STM3210C_EVAL BSP User Manual
|
Functions | |
uint8_t | BSP_SD_Init (void) |
Initializes the SD/SD communication. | |
uint8_t | BSP_SD_IsDetected (void) |
Detects if SD card is correctly plugged in the memory slot or not. | |
uint8_t | BSP_SD_GetCardInfo (SD_CardInfo *pCardInfo) |
Returns information about specific card. | |
uint8_t | BSP_SD_ReadBlocks (uint32_t *pData, uint32_t ReadAddr, uint32_t NumOfBlocks, uint32_t Timeout) |
Reads block(s) from a specified address in the SD card, in polling mode. | |
uint8_t | BSP_SD_WriteBlocks (uint32_t *pData, uint32_t WriteAddr, uint32_t NumOfBlocks, uint32_t Timeout) |
Writes block(s) to a specified address in the SD card, in polling mode. | |
uint8_t | BSP_SD_Erase (uint32_t StartAddr, uint32_t EndAddr) |
Erases the specified memory area of the given SD card. | |
uint8_t | BSP_SD_GetCardState (void) |
Returns the SD status. | |
void | SD_IO_Init (void) |
Initializes the SD Card and put it into StandBy State (Ready for data transfer). | |
void | SD_IO_CSState (uint8_t state) |
Set the SD_CS pin. | |
void | SD_IO_WriteReadData (const uint8_t *DataIn, uint8_t *DataOut, uint16_t DataLength) |
Write a byte on the SD. | |
void | SD_IO_WriteData (const uint8_t *Data, uint16_t DataLength) |
Write a byte on the SD. | |
void | SD_IO_ReadData (const uint8_t *Data, uint16_t DataLength) |
Read a byte from the SD. | |
uint8_t | SD_IO_WriteByte (uint8_t Data) |
Writes a byte on the SD. | |
void | HAL_Delay (__IO uint32_t Delay) |
static uint8_t | SD_GetCSDRegister (SD_CSD *Csd) |
Reads the SD card SCD register. | |
static uint8_t | SD_GetCIDRegister (SD_CID *Cid) |
Reads the SD card CID register. | |
static SD_CmdAnswer_typedef | SD_SendCmd (uint8_t Cmd, uint32_t Arg, uint8_t Crc, uint8_t Answer) |
Sends 5 bytes command to the SD card and get response. | |
static uint8_t | SD_GetDataResponse (void) |
Gets the SD card data response and check the busy flag. | |
static uint8_t | SD_GoIdleState (void) |
Put the SD in Idle state. | |
static uint8_t | SD_ReadData (void) |
Waits a data until a value different from SD_DUMMY_BITE. | |
static uint8_t | SD_WaitData (uint8_t data) |
Waits a data from the SD card. |
Function Documentation
uint8_t BSP_SD_Erase | ( | uint32_t | StartAddr, |
uint32_t | EndAddr | ||
) |
Erases the specified memory area of the given SD card.
- Parameters:
-
StartAddr,: Start address in Blocks (Size of a block is 512bytes) EndAddr,: End address in Blocks (Size of a block is 512bytes)
- Return values:
-
SD status
Definition at line 492 of file stm3210c_eval_sd.c.
References BSP_SD_ERROR, BSP_SD_OK, SD_CmdAnswer_typedef::r1, SD_ANSWER_R1_EXPECTED, SD_ANSWER_R1B_EXPECTED, SD_CMD_ERASE, SD_CMD_SD_ERASE_GRP_END, SD_CMD_SD_ERASE_GRP_START, SD_DUMMY_BYTE, SD_IO_CSState(), SD_IO_WriteByte(), SD_R1_NO_ERROR, and SD_SendCmd().
uint8_t BSP_SD_GetCardInfo | ( | SD_CardInfo * | pCardInfo | ) |
Returns information about specific card.
- Parameters:
-
pCardInfo,: pointer to a SD_CardInfo structure that contains all SD card information.
- Return values:
-
The SD Response: - MSD_ERROR : Sequence failed
- MSD_OK : Sequence succeed
Definition at line 316 of file stm3210c_eval_sd.c.
References SD_CardInfo::CardBlockSize, SD_CardInfo::CardCapacity, SD_CardInfo::Cid, SD_CardInfo::Csd, struct_v1::DeviceSize, struct_v2::DeviceSize, struct_v1::DeviceSizeMul, SD_CardInfo::LogBlockNbr, SD_CardInfo::LogBlockSize, SD_CSD::RdBlockLen, SD_GetCIDRegister(), SD_GetCSDRegister(), SD_CSD::csd_version::v1, SD_CSD::csd_version::v2, and SD_CSD::version.
uint8_t BSP_SD_GetCardState | ( | void | ) |
Returns the SD status.
- Parameters:
-
None
- Return values:
-
The SD status.
Definition at line 529 of file stm3210c_eval_sd.c.
References BSP_SD_ERROR, BSP_SD_OK, SD_CmdAnswer_typedef::r1, SD_CmdAnswer_typedef::r2, SD_ANSWER_R2_EXPECTED, SD_CMD_SEND_STATUS, SD_DUMMY_BYTE, SD_IO_CSState(), SD_IO_WriteByte(), SD_R1_NO_ERROR, SD_R2_NO_ERROR, and SD_SendCmd().
uint8_t BSP_SD_Init | ( | void | ) |
Initializes the SD/SD communication.
- Return values:
-
The SD Response: - MSD_ERROR : Sequence failed
- MSD_OK : Sequence succeed
Definition at line 272 of file stm3210c_eval_sd.c.
References BSP_SD_IsDetected(), SD_GoIdleState(), SD_IO_Init(), SD_NOT_PRESENT, and SD_PRESENT.
uint8_t BSP_SD_IsDetected | ( | void | ) |
Detects if SD card is correctly plugged in the memory slot or not.
- Return values:
-
Returns if SD is detected or not
Definition at line 295 of file stm3210c_eval_sd.c.
References SD_DETECT_GPIO_PORT, SD_DETECT_PIN, SD_NOT_PRESENT, and SD_PRESENT.
Referenced by BSP_SD_Init().
uint8_t BSP_SD_ReadBlocks | ( | uint32_t * | pData, |
uint32_t | ReadAddr, | ||
uint32_t | NumOfBlocks, | ||
uint32_t | Timeout | ||
) |
Reads block(s) from a specified address in the SD card, in polling mode.
- Parameters:
-
pData,: Pointer to the buffer that will contain the data to transmit ReadAddr,: Address from where data is to be read. The address is counted in blocks of 512bytes NumOfBlocks,: Number of SD blocks to read Timeout,: This parameter is used for compatibility with BSP implementation
- Return values:
-
SD status
Definition at line 350 of file stm3210c_eval_sd.c.
References BSP_SD_ERROR, BSP_SD_OK, SD_CmdAnswer_typedef::r1, SD_ANSWER_R1_EXPECTED, SD_CMD_READ_SINGLE_BLOCK, SD_CMD_SET_BLOCKLEN, SD_DUMMY_BYTE, SD_IO_CSState(), SD_IO_ReadData(), SD_IO_WriteByte(), SD_R1_NO_ERROR, SD_SendCmd(), SD_TOKEN_START_DATA_SINGLE_BLOCK_READ, and SD_WaitData().
uint8_t BSP_SD_WriteBlocks | ( | uint32_t * | pData, |
uint32_t | WriteAddr, | ||
uint32_t | NumOfBlocks, | ||
uint32_t | Timeout | ||
) |
Writes block(s) to a specified address in the SD card, in polling mode.
- Parameters:
-
pData,: Pointer to the buffer that will contain the data to transmit WriteAddr,: Address from where data is to be written. The address is counted in blocks of 512bytes NumOfBlocks,: Number of SD blocks to write Timeout,: This parameter is used for compatibility with BSP implementation
- Return values:
-
SD status
Definition at line 420 of file stm3210c_eval_sd.c.
References BSP_SD_ERROR, BSP_SD_OK, SD_CmdAnswer_typedef::r1, SD_ANSWER_R1_EXPECTED, SD_CMD_SET_BLOCKLEN, SD_CMD_WRITE_SINGLE_BLOCK, SD_DATA_OK, SD_DUMMY_BYTE, SD_GetDataResponse(), SD_IO_CSState(), SD_IO_WriteByte(), SD_IO_WriteData(), SD_R1_NO_ERROR, SD_SendCmd(), and SD_TOKEN_START_DATA_SINGLE_BLOCK_WRITE.
void HAL_Delay | ( | __IO uint32_t | Delay | ) |
Referenced by AUDIO_IO_Init(), BSP_AUDIO_OUT_Stop(), IOE_Delay(), LCD_Delay(), LCD_IO_ReadData(), and SD_SendCmd().
uint8_t SD_GetCIDRegister | ( | SD_CID * | Cid | ) | [static] |
Reads the SD card CID register.
Reading the contents of the CID register in SPI mode is a simple read-block transaction.
- Parameters:
-
Cid,: pointer on an CID register structure
- Return values:
-
SD status
Definition at line 668 of file stm3210c_eval_sd.c.
References BSP_SD_ERROR, BSP_SD_OK, SD_CID::CID_CRC, SD_CID::ManufactDate, SD_CID::ManufacturerID, SD_CID::OEM_AppliID, SD_CID::ProdName1, SD_CID::ProdName2, SD_CID::ProdRev, SD_CID::ProdSN, SD_CmdAnswer_typedef::r1, SD_CID::Reserved1, SD_CID::Reserved2, SD_ANSWER_R1_EXPECTED, SD_CMD_SEND_CID, SD_DUMMY_BYTE, SD_IO_CSState(), SD_IO_WriteByte(), SD_R1_NO_ERROR, SD_SendCmd(), SD_TOKEN_START_DATA_SINGLE_BLOCK_READ, and SD_WaitData().
Referenced by BSP_SD_GetCardInfo().
uint8_t SD_GetCSDRegister | ( | SD_CSD * | Csd | ) | [static] |
Reads the SD card SCD register.
Reading the contents of the CSD register in SPI mode is a simple read-block transaction.
- Parameters:
-
Csd,: pointer on an SCD register structure
- Return values:
-
SD status
Definition at line 554 of file stm3210c_eval_sd.c.
References BSP_SD_ERROR, BSP_SD_OK, SD_CSD::CardComdClasses, SD_CSD::CopyFlag, SD_CSD::crc, SD_CSD::CSDStruct, struct_v1::DeviceSize, struct_v2::DeviceSize, struct_v1::DeviceSizeMul, SD_CSD::DSRImpl, SD_CSD::EraseSectorSize, SD_CSD::EraseSingleBlockEnable, SD_CSD::FileFormat, SD_CSD::FileFormatGrouop, SD_CSD::MaxBusClkFrec, struct_v1::MaxRdCurrentVDDMax, struct_v1::MaxRdCurrentVDDMin, SD_CSD::MaxWrBlockLen, struct_v1::MaxWrCurrentVDDMax, struct_v1::MaxWrCurrentVDDMin, SD_CSD::NSAC, SD_CSD::PartBlockRead, SD_CSD::PermWrProtect, SD_CmdAnswer_typedef::r1, SD_CSD::RdBlockLen, SD_CSD::RdBlockMisalign, struct_v1::Reserved1, struct_v2::Reserved1, SD_CSD::Reserved1, struct_v2::Reserved2, SD_CSD::Reserved2, SD_CSD::Reserved3, SD_CSD::Reserved4, SD_CSD::Reserved5, SD_ANSWER_R1_EXPECTED, SD_CMD_SEND_CSD, SD_DUMMY_BYTE, SD_IO_CSState(), SD_IO_WriteByte(), SD_R1_NO_ERROR, SD_SendCmd(), SD_TOKEN_START_DATA_SINGLE_BLOCK_READ, SD_WaitData(), SD_CSD::TAAC, SD_CSD::TempWrProtect, SD_CSD::csd_version::v1, SD_CSD::csd_version::v2, SD_CSD::version, SD_CSD::WrBlockMisalign, SD_CSD::WriteBlockPartial, SD_CSD::WrProtectGrEnable, SD_CSD::WrProtectGrSize, and SD_CSD::WrSpeedFact.
Referenced by BSP_SD_GetCardInfo().
uint8_t SD_GetDataResponse | ( | void | ) | [static] |
Gets the SD card data response and check the busy flag.
- Return values:
-
The SD status: Read data response xxx0<status>1 - status 010: Data accecpted
- status 101: Data rejected due to a crc error
- status 110: Data rejected due to a Write error.
- status 111: Data rejected due to other error.
Definition at line 825 of file stm3210c_eval_sd.c.
References SD_DATA_CRC_ERROR, SD_DATA_OK, SD_DATA_OTHER_ERROR, SD_DATA_WRITE_ERROR, SD_DUMMY_BYTE, SD_IO_CSState(), and SD_IO_WriteByte().
Referenced by BSP_SD_WriteBlocks().
uint8_t SD_GoIdleState | ( | void | ) | [static] |
Put the SD in Idle state.
- Return values:
-
SD status
Definition at line 866 of file stm3210c_eval_sd.c.
References BSP_SD_ERROR, BSP_SD_OK, SD_CmdAnswer_typedef::r1, SD_CmdAnswer_typedef::r2, SD_ANSWER_R1_EXPECTED, SD_ANSWER_R3_EXPECTED, SD_ANSWER_R7_EXPECTED, SD_CMD_APP_CMD, SD_CMD_GO_IDLE_STATE, SD_CMD_READ_OCR, SD_CMD_SD_APP_OP_COND, SD_CMD_SEND_IF_COND, SD_DUMMY_BYTE, SD_IO_CSState(), SD_IO_WriteByte(), SD_MAX_TRY, SD_R1_ILLEGAL_COMMAND, SD_R1_IN_IDLE_STATE, SD_R1_NO_ERROR, and SD_SendCmd().
Referenced by BSP_SD_Init().
void SD_IO_CSState | ( | uint8_t | val | ) |
Set the SD_CS pin.
- Parameters:
-
pin value.
- Return values:
-
None
Definition at line 1197 of file stm3210c_eval.c.
References SD_CS_HIGH, and SD_CS_LOW.
Referenced by BSP_SD_Erase(), BSP_SD_GetCardState(), BSP_SD_ReadBlocks(), BSP_SD_WriteBlocks(), SD_GetCIDRegister(), SD_GetCSDRegister(), SD_GetDataResponse(), SD_GoIdleState(), and SD_SendCmd().
void SD_IO_Init | ( | void | ) |
Initializes the SD Card and put it into StandBy State (Ready for data transfer).
Definition at line 1154 of file stm3210c_eval.c.
References SD_CS_GPIO_CLK_ENABLE, SD_CS_GPIO_PORT, SD_CS_HIGH, SD_CS_PIN, SD_DETECT_GPIO_CLK_ENABLE, SD_DETECT_GPIO_PORT, SD_DETECT_PIN, SD_DUMMY_BYTE, SD_IO_WriteByte(), and SPIx_Init().
Referenced by BSP_SD_Init().
void SD_IO_ReadData | ( | const uint8_t * | Data, |
uint16_t | DataLength | ||
) |
Read a byte from the SD.
- Parameters:
-
Data,: value to be read DataLength,: length of data
Definition at line 1237 of file stm3210c_eval.c.
References SPIx_ReadData().
Referenced by BSP_SD_ReadBlocks().
uint8_t SD_IO_WriteByte | ( | uint8_t | Data | ) |
Writes a byte on the SD.
- Parameters:
-
Data,: byte to send.
Definition at line 1247 of file stm3210c_eval.c.
References SPIx_WriteReadData().
Referenced by BSP_SD_Erase(), BSP_SD_GetCardState(), BSP_SD_ReadBlocks(), BSP_SD_WriteBlocks(), SD_GetCIDRegister(), SD_GetCSDRegister(), SD_GetDataResponse(), SD_GoIdleState(), SD_IO_Init(), SD_ReadData(), SD_SendCmd(), and SD_WaitData().
void SD_IO_WriteData | ( | const uint8_t * | Data, |
uint16_t | DataLength | ||
) |
Write a byte on the SD.
- Parameters:
-
Data,: value to be written DataLength,: length of data
Definition at line 1226 of file stm3210c_eval.c.
References SPIx_WriteData().
Referenced by BSP_SD_WriteBlocks().
void SD_IO_WriteReadData | ( | const uint8_t * | DataIn, |
uint8_t * | DataOut, | ||
uint16_t | DataLength | ||
) |
Write a byte on the SD.
- Parameters:
-
DataIn,: value to be written DataOut,: value to be read DataLength,: length of data
Definition at line 1215 of file stm3210c_eval.c.
References SPIx_WriteReadData().
Referenced by SD_SendCmd().
uint8_t SD_ReadData | ( | void | ) | [static] |
Waits a data until a value different from SD_DUMMY_BITE.
- Parameters:
-
None
- Return values:
-
the value read
Definition at line 968 of file stm3210c_eval_sd.c.
References SD_DUMMY_BYTE, and SD_IO_WriteByte().
Referenced by SD_SendCmd().
SD_CmdAnswer_typedef SD_SendCmd | ( | uint8_t | Cmd, |
uint32_t | Arg, | ||
uint8_t | Crc, | ||
uint8_t | Answer | ||
) | [static] |
Sends 5 bytes command to the SD card and get response.
- Parameters:
-
Cmd,: The user expected command to send to SD card. Arg,: The command argument. Crc,: The CRC. Answer,: SD_ANSWER_NOT_EXPECTED or SD_ANSWER_EXPECTED
- Return values:
-
SD status
Definition at line 761 of file stm3210c_eval_sd.c.
References HAL_Delay(), SD_CmdAnswer_typedef::r1, SD_CmdAnswer_typedef::r2, SD_CmdAnswer_typedef::r3, SD_CmdAnswer_typedef::r4, SD_CmdAnswer_typedef::r5, SD_ANSWER_R1_EXPECTED, SD_ANSWER_R1B_EXPECTED, SD_ANSWER_R2_EXPECTED, SD_ANSWER_R3_EXPECTED, SD_ANSWER_R7_EXPECTED, SD_CMD_LENGTH, SD_DUMMY_BYTE, SD_IO_CSState(), SD_IO_WriteByte(), SD_IO_WriteReadData(), and SD_ReadData().
Referenced by BSP_SD_Erase(), BSP_SD_GetCardState(), BSP_SD_ReadBlocks(), BSP_SD_WriteBlocks(), SD_GetCIDRegister(), SD_GetCSDRegister(), and SD_GoIdleState().
uint8_t SD_WaitData | ( | uint8_t | data | ) | [static] |
Waits a data from the SD card.
- Parameters:
-
data : Expected data from the SD card
- Return values:
-
BSP_SD_OK or BSP_SD_TIMEOUT
Definition at line 989 of file stm3210c_eval_sd.c.
References BSP_SD_OK, BSP_SD_TIMEOUT, SD_DUMMY_BYTE, and SD_IO_WriteByte().
Referenced by BSP_SD_ReadBlocks(), SD_GetCIDRegister(), and SD_GetCSDRegister().
Generated on Fri Apr 14 2017 13:00:47 for STM3210C_EVAL BSP User Manual by
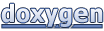