STM3210C_EVAL BSP User Manual
|
stm3210c_eval_audio.h
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm3210c_eval_audio.h 00004 * @author MCD Application Team 00005 * @version V7.0.0 00006 * @date 14-April-2017 00007 * @brief This file contains the common defines and functions prototypes for 00008 * stm3210c_eval_audio.c driver. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* Define to prevent recursive inclusion -------------------------------------*/ 00040 #ifndef __STM3210C_EVAL_AUDIO_H 00041 #define __STM3210C_EVAL_AUDIO_H 00042 00043 #ifdef __cplusplus 00044 extern "C" { 00045 #endif 00046 00047 /* Includes ------------------------------------------------------------------*/ 00048 /* Include audio component Driver */ 00049 #include "../Components/cs43l22/cs43l22.h" 00050 #include "stm3210c_eval.h" 00051 00052 /** @addtogroup BSP 00053 * @{ 00054 */ 00055 00056 /** @addtogroup STM3210C_EVAL 00057 * @{ 00058 */ 00059 00060 /** @addtogroup STM3210C_EVAL_AUDIO 00061 * @{ 00062 */ 00063 00064 /** @defgroup STM3210C_EVAL_AUDIO_OUT_Exported_Constants STM3210C EVAL AUDIO OUT Exported Constants 00065 * @{ 00066 */ 00067 00068 00069 /*------------------------------------------------------------------------------ 00070 AUDIO OUT CONFIGURATION 00071 ------------------------------------------------------------------------------*/ 00072 00073 /* I2S peripheral configuration defines */ 00074 #define I2SOUT SPI2 00075 #define I2SOUT_CLK_ENABLE() __HAL_RCC_SPI2_CLK_ENABLE() 00076 #define I2SOUT_CLK_DISABLE() __HAL_RCC_SPI2_CLK_DISABLE() 00077 #define I2SOUT_SCK_SD_CLK_ENABLE() __HAL_RCC_GPIOB_CLK_ENABLE() 00078 #define I2SOUT_MCK_CLK_ENABLE() __HAL_RCC_GPIOC_CLK_ENABLE() 00079 #define I2SOUT_WS_CLK_ENABLE() __HAL_RCC_GPIOB_CLK_ENABLE() 00080 #define I2SOUT_WS_PIN GPIO_PIN_12 /* PB.12*/ 00081 #define I2SOUT_SCK_PIN GPIO_PIN_13 /* PB.13*/ 00082 #define I2SOUT_SD_PIN GPIO_PIN_15 /* PB.15*/ 00083 #define I2SOUT_MCK_PIN GPIO_PIN_6 /* PC.06*/ 00084 #define I2SOUT_SCK_SD_GPIO_PORT GPIOB 00085 #define I2SOUT_WS_GPIO_PORT GPIOB 00086 #define I2SOUT_MCK_GPIO_PORT GPIOC 00087 00088 /* I2S DMA Channel definitions */ 00089 #define I2SOUT_DMAx_CLK_ENABLE() __HAL_RCC_DMA1_CLK_ENABLE() 00090 #define I2SOUT_DMAx_CHANNEL DMA1_Channel5 00091 #define I2SOUT_DMAx_IRQ DMA1_Channel5_IRQn 00092 #define I2SOUT_DMAx_PERIPH_DATA_SIZE DMA_PDATAALIGN_HALFWORD 00093 #define I2SOUT_DMAx_MEM_DATA_SIZE DMA_MDATAALIGN_HALFWORD 00094 #define DMA_MAX_SZE 0xFFFF 00095 00096 #define I2SOUT_IRQHandler DMA1_Channel5_IRQHandler 00097 00098 /* Select the interrupt preemption priority and subpriority for the DMA interrupt */ 00099 #define AUDIO_OUT_IRQ_PREPRIO 0xE /* Select the preemption priority level(0 is the highest) */ 00100 00101 /*------------------------------------------------------------------------------ 00102 CONFIGURATION: Audio Driver Configuration parameters 00103 ------------------------------------------------------------------------------*/ 00104 00105 /* Audio status definition */ 00106 #define AUDIO_OK 0 00107 #define AUDIO_ERROR 1 00108 #define AUDIO_TIMEOUT 2 00109 00110 /* PDM buffer input size */ 00111 #define INTERNAL_BUFF_SIZE 128*DEFAULT_AUDIO_IN_FREQ/16000*DEFAULT_AUDIO_IN_CHANNEL_NBR 00112 00113 /*------------------------------------------------------------------------------ 00114 OPTIONAL Configuration defines parameters 00115 ------------------------------------------------------------------------------*/ 00116 00117 00118 /** 00119 * @} 00120 */ 00121 00122 /** @defgroup STM3210C_EVAL_AUDIO_Exported_Macros STM3210C EVAL AUDIO Exported Macros 00123 * @{ 00124 */ 00125 #define DMA_MAX(_X_) (((_X_) <= DMA_MAX_SZE)? (_X_):DMA_MAX_SZE) 00126 00127 /** 00128 * @} 00129 */ 00130 00131 /** @addtogroup STM3210C_EVAL_AUDIO_OUT_Exported_Functions 00132 * @{ 00133 */ 00134 uint8_t BSP_AUDIO_OUT_Init(uint16_t OutputDevice, uint8_t Volume, uint32_t AudioFreq); 00135 void BSP_AUDIO_OUT_DeInit(void); 00136 uint8_t BSP_AUDIO_OUT_Play(uint16_t* pBuffer, uint32_t Size); 00137 void BSP_AUDIO_OUT_ChangeBuffer(uint16_t *pData, uint16_t Size); 00138 uint8_t BSP_AUDIO_OUT_Pause(void); 00139 uint8_t BSP_AUDIO_OUT_Resume(void); 00140 uint8_t BSP_AUDIO_OUT_Stop(uint32_t Option); 00141 uint8_t BSP_AUDIO_OUT_SetVolume(uint8_t Volume); 00142 void BSP_AUDIO_OUT_SetFrequency(uint32_t AudioFreq); 00143 uint8_t BSP_AUDIO_OUT_SetMute(uint32_t Command); 00144 uint8_t BSP_AUDIO_OUT_SetOutputMode(uint8_t Output); 00145 00146 /* User Callbacks: user has to implement these functions in his code if they are needed. */ 00147 /* This function is called when the requested data has been completely transferred.*/ 00148 void BSP_AUDIO_OUT_TransferComplete_CallBack(void); 00149 00150 /* This function is called when half of the requested buffer has been transferred. */ 00151 void BSP_AUDIO_OUT_HalfTransfer_CallBack(void); 00152 00153 /* This function is called when an Interrupt due to transfer error on or peripheral 00154 error occurs. */ 00155 void BSP_AUDIO_OUT_Error_CallBack(void); 00156 00157 /* These function can be modified in case the current settings 00158 need to be changed for specific application needs */ 00159 void BSP_AUDIO_OUT_MspInit(I2S_HandleTypeDef *hi2s, void *Params); 00160 void BSP_AUDIO_OUT_MspDeInit(I2S_HandleTypeDef *hi2s, void *Params); 00161 00162 /** 00163 * @} 00164 */ 00165 00166 00167 00168 /** 00169 * @} 00170 */ 00171 00172 /** 00173 * @} 00174 */ 00175 00176 /** 00177 * @} 00178 */ 00179 00180 #ifdef __cplusplus 00181 } 00182 #endif 00183 00184 #endif /* __STM3210C_EVAL_AUDIO_H */ 00185 00186 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Fri Apr 14 2017 13:00:47 for STM3210C_EVAL BSP User Manual by
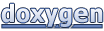