STM3210C_EVAL BSP User Manual
|
Functions | |
static void | I2Cx_MspInit (I2C_HandleTypeDef *hi2c) |
Eval I2Cx MSP Initialization. | |
static HAL_StatusTypeDef | I2Cx_ReadMultiple (uint8_t Addr, uint16_t Reg, uint16_t MemAddress, uint8_t *Buffer, uint16_t Length) |
Reads multiple data. | |
static void | I2Cx_WriteData (uint16_t Addr, uint8_t Reg, uint8_t Value) |
Write a value in a register of the device through BUS. | |
static HAL_StatusTypeDef | I2Cx_WriteBuffer (uint16_t Addr, uint8_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length) |
Write a value in a register of the device through BUS. | |
static uint8_t | I2Cx_ReadData (uint16_t Addr, uint8_t Reg) |
Read a value in a register of the device through BUS. | |
static HAL_StatusTypeDef | I2Cx_ReadBuffer (uint16_t Addr, uint8_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length) |
Reads multiple data on the BUS. | |
static HAL_StatusTypeDef | I2Cx_IsDeviceReady (uint16_t DevAddress, uint32_t Trials) |
Checks if target device is ready for communication. | |
static void | I2Cx_Error (uint8_t Addr) |
Manages error callback by re-initializing I2C. | |
static uint32_t | SPIx_Read (void) |
SPI Read 4 bytes from device. | |
static void | SPIx_WriteReadData (const uint8_t *DataIn, uint8_t *DataOut, uint16_t DataLength) |
SPI Write a byte to device. | |
static void | SPIx_WriteData (const uint8_t *Data, uint16_t DataLength) |
SPI Write Data to device. | |
static void | SPIx_ReadData (const uint8_t *Data, uint16_t DataLength) |
SPI Read Data from device. | |
static void | SPIx_Write (uint8_t Value) |
SPI Write a byte to device. | |
static void | SPIx_Error (void) |
SPI error treatment function. |
Function Documentation
static void I2Cx_Error | ( | uint8_t | Addr | ) | [static] |
Manages error callback by re-initializing I2C.
- Parameters:
-
Addr,: I2C Address
Definition at line 755 of file stm3210c_eval.c.
References heval_I2c, and I2Cx_Init().
Referenced by I2Cx_ReadBuffer(), I2Cx_ReadData(), I2Cx_ReadMultiple(), I2Cx_WriteBuffer(), and I2Cx_WriteData().
static HAL_StatusTypeDef I2Cx_IsDeviceReady | ( | uint16_t | DevAddress, |
uint32_t | Trials | ||
) | [static] |
Checks if target device is ready for communication.
- Note:
- This function is used with Memory devices
- Parameters:
-
DevAddress,: Target device address Trials,: Number of trials
- Return values:
-
HAL status
Definition at line 746 of file stm3210c_eval.c.
References heval_I2c, and I2cxTimeout.
Referenced by EEPROM_I2C_IO_IsDeviceReady(), and TSENSOR_IO_IsDeviceReady().
static void I2Cx_MspInit | ( | I2C_HandleTypeDef * | hi2c | ) | [static] |
Eval I2Cx MSP Initialization.
- Parameters:
-
hi2c,: I2C handle
Definition at line 529 of file stm3210c_eval.c.
References EVAL_I2Cx, EVAL_I2Cx_CLK_ENABLE, EVAL_I2Cx_ER_IRQn, EVAL_I2Cx_EV_IRQn, EVAL_I2Cx_FORCE_RESET, EVAL_I2Cx_RELEASE_RESET, EVAL_I2Cx_SCL_GPIO_CLK_ENABLE, EVAL_I2Cx_SCL_GPIO_PORT, EVAL_I2Cx_SCL_PIN, EVAL_I2Cx_SDA_GPIO_CLK_ENABLE, EVAL_I2Cx_SDA_GPIO_PORT, and EVAL_I2Cx_SDA_PIN.
Referenced by I2Cx_Init().
static HAL_StatusTypeDef I2Cx_ReadBuffer | ( | uint16_t | Addr, |
uint8_t | Reg, | ||
uint16_t | RegSize, | ||
uint8_t * | pBuffer, | ||
uint16_t | Length | ||
) | [static] |
Reads multiple data on the BUS.
- Parameters:
-
Addr,: I2C Address Reg,: Reg Address RegSize : The target register size (can be 8BIT or 16BIT) pBuffer,: pointer to read data buffer Length,: length of the data
- Return values:
-
0 if no problems to read multiple data
Definition at line 724 of file stm3210c_eval.c.
References heval_I2c, I2Cx_Error(), and I2cxTimeout.
Referenced by ACCELERO_IO_Read(), EEPROM_I2C_IO_ReadData(), and TSENSOR_IO_Read().
static uint8_t I2Cx_ReadData | ( | uint16_t | Addr, |
uint8_t | Reg | ||
) | [static] |
Read a value in a register of the device through BUS.
- Parameters:
-
Addr,: Device address on BUS Bus. Reg,: The target register address to write
- Return values:
-
Data read at register @
Definition at line 698 of file stm3210c_eval.c.
References heval_I2c, I2Cx_Error(), and I2cxTimeout.
Referenced by AUDIO_IO_Read(), and IOE_Read().
static HAL_StatusTypeDef I2Cx_ReadMultiple | ( | uint8_t | Addr, |
uint16_t | Reg, | ||
uint16_t | MemAddress, | ||
uint8_t * | Buffer, | ||
uint16_t | Length | ||
) | [static] |
Reads multiple data.
- Parameters:
-
Addr,: I2C address Reg,: Reg address MemAddress,: Internal memory address Buffer,: Pointer to data buffer Length,: Length of the data
- Return values:
-
Number of read data
Definition at line 634 of file stm3210c_eval.c.
References heval_I2c, I2Cx_Error(), and I2cxTimeout.
Referenced by IOE_ReadMultiple().
static HAL_StatusTypeDef I2Cx_WriteBuffer | ( | uint16_t | Addr, |
uint8_t | Reg, | ||
uint16_t | RegSize, | ||
uint8_t * | pBuffer, | ||
uint16_t | Length | ||
) | [static] |
Write a value in a register of the device through BUS.
- Parameters:
-
Addr,: Device address on BUS Bus. Reg,: The target register address to write RegSize,: The target register size (can be 8BIT or 16BIT) pBuffer,: The target register value to be written Length,: buffer size to be written
Definition at line 677 of file stm3210c_eval.c.
References heval_I2c, I2Cx_Error(), and I2cxTimeout.
Referenced by ACCELERO_IO_Write(), EEPROM_I2C_IO_WriteData(), and TSENSOR_IO_Write().
static void I2Cx_WriteData | ( | uint16_t | Addr, |
uint8_t | Reg, | ||
uint8_t | Value | ||
) | [static] |
Write a value in a register of the device through BUS.
- Parameters:
-
Addr,: Device address on BUS Bus. Reg,: The target register address to write Value,: The target register value to be written
Definition at line 655 of file stm3210c_eval.c.
References heval_I2c, I2Cx_Error(), and I2cxTimeout.
Referenced by AUDIO_IO_Write(), and IOE_Write().
static void SPIx_Error | ( | void | ) | [static] |
SPI error treatment function.
- Return values:
-
None
Definition at line 935 of file stm3210c_eval.c.
References heval_Spi, and SPIx_Init().
Referenced by SPIx_Read(), SPIx_ReadData(), SPIx_Write(), SPIx_WriteData(), and SPIx_WriteReadData().
static uint32_t SPIx_Read | ( | void | ) | [static] |
SPI Read 4 bytes from device.
- Return values:
-
Read data
Definition at line 836 of file stm3210c_eval.c.
References heval_Spi, SPIx_Error(), and SpixTimeout.
Referenced by LCD_IO_ReadData().
static void SPIx_ReadData | ( | const uint8_t * | Data, |
uint16_t | DataLength | ||
) | [static] |
SPI Read Data from device.
- Parameters:
-
Data,: value to be read DataLength,: length of data
Definition at line 898 of file stm3210c_eval.c.
References heval_Spi, SPIx_Error(), and SpixTimeout.
Referenced by SD_IO_ReadData().
static void SPIx_Write | ( | uint8_t | Value | ) | [static] |
SPI Write a byte to device.
- Parameters:
-
Value,: value to be written
Definition at line 916 of file stm3210c_eval.c.
References heval_Spi, SPIx_Error(), and SpixTimeout.
Referenced by LCD_IO_ReadData(), LCD_IO_WriteMultipleData(), and LCD_IO_WriteReg().
static void SPIx_WriteData | ( | const uint8_t * | Data, |
uint16_t | DataLength | ||
) | [static] |
SPI Write Data to device.
- Parameters:
-
Data,: value to be written DataLength,: length of data
Definition at line 879 of file stm3210c_eval.c.
References heval_Spi, SPIx_Error(), and SpixTimeout.
Referenced by SD_IO_WriteData().
static void SPIx_WriteReadData | ( | const uint8_t * | DataIn, |
uint8_t * | DataOut, | ||
uint16_t | DataLength | ||
) | [static] |
SPI Write a byte to device.
- Parameters:
-
DataIn,: value to be written DataOut,: value to be read DataLength,: length of data
Definition at line 860 of file stm3210c_eval.c.
References heval_Spi, SPIx_Error(), and SpixTimeout.
Referenced by SD_IO_WriteByte(), and SD_IO_WriteReadData().
Generated on Fri Apr 14 2017 13:00:47 for STM3210C_EVAL BSP User Manual by
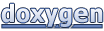