STM3210C_EVAL BSP User Manual
|
stm3210c_eval_accelerometer.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm3210c_eval_accelerometer.c 00004 * @author MCD Application Team 00005 * @version V7.0.0 00006 * @date 14-April-2017 00007 * @brief This file provides a set of functions needed to manage the ACCELEROMETER 00008 * MEMS accelerometer available on STM3210C_EVAL board. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* Includes ------------------------------------------------------------------*/ 00040 #include "stm3210c_eval_accelerometer.h" 00041 00042 /** @addtogroup BSP 00043 * @{ 00044 */ 00045 00046 /** @addtogroup STM3210C_EVAL 00047 * @{ 00048 */ 00049 00050 /** @defgroup STM3210C_EVAL_ACCELEROMETER STM3210C EVAL ACCELEROMETER 00051 * @brief This file includes the motion sensor driver for ACCELEROMETER motion sensor 00052 * devices. 00053 * @{ 00054 */ 00055 00056 /** @defgroup STM3210C_EVAL_ACCELEROMETER_Private_Variables STM3210C EVAL ACCELEROMETER Private Variables 00057 * @{ 00058 */ 00059 static ACCELERO_DrvTypeDef *AcceleroDrv; 00060 00061 /** 00062 * @} 00063 */ 00064 00065 00066 /** @defgroup STM3210C_EVAL_ACCELEROMETER_Exported_Functions STM3210C EVAL ACCELEROMETER Exported Functions 00067 * @{ 00068 */ 00069 00070 /** 00071 * @brief Set ACCELEROMETER Initialization. 00072 */ 00073 uint8_t BSP_ACCELERO_Init(void) 00074 { 00075 uint8_t ret = ACCELERO_ERROR; 00076 uint16_t ctrl = 0x0000; 00077 LIS302DL_InitTypeDef lis302dl_initstruct; 00078 LIS302DL_FilterConfigTypeDef lis302dl_filter={0,0,0}; 00079 00080 if(Lis302dlDrv.ReadID() == I_AM_LIS302DL) 00081 { 00082 /* Initialize the gyroscope driver structure */ 00083 AcceleroDrv = &Lis302dlDrv; 00084 00085 /* Set configuration of LIS302DL MEMS Accelerometer *********************/ 00086 lis302dl_initstruct.Power_Mode = LIS302DL_LOWPOWERMODE_ACTIVE; 00087 lis302dl_initstruct.Output_DataRate = LIS302DL_DATARATE_100; 00088 lis302dl_initstruct.Axes_Enable = LIS302DL_XYZ_ENABLE; 00089 lis302dl_initstruct.Full_Scale = LIS302DL_FULLSCALE_2_3; 00090 lis302dl_initstruct.Self_Test = LIS302DL_SELFTEST_NORMAL; 00091 00092 /* Configure MEMS: data rate, power mode, full scale, self test and axes */ 00093 ctrl = (uint16_t) (lis302dl_initstruct.Output_DataRate | lis302dl_initstruct.Power_Mode | \ 00094 lis302dl_initstruct.Full_Scale | lis302dl_initstruct.Self_Test | \ 00095 lis302dl_initstruct.Axes_Enable); 00096 00097 /* Configure the accelerometer main parameters */ 00098 AcceleroDrv->Init(ctrl); 00099 00100 /* MEMS High Pass Filter configuration */ 00101 lis302dl_filter.HighPassFilter_Data_Selection = LIS302DL_FILTEREDDATASELECTION_OUTPUTREGISTER; 00102 lis302dl_filter.HighPassFilter_CutOff_Frequency = LIS302DL_HIGHPASSFILTER_LEVEL_1; 00103 lis302dl_filter.HighPassFilter_Interrupt = LIS302DL_HIGHPASSFILTERINTERRUPT_1_2; 00104 00105 /* Configure MEMS high pass filter cut-off level, interrupt and data selection bits */ 00106 ctrl = (uint8_t)(lis302dl_filter.HighPassFilter_Data_Selection | \ 00107 lis302dl_filter.HighPassFilter_CutOff_Frequency | \ 00108 lis302dl_filter.HighPassFilter_Interrupt); 00109 00110 /* Configure the accelerometer LPF main parameters */ 00111 AcceleroDrv->FilterConfig(ctrl); 00112 00113 ret = ACCELERO_OK; 00114 } 00115 else 00116 { 00117 ret = ACCELERO_ERROR; 00118 } 00119 00120 return ret; 00121 } 00122 00123 /** 00124 * @brief Read ID of Accelerometer component 00125 * @retval ID 00126 */ 00127 uint8_t BSP_ACCELERO_ReadID(void) 00128 { 00129 uint8_t id = 0x00; 00130 00131 if(AcceleroDrv->ReadID != NULL) 00132 { 00133 id = AcceleroDrv->ReadID(); 00134 } 00135 return id; 00136 } 00137 00138 /** 00139 * @brief Reboot memory content of ACCELEROMETER 00140 */ 00141 void BSP_ACCELERO_Reset(void) 00142 { 00143 if(AcceleroDrv->Reset != NULL) 00144 { 00145 AcceleroDrv->Reset(); 00146 } 00147 } 00148 00149 /** 00150 * @brief Config Accelerometer click IT 00151 */ 00152 void BSP_ACCELERO_Click_ITConfig(void) 00153 { 00154 if(AcceleroDrv->ConfigIT!= NULL) 00155 { 00156 AcceleroDrv->ConfigIT(); 00157 } 00158 } 00159 00160 00161 /** 00162 * @brief Clear Accelerometer click IT 00163 */ 00164 void BSP_ACCELERO_Click_ITClear(void) 00165 { 00166 if(AcceleroDrv->ClearIT!= NULL) 00167 { 00168 AcceleroDrv->ClearIT(); 00169 } 00170 } 00171 00172 /** 00173 * @brief Get XYZ acceleration 00174 * @param pDataXYZ: angular acceleration on X/Y/Z axis 00175 */ 00176 void BSP_ACCELERO_GetXYZ(int16_t *pDataXYZ) 00177 { 00178 int16_t SwitchXY = 0; 00179 00180 if(AcceleroDrv->GetXYZ!= NULL) 00181 { 00182 AcceleroDrv->GetXYZ(pDataXYZ); 00183 00184 /* Switch X and Y Axis in case of MEMS LIS302DL */ 00185 if(AcceleroDrv == &Lis302dlDrv) 00186 { 00187 SwitchXY = pDataXYZ[0]; 00188 pDataXYZ[0] = pDataXYZ[1]; 00189 /* Invert Y Axis to be conpliant with LIS3DSH */ 00190 pDataXYZ[1] = -SwitchXY; 00191 } 00192 } 00193 } 00194 00195 00196 /** 00197 * @} 00198 */ 00199 00200 /** 00201 * @} 00202 */ 00203 00204 /** 00205 * @} 00206 */ 00207 00208 /** 00209 * @} 00210 */ 00211 00212 00213 /******************* (C) COPYRIGHT 2011 STMicroelectronics *****END OF FILE****/
Generated on Fri Apr 14 2017 13:00:47 for STM3210C_EVAL BSP User Manual by
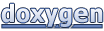