STM3210E_EVAL BSP User Manual
|
stm3210e_eval_nand.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm3210e_eval_nand.c 00004 * @author MCD Application Team 00005 * @version V6.0.2 00006 * @date 29-April-2016 00007 * @brief This file includes a standard driver for the NAND512W3A2CN6E NAND flash memory 00008 * device mounted on STM3210E-EVAL evaluation board. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* File Info : ----------------------------------------------------------------- 00040 User NOTES 00041 1. How To use this driver: 00042 -------------------------- 00043 - This driver is used to drive the NAND512W3A2CN6E NAND flash external memory mounted 00044 on STM3210E-EVAL evaluation board. 00045 - This driver does not need a specific component driver for the NAND device 00046 to be included with. 00047 00048 2. Driver description: 00049 --------------------- 00050 + Initialization steps: 00051 o Initialize the NAND external memory using the BSP_NAND_Init() function. This 00052 function includes the MSP layer hardware resources initialization and the 00053 FMC controller configuration to interface with the external NAND memory. 00054 00055 + NAND flash operations 00056 o NAND external memory can be accessed with read/write operations once it is 00057 initialized. 00058 Read/write operation can be performed with AHB access using the functions 00059 BSP_NAND_ReadData()/BSP_NAND_WriteData(). The BSP_NAND_WriteData() performs write operation 00060 of an amount of data by unit (halfword). 00061 o The function BSP_NAND_Read_ID() returns the chip IDs stored in the structure 00062 "NAND_IDTypeDef". (see the NAND IDs in the memory data sheet) 00063 o Perform erase block operation using the function BSP_NAND_Erase_Block() and by 00064 specifying the block address. 00065 00066 ------------------------------------------------------------------------------*/ 00067 00068 /* Includes ------------------------------------------------------------------*/ 00069 #include "stm3210e_eval_nand.h" 00070 00071 /** @addtogroup BSP 00072 * @{ 00073 */ 00074 00075 /** @addtogroup STM3210E_EVAL 00076 * @{ 00077 */ 00078 00079 /** @defgroup STM3210E_EVAL_NAND STM3210E EVAL NAND 00080 * @{ 00081 */ 00082 00083 /* Private variables ---------------------------------------------------------*/ 00084 00085 /** @defgroup STM3210E_EVAL_NAND_Private_Variables STM3210E EVAL NAND Private Variables 00086 * @{ 00087 */ 00088 static NAND_HandleTypeDef nandHandle; 00089 static FSMC_NAND_PCC_TimingTypeDef Timing; 00090 00091 /** 00092 * @} 00093 */ 00094 00095 /* Private function prototypes -----------------------------------------------*/ 00096 00097 /** @defgroup STM3210E_EVAL_NAND_Private_Function_Prototypes STM3210E EVAL NAND Private Function Prototypes 00098 * @{ 00099 */ 00100 static void NAND_MspInit(void); 00101 00102 /** 00103 * @} 00104 */ 00105 00106 /* Private functions ---------------------------------------------------------*/ 00107 00108 /** @defgroup STM3210E_EVAL_NAND_Exported_Functions STM3210E EVAL NAND Exported Functions 00109 * @{ 00110 */ 00111 00112 /** 00113 * @brief Initializes the NAND device. 00114 * @retval NAND memory status 00115 */ 00116 uint8_t BSP_NAND_Init(void) 00117 { 00118 nandHandle.Instance = FSMC_NAND_DEVICE; 00119 00120 /*NAND Configuration */ 00121 Timing.SetupTime = 0; 00122 Timing.WaitSetupTime = 2; 00123 Timing.HoldSetupTime = 1; 00124 Timing.HiZSetupTime = 0; 00125 00126 nandHandle.Init.NandBank = FSMC_NAND_BANK2; 00127 nandHandle.Init.Waitfeature = FSMC_NAND_PCC_WAIT_FEATURE_ENABLE; 00128 nandHandle.Init.MemoryDataWidth = FSMC_NAND_PCC_MEM_BUS_WIDTH_8; 00129 nandHandle.Init.EccComputation = FSMC_NAND_ECC_ENABLE; 00130 nandHandle.Init.ECCPageSize = FSMC_NAND_ECC_PAGE_SIZE_512BYTE; 00131 nandHandle.Init.TCLRSetupTime = 0; 00132 nandHandle.Init.TARSetupTime = 0; 00133 00134 nandHandle.Info.BlockNbr = NAND_MAX_ZONE; 00135 nandHandle.Info.BlockSize = NAND_BLOCK_SIZE; 00136 nandHandle.Info.ZoneSize = NAND_ZONE_SIZE; 00137 nandHandle.Info.PageSize = NAND_PAGE_SIZE; 00138 nandHandle.Info.SpareAreaSize = NAND_SPARE_AREA_SIZE; 00139 00140 /* NAND controller initialization */ 00141 NAND_MspInit(); 00142 00143 if(HAL_NAND_Init(&nandHandle, &Timing, &Timing) != HAL_OK) 00144 { 00145 return NAND_ERROR; 00146 } 00147 else 00148 { 00149 return NAND_OK; 00150 } 00151 } 00152 00153 /** 00154 * @brief Reads an amount of data from the NAND device. 00155 * @param BlockAddress: Block address to Read 00156 * @param pData: Pointer to data to be read 00157 * @param uwNumPage: Number of Pages to read to Block 00158 * @retval NAND memory status 00159 */ 00160 uint8_t BSP_NAND_ReadData(NAND_AddressTypeDef BlockAddress, uint8_t* pData, uint32_t uwNumPage) 00161 { 00162 /* Read data from NAND */ 00163 if (HAL_NAND_Read_Page(&nandHandle, &BlockAddress, pData, uwNumPage) != HAL_OK) 00164 { 00165 return NAND_ERROR; 00166 } 00167 00168 return NAND_OK; 00169 } 00170 00171 /** 00172 * @brief Writes an amount of data to the NAND device. 00173 * @param BlockAddress: Block address to Write 00174 * @param pData: Pointer to data to be written 00175 * @param uwNumPage: Number of Pages to write to Block 00176 * @retval NAND memory status 00177 */ 00178 uint8_t BSP_NAND_WriteData(NAND_AddressTypeDef BlockAddress, uint8_t* pData, uint32_t uwNumPage) 00179 { 00180 /* Write data to NAND */ 00181 if (HAL_NAND_Write_Page(&nandHandle, &BlockAddress, pData, uwNumPage) != HAL_OK) 00182 { 00183 return NAND_ERROR; 00184 } 00185 00186 return NAND_OK; 00187 } 00188 00189 /** 00190 * @brief Erases the specified block of the NAND device. 00191 * @param BlockAddress: Block address to Erase 00192 * @retval NAND memory status 00193 */ 00194 uint8_t BSP_NAND_Erase_Block(NAND_AddressTypeDef BlockAddress) 00195 { 00196 /* Send NAND erase block operation */ 00197 if (HAL_NAND_Erase_Block(&nandHandle, &BlockAddress) != HAL_OK) 00198 { 00199 return NAND_ERROR; 00200 } 00201 00202 return NAND_OK; 00203 } 00204 00205 /** 00206 * @brief Reads NAND flash IDs. 00207 * @param pNAND_ID : Pointer to NAND ID structure 00208 * @retval NAND memory status 00209 */ 00210 uint8_t BSP_NAND_Read_ID(NAND_IDTypeDef *pNAND_ID) 00211 { 00212 if(HAL_NAND_Read_ID(&nandHandle, pNAND_ID) != HAL_OK) 00213 { 00214 return NAND_ERROR; 00215 } 00216 else 00217 { 00218 return NAND_OK; 00219 } 00220 } 00221 00222 /** 00223 * @brief Initializes the NAND MSP. 00224 */ 00225 static void NAND_MspInit(void) 00226 { 00227 GPIO_InitTypeDef gpioinitstruct = {0}; 00228 00229 /* Enable FSMC clock */ 00230 __HAL_RCC_FSMC_CLK_ENABLE(); 00231 00232 /* Enable GPIOs clock */ 00233 __HAL_RCC_GPIOD_CLK_ENABLE(); 00234 __HAL_RCC_GPIOE_CLK_ENABLE(); 00235 __HAL_RCC_GPIOF_CLK_ENABLE(); 00236 __HAL_RCC_GPIOG_CLK_ENABLE(); 00237 00238 /* Common GPIO configuration */ 00239 gpioinitstruct.Mode = GPIO_MODE_AF_PP; 00240 gpioinitstruct.Pull = GPIO_PULLUP; 00241 gpioinitstruct.Speed = GPIO_SPEED_FREQ_HIGH; 00242 00243 /*-- GPIO Configuration ------------------------------------------------------*/ 00244 /*!< CLE, ALE, D0->D3, NOE, NWE and NCE2 NAND pin configuration */ 00245 gpioinitstruct.Pin = GPIO_PIN_11 | GPIO_PIN_12 | GPIO_PIN_14 | GPIO_PIN_15 | 00246 GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_4 | GPIO_PIN_5 | 00247 GPIO_PIN_7; 00248 HAL_GPIO_Init(GPIOD, &gpioinitstruct); 00249 00250 /*!< D4->D7 NAND pin configuration */ 00251 gpioinitstruct.Pin = GPIO_PIN_7 | GPIO_PIN_8 | GPIO_PIN_9 | GPIO_PIN_10; 00252 00253 HAL_GPIO_Init(GPIOE, &gpioinitstruct); 00254 00255 00256 /*!< NWAIT NAND pin configuration */ 00257 gpioinitstruct.Pin = GPIO_PIN_6; 00258 gpioinitstruct.Mode = GPIO_MODE_INPUT; 00259 00260 HAL_GPIO_Init(GPIOD, &gpioinitstruct); 00261 00262 /*!< INT2 NAND pin configuration */ 00263 gpioinitstruct.Pin = GPIO_PIN_6; 00264 HAL_GPIO_Init(GPIOG, &gpioinitstruct); 00265 } 00266 00267 /** 00268 * @} 00269 */ 00270 00271 /** 00272 * @} 00273 */ 00274 00275 /** 00276 * @} 00277 */ 00278 00279 /** 00280 * @} 00281 */ 00282 00283 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Fri Feb 24 2017 17:15:11 for STM3210E_EVAL BSP User Manual by
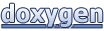