STM32303C_EVAL BSP User Manual
|
stm32303c_eval_lcd.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32303c_eval_lcd.c 00004 * @author MCD Application Team 00005 * @brief This file includes the driver for Liquid Crystal Display modules 00006 * mounted on STM32303C-EVAL evaluation board. 00007 ****************************************************************************** 00008 * @attention 00009 * 00010 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00011 * 00012 * Redistribution and use in source and binary forms, with or without modification, 00013 * are permitted provided that the following conditions are met: 00014 * 1. Redistributions of source code must retain the above copyright notice, 00015 * this list of conditions and the following disclaimer. 00016 * 2. Redistributions in binary form must reproduce the above copyright notice, 00017 * this list of conditions and the following disclaimer in the documentation 00018 * and/or other materials provided with the distribution. 00019 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00020 * may be used to endorse or promote products derived from this software 00021 * without specific prior written permission. 00022 * 00023 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00024 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00025 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00026 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00027 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00028 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00029 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00030 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00031 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00032 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00033 * 00034 ****************************************************************************** 00035 */ 00036 00037 /* File Info : ----------------------------------------------------------------- 00038 User NOTES 00039 1. How To use this driver: 00040 -------------------------- 00041 - This driver is used to drive indirectly an LCD TFT. 00042 - This driver supports the AM-240320L8TNQW00H (ILI9320), 00043 AM-240320LDTNQW00H (SPFD5408B) and AM240320LGTNQW00H (HX8347D) LCD 00044 mounted on MB895 daughter board 00045 - The ILI9320, SPFD5408B and HX8347D components driver MUST be included with this driver. 00046 00047 2. Driver description: 00048 --------------------- 00049 + Initialization steps: 00050 o Initialize the LCD using the LCD_Init() function. 00051 00052 + Display on LCD 00053 o Clear the hole LCD using yhe LCD_Clear() function or only one specified 00054 string line using the LCD_ClearStringLine() function. 00055 o Display a character on the specified line and column using the LCD_DisplayChar() 00056 function or a complete string line using the LCD_DisplayStringAtLine() function. 00057 o Display a string line on the specified position (x,y in pixel) and align mode 00058 using the LCD_DisplayStringAtLine() function. 00059 o Draw and fill a basic shapes (dot, line, rectangle, circle, ellipse, .. bitmap) 00060 on LCD using a set of functions. 00061 00062 ------------------------------------------------------------------------------*/ 00063 00064 /* Includes ------------------------------------------------------------------*/ 00065 #include "stm32303c_eval_lcd.h" 00066 #include "../../../Utilities/Fonts/fonts.h" 00067 #include "../../../Utilities/Fonts/font24.c" 00068 #include "../../../Utilities/Fonts/font20.c" 00069 #include "../../../Utilities/Fonts/font16.c" 00070 #include "../../../Utilities/Fonts/font12.c" 00071 #include "../../../Utilities/Fonts/font8.c" 00072 00073 /** @addtogroup BSP 00074 * @{ 00075 */ 00076 00077 /** @addtogroup STM32303C_EVAL 00078 * @{ 00079 */ 00080 00081 /** @addtogroup STM32303C_EVAL_LCD 00082 * @{ 00083 */ 00084 00085 /** @addtogroup STM32303C_EVAL_LCD_Private_Defines 00086 * @{ 00087 */ 00088 #define POLY_X(Z) ((int32_t)((pPoints + (Z))->X)) 00089 #define POLY_Y(Z) ((int32_t)((pPoints + (Z))->Y)) 00090 00091 #define MAX_HEIGHT_FONT 17 00092 #define MAX_WIDTH_FONT 24 00093 #define OFFSET_BITMAP 54 00094 /** 00095 * @} 00096 */ 00097 00098 /** @addtogroup STM32303C_EVAL_LCD_Private_Macros 00099 * @{ 00100 */ 00101 #define ABS(X) ((X) > 0 ? (X) : -(X)) 00102 00103 /** 00104 * @} 00105 */ 00106 00107 /** @addtogroup STM32303C_EVAL_LCD_Private_Variables STM32303C_EVAL_LCD_Private_Variables 00108 * @{ 00109 */ 00110 LCD_DrawPropTypeDef DrawProp; 00111 00112 static LCD_DrvTypeDef *lcd_drv; 00113 00114 /* Max size of bitmap will based on a font24 (17x24) */ 00115 static uint8_t bitmap[MAX_HEIGHT_FONT*MAX_WIDTH_FONT*2+OFFSET_BITMAP] = {0}; 00116 00117 /** 00118 * @} 00119 */ 00120 00121 /** @addtogroup STM32303C_EVAL_LCD_Private_Functions 00122 * @{ 00123 */ 00124 static void LCD_DrawPixel(uint16_t Xpos, uint16_t Ypos, uint16_t RGBCode); 00125 static void LCD_DrawChar(uint16_t Xpos, uint16_t Ypos, const uint8_t *pChar); 00126 static void LCD_SetDisplayWindow(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height); 00127 /** 00128 * @} 00129 */ 00130 00131 /** @addtogroup STM32303C_EVAL_LCD_Exported_Functions 00132 * @{ 00133 */ 00134 00135 /** 00136 * @brief Initializes the LCD. 00137 * @retval LCD state 00138 */ 00139 uint8_t BSP_LCD_Init(void) 00140 { 00141 uint8_t ret = LCD_ERROR; 00142 00143 /* Default value for draw propriety */ 00144 DrawProp.BackColor = 0xFFFF; 00145 DrawProp.pFont = &Font24; 00146 DrawProp.TextColor = 0x0000; 00147 00148 if(spfd5408_drv.ReadID() == SPFD5408_ID) 00149 { 00150 lcd_drv = &spfd5408_drv; 00151 ret = LCD_OK; 00152 } 00153 else 00154 { 00155 /*HX8347D_ID connected*/ 00156 lcd_drv = &hx8347d_drv; 00157 ret = LCD_OK; 00158 } 00159 00160 if(ret != LCD_ERROR) 00161 { 00162 /* LCD Init */ 00163 lcd_drv->Init(); 00164 00165 /* Initialize the font */ 00166 BSP_LCD_SetFont(&LCD_DEFAULT_FONT); 00167 } 00168 00169 return ret; 00170 } 00171 00172 /** 00173 * @brief Gets the LCD X size. 00174 * @retval Used LCD X size 00175 */ 00176 uint32_t BSP_LCD_GetXSize(void) 00177 { 00178 return(lcd_drv->GetLcdPixelWidth()); 00179 } 00180 00181 /** 00182 * @brief Gets the LCD Y size. 00183 * @retval Used LCD Y size 00184 */ 00185 uint32_t BSP_LCD_GetYSize(void) 00186 { 00187 return(lcd_drv->GetLcdPixelHeight()); 00188 } 00189 00190 /** 00191 * @brief Gets the LCD text color. 00192 * @retval Used text color. 00193 */ 00194 uint16_t BSP_LCD_GetTextColor(void) 00195 { 00196 return DrawProp.TextColor; 00197 } 00198 00199 /** 00200 * @brief Gets the LCD background color. 00201 * @retval Used background color 00202 */ 00203 uint16_t BSP_LCD_GetBackColor(void) 00204 { 00205 return DrawProp.BackColor; 00206 } 00207 00208 /** 00209 * @brief Sets the LCD text color. 00210 * @param Color Text color code RGB(5-6-5) 00211 * @retval None 00212 */ 00213 void BSP_LCD_SetTextColor(uint16_t Color) 00214 { 00215 DrawProp.TextColor = Color; 00216 } 00217 00218 /** 00219 * @brief Sets the LCD background color. 00220 * @param Color Background color code RGB(5-6-5) 00221 * @retval None 00222 */ 00223 void BSP_LCD_SetBackColor(uint16_t Color) 00224 { 00225 DrawProp.BackColor = Color; 00226 } 00227 00228 /** 00229 * @brief Sets the LCD text font. 00230 * @param pFonts Font to be used 00231 * @retval None 00232 */ 00233 void BSP_LCD_SetFont(sFONT *pFonts) 00234 { 00235 DrawProp.pFont = pFonts; 00236 } 00237 00238 /** 00239 * @brief Gets the LCD text font. 00240 * @retval Used font 00241 */ 00242 sFONT *BSP_LCD_GetFont(void) 00243 { 00244 return DrawProp.pFont; 00245 } 00246 00247 /** 00248 * @brief Clears the hole LCD. 00249 * @param Color Color of the background 00250 * @retval None 00251 */ 00252 void BSP_LCD_Clear(uint16_t Color) 00253 { 00254 uint32_t counter = 0; 00255 00256 uint32_t color_backup = DrawProp.TextColor; 00257 DrawProp.TextColor = Color; 00258 00259 for(counter = 0; counter < BSP_LCD_GetYSize(); counter++) 00260 { 00261 BSP_LCD_DrawHLine(0, counter, BSP_LCD_GetXSize()); 00262 } 00263 00264 DrawProp.TextColor = color_backup; 00265 BSP_LCD_SetTextColor(DrawProp.TextColor); 00266 } 00267 00268 /** 00269 * @brief Clears the selected line. 00270 * @param Line Line to be cleared 00271 * This parameter can be one of the following values: 00272 * @arg 0..9: if the Current fonts is Font16x24 00273 * @arg 0..19: if the Current fonts is Font12x12 or Font8x12 00274 * @arg 0..29: if the Current fonts is Font8x8 00275 * @retval None 00276 */ 00277 void BSP_LCD_ClearStringLine(uint16_t Line) 00278 { 00279 uint32_t colorbackup = DrawProp.TextColor; 00280 DrawProp.TextColor = DrawProp.BackColor;; 00281 00282 /* Draw a rectangle with background color */ 00283 BSP_LCD_FillRect(0, (Line * DrawProp.pFont->Height), BSP_LCD_GetXSize(), DrawProp.pFont->Height); 00284 00285 DrawProp.TextColor = colorbackup; 00286 BSP_LCD_SetTextColor(DrawProp.TextColor); 00287 } 00288 00289 /** 00290 * @brief Displays one character. 00291 * @param Xpos Start column address 00292 * @param Ypos Line where to display the character shape. 00293 * @param Ascii Character ascii code 00294 * This parameter must be a number between Min_Data = 0x20 and Max_Data = 0x7E 00295 * @retval None 00296 */ 00297 void BSP_LCD_DisplayChar(uint16_t Xpos, uint16_t Ypos, uint8_t Ascii) 00298 { 00299 LCD_DrawChar(Ypos, Xpos, &DrawProp.pFont->table[(Ascii-' ') *\ 00300 DrawProp.pFont->Height * ((DrawProp.pFont->Width + 7) / 8)]); 00301 } 00302 00303 /** 00304 * @brief Displays characters on the LCD. 00305 * @param Xpos X position (in pixel) 00306 * @param Ypos Y position (in pixel) 00307 * @param pText Pointer to string to display on LCD 00308 * @param Mode Display mode 00309 * This parameter can be one of the following values: 00310 * @arg CENTER_MODE 00311 * @arg RIGHT_MODE 00312 * @arg LEFT_MODE 00313 * @retval None 00314 */ 00315 void BSP_LCD_DisplayStringAt(uint16_t Xpos, uint16_t Ypos, uint8_t *pText, Line_ModeTypdef Mode) 00316 { 00317 uint16_t refcolumn = 1, counter = 0; 00318 uint32_t size = 0, ysize = 0; 00319 uint8_t *ptr = pText; 00320 00321 /* Get the text size */ 00322 while (*ptr++) size ++ ; 00323 00324 /* Characters number per line */ 00325 ysize = (BSP_LCD_GetXSize()/DrawProp.pFont->Width); 00326 00327 switch (Mode) 00328 { 00329 case CENTER_MODE: 00330 { 00331 refcolumn = Xpos + ((ysize - size)* DrawProp.pFont->Width) / 2; 00332 break; 00333 } 00334 case LEFT_MODE: 00335 { 00336 refcolumn = Xpos; 00337 break; 00338 } 00339 case RIGHT_MODE: 00340 { 00341 refcolumn = Xpos + ((ysize - size)*DrawProp.pFont->Width); 00342 break; 00343 } 00344 default: 00345 { 00346 refcolumn = Xpos; 00347 break; 00348 } 00349 } 00350 00351 /* Send the string character by character on lCD */ 00352 while ((*pText != 0) & (((BSP_LCD_GetXSize() - (counter*DrawProp.pFont->Width)) & 0xFFFF) >= DrawProp.pFont->Width)) 00353 { 00354 /* Display one character on LCD */ 00355 BSP_LCD_DisplayChar(refcolumn, Ypos, *pText); 00356 /* Decrement the column position by 16 */ 00357 refcolumn += DrawProp.pFont->Width; 00358 /* Point on the next character */ 00359 pText++; 00360 counter++; 00361 } 00362 } 00363 00364 /** 00365 * @brief Displays a character on the LCD. 00366 * @param Line Line where to display the character shape 00367 * This parameter can be one of the following values: 00368 * @arg 0..9: if the Current fonts is Font16x24 00369 * @arg 0..19: if the Current fonts is Font12x12 or Font8x12 00370 * @arg 0..29: if the Current fonts is Font8x8 00371 * @param pText Pointer to string to display on LCD 00372 * @retval None 00373 */ 00374 void BSP_LCD_DisplayStringAtLine(uint16_t Line, uint8_t *pText) 00375 { 00376 BSP_LCD_DisplayStringAt(0, LINE(Line),pText, LEFT_MODE); 00377 } 00378 00379 /** 00380 * @brief Reads an LCD pixel. 00381 * @param Xpos X position 00382 * @param Ypos Y position 00383 * @retval RGB pixel color 00384 */ 00385 uint16_t BSP_LCD_ReadPixel(uint16_t Xpos, uint16_t Ypos) 00386 { 00387 uint16_t ret = 0; 00388 00389 if(lcd_drv->ReadPixel != NULL) 00390 { 00391 ret = lcd_drv->ReadPixel(Xpos, Ypos); 00392 } 00393 00394 return ret; 00395 } 00396 00397 /** 00398 * @brief Draws an horizontal line. 00399 * @param Xpos X position 00400 * @param Ypos Y position 00401 * @param Length Line length 00402 * @retval None 00403 */ 00404 void BSP_LCD_DrawHLine(uint16_t Xpos, uint16_t Ypos, uint16_t Length) 00405 { 00406 uint32_t index = 0; 00407 00408 if(lcd_drv->DrawHLine != NULL) 00409 { 00410 lcd_drv->DrawHLine(DrawProp.TextColor, Ypos, Xpos, Length); 00411 } 00412 else 00413 { 00414 for(index = 0; index < Length; index++) 00415 { 00416 LCD_DrawPixel((Ypos + index), Xpos, DrawProp.TextColor); 00417 } 00418 } 00419 } 00420 00421 /** 00422 * @brief Draws a vertical line. 00423 * @param Xpos X position 00424 * @param Ypos Y position 00425 * @param Length Line length 00426 * @retval None 00427 */ 00428 void BSP_LCD_DrawVLine(uint16_t Xpos, uint16_t Ypos, uint16_t Length) 00429 { 00430 uint32_t index = 0; 00431 00432 if(lcd_drv->DrawVLine != NULL) 00433 { 00434 LCD_SetDisplayWindow(Ypos, Xpos, 1, Length); 00435 lcd_drv->DrawVLine(DrawProp.TextColor, Ypos, Xpos, Length); 00436 LCD_SetDisplayWindow(0, 0, BSP_LCD_GetXSize(), BSP_LCD_GetYSize()); 00437 } 00438 else 00439 { 00440 for(index = 0; index < Length; index++) 00441 { 00442 LCD_DrawPixel(Ypos, Xpos + index, DrawProp.TextColor); 00443 } 00444 } 00445 } 00446 00447 /** 00448 * @brief Draws an uni-line (between two points). 00449 * @param X1 Point 1 X position 00450 * @param Y1 Point 1 Y position 00451 * @param X2 Point 2 X position 00452 * @param Y2 Point 2 Y position 00453 * @retval None 00454 */ 00455 void BSP_LCD_DrawLine(uint16_t X1, uint16_t Y1, uint16_t X2, uint16_t Y2) 00456 { 00457 int16_t deltax = 0, deltay = 0, x = 0, y = 0, xinc1 = 0, xinc2 = 0, 00458 yinc1 = 0, yinc2 = 0, den = 0, num = 0, numadd = 0, numpixels = 0, 00459 curpixel = 0; 00460 00461 deltax = ABS(Y2 - Y1); /* The difference between the x's */ 00462 deltay = ABS(X2 - X1); /* The difference between the y's */ 00463 x = Y1; /* Start x off at the first pixel */ 00464 y = X1; /* Start y off at the first pixel */ 00465 00466 if (Y2 >= Y1) /* The x-values are increasing */ 00467 { 00468 xinc1 = 1; 00469 xinc2 = 1; 00470 } 00471 else /* The x-values are decreasing */ 00472 { 00473 xinc1 = -1; 00474 xinc2 = -1; 00475 } 00476 00477 if (X2 >= X1) /* The y-values are increasing */ 00478 { 00479 yinc1 = 1; 00480 yinc2 = 1; 00481 } 00482 else /* The y-values are decreasing */ 00483 { 00484 yinc1 = -1; 00485 yinc2 = -1; 00486 } 00487 00488 if (deltax >= deltay) /* There is at least one x-value for every y-value */ 00489 { 00490 xinc1 = 0; /* Don't change the x when numerator >= denominator */ 00491 yinc2 = 0; /* Don't change the y for every iteration */ 00492 den = deltax; 00493 num = deltax / 2; 00494 numadd = deltay; 00495 numpixels = deltax; /* There are more x-values than y-values */ 00496 } 00497 else /* There is at least one y-value for every x-value */ 00498 { 00499 xinc2 = 0; /* Don't change the x for every iteration */ 00500 yinc1 = 0; /* Don't change the y when numerator >= denominator */ 00501 den = deltay; 00502 num = deltay / 2; 00503 numadd = deltax; 00504 numpixels = deltay; /* There are more y-values than x-values */ 00505 } 00506 00507 for (curpixel = 0; curpixel <= numpixels; curpixel++) 00508 { 00509 LCD_DrawPixel(x, y, DrawProp.TextColor); /* Draw the current pixel */ 00510 num += numadd; /* Increase the numerator by the top of the fraction */ 00511 if (num >= den) /* Check if numerator >= denominator */ 00512 { 00513 num -= den; /* Calculate the new numerator value */ 00514 x += xinc1; /* Change the x as appropriate */ 00515 y += yinc1; /* Change the y as appropriate */ 00516 } 00517 x += xinc2; /* Change the x as appropriate */ 00518 y += yinc2; /* Change the y as appropriate */ 00519 } 00520 } 00521 00522 /** 00523 * @brief Draws a rectangle. 00524 * @param Xpos X position 00525 * @param Ypos Y position 00526 * @param Width Rectangle width 00527 * @param Height Rectangle height 00528 * @retval None 00529 */ 00530 void BSP_LCD_DrawRect(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) 00531 { 00532 /* Draw horizontal lines */ 00533 BSP_LCD_DrawHLine(Xpos, Ypos, Width); 00534 BSP_LCD_DrawHLine(Xpos, (Ypos+ Height), Width); 00535 00536 /* Draw vertical lines */ 00537 BSP_LCD_DrawVLine(Xpos, Ypos, Height); 00538 BSP_LCD_DrawVLine((Xpos + Width), Ypos, Height); 00539 } 00540 00541 /** 00542 * @brief Draws a circle. 00543 * @param Xpos X position 00544 * @param Ypos Y position 00545 * @param Radius Circle radius 00546 * @retval None 00547 */ 00548 void BSP_LCD_DrawCircle(uint16_t Xpos, uint16_t Ypos, uint16_t Radius) 00549 { 00550 int32_t decision; /* Decision Variable */ 00551 uint32_t curx; /* Current X Value */ 00552 uint32_t cury; /* Current Y Value */ 00553 00554 decision = 3 - (Radius << 1); 00555 curx = 0; 00556 cury = Radius; 00557 00558 while (curx <= cury) 00559 { 00560 LCD_DrawPixel((Ypos + curx), (Xpos - cury), DrawProp.TextColor); 00561 00562 LCD_DrawPixel((Ypos - curx), (Xpos - cury), DrawProp.TextColor); 00563 00564 LCD_DrawPixel((Ypos + cury), (Xpos - curx), DrawProp.TextColor); 00565 00566 LCD_DrawPixel((Ypos - cury), (Xpos - curx), DrawProp.TextColor); 00567 00568 LCD_DrawPixel((Ypos + curx), (Xpos + cury), DrawProp.TextColor); 00569 00570 LCD_DrawPixel((Ypos - curx), (Xpos + cury), DrawProp.TextColor); 00571 00572 LCD_DrawPixel((Ypos + cury), (Xpos + curx), DrawProp.TextColor); 00573 00574 LCD_DrawPixel((Ypos - cury), (Xpos + curx), DrawProp.TextColor); 00575 00576 /* Initialize the font */ 00577 BSP_LCD_SetFont(&LCD_DEFAULT_FONT); 00578 00579 if (decision < 0) 00580 { 00581 decision += (curx << 2) + 6; 00582 } 00583 else 00584 { 00585 decision += ((curx - cury) << 2) + 10; 00586 cury--; 00587 } 00588 curx++; 00589 } 00590 } 00591 00592 /** 00593 * @brief Draws an poly-line (between many points). 00594 * @param pPoints Pointer to the points array 00595 * @param PointCount Number of points 00596 * @retval None 00597 */ 00598 void BSP_LCD_DrawPolygon(pPoint pPoints, uint16_t PointCount) 00599 { 00600 int16_t x = 0, y = 0; 00601 00602 if(PointCount < 2) 00603 { 00604 return; 00605 } 00606 00607 BSP_LCD_DrawLine(pPoints->X, pPoints->Y, (pPoints+PointCount-1)->X, (pPoints+PointCount-1)->Y); 00608 00609 while(--PointCount) 00610 { 00611 x = pPoints->X; 00612 y = pPoints->Y; 00613 pPoints++; 00614 BSP_LCD_DrawLine(x, y, pPoints->X, pPoints->Y); 00615 } 00616 00617 } 00618 00619 /** 00620 * @brief Draws an ellipse on LCD. 00621 * @param Xpos X position 00622 * @param Ypos Y position 00623 * @param XRadius Ellipse X radius 00624 * @param YRadius Ellipse Y radius 00625 * @retval None 00626 */ 00627 void BSP_LCD_DrawEllipse(int Xpos, int Ypos, int XRadius, int YRadius) 00628 { 00629 int x = 0, y = -XRadius, err = 2-2*YRadius, e2; 00630 float k = 0, rad1 = 0, rad2 = 0; 00631 00632 rad1 = YRadius; 00633 rad2 = XRadius; 00634 00635 k = (float)(rad2/rad1); 00636 00637 do { 00638 LCD_DrawPixel((Ypos-(uint16_t)(x/k)), (Xpos+y), DrawProp.TextColor); 00639 LCD_DrawPixel((Ypos+(uint16_t)(x/k)), (Xpos+y), DrawProp.TextColor); 00640 LCD_DrawPixel((Ypos+(uint16_t)(x/k)), (Xpos-y), DrawProp.TextColor); 00641 LCD_DrawPixel((Ypos-(uint16_t)(x/k)), (Xpos-y), DrawProp.TextColor); 00642 00643 e2 = err; 00644 if (e2 <= x) { 00645 err += ++x*2+1; 00646 if (-y == x && e2 <= y) e2 = 0; 00647 } 00648 if (e2 > y) err += ++y*2+1; 00649 } 00650 while (y <= 0); 00651 } 00652 00653 /** 00654 * @brief Draws a bitmap picture loaded in the internal Flash (32 bpp). 00655 * @param Xpos Bmp X position in the LCD 00656 * @param Ypos Bmp Y position in the LCD 00657 * @param pBmp Pointer to Bmp picture address in the internal Flash 00658 * @retval None 00659 */ 00660 void BSP_LCD_DrawBitmap(uint16_t Xpos, uint16_t Ypos, uint8_t *pBmp) 00661 { 00662 uint32_t height = 0, width = 0; 00663 00664 /* Read bitmap width */ 00665 width = *(uint16_t *) (pBmp + 18); 00666 width |= (*(uint16_t *) (pBmp + 20)) << 16; 00667 00668 /* Read bitmap height */ 00669 height = *(uint16_t *) (pBmp + 22); 00670 height |= (*(uint16_t *) (pBmp + 24)) << 16; 00671 00672 /* Remap Ypos, hx8347d works with inverted X in case of bitmap */ 00673 /* X = 0, cursor is on Bottom corner */ 00674 if(lcd_drv == &hx8347d_drv) 00675 { 00676 Ypos = BSP_LCD_GetYSize() - Ypos - height; 00677 } 00678 00679 LCD_SetDisplayWindow(Ypos, Xpos, width, height); 00680 00681 if(lcd_drv->DrawBitmap != NULL) 00682 { 00683 lcd_drv->DrawBitmap(Ypos, Xpos, pBmp); 00684 } 00685 LCD_SetDisplayWindow(0, 0, BSP_LCD_GetXSize(), BSP_LCD_GetYSize()); 00686 } 00687 00688 /** 00689 * @brief Draws a full rectangle. 00690 * @param Xpos X position 00691 * @param Ypos Y position 00692 * @param Width Rectangle width 00693 * @param Height Rectangle height 00694 * @retval None 00695 */ 00696 void BSP_LCD_FillRect(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) 00697 { 00698 BSP_LCD_SetTextColor(DrawProp.TextColor); 00699 do 00700 { 00701 BSP_LCD_DrawHLine(Xpos, Ypos++, Width); 00702 } 00703 while(Height--); 00704 } 00705 00706 /** 00707 * @brief Draws a full circle. 00708 * @param Xpos X position 00709 * @param Ypos Y position 00710 * @param Radius Circle radius 00711 * @retval None 00712 */ 00713 void BSP_LCD_FillCircle(uint16_t Xpos, uint16_t Ypos, uint16_t Radius) 00714 { 00715 int32_t decision; /* Decision Variable */ 00716 uint32_t curx; /* Current X Value */ 00717 uint32_t cury; /* Current Y Value */ 00718 00719 decision = 3 - (Radius << 1); 00720 00721 curx = 0; 00722 cury = Radius; 00723 00724 BSP_LCD_SetTextColor(DrawProp.TextColor); 00725 00726 while (curx <= cury) 00727 { 00728 if(cury > 0) 00729 { 00730 BSP_LCD_DrawVLine(Xpos + curx, Ypos - cury, 2*cury); 00731 BSP_LCD_DrawVLine(Xpos - curx, Ypos - cury, 2*cury); 00732 } 00733 00734 if(curx > 0) 00735 { 00736 BSP_LCD_DrawVLine(Xpos - cury, Ypos - curx, 2*curx); 00737 BSP_LCD_DrawVLine(Xpos + cury, Ypos - curx, 2*curx); 00738 } 00739 if (decision < 0) 00740 { 00741 decision += (curx << 2) + 6; 00742 } 00743 else 00744 { 00745 decision += ((curx - cury) << 2) + 10; 00746 cury--; 00747 } 00748 curx++; 00749 } 00750 00751 BSP_LCD_SetTextColor(DrawProp.TextColor); 00752 BSP_LCD_DrawCircle(Xpos, Ypos, Radius); 00753 } 00754 00755 /** 00756 * @brief Draws a full ellipse. 00757 * @param Xpos X position 00758 * @param Ypos Y position 00759 * @param XRadius Ellipse X radius 00760 * @param YRadius Ellipse Y radius 00761 * @retval None 00762 */ 00763 void BSP_LCD_FillEllipse(int Xpos, int Ypos, int XRadius, int YRadius) 00764 { 00765 int x = 0, y = -XRadius, err = 2-2*YRadius, e2; 00766 float k = 0, rad1 = 0, rad2 = 0; 00767 00768 rad1 = YRadius; 00769 rad2 = XRadius; 00770 00771 k = (float)(rad2/rad1); 00772 00773 do 00774 { 00775 BSP_LCD_DrawVLine((Xpos+y), (Ypos-(uint16_t)(x/k)), (2*(uint16_t)(x/k) + 1)); 00776 BSP_LCD_DrawVLine((Xpos-y), (Ypos-(uint16_t)(x/k)), (2*(uint16_t)(x/k) + 1)); 00777 00778 e2 = err; 00779 if (e2 <= x) 00780 { 00781 err += ++x*2+1; 00782 if (-y == x && e2 <= y) e2 = 0; 00783 } 00784 if (e2 > y) err += ++y*2+1; 00785 } 00786 while (y <= 0); 00787 } 00788 00789 /** 00790 * @brief Enables the display. 00791 * @retval None 00792 */ 00793 void BSP_LCD_DisplayOn(void) 00794 { 00795 lcd_drv->DisplayOn(); 00796 } 00797 00798 /** 00799 * @brief Disables the display. 00800 * @retval None 00801 */ 00802 void BSP_LCD_DisplayOff(void) 00803 { 00804 lcd_drv->DisplayOff(); 00805 } 00806 00807 /** 00808 * @} 00809 */ 00810 00811 /****************************************************************************** 00812 Static Function 00813 *******************************************************************************/ 00814 /** @addtogroup STM32303C_EVAL_LCD_Private_Functions 00815 * @{ 00816 */ 00817 00818 /** 00819 * @brief Draws a pixel on LCD. 00820 * @param Xpos X position 00821 * @param Ypos Y position 00822 * @param RGBCode Pixel color in RGB mode (5-6-5) 00823 * @retval None 00824 */ 00825 static void LCD_DrawPixel(uint16_t Xpos, uint16_t Ypos, uint16_t RGBCode) 00826 { 00827 if(lcd_drv->WritePixel != NULL) 00828 { 00829 lcd_drv->WritePixel(Xpos, Ypos, RGBCode); 00830 } 00831 } 00832 00833 /** 00834 * @brief Draws a character on LCD. 00835 * @param Xpos Line where to display the character shape 00836 * @param Ypos Start column address 00837 * @param pChar Pointer to the character data 00838 * @retval None 00839 */ 00840 static void LCD_DrawChar(uint16_t Xpos, uint16_t Ypos, const uint8_t *pChar) 00841 { 00842 uint32_t counterh = 0, counterw = 0, index = 0; 00843 uint16_t height = 0, width = 0; 00844 uint8_t offset = 0; 00845 uint8_t *pchar = NULL; 00846 uint32_t line = 0; 00847 00848 height = DrawProp.pFont->Height; 00849 width = DrawProp.pFont->Width; 00850 00851 /* Fill bitmap header*/ 00852 *(uint16_t *) (bitmap + 2) = (uint16_t)(height*width*2+OFFSET_BITMAP); 00853 *(uint16_t *) (bitmap + 4) = (uint16_t)((height*width*2+OFFSET_BITMAP)>>16); 00854 *(uint16_t *) (bitmap + 10) = OFFSET_BITMAP; 00855 *(uint16_t *) (bitmap + 18) = (uint16_t)(width); 00856 *(uint16_t *) (bitmap + 20) = (uint16_t)((width)>>16); 00857 *(uint16_t *) (bitmap + 22) = (uint16_t)(height); 00858 *(uint16_t *) (bitmap + 24) = (uint16_t)((height)>>16); 00859 00860 offset = 8 *((width + 7)/8) - width ; 00861 00862 for(counterh = 0; counterh < height; counterh++) 00863 { 00864 pchar = ((uint8_t *)pChar + (width + 7)/8 * counterh); 00865 00866 if(((width + 7)/8) == 3) 00867 { 00868 line = (pchar[0]<< 16) | (pchar[1]<< 8) | pchar[2]; 00869 } 00870 00871 if(((width + 7)/8) == 2) 00872 { 00873 line = (pchar[0]<< 8) | pchar[1]; 00874 } 00875 00876 if(((width + 7)/8) == 1) 00877 { 00878 line = pchar[0]; 00879 } 00880 00881 for (counterw = 0; counterw < width; counterw++) 00882 { 00883 /* Image in the bitmap is written from the bottom to the top */ 00884 /* Need to invert image in the bitmap */ 00885 index = (((height-counterh-1)*width)+(counterw))*2+OFFSET_BITMAP; 00886 if(line & (1 << (width- counterw + offset- 1))) 00887 { 00888 bitmap[index] = (uint8_t)DrawProp.TextColor; 00889 bitmap[index+1] = (uint8_t)(DrawProp.TextColor >> 8); 00890 } 00891 else 00892 { 00893 bitmap[index] = (uint8_t)DrawProp.BackColor; 00894 bitmap[index+1] = (uint8_t)(DrawProp.BackColor >> 8); 00895 } 00896 } 00897 } 00898 00899 BSP_LCD_DrawBitmap(Ypos, Xpos, bitmap); 00900 } 00901 00902 /** 00903 * @brief Sets display window. 00904 * @param Xpos LCD X position 00905 * @param Ypos LCD Y position 00906 * @param Width LCD window width 00907 * @param Height LCD window height 00908 * @retval None 00909 */ 00910 static void LCD_SetDisplayWindow(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) 00911 { 00912 if(lcd_drv->SetDisplayWindow != NULL) 00913 { 00914 lcd_drv->SetDisplayWindow(Xpos, Ypos, Width, Height); 00915 } 00916 } 00917 /** 00918 * @} 00919 */ 00920 00921 /** 00922 * @} 00923 */ 00924 00925 /** 00926 * @} 00927 */ 00928 00929 /** 00930 * @} 00931 */ 00932 00933 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Wed May 31 2017 10:00:44 for STM32303C_EVAL BSP User Manual by
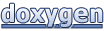