STM3210C_EVAL BSP User Manual
|
stm3210c_eval_audio.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm3210c_eval_audio.c 00004 * @author MCD Application Team 00005 * @version V7.0.0 00006 * @date 14-April-2017 00007 * @brief This file provides the Audio driver for the STM3210C-Eval 00008 * board. 00009 @verbatim 00010 ============================================================================== 00011 ##### How to use this driver ##### 00012 ============================================================================== 00013 [..] 00014 (#) This driver supports STM32F107xC devices on STM3210C-Eval Kit: 00015 (++) to play an audio file (all functions names start by BSP_AUDIO_OUT_xxx) 00016 00017 [..] 00018 (#) PLAY A FILE: 00019 (++) Call the function BSP_AUDIO_OUT_Init( 00020 OutputDevice: physical output mode (OUTPUT_DEVICE_SPEAKER, 00021 OUTPUT_DEVICE_HEADPHONE, OUTPUT_DEVICE_AUTO or 00022 OUTPUT_DEVICE_BOTH) 00023 Volume: initial volume to be set (0 is min (mute), 100 is max (100%) 00024 AudioFreq: Audio frequency in Hz (8000, 16000, 22500, 32000 ...) 00025 this parameter is relative to the audio file/stream type. 00026 ) 00027 This function configures all the hardware required for the audio application 00028 (codec, I2C, I2S, GPIOs, DMA and interrupt if needed). This function returns 0 00029 if configuration is OK. 00030 If the returned value is different from 0 or the function is stuck then the 00031 communication with the codec (try to un-plug the power or reset device in this case). 00032 (+++) OUTPUT_DEVICE_SPEAKER: only speaker will be set as output for the 00033 audio stream. 00034 (+++) OUTPUT_DEVICE_HEADPHONE: only headphones will be set as output for 00035 the audio stream. 00036 (+++) OUTPUT_DEVICE_AUTO: Selection of output device is made through external 00037 switch (implemented into the audio jack on the evaluation board). 00038 When the Headphone is connected it is used as output. 00039 When the headphone is disconnected from the audio jack, the output is 00040 automatically switched to Speaker. 00041 (+++) OUTPUT_DEVICE_BOTH: both Speaker and Headphone are used as outputs 00042 for the audio stream at the same time. 00043 (++) Call the function BSP_AUDIO_OUT_Play( 00044 pBuffer: pointer to the audio data file address 00045 Size: size of the buffer to be sent in Bytes 00046 ) 00047 to start playing (for the first time) from the audio file/stream. 00048 (++) Call the function BSP_AUDIO_OUT_Pause() to pause playing 00049 (++) Call the function BSP_AUDIO_OUT_Resume() to resume playing. 00050 Note. After calling BSP_AUDIO_OUT_Pause() function for pause, 00051 only BSP_AUDIO_OUT_Resume() should be called for resume 00052 (it is not allowed to call BSP_AUDIO_OUT_Play() in this case). 00053 Note. This function should be called only when the audio file is played 00054 or paused (not stopped). 00055 (++) For each mode, you may need to implement the relative callback functions 00056 into your code. 00057 The Callback functions are named BSP_AUDIO_OUT_XXXCallBack() and only 00058 their prototypes are declared in the stm3210c_eval_audio.h file. 00059 (refer to the example for more details on the callbacks implementations) 00060 (++) To Stop playing, to modify the volume level, the frequency or to mute, 00061 use the functions BSP_AUDIO_OUT_Stop(), BSP_AUDIO_OUT_SetVolume(), 00062 AUDIO_OUT_SetFrequency() BSP_AUDIO_OUT_SetOutputMode and BSP_AUDIO_OUT_SetMute(). 00063 (++) The driver API and the callback functions are at the end of the 00064 stm3210c_eval_audio.h file. 00065 00066 (++) This driver provide the High Audio Layer: consists of the function API 00067 exported in the stm3210c_eval_audio.h file (BSP_AUDIO_OUT_Init(), 00068 BSP_AUDIO_OUT_Play() ...) 00069 (++) This driver provide also the Media Access Layer (MAL): which consists 00070 of functions allowing to access the media containing/providing the 00071 audio file/stream. These functions are also included as local functions into 00072 the stm3210c_eval_audio.c file (I2SOUT_Init()...) 00073 00074 [..] 00075 ##### Known Limitations ##### 00076 ============================================================================== 00077 (#) When using the Speaker, if the audio file quality is not high enough, the 00078 speaker output may produce high and uncomfortable noise level. To avoid 00079 this issue, to use speaker output properly, try to increase audio file 00080 sampling rate (typically higher than 48KHz). 00081 This operation will lead to larger file size. 00082 00083 (#) Communication with the audio codec (through I2C) may be corrupted if it 00084 is interrupted by some user interrupt routines (in this case, interrupts 00085 could be disabled just before the start of communication then re-enabled 00086 when it is over). Note that this communication is only done at the 00087 configuration phase (BSP_AUDIO_OUT_Init() or BSP_AUDIO_OUT_Stop()) 00088 and when Volume control modification is performed (BSP_AUDIO_OUT_SetVolume() 00089 or BSP_AUDIO_OUT_SetMute()or BSP_AUDIO_OUT_SetOutputMode()). 00090 When the audio data is played, no communication is required with the audio codec. 00091 00092 (#) Parsing of audio file is not implemented (in order to determine audio file 00093 properties: Mono/Stereo, Data size, File size, Audio Frequency, Audio Data 00094 header size ...). The configuration is fixed for the given audio file. 00095 00096 (#) Mono audio streaming is not supported (in order to play mono audio streams, 00097 each data should be sent twice on the I2S or should be duplicated on the 00098 source buffer. Or convert the stream in stereo before playing). 00099 00100 (#) Supports only 16-bit audio data size. 00101 00102 @endverbatim 00103 ****************************************************************************** 00104 * @attention 00105 * 00106 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00107 * 00108 * Redistribution and use in source and binary forms, with or without modification, 00109 * are permitted provided that the following conditions are met: 00110 * 1. Redistributions of source code must retain the above copyright notice, 00111 * this list of conditions and the following disclaimer. 00112 * 2. Redistributions in binary form must reproduce the above copyright notice, 00113 * this list of conditions and the following disclaimer in the documentation 00114 * and/or other materials provided with the distribution. 00115 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00116 * may be used to endorse or promote products derived from this software 00117 * without specific prior written permission. 00118 * 00119 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00120 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00121 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00122 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00123 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00124 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00125 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00126 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00127 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00128 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00129 * 00130 ****************************************************************************** 00131 */ 00132 00133 /* Includes ------------------------------------------------------------------*/ 00134 #include "stm3210c_eval_audio.h" 00135 00136 /** @addtogroup BSP 00137 * @{ 00138 */ 00139 00140 /** @addtogroup STM3210C_EVAL 00141 * @{ 00142 */ 00143 00144 /** @defgroup STM3210C_EVAL_AUDIO STM3210C EVAL AUDIO 00145 * @brief This file includes the low layer audio driver available on STM3210C-Eval 00146 * eval board. 00147 * @{ 00148 */ 00149 00150 /** @defgroup STM3210C_EVAL_AUDIO_Private_Variables STM3210C EVAL AUDIO Private Variables 00151 * @{ 00152 */ 00153 /*### PLAY ###*/ 00154 static AUDIO_DrvTypeDef *pAudioDrv; 00155 I2S_HandleTypeDef hAudioOutI2s; 00156 00157 /** 00158 * @} 00159 */ 00160 00161 /** @defgroup STM3210C_EVAL_AUDIO_Private_Function_Prototypes STM3210C EVAL AUDIO Private Function Prototypes 00162 * @{ 00163 */ 00164 static void I2SOUT_Init(uint32_t AudioFreq); 00165 static void I2SOUT_DeInit(void); 00166 00167 /** 00168 * @} 00169 */ 00170 00171 /** @defgroup STM3210C_EVAL_AUDIO_OUT_Exported_Functions STM3210C EVAL AUDIO OUT Exported Functions 00172 * @{ 00173 */ 00174 00175 /** 00176 * @brief Configure the audio peripherals. 00177 * @param OutputDevice: OUTPUT_DEVICE_SPEAKER, OUTPUT_DEVICE_HEADPHONE, 00178 * OUTPUT_DEVICE_BOTH or OUTPUT_DEVICE_AUTO . 00179 * @param Volume: Initial volume level (from 0 (Mute) to 100 (Max)) 00180 * @param AudioFreq: Audio frequency used to play the audio stream. 00181 * @retval 0 if correct communication, else wrong communication 00182 */ 00183 uint8_t BSP_AUDIO_OUT_Init(uint16_t OutputDevice, uint8_t Volume, uint32_t AudioFreq) 00184 { 00185 uint8_t ret = AUDIO_ERROR; 00186 00187 /* Disable I2S */ 00188 I2SOUT_DeInit(); 00189 00190 /* I2S data transfer preparation: 00191 Prepare the Media to be used for the audio transfer from memory to I2S peripheral */ 00192 hAudioOutI2s.Instance = I2SOUT; 00193 if(HAL_I2S_GetState(&hAudioOutI2s) == HAL_I2S_STATE_RESET) 00194 { 00195 /* Init the I2S MSP: this __weak function can be redefined by the application*/ 00196 BSP_AUDIO_OUT_MspInit(&hAudioOutI2s, NULL); 00197 } 00198 /* Configure the I2S peripheral */ 00199 I2SOUT_Init(AudioFreq); 00200 00201 if(((cs43l22_drv.ReadID(AUDIO_I2C_ADDRESS)) & CS43L22_ID_MASK) == CS43L22_ID) 00202 { 00203 /* Initialize the audio driver structure */ 00204 pAudioDrv = &cs43l22_drv; 00205 ret = AUDIO_OK; 00206 } 00207 else 00208 { 00209 ret = AUDIO_ERROR; 00210 } 00211 00212 if(ret == AUDIO_OK) 00213 { 00214 pAudioDrv->Init(AUDIO_I2C_ADDRESS, OutputDevice, Volume, AudioFreq); 00215 } 00216 00217 return ret; 00218 } 00219 00220 /** 00221 * @brief De-initialize the audio peripherals. 00222 */ 00223 void BSP_AUDIO_OUT_DeInit(void) 00224 { 00225 I2SOUT_DeInit(); 00226 /* DeInit the I2S MSP : this __weak function can be rewritten by the application */ 00227 BSP_AUDIO_OUT_MspDeInit(&hAudioOutI2s, NULL); 00228 } 00229 00230 /** 00231 * @brief Starts playing audio stream from a data buffer for a determined size. 00232 * @param pBuffer: Pointer to the buffer 00233 * @param Size: Number of audio data BYTES. 00234 * @retval AUDIO_OK if correct communication, else wrong communication 00235 */ 00236 uint8_t BSP_AUDIO_OUT_Play(uint16_t* pBuffer, uint32_t Size) 00237 { 00238 /* Call the audio Codec Play function */ 00239 if(pAudioDrv->Play(AUDIO_I2C_ADDRESS, pBuffer, Size) != 0) 00240 { 00241 return AUDIO_ERROR; 00242 } 00243 else 00244 { 00245 /* Update the Media layer and enable it for play */ 00246 HAL_I2S_Transmit_DMA(&hAudioOutI2s, pBuffer, DMA_MAX(Size)); 00247 00248 return AUDIO_OK; 00249 } 00250 } 00251 00252 /** 00253 * @brief Sends n-Bytes on the I2S interface. 00254 * @param pData: pointer on data address 00255 * @param Size: number of data to be written 00256 */ 00257 void BSP_AUDIO_OUT_ChangeBuffer(uint16_t *pData, uint16_t Size) 00258 { 00259 HAL_I2S_Transmit_DMA(&hAudioOutI2s, pData, Size); 00260 } 00261 00262 /** 00263 * @brief This function Pauses the audio file stream. In case 00264 * of using DMA, the DMA Pause feature is used. 00265 * @note When calling BSP_AUDIO_OUT_Pause() function for pause, only 00266 * BSP_AUDIO_OUT_Resume() function should be called for resume (use of BSP_AUDIO_OUT_Play() 00267 * function for resume could lead to unexpected behavior). 00268 * @retval AUDIO_OK if correct communication, else wrong communication 00269 */ 00270 uint8_t BSP_AUDIO_OUT_Pause(void) 00271 { 00272 /* Call the Audio Codec Pause/Resume function */ 00273 if(pAudioDrv->Pause(AUDIO_I2C_ADDRESS) != 0) 00274 { 00275 return AUDIO_ERROR; 00276 } 00277 else 00278 { 00279 /* Call the Media layer pause function */ 00280 HAL_I2S_DMAPause(&hAudioOutI2s); 00281 00282 /* Return AUDIO_OK if all operations are OK */ 00283 return AUDIO_OK; 00284 } 00285 } 00286 00287 /** 00288 * @brief This function Resumes the audio file stream. 00289 * @note When calling BSP_AUDIO_OUT_Pause() function for pause, only 00290 * BSP_AUDIO_OUT_Resume() function should be called for resume (use of BSP_AUDIO_OUT_Play() 00291 * function for resume could lead to unexpected behavior). 00292 * @retval AUDIO_OK if correct communication, else wrong communication 00293 */ 00294 uint8_t BSP_AUDIO_OUT_Resume(void) 00295 { 00296 /* Call the Audio Codec Pause/Resume function */ 00297 if(pAudioDrv->Resume(AUDIO_I2C_ADDRESS) != 0) 00298 { 00299 return AUDIO_ERROR; 00300 } 00301 else 00302 { 00303 /* Call the Media layer resume function */ 00304 HAL_I2S_DMAResume(&hAudioOutI2s); 00305 /* Return AUDIO_OK if all operations are OK */ 00306 return AUDIO_OK; 00307 } 00308 } 00309 00310 /** 00311 * @brief Stops audio playing and Power down the Audio Codec. 00312 * @param Option: could be one of the following parameters 00313 * - CODEC_PDWN_HW: completely shut down the codec (physically). 00314 * Then need to reconfigure the Codec after power on. 00315 * @retval AUDIO_OK if correct communication, else wrong communication 00316 */ 00317 uint8_t BSP_AUDIO_OUT_Stop(uint32_t Option) 00318 { 00319 /* Call DMA Stop to disable DMA stream before stopping codec */ 00320 HAL_I2S_DMAStop(&hAudioOutI2s); 00321 00322 /* Call Audio Codec Stop function */ 00323 if(pAudioDrv->Stop(AUDIO_I2C_ADDRESS, Option) != 0) 00324 { 00325 return AUDIO_ERROR; 00326 } 00327 else 00328 { 00329 if(Option == CODEC_PDWN_HW) 00330 { 00331 /* Wait at least 100us */ 00332 HAL_Delay(1); 00333 00334 /* Power Down the codec */ 00335 BSP_IO_WritePin(AUDIO_RESET_PIN, GPIO_PIN_RESET); 00336 00337 } 00338 /* Return AUDIO_OK when all operations are correctly done */ 00339 return AUDIO_OK; 00340 } 00341 } 00342 00343 /** 00344 * @brief Controls the current audio volume level. 00345 * @param Volume: Volume level to be set in percentage from 0% to 100% (0 for 00346 * Mute and 100 for Max volume level). 00347 * @retval AUDIO_OK if correct communication, else wrong communication 00348 */ 00349 uint8_t BSP_AUDIO_OUT_SetVolume(uint8_t Volume) 00350 { 00351 /* Call the codec volume control function with converted volume value */ 00352 if(pAudioDrv->SetVolume(AUDIO_I2C_ADDRESS, Volume) != 0) 00353 { 00354 return AUDIO_ERROR; 00355 } 00356 else 00357 { 00358 /* Return AUDIO_OK when all operations are correctly done */ 00359 return AUDIO_OK; 00360 } 00361 } 00362 00363 /** 00364 * @brief Enables or disables the MUTE mode by software 00365 * @param Cmd: could be AUDIO_MUTE_ON to mute sound or AUDIO_MUTE_OFF to 00366 * unmute the codec and restore previous volume level. 00367 * @retval AUDIO_OK if correct communication, else wrong communication 00368 */ 00369 uint8_t BSP_AUDIO_OUT_SetMute(uint32_t Cmd) 00370 { 00371 /* Call the Codec Mute function */ 00372 if(pAudioDrv->SetMute(AUDIO_I2C_ADDRESS, Cmd) != 0) 00373 { 00374 return AUDIO_ERROR; 00375 } 00376 else 00377 { 00378 /* Return AUDIO_OK when all operations are correctly done */ 00379 return AUDIO_OK; 00380 } 00381 } 00382 00383 /** 00384 * @brief Switch dynamically (while audio file is played) the output target 00385 * (speaker or headphone). 00386 * @note This function modifies a global variable of the audio codec driver: OutputDev. 00387 * @param Output: specifies the audio output target: OUTPUT_DEVICE_SPEAKER, 00388 * OUTPUT_DEVICE_HEADPHONE, OUTPUT_DEVICE_BOTH or OUTPUT_DEVICE_AUTO 00389 * @retval AUDIO_OK if correct communication, else wrong communication 00390 */ 00391 uint8_t BSP_AUDIO_OUT_SetOutputMode(uint8_t Output) 00392 { 00393 /* Call the Codec output Device function */ 00394 if(pAudioDrv->SetOutputMode(AUDIO_I2C_ADDRESS, Output) != 0) 00395 { 00396 return AUDIO_ERROR; 00397 } 00398 else 00399 { 00400 /* Return AUDIO_OK when all operations are correctly done */ 00401 return AUDIO_OK; 00402 } 00403 } 00404 00405 /** 00406 * @brief Update the audio frequency. 00407 * @param AudioFreq: Audio frequency used to play the audio stream. 00408 * @note This API should be called after the BSP_AUDIO_OUT_Init() to adjust the 00409 * audio frequency. 00410 */ 00411 void BSP_AUDIO_OUT_SetFrequency(uint32_t AudioFreq) 00412 { 00413 /* Update the I2S audio frequency configuration */ 00414 I2SOUT_Init(AudioFreq); 00415 } 00416 00417 /** 00418 * @brief Initializes BSP_AUDIO_OUT MSP. 00419 * @param hi2s: I2S handle 00420 * @param Params 00421 */ 00422 __weak void BSP_AUDIO_OUT_MspInit(I2S_HandleTypeDef *hi2s, void *Params) 00423 { 00424 static DMA_HandleTypeDef hdma_i2stx; 00425 GPIO_InitTypeDef gpioinitstruct = {0}; 00426 00427 /* Enable I2SOUT clock */ 00428 I2SOUT_CLK_ENABLE(); 00429 00430 /*** Configure the GPIOs ***/ 00431 /* Enable I2S GPIO clocks */ 00432 I2SOUT_SCK_SD_CLK_ENABLE(); 00433 I2SOUT_WS_CLK_ENABLE(); 00434 00435 /* I2SOUT pins configuration: WS, SCK and SD pins -----------------------------*/ 00436 gpioinitstruct.Pin = I2SOUT_SCK_PIN | I2SOUT_SD_PIN; 00437 gpioinitstruct.Mode = GPIO_MODE_AF_PP; 00438 gpioinitstruct.Pull = GPIO_NOPULL; 00439 gpioinitstruct.Speed = GPIO_SPEED_FREQ_MEDIUM; 00440 HAL_GPIO_Init(I2SOUT_SCK_SD_GPIO_PORT, &gpioinitstruct); 00441 00442 gpioinitstruct.Pin = I2SOUT_WS_PIN ; 00443 HAL_GPIO_Init(I2SOUT_WS_GPIO_PORT, &gpioinitstruct); 00444 00445 /* I2SOUT pins configuration: MCK pin */ 00446 I2SOUT_MCK_CLK_ENABLE(); 00447 gpioinitstruct.Pin = I2SOUT_MCK_PIN; 00448 HAL_GPIO_Init(I2SOUT_MCK_GPIO_PORT, &gpioinitstruct); 00449 00450 /* Enable the I2S DMA clock */ 00451 I2SOUT_DMAx_CLK_ENABLE(); 00452 00453 if(hi2s->Instance == I2SOUT) 00454 { 00455 /* Configure the hdma_i2stx handle parameters */ 00456 hdma_i2stx.Init.Direction = DMA_MEMORY_TO_PERIPH; 00457 hdma_i2stx.Init.PeriphInc = DMA_PINC_DISABLE; 00458 hdma_i2stx.Init.MemInc = DMA_MINC_ENABLE; 00459 hdma_i2stx.Init.PeriphDataAlignment = I2SOUT_DMAx_PERIPH_DATA_SIZE; 00460 hdma_i2stx.Init.MemDataAlignment = I2SOUT_DMAx_MEM_DATA_SIZE; 00461 hdma_i2stx.Init.Mode = DMA_NORMAL; 00462 hdma_i2stx.Init.Priority = DMA_PRIORITY_HIGH; 00463 00464 hdma_i2stx.Instance = I2SOUT_DMAx_CHANNEL; 00465 00466 /* Associate the DMA handle */ 00467 __HAL_LINKDMA(hi2s, hdmatx, hdma_i2stx); 00468 00469 /* Deinitialize the Channel for new transfer */ 00470 HAL_DMA_DeInit(&hdma_i2stx); 00471 00472 /* Configure the DMA Channel */ 00473 HAL_DMA_Init(&hdma_i2stx); 00474 } 00475 00476 /* I2S DMA IRQ Channel configuration */ 00477 HAL_NVIC_SetPriority(I2SOUT_DMAx_IRQ, AUDIO_OUT_IRQ_PREPRIO, 0); 00478 HAL_NVIC_EnableIRQ(I2SOUT_DMAx_IRQ); 00479 } 00480 00481 /** 00482 * @brief De-Initializes BSP_AUDIO_OUT MSP. 00483 * @param hi2s: I2S handle 00484 * @param Params 00485 */ 00486 __weak void BSP_AUDIO_OUT_MspDeInit(I2S_HandleTypeDef *hi2s, void *Params) 00487 { 00488 GPIO_InitTypeDef gpioinitstruct; 00489 00490 /* Disable I2S clock */ 00491 I2SOUT_CLK_DISABLE(); 00492 00493 /* CODEC_I2S pins configuration: WS, SCK and SD pins */ 00494 gpioinitstruct.Pin = I2SOUT_WS_PIN | I2SOUT_SCK_PIN | I2SOUT_SD_PIN; 00495 HAL_GPIO_DeInit(I2SOUT_SCK_SD_GPIO_PORT, gpioinitstruct.Pin); 00496 00497 /* CODEC_I2S pins configuration: MCK pin */ 00498 gpioinitstruct.Pin = I2SOUT_MCK_PIN; 00499 HAL_GPIO_DeInit(I2SOUT_MCK_GPIO_PORT, gpioinitstruct.Pin); 00500 } 00501 00502 /** 00503 * @brief Tx Transfer completed callbacks 00504 * @param hi2s: I2S handle 00505 */ 00506 void HAL_I2S_TxCpltCallback(I2S_HandleTypeDef *hi2s) 00507 { 00508 if(hi2s->Instance == I2SOUT) 00509 { 00510 /* Manage the remaining file size and new address offset: This function 00511 should be coded by user (its prototype is already declared in stm3210c_eval_audio.h) */ 00512 BSP_AUDIO_OUT_TransferComplete_CallBack(); 00513 } 00514 } 00515 00516 /** 00517 * @brief Tx Transfer Half completed callbacks 00518 * @param hi2s: I2S handle 00519 */ 00520 void HAL_I2S_TxHalfCpltCallback(I2S_HandleTypeDef *hi2s) 00521 { 00522 if(hi2s->Instance == I2SOUT) 00523 { 00524 /* Manage the remaining file size and new address offset: This function 00525 should be coded by user (its prototype is already declared in stm3210c_eval_audio.h) */ 00526 BSP_AUDIO_OUT_HalfTransfer_CallBack(); 00527 } 00528 } 00529 00530 /** 00531 * @brief I2S error callbacks 00532 * @param hi2s: I2S handle 00533 */ 00534 void HAL_I2S_ErrorCallback(I2S_HandleTypeDef *hi2s) 00535 { 00536 /* Manage the error generated on DMA FIFO: This function 00537 should be coded by user (its prototype is already declared in stm3210c_eval_audio.h) */ 00538 if(hi2s->Instance == I2SOUT) 00539 { 00540 BSP_AUDIO_OUT_Error_CallBack(); 00541 } 00542 } 00543 00544 /** 00545 * @brief Manages the DMA full Transfer complete event. 00546 */ 00547 __weak void BSP_AUDIO_OUT_TransferComplete_CallBack(void) 00548 { 00549 } 00550 00551 /** 00552 * @brief Manages the DMA Half Transfer complete event. 00553 */ 00554 __weak void BSP_AUDIO_OUT_HalfTransfer_CallBack(void) 00555 { 00556 } 00557 00558 /** 00559 * @brief Manages the DMA FIFO error event. 00560 */ 00561 __weak void BSP_AUDIO_OUT_Error_CallBack(void) 00562 { 00563 } 00564 00565 /****************************************************************************** 00566 Static Function 00567 *******************************************************************************/ 00568 00569 /** 00570 * @brief Initializes the Audio Codec audio interface (I2S) 00571 * @param AudioFreq: Audio frequency to be configured for the I2S peripheral. 00572 */ 00573 static void I2SOUT_Init(uint32_t AudioFreq) 00574 { 00575 /* Initialize the hAudioOutI2s Instance parameter */ 00576 hAudioOutI2s.Instance = I2SOUT; 00577 00578 /* Disable I2S block */ 00579 __HAL_I2S_DISABLE(&hAudioOutI2s); 00580 00581 /* I2SOUT peripheral configuration */ 00582 hAudioOutI2s.Init.AudioFreq = AudioFreq; 00583 hAudioOutI2s.Init.CPOL = I2S_CPOL_LOW; 00584 hAudioOutI2s.Init.DataFormat = I2S_DATAFORMAT_16B; 00585 hAudioOutI2s.Init.MCLKOutput = I2S_MCLKOUTPUT_ENABLE; 00586 hAudioOutI2s.Init.Mode = I2S_MODE_MASTER_TX; 00587 hAudioOutI2s.Init.Standard = I2S_STANDARD; 00588 00589 /* Initialize the I2S peripheral with the structure above */ 00590 HAL_I2S_Init(&hAudioOutI2s); 00591 } 00592 00593 /** 00594 * @brief Deinitialize the Audio Codec audio interface (I2S). 00595 */ 00596 static void I2SOUT_DeInit(void) 00597 { 00598 /* Initialize the hAudioOutI2s Instance parameter */ 00599 hAudioOutI2s.Instance = I2SOUT; 00600 00601 /* Disable I2S peripheral */ 00602 __HAL_I2S_DISABLE(&hAudioOutI2s); 00603 00604 HAL_I2S_DeInit(&hAudioOutI2s); 00605 } 00606 00607 /** 00608 * @} 00609 */ 00610 00611 /** 00612 * @} 00613 */ 00614 00615 /** 00616 * @} 00617 */ 00618 00619 /** 00620 * @} 00621 */ 00622 00623 /** 00624 * @} 00625 */ 00626 00627 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Fri Apr 14 2017 13:00:47 for STM3210C_EVAL BSP User Manual by
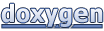