STM3210C_EVAL BSP User Manual
|
stm3210c_eval_io.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm3210c_eval_io.c 00004 * @author MCD Application Team 00005 * @version V7.0.0 00006 * @date 14-April-2017 00007 * @brief This file provides a set of functions needed to manage the IO pins 00008 * on STM3210C-EVAL evaluation board. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* File Info : ----------------------------------------------------------------- 00040 User NOTES 00041 1. How To use this driver: 00042 -------------------------- 00043 - This driver is used to drive the IO module of the STM3210C-EVAL evaluation 00044 board. 00045 - The STMPE811 IO expander device component driver must be included with this 00046 driver in order to run the IO functionalities commanded by the IO expander 00047 device mounted on the evaluation board. 00048 00049 2. Driver description: 00050 --------------------- 00051 + Initialization steps: 00052 o Initialize the IO module using the BSP_IO_Init() function. This 00053 function includes the MSP layer hardware resources initialization and the 00054 communication layer configuration to start the IO functionalities use. 00055 00056 + IO functionalities use 00057 o The IO pin mode is configured when calling the function BSP_IO_ConfigPin(), you 00058 must specify the desired IO mode by choosing the "IO_ModeTypedef" parameter 00059 predefined value. 00060 o If an IO pin is used in interrupt mode, the function BSP_IO_ITGetStatus() is 00061 needed to get the interrupt status. To clear the IT pending bits, you should 00062 call the function BSP_IO_ITClear() with specifying the IO pending bit to clear. 00063 o The IT is handled using the corresponding external interrupt IRQ handler, 00064 the user IT callback treatment is implemented on the same external interrupt 00065 callback. 00066 o To get/set an IO pin combination state you can use the functions 00067 BSP_IO_ReadPin()/BSP_IO_WritePin() or the function BSP_IO_TogglePin() to toggle the pin 00068 state. 00069 00070 ------------------------------------------------------------------------------*/ 00071 00072 /* Includes ------------------------------------------------------------------*/ 00073 #include "stm3210c_eval_io.h" 00074 00075 /** @addtogroup BSP 00076 * @{ 00077 */ 00078 00079 /** @addtogroup STM3210C_EVAL 00080 * @{ 00081 */ 00082 00083 /** @defgroup STM3210C_EVAL_IO STM3210C EVAL IO Expander 00084 * @{ 00085 */ 00086 00087 /* Private variables ---------------------------------------------------------*/ 00088 00089 /** @defgroup STM3210C_EVAL_IO_Private_Variables STM3210C EVAL IO Private Variables 00090 * @{ 00091 */ 00092 static IO_DrvTypeDef *io1_driver; 00093 static IO_DrvTypeDef *io2_driver; 00094 00095 00096 /** 00097 * @} 00098 */ 00099 00100 /* Private functions ---------------------------------------------------------*/ 00101 00102 /** @defgroup STM3210C_EVAL_IO_Exported_Functions STM3210C EVAL IO Exported Functions 00103 * @{ 00104 */ 00105 00106 /** 00107 * @brief Initializes and configures the IO functionalities and configures all 00108 * necessary hardware resources (GPIOs, clocks..). 00109 * @note BSP_IO_Init() is using HAL_Delay() function to ensure that stmpe811 00110 * IO Expander is correctly reset. HAL_Delay() function provides accurate 00111 * delay (in milliseconds) based on variable incremented in SysTick ISR. 00112 * This implies that if BSP_IO_Init() is called from a peripheral ISR process, 00113 * then the SysTick interrupt must have higher priority (numerically lower) 00114 * than the peripheral interrupt. Otherwise the caller ISR process will be blocked. 00115 * @retval IO_OK: if all initializations are OK. Other value if error. 00116 */ 00117 uint8_t BSP_IO_Init(void) 00118 { 00119 uint8_t ret = IO_ERROR; 00120 00121 /* Initialize IO Expander 1*/ 00122 if(stmpe811_io_drv.ReadID(IO1_I2C_ADDRESS) == STMPE811_ID) 00123 { 00124 /* Initialize the IO Expander 1 driver structure */ 00125 io1_driver = &stmpe811_io_drv; 00126 00127 io1_driver->Init(IO1_I2C_ADDRESS); 00128 io1_driver->Start(IO1_I2C_ADDRESS, IO1_PIN_ALL >> IO1_PIN_OFFSET); 00129 00130 ret = IO_OK; 00131 } 00132 00133 /* Initialize IO Expander 2*/ 00134 if(stmpe811_io_drv.ReadID(IO2_I2C_ADDRESS) == STMPE811_ID) 00135 { 00136 /* Initialize the IO Expander 2 driver structure */ 00137 io2_driver = &stmpe811_io_drv; 00138 00139 io2_driver->Init(IO2_I2C_ADDRESS); 00140 io2_driver->Start(IO2_I2C_ADDRESS, IO2_PIN_ALL >> IO2_PIN_OFFSET); 00141 00142 ret = IO_OK; 00143 } 00144 00145 return ret; 00146 } 00147 00148 /** 00149 * @brief Gets the selected pins IT status. 00150 * @param IO_Pin: Selected pins to check the status. 00151 * This parameter can be any combination of the IO pins. 00152 * @retval Status of the checked IO pin(s). 00153 */ 00154 uint32_t BSP_IO_ITGetStatus(uint32_t IO_Pin) 00155 { 00156 uint32_t status = 0; 00157 uint32_t io1_pin = 0; 00158 uint32_t io2_pin = 0; 00159 00160 io1_pin = (IO_Pin & IO1_PIN_ALL) >> IO1_PIN_OFFSET; 00161 io2_pin = (IO_Pin & IO2_PIN_ALL) >> IO2_PIN_OFFSET; 00162 00163 if (io1_pin != 0) 00164 { 00165 /* Return the IO Expander 1 Pin IT status */ 00166 status |= (io1_driver->ITStatus(IO1_I2C_ADDRESS, io1_pin)) << IO1_PIN_OFFSET; 00167 } 00168 00169 if (io2_pin != 0) 00170 { 00171 /* Return the IO Expander 2 Pin IT status */ 00172 status |= (io2_driver->ITStatus(IO2_I2C_ADDRESS, io2_pin)) << IO2_PIN_OFFSET; 00173 } 00174 00175 return status; 00176 } 00177 00178 /** 00179 * @brief Clears the selected IO IT pending bit. 00180 * @param IO_Pin: Selected pins to check the status. 00181 * This parameter can be any combination of the IO pins. 00182 */ 00183 void BSP_IO_ITClear(uint32_t IO_Pin) 00184 { 00185 uint32_t io1_pin = 0; 00186 uint32_t io2_pin = 0; 00187 00188 io1_pin = (IO_Pin & IO1_PIN_ALL) >> IO1_PIN_OFFSET; 00189 io2_pin = (IO_Pin & IO2_PIN_ALL) >> IO2_PIN_OFFSET; 00190 00191 if (io1_pin != 0) 00192 { 00193 /* Clears the selected IO Expander 1 pin(s) mode */ 00194 io1_driver->ClearIT(IO1_I2C_ADDRESS, io1_pin); 00195 } 00196 00197 if (io2_pin != 0) 00198 { 00199 /* Clears the selected IO Expander 2 pin(s) mode */ 00200 io2_driver->ClearIT(IO2_I2C_ADDRESS, io2_pin); 00201 } 00202 } 00203 00204 /** 00205 * @brief Configures the IO pin(s) according to IO mode structure value. 00206 * @param IO_Pin: Output pin to be set or reset. 00207 * This parameter can be any combination of the IO pins. 00208 * @param IO_Mode: IO pin mode to configure 00209 * This parameter can be one of the following values: 00210 * @arg IO_MODE_INPUT 00211 * @arg IO_MODE_OUTPUT 00212 * @arg IO_MODE_IT_RISING_EDGE 00213 * @arg IO_MODE_IT_FALLING_EDGE 00214 * @arg IO_MODE_IT_LOW_LEVEL 00215 * @arg IO_MODE_IT_HIGH_LEVEL 00216 * @retval IO_OK: if all initializations are OK. Other value if error. 00217 */ 00218 uint8_t BSP_IO_ConfigPin(uint32_t IO_Pin, IO_ModeTypedef IO_Mode) 00219 { 00220 uint32_t io1_pin = 0; 00221 uint32_t io2_pin = 0; 00222 00223 io1_pin = (IO_Pin & IO1_PIN_ALL) >> IO1_PIN_OFFSET; 00224 io2_pin = (IO_Pin & IO2_PIN_ALL) >> IO2_PIN_OFFSET; 00225 00226 if (io1_pin != 0) 00227 { 00228 /* Configure the selected IO Expander 1 pin(s) mode */ 00229 io1_driver->Config(IO1_I2C_ADDRESS, io1_pin, IO_Mode); 00230 } 00231 00232 if (io2_pin != 0) 00233 { 00234 /* Configure the selected IO Expander 2 pin(s) mode */ 00235 io2_driver->Config(IO2_I2C_ADDRESS, io2_pin, IO_Mode); 00236 } 00237 00238 return IO_OK; 00239 } 00240 00241 /** 00242 * @brief Sets the selected pins state. 00243 * @param IO_Pin: Selected pins to write. 00244 * This parameter can be any combination of the IO pins. 00245 * @param PinState: New pins state to write 00246 */ 00247 void BSP_IO_WritePin(uint32_t IO_Pin, uint8_t PinState) 00248 { 00249 uint32_t io1_pin = 0; 00250 uint32_t io2_pin = 0; 00251 00252 io1_pin = (IO_Pin & IO1_PIN_ALL) >> IO1_PIN_OFFSET; 00253 io2_pin = (IO_Pin & IO2_PIN_ALL) >> IO2_PIN_OFFSET; 00254 00255 if (io1_pin != 0) 00256 { 00257 /* Sets the IO Expander 1 selected pins state */ 00258 io1_driver->WritePin(IO1_I2C_ADDRESS, io1_pin, PinState); 00259 } 00260 00261 if (io2_pin != 0) 00262 { 00263 /* Sets the IO Expander 2 selected pins state */ 00264 io2_driver->WritePin(IO2_I2C_ADDRESS, io2_pin, PinState); 00265 } 00266 } 00267 00268 /** 00269 * @brief Gets the selected pins current state. 00270 * @param IO_Pin: Selected pins to read. 00271 * This parameter can be any combination of the IO pins. 00272 * @retval The current pins state 00273 */ 00274 uint32_t BSP_IO_ReadPin(uint32_t IO_Pin) 00275 { 00276 uint32_t pin_state = 0; 00277 uint32_t io1_pin = 0; 00278 uint32_t io2_pin = 0; 00279 00280 io1_pin = (IO_Pin & IO1_PIN_ALL) >> IO1_PIN_OFFSET; 00281 io2_pin = (IO_Pin & IO2_PIN_ALL) >> IO2_PIN_OFFSET; 00282 00283 if (io1_pin != 0) 00284 { 00285 /* Gets the IO Expander 1 selected pins current state */ 00286 pin_state |= (io1_driver->ReadPin(IO1_I2C_ADDRESS, io1_pin)) << IO1_PIN_OFFSET; 00287 } 00288 00289 if (io2_pin != 0) 00290 { 00291 /* Gets the IO Expander 2 selected pins current state */ 00292 pin_state |= (io2_driver->ReadPin(IO2_I2C_ADDRESS, io2_pin)) << IO2_PIN_OFFSET; 00293 } 00294 00295 return pin_state; 00296 } 00297 00298 /** 00299 * @brief Toggles the selected pins state 00300 * @param IO_Pin: Selected pins to toggle. 00301 * This parameter can be any combination of the IO pins. 00302 */ 00303 void BSP_IO_TogglePin(uint32_t IO_Pin) 00304 { 00305 uint32_t io1_pin = 0; 00306 uint32_t io2_pin = 0; 00307 00308 io1_pin = (IO_Pin & IO1_PIN_ALL) >> IO1_PIN_OFFSET; 00309 io2_pin = (IO_Pin & IO2_PIN_ALL) >> IO2_PIN_OFFSET; 00310 00311 if (io1_pin != 0) 00312 { 00313 /* Toggles the IO Expander 1 selected pins state */ 00314 if(io1_driver->ReadPin(IO1_I2C_ADDRESS, io1_pin) == RESET) /* Set */ 00315 { 00316 BSP_IO_WritePin(io1_pin, GPIO_PIN_SET); /* Reset */ 00317 } 00318 else 00319 { 00320 BSP_IO_WritePin(io1_pin, GPIO_PIN_RESET); /* Set */ 00321 } 00322 } 00323 00324 if (io2_pin != 0) 00325 { 00326 /* Toggles the IO Expander 2 selected pins state */ 00327 if(io2_driver->ReadPin(IO2_I2C_ADDRESS, io2_pin) == RESET) /* Set */ 00328 { 00329 BSP_IO_WritePin(io2_pin, GPIO_PIN_SET); /* Reset */ 00330 } 00331 else 00332 { 00333 BSP_IO_WritePin(io2_pin, GPIO_PIN_RESET); /* Set */ 00334 } 00335 } 00336 } 00337 00338 /** 00339 * @} 00340 */ 00341 00342 /** 00343 * @} 00344 */ 00345 00346 /** 00347 * @} 00348 */ 00349 00350 /** 00351 * @} 00352 */ 00353 00354 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Fri Apr 14 2017 13:00:47 for STM3210C_EVAL BSP User Manual by
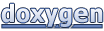