STM32446E_EVAL BSP User Manual
|
stm32446e_eval_lcd.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32446e_eval_lcd.c 00004 * @author MCD Application Team 00005 * @version V1.1.1 00006 * @date 13-January-2016 00007 * @brief This file includes the driver for Liquid Crystal Display (LCD) module 00008 * mounted on STM32446E-EVAL evaluation board. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* File Info : ----------------------------------------------------------------- 00040 User NOTES 00041 1. How To use this driver: 00042 -------------------------- 00043 - This driver is used to drive indirectly an LCD TFT. 00044 - This driver supports the ILI9325 LCD mounted on MB785 daughter board 00045 - The ILI9325 component driver MUST be included with this driver. 00046 00047 2. Driver description: 00048 --------------------- 00049 + Initialization steps: 00050 o Initialize the LCD using the BSP_LCD_Init() function. 00051 00052 + Display on LCD 00053 o Clear the hole LCD using BSP_LCD_Clear() function or only one specified string 00054 line using the BSP_LCD_ClearStringLine() function. 00055 o Display a character on the specified line and column using the BSP_LCD_DisplayChar() 00056 function or a complete string line using the BSP_LCD_DisplayStringAtLine() function. 00057 o Display a string line on the specified position (x,y in pixel) and align mode 00058 using the BSP_LCD_DisplayStringAtLine() function. 00059 o Draw and fill a basic shapes (dot, line, rectangle, circle, ellipse, .. bitmap) 00060 on LCD using the available set of functions. 00061 00062 ------------------------------------------------------------------------------*/ 00063 00064 /* Includes ------------------------------------------------------------------*/ 00065 #include "stm32446e_eval_lcd.h" 00066 #include "../../../Utilities/Fonts/fonts.h" 00067 #include "../../../Utilities/Fonts/font24.c" 00068 #include "../../../Utilities/Fonts/font20.c" 00069 #include "../../../Utilities/Fonts/font16.c" 00070 #include "../../../Utilities/Fonts/font12.c" 00071 #include "../../../Utilities/Fonts/font8.c" 00072 00073 /** @addtogroup BSP 00074 * @{ 00075 */ 00076 00077 /** @addtogroup STM32446E_EVAL 00078 * @{ 00079 */ 00080 00081 /** @defgroup STM32446E_EVAL_LCD STM32446E EVAL LCD 00082 * @{ 00083 */ 00084 00085 /** @defgroup STM32446E_EVAL_LCD_Private_TypesDefinitions STM32446E EVAL LCD Private TypesDefinitions 00086 * @{ 00087 */ 00088 /** 00089 * @} 00090 */ 00091 00092 /** @defgroup STM32446E_EVAL_LCD_Private_Defines STM32446E EVAL LCD Private Defines 00093 * @{ 00094 */ 00095 #define POLY_X(Z) ((int32_t)((Points + Z)->X)) 00096 #define POLY_Y(Z) ((int32_t)((Points + Z)->Y)) 00097 /** 00098 * @} 00099 */ 00100 00101 /** @defgroup STM32446E_EVAL_LCD_Private_Macros STM32446E EVAL LCD Private Macros 00102 * @{ 00103 */ 00104 #define ABS(X) ((X) > 0 ? (X) : -(X)) 00105 /** 00106 * @} 00107 */ 00108 00109 /** @defgroup STM32446E_EVAL_LCD_Private_Variables STM32446E EVAL LCD Private Variables 00110 * @{ 00111 */ 00112 LCD_DrawPropTypeDef DrawProp; 00113 static LCD_DrvTypeDef *LcdDrv; 00114 /** 00115 * @} 00116 */ 00117 00118 /** @defgroup STM32446E_EVAL_LCD_Private_FunctionPrototypes STM32446E EVAL LCD Private FunctionPrototypes 00119 * @{ 00120 */ 00121 static void DrawChar(uint16_t Xpos, uint16_t Ypos, const uint8_t *c); 00122 static void SetDisplayWindow(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height); 00123 static void FillTriangle(uint16_t x1, uint16_t x2, uint16_t x3, uint16_t y1, uint16_t y2, uint16_t y3); 00124 /** 00125 * @} 00126 */ 00127 00128 /** @defgroup STM32446E_EVAL_LCD_Private_Functions STM32446E EVAL LCD Private Functions 00129 * @{ 00130 */ 00131 00132 /** 00133 * @brief Initializes the LCD. 00134 * @retval LCD state 00135 */ 00136 uint8_t BSP_LCD_Init(void) 00137 { 00138 uint8_t ret = LCD_ERROR; 00139 00140 /* Default value for draw propriety */ 00141 DrawProp.BackColor = 0xFFFF; 00142 DrawProp.pFont = &Font24; 00143 DrawProp.TextColor = 0x0000; 00144 00145 if(ili9325_drv.ReadID() == ILI9325_ID) 00146 { 00147 LcdDrv = &ili9325_drv; 00148 00149 /* LCD Init */ 00150 LcdDrv->Init(); 00151 00152 /* Initialize the font */ 00153 BSP_LCD_SetFont(&LCD_DEFAULT_FONT); 00154 00155 ret = LCD_OK; 00156 } 00157 00158 return ret; 00159 } 00160 00161 /** 00162 * @brief DeInitializes the LCD. 00163 * @retval LCD state 00164 */ 00165 uint8_t BSP_LCD_DeInit(void) 00166 { 00167 /* Actually LcdDrv does not provide a DeInit function */ 00168 return LCD_OK; 00169 } 00170 00171 /** 00172 * @brief Gets the LCD X size. 00173 * @retval Used LCD X size 00174 */ 00175 uint32_t BSP_LCD_GetXSize(void) 00176 { 00177 return(LcdDrv->GetLcdPixelWidth()); 00178 } 00179 00180 /** 00181 * @brief Gets the LCD Y size. 00182 * @retval Used LCD Y size 00183 */ 00184 uint32_t BSP_LCD_GetYSize(void) 00185 { 00186 return(LcdDrv->GetLcdPixelHeight()); 00187 } 00188 00189 /** 00190 * @brief Gets the LCD text color. 00191 * @retval Used text color. 00192 */ 00193 uint16_t BSP_LCD_GetTextColor(void) 00194 { 00195 return DrawProp.TextColor; 00196 } 00197 00198 /** 00199 * @brief Gets the LCD background color. 00200 * @retval Used background color 00201 */ 00202 uint16_t BSP_LCD_GetBackColor(void) 00203 { 00204 return DrawProp.BackColor; 00205 } 00206 00207 /** 00208 * @brief Sets the LCD text color. 00209 * @param Color: Text color code RGB(5-6-5) 00210 */ 00211 void BSP_LCD_SetTextColor(uint16_t Color) 00212 { 00213 DrawProp.TextColor = Color; 00214 } 00215 00216 /** 00217 * @brief Sets the LCD background color. 00218 * @param Color: Background color code RGB(5-6-5) 00219 */ 00220 void BSP_LCD_SetBackColor(uint16_t Color) 00221 { 00222 DrawProp.BackColor = Color; 00223 } 00224 00225 /** 00226 * @brief Sets the LCD text font. 00227 * @param fonts: Font to be used 00228 */ 00229 void BSP_LCD_SetFont(sFONT *fonts) 00230 { 00231 DrawProp.pFont = fonts; 00232 } 00233 00234 /** 00235 * @brief Gets the LCD text font. 00236 * @retval Used font 00237 */ 00238 sFONT *BSP_LCD_GetFont(void) 00239 { 00240 return DrawProp.pFont; 00241 } 00242 00243 /** 00244 * @brief Clears the hole LCD. 00245 * @param Color: Color of the background 00246 */ 00247 void BSP_LCD_Clear(uint16_t Color) 00248 { 00249 uint32_t counter = 0; 00250 uint32_t y_size = 0; 00251 uint32_t color_backup = DrawProp.TextColor; 00252 00253 DrawProp.TextColor = Color; 00254 y_size = BSP_LCD_GetYSize(); 00255 00256 for(counter = 0; counter < y_size; counter++) 00257 { 00258 BSP_LCD_DrawHLine(0, counter, BSP_LCD_GetXSize()); 00259 } 00260 DrawProp.TextColor = color_backup; 00261 BSP_LCD_SetTextColor(DrawProp.TextColor); 00262 } 00263 00264 /** 00265 * @brief Clears the selected line. 00266 * @param Line: Line to be cleared 00267 * This parameter can be one of the following values: 00268 * @arg 0..9: if the Current fonts is Font16x24 00269 * @arg 0..19: if the Current fonts is Font12x12 or Font8x12 00270 * @arg 0..29: if the Current fonts is Font8x8 00271 */ 00272 void BSP_LCD_ClearStringLine(uint16_t Line) 00273 { 00274 uint32_t color_backup = DrawProp.TextColor; 00275 00276 DrawProp.TextColor = DrawProp.BackColor;; 00277 00278 /* Draw a rectangle with background color */ 00279 BSP_LCD_FillRect(0, (Line * DrawProp.pFont->Height), BSP_LCD_GetXSize(), DrawProp.pFont->Height); 00280 00281 DrawProp.TextColor = color_backup; 00282 BSP_LCD_SetTextColor(DrawProp.TextColor); 00283 } 00284 00285 /** 00286 * @brief Displays one character. 00287 * @param Xpos: Start column address 00288 * @param Ypos: Line where to display the character shape. 00289 * @param Ascii: Character ascii code 00290 * This parameter must be a number between Min_Data = 0x20 and Max_Data = 0x7E 00291 */ 00292 void BSP_LCD_DisplayChar(uint16_t Xpos, uint16_t Ypos, uint8_t Ascii) 00293 { 00294 DrawChar(Xpos, Ypos, &DrawProp.pFont->table[(Ascii-' ') *\ 00295 DrawProp.pFont->Height * ((DrawProp.pFont->Width + 7) / 8)]); 00296 } 00297 00298 /** 00299 * @brief Displays characters on the LCD. 00300 * @param Xpos: X position (in pixel) 00301 * @param Ypos: Y position (in pixel) 00302 * @param Text: Pointer to string to display on LCD 00303 * @param Mode: Display mode 00304 * This parameter can be one of the following values: 00305 * @arg CENTER_MODE 00306 * @arg RIGHT_MODE 00307 * @arg LEFT_MODE 00308 */ 00309 void BSP_LCD_DisplayStringAt(uint16_t Xpos, uint16_t Ypos, uint8_t *Text, Line_ModeTypdef Mode) 00310 { 00311 uint16_t refcolumn = 1, i = 0; 00312 uint32_t size = 0, xsize = 0; 00313 uint8_t *ptr = Text; 00314 00315 /* Get the text size */ 00316 while (*ptr++) size ++ ; 00317 00318 /* Characters number per line */ 00319 xsize = (BSP_LCD_GetXSize()/DrawProp.pFont->Width); 00320 00321 switch (Mode) 00322 { 00323 case CENTER_MODE: 00324 { 00325 refcolumn = Xpos + ((xsize - size)* DrawProp.pFont->Width) / 2; 00326 break; 00327 } 00328 case LEFT_MODE: 00329 { 00330 refcolumn = Xpos; 00331 break; 00332 } 00333 case RIGHT_MODE: 00334 { 00335 refcolumn = - Xpos + ((xsize - size)*DrawProp.pFont->Width); 00336 break; 00337 } 00338 default: 00339 { 00340 refcolumn = Xpos; 00341 break; 00342 } 00343 } 00344 00345 /* Check that the Start column is located in the screen */ 00346 if ((refcolumn < 1) || (refcolumn >= 0x8000)) 00347 { 00348 refcolumn = 1; 00349 } 00350 00351 /* Send the string character by character on lCD */ 00352 while ((*Text != 0) & (((BSP_LCD_GetXSize() - (i*DrawProp.pFont->Width)) & 0xFFFF) >= DrawProp.pFont->Width)) 00353 { 00354 /* Display one character on LCD */ 00355 BSP_LCD_DisplayChar(refcolumn, Ypos, *Text); 00356 /* Decrement the column position by 16 */ 00357 refcolumn += DrawProp.pFont->Width; 00358 /* Point on the next character */ 00359 Text++; 00360 i++; 00361 } 00362 } 00363 00364 /** 00365 * @brief Displays a character on the LCD. 00366 * @param Line: Line where to display the character shape 00367 * This parameter can be one of the following values: 00368 * @arg 0..9: if the Current fonts is Font16x24 00369 * @arg 0..19: if the Current fonts is Font12x12 or Font8x12 00370 * @arg 0..29: if the Current fonts is Font8x8 00371 * @param ptr: Pointer to string to display on LCD 00372 */ 00373 void BSP_LCD_DisplayStringAtLine(uint16_t Line, uint8_t *ptr) 00374 { 00375 BSP_LCD_DisplayStringAt(0, LINE(Line), ptr, LEFT_MODE); 00376 } 00377 00378 /** 00379 * @brief Reads an LCD pixel. 00380 * @param Xpos: X position 00381 * @param Ypos: Y position 00382 * @retval RGB pixel color 00383 */ 00384 uint16_t BSP_LCD_ReadPixel(uint16_t Xpos, uint16_t Ypos) 00385 { 00386 uint16_t ret = 0; 00387 00388 if(LcdDrv->ReadPixel != NULL) 00389 { 00390 ret = LcdDrv->ReadPixel(Xpos, Ypos); 00391 } 00392 00393 return ret; 00394 } 00395 00396 /** 00397 * @brief Draws a pixel on LCD. 00398 * @param Xpos: X position 00399 * @param Ypos: Y position 00400 * @param RGB_Code: Pixel color in RGB mode (5-6-5) 00401 */ 00402 void BSP_LCD_DrawPixel(uint16_t Xpos, uint16_t Ypos, uint16_t RGB_Code) 00403 { 00404 if(LcdDrv->WritePixel != NULL) 00405 { 00406 LcdDrv->WritePixel(Xpos, Ypos, RGB_Code); 00407 } 00408 } 00409 00410 /** 00411 * @brief Draws an horizontal line. 00412 * @param Xpos: X position 00413 * @param Ypos: Y position 00414 * @param Length: Line length 00415 */ 00416 void BSP_LCD_DrawHLine(uint16_t Xpos, uint16_t Ypos, uint16_t Length) 00417 { 00418 uint32_t index = 0; 00419 00420 if(LcdDrv->DrawHLine != NULL) 00421 { 00422 LcdDrv->DrawHLine(DrawProp.TextColor, Xpos, Ypos, Length); 00423 } 00424 else 00425 { 00426 for(index = 0; index < Length; index++) 00427 { 00428 BSP_LCD_DrawPixel((Xpos + index), Ypos, DrawProp.TextColor); 00429 } 00430 } 00431 } 00432 00433 /** 00434 * @brief Draws a vertical line. 00435 * @param Xpos: X position 00436 * @param Ypos: Y position 00437 * @param Length: Line length 00438 */ 00439 void BSP_LCD_DrawVLine(uint16_t Xpos, uint16_t Ypos, uint16_t Length) 00440 { 00441 uint32_t index = 0; 00442 00443 if(LcdDrv->DrawVLine != NULL) 00444 { 00445 LcdDrv->DrawVLine(DrawProp.TextColor, Xpos, Ypos, Length); 00446 } 00447 else 00448 { 00449 for(index = 0; index < Length; index++) 00450 { 00451 BSP_LCD_DrawPixel(Xpos, Ypos + index, DrawProp.TextColor); 00452 } 00453 } 00454 } 00455 00456 /** 00457 * @brief Draws an uni-line (between two points). 00458 * @param x1: Point 1 X position 00459 * @param y1: Point 1 Y position 00460 * @param x2: Point 2 X position 00461 * @param y2: Point 2 Y position 00462 */ 00463 void BSP_LCD_DrawLine(uint16_t x1, uint16_t y1, uint16_t x2, uint16_t y2) 00464 { 00465 int16_t deltax = 0, deltay = 0, x = 0, y = 0, xinc1 = 0, xinc2 = 0, 00466 yinc1 = 0, yinc2 = 0, den = 0, num = 0, numadd = 0, numpixels = 0, 00467 curpixel = 0; 00468 00469 deltax = ABS(x2 - x1); /* The difference between the x's */ 00470 deltay = ABS(y2 - y1); /* The difference between the y's */ 00471 x = x1; /* Start x off at the first pixel */ 00472 y = y1; /* Start y off at the first pixel */ 00473 00474 if (x2 >= x1) /* The x-values are increasing */ 00475 { 00476 xinc1 = 1; 00477 xinc2 = 1; 00478 } 00479 else /* The x-values are decreasing */ 00480 { 00481 xinc1 = -1; 00482 xinc2 = -1; 00483 } 00484 00485 if (y2 >= y1) /* The y-values are increasing */ 00486 { 00487 yinc1 = 1; 00488 yinc2 = 1; 00489 } 00490 else /* The y-values are decreasing */ 00491 { 00492 yinc1 = -1; 00493 yinc2 = -1; 00494 } 00495 00496 if (deltax >= deltay) /* There is at least one x-value for every y-value */ 00497 { 00498 xinc1 = 0; /* Don't change the x when numerator >= denominator */ 00499 yinc2 = 0; /* Don't change the y for every iteration */ 00500 den = deltax; 00501 num = deltax / 2; 00502 numadd = deltay; 00503 numpixels = deltax; /* There are more x-values than y-values */ 00504 } 00505 else /* There is at least one y-value for every x-value */ 00506 { 00507 xinc2 = 0; /* Don't change the x for every iteration */ 00508 yinc1 = 0; /* Don't change the y when numerator >= denominator */ 00509 den = deltay; 00510 num = deltay / 2; 00511 numadd = deltax; 00512 numpixels = deltay; /* There are more y-values than x-values */ 00513 } 00514 00515 for (curpixel = 0; curpixel <= numpixels; curpixel++) 00516 { 00517 BSP_LCD_DrawPixel(x, y, DrawProp.TextColor); /* Draw the current pixel */ 00518 num += numadd; /* Increase the numerator by the top of the fraction */ 00519 if (num >= den) /* Check if numerator >= denominator */ 00520 { 00521 num -= den; /* Calculate the new numerator value */ 00522 x += xinc1; /* Change the x as appropriate */ 00523 y += yinc1; /* Change the y as appropriate */ 00524 } 00525 x += xinc2; /* Change the x as appropriate */ 00526 y += yinc2; /* Change the y as appropriate */ 00527 } 00528 } 00529 00530 /** 00531 * @brief Draws a rectangle. 00532 * @param Xpos: X position 00533 * @param Ypos: Y position 00534 * @param Width: Rectangle width 00535 * @param Height: Rectangle height 00536 */ 00537 void BSP_LCD_DrawRect(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) 00538 { 00539 /* Draw horizontal lines */ 00540 BSP_LCD_DrawHLine(Xpos, Ypos, Width); 00541 BSP_LCD_DrawHLine(Xpos, (Ypos+ Height), Width); 00542 00543 /* Draw vertical lines */ 00544 BSP_LCD_DrawVLine(Xpos, Ypos, Height); 00545 BSP_LCD_DrawVLine((Xpos + Width), Ypos, Height); 00546 } 00547 00548 /** 00549 * @brief Draws a circle. 00550 * @param Xpos: X position 00551 * @param Ypos: Y position 00552 * @param Radius: Circle radius 00553 */ 00554 void BSP_LCD_DrawCircle(uint16_t Xpos, uint16_t Ypos, uint16_t Radius) 00555 { 00556 int32_t decision; /* Decision Variable */ 00557 uint32_t current_x; /* Current X Value */ 00558 uint32_t current_y; /* Current Y Value */ 00559 00560 decision = 3 - (Radius << 1); 00561 current_x = 0; 00562 current_y = Radius; 00563 00564 while (current_x <= current_y) 00565 { 00566 BSP_LCD_DrawPixel((Xpos + current_x), (Ypos - current_y), DrawProp.TextColor); 00567 00568 BSP_LCD_DrawPixel((Xpos - current_x), (Ypos - current_y), DrawProp.TextColor); 00569 00570 BSP_LCD_DrawPixel((Xpos + current_y), (Ypos - current_x), DrawProp.TextColor); 00571 00572 BSP_LCD_DrawPixel((Xpos - current_y), (Ypos - current_x), DrawProp.TextColor); 00573 00574 BSP_LCD_DrawPixel((Xpos + current_x), (Ypos + current_y), DrawProp.TextColor); 00575 00576 BSP_LCD_DrawPixel((Xpos - current_x), (Ypos + current_y), DrawProp.TextColor); 00577 00578 BSP_LCD_DrawPixel((Xpos + current_y), (Ypos + current_x), DrawProp.TextColor); 00579 00580 BSP_LCD_DrawPixel((Xpos - current_y), (Ypos + current_x), DrawProp.TextColor); 00581 00582 /* Initialize the font */ 00583 BSP_LCD_SetFont(&LCD_DEFAULT_FONT); 00584 00585 if (decision < 0) 00586 { 00587 decision += (current_x << 2) + 6; 00588 } 00589 else 00590 { 00591 decision += ((current_x - current_y) << 2) + 10; 00592 current_y--; 00593 } 00594 current_x++; 00595 } 00596 } 00597 00598 /** 00599 * @brief Draws an poly-line (between many points). 00600 * @param Points: Pointer to the points array 00601 * @param PointCount: Number of points 00602 */ 00603 void BSP_LCD_DrawPolygon(pPoint Points, uint16_t PointCount) 00604 { 00605 int16_t x = 0, y = 0; 00606 00607 if(PointCount < 2) 00608 { 00609 return; 00610 } 00611 00612 BSP_LCD_DrawLine(Points->X, Points->Y, (Points+PointCount-1)->X, (Points+PointCount-1)->Y); 00613 00614 while(--PointCount) 00615 { 00616 x = Points->X; 00617 y = Points->Y; 00618 Points++; 00619 BSP_LCD_DrawLine(x, y, Points->X, Points->Y); 00620 } 00621 } 00622 00623 /** 00624 * @brief Draws an ellipse on LCD. 00625 * @param Xpos: X position 00626 * @param Ypos: Y position 00627 * @param XRadius: Ellipse X radius 00628 * @param YRadius: Ellipse Y radius 00629 */ 00630 void BSP_LCD_DrawEllipse(int Xpos, int Ypos, int XRadius, int YRadius) 00631 { 00632 int x = 0, y = -YRadius, err = 2-2*XRadius, e2; 00633 float k = 0, rad1 = 0, rad2 = 0; 00634 00635 rad1 = XRadius; 00636 rad2 = YRadius; 00637 00638 k = (float)(rad2/rad1); 00639 00640 do { 00641 BSP_LCD_DrawPixel((Xpos-(uint16_t)(x/k)), (Ypos+y), DrawProp.TextColor); 00642 BSP_LCD_DrawPixel((Xpos+(uint16_t)(x/k)), (Ypos+y), DrawProp.TextColor); 00643 BSP_LCD_DrawPixel((Xpos+(uint16_t)(x/k)), (Ypos-y), DrawProp.TextColor); 00644 BSP_LCD_DrawPixel((Xpos-(uint16_t)(x/k)), (Ypos-y), DrawProp.TextColor); 00645 00646 e2 = err; 00647 if (e2 <= x) { 00648 err += ++x*2+1; 00649 if (-y == x && e2 <= y) e2 = 0; 00650 } 00651 if (e2 > y) err += ++y*2+1; 00652 } 00653 while (y <= 0); 00654 } 00655 00656 /** 00657 * @brief Draws a bitmap picture (16 bpp). 00658 * @param Xpos: Bmp X position in the LCD 00659 * @param Ypos: Bmp Y position in the LCD 00660 * @param pbmp: Pointer to Bmp picture address. 00661 */ 00662 void BSP_LCD_DrawBitmap(uint16_t Xpos, uint16_t Ypos, uint8_t *pbmp) 00663 { 00664 uint32_t height = 0; 00665 uint32_t width = 0; 00666 00667 00668 /* Read bitmap width */ 00669 width = *(uint16_t *) (pbmp + 18); 00670 width |= (*(uint16_t *) (pbmp + 20)) << 16; 00671 00672 /* Read bitmap height */ 00673 height = *(uint16_t *) (pbmp + 22); 00674 height |= (*(uint16_t *) (pbmp + 24)) << 16; 00675 00676 SetDisplayWindow(Xpos, Ypos, width, height); 00677 00678 if(LcdDrv->DrawBitmap != NULL) 00679 { 00680 LcdDrv->DrawBitmap(Xpos, Ypos, pbmp); 00681 } 00682 SetDisplayWindow(0, 0, BSP_LCD_GetXSize(), BSP_LCD_GetYSize()); 00683 } 00684 00685 /** 00686 * @brief Draws RGB Image (16 bpp). 00687 * @param Xpos: X position in the LCD 00688 * @param Ypos: Y position in the LCD 00689 * @param Xsize: X size in the LCD 00690 * @param Ysize: Y size in the LCD 00691 * @param pdata: Pointer to the RGB Image address. 00692 */ 00693 void BSP_LCD_DrawRGBImage(uint16_t Xpos, uint16_t Ypos, uint16_t Xsize, uint16_t Ysize, uint8_t *pdata) 00694 { 00695 00696 SetDisplayWindow(Xpos, Ypos, Xsize, Ysize); 00697 00698 if(LcdDrv->DrawRGBImage != NULL) 00699 { 00700 LcdDrv->DrawRGBImage(Xpos, Ypos, Xsize, Ysize, pdata); 00701 } 00702 SetDisplayWindow(0, 0, BSP_LCD_GetXSize(), BSP_LCD_GetYSize()); 00703 } 00704 00705 /** 00706 * @brief Draws a full rectangle. 00707 * @param Xpos: X position 00708 * @param Ypos: Y position 00709 * @param Width: Rectangle width 00710 * @param Height: Rectangle height 00711 */ 00712 void BSP_LCD_FillRect(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) 00713 { 00714 BSP_LCD_SetTextColor(DrawProp.TextColor); 00715 do 00716 { 00717 BSP_LCD_DrawHLine(Xpos, Ypos++, Width); 00718 } 00719 while(Height--); 00720 } 00721 00722 /** 00723 * @brief Draws a full circle. 00724 * @param Xpos: X position 00725 * @param Ypos: Y position 00726 * @param Radius: Circle radius 00727 */ 00728 void BSP_LCD_FillCircle(uint16_t Xpos, uint16_t Ypos, uint16_t Radius) 00729 { 00730 int32_t decision; /* Decision Variable */ 00731 uint32_t current_x; /* Current X Value */ 00732 uint32_t current_y; /* Current Y Value */ 00733 00734 decision = 3 - (Radius << 1); 00735 00736 current_x = 0; 00737 current_y = Radius; 00738 00739 BSP_LCD_SetTextColor(DrawProp.TextColor); 00740 00741 while (current_x <= current_y) 00742 { 00743 if(current_y > 0) 00744 { 00745 BSP_LCD_DrawHLine(Xpos - current_y, Ypos + current_x, 2*current_y); 00746 BSP_LCD_DrawHLine(Xpos - current_y, Ypos - current_x, 2*current_y); 00747 } 00748 00749 if(current_x > 0) 00750 { 00751 BSP_LCD_DrawHLine(Xpos - current_x, Ypos - current_y, 2*current_x); 00752 BSP_LCD_DrawHLine(Xpos - current_x, Ypos + current_y, 2*current_x); 00753 } 00754 if (decision < 0) 00755 { 00756 decision += (current_x << 2) + 6; 00757 } 00758 else 00759 { 00760 decision += ((current_x - current_y) << 2) + 10; 00761 current_y--; 00762 } 00763 current_x++; 00764 } 00765 00766 BSP_LCD_SetTextColor(DrawProp.TextColor); 00767 BSP_LCD_DrawCircle(Xpos, Ypos, Radius); 00768 } 00769 00770 /** 00771 * @brief Draws a full poly-line (between many points). 00772 * @param Points: Pointer to the points array 00773 * @param PointCount: Number of points 00774 */ 00775 void BSP_LCD_FillPolygon(pPoint Points, uint16_t PointCount) 00776 { 00777 int16_t X = 0, Y = 0, X2 = 0, Y2 = 0, X_center = 0, Y_center = 0, X_first = 0, Y_first = 0, pixelX = 0, pixelY = 0, counter = 0; 00778 uint16_t IMAGE_LEFT = 0, IMAGE_RIGHT = 0, IMAGE_TOP = 0, IMAGE_BOTTOM = 0; 00779 00780 IMAGE_LEFT = IMAGE_RIGHT = Points->X; 00781 IMAGE_TOP= IMAGE_BOTTOM = Points->Y; 00782 00783 for(counter = 1; counter < PointCount; counter++) 00784 { 00785 pixelX = POLY_X(counter); 00786 if(pixelX < IMAGE_LEFT) 00787 { 00788 IMAGE_LEFT = pixelX; 00789 } 00790 if(pixelX > IMAGE_RIGHT) 00791 { 00792 IMAGE_RIGHT = pixelX; 00793 } 00794 00795 pixelY = POLY_Y(counter); 00796 if(pixelY < IMAGE_TOP) 00797 { 00798 IMAGE_TOP = pixelY; 00799 } 00800 if(pixelY > IMAGE_BOTTOM) 00801 { 00802 IMAGE_BOTTOM = pixelY; 00803 } 00804 } 00805 00806 if(PointCount < 2) 00807 { 00808 return; 00809 } 00810 00811 X_center = (IMAGE_LEFT + IMAGE_RIGHT)/2; 00812 Y_center = (IMAGE_BOTTOM + IMAGE_TOP)/2; 00813 00814 X_first = Points->X; 00815 Y_first = Points->Y; 00816 00817 while(--PointCount) 00818 { 00819 X = Points->X; 00820 Y = Points->Y; 00821 Points++; 00822 X2 = Points->X; 00823 Y2 = Points->Y; 00824 00825 FillTriangle(X, X2, X_center, Y, Y2, Y_center); 00826 FillTriangle(X, X_center, X2, Y, Y_center, Y2); 00827 FillTriangle(X_center, X2, X, Y_center, Y2, Y); 00828 } 00829 00830 FillTriangle(X_first, X2, X_center, Y_first, Y2, Y_center); 00831 FillTriangle(X_first, X_center, X2, Y_first, Y_center, Y2); 00832 FillTriangle(X_center, X2, X_first, Y_center, Y2, Y_first); 00833 } 00834 00835 /** 00836 * @brief Draws a full ellipse. 00837 * @param Xpos: X position 00838 * @param Ypos: Y position 00839 * @param XRadius: Ellipse X radius 00840 * @param YRadius: Ellipse Y radius 00841 */ 00842 void BSP_LCD_FillEllipse(int Xpos, int Ypos, int XRadius, int YRadius) 00843 { 00844 int x = 0, y = -YRadius, err = 2-2*XRadius, e2; 00845 float k = 0, rad1 = 0, rad2 = 0; 00846 00847 rad1 = XRadius; 00848 rad2 = YRadius; 00849 00850 k = (float)(rad2/rad1); 00851 00852 do 00853 { 00854 BSP_LCD_DrawHLine((Xpos-(uint16_t)(x/k)), (Ypos+y), (2*(uint16_t)(x/k) + 1)); 00855 BSP_LCD_DrawHLine((Xpos-(uint16_t)(x/k)), (Ypos-y), (2*(uint16_t)(x/k) + 1)); 00856 00857 e2 = err; 00858 if (e2 <= x) 00859 { 00860 err += ++x*2+1; 00861 if (-y == x && e2 <= y) e2 = 0; 00862 } 00863 if (e2 > y) err += ++y*2+1; 00864 } 00865 while (y <= 0); 00866 } 00867 00868 /** 00869 * @brief Enables the display. 00870 */ 00871 void BSP_LCD_DisplayOn(void) 00872 { 00873 LcdDrv->DisplayOn(); 00874 } 00875 00876 /** 00877 * @brief Disables the display. 00878 */ 00879 void BSP_LCD_DisplayOff(void) 00880 { 00881 LcdDrv->DisplayOff(); 00882 } 00883 00884 /****************************************************************************** 00885 Static Functions 00886 *******************************************************************************/ 00887 00888 /** 00889 * @brief Draws a character on LCD. 00890 * @param Xpos: Line where to display the character shape 00891 * @param Ypos: Start column address 00892 * @param c: Pointer to the character data 00893 */ 00894 static void DrawChar(uint16_t Xpos, uint16_t Ypos, const uint8_t *c) 00895 { 00896 uint32_t i = 0, j = 0; 00897 uint16_t height, width; 00898 uint8_t offset; 00899 uint8_t *pchar; 00900 uint32_t line; 00901 00902 height = DrawProp.pFont->Height; 00903 width = DrawProp.pFont->Width; 00904 00905 offset = 8 *((width + 7)/8) - width ; 00906 00907 for(i = 0; i < height; i++) 00908 { 00909 pchar = ((uint8_t *)c + (width + 7)/8 * i); 00910 00911 switch(((width + 7)/8)) 00912 { 00913 case 1: 00914 line = pchar[0]; 00915 break; 00916 00917 case 2: 00918 line = (pchar[0]<< 8) | pchar[1]; 00919 break; 00920 00921 case 3: 00922 default: 00923 line = (pchar[0]<< 16) | (pchar[1]<< 8) | pchar[2]; 00924 break; 00925 } 00926 00927 for (j = 0; j < width; j++) 00928 { 00929 if(line & (1 << (width- j + offset- 1))) 00930 { 00931 BSP_LCD_DrawPixel((Xpos + j), Ypos, DrawProp.TextColor); 00932 } 00933 else 00934 { 00935 BSP_LCD_DrawPixel((Xpos + j), Ypos, DrawProp.BackColor); 00936 } 00937 } 00938 Ypos++; 00939 } 00940 } 00941 00942 /** 00943 * @brief Sets display window. 00944 * @param Xpos: LCD X position 00945 * @param Ypos: LCD Y position 00946 * @param Width: LCD window width 00947 * @param Height: LCD window height 00948 */ 00949 static void SetDisplayWindow(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) 00950 { 00951 if(LcdDrv->SetDisplayWindow != NULL) 00952 { 00953 LcdDrv->SetDisplayWindow(Xpos, Ypos, Width, Height); 00954 } 00955 } 00956 00957 /** 00958 * @brief Fills a triangle (between 3 points). 00959 * @param x1: Point 1 X position 00960 * @param y1: Point 1 Y position 00961 * @param x2: Point 2 X position 00962 * @param y2: Point 2 Y position 00963 * @param x3: Point 3 X position 00964 * @param y3: Point 3 Y position 00965 */ 00966 static void FillTriangle(uint16_t x1, uint16_t x2, uint16_t x3, uint16_t y1, uint16_t y2, uint16_t y3) 00967 { 00968 int16_t deltax = 0, deltay = 0, x = 0, y = 0, xinc1 = 0, xinc2 = 0, 00969 yinc1 = 0, yinc2 = 0, den = 0, num = 0, numadd = 0, numpixels = 0, 00970 curpixel = 0; 00971 00972 deltax = ABS(x2 - x1); /* The difference between the x's */ 00973 deltay = ABS(y2 - y1); /* The difference between the y's */ 00974 x = x1; /* Start x off at the first pixel */ 00975 y = y1; /* Start y off at the first pixel */ 00976 00977 if (x2 >= x1) /* The x-values are increasing */ 00978 { 00979 xinc1 = 1; 00980 xinc2 = 1; 00981 } 00982 else /* The x-values are decreasing */ 00983 { 00984 xinc1 = -1; 00985 xinc2 = -1; 00986 } 00987 00988 if (y2 >= y1) /* The y-values are increasing */ 00989 { 00990 yinc1 = 1; 00991 yinc2 = 1; 00992 } 00993 else /* The y-values are decreasing */ 00994 { 00995 yinc1 = -1; 00996 yinc2 = -1; 00997 } 00998 00999 if (deltax >= deltay) /* There is at least one x-value for every y-value */ 01000 { 01001 xinc1 = 0; /* Don't change the x when numerator >= denominator */ 01002 yinc2 = 0; /* Don't change the y for every iteration */ 01003 den = deltax; 01004 num = deltax / 2; 01005 numadd = deltay; 01006 numpixels = deltax; /* There are more x-values than y-values */ 01007 } 01008 else /* There is at least one y-value for every x-value */ 01009 { 01010 xinc2 = 0; /* Don't change the x for every iteration */ 01011 yinc1 = 0; /* Don't change the y when numerator >= denominator */ 01012 den = deltay; 01013 num = deltay / 2; 01014 numadd = deltax; 01015 numpixels = deltay; /* There are more y-values than x-values */ 01016 } 01017 01018 for (curpixel = 0; curpixel <= numpixels; curpixel++) 01019 { 01020 BSP_LCD_DrawLine(x, y, x3, y3); 01021 01022 num += numadd; /* Increase the numerator by the top of the fraction */ 01023 if (num >= den) /* Check if numerator >= denominator */ 01024 { 01025 num -= den; /* Calculate the new numerator value */ 01026 x += xinc1; /* Change the x as appropriate */ 01027 y += yinc1; /* Change the y as appropriate */ 01028 } 01029 x += xinc2; /* Change the x as appropriate */ 01030 y += yinc2; /* Change the y as appropriate */ 01031 } 01032 } 01033 01034 /** 01035 * @} 01036 */ 01037 01038 /** 01039 * @} 01040 */ 01041 01042 /** 01043 * @} 01044 */ 01045 01046 /** 01047 * @} 01048 */ 01049 01050 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Fri Jan 15 2016 10:06:21 for STM32446E_EVAL BSP User Manual by
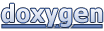