STM32303C_EVAL BSP User Manual
|
stm32303c_eval_lcd.h
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32303c_eval_lcd.h 00004 * @author MCD Application Team 00005 * @brief This file contains all the functions prototypes for the 00006 * stm32303c_eval_lcd.c driver. 00007 ****************************************************************************** 00008 * @attention 00009 * 00010 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00011 * 00012 * Redistribution and use in source and binary forms, with or without modification, 00013 * are permitted provided that the following conditions are met: 00014 * 1. Redistributions of source code must retain the above copyright notice, 00015 * this list of conditions and the following disclaimer. 00016 * 2. Redistributions in binary form must reproduce the above copyright notice, 00017 * this list of conditions and the following disclaimer in the documentation 00018 * and/or other materials provided with the distribution. 00019 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00020 * may be used to endorse or promote products derived from this software 00021 * without specific prior written permission. 00022 * 00023 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00024 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00025 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00026 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00027 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00028 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00029 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00030 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00031 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00032 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00033 * 00034 ****************************************************************************** 00035 */ 00036 00037 /* Define to prevent recursive inclusion -------------------------------------*/ 00038 #ifndef __STM32303C_EVAL_LCD_H 00039 #define __STM32303C_EVAL_LCD_H 00040 00041 #ifdef __cplusplus 00042 extern "C" { 00043 #endif 00044 00045 /* Includes ------------------------------------------------------------------*/ 00046 #include "stm32303c_eval.h" 00047 #include "../Components/hx8347d/hx8347d.h" 00048 #include "../Components/spfd5408/spfd5408.h" 00049 #include "../../../Utilities/Fonts/fonts.h" 00050 /** @addtogroup BSP 00051 * @{ 00052 */ 00053 00054 /** @addtogroup STM32303C_EVAL 00055 * @{ 00056 */ 00057 00058 /** @defgroup STM32303C_EVAL_LCD STM32303C-EVAL LCD 00059 * @{ 00060 */ 00061 00062 /** @defgroup STM32303C_EVAL_LCD_Private_Defines Private Defines 00063 * @{ 00064 */ 00065 /** 00066 * @} 00067 */ 00068 00069 /** @defgroup STM32303C_EVAL_LCD_Private_Macros Private Macros 00070 * @{ 00071 */ 00072 /** 00073 * @} 00074 */ 00075 00076 /** @defgroup STM32303C_EVAL_LCD_Private_Variables Private Variables 00077 * @{ 00078 */ 00079 /** 00080 * @} 00081 */ 00082 00083 /** @defgroup STM32303C_EVAL_LCD_Private_Functions Private Functions 00084 * @{ 00085 */ 00086 /** 00087 * @} 00088 */ 00089 00090 /** @defgroup STM32303C_EVAL_LCD_Exported_Types Exported Types 00091 * @{ 00092 */ 00093 typedef struct 00094 { 00095 uint32_t TextColor; 00096 uint32_t BackColor; 00097 sFONT *pFont; 00098 00099 }LCD_DrawPropTypeDef; 00100 /** 00101 * @} 00102 */ 00103 00104 /** @defgroup STM32303C_EVAL_LCD_Exported_Constants Exported Constants 00105 * @{ 00106 */ 00107 /** 00108 * @brief LCD status structure definition 00109 */ 00110 #define LCD_OK 0x00 00111 #define LCD_ERROR 0x01 00112 #define LCD_TIMEOUT 0x02 00113 00114 typedef struct 00115 { 00116 int16_t X; 00117 int16_t Y; 00118 00119 }Point, * pPoint; 00120 00121 /** 00122 * @brief Line mode structures definition 00123 */ 00124 typedef enum 00125 { 00126 CENTER_MODE = 0x01, /*!< Center mode */ 00127 RIGHT_MODE = 0x02, /*!< Right mode */ 00128 LEFT_MODE = 0x03 /*!< Left mode */ 00129 00130 }Line_ModeTypdef; 00131 00132 /** 00133 * @brief LCD color 00134 */ 00135 #define LCD_COLOR_BLUE 0x001F 00136 #define LCD_COLOR_GREEN 0x07E0 00137 #define LCD_COLOR_RED 0xF800 00138 #define LCD_COLOR_CYAN 0x07FF 00139 #define LCD_COLOR_MAGENTA 0xF81F 00140 #define LCD_COLOR_YELLOW 0xFFE0 00141 #define LCD_COLOR_LIGHTBLUE 0x841F 00142 #define LCD_COLOR_LIGHTGREEN 0x87F0 00143 #define LCD_COLOR_LIGHTRED 0xFC10 00144 #define LCD_COLOR_LIGHTCYAN 0x87FF 00145 #define LCD_COLOR_LIGHTMAGENTA 0xFC1F 00146 #define LCD_COLOR_LIGHTYELLOW 0xFFF0 00147 #define LCD_COLOR_DARKBLUE 0x0010 00148 #define LCD_COLOR_DARKGREEN 0x0400 00149 #define LCD_COLOR_DARKRED 0x8000 00150 #define LCD_COLOR_DARKCYAN 0x0410 00151 #define LCD_COLOR_DARKMAGENTA 0x8010 00152 #define LCD_COLOR_DARKYELLOW 0x8400 00153 #define LCD_COLOR_WHITE 0xFFFF 00154 #define LCD_COLOR_LIGHTGRAY 0xD69A 00155 #define LCD_COLOR_GRAY 0x8410 00156 #define LCD_COLOR_DARKGRAY 0x4208 00157 #define LCD_COLOR_BLACK 0x0000 00158 #define LCD_COLOR_BROWN 0xA145 00159 #define LCD_COLOR_ORANGE 0xFD20 00160 00161 /** 00162 * @brief LCD default font 00163 */ 00164 #define LCD_DEFAULT_FONT Font24 00165 00166 /** 00167 * @} 00168 */ 00169 00170 /** @defgroup STM32303C_EVAL_LCD_Exported_Functions Exported Functions 00171 * @{ 00172 */ 00173 uint8_t BSP_LCD_Init(void); 00174 uint32_t BSP_LCD_GetXSize(void); 00175 uint32_t BSP_LCD_GetYSize(void); 00176 00177 uint16_t BSP_LCD_GetTextColor(void); 00178 uint16_t BSP_LCD_GetBackColor(void); 00179 void BSP_LCD_SetTextColor(__IO uint16_t Color); 00180 void BSP_LCD_SetBackColor(__IO uint16_t Color); 00181 void BSP_LCD_SetFont(sFONT *pFonts); 00182 sFONT *BSP_LCD_GetFont(void); 00183 00184 void BSP_LCD_Clear(uint16_t Color); 00185 void BSP_LCD_ClearStringLine(uint16_t Line); 00186 void BSP_LCD_DisplayStringAtLine(uint16_t Line, uint8_t *pText); 00187 void BSP_LCD_DisplayStringAt(uint16_t Xpos, uint16_t Ypos, uint8_t *pText, Line_ModeTypdef Mode); 00188 void BSP_LCD_DisplayChar(uint16_t Xpos, uint16_t Ypos, uint8_t Ascii); 00189 00190 uint16_t BSP_LCD_ReadPixel(uint16_t Xpos, uint16_t Ypos); 00191 void BSP_LCD_DrawHLine(uint16_t Xpos, uint16_t Ypos, uint16_t Length); 00192 void BSP_LCD_DrawVLine(uint16_t Xpos, uint16_t Ypos, uint16_t Length); 00193 void BSP_LCD_DrawLine(uint16_t X1, uint16_t Y1, uint16_t X2, uint16_t Y2); 00194 void BSP_LCD_DrawRect(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height); 00195 void BSP_LCD_DrawCircle(uint16_t Xpos, uint16_t Ypos, uint16_t Radius); 00196 void BSP_LCD_DrawPolygon(pPoint pPoints, uint16_t PointCount); 00197 void BSP_LCD_DrawEllipse(int Xpos, int Ypos, int XRadius, int YRadius); 00198 void BSP_LCD_DrawBitmap(uint16_t Xpos, uint16_t Ypos, uint8_t *pBmp); 00199 00200 void BSP_LCD_FillRect(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height); 00201 void BSP_LCD_FillCircle(uint16_t Xpos, uint16_t Ypos, uint16_t Radius); 00202 void BSP_LCD_FillEllipse(int Xpos, int Ypos, int XRadius, int YRadius); 00203 00204 void BSP_LCD_DisplayOff(void); 00205 void BSP_LCD_DisplayOn(void); 00206 00207 /** 00208 * @} 00209 */ 00210 00211 /** 00212 * @} 00213 */ 00214 00215 /** 00216 * @} 00217 */ 00218 00219 /** 00220 * @} 00221 */ 00222 00223 #ifdef __cplusplus 00224 } 00225 #endif 00226 00227 #endif /* __STM32303C_EVAL_LCD_H */ 00228 00229 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Wed May 31 2017 10:00:44 for STM32303C_EVAL BSP User Manual by
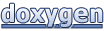