STM32303C_EVAL BSP User Manual
|
stm32303c_eval_eeprom.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32303c_eval_eeprom.c 00004 * @author MCD Application Team 00005 * @brief This file provides a set of functions needed to manage a M24LR64 00006 * or M24M01 I2C EEPROM memory, or a M95M01 SPI EEPROM memory. 00007 * 00008 * =================================================================== 00009 * Notes: 00010 * - This driver is intended for STM32F30x families devices only. 00011 * - The I2C EEPROM memory (M24LR64) is available on separate daughter 00012 * board ANT7-M24LR-A, which is provided with the STM32F303C 00013 * EVAL board. 00014 * To use this driver with M24LR64, you have to connect 00015 * the ANT7-M24LR-A to CN1 connector of STM32F303C EVAL board. 00016 * Jumper JP5 and JP6 needs to be set in I2C2 position. 00017 * Jumper JP8 (E2P WC) needs to be set. 00018 * 00019 * - The I2C EEPROM memory (M24M01) is mounted on the STM32F303C 00020 * EVAL board. 00021 * Jumper JP5 and JP6 needs to be set in I2C2_F position. 00022 * Jumper JP8 (E2P WC) needs to be set. 00023 * 00024 * - The SPI EEPROM memory (M95M01) is available directly 00025 * on STM32F303C EVAL board. 00026 * =================================================================== 00027 * 00028 * It implements a high level communication layer for read and write 00029 * from/to this memory. The needed STM32F30x hardware resources (I2C, 00030 * SPI and GPIO) are defined in stm32303c_eval.h file, 00031 * and the initialization is performed depending of EEPROMs 00032 * in EEPROM_I2C_IO_Init() or EEPROM_SPI_IO_Init() functions 00033 * declared in stm32303c_eval.c file. 00034 * You can easily tailor this driver to any other development board, 00035 * by just adapting the defines for hardware resources and 00036 * EEPROM_I2C_IO_Init() or EEPROM_SPI_IO_Init() functions. 00037 * 00038 * @note In this driver, basic read and write functions 00039 * (BSP_EEPROM_ReadBuffer() and BSP_EEPROM_WriteBuffer()) 00040 * use Polling mode to perform the data transfer to/from EEPROM memories. 00041 * +-----------------------------------------------------------------+ 00042 * | Pin assignment for M24M01 EEPROM | 00043 * +---------------------------------------+-----------+-------------+ 00044 * | STM32F30x I2C Pins | EEPROM | Pin | 00045 * +---------------------------------------+-----------+-------------+ 00046 * | . | NC | 1 | 00047 * | . | E1(VDD) | 2 (3.3V) | 00048 * | . | E2(GND) | 3 (0V) | 00049 * | . | VSS | 4 (0V) | 00050 * | EEPROM_I2C_SDA_PIN/ SDA | SDA | 5 | 00051 * | EEPROM_I2C_SCL_PIN/ SCL | SCL | 6 | 00052 * | . | /WC(VSS)| 7 (0V) | 00053 * | . | VDD | 8 (3.3V) | 00054 * +---------------------------------------+-----------+-------------+ 00055 * +-----------------------------------------------------------------+ 00056 * | Pin assignment for M24LR64 EEPROM | 00057 * +---------------------------------------+-----------+-------------+ 00058 * | STM32F30x I2C Pins | EEPROM | Pin | 00059 * +---------------------------------------+-----------+-------------+ 00060 * | . | E0(GND) | 1 (0V) | 00061 * | . | AC0 | 2 | 00062 * | . | AC1 | 3 | 00063 * | . | VSS | 4 (0V) | 00064 * | EEPROM_I2C_SDA_PIN/ SDA | SDA | 5 | 00065 * | EEPROM_I2C_SCL_PIN/ SCL | SCL | 6 | 00066 * | . | E1(GND) | 7 (0V) | 00067 * | . | VDD | 8 (3.3V) | 00068 * +---------------------------------------+-----------+-------------+ 00069 * 00070 * +-----------------------------------------------------------------+ 00071 * | Pin assignment for M95M01 EEPROM | 00072 * +---------------------------------------+-----------+-------------+ 00073 * | STM32F30x SPI Pins | EEPROM | Pin | 00074 * +---------------------------------------+-----------+-------------+ 00075 * | EEPROM_CS_PIN | ChipSelect| 1 (/S) | 00076 * | EEPROM_SPI_MISO_PIN/ MISO | DataOut | 2 (Q) | 00077 * | EEPROM_SPI_WP_PIN | WR Protect| 3 (/W) | 00078 * | . | GND | 4 (0 V) | 00079 * | EEPROM_SPI_MOSI_PIN/ MOSI | DataIn | 5 (D) | 00080 * | EEPROM_SPI_SCK_PIN/ SCLK | Clock | 6 (C) | 00081 * | . | Hold | 7 (/HOLD)| 00082 * | . | VCC | 8 (3.3 V)| 00083 * +---------------------------------------+-----------+-------------+ 00084 ****************************************************************************** 00085 * @attention 00086 * 00087 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00088 * 00089 * Redistribution and use in source and binary forms, with or without modification, 00090 * are permitted provided that the following conditions are met: 00091 * 1. Redistributions of source code must retain the above copyright notice, 00092 * this list of conditions and the following disclaimer. 00093 * 2. Redistributions in binary form must reproduce the above copyright notice, 00094 * this list of conditions and the following disclaimer in the documentation 00095 * and/or other materials provided with the distribution. 00096 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00097 * may be used to endorse or promote products derived from this software 00098 * without specific prior written permission. 00099 * 00100 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00101 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00102 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00103 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00104 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00105 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00106 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00107 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00108 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00109 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00110 * 00111 ****************************************************************************** 00112 */ 00113 00114 /* Includes ------------------------------------------------------------------*/ 00115 #include "stm32303c_eval_eeprom.h" 00116 00117 00118 /** @addtogroup BSP 00119 * @{ 00120 */ 00121 00122 /** @addtogroup STM32303C_EVAL 00123 * @{ 00124 */ 00125 00126 /** @addtogroup STM32303C_EVAL_EEPROM 00127 * @brief This file includes the I2C EEPROM and SPI EEPROM driver 00128 * of STM32303C-EVAL board. 00129 * @{ 00130 */ 00131 00132 /** @addtogroup STM32303C_EVAL_EEPROM_Private_Variables 00133 * @{ 00134 */ 00135 __IO uint16_t EEPROMAddress = 0; 00136 __IO uint16_t EEPROMPageSize = 0; 00137 __IO uint16_t EEPROMDataRead; 00138 __IO uint8_t EEPROMDataWrite; 00139 00140 static EEPROM_DrvTypeDef *EEPROM_SelectedDevice = 0; 00141 /** 00142 * @} 00143 */ 00144 00145 /** @addtogroup STM32303C_EVAL_EEPROM_Private_Functions 00146 * @{ 00147 */ 00148 static uint32_t EEPROM_I2C_Init(void); 00149 static uint32_t EEPROM_I2C_ReadBuffer(uint8_t* pBuffer, uint16_t ReadAddr, uint32_t* NumByteToRead); 00150 static uint32_t EEPROM_I2C_WritePage(uint8_t* pBuffer, uint16_t WriteAddr, uint32_t* NumByteToWrite); 00151 static uint32_t EEPROM_I2C_WaitEepromStandbyState(void); 00152 00153 static uint32_t EEPROM_SPI_Init(void); 00154 static uint32_t EEPROM_SPI_ReadBuffer(uint8_t* pBuffer, uint16_t ReadAddr, uint32_t* NumByteToRead); 00155 static uint32_t EEPROM_SPI_WritePage(uint8_t* pBuffer, uint16_t WriteAddr, uint32_t* NumByteToWrite); 00156 static uint32_t EEPROM_SPI_WaitEepromStandbyState(void); 00157 /** 00158 * @} 00159 */ 00160 00161 /** @defgroup STM32303C_EVAL_EEPROM_Private_Types Private Types 00162 * @{ 00163 */ 00164 00165 /* EEPROM I2C driver typedef */ 00166 EEPROM_DrvTypeDef EEPROM_I2C_Drv = 00167 { 00168 EEPROM_I2C_Init, 00169 EEPROM_I2C_ReadBuffer, 00170 EEPROM_I2C_WritePage 00171 }; 00172 00173 /* EEPROM SPI driver typedef */ 00174 EEPROM_DrvTypeDef EEPROM_SPI_Drv = 00175 { 00176 EEPROM_SPI_Init, 00177 EEPROM_SPI_ReadBuffer, 00178 EEPROM_SPI_WritePage 00179 }; 00180 /** 00181 * @} 00182 */ 00183 00184 /** @addtogroup STM32303C_EVAL_EEPROM_Exported_Functions 00185 * @{ 00186 */ 00187 00188 /** 00189 * @brief Initializes peripherals used by the EEPROM device selected. 00190 * @retval EEPROM_OK (0) if operation is correctly performed, else return value 00191 * different from EEPROM_OK (0) 00192 */ 00193 uint32_t BSP_EEPROM_Init(void) 00194 { 00195 if(EEPROM_SelectedDevice->Init != 0) 00196 { 00197 return (EEPROM_SelectedDevice->Init()); 00198 } 00199 else 00200 { 00201 return EEPROM_FAIL; 00202 } 00203 } 00204 00205 /** 00206 * @brief Select the EEPROM device to communicate. 00207 * @param DeviceID Specifies the EEPROM device to be selected. 00208 * This parameter can be one of following parameters: 00209 * @arg BSP_EEPROM_M24LR64 00210 * @arg BSP_EEPROM_M24M01 00211 * @arg BSP_EEPROM_M95M01 00212 * 00213 * @retval EEPROM_OK (0) if operation is correctly performed, else return value 00214 * different from EEPROM_OK (0) 00215 */ 00216 void BSP_EEPROM_SelectDevice(uint8_t DeviceID) 00217 { 00218 switch(DeviceID) 00219 { 00220 case BSP_EEPROM_M24LR64 : 00221 EEPROM_SelectedDevice = &EEPROM_I2C_Drv; 00222 break; 00223 00224 case BSP_EEPROM_M24M01 : 00225 EEPROM_SelectedDevice = &EEPROM_I2C_Drv; 00226 break; 00227 00228 case BSP_EEPROM_M95M01 : 00229 EEPROM_SelectedDevice = &EEPROM_SPI_Drv; 00230 break; 00231 00232 default: 00233 break; 00234 } 00235 } 00236 00237 /** 00238 * @brief Reads a block of data from the EEPROM device selected. 00239 * @param pBuffer pointer to the buffer that receives the data read from 00240 * the EEPROM. 00241 * @param ReadAddr EEPROM's internal address to start reading from. 00242 * @param NumByteToRead pointer to the variable holding number of bytes to 00243 * be read from the EEPROM. 00244 * 00245 * @note The variable pointed by NumByteToRead is reset to 0 when all the 00246 * data are read from the EEPROM. Application should monitor this 00247 * variable in order know when the transfer is complete. 00248 * 00249 * @retval EEPROM_OK (0) if operation is correctly performed, else return value 00250 * different from EEPROM_OK (0) or the timeout user callback. 00251 */ 00252 uint32_t BSP_EEPROM_ReadBuffer(uint8_t* pBuffer, uint16_t ReadAddr, uint32_t* NumByteToRead) 00253 { 00254 if(EEPROM_SelectedDevice->ReadBuffer != 0) 00255 { 00256 return (EEPROM_SelectedDevice->ReadBuffer(pBuffer, ReadAddr, NumByteToRead)); 00257 } 00258 else 00259 { 00260 return EEPROM_FAIL; 00261 } 00262 } 00263 00264 /** 00265 * @brief Writes buffer of data to the EEPROM device selected. 00266 * @param pBuffer pointer to the buffer containing the data to be written 00267 * to the EEPROM. 00268 * @param WriteAddr EEPROM's internal address to write to. 00269 * @param NumByteToWrite number of bytes to write to the EEPROM. 00270 * @retval EEPROM_OK (0) if operation is correctly performed, else return value 00271 * different from EEPROM_OK (0) or the timeout user callback. 00272 */ 00273 uint32_t BSP_EEPROM_WriteBuffer(uint8_t* pBuffer, uint16_t WriteAddr, uint32_t NumByteToWrite) 00274 { 00275 uint16_t numofpage = 0, numofsingle = 0, count = 0; 00276 uint16_t addr = 0; 00277 uint32_t dataindex = 0; 00278 uint32_t status = EEPROM_OK; 00279 00280 addr = WriteAddr % EEPROMPageSize; 00281 count = EEPROMPageSize - addr; 00282 numofpage = NumByteToWrite / EEPROMPageSize; 00283 numofsingle = NumByteToWrite % EEPROMPageSize; 00284 00285 if(EEPROM_SelectedDevice->WritePage == 0) 00286 { 00287 return EEPROM_FAIL; 00288 } 00289 00290 /*!< If WriteAddr is EEPROM_PAGESIZE aligned */ 00291 if(addr == 0) 00292 { 00293 /*!< If NumByteToWrite < EEPROM_PAGESIZE */ 00294 if(numofpage == 0) 00295 { 00296 /* Store the number of data to be written */ 00297 dataindex = numofsingle; 00298 /* Start writing data */ 00299 status = EEPROM_SelectedDevice->WritePage(pBuffer, WriteAddr, (uint32_t*)(&dataindex)); 00300 if (status != EEPROM_OK) 00301 { 00302 return status; 00303 } 00304 } 00305 /*!< If NumByteToWrite > EEPROM_PAGESIZE */ 00306 else 00307 { 00308 while(numofpage--) 00309 { 00310 /* Store the number of data to be written */ 00311 dataindex = EEPROMPageSize; 00312 status = EEPROM_SelectedDevice->WritePage(pBuffer, WriteAddr, (uint32_t*)(&dataindex)); 00313 if (status != EEPROM_OK) 00314 { 00315 return status; 00316 } 00317 00318 WriteAddr += EEPROMPageSize; 00319 pBuffer += EEPROMPageSize; 00320 } 00321 00322 if(numofsingle!=0) 00323 { 00324 /* Store the number of data to be written */ 00325 dataindex = numofsingle; 00326 status = EEPROM_SelectedDevice->WritePage(pBuffer, WriteAddr, (uint32_t*)(&dataindex)); 00327 if (status != EEPROM_OK) 00328 { 00329 return status; 00330 } 00331 } 00332 } 00333 } 00334 /*!< If WriteAddr is not EEPROM_PAGESIZE aligned */ 00335 else 00336 { 00337 /*!< If NumByteToWrite < EEPROM_PAGESIZE */ 00338 if(numofpage== 0) 00339 { 00340 /*!< If the number of data to be written is more than the remaining space 00341 in the current page: */ 00342 if (NumByteToWrite > count) 00343 { 00344 /* Store the number of data to be written */ 00345 dataindex = count; 00346 /*!< Write the data contained in same page */ 00347 status = EEPROM_SelectedDevice->WritePage(pBuffer, WriteAddr, (uint32_t*)(&dataindex)); 00348 if (status != EEPROM_OK) 00349 { 00350 return status; 00351 } 00352 00353 /* Store the number of data to be written */ 00354 dataindex = (NumByteToWrite - count); 00355 /*!< Write the remaining data in the following page */ 00356 status = EEPROM_SelectedDevice->WritePage((uint8_t*)(pBuffer + count), (WriteAddr + count), (uint32_t*)(&dataindex)); 00357 if (status != EEPROM_OK) 00358 { 00359 return status; 00360 } 00361 } 00362 else 00363 { 00364 /* Store the number of data to be written */ 00365 dataindex = numofsingle; 00366 status = EEPROM_SelectedDevice->WritePage(pBuffer, WriteAddr, (uint32_t*)(&dataindex)); 00367 if (status != EEPROM_OK) 00368 { 00369 return status; 00370 } 00371 } 00372 } 00373 /*!< If NumByteToWrite > EEPROM_PAGESIZE */ 00374 else 00375 { 00376 NumByteToWrite -= count; 00377 numofpage = NumByteToWrite / EEPROMPageSize; 00378 numofsingle = NumByteToWrite % EEPROMPageSize; 00379 00380 if(count != 0) 00381 { 00382 /* Store the number of data to be written */ 00383 dataindex = count; 00384 status = EEPROM_SelectedDevice->WritePage(pBuffer, WriteAddr, (uint32_t*)(&dataindex)); 00385 if (status != EEPROM_OK) 00386 { 00387 return status; 00388 } 00389 WriteAddr += count; 00390 pBuffer += count; 00391 } 00392 00393 while(numofpage--) 00394 { 00395 /* Store the number of data to be written */ 00396 dataindex = EEPROMPageSize; 00397 status = EEPROM_SelectedDevice->WritePage(pBuffer, WriteAddr, (uint32_t*)(&dataindex)); 00398 if (status != EEPROM_OK) 00399 { 00400 return status; 00401 } 00402 WriteAddr += EEPROMPageSize; 00403 pBuffer += EEPROMPageSize; 00404 } 00405 if(numofsingle != 0) 00406 { 00407 /* Store the number of data to be written */ 00408 dataindex = numofsingle; 00409 status = EEPROM_SelectedDevice->WritePage(pBuffer, WriteAddr, (uint32_t*)(&dataindex)); 00410 if (status != EEPROM_OK) 00411 { 00412 return status; 00413 } 00414 } 00415 } 00416 } 00417 00418 /* If all operations OK, return EEPROM_OK (0) */ 00419 return EEPROM_OK; 00420 } 00421 00422 /** 00423 * @brief Basic management of the timeout situation. 00424 * @retval None. 00425 */ 00426 __weak void BSP_EEPROM_TIMEOUT_UserCallback(void) 00427 { 00428 } 00429 /** 00430 * @} 00431 */ 00432 00433 /** @addtogroup STM32303C_EVAL_EEPROM_Private_Functions 00434 * @{ 00435 */ 00436 00437 /** 00438 * @brief Initializes peripherals used by the I2C EEPROM driver. 00439 * @note There are 2 different versions of M24LR64 (A01 & A02). 00440 * Then try to connect on 1st one (EEPROM_I2C_ADDRESS_A01) 00441 * and if problem, check the 2nd one (EEPROM_I2C_ADDRESS_A02) 00442 * @retval EEPROM_OK (0) if operation is correctly performed, else return value 00443 * different from EEPROM_OK (0) 00444 */ 00445 static uint32_t EEPROM_I2C_Init(void) 00446 { 00447 EEPROM_I2C_IO_Init(); 00448 00449 /*Select the EEPROM address for M24LR64 A02 and check if OK*/ 00450 EEPROMAddress = EEPROM_ADDRESS_M24LR64_A01; 00451 EEPROMPageSize = EEPROM_PAGESIZE_M24LR64; 00452 if (EEPROM_I2C_IO_IsDeviceReady(EEPROMAddress, EEPROM_MAX_TRIALS) != HAL_OK) 00453 { 00454 /*Select the EEPROM address for M24LR64 A01 and check if OK*/ 00455 EEPROMAddress = EEPROM_ADDRESS_M24LR64_A02; 00456 EEPROMPageSize = EEPROM_PAGESIZE_M24LR64; 00457 if (EEPROM_I2C_IO_IsDeviceReady(EEPROMAddress, EEPROM_MAX_TRIALS) != HAL_OK) 00458 { 00459 /*Select the EEPROM address for M24M01 and check if OK*/ 00460 EEPROMAddress = EEPROM_ADDRESS_M24M01; 00461 EEPROMPageSize = EEPROM_PAGESIZE_M24M01; 00462 if (EEPROM_I2C_IO_IsDeviceReady(EEPROMAddress, EEPROM_MAX_TRIALS) != HAL_OK) 00463 { 00464 return EEPROM_FAIL; 00465 } 00466 } 00467 } 00468 00469 return EEPROM_OK; 00470 } 00471 00472 /** 00473 * @brief Reads a block of data from the I2C EEPROM. 00474 * @param pBuffer pointer to the buffer that receives the data read from 00475 * the EEPROM. 00476 * @param ReadAddr EEPROM's internal address to start reading from. 00477 * @param NumByteToRead pointer to the variable holding number of bytes to 00478 * be read from the EEPROM. 00479 * 00480 * @retval EEPROM_OK (0) if operation is correctly performed, else return value 00481 * different from EEPROM_OK (0) or the timeout user callback. 00482 */ 00483 static uint32_t EEPROM_I2C_ReadBuffer(uint8_t* pBuffer, uint16_t ReadAddr, uint32_t* NumByteToRead) 00484 { 00485 uint32_t buffersize = *NumByteToRead; 00486 00487 if (EEPROM_I2C_IO_ReadData(EEPROMAddress, ReadAddr, pBuffer, buffersize) != HAL_OK) 00488 { 00489 return EEPROM_FAIL; 00490 } 00491 00492 /* If all operations OK, return EEPROM_OK (0) */ 00493 return EEPROM_OK; 00494 } 00495 00496 /** 00497 * @brief Writes more than one byte to the EEPROM with a single WRITE cycle. 00498 * 00499 * @note The number of bytes (combined to write start address) must not 00500 * cross the EEPROM page boundary. This function can only write into 00501 * the boundaries of an EEPROM page. 00502 * This function doesn't check on boundaries condition (in this driver 00503 * the function BSP_EEPROM_WriteBuffer() which calls EEPROM_WritePage() is 00504 * responsible of checking on Page boundaries). 00505 * 00506 * @param pBuffer pointer to the buffer containing the data to be written to 00507 * the EEPROM. 00508 * @param WriteAddr EEPROM's internal address to write to. 00509 * @param NumByteToWrite pointer to the variable holding number of bytes to 00510 * be written into the EEPROM. 00511 * 00512 * @note The variable pointed by NumByteToWrite is reset to 0 when all the 00513 * data are written to the EEPROM. Application should monitor this 00514 * variable in order know when the transfer is complete. 00515 * 00516 * 00517 * @retval EEPROM_OK (0) if operation is correctly performed, else return value 00518 * different from EEPROM_OK (0) or the timeout user callback. 00519 */ 00520 static uint32_t EEPROM_I2C_WritePage(uint8_t* pBuffer, uint16_t WriteAddr, uint32_t* NumByteToWrite) 00521 { 00522 uint32_t buffersize = *NumByteToWrite; 00523 00524 if (EEPROM_I2C_IO_WriteData(EEPROMAddress, WriteAddr, pBuffer, buffersize) != HAL_OK) 00525 { 00526 return EEPROM_FAIL; 00527 } 00528 00529 /* Wait for EEPROM Standby state */ 00530 if (EEPROM_I2C_WaitEepromStandbyState() != EEPROM_OK) 00531 { 00532 return EEPROM_FAIL; 00533 } 00534 00535 return EEPROM_OK; 00536 } 00537 00538 /** 00539 * @brief Wait for EEPROM I2C Standby state. 00540 * 00541 * @note This function allows to wait and check that EEPROM has finished the 00542 * last operation. It is mostly used after Write operation: after receiving 00543 * the buffer to be written, the EEPROM may need additional time to actually 00544 * perform the write operation. During this time, it doesn't answer to 00545 * I2C packets addressed to it. Once the write operation is complete 00546 * the EEPROM responds to its address. 00547 * 00548 * @retval EEPROM_OK (0) if operation is correctly performed, else return value 00549 * different from EEPROM_OK (0) or the timeout user callback. 00550 */ 00551 static uint32_t EEPROM_I2C_WaitEepromStandbyState(void) 00552 { 00553 /* Check if the maximum allowed number of trials has bee reached */ 00554 if (EEPROM_I2C_IO_IsDeviceReady(EEPROMAddress, EEPROM_MAX_TRIALS) != HAL_OK) 00555 { 00556 /* If the maximum number of trials has been reached, exit the function */ 00557 BSP_EEPROM_TIMEOUT_UserCallback(); 00558 return EEPROM_TIMEOUT; 00559 } 00560 return EEPROM_OK; 00561 } 00562 00563 /** 00564 * @brief Initializes peripherals used by the SPI EEPROM driver. 00565 * @retval EEPROM_OK (0) if operation is correctly performed, else return value 00566 * different from EEPROM_OK (0) 00567 */ 00568 static uint32_t EEPROM_SPI_Init(void) 00569 { 00570 EEPROM_SPI_IO_Init(); 00571 00572 /* Check if EEPROM SPI is ready to use */ 00573 EEPROMPageSize = EEPROM_PAGESIZE_M95M01; 00574 if(EEPROM_SPI_WaitEepromStandbyState() != HAL_OK) 00575 { 00576 return EEPROM_FAIL; 00577 } 00578 return EEPROM_OK; 00579 } 00580 00581 /** 00582 * @brief Reads a block of data from the SPI EEPROM. 00583 * @param pBuffer pointer to the buffer that receives the data read from 00584 * the EEPROM. 00585 * @param ReadAddr EEPROM's internal address to start reading from. 00586 * @param NumByteToRead pointer to the variable holding number of bytes to 00587 * be read from the EEPROM. 00588 * 00589 * @note The variable pointed by NumByteToRead is reset to 0 when all the 00590 * data are read from the EEPROM. Application should monitor this 00591 * variable in order know when the transfer is complete. 00592 * 00593 * @retval EEPROM_OK (0) if operation is correctly performed, else return value 00594 * different from EEPROM_OK (0) or the timeout user callback. 00595 */ 00596 static uint32_t EEPROM_SPI_ReadBuffer(uint8_t* pBuffer, uint16_t ReadAddr, uint32_t* NumByteToRead) 00597 { 00598 uint32_t buffersize = *NumByteToRead; 00599 EEPROM_SPI_IO_ReadData(ReadAddr, pBuffer, buffersize); 00600 00601 /* Wait for EEPROM Standby state */ 00602 if (EEPROM_SPI_WaitEepromStandbyState() != EEPROM_OK) 00603 { 00604 return EEPROM_FAIL; 00605 } 00606 00607 return EEPROM_OK; 00608 } 00609 00610 /** 00611 * @brief Writes more than one byte to the EEPROM with a single WRITE cycle. 00612 * 00613 * @note The number of bytes (combined to write start address) must not 00614 * cross the EEPROM page boundary. This function can only write into 00615 * the boundaries of an EEPROM page. 00616 * This function doesn't check on boundaries condition (in this driver 00617 * the function BSP_EEPROM_WriteBuffer() which calls EEPROM_WritePage() is 00618 * responsible of checking on Page boundaries). 00619 * 00620 * @param pBuffer pointer to the buffer containing the data to be written to 00621 * the EEPROM. 00622 * @param WriteAddr EEPROM's internal address to write to. 00623 * @param NumByteToWrite pointer to the variable holding number of bytes to 00624 * be written into the EEPROM. 00625 * 00626 * @note The variable pointed by NumByteToWrite is reset to 0 when all the 00627 * data are written to the EEPROM. Application should monitor this 00628 * variable in order know when the transfer is complete. 00629 * 00630 * 00631 * @retval EEPROM_OK (0) if operation is correctly performed, else return value 00632 * different from EEPROM_OK (0) or the timeout user callback. 00633 */ 00634 static uint32_t EEPROM_SPI_WritePage(uint8_t* pBuffer, uint16_t WriteAddr, uint32_t* NumByteToWrite) 00635 { 00636 uint32_t buffersize = *NumByteToWrite; 00637 00638 if (EEPROM_SPI_IO_WriteData(WriteAddr, pBuffer, buffersize) != HAL_OK) 00639 { 00640 return EEPROM_FAIL; 00641 } 00642 00643 /* Wait for EEPROM Standby state */ 00644 if (EEPROM_SPI_WaitEepromStandbyState() != EEPROM_OK) 00645 { 00646 return EEPROM_FAIL; 00647 } 00648 00649 return EEPROM_OK; 00650 } 00651 00652 /** 00653 * @brief Wait for EEPROM SPI Standby state. 00654 * 00655 * @note This function allows to wait and check that EEPROM has finished the 00656 * last operation. It is mostly used after Write operation. 00657 * 00658 * @retval EEPROM_OK (0) if operation is correctly performed, else return value 00659 * different from EEPROM_OK (0) or the timeout user callback. 00660 */ 00661 static uint32_t EEPROM_SPI_WaitEepromStandbyState(void) 00662 { 00663 if(EEPROM_SPI_IO_WaitEepromStandbyState() != HAL_OK) 00664 { 00665 BSP_EEPROM_TIMEOUT_UserCallback(); 00666 return EEPROM_TIMEOUT; 00667 } 00668 return EEPROM_OK; 00669 } 00670 00671 /** 00672 * @} 00673 */ 00674 00675 /** 00676 * @} 00677 */ 00678 00679 /** 00680 * @} 00681 */ 00682 00683 /** 00684 * @} 00685 */ 00686 00687 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Wed May 31 2017 10:00:44 for STM32303C_EVAL BSP User Manual by
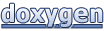