STM32303C_EVAL BSP User Manual
|
stm32303c_eval_sd.h
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32303c_eval_sd.h 00004 * @author MCD Application Team 00005 * @brief This file contains the common defines and functions prototypes for 00006 * the stm32303c_eval_sd.c driver. 00007 ****************************************************************************** 00008 * @attention 00009 * 00010 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00011 * 00012 * Redistribution and use in source and binary forms, with or without modification, 00013 * are permitted provided that the following conditions are met: 00014 * 1. Redistributions of source code must retain the above copyright notice, 00015 * this list of conditions and the following disclaimer. 00016 * 2. Redistributions in binary form must reproduce the above copyright notice, 00017 * this list of conditions and the following disclaimer in the documentation 00018 * and/or other materials provided with the distribution. 00019 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00020 * may be used to endorse or promote products derived from this software 00021 * without specific prior written permission. 00022 * 00023 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00024 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00025 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00026 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00027 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00028 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00029 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00030 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00031 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00032 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00033 * 00034 ****************************************************************************** 00035 */ 00036 00037 /* Define to prevent recursive inclusion -------------------------------------*/ 00038 #ifndef __STM32303C_EVAL_SD_H 00039 #define __STM32303C_EVAL_SD_H 00040 00041 #ifdef __cplusplus 00042 extern "C" { 00043 #endif 00044 00045 /* Includes ------------------------------------------------------------------*/ 00046 #include "stm32303c_eval.h" 00047 00048 /** @addtogroup BSP 00049 * @{ 00050 */ 00051 00052 /** @addtogroup STM32303C_EVAL 00053 * @{ 00054 */ 00055 00056 /** @defgroup STM32303C_EVAL_SD STM32303C-EVAL SD 00057 * @{ 00058 */ 00059 00060 /* Private define ------------------------------------------------------------*/ 00061 00062 /** @defgroup STM32303C_EVAL_SD_Private_Defines Private Constants 00063 * @{ 00064 */ 00065 /** 00066 * @} 00067 */ 00068 /* Private variables ---------------------------------------------------------*/ 00069 00070 /** @defgroup STM32303C_EVAL_SD_Private_Variables Private Variables 00071 * @{ 00072 */ 00073 /** 00074 * @} 00075 */ 00076 00077 /** @defgroup STM32303C_EVAL_SD_Exported_Types Exported Types 00078 * @{ 00079 */ 00080 00081 /** 00082 * @brief SD status structure definition 00083 */ 00084 #define MSD_OK 0x00 00085 #define MSD_ERROR 0x01 00086 00087 typedef enum 00088 { 00089 /** 00090 * @brief SD reponses and error flags 00091 */ 00092 SD_RESPONSE_NO_ERROR = (0x00), 00093 SD_IN_IDLE_STATE = (0x01), 00094 SD_ERASE_RESET = (0x02), 00095 SD_ILLEGAL_COMMAND = (0x04), 00096 SD_COM_CRC_ERROR = (0x08), 00097 SD_ERASE_SEQUENCE_ERROR = (0x10), 00098 SD_ADDRESS_ERROR = (0x20), 00099 SD_PARAMETER_ERROR = (0x40), 00100 SD_RESPONSE_FAILURE = (0xFF), 00101 00102 /** 00103 * @brief Data response error 00104 */ 00105 SD_DATA_OK = (0x05), 00106 SD_DATA_CRC_ERROR = (0x0B), 00107 SD_DATA_WRITE_ERROR = (0x0D), 00108 SD_DATA_OTHER_ERROR = (0xFF) 00109 }SD_Info; 00110 00111 /** 00112 * @brief Card Specific Data: CSD Register 00113 */ 00114 typedef struct 00115 { 00116 __IO uint8_t CSDStruct; /* CSD structure */ 00117 __IO uint8_t SysSpecVersion; /* System specification version */ 00118 __IO uint8_t Reserved1; /* Reserved */ 00119 __IO uint8_t TAAC; /* Data read access-time 1 */ 00120 __IO uint8_t NSAC; /* Data read access-time 2 in CLK cycles */ 00121 __IO uint8_t MaxBusClkFrec; /* Max. bus clock frequency */ 00122 __IO uint16_t CardComdClasses; /* Card command classes */ 00123 __IO uint8_t RdBlockLen; /* Max. read data block length */ 00124 __IO uint8_t PartBlockRead; /* Partial blocks for read allowed */ 00125 __IO uint8_t WrBlockMisalign; /* Write block misalignment */ 00126 __IO uint8_t RdBlockMisalign; /* Read block misalignment */ 00127 __IO uint8_t DSRImpl; /* DSR implemented */ 00128 __IO uint8_t Reserved2; /* Reserved */ 00129 __IO uint32_t DeviceSize; /* Device Size */ 00130 __IO uint8_t MaxRdCurrentVDDMin; /* Max. read current @ VDD min */ 00131 __IO uint8_t MaxRdCurrentVDDMax; /* Max. read current @ VDD max */ 00132 __IO uint8_t MaxWrCurrentVDDMin; /* Max. write current @ VDD min */ 00133 __IO uint8_t MaxWrCurrentVDDMax; /* Max. write current @ VDD max */ 00134 __IO uint8_t DeviceSizeMul; /* Device size multiplier */ 00135 __IO uint8_t EraseGrSize; /* Erase group size */ 00136 __IO uint8_t EraseGrMul; /* Erase group size multiplier */ 00137 __IO uint8_t WrProtectGrSize; /* Write protect group size */ 00138 __IO uint8_t WrProtectGrEnable; /* Write protect group enable */ 00139 __IO uint8_t ManDeflECC; /* Manufacturer default ECC */ 00140 __IO uint8_t WrSpeedFact; /* Write speed factor */ 00141 __IO uint8_t MaxWrBlockLen; /* Max. write data block length */ 00142 __IO uint8_t WriteBlockPaPartial; /* Partial blocks for write allowed */ 00143 __IO uint8_t Reserved3; /* Reserded */ 00144 __IO uint8_t ContentProtectAppli; /* Content protection application */ 00145 __IO uint8_t FileFormatGrouop; /* File format group */ 00146 __IO uint8_t CopyFlag; /* Copy flag (OTP) */ 00147 __IO uint8_t PermWrProtect; /* Permanent write protection */ 00148 __IO uint8_t TempWrProtect; /* Temporary write protection */ 00149 __IO uint8_t FileFormat; /* File Format */ 00150 __IO uint8_t ECC; /* ECC code */ 00151 __IO uint8_t CSD_CRC; /* CSD CRC */ 00152 __IO uint8_t Reserved4; /* always 1*/ 00153 } SD_CSD; 00154 00155 /** 00156 * @brief Card Identification Data: CID Register 00157 */ 00158 typedef struct 00159 { 00160 __IO uint8_t ManufacturerID; /* ManufacturerID */ 00161 __IO uint16_t OEM_AppliID; /* OEM/Application ID */ 00162 __IO uint32_t ProdName1; /* Product Name part1 */ 00163 __IO uint8_t ProdName2; /* Product Name part2*/ 00164 __IO uint8_t ProdRev; /* Product Revision */ 00165 __IO uint32_t ProdSN; /* Product Serial Number */ 00166 __IO uint8_t Reserved1; /* Reserved1 */ 00167 __IO uint16_t ManufactDate; /* Manufacturing Date */ 00168 __IO uint8_t CID_CRC; /* CID CRC */ 00169 __IO uint8_t Reserved2; /* always 1 */ 00170 } SD_CID; 00171 00172 /** 00173 * @brief SD Card information 00174 */ 00175 typedef struct 00176 { 00177 SD_CSD Csd; 00178 SD_CID Cid; 00179 uint32_t CardCapacity; /* Card Capacity */ 00180 uint32_t CardBlockSize; /* Card Block Size */ 00181 } SD_CardInfo; 00182 00183 /** 00184 * @} 00185 */ 00186 00187 /** @defgroup STM32303C_EVAL_SD_Exported_Constants Exported Constants 00188 * @{ 00189 */ 00190 00191 /** 00192 * @brief Start Data tokens: 00193 * Tokens (necessary because at nop/idle (and CS active) only 0xff is 00194 * on the data/command line) 00195 */ 00196 #define SD_START_DATA_SINGLE_BLOCK_READ 0xFE /* Data token start byte, Start Single Block Read */ 00197 #define SD_START_DATA_MULTIPLE_BLOCK_READ 0xFE /* Data token start byte, Start Multiple Block Read */ 00198 #define SD_START_DATA_SINGLE_BLOCK_WRITE 0xFE /* Data token start byte, Start Single Block Write */ 00199 #define SD_START_DATA_MULTIPLE_BLOCK_WRITE 0xFD /* Data token start byte, Start Multiple Block Write */ 00200 #define SD_STOP_DATA_MULTIPLE_BLOCK_WRITE 0xFD /* Data toke stop byte, Stop Multiple Block Write */ 00201 00202 /** 00203 * @brief SD detection on its memory slot 00204 */ 00205 #define SD_PRESENT ((uint8_t)0x01) 00206 #define SD_NOT_PRESENT ((uint8_t)0x00) 00207 00208 /** 00209 * @brief Commands: CMDxx = CMD-number | 0x40 00210 */ 00211 #define SD_CMD_GO_IDLE_STATE 0 /* CMD0 = 0x40 */ 00212 #define SD_CMD_SEND_OP_COND 1 /* CMD1 = 0x41 */ 00213 #define SD_CMD_SEND_CSD 9 /* CMD9 = 0x49 */ 00214 #define SD_CMD_SEND_CID 10 /* CMD10 = 0x4A */ 00215 #define SD_CMD_STOP_TRANSMISSION 12 /* CMD12 = 0x4C */ 00216 #define SD_CMD_SEND_STATUS 13 /* CMD13 = 0x4D */ 00217 #define SD_CMD_SET_BLOCKLEN 16 /* CMD16 = 0x50 */ 00218 #define SD_CMD_READ_SINGLE_BLOCK 17 /* CMD17 = 0x51 */ 00219 #define SD_CMD_READ_MULT_BLOCK 18 /* CMD18 = 0x52 */ 00220 #define SD_CMD_SET_BLOCK_COUNT 23 /* CMD23 = 0x57 */ 00221 #define SD_CMD_WRITE_SINGLE_BLOCK 24 /* CMD24 = 0x58 */ 00222 #define SD_CMD_WRITE_MULT_BLOCK 25 /* CMD25 = 0x59 */ 00223 #define SD_CMD_PROG_CSD 27 /* CMD27 = 0x5B */ 00224 #define SD_CMD_SET_WRITE_PROT 28 /* CMD28 = 0x5C */ 00225 #define SD_CMD_CLR_WRITE_PROT 29 /* CMD29 = 0x5D */ 00226 #define SD_CMD_SEND_WRITE_PROT 30 /* CMD30 = 0x5E */ 00227 #define SD_CMD_SD_ERASE_GRP_START 32 /* CMD32 = 0x60 */ 00228 #define SD_CMD_SD_ERASE_GRP_END 33 /* CMD33 = 0x61 */ 00229 #define SD_CMD_UNTAG_SECTOR 34 /* CMD34 = 0x62 */ 00230 #define SD_CMD_ERASE_GRP_START 35 /* CMD35 = 0x63 */ 00231 #define SD_CMD_ERASE_GRP_END 36 /* CMD36 = 0x64 */ 00232 #define SD_CMD_UNTAG_ERASE_GROUP 37 /* CMD37 = 0x65 */ 00233 #define SD_CMD_ERASE 38 /* CMD38 = 0x66 */ 00234 00235 /** 00236 * @} 00237 */ 00238 00239 /** @defgroup STM32303C_EVAL_SD_Exported_Macro Exported Macro 00240 * @{ 00241 */ 00242 00243 /** 00244 * @} 00245 */ 00246 00247 /** @defgroup STM32303C_EVAL_SD_Exported_Functions Exported Functions 00248 * @{ 00249 */ 00250 uint8_t BSP_SD_Init(void); 00251 uint8_t BSP_SD_IsDetected(void); 00252 uint8_t BSP_SD_ReadBlocks(uint32_t* p32Data, uint64_t ReadAddr, uint16_t BlockSize, uint32_t NumberOfBlocks); 00253 uint8_t BSP_SD_WriteBlocks(uint32_t* p32Data, uint64_t WriteAddr, uint16_t BlockSize, uint32_t NumberOfBlocks); 00254 uint8_t BSP_SD_Erase(uint32_t StartAddr, uint32_t EndAddr); 00255 uint8_t BSP_SD_GetStatus(void); 00256 uint8_t BSP_SD_GetCardInfo(SD_CardInfo *pCardInfo); 00257 00258 /* Link functions for SD Card peripheral*/ 00259 void SD_IO_Init(void); 00260 void SD_IO_WriteByte(uint8_t Data); 00261 uint8_t SD_IO_ReadByte(void); 00262 HAL_StatusTypeDef SD_IO_WriteCmd(uint8_t Cmd, uint32_t Arg, uint8_t Crc, uint8_t Response); 00263 HAL_StatusTypeDef SD_IO_WaitResponse(uint8_t Response); 00264 void SD_IO_WriteDummy(void); 00265 00266 /** 00267 * @} 00268 */ 00269 00270 /** @defgroup STM32303C_EVAL_SD_Private_Functions Private Functions 00271 * @{ 00272 */ 00273 00274 /** 00275 * @} 00276 */ 00277 00278 /** 00279 * @} 00280 */ 00281 00282 /** 00283 * @} 00284 */ 00285 00286 /** 00287 * @} 00288 */ 00289 00290 #ifdef __cplusplus 00291 } 00292 #endif 00293 00294 #endif /* __H */ 00295 00296 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Wed May 31 2017 10:00:44 for STM32303C_EVAL BSP User Manual by
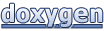