STM3210E_EVAL BSP User Manual
|
stm3210e_eval_audio.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm3210e_eval_audio.c 00004 * @author MCD Application Team 00005 * @version V6.0.2 00006 * @date 29-April-2016 00007 * @brief This file provides the Audio driver for the STM3210E-Eval 00008 * board. 00009 @verbatim 00010 ============================================================================== 00011 ##### How to use this driver ##### 00012 ============================================================================== 00013 [..] 00014 (#) This driver supports STM32F103xE devices on STM3210E-Eval Kit: 00015 (++) to play an audio file (all functions names start by BSP_AUDIO_OUT_xxx) 00016 00017 [..] 00018 (#) PLAY A FILE: 00019 (++) Call the function BSP_AUDIO_OUT_Init( 00020 OutputDevice: physical output mode (OUTPUT_DEVICE_SPEAKER, 00021 OUTPUT_DEVICE_HEADPHONE, OUTPUT_DEVICE_BOTH) 00022 Volume: initial volume to be set (0 is min (mute), 100 is max (100%) 00023 AudioFreq: Audio frequency in Hz (8000, 16000, 22500, 32000 ...) 00024 this parameter is relative to the audio file/stream type. 00025 ) 00026 This function configures all the hardware required for the audio application 00027 (codec, I2C, I2S, GPIOs, DMA and interrupt if needed). This function returns 0 00028 if configuration is OK. 00029 If the returned value is different from 0 or the function is stuck then the 00030 communication with the codec (try to un-plug the power or reset device in this case). 00031 (+++) OUTPUT_DEVICE_SPEAKER: only speaker will be set as output for the 00032 audio stream. 00033 (+++) OUTPUT_DEVICE_HEADPHONE: only headphones will be set as output for 00034 the audio stream. 00035 (+++) OUTPUT_DEVICE_BOTH: both Speaker and Headphone are used as outputs 00036 for the audio stream at the same time. 00037 (++) Call the function BSP_AUDIO_OUT_Play( 00038 pBuffer: pointer to the audio data file address 00039 Size: size of the buffer to be sent in Bytes 00040 ) 00041 to start playing (for the first time) from the audio file/stream. 00042 (++) Call the function BSP_AUDIO_OUT_Pause() to pause playing 00043 (++) Call the function BSP_AUDIO_OUT_Resume() to resume playing. 00044 Note. After calling BSP_AUDIO_OUT_Pause() function for pause, 00045 only BSP_AUDIO_OUT_Resume() should be called for resume 00046 (it is not allowed to call BSP_AUDIO_OUT_Play() in this case). 00047 Note. This function should be called only when the audio file is played 00048 or paused (not stopped). 00049 (++) For each mode, you may need to implement the relative callback functions 00050 into your code. 00051 The Callback functions are named BSP_AUDIO_OUT_XXXCallBack() and only 00052 their prototypes are declared in the stm3210e_eval_audio.h file. 00053 (refer to the example for more details on the callbacks implementations) 00054 (++) To Stop playing, to modify the volume level, the frequency or to mute, 00055 use the functions BSP_AUDIO_OUT_Stop(), BSP_AUDIO_OUT_SetVolume(), 00056 AUDIO_OUT_SetFrequency() BSP_AUDIO_OUT_SetOutputMode and BSP_AUDIO_OUT_SetMute(). 00057 (++) The driver API and the callback functions are at the end of the 00058 stm3210e_eval_audio.h file. 00059 00060 (++) This driver provide the High Audio Layer: consists of the function API 00061 exported in the stm3210e_eval_audio.h file (BSP_AUDIO_OUT_Init(), 00062 BSP_AUDIO_OUT_Play() ...) 00063 (++) This driver provide also the Media Access Layer (MAL): which consists 00064 of functions allowing to access the media containing/providing the 00065 audio file/stream. These functions are also included as local functions into 00066 the stm3210e_eval_audio.c file (I2SOUT_Init()...) 00067 00068 [..] 00069 ##### Known Limitations ##### 00070 ============================================================================== 00071 (#) When using the Speaker, if the audio file quality is not high enough, the 00072 speaker output may produce high and uncomfortable noise level. To avoid 00073 this issue, to use speaker output properly, try to increase audio file 00074 sampling rate (typically higher than 48KHz). 00075 This operation will lead to larger file size. 00076 00077 (#) Communication with the audio codec (through I2C) may be corrupted if it 00078 is interrupted by some user interrupt routines (in this case, interrupts 00079 could be disabled just before the start of communication then re-enabled 00080 when it is over). Note that this communication is only done at the 00081 configuration phase (BSP_AUDIO_OUT_Init() or BSP_AUDIO_OUT_Stop()) 00082 and when Volume control modification is performed (BSP_AUDIO_OUT_SetVolume() 00083 or BSP_AUDIO_OUT_SetMute()or BSP_AUDIO_OUT_SetOutputMode()). 00084 When the audio data is played, no communication is required with the audio codec. 00085 00086 (#) Parsing of audio file is not implemented (in order to determine audio file 00087 properties: Mono/Stereo, Data size, File size, Audio Frequency, Audio Data 00088 header size ...). The configuration is fixed for the given audio file. 00089 00090 (#) Mono audio streaming is not supported (in order to play mono audio streams, 00091 each data should be sent twice on the I2S or should be duplicated on the 00092 source buffer. Or convert the stream in stereo before playing). 00093 00094 (#) Supports only 16-bit audio data size. 00095 00096 @endverbatim 00097 ****************************************************************************** 00098 * @attention 00099 * 00100 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00101 * 00102 * Redistribution and use in source and binary forms, with or without modification, 00103 * are permitted provided that the following conditions are met: 00104 * 1. Redistributions of source code must retain the above copyright notice, 00105 * this list of conditions and the following disclaimer. 00106 * 2. Redistributions in binary form must reproduce the above copyright notice, 00107 * this list of conditions and the following disclaimer in the documentation 00108 * and/or other materials provided with the distribution. 00109 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00110 * may be used to endorse or promote products derived from this software 00111 * without specific prior written permission. 00112 * 00113 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00114 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00115 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00116 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00117 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00118 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00119 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00120 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00121 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00122 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00123 * 00124 ****************************************************************************** 00125 */ 00126 00127 /* Includes ------------------------------------------------------------------*/ 00128 #include "stm32f1xx_hal.h" /* Include HAL driver definitions to have access to I2S literals in audio driver */ 00129 #include "stm3210e_eval_audio.h" 00130 00131 /* Private variables ---------------------------------------------------------*/ 00132 00133 /** @addtogroup BSP 00134 * @{ 00135 */ 00136 00137 /** @addtogroup STM3210E_EVAL 00138 * @{ 00139 */ 00140 00141 /** @defgroup STM3210E_EVAL_AUDIO STM3210E EVAL AUDIO 00142 * @brief This file includes the low layer audio driver available on STM3210E-Eval 00143 * eval board. 00144 * @{ 00145 */ 00146 00147 /** @defgroup STM3210E_EVAL_AUDIO_Private_Macros STM3210E EVAL AUDIO Private Macros 00148 * @{ 00149 */ 00150 /** 00151 * @} 00152 */ 00153 00154 /** @defgroup STM3210E_EVAL_AUDIO_Private_Variables STM3210E EVAL AUDIO Private Variables 00155 * @{ 00156 */ 00157 #define AUDIO_SAMPLE_SENDDUMMYDATA_SIZE 8 00158 00159 const uint16_t audio_sample_SendDummyData[] = 00160 {0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000, 0x0000}; 00161 00162 static __IO uint32_t SendDummyData = DISABLE; /* Variable to switch between sending dummy data or audio data on bus I2S */ 00163 00164 00165 /*### PLAY ###*/ 00166 static AUDIO_DrvTypeDef *pAudioDrv; 00167 I2S_HandleTypeDef hAudioOutI2s; 00168 00169 /** 00170 * @} 00171 */ 00172 00173 /** @defgroup STM3210E_EVAL_AUDIO_Private_Function_Prototypes STM3210E EVAL AUDIO Private Function Prototypes 00174 * @{ 00175 */ 00176 static void I2SOUT_Init(uint32_t AudioFreq); 00177 static void I2SOUT_DeInit(void); 00178 static uint8_t I2SOUT_SendDummyData_Start(uint16_t* pBuffer, uint32_t Size); 00179 static uint8_t I2SOUT_SendDummyData_Stop(void); 00180 00181 /** 00182 * @} 00183 */ 00184 00185 /** @defgroup STM3210E_EVAL_AUDIO_OUT_Exported_Functions STM3210E EVAL AUDIO OUT Exported Functions 00186 * @{ 00187 */ 00188 00189 /** 00190 * @brief Configure the audio peripherals. 00191 * @param OutputDevice: OUTPUT_DEVICE_SPEAKER, OUTPUT_DEVICE_HEADPHONE, 00192 * OUTPUT_DEVICE_BOTH. 00193 * @param Volume: Initial volume level (from 0 (Mute) to 100 (Max)) 00194 * @param AudioFreq: Audio frequency used to play the audio stream. 00195 * @retval 0 if correct communication, else wrong communication 00196 */ 00197 uint8_t BSP_AUDIO_OUT_Init(uint16_t OutputDevice, uint8_t Volume, uint32_t AudioFreq) 00198 { 00199 uint8_t ret = AUDIO_ERROR; 00200 00201 /* Disable I2S */ 00202 I2SOUT_DeInit(); 00203 00204 /* I2S data transfer preparation: 00205 Prepare the Media to be used for the audio transfer from memory to I2S peripheral */ 00206 hAudioOutI2s.Instance = I2SOUT; 00207 if(HAL_I2S_GetState(&hAudioOutI2s) == HAL_I2S_STATE_RESET) 00208 { 00209 /* Init the I2S MSP: this __weak function can be redefined by the application*/ 00210 BSP_AUDIO_OUT_MspInit(&hAudioOutI2s, NULL); 00211 } 00212 00213 /* I2S data transfer preparation: */ 00214 /* Prepare the Media to be used for the audio transfer from memory to I2S */ 00215 /* peripheral. */ 00216 /* Note: This function must be called before audio codec init because */ 00217 /* I2S initialisation parameter "MCLKOutput" determines whether the */ 00218 /* audio Codec is driven by I2S clock (I2S_MCLKOUTPUT_ENABLE) */ 00219 /* or by its own internal PLL (I2S_MCLKOUTPUT_DISABLE). */ 00220 /* Configure the I2S peripheral */ 00221 00222 /* Switch to send dummy data on bus I2S (switch used in I2SOUT_Init() */ 00223 SendDummyData = ENABLE; 00224 00225 I2SOUT_Init(AudioFreq); 00226 00227 /* Assign value to codec driver parameter I2S_MCLKOutput in function of */ 00228 /* I2S initialisation parameter "MCLKOutput". */ 00229 if (hAudioOutI2s.Init.MCLKOutput == I2S_MCLKOUTPUT_DISABLE) 00230 { 00231 ak4343_MCLKOutput(0); 00232 } 00233 else 00234 { 00235 ak4343_MCLKOutput(1); 00236 } 00237 00238 /* Initialize the audio driver structure */ 00239 pAudioDrv = &ak4343_drv; 00240 00241 /* Generate I2S master clock MCK */ 00242 /* (Start the audio player to send dummy data on I2S bus) */ 00243 if(I2SOUT_SendDummyData_Start((uint16_t *)audio_sample_SendDummyData, AUDIO_SAMPLE_SENDDUMMYDATA_SIZE) == 0) 00244 { 00245 if (pAudioDrv->Init(AUDIO_I2C_ADDRESS, OutputDevice, Volume, AudioFreq) == 0) 00246 { 00247 ret = AUDIO_OK; 00248 } 00249 else 00250 { 00251 ret = AUDIO_ERROR; 00252 } 00253 00254 I2SOUT_SendDummyData_Stop(); 00255 00256 /* Switch to send audio data on bus I2S */ 00257 SendDummyData = DISABLE; 00258 00259 I2SOUT_Init(AudioFreq); 00260 } 00261 else 00262 { 00263 ret = AUDIO_ERROR; 00264 } 00265 00266 00267 return ret; 00268 } 00269 00270 /** 00271 * @brief De-initialize the audio peripherals. 00272 */ 00273 void BSP_AUDIO_OUT_DeInit(void) 00274 { 00275 I2SOUT_DeInit(); 00276 /* DeInit the I2S MSP : this __weak function can be rewritten by the application */ 00277 BSP_AUDIO_OUT_MspDeInit(&hAudioOutI2s, NULL); 00278 } 00279 00280 /** 00281 * @brief Starts playing audio stream from a data buffer for a determined size. 00282 * @param pBuffer: Pointer to the buffer 00283 * @param Size: Number of audio data BYTES. 00284 * @retval AUDIO_OK if correct communication, else wrong communication 00285 */ 00286 uint8_t BSP_AUDIO_OUT_Play(uint16_t* pBuffer, uint32_t Size) 00287 { 00288 /* Call the audio Codec Play function */ 00289 if(pAudioDrv->Play(AUDIO_I2C_ADDRESS, pBuffer, Size) != 0) 00290 { 00291 return AUDIO_ERROR; 00292 } 00293 else 00294 { 00295 /* Update the Media layer and enable it for play */ 00296 HAL_I2S_Transmit_DMA(&hAudioOutI2s, pBuffer, DMA_MAX(Size)); 00297 00298 return AUDIO_OK; 00299 } 00300 } 00301 00302 /** 00303 * @brief Sends n-Bytes on the I2S interface. 00304 * @param pData: pointer on data address 00305 * @param Size: number of data to be written 00306 */ 00307 void BSP_AUDIO_OUT_ChangeBuffer(uint16_t *pData, uint16_t Size) 00308 { 00309 HAL_I2S_Transmit_DMA(&hAudioOutI2s, pData, Size); 00310 } 00311 00312 /** 00313 * @brief This function Pauses the audio file stream. In case 00314 * of using DMA, the DMA Pause feature is used. 00315 * @note When calling BSP_AUDIO_OUT_Pause() function for pause, only 00316 * BSP_AUDIO_OUT_Resume() function should be called for resume (use of BSP_AUDIO_OUT_Play() 00317 * function for resume could lead to unexpected behavior). 00318 * @retval AUDIO_OK if correct communication, else wrong communication 00319 */ 00320 uint8_t BSP_AUDIO_OUT_Pause(void) 00321 { 00322 /* Call the Audio Codec Pause/Resume function */ 00323 if(pAudioDrv->Pause(AUDIO_I2C_ADDRESS) != 0) 00324 { 00325 return AUDIO_ERROR; 00326 } 00327 else 00328 { 00329 /* Call the Media layer pause function */ 00330 HAL_I2S_DMAPause(&hAudioOutI2s); 00331 00332 /* Return AUDIO_OK if all operations are OK */ 00333 return AUDIO_OK; 00334 } 00335 } 00336 00337 /** 00338 * @brief This function Resumes the audio file stream. 00339 * @note When calling BSP_AUDIO_OUT_Pause() function for pause, only 00340 * BSP_AUDIO_OUT_Resume() function should be called for resume (use of BSP_AUDIO_OUT_Play() 00341 * function for resume could lead to unexpected behavior). 00342 * @retval AUDIO_OK if correct communication, else wrong communication 00343 */ 00344 uint8_t BSP_AUDIO_OUT_Resume(void) 00345 { 00346 /* Call the Audio Codec Pause/Resume function */ 00347 if(pAudioDrv->Resume(AUDIO_I2C_ADDRESS) != 0) 00348 { 00349 return AUDIO_ERROR; 00350 } 00351 else 00352 { 00353 /* Call the Media layer resume function */ 00354 HAL_I2S_DMAResume(&hAudioOutI2s); 00355 /* Return AUDIO_OK if all operations are OK */ 00356 return AUDIO_OK; 00357 } 00358 } 00359 00360 /** 00361 * @brief Stops audio playing and Power down the Audio Codec. 00362 * @param Option: could be one of the following parameters 00363 * - CODEC_PDWN_HW: completely shut down the codec (physically). 00364 * Then need to reconfigure the Codec after power on. 00365 * @retval AUDIO_OK if correct communication, else wrong communication 00366 */ 00367 uint8_t BSP_AUDIO_OUT_Stop(uint32_t Option) 00368 { 00369 /* Call DMA Stop to disable DMA stream before stopping codec */ 00370 HAL_I2S_DMAStop(&hAudioOutI2s); 00371 00372 /* Call Audio Codec Stop function */ 00373 if(pAudioDrv->Stop(AUDIO_I2C_ADDRESS, Option) != 0) 00374 { 00375 return AUDIO_ERROR; 00376 } 00377 else 00378 { 00379 if(Option == CODEC_PDWN_HW) 00380 { 00381 /* Wait at least 100us */ 00382 HAL_Delay(1); 00383 00384 /* Power Down the codec */ 00385 HAL_GPIO_WritePin(AUDIO_RESET_GPIO, AUDIO_RESET_PIN, GPIO_PIN_RESET); 00386 00387 } 00388 /* Return AUDIO_OK when all operations are correctly done */ 00389 return AUDIO_OK; 00390 } 00391 } 00392 00393 /** 00394 * @brief Controls the current audio volume level. 00395 * @param Volume: Volume level to be set in percentage from 0% to 100% (0 for 00396 * Mute and 100 for Max volume level). 00397 * @retval AUDIO_OK if correct communication, else wrong communication 00398 */ 00399 uint8_t BSP_AUDIO_OUT_SetVolume(uint8_t Volume) 00400 { 00401 /* Call the codec volume control function with converted volume value */ 00402 if(pAudioDrv->SetVolume(AUDIO_I2C_ADDRESS, Volume) != 0) 00403 { 00404 return AUDIO_ERROR; 00405 } 00406 else 00407 { 00408 /* Return AUDIO_OK when all operations are correctly done */ 00409 return AUDIO_OK; 00410 } 00411 } 00412 00413 /** 00414 * @brief Enables or disables the MUTE mode by software 00415 * @param Cmd: could be AUDIO_MUTE_ON to mute sound or AUDIO_MUTE_OFF to 00416 * unmute the codec and restore previous volume level. 00417 * @retval AUDIO_OK if correct communication, else wrong communication 00418 */ 00419 uint8_t BSP_AUDIO_OUT_SetMute(uint32_t Cmd) 00420 { 00421 /* Call the Codec Mute function */ 00422 if(pAudioDrv->SetMute(AUDIO_I2C_ADDRESS, Cmd) != 0) 00423 { 00424 return AUDIO_ERROR; 00425 } 00426 else 00427 { 00428 /* Return AUDIO_OK when all operations are correctly done */ 00429 return AUDIO_OK; 00430 } 00431 } 00432 00433 /** 00434 * @brief Switch dynamically (while audio file is played) the output target 00435 * (speaker or headphone). 00436 * @param Output: specifies the audio output target: OUTPUT_DEVICE_SPEAKER, 00437 * OUTPUT_DEVICE_HEADPHONE, OUTPUT_DEVICE_BOTH 00438 * @retval AUDIO_OK if correct communication, else wrong communication 00439 */ 00440 uint8_t BSP_AUDIO_OUT_SetOutputMode(uint8_t Output) 00441 { 00442 /* Call the Codec output Device function */ 00443 if(pAudioDrv->SetOutputMode(AUDIO_I2C_ADDRESS, Output) != 0) 00444 { 00445 return AUDIO_ERROR; 00446 } 00447 else 00448 { 00449 /* Return AUDIO_OK when all operations are correctly done */ 00450 return AUDIO_OK; 00451 } 00452 } 00453 00454 /** 00455 * @brief Update the audio frequency. 00456 * @param AudioFreq: Audio frequency used to play the audio stream. 00457 * @retval None 00458 * @note This API should be called after the BSP_AUDIO_OUT_Init() to adjust the 00459 * audio frequency. 00460 */ 00461 void BSP_AUDIO_OUT_SetFrequency(uint32_t AudioFreq) 00462 { 00463 /* Update the I2S audio frequency configuration */ 00464 I2SOUT_Init(AudioFreq); 00465 } 00466 00467 /** 00468 * @brief Initializes BSP_AUDIO_OUT MSP. 00469 * @param hi2s: I2S handle 00470 * @param Params 00471 */ 00472 __weak void BSP_AUDIO_OUT_MspInit(I2S_HandleTypeDef *hi2s, void *Params) 00473 { 00474 static DMA_HandleTypeDef hdma_i2stx; 00475 GPIO_InitTypeDef gpioinitstruct = {0}; 00476 00477 /* Enable I2SOUT clock */ 00478 I2SOUT_CLK_ENABLE(); 00479 00480 /*** Configure the GPIOs ***/ 00481 /* Enable I2S GPIO clocks */ 00482 I2SOUT_SCK_SD_CLK_ENABLE(); 00483 I2SOUT_WS_CLK_ENABLE(); 00484 00485 /* I2SOUT pins configuration: WS, SCK and SD pins -----------------------------*/ 00486 gpioinitstruct.Pin = I2SOUT_SCK_PIN | I2SOUT_SD_PIN; 00487 gpioinitstruct.Mode = GPIO_MODE_AF_PP; 00488 gpioinitstruct.Pull = GPIO_NOPULL; 00489 gpioinitstruct.Speed = GPIO_SPEED_FREQ_MEDIUM; 00490 HAL_GPIO_Init(I2SOUT_SCK_SD_GPIO_PORT, &gpioinitstruct); 00491 00492 gpioinitstruct.Pin = I2SOUT_WS_PIN ; 00493 HAL_GPIO_Init(I2SOUT_WS_GPIO_PORT, &gpioinitstruct); 00494 00495 /* I2SOUT pins configuration: MCK pin */ 00496 I2SOUT_MCK_CLK_ENABLE(); 00497 gpioinitstruct.Pin = I2SOUT_MCK_PIN; 00498 HAL_GPIO_Init(I2SOUT_MCK_GPIO_PORT, &gpioinitstruct); 00499 00500 /* Enable the I2S DMA clock */ 00501 I2SOUT_DMAx_CLK_ENABLE(); 00502 00503 if(hi2s->Instance == I2SOUT) 00504 { 00505 /* Configure the hdma_i2stx handle parameters */ 00506 hdma_i2stx.Init.Direction = DMA_MEMORY_TO_PERIPH; 00507 hdma_i2stx.Init.PeriphInc = DMA_PINC_DISABLE; 00508 hdma_i2stx.Init.MemInc = DMA_MINC_ENABLE; 00509 hdma_i2stx.Init.PeriphDataAlignment = I2SOUT_DMAx_PERIPH_DATA_SIZE; 00510 hdma_i2stx.Init.MemDataAlignment = I2SOUT_DMAx_MEM_DATA_SIZE; 00511 /* Configuration change depending on sending dummy data or audio data on bus I2S */ 00512 if (SendDummyData != ENABLE) 00513 { 00514 hdma_i2stx.Init.Mode = DMA_NORMAL; 00515 } 00516 else 00517 { 00518 hdma_i2stx.Init.Mode = DMA_CIRCULAR; 00519 } 00520 hdma_i2stx.Init.Priority = DMA_PRIORITY_HIGH; 00521 00522 hdma_i2stx.Instance = I2SOUT_DMAx_CHANNEL; 00523 00524 /* Associate the DMA handle */ 00525 __HAL_LINKDMA(hi2s, hdmatx, hdma_i2stx); 00526 00527 /* Deinitialize the Channel for new transfer */ 00528 HAL_DMA_DeInit(&hdma_i2stx); 00529 00530 /* Configure the DMA Channel */ 00531 HAL_DMA_Init(&hdma_i2stx); 00532 } 00533 00534 /* I2S DMA IRQ Channel configuration */ 00535 HAL_NVIC_SetPriority(I2SOUT_DMAx_IRQ, AUDIO_OUT_IRQ_PREPRIO, 0); 00536 HAL_NVIC_EnableIRQ(I2SOUT_DMAx_IRQ); 00537 } 00538 00539 /** 00540 * @brief De-Initializes BSP_AUDIO_OUT MSP. 00541 * @param hi2s: I2S handle 00542 * @param Params 00543 */ 00544 __weak void BSP_AUDIO_OUT_MspDeInit(I2S_HandleTypeDef *hi2s, void *Params) 00545 { 00546 GPIO_InitTypeDef gpioinitstruct; 00547 00548 /* Disable I2S clock */ 00549 I2SOUT_CLK_DISABLE(); 00550 00551 /* CODEC_I2S pins configuration: WS, SCK and SD pins */ 00552 gpioinitstruct.Pin = I2SOUT_WS_PIN | I2SOUT_SCK_PIN | I2SOUT_SD_PIN; 00553 HAL_GPIO_DeInit(I2SOUT_SCK_SD_GPIO_PORT, gpioinitstruct.Pin); 00554 00555 /* CODEC_I2S pins configuration: MCK pin */ 00556 gpioinitstruct.Pin = I2SOUT_MCK_PIN; 00557 HAL_GPIO_DeInit(I2SOUT_MCK_GPIO_PORT, gpioinitstruct.Pin); 00558 } 00559 00560 /** 00561 * @brief Tx Transfer completed callbacks 00562 * @param hi2s: I2S handle 00563 */ 00564 void HAL_I2S_TxCpltCallback(I2S_HandleTypeDef *hi2s) 00565 { 00566 if(hi2s->Instance == I2SOUT) 00567 { 00568 /* Configuration change depending on sending dummy data or audio data on bus I2S */ 00569 if (SendDummyData != ENABLE) 00570 { 00571 /* Call the user function which will manage directly transfer complete */ 00572 BSP_AUDIO_OUT_TransferComplete_CallBack(); 00573 } 00574 else 00575 { 00576 /* No action */ 00577 } 00578 } 00579 00580 } 00581 00582 /** 00583 * @brief Tx Transfer Half completed callbacks 00584 * @param hi2s: I2S handle 00585 */ 00586 void HAL_I2S_TxHalfCpltCallback(I2S_HandleTypeDef *hi2s) 00587 { 00588 if(hi2s->Instance == I2SOUT) 00589 { 00590 /* Manage the remaining file size and new address offset: This function 00591 should be coded by user (its prototype is already declared in stm3210e_eval_audio.h) */ 00592 BSP_AUDIO_OUT_HalfTransfer_CallBack(); 00593 } 00594 } 00595 00596 /** 00597 * @brief I2S error callbacks 00598 * @param hi2s: I2S handle 00599 */ 00600 void HAL_I2S_ErrorCallback(I2S_HandleTypeDef *hi2s) 00601 { 00602 /* Manage the error generated on DMA FIFO: This function 00603 should be coded by user (its prototype is already declared in stm3210e_eval_audio.h) */ 00604 if(hi2s->Instance == I2SOUT) 00605 { 00606 BSP_AUDIO_OUT_Error_CallBack(); 00607 } 00608 } 00609 00610 /** 00611 * @brief Manages the DMA full Transfer complete event. 00612 */ 00613 __weak void BSP_AUDIO_OUT_TransferComplete_CallBack(void) 00614 { 00615 } 00616 00617 /** 00618 * @brief Manages the DMA Half Transfer complete event. 00619 */ 00620 __weak void BSP_AUDIO_OUT_HalfTransfer_CallBack(void) 00621 { 00622 } 00623 00624 /** 00625 * @brief Manages the DMA FIFO error event. 00626 */ 00627 __weak void BSP_AUDIO_OUT_Error_CallBack(void) 00628 { 00629 } 00630 00631 /****************************************************************************** 00632 Static Function 00633 *******************************************************************************/ 00634 00635 /** 00636 * @brief Initializes the Audio Codec audio interface (I2S) 00637 * @param AudioFreq: Audio frequency to be configured for the I2S peripheral. 00638 */ 00639 static void I2SOUT_Init(uint32_t AudioFreq) 00640 { 00641 /* Initialize the hAudioOutI2s Instance parameter */ 00642 hAudioOutI2s.Instance = I2SOUT; 00643 00644 /* Disable I2S block */ 00645 __HAL_I2S_DISABLE(&hAudioOutI2s); 00646 00647 /* I2SOUT peripheral configuration */ 00648 hAudioOutI2s.Init.AudioFreq = AudioFreq; 00649 hAudioOutI2s.Init.CPOL = I2S_CPOL_LOW; 00650 hAudioOutI2s.Init.DataFormat = I2S_DATAFORMAT_16B; 00651 hAudioOutI2s.Init.MCLKOutput = I2S_MCLKOUTPUT_ENABLE; 00652 hAudioOutI2s.Init.Mode = I2S_MODE_MASTER_TX; 00653 hAudioOutI2s.Init.Standard = I2S_STANDARD; 00654 00655 /* Initialize the I2S peripheral with the structure above */ 00656 HAL_I2S_Init(&hAudioOutI2s); 00657 00658 } 00659 00660 /** 00661 * @brief Deinitialize the Audio Codec audio interface (I2S). 00662 */ 00663 static void I2SOUT_DeInit(void) 00664 { 00665 /* Initialize the hAudioOutI2s Instance parameter */ 00666 hAudioOutI2s.Instance = I2SOUT; 00667 00668 /* Disable I2S peripheral */ 00669 __HAL_I2S_DISABLE(&hAudioOutI2s); 00670 00671 HAL_I2S_DeInit(&hAudioOutI2s); 00672 } 00673 00674 /** 00675 * @brief Starts sending dummy data as audio stream: function used to 00676 generate I2S master clock MCK for audio codec device. 00677 * @param pBuffer: Pointer to the buffer 00678 * @param Size: Number of audio data BYTES. 00679 * @retval AUDIO_OK if correct communication, else wrong communication 00680 */ 00681 static uint8_t I2SOUT_SendDummyData_Start(uint16_t* pBuffer, uint32_t Size) 00682 { 00683 /* Update the Media layer and enable it for play */ 00684 HAL_I2S_Transmit_DMA(&hAudioOutI2s, pBuffer, DMA_MAX(Size)); 00685 00686 return AUDIO_OK; 00687 00688 } 00689 00690 /** 00691 * @brief Stops sending dummy data as audio stream: function used to stop to 00692 generate I2S master clock MCK for audio codec device. 00693 * @retval AUDIO_OK if correct communication, else wrong communication 00694 */ 00695 static uint8_t I2SOUT_SendDummyData_Stop(void) 00696 { 00697 /* Call DMA Stop to disable DMA stream before stopping codec */ 00698 HAL_I2S_DMAStop(&hAudioOutI2s); 00699 00700 return AUDIO_OK; 00701 00702 } 00703 00704 00705 /** 00706 * @} 00707 */ 00708 00709 /** 00710 * @} 00711 */ 00712 00713 /** 00714 * @} 00715 */ 00716 00717 /** 00718 * @} 00719 */ 00720 00721 /** 00722 * @} 00723 */ 00724 00725 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Fri Feb 24 2017 17:15:11 for STM3210E_EVAL BSP User Manual by
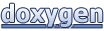