STM3210E_EVAL BSP User Manual
|
stm3210e_eval_sd.h
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm3210e_eval_sd.c 00004 * @author MCD Application Team 00005 * @version V6.0.2 00006 * @date 29-April-2016 00007 * @brief This file includes the uSD card driver. 00008 ****************************************************************************** 00009 * @attention 00010 * 00011 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00012 * 00013 * Redistribution and use in source and binary forms, with or without modification, 00014 * are permitted provided that the following conditions are met: 00015 * 1. Redistributions of source code must retain the above copyright notice, 00016 * this list of conditions and the following disclaimer. 00017 * 2. Redistributions in binary form must reproduce the above copyright notice, 00018 * this list of conditions and the following disclaimer in the documentation 00019 * and/or other materials provided with the distribution. 00020 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00021 * may be used to endorse or promote products derived from this software 00022 * without specific prior written permission. 00023 * 00024 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00025 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00026 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00027 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00028 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00029 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00030 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00031 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00032 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 * 00035 ****************************************************************************** 00036 */ 00037 00038 /* Define to prevent recursive inclusion -------------------------------------*/ 00039 #ifndef __STM3210E_EVAL_SD_H 00040 #define __STM3210E_EVAL_SD_H 00041 00042 #ifdef __cplusplus 00043 extern "C" { 00044 #endif 00045 00046 /* Includes ------------------------------------------------------------------*/ 00047 #include "stm32f1xx_hal.h" 00048 00049 /** @addtogroup BSP 00050 * @{ 00051 */ 00052 00053 /** @addtogroup STM3210E_EVAL 00054 * @{ 00055 */ 00056 00057 /** @addtogroup STM3210E_EVAL_SD 00058 * @{ 00059 */ 00060 00061 /* Exported types ------------------------------------------------------------*/ 00062 00063 /** @defgroup STM3210E_EVAL_SD_Exported_Types STM3210E EVAL SD Exported Types 00064 * @{ 00065 */ 00066 00067 /** 00068 * @brief SD Card information structure 00069 */ 00070 #define SD_CardInfo HAL_SD_CardInfoTypedef 00071 00072 /** 00073 * @brief SD status structure definition 00074 */ 00075 #define MSD_OK 0x00 00076 #define MSD_ERROR 0x01 00077 00078 /** 00079 * @} 00080 */ 00081 00082 00083 /* Exported constants --------------------------------------------------------*/ 00084 00085 /** @defgroup STM3210E_EVAL_SD_Exported_Constants STM3210E EVAL SD Exported Constants 00086 * @{ 00087 */ 00088 #define SD_DETECT_PIN GPIO_PIN_11 00089 #define SD_DETECT_GPIO_PORT GPIOF 00090 #define __SD_DETECT_GPIO_CLK_ENABLE() __HAL_RCC_GPIOF_CLK_ENABLE() 00091 #define SD_DETECT_IRQn EXTI15_10_IRQn 00092 00093 #define SD_DATATIMEOUT ((uint32_t)100000000) 00094 00095 #define SD_PRESENT ((uint8_t)0x01) 00096 #define SD_NOT_PRESENT ((uint8_t)0x00) 00097 00098 /* DMA definitions for SD DMA transfer */ 00099 #define __DMAx_TxRx_CLK_ENABLE __HAL_RCC_DMA2_CLK_ENABLE 00100 #define SD_DMAx_Tx_INSTANCE DMA2_Channel4 00101 #define SD_DMAx_Rx_INSTANCE DMA2_Channel4 00102 #define SD_DMAx_Tx_IRQn DMA2_Channel4_5_IRQn 00103 #define SD_DMAx_Rx_IRQn DMA2_Channel4_5_IRQn 00104 #define SD_DMAx_Tx_IRQHandler DMA2_Channel4_5_IRQHandler 00105 #define SD_DMAx_Rx_IRQHandler DMA2_Channel4_5_IRQHandler 00106 00107 /** 00108 * @} 00109 */ 00110 00111 00112 /* Exported functions --------------------------------------------------------*/ 00113 00114 /** @addtogroup STM3210E_EVAL_SD_Exported_Functions 00115 * @{ 00116 */ 00117 uint8_t BSP_SD_Init(void); 00118 uint8_t BSP_SD_ITConfig(void); 00119 void BSP_SD_DetectIT(void); 00120 void BSP_SD_DetectCallback(void); 00121 uint8_t BSP_SD_ReadBlocks(uint32_t *pData, uint64_t ReadAddr, uint32_t BlockSize, uint32_t NumOfBlocks); 00122 uint8_t BSP_SD_WriteBlocks(uint32_t *pData, uint64_t WriteAddr, uint32_t BlockSize, uint32_t NumOfBlocks); 00123 uint8_t BSP_SD_ReadBlocks_DMA(uint32_t *pData, uint64_t ReadAddr, uint32_t BlockSize, uint32_t NumOfBlocks); 00124 uint8_t BSP_SD_WriteBlocks_DMA(uint32_t *pData, uint64_t WriteAddr, uint32_t BlockSize, uint32_t NumOfBlocks); 00125 uint8_t BSP_SD_Erase(uint64_t StartAddr, uint64_t EndAddr); 00126 void BSP_SD_IRQHandler(void); 00127 void BSP_SD_DMA_Tx_IRQHandler(void); 00128 void BSP_SD_DMA_Rx_IRQHandler(void); 00129 HAL_SD_TransferStateTypedef BSP_SD_GetStatus(void); 00130 void BSP_SD_GetCardInfo(HAL_SD_CardInfoTypedef *CardInfo); 00131 uint8_t BSP_SD_IsDetected(void); 00132 00133 /** 00134 * @} 00135 */ 00136 00137 /** 00138 * @} 00139 */ 00140 00141 /** 00142 * @} 00143 */ 00144 00145 /** 00146 * @} 00147 */ 00148 00149 #ifdef __cplusplus 00150 } 00151 #endif 00152 00153 #endif /* __STM3210E_EVAL_SD_H */ 00154 00155 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Fri Feb 24 2017 17:15:11 for STM3210E_EVAL BSP User Manual by
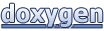