STM3210E_EVAL BSP User Manual
|
stm3210e_eval_lcd.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm3210e_eval_lcd.c 00004 * @author MCD Application Team 00005 * @version V6.0.2 00006 * @date 29-April-2016 00007 * @brief This file includes the driver for Liquid Crystal Display (LCD) module 00008 * mounted on STM3210E-EVAL evaluation board. 00009 @verbatim 00010 ============================================================================== 00011 ##### How to use this driver ##### 00012 ============================================================================== 00013 [..] 00014 (#) This driver is used to drive indirectly an LCD TFT. 00015 00016 (#) This driver supports the AM-240320L8TNQW00H (ILI9320), AM-240320LDTNQW-05H (ILI9325) 00017 AM-240320LDTNQW-02H (SPFD5408B) and AM240320LGTNQW-01H (HX8347D) LCD 00018 mounted on MB895 daughter board 00019 00020 (#) The ILI9320, ILI9325, SPFD5408B and HX8347D components driver MUST be included with this driver. 00021 00022 (#) Initialization steps: 00023 (++) Initialize the LCD using the BSP_LCD_Init() function. 00024 00025 (#) Display on LCD 00026 (++) Clear the hole LCD using yhe BSP_LCD_Clear() function or only one specified 00027 string line using the BSP_LCD_ClearStringLine() function. 00028 (++) Display a character on the specified line and column using the BSP_LCD_DisplayChar() 00029 function or a complete string line using the BSP_LCD_DisplayStringAtLine() function. 00030 (++) Display a string line on the specified position (x,y in pixel) and align mode 00031 using the BSP_LCD_DisplayStringAtLine() function. 00032 (++) Draw and fill a basic shapes (dot, line, rectangle, circle, ellipse, .. bitmap, raw picture) 00033 on LCD using a set of functions. 00034 @endverbatim 00035 ****************************************************************************** 00036 * @attention 00037 * 00038 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00039 * 00040 * Redistribution and use in source and binary forms, with or without modification, 00041 * are permitted provided that the following conditions are met: 00042 * 1. Redistributions of source code must retain the above copyright notice, 00043 * this list of conditions and the following disclaimer. 00044 * 2. Redistributions in binary form must reproduce the above copyright notice, 00045 * this list of conditions and the following disclaimer in the documentation 00046 * and/or other materials provided with the distribution. 00047 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00048 * may be used to endorse or promote products derived from this software 00049 * without specific prior written permission. 00050 * 00051 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00052 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00053 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00054 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00055 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00056 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00057 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00058 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00059 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00060 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00061 * 00062 ****************************************************************************** 00063 */ 00064 00065 /* Includes ------------------------------------------------------------------*/ 00066 #include "stm3210e_eval_lcd.h" 00067 #include "../../../Utilities/Fonts/fonts.h" 00068 #include "../../../Utilities/Fonts/font24.c" 00069 #include "../../../Utilities/Fonts/font20.c" 00070 #include "../../../Utilities/Fonts/font16.c" 00071 #include "../../../Utilities/Fonts/font12.c" 00072 #include "../../../Utilities/Fonts/font8.c" 00073 00074 /** @addtogroup BSP 00075 * @{ 00076 */ 00077 00078 /** @addtogroup STM3210E_EVAL 00079 * @{ 00080 */ 00081 00082 /** @defgroup STM3210E_EVAL_LCD STM3210E EVAL LCD 00083 * @{ 00084 */ 00085 00086 00087 /** @defgroup STM3210E_EVAL_LCD_Private_Defines STM3210E EVAL LCD Private Defines 00088 * @{ 00089 */ 00090 #define POLY_X(Z) ((int32_t)((pPoints + (Z))->X)) 00091 #define POLY_Y(Z) ((int32_t)((pPoints + (Z))->Y)) 00092 00093 #define MAX_HEIGHT_FONT 17 00094 #define MAX_WIDTH_FONT 24 00095 #define OFFSET_BITMAP 54 00096 /** 00097 * @} 00098 */ 00099 00100 /** @defgroup STM3210E_EVAL_LCD_Private_Macros STM3210E EVAL LCD Private Macros 00101 * @{ 00102 */ 00103 #define ABS(X) ((X) > 0 ? (X) : -(X)) 00104 00105 /** 00106 * @} 00107 */ 00108 00109 /** @defgroup STM3210E_EVAL_LCD_Private_Variables STM3210E EVAL LCD Private Variables 00110 * @{ 00111 */ 00112 LCD_DrawPropTypeDef DrawProp; 00113 00114 static LCD_DrvTypeDef *lcd_drv; 00115 00116 /* Max size of bitmap will based on a font24 (17x24) */ 00117 static uint8_t bitmap[MAX_HEIGHT_FONT*MAX_WIDTH_FONT*2+OFFSET_BITMAP] = {0}; 00118 00119 static uint32_t LCD_SwapXY = 0; 00120 /** 00121 * @} 00122 */ 00123 00124 /** @defgroup STM3210E_EVAL_LCD_Private_Functions STM3210E EVAL LCD Private Functions 00125 * @{ 00126 */ 00127 static void LCD_DrawPixel(uint16_t Xpos, uint16_t Ypos, uint16_t RGBCode); 00128 static void LCD_DrawChar(uint16_t Xpos, uint16_t Ypos, const uint8_t *pChar); 00129 static void LCD_SetDisplayWindow(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height); 00130 /** 00131 * @} 00132 */ 00133 00134 00135 /** @defgroup STM3210E_EVAL_LCD_Exported_Functions STM3210E EVAL LCD Exported Functions 00136 * @{ 00137 */ 00138 00139 /** 00140 * @brief Initializes the LCD. 00141 * @retval LCD state 00142 */ 00143 uint8_t BSP_LCD_Init(void) 00144 { 00145 uint8_t ret = LCD_ERROR; 00146 00147 /* Default value for draw propriety */ 00148 DrawProp.BackColor = 0xFFFF; 00149 DrawProp.pFont = &Font24; 00150 DrawProp.TextColor = 0x0000; 00151 00152 if(hx8347d_drv.ReadID() == HX8347D_ID) 00153 { 00154 lcd_drv = &hx8347d_drv; 00155 ret = LCD_OK; 00156 } 00157 else if(spfd5408_drv.ReadID() == SPFD5408_ID) 00158 { 00159 lcd_drv = &spfd5408_drv; 00160 ret = LCD_OK; 00161 } 00162 else if(ili9320_drv.ReadID() == ILI9320_ID) 00163 { 00164 lcd_drv = &ili9320_drv; 00165 LCD_SwapXY = 1; 00166 ret = LCD_OK; 00167 } 00168 00169 if(ret != LCD_ERROR) 00170 { 00171 /* LCD Init */ 00172 lcd_drv->Init(); 00173 00174 /* Initialize the font */ 00175 BSP_LCD_SetFont(&LCD_DEFAULT_FONT); 00176 } 00177 00178 #if defined(AFIO_MAPR2_FSMC_NADV_REMAP) 00179 /* Disconnect FSMC pin NADV. */ 00180 /* FSMC and I2C share the same I/O pin: NADV/SDA (PB7). Since this pin */ 00181 /* is not used by FSMC on STM32F10E_EVAL board, disconnect it internaly */ 00182 /* from FSMC to let it dedicated to I2C1 without sharing constraints. */ 00183 __HAL_AFIO_FSMCNADV_DISCONNECTED(); 00184 #else 00185 #error "Warning: FSMC pin NADV cannot be disconnected by internal remap. \ 00186 Therefore, I2C1 will have a shared pin in conflict with FSMC. \ 00187 Use macro __HAL_RCC_FSMC_CLK_ENABLE() and \ 00188 __HAL_RCC_FSMC_CLK_DISABLE() to manage the FSMC and I2C1 usage \ 00189 alternatively." 00190 #endif 00191 00192 return ret; 00193 } 00194 00195 /** 00196 * @brief Gets the LCD X size. 00197 * @retval Used LCD X size 00198 */ 00199 uint32_t BSP_LCD_GetXSize(void) 00200 { 00201 return(lcd_drv->GetLcdPixelWidth()); 00202 } 00203 00204 /** 00205 * @brief Gets the LCD Y size. 00206 * @retval Used LCD Y size 00207 */ 00208 uint32_t BSP_LCD_GetYSize(void) 00209 { 00210 return(lcd_drv->GetLcdPixelHeight()); 00211 } 00212 00213 /** 00214 * @brief Gets the LCD text color. 00215 * @retval Used text color. 00216 */ 00217 uint16_t BSP_LCD_GetTextColor(void) 00218 { 00219 return DrawProp.TextColor; 00220 } 00221 00222 /** 00223 * @brief Gets the LCD background color. 00224 * @retval Used background color 00225 */ 00226 uint16_t BSP_LCD_GetBackColor(void) 00227 { 00228 return DrawProp.BackColor; 00229 } 00230 00231 /** 00232 * @brief Sets the LCD text color. 00233 * @param Color: Text color code RGB(5-6-5) 00234 * @retval None 00235 */ 00236 void BSP_LCD_SetTextColor(uint16_t Color) 00237 { 00238 DrawProp.TextColor = Color; 00239 } 00240 00241 /** 00242 * @brief Sets the LCD background color. 00243 * @param Color: Background color code RGB(5-6-5) 00244 */ 00245 void BSP_LCD_SetBackColor(uint16_t Color) 00246 { 00247 DrawProp.BackColor = Color; 00248 } 00249 00250 /** 00251 * @brief Sets the LCD text font. 00252 * @param pFonts: Font to be used 00253 */ 00254 void BSP_LCD_SetFont(sFONT *pFonts) 00255 { 00256 DrawProp.pFont = pFonts; 00257 } 00258 00259 /** 00260 * @brief Gets the LCD text font. 00261 * @retval Used font 00262 */ 00263 sFONT *BSP_LCD_GetFont(void) 00264 { 00265 return DrawProp.pFont; 00266 } 00267 00268 /** 00269 * @brief Clears the hole LCD. 00270 * @param Color: Color of the background 00271 */ 00272 void BSP_LCD_Clear(uint16_t Color) 00273 { 00274 uint32_t counter = 0; 00275 00276 uint32_t color_backup = DrawProp.TextColor; 00277 DrawProp.TextColor = Color; 00278 00279 for(counter = 0; counter < BSP_LCD_GetYSize(); counter++) 00280 { 00281 BSP_LCD_DrawHLine(0, counter, BSP_LCD_GetXSize()); 00282 } 00283 00284 DrawProp.TextColor = color_backup; 00285 BSP_LCD_SetTextColor(DrawProp.TextColor); 00286 } 00287 00288 /** 00289 * @brief Clears the selected line. 00290 * @param Line: Line to be cleared 00291 * This parameter can be one of the following values: 00292 * @arg 0..9: if the Current fonts is Font16x24 00293 * @arg 0..19: if the Current fonts is Font12x12 or Font8x12 00294 * @arg 0..29: if the Current fonts is Font8x8 00295 */ 00296 void BSP_LCD_ClearStringLine(uint16_t Line) 00297 { 00298 uint32_t colorbackup = DrawProp.TextColor; 00299 DrawProp.TextColor = DrawProp.BackColor;; 00300 00301 /* Draw a rectangle with background color */ 00302 BSP_LCD_FillRect(0, (Line * DrawProp.pFont->Height), BSP_LCD_GetXSize(), DrawProp.pFont->Height); 00303 00304 DrawProp.TextColor = colorbackup; 00305 BSP_LCD_SetTextColor(DrawProp.TextColor); 00306 } 00307 00308 /** 00309 * @brief Displays one character. 00310 * @param Xpos: Start column address 00311 * @param Ypos: Line where to display the character shape. 00312 * @param Ascii: Character ascii code 00313 * This parameter must be a number between Min_Data = 0x20 and Max_Data = 0x7E 00314 */ 00315 void BSP_LCD_DisplayChar(uint16_t Xpos, uint16_t Ypos, uint8_t Ascii) 00316 { 00317 LCD_DrawChar(Ypos, Xpos, &DrawProp.pFont->table[(Ascii-' ') *\ 00318 DrawProp.pFont->Height * ((DrawProp.pFont->Width + 7) / 8)]); 00319 } 00320 00321 /** 00322 * @brief Displays characters on the LCD. 00323 * @param Xpos: X position (in pixel) 00324 * @param Ypos: Y position (in pixel) 00325 * @param pText: Pointer to string to display on LCD 00326 * @param Mode: Display mode 00327 * This parameter can be one of the following values: 00328 * @arg CENTER_MODE 00329 * @arg RIGHT_MODE 00330 * @arg LEFT_MODE 00331 */ 00332 void BSP_LCD_DisplayStringAt(uint16_t Xpos, uint16_t Ypos, uint8_t *pText, Line_ModeTypdef Mode) 00333 { 00334 uint16_t refcolumn = 1, counter = 0; 00335 uint32_t size = 0, ysize = 0; 00336 uint8_t *ptr = pText; 00337 00338 /* Get the text size */ 00339 while (*ptr++) size ++ ; 00340 00341 /* Characters number per line */ 00342 ysize = (BSP_LCD_GetXSize()/DrawProp.pFont->Width); 00343 00344 switch (Mode) 00345 { 00346 case CENTER_MODE: 00347 { 00348 refcolumn = Xpos + ((ysize - size)* DrawProp.pFont->Width) / 2; 00349 break; 00350 } 00351 case LEFT_MODE: 00352 { 00353 refcolumn = Xpos; 00354 break; 00355 } 00356 case RIGHT_MODE: 00357 { 00358 refcolumn = -Xpos + ((ysize - size)*DrawProp.pFont->Width); 00359 break; 00360 } 00361 default: 00362 { 00363 refcolumn = Xpos; 00364 break; 00365 } 00366 } 00367 00368 /* Send the string character by character on lCD */ 00369 while ((*pText != 0) & (((BSP_LCD_GetXSize() - (counter*DrawProp.pFont->Width)) & 0xFFFF) >= DrawProp.pFont->Width)) 00370 { 00371 /* Display one character on LCD */ 00372 BSP_LCD_DisplayChar(refcolumn, Ypos, *pText); 00373 /* Decrement the column position by 16 */ 00374 refcolumn += DrawProp.pFont->Width; 00375 /* Point on the next character */ 00376 pText++; 00377 counter++; 00378 } 00379 } 00380 00381 /** 00382 * @brief Displays a character on the LCD. 00383 * @param Line: Line where to display the character shape 00384 * This parameter can be one of the following values: 00385 * @arg 0..9: if the Current fonts is Font16x24 00386 * @arg 0..19: if the Current fonts is Font12x12 or Font8x12 00387 * @arg 0..29: if the Current fonts is Font8x8 00388 * @param pText: Pointer to string to display on LCD 00389 */ 00390 void BSP_LCD_DisplayStringAtLine(uint16_t Line, uint8_t *pText) 00391 { 00392 BSP_LCD_DisplayStringAt(0, LINE(Line),pText, LEFT_MODE); 00393 } 00394 00395 /** 00396 * @brief Reads an LCD pixel. 00397 * @param Xpos: X position 00398 * @param Ypos: Y position 00399 * @retval RGB pixel color 00400 */ 00401 uint16_t BSP_LCD_ReadPixel(uint16_t Xpos, uint16_t Ypos) 00402 { 00403 uint16_t ret = 0; 00404 00405 if(lcd_drv->ReadPixel != NULL) 00406 { 00407 ret = lcd_drv->ReadPixel(Xpos, Ypos); 00408 } 00409 00410 return ret; 00411 } 00412 00413 /** 00414 * @brief Draws an horizontal line. 00415 * @param Xpos: X position 00416 * @param Ypos: Y position 00417 * @param Length: Line length 00418 */ 00419 void BSP_LCD_DrawHLine(uint16_t Xpos, uint16_t Ypos, uint16_t Length) 00420 { 00421 uint32_t index = 0; 00422 00423 if(lcd_drv->DrawHLine != NULL) 00424 { 00425 if (LCD_SwapXY) 00426 { 00427 uint16_t tmp = Ypos; 00428 Ypos = Xpos; 00429 Xpos = tmp; 00430 } 00431 00432 lcd_drv->DrawHLine(DrawProp.TextColor, Ypos, Xpos, Length); 00433 } 00434 else 00435 { 00436 for(index = 0; index < Length; index++) 00437 { 00438 LCD_DrawPixel((Ypos + index), Xpos, DrawProp.TextColor); 00439 } 00440 } 00441 } 00442 00443 /** 00444 * @brief Draws a vertical line. 00445 * @param Xpos: X position 00446 * @param Ypos: Y position 00447 * @param Length: Line length 00448 */ 00449 void BSP_LCD_DrawVLine(uint16_t Xpos, uint16_t Ypos, uint16_t Length) 00450 { 00451 uint32_t index = 0; 00452 00453 if(lcd_drv->DrawVLine != NULL) 00454 { 00455 if (LCD_SwapXY) 00456 { 00457 uint16_t tmp = Ypos; 00458 Ypos = Xpos; 00459 Xpos = tmp; 00460 } 00461 00462 LCD_SetDisplayWindow(Ypos, Xpos, 1, Length); 00463 lcd_drv->DrawVLine(DrawProp.TextColor, Ypos, Xpos, Length); 00464 LCD_SetDisplayWindow(0, 0, BSP_LCD_GetXSize(), BSP_LCD_GetYSize()); 00465 } 00466 else 00467 { 00468 for(index = 0; index < Length; index++) 00469 { 00470 LCD_DrawPixel(Ypos, Xpos + index, DrawProp.TextColor); 00471 } 00472 } 00473 } 00474 00475 /** 00476 * @brief Draws an uni-line (between two points). 00477 * @param X1: Point 1 X position 00478 * @param Y1: Point 1 Y position 00479 * @param X2: Point 2 X position 00480 * @param Y2: Point 2 Y position 00481 */ 00482 void BSP_LCD_DrawLine(uint16_t X1, uint16_t Y1, uint16_t X2, uint16_t Y2) 00483 { 00484 int16_t deltax = 0, deltay = 0, x = 0, y = 0, xinc1 = 0, xinc2 = 0, 00485 yinc1 = 0, yinc2 = 0, den = 0, num = 0, numadd = 0, numpixels = 0, 00486 curpixel = 0; 00487 00488 deltax = ABS(Y2 - Y1); /* The difference between the x's */ 00489 deltay = ABS(X2 - X1); /* The difference between the y's */ 00490 x = Y1; /* Start x off at the first pixel */ 00491 y = X1; /* Start y off at the first pixel */ 00492 00493 if (Y2 >= Y1) /* The x-values are increasing */ 00494 { 00495 xinc1 = 1; 00496 xinc2 = 1; 00497 } 00498 else /* The x-values are decreasing */ 00499 { 00500 xinc1 = -1; 00501 xinc2 = -1; 00502 } 00503 00504 if (X2 >= X1) /* The y-values are increasing */ 00505 { 00506 yinc1 = 1; 00507 yinc2 = 1; 00508 } 00509 else /* The y-values are decreasing */ 00510 { 00511 yinc1 = -1; 00512 yinc2 = -1; 00513 } 00514 00515 if (deltax >= deltay) /* There is at least one x-value for every y-value */ 00516 { 00517 xinc1 = 0; /* Don't change the x when numerator >= denominator */ 00518 yinc2 = 0; /* Don't change the y for every iteration */ 00519 den = deltax; 00520 num = deltax / 2; 00521 numadd = deltay; 00522 numpixels = deltax; /* There are more x-values than y-values */ 00523 } 00524 else /* There is at least one y-value for every x-value */ 00525 { 00526 xinc2 = 0; /* Don't change the x for every iteration */ 00527 yinc1 = 0; /* Don't change the y when numerator >= denominator */ 00528 den = deltay; 00529 num = deltay / 2; 00530 numadd = deltax; 00531 numpixels = deltay; /* There are more y-values than x-values */ 00532 } 00533 00534 for (curpixel = 0; curpixel <= numpixels; curpixel++) 00535 { 00536 LCD_DrawPixel(x, y, DrawProp.TextColor); /* Draw the current pixel */ 00537 num += numadd; /* Increase the numerator by the top of the fraction */ 00538 if (num >= den) /* Check if numerator >= denominator */ 00539 { 00540 num -= den; /* Calculate the new numerator value */ 00541 x += xinc1; /* Change the x as appropriate */ 00542 y += yinc1; /* Change the y as appropriate */ 00543 } 00544 x += xinc2; /* Change the x as appropriate */ 00545 y += yinc2; /* Change the y as appropriate */ 00546 } 00547 } 00548 00549 /** 00550 * @brief Draws a rectangle. 00551 * @param Xpos: X position 00552 * @param Ypos: Y position 00553 * @param Width: Rectangle width 00554 * @param Height: Rectangle height 00555 */ 00556 void BSP_LCD_DrawRect(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) 00557 { 00558 /* Draw horizontal lines */ 00559 BSP_LCD_DrawHLine(Xpos, Ypos, Width); 00560 BSP_LCD_DrawHLine(Xpos, (Ypos+ Height), Width); 00561 00562 /* Draw vertical lines */ 00563 BSP_LCD_DrawVLine(Xpos, Ypos, Height); 00564 BSP_LCD_DrawVLine((Xpos + Width), Ypos, Height); 00565 } 00566 00567 /** 00568 * @brief Draws a circle. 00569 * @param Xpos: X position 00570 * @param Ypos: Y position 00571 * @param Radius: Circle radius 00572 */ 00573 void BSP_LCD_DrawCircle(uint16_t Xpos, uint16_t Ypos, uint16_t Radius) 00574 { 00575 int32_t decision; /* Decision Variable */ 00576 uint32_t curx; /* Current X Value */ 00577 uint32_t cury; /* Current Y Value */ 00578 00579 decision = 3 - (Radius << 1); 00580 curx = 0; 00581 cury = Radius; 00582 00583 while (curx <= cury) 00584 { 00585 LCD_DrawPixel((Ypos + curx), (Xpos - cury), DrawProp.TextColor); 00586 00587 LCD_DrawPixel((Ypos - curx), (Xpos - cury), DrawProp.TextColor); 00588 00589 LCD_DrawPixel((Ypos + cury), (Xpos - curx), DrawProp.TextColor); 00590 00591 LCD_DrawPixel((Ypos - cury), (Xpos - curx), DrawProp.TextColor); 00592 00593 LCD_DrawPixel((Ypos + curx), (Xpos + cury), DrawProp.TextColor); 00594 00595 LCD_DrawPixel((Ypos - curx), (Xpos + cury), DrawProp.TextColor); 00596 00597 LCD_DrawPixel((Ypos + cury), (Xpos + curx), DrawProp.TextColor); 00598 00599 LCD_DrawPixel((Ypos - cury), (Xpos + curx), DrawProp.TextColor); 00600 00601 /* Initialize the font */ 00602 BSP_LCD_SetFont(&LCD_DEFAULT_FONT); 00603 00604 if (decision < 0) 00605 { 00606 decision += (curx << 2) + 6; 00607 } 00608 else 00609 { 00610 decision += ((curx - cury) << 2) + 10; 00611 cury--; 00612 } 00613 curx++; 00614 } 00615 } 00616 00617 /** 00618 * @brief Draws an poly-line (between many points). 00619 * @param pPoints: Pointer to the points array 00620 * @param PointCount: Number of points 00621 */ 00622 void BSP_LCD_DrawPolygon(pPoint pPoints, uint16_t PointCount) 00623 { 00624 int16_t x = 0, y = 0; 00625 00626 if(PointCount < 2) 00627 { 00628 return; 00629 } 00630 00631 BSP_LCD_DrawLine(pPoints->X, pPoints->Y, (pPoints+PointCount-1)->X, (pPoints+PointCount-1)->Y); 00632 00633 while(--PointCount) 00634 { 00635 x = pPoints->X; 00636 y = pPoints->Y; 00637 pPoints++; 00638 BSP_LCD_DrawLine(x, y, pPoints->X, pPoints->Y); 00639 } 00640 00641 } 00642 00643 /** 00644 * @brief Draws an ellipse on LCD. 00645 * @param Xpos: X position 00646 * @param Ypos: Y position 00647 * @param XRadius: Ellipse X radius 00648 * @param YRadius: Ellipse Y radius 00649 */ 00650 void BSP_LCD_DrawEllipse(int Xpos, int Ypos, int XRadius, int YRadius) 00651 { 00652 int x = 0, y = -XRadius, err = 2-2*YRadius, e2; 00653 float k = 0, rad1 = 0, rad2 = 0; 00654 00655 rad1 = YRadius; 00656 rad2 = XRadius; 00657 00658 k = (float)(rad2/rad1); 00659 00660 do { 00661 LCD_DrawPixel((Ypos-(uint16_t)(x/k)), (Xpos+y), DrawProp.TextColor); 00662 LCD_DrawPixel((Ypos+(uint16_t)(x/k)), (Xpos+y), DrawProp.TextColor); 00663 LCD_DrawPixel((Ypos+(uint16_t)(x/k)), (Xpos-y), DrawProp.TextColor); 00664 LCD_DrawPixel((Ypos-(uint16_t)(x/k)), (Xpos-y), DrawProp.TextColor); 00665 00666 e2 = err; 00667 if (e2 <= x) { 00668 err += ++x*2+1; 00669 if (-y == x && e2 <= y) e2 = 0; 00670 } 00671 if (e2 > y) err += ++y*2+1; 00672 } 00673 while (y <= 0); 00674 } 00675 00676 /** 00677 * @brief Draws a bitmap picture loaded in the internal Flash (32 bpp). 00678 * @param Xpos: Bmp X position in the LCD 00679 * @param Ypos: Bmp Y position in the LCD 00680 * @param pBmp: Pointer to Bmp picture address in the internal Flash 00681 */ 00682 void BSP_LCD_DrawBitmap(uint16_t Xpos, uint16_t Ypos, uint8_t *pBmp) 00683 { 00684 uint32_t height = 0, width = 0; 00685 00686 if (LCD_SwapXY) 00687 { 00688 uint16_t tmp = Ypos; 00689 Ypos = Xpos; 00690 Xpos = tmp; 00691 } 00692 00693 /* Read bitmap width */ 00694 width = *(uint16_t *) (pBmp + 18); 00695 width |= (*(uint16_t *) (pBmp + 20)) << 16; 00696 00697 /* Read bitmap height */ 00698 height = *(uint16_t *) (pBmp + 22); 00699 height |= (*(uint16_t *) (pBmp + 24)) << 16; 00700 00701 /* Remap Ypos, hx8347d works with inverted X in case of bitmap */ 00702 /* X = 0, cursor is on Bottom corner */ 00703 if(lcd_drv == &hx8347d_drv) 00704 { 00705 Ypos = BSP_LCD_GetYSize() - Ypos - height; 00706 } 00707 00708 LCD_SetDisplayWindow(Ypos, Xpos, width, height); 00709 00710 if(lcd_drv->DrawBitmap != NULL) 00711 { 00712 lcd_drv->DrawBitmap(Ypos, Xpos, pBmp); 00713 } 00714 LCD_SetDisplayWindow(0, 0, BSP_LCD_GetXSize(), BSP_LCD_GetYSize()); 00715 } 00716 00717 /** 00718 * @brief Draws a full rectangle. 00719 * @param Xpos: X position 00720 * @param Ypos: Y position 00721 * @param Width: Rectangle width 00722 * @param Height: Rectangle height 00723 */ 00724 void BSP_LCD_FillRect(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) 00725 { 00726 BSP_LCD_SetTextColor(DrawProp.TextColor); 00727 do 00728 { 00729 BSP_LCD_DrawHLine(Xpos, Ypos++, Width); 00730 } 00731 while(Height--); 00732 } 00733 00734 /** 00735 * @brief Draws a full circle. 00736 * @param Xpos: X position 00737 * @param Ypos: Y position 00738 * @param Radius: Circle radius 00739 */ 00740 void BSP_LCD_FillCircle(uint16_t Xpos, uint16_t Ypos, uint16_t Radius) 00741 { 00742 int32_t decision; /* Decision Variable */ 00743 uint32_t curx; /* Current X Value */ 00744 uint32_t cury; /* Current Y Value */ 00745 00746 decision = 3 - (Radius << 1); 00747 00748 curx = 0; 00749 cury = Radius; 00750 00751 BSP_LCD_SetTextColor(DrawProp.TextColor); 00752 00753 while (curx <= cury) 00754 { 00755 if(cury > 0) 00756 { 00757 BSP_LCD_DrawVLine(Xpos + curx, Ypos - cury, 2*cury); 00758 BSP_LCD_DrawVLine(Xpos - curx, Ypos - cury, 2*cury); 00759 } 00760 00761 if(curx > 0) 00762 { 00763 BSP_LCD_DrawVLine(Xpos - cury, Ypos - curx, 2*curx); 00764 BSP_LCD_DrawVLine(Xpos + cury, Ypos - curx, 2*curx); 00765 } 00766 if (decision < 0) 00767 { 00768 decision += (curx << 2) + 6; 00769 } 00770 else 00771 { 00772 decision += ((curx - cury) << 2) + 10; 00773 cury--; 00774 } 00775 curx++; 00776 } 00777 00778 BSP_LCD_SetTextColor(DrawProp.TextColor); 00779 BSP_LCD_DrawCircle(Xpos, Ypos, Radius); 00780 } 00781 00782 /** 00783 * @brief Fill triangle. 00784 * @param X1: specifies the point 1 x position. 00785 * @param Y1: specifies the point 1 y position. 00786 * @param X2: specifies the point 2 x position. 00787 * @param Y2: specifies the point 2 y position. 00788 * @param X3: specifies the point 3 x position. 00789 * @param Y3: specifies the point 3 y position. 00790 */ 00791 void BSP_LCD_FillTriangle(uint16_t X1, uint16_t X2, uint16_t X3, uint16_t Y1, uint16_t Y2, uint16_t Y3) 00792 { 00793 int16_t deltax = 0, deltay = 0, x = 0, y = 0, xinc1 = 0, xinc2 = 0, 00794 yinc1 = 0, yinc2 = 0, den = 0, num = 0, numadd = 0, numpixels = 0, 00795 curpixel = 0; 00796 00797 deltax = ABS(X2 - X1); /* The difference between the x's */ 00798 deltay = ABS(Y2 - Y1); /* The difference between the y's */ 00799 x = X1; /* Start x off at the first pixel */ 00800 y = Y1; /* Start y off at the first pixel */ 00801 00802 if (X2 >= X1) /* The x-values are increasing */ 00803 { 00804 xinc1 = 1; 00805 xinc2 = 1; 00806 } 00807 else /* The x-values are decreasing */ 00808 { 00809 xinc1 = -1; 00810 xinc2 = -1; 00811 } 00812 00813 if (Y2 >= Y1) /* The y-values are increasing */ 00814 { 00815 yinc1 = 1; 00816 yinc2 = 1; 00817 } 00818 else /* The y-values are decreasing */ 00819 { 00820 yinc1 = -1; 00821 yinc2 = -1; 00822 } 00823 00824 if (deltax >= deltay) /* There is at least one x-value for every y-value */ 00825 { 00826 xinc1 = 0; /* Don't change the x when numerator >= denominator */ 00827 yinc2 = 0; /* Don't change the y for every iteration */ 00828 den = deltax; 00829 num = deltax / 2; 00830 numadd = deltay; 00831 numpixels = deltax; /* There are more x-values than y-values */ 00832 } 00833 else /* There is at least one y-value for every x-value */ 00834 { 00835 xinc2 = 0; /* Don't change the x for every iteration */ 00836 yinc1 = 0; /* Don't change the y when numerator >= denominator */ 00837 den = deltay; 00838 num = deltay / 2; 00839 numadd = deltax; 00840 numpixels = deltay; /* There are more y-values than x-values */ 00841 } 00842 00843 for (curpixel = 0; curpixel <= numpixels; curpixel++) 00844 { 00845 BSP_LCD_DrawLine(x, y, X3, Y3); 00846 00847 num += numadd; /* Increase the numerator by the top of the fraction */ 00848 if (num >= den) /* Check if numerator >= denominator */ 00849 { 00850 num -= den; /* Calculate the new numerator value */ 00851 x += xinc1; /* Change the x as appropriate */ 00852 y += yinc1; /* Change the y as appropriate */ 00853 } 00854 x += xinc2; /* Change the x as appropriate */ 00855 y += yinc2; /* Change the y as appropriate */ 00856 } 00857 } 00858 00859 /** 00860 * @brief Displays a full poly-line (between many points). 00861 * @param pPoints: pointer to the points array. 00862 * @param PointCount: Number of points. 00863 */ 00864 void BSP_LCD_FillPolygon(pPoint pPoints, uint16_t PointCount) 00865 { 00866 00867 int16_t x = 0, y = 0, x2 = 0, y2 = 0, xcenter = 0, ycenter = 0, xfirst = 0, yfirst = 0, pixelx = 0, pixely = 0, counter = 0; 00868 uint16_t imageleft = 0, imageright = 0, imagetop = 0, imagebottom = 0; 00869 00870 imageleft = imageright = pPoints->X; 00871 imagetop= imagebottom = pPoints->Y; 00872 00873 for(counter = 1; counter < PointCount; counter++) 00874 { 00875 pixelx = POLY_X(counter); 00876 if(pixelx < imageleft) 00877 { 00878 imageleft = pixelx; 00879 } 00880 if(pixelx > imageright) 00881 { 00882 imageright = pixelx; 00883 } 00884 00885 pixely = POLY_Y(counter); 00886 if(pixely < imagetop) 00887 { 00888 imagetop = pixely; 00889 } 00890 if(pixely > imagebottom) 00891 { 00892 imagebottom = pixely; 00893 } 00894 } 00895 00896 if(PointCount < 2) 00897 { 00898 return; 00899 } 00900 00901 xcenter = (imageleft + imageright)/2; 00902 ycenter = (imagebottom + imagetop)/2; 00903 00904 xfirst = pPoints->X; 00905 yfirst = pPoints->Y; 00906 00907 while(--PointCount) 00908 { 00909 x = pPoints->X; 00910 y = pPoints->Y; 00911 pPoints++; 00912 x2 = pPoints->X; 00913 y2 = pPoints->Y; 00914 00915 BSP_LCD_FillTriangle(x, x2, xcenter, y, y2, ycenter); 00916 BSP_LCD_FillTriangle(x, xcenter, x2, y, ycenter, y2); 00917 BSP_LCD_FillTriangle(xcenter, x2, x, ycenter, y2, y); 00918 } 00919 00920 BSP_LCD_FillTriangle(xfirst, x2, xcenter, yfirst, y2, ycenter); 00921 BSP_LCD_FillTriangle(xfirst, xcenter, x2, yfirst, ycenter, y2); 00922 BSP_LCD_FillTriangle(xcenter, x2, xfirst, ycenter, y2, yfirst); 00923 } 00924 00925 /** 00926 * @brief Draws a full ellipse. 00927 * @param Xpos: X position 00928 * @param Ypos: Y position 00929 * @param XRadius: Ellipse X radius 00930 * @param YRadius: Ellipse Y radius 00931 */ 00932 void BSP_LCD_FillEllipse(int Xpos, int Ypos, int XRadius, int YRadius) 00933 { 00934 int x = 0, y = -XRadius, err = 2-2*YRadius, e2; 00935 float k = 0, rad1 = 0, rad2 = 0; 00936 00937 rad1 = YRadius; 00938 rad2 = XRadius; 00939 00940 k = (float)(rad2/rad1); 00941 00942 do 00943 { 00944 BSP_LCD_DrawVLine((Xpos+y), (Ypos-(uint16_t)(x/k)), (2*(uint16_t)(x/k) + 1)); 00945 BSP_LCD_DrawVLine((Xpos-y), (Ypos-(uint16_t)(x/k)), (2*(uint16_t)(x/k) + 1)); 00946 00947 e2 = err; 00948 if (e2 <= x) 00949 { 00950 err += ++x*2+1; 00951 if (-y == x && e2 <= y) e2 = 0; 00952 } 00953 if (e2 > y) err += ++y*2+1; 00954 } 00955 while (y <= 0); 00956 } 00957 00958 /** 00959 * @brief Enables the display. 00960 */ 00961 void BSP_LCD_DisplayOn(void) 00962 { 00963 lcd_drv->DisplayOn(); 00964 } 00965 00966 /** 00967 * @brief Disables the display. 00968 */ 00969 void BSP_LCD_DisplayOff(void) 00970 { 00971 lcd_drv->DisplayOff(); 00972 } 00973 00974 /** 00975 * @} 00976 */ 00977 00978 /** @addtogroup STM3210E_EVAL_LCD_Private_Functions 00979 * @{ 00980 */ 00981 00982 /****************************************************************************** 00983 Static Function 00984 *******************************************************************************/ 00985 /** 00986 * @brief Draws a pixel on LCD. 00987 * @param Xpos: X position 00988 * @param Ypos: Y position 00989 * @param RGBCode: Pixel color in RGB mode (5-6-5) 00990 */ 00991 static void LCD_DrawPixel(uint16_t Xpos, uint16_t Ypos, uint16_t RGBCode) 00992 { 00993 if (LCD_SwapXY) 00994 { 00995 uint16_t tmp = Ypos; 00996 Ypos = Xpos; 00997 Xpos = tmp; 00998 } 00999 01000 if(lcd_drv->WritePixel != NULL) 01001 { 01002 lcd_drv->WritePixel(Xpos, Ypos, RGBCode); 01003 } 01004 } 01005 01006 /** 01007 * @brief Draws a character on LCD. 01008 * @param Xpos: Line where to display the character shape 01009 * @param Ypos: Start column address 01010 * @param pChar: Pointer to the character data 01011 */ 01012 static void LCD_DrawChar(uint16_t Xpos, uint16_t Ypos, const uint8_t *pChar) 01013 { 01014 uint32_t counterh = 0, counterw = 0, index = 0; 01015 uint16_t height = 0, width = 0; 01016 uint8_t offset = 0; 01017 uint8_t *pchar = NULL; 01018 uint32_t line = 0; 01019 01020 01021 height = DrawProp.pFont->Height; 01022 width = DrawProp.pFont->Width; 01023 01024 /* Fill bitmap header*/ 01025 *(uint16_t *) (bitmap + 2) = (uint16_t)(height*width*2+OFFSET_BITMAP); 01026 *(uint16_t *) (bitmap + 4) = (uint16_t)((height*width*2+OFFSET_BITMAP)>>16); 01027 *(uint16_t *) (bitmap + 10) = OFFSET_BITMAP; 01028 *(uint16_t *) (bitmap + 18) = (uint16_t)(width); 01029 *(uint16_t *) (bitmap + 20) = (uint16_t)((width)>>16); 01030 *(uint16_t *) (bitmap + 22) = (uint16_t)(height); 01031 *(uint16_t *) (bitmap + 24) = (uint16_t)((height)>>16); 01032 01033 offset = 8 *((width + 7)/8) - width ; 01034 01035 for(counterh = 0; counterh < height; counterh++) 01036 { 01037 pchar = ((uint8_t *)pChar + (width + 7)/8 * counterh); 01038 01039 if(((width + 7)/8) == 3) 01040 { 01041 line = (pchar[0]<< 16) | (pchar[1]<< 8) | pchar[2]; 01042 } 01043 01044 if(((width + 7)/8) == 2) 01045 { 01046 line = (pchar[0]<< 8) | pchar[1]; 01047 } 01048 01049 if(((width + 7)/8) == 1) 01050 { 01051 line = pchar[0]; 01052 } 01053 01054 for (counterw = 0; counterw < width; counterw++) 01055 { 01056 /* Image in the bitmap is written from the bottom to the top */ 01057 /* Need to invert image in the bitmap */ 01058 index = (((height-counterh-1)*width)+(counterw))*2+OFFSET_BITMAP; 01059 if(line & (1 << (width- counterw + offset- 1))) 01060 { 01061 bitmap[index] = (uint8_t)DrawProp.TextColor; 01062 bitmap[index+1] = (uint8_t)(DrawProp.TextColor >> 8); 01063 } 01064 else 01065 { 01066 bitmap[index] = (uint8_t)DrawProp.BackColor; 01067 bitmap[index+1] = (uint8_t)(DrawProp.BackColor >> 8); 01068 } 01069 } 01070 } 01071 01072 BSP_LCD_DrawBitmap(Ypos, Xpos, bitmap); 01073 } 01074 01075 /** 01076 * @brief Sets display window. 01077 * @param Xpos: LCD X position 01078 * @param Ypos: LCD Y position 01079 * @param Width: LCD window width 01080 * @param Height: LCD window height 01081 */ 01082 static void LCD_SetDisplayWindow(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) 01083 { 01084 if(lcd_drv->SetDisplayWindow != NULL) 01085 { 01086 lcd_drv->SetDisplayWindow(Xpos, Ypos, Width, Height); 01087 } 01088 } 01089 01090 /** 01091 * @} 01092 */ 01093 01094 /** 01095 * @} 01096 */ 01097 01098 /** 01099 * @} 01100 */ 01101 01102 /** 01103 * @} 01104 */ 01105 01106 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Fri Feb 24 2017 17:15:11 for STM3210E_EVAL BSP User Manual by
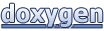