STM3210E_EVAL BSP User Manual
|
stm3210e_eval_sram.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm3210e_eval_sram.c 00004 * @author MCD Application Team 00005 * @version V6.0.2 00006 * @date 29-April-2016 00007 * @brief This file includes the SRAM driver for the IS61WV51216BLL-10M memory 00008 * device mounted on STM3210E-EVAL evaluation board. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* File Info : ----------------------------------------------------------------- 00040 User NOTES 00041 1. How To use this driver: 00042 -------------------------- 00043 - This driver is used to drive the IS61WV51216BLL-10M SRAM external memory mounted 00044 on STM3210E-EVAL evaluation board. 00045 - This driver does not need a specific component driver for the SRAM device 00046 to be included with. 00047 00048 2. Driver description: 00049 --------------------- 00050 + Initialization steps: 00051 o Initialize the SRAM external memory using the BSP_SRAM_Init() function. This 00052 function includes the MSP layer hardware resources initialization and the 00053 FSMC controller configuration to interface with the external SRAM memory. 00054 00055 + SRAM read/write operations 00056 o SRAM external memory can be accessed with read/write operations once it is 00057 initialized. 00058 Read/write operation can be performed with AHB access using the functions 00059 BSP_SRAM_ReadData()/BSP_SRAM_WriteData(), or by DMA transfer using the functions 00060 BSP_SRAM_ReadData_DMA()/BSP_SRAM_WriteData_DMA(). 00061 o The AHB access is performed with 16-bit width transaction, the DMA transfer 00062 configuration is fixed at single (no burst) halfword transfer 00063 (see the SRAM_MspInit() static function). 00064 o User can implement his own functions for read/write access with his desired 00065 configurations. 00066 o If interrupt mode is used for DMA transfer, the function BSP_SRAM_DMA_IRQHandler() 00067 is called in IRQ handler file, to serve the generated interrupt once the DMA 00068 transfer is complete. 00069 00070 ------------------------------------------------------------------------------*/ 00071 00072 /* Includes ------------------------------------------------------------------*/ 00073 #include "stm3210e_eval_sram.h" 00074 00075 /** @addtogroup BSP 00076 * @{ 00077 */ 00078 00079 /** @addtogroup STM3210E_EVAL 00080 * @{ 00081 */ 00082 00083 /** @defgroup STM3210E_EVAL_SRAM STM3210E EVAL SRAM 00084 * @{ 00085 */ 00086 00087 /* Private variables ---------------------------------------------------------*/ 00088 00089 /** @defgroup STM3210E_EVAL_SRAM_Private_Variables STM3210E EVAL SRAM Private Variables 00090 * @{ 00091 */ 00092 SRAM_HandleTypeDef sramHandle; 00093 static FSMC_NORSRAM_TimingTypeDef Timing; 00094 00095 /** 00096 * @} 00097 */ 00098 00099 /* Private function prototypes -----------------------------------------------*/ 00100 00101 /** @defgroup STM3210E_EVAL_SRAM_Private_Function_Prototypes STM3210E EVAL SRAM Private Function Prototypes 00102 * @{ 00103 */ 00104 static void SRAM_MspInit(void); 00105 00106 /** 00107 * @} 00108 */ 00109 00110 /* Private functions ---------------------------------------------------------*/ 00111 00112 /** @defgroup STM3210E_EVAL_SRAM_Exported_Functions STM3210E EVAL SRAM Exported Functions 00113 * @{ 00114 */ 00115 00116 /** 00117 * @brief Initializes the SRAM device. 00118 * @retval SRAM status 00119 */ 00120 uint8_t BSP_SRAM_Init(void) 00121 { 00122 sramHandle.Instance = FSMC_NORSRAM_DEVICE; 00123 sramHandle.Extended = FSMC_NORSRAM_EXTENDED_DEVICE; 00124 00125 /* SRAM device configuration */ 00126 Timing.AddressSetupTime = 2; 00127 Timing.AddressHoldTime = 1; 00128 Timing.DataSetupTime = 2; 00129 Timing.BusTurnAroundDuration = 1; 00130 Timing.CLKDivision = 2; 00131 Timing.DataLatency = 2; 00132 Timing.AccessMode = FSMC_ACCESS_MODE_A; 00133 00134 sramHandle.Init.NSBank = FSMC_NORSRAM_BANK3; 00135 sramHandle.Init.DataAddressMux = FSMC_DATA_ADDRESS_MUX_DISABLE; 00136 sramHandle.Init.MemoryType = FSMC_MEMORY_TYPE_SRAM; 00137 sramHandle.Init.MemoryDataWidth = SRAM_MEMORY_WIDTH; 00138 sramHandle.Init.BurstAccessMode = SRAM_BURSTACCESS; 00139 sramHandle.Init.WaitSignalPolarity = FSMC_WAIT_SIGNAL_POLARITY_LOW; 00140 sramHandle.Init.WrapMode = FSMC_WRAP_MODE_DISABLE; 00141 sramHandle.Init.WaitSignalActive = FSMC_WAIT_TIMING_BEFORE_WS; 00142 sramHandle.Init.WriteOperation = FSMC_WRITE_OPERATION_ENABLE; 00143 sramHandle.Init.WaitSignal = FSMC_WAIT_SIGNAL_DISABLE; 00144 sramHandle.Init.ExtendedMode = FSMC_EXTENDED_MODE_DISABLE; 00145 sramHandle.Init.AsynchronousWait = FSMC_ASYNCHRONOUS_WAIT_DISABLE; 00146 sramHandle.Init.WriteBurst = SRAM_WRITEBURST; 00147 00148 /* SRAM controller initialization */ 00149 BSP_SRAM_MspInit(); 00150 00151 if(HAL_SRAM_Init(&sramHandle, &Timing, &Timing) != HAL_OK) 00152 { 00153 return SRAM_ERROR; 00154 } 00155 else 00156 { 00157 return SRAM_OK; 00158 } 00159 } 00160 00161 /** 00162 * @brief Reads an amount of data from the SRAM device in polling mode. 00163 * @param uwStartAddress: Read start address 00164 * @param pData: Pointer to data to be read 00165 * @param uwDataSize: Size of read data from the memory 00166 * @retval SRAM status 00167 */ 00168 uint8_t BSP_SRAM_ReadData(uint32_t uwStartAddress, uint16_t *pData, uint32_t uwDataSize) 00169 { 00170 if(HAL_SRAM_Read_16b(&sramHandle, (uint32_t *)uwStartAddress, pData, uwDataSize) != HAL_OK) 00171 { 00172 return SRAM_ERROR; 00173 } 00174 else 00175 { 00176 return SRAM_OK; 00177 } 00178 } 00179 00180 /** 00181 * @brief Reads an amount of data from the SRAM device in DMA mode. 00182 * @param uwStartAddress: Read start address 00183 * @param pData: Pointer to data to be read 00184 * @param uwDataSize: Size of read data from the memory 00185 * @retval SRAM status 00186 */ 00187 uint8_t BSP_SRAM_ReadData_DMA(uint32_t uwStartAddress, uint16_t *pData, uint32_t uwDataSize) 00188 { 00189 if(HAL_SRAM_Read_DMA(&sramHandle, (uint32_t *)uwStartAddress, (uint32_t *)pData, uwDataSize) != HAL_OK) 00190 { 00191 return SRAM_ERROR; 00192 } 00193 else 00194 { 00195 return SRAM_OK; 00196 } 00197 } 00198 00199 /** 00200 * @brief Writes an amount of data from the SRAM device in polling mode. 00201 * @param uwStartAddress: Write start address 00202 * @param pData: Pointer to data to be written 00203 * @param uwDataSize: Size of written data from the memory 00204 * @retval SRAM status 00205 */ 00206 uint8_t BSP_SRAM_WriteData(uint32_t uwStartAddress, uint16_t *pData, uint32_t uwDataSize) 00207 { 00208 if(HAL_SRAM_Write_16b(&sramHandle, (uint32_t *)uwStartAddress, pData, uwDataSize) != HAL_OK) 00209 { 00210 return SRAM_ERROR; 00211 } 00212 else 00213 { 00214 return SRAM_OK; 00215 } 00216 } 00217 00218 /** 00219 * @brief Writes an amount of data from the SRAM device in DMA mode. 00220 * @param uwStartAddress: Write start address 00221 * @param pData: Pointer to data to be written 00222 * @param uwDataSize: Size of written data from the memory 00223 * @retval SRAM status 00224 */ 00225 uint8_t BSP_SRAM_WriteData_DMA(uint32_t uwStartAddress, uint16_t *pData, uint32_t uwDataSize) 00226 { 00227 if(HAL_SRAM_Write_DMA(&sramHandle, (uint32_t *)uwStartAddress, (uint32_t *)pData, uwDataSize) != HAL_OK) 00228 { 00229 return SRAM_ERROR; 00230 } 00231 else 00232 { 00233 return SRAM_OK; 00234 } 00235 } 00236 00237 /** 00238 * @brief Handles SRAM DMA transfer interrupt request. 00239 * @retval None 00240 */ 00241 void BSP_SRAM_DMA_IRQHandler(void) 00242 { 00243 HAL_DMA_IRQHandler(sramHandle.hdma); 00244 } 00245 00246 /** 00247 * @brief Initializes SRAM MSP. 00248 * @retval None 00249 */ 00250 __weak void BSP_SRAM_MspInit(void) 00251 { 00252 static DMA_HandleTypeDef hdma1; 00253 GPIO_InitTypeDef gpioinitstruct = {0}; 00254 /* Enable FSMC clock */ 00255 __HAL_RCC_FSMC_CLK_ENABLE(); 00256 00257 /* Enable DMA1 and DMA2 clocks */ 00258 __HAL_RCC_DMA1_CLK_ENABLE(); 00259 00260 /* Enable GPIOs clock */ 00261 __HAL_RCC_GPIOD_CLK_ENABLE(); 00262 __HAL_RCC_GPIOE_CLK_ENABLE(); 00263 __HAL_RCC_GPIOF_CLK_ENABLE(); 00264 __HAL_RCC_GPIOG_CLK_ENABLE(); 00265 00266 /* Common GPIO configuration */ 00267 gpioinitstruct.Mode = GPIO_MODE_AF_PP; 00268 gpioinitstruct.Pull = GPIO_NOPULL; 00269 gpioinitstruct.Speed = GPIO_SPEED_FREQ_HIGH; 00270 00271 /*-- GPIO Configuration ------------------------------------------------------*/ 00272 /*!< SRAM Data lines configuration */ 00273 gpioinitstruct.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_8 | GPIO_PIN_9 | 00274 GPIO_PIN_10 | GPIO_PIN_14 | GPIO_PIN_15; 00275 HAL_GPIO_Init(GPIOD, &gpioinitstruct); 00276 00277 gpioinitstruct.Pin = GPIO_PIN_7 | GPIO_PIN_8 | GPIO_PIN_9 | GPIO_PIN_10 | 00278 GPIO_PIN_11 | GPIO_PIN_12 | GPIO_PIN_13 | GPIO_PIN_14 | 00279 GPIO_PIN_15; 00280 HAL_GPIO_Init(GPIOE, &gpioinitstruct); 00281 00282 /*!< SRAM Address lines configuration */ 00283 gpioinitstruct.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_2 | GPIO_PIN_3 | 00284 GPIO_PIN_4 | GPIO_PIN_5 | GPIO_PIN_12 | GPIO_PIN_13 | 00285 GPIO_PIN_14 | GPIO_PIN_15; 00286 HAL_GPIO_Init(GPIOF, &gpioinitstruct); 00287 00288 gpioinitstruct.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_2 | GPIO_PIN_3 | 00289 GPIO_PIN_4 | GPIO_PIN_5; 00290 HAL_GPIO_Init(GPIOG, &gpioinitstruct); 00291 00292 gpioinitstruct.Pin = GPIO_PIN_11 | GPIO_PIN_12 | GPIO_PIN_13; 00293 HAL_GPIO_Init(GPIOD, &gpioinitstruct); 00294 00295 /*!< NOE and NWE configuration */ 00296 gpioinitstruct.Pin = GPIO_PIN_4 |GPIO_PIN_5; 00297 HAL_GPIO_Init(GPIOD, &gpioinitstruct); 00298 00299 /*!< NE3 configuration */ 00300 gpioinitstruct.Pin = GPIO_PIN_10 |GPIO_PIN_12; 00301 HAL_GPIO_Init(GPIOG, &gpioinitstruct); 00302 00303 /*!< NBL0, NBL1 configuration */ 00304 gpioinitstruct.Pin = GPIO_PIN_0 | GPIO_PIN_1; 00305 HAL_GPIO_Init(GPIOE, &gpioinitstruct); 00306 00307 /* Configure common DMA parameters */ 00308 hdma1.Init.Direction = DMA_MEMORY_TO_MEMORY; 00309 hdma1.Init.PeriphInc = DMA_PINC_ENABLE; 00310 hdma1.Init.MemInc = DMA_MINC_ENABLE; 00311 hdma1.Init.PeriphDataAlignment = DMA_PDATAALIGN_HALFWORD; 00312 hdma1.Init.MemDataAlignment = DMA_MDATAALIGN_HALFWORD; 00313 hdma1.Init.Mode = DMA_NORMAL; 00314 hdma1.Init.Priority = DMA_PRIORITY_HIGH; 00315 00316 hdma1.Instance = DMA1_Channel1; 00317 00318 /* Deinitialize the Stream for new transfer */ 00319 HAL_DMA_DeInit(&hdma1); 00320 00321 /* Configure the DMA Stream */ 00322 HAL_DMA_Init(&hdma1); 00323 00324 /* Associate the DMA handle to the FSMC SRAM one */ 00325 sramHandle.hdma = &hdma1; 00326 00327 /* NVIC configuration for DMA transfer complete interrupt */ 00328 HAL_NVIC_SetPriority(DMA1_Channel1_IRQn, 0xC, 0); 00329 HAL_NVIC_EnableIRQ(DMA1_Channel1_IRQn); 00330 00331 } 00332 /** 00333 * @} 00334 */ 00335 00336 /** 00337 * @} 00338 */ 00339 00340 /** 00341 * @} 00342 */ 00343 00344 /** 00345 * @} 00346 */ 00347 00348 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Fri Feb 24 2017 17:15:11 for STM3210E_EVAL BSP User Manual by
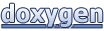