufferSize)); 01280 } 01281 01282 /** 01283 * @brief Checks if target device is ready for communication. 01284 * @note This function is used with Memory devices 01285 * @param DevAddress: Target device address 01286 * @param Trials: Number of trials 01287 * @retval HAL status 01288 */ 01289 HAL_StatusTypeDef EEPROM_IO_IsDeviceReady(uint16_t DevAddress, uint32_t Trials) 01290 { 01291 return (I2Cx_IsDeviceReady(DevAddress, Trials)); 01292 } 01293 01294 01295 01296 /** 01297 * @} 01298 */ 01299 01300 /** 01301 * @} 01302 */ 01303 01304 /** 01305 * @} 01306 */ 01307 01308 /** 01309 * @} 01310 */ 01311 01312 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Fri Jan 15 2016 10:06:21 for STM32446E_EVAL BSP User Manual by
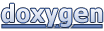