STM3210C_EVAL BSP User Manual
|
stm3210c_eval.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm3210c_eval.c 00004 * @author MCD Application Team 00005 * @version V7.0.0 00006 * @date 14-April-2017 00007 * @brief This file provides a set of firmware functions to manage Leds, 00008 * push-button and COM ports for STM3210C_EVAL 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* Includes ------------------------------------------------------------------*/ 00040 #include "stm3210c_eval.h" 00041 00042 /** @addtogroup BSP 00043 * @{ 00044 */ 00045 00046 /** @defgroup STM3210C_EVAL STM3210C EVAL 00047 * @{ 00048 */ 00049 00050 /** @defgroup STM3210C_EVAL_COMMON STM3210C EVAL Common 00051 * @{ 00052 */ 00053 00054 /** @defgroup STM3210C_EVAL_Private_TypesDefinitions STM3210C EVAL Private TypesDefinitions 00055 * @{ 00056 */ 00057 00058 typedef struct 00059 { 00060 __IO uint16_t LCD_REG_R; /* Read Register */ 00061 __IO uint16_t LCD_RAM_R; /* Read RAM */ 00062 __IO uint16_t LCD_REG_W; /* Write Register */ 00063 __IO uint16_t LCD_RAM_W; /* Write RAM */ 00064 } TFT_LCD_TypeDef; 00065 00066 /** 00067 * @} 00068 */ 00069 00070 /** @defgroup STM3210C_EVAL_Private_Defines STM3210C EVAL Private Defines 00071 * @{ 00072 */ 00073 00074 /* LINK LCD */ 00075 #define START_BYTE 0x70 00076 #define SET_INDEX 0x00 00077 #define READ_STATUS 0x01 00078 #define LCD_WRITE_REG 0x02 00079 #define LCD_READ_REG 0x03 00080 00081 /* LINK SD Card */ 00082 #define SD_DUMMY_BYTE 0xFF 00083 #define SD_NO_RESPONSE_EXPECTED 0x80 00084 00085 /** 00086 * @brief STM3210C EVAL BSP Driver version number 00087 */ 00088 #define __STM3210C_EVAL_BSP_VERSION_MAIN (0x07) /*!< [31:24] main version */ 00089 #define __STM3210C_EVAL_BSP_VERSION_SUB1 (0x00) /*!< [23:16] sub1 version */ 00090 #define __STM3210C_EVAL_BSP_VERSION_SUB2 (0x00) /*!< [15:8] sub2 version */ 00091 #define __STM3210C_EVAL_BSP_VERSION_RC (0x00) /*!< [7:0] release candidate */ 00092 #define __STM3210C_EVAL_BSP_VERSION ((__STM3210C_EVAL_BSP_VERSION_MAIN << 24)\ 00093 |(__STM3210C_EVAL_BSP_VERSION_SUB1 << 16)\ 00094 |(__STM3210C_EVAL_BSP_VERSION_SUB2 << 8 )\ 00095 |(__STM3210C_EVAL_BSP_VERSION_RC)) 00096 00097 00098 /* Note: LCD /CS is CE4 - Bank 4 of NOR/SRAM Bank 1~4 */ 00099 #define TFT_LCD_BASE ((uint32_t)(0x60000000 | 0x0C000000)) 00100 #define TFT_LCD ((TFT_LCD_TypeDef *) TFT_LCD_BASE) 00101 00102 /** 00103 * @} 00104 */ 00105 00106 00107 /** @defgroup STM3210C_EVAL_Private_Variables STM3210C EVAL Private Variables 00108 * @{ 00109 */ 00110 /** 00111 * @brief LED variables 00112 */ 00113 GPIO_TypeDef* LED_PORT[LEDn] = {LED1_GPIO_PORT, 00114 LED2_GPIO_PORT, 00115 LED3_GPIO_PORT, 00116 LED4_GPIO_PORT}; 00117 00118 const uint16_t LED_PIN[LEDn] = {LED1_PIN, 00119 LED2_PIN, 00120 LED3_PIN, 00121 LED4_PIN}; 00122 00123 /** 00124 * @brief BUTTON variables 00125 */ 00126 GPIO_TypeDef* BUTTON_PORT[BUTTONn] = {WAKEUP_BUTTON_GPIO_PORT, 00127 TAMPER_BUTTON_GPIO_PORT, 00128 KEY_BUTTON_GPIO_PORT}; 00129 00130 const uint16_t BUTTON_PIN[BUTTONn] = {WAKEUP_BUTTON_PIN, 00131 TAMPER_BUTTON_PIN, 00132 KEY_BUTTON_PIN}; 00133 00134 const uint16_t BUTTON_IRQn[BUTTONn] = {WAKEUP_BUTTON_EXTI_IRQn, 00135 TAMPER_BUTTON_EXTI_IRQn, 00136 KEY_BUTTON_EXTI_IRQn}; 00137 00138 00139 /** 00140 * @brief COM variables 00141 */ 00142 USART_TypeDef* COM_USART[COMn] = {EVAL_COM1}; 00143 00144 GPIO_TypeDef* COM_TX_PORT[COMn] = {EVAL_COM1_TX_GPIO_PORT}; 00145 00146 GPIO_TypeDef* COM_RX_PORT[COMn] = {EVAL_COM1_RX_GPIO_PORT}; 00147 00148 const uint16_t COM_TX_PIN[COMn] = {EVAL_COM1_TX_PIN}; 00149 00150 const uint16_t COM_RX_PIN[COMn] = {EVAL_COM1_RX_PIN}; 00151 00152 /** 00153 * @brief BUS variables 00154 */ 00155 #ifdef HAL_SPI_MODULE_ENABLED 00156 uint32_t SpixTimeout = EVAL_SPIx_TIMEOUT_MAX; /*<! Value of Timeout when SPI communication fails */ 00157 static SPI_HandleTypeDef heval_Spi; 00158 #endif /* HAL_SPI_MODULE_ENABLED */ 00159 00160 #ifdef HAL_I2C_MODULE_ENABLED 00161 uint32_t I2cxTimeout = EVAL_I2Cx_TIMEOUT_MAX; /*<! Value of Timeout when I2C communication fails */ 00162 I2C_HandleTypeDef heval_I2c; 00163 #endif /* HAL_I2C_MODULE_ENABLED */ 00164 00165 /** 00166 * @} 00167 */ 00168 00169 /* I2Cx bus function */ 00170 #ifdef HAL_I2C_MODULE_ENABLED 00171 /* Link function for I2C EEPROM peripheral */ 00172 static void I2Cx_Init(void); 00173 static void I2Cx_ITConfig(void); 00174 static HAL_StatusTypeDef I2Cx_ReadMultiple(uint8_t Addr, uint16_t Reg, uint16_t MemAddress, uint8_t *Buffer, uint16_t Length); 00175 static HAL_StatusTypeDef I2Cx_ReadBuffer(uint16_t Addr, uint8_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length); 00176 static void I2Cx_WriteData(uint16_t Addr, uint8_t Reg, uint8_t Value); 00177 static HAL_StatusTypeDef I2Cx_WriteBuffer(uint16_t Addr, uint8_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length); 00178 static uint8_t I2Cx_ReadData(uint16_t Addr, uint8_t Reg); 00179 static HAL_StatusTypeDef I2Cx_IsDeviceReady(uint16_t DevAddress, uint32_t Trials); 00180 static void I2Cx_Error(uint8_t Addr); 00181 static void I2Cx_MspInit(I2C_HandleTypeDef *hi2c); 00182 00183 /* Link function for IO Expander over I2C */ 00184 void IOE_Init(void); 00185 void IOE_ITConfig(void); 00186 void IOE_Delay(uint32_t Delay); 00187 void IOE_Write(uint8_t Addr, uint8_t Reg, uint8_t Value); 00188 uint8_t IOE_Read(uint8_t Addr, uint8_t Reg); 00189 uint16_t IOE_ReadMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length); 00190 00191 /* Link function for EEPROM peripheral over I2C */ 00192 void EEPROM_I2C_IO_Init(void); 00193 HAL_StatusTypeDef EEPROM_I2C_IO_WriteData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize); 00194 HAL_StatusTypeDef EEPROM_I2C_IO_ReadData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize); 00195 HAL_StatusTypeDef EEPROM_I2C_IO_IsDeviceReady(uint16_t DevAddress, uint32_t Trials); 00196 00197 /* Link functions for Temperature Sensor peripheral */ 00198 void TSENSOR_IO_Init(void); 00199 void TSENSOR_IO_Write(uint16_t DevAddress, uint8_t* pBuffer, uint8_t WriteAddr, uint16_t Length); 00200 void TSENSOR_IO_Read(uint16_t DevAddress, uint8_t* pBuffer, uint8_t ReadAddr, uint16_t Length); 00201 uint16_t TSENSOR_IO_IsDeviceReady(uint16_t DevAddress, uint32_t Trials); 00202 00203 /* Link function for Audio peripheral */ 00204 void AUDIO_IO_Init(void); 00205 void AUDIO_IO_DeInit(void); 00206 void AUDIO_IO_Write(uint8_t Addr, uint8_t Reg, uint8_t Value); 00207 uint8_t AUDIO_IO_Read(uint8_t Addr, uint8_t Reg); 00208 00209 /* Link function for Accelero peripheral */ 00210 void ACCELERO_IO_Init(void); 00211 void ACCELERO_IO_ITConfig(void); 00212 void ACCELERO_IO_Write(uint8_t* pBuffer, uint8_t WriteAddr, uint16_t NumByteToWrite); 00213 void ACCELERO_IO_Read(uint8_t* pBuffer, uint8_t ReadAddr, uint16_t NumByteToRead); 00214 00215 #endif /* HAL_I2C_MODULE_ENABLED */ 00216 00217 /* SPIx bus function */ 00218 #ifdef HAL_SPI_MODULE_ENABLED 00219 static void SPIx_Init(void); 00220 static void SPIx_Write(uint8_t Value); 00221 static uint32_t SPIx_Read(void); 00222 static void SPIx_WriteReadData(const uint8_t *DataIn, uint8_t *DataOut, uint16_t DataLength); 00223 static void SPIx_WriteData(const uint8_t *Data, uint16_t DataLength); 00224 static void SPIx_ReadData(const uint8_t *Data, uint16_t DataLength); 00225 static void SPIx_Error(void); 00226 static void SPIx_MspInit(void); 00227 00228 /* Link function for LCD peripheral over SPI */ 00229 void LCD_IO_Init(void); 00230 void LCD_IO_WriteMultipleData(uint8_t *pData, uint32_t Size); 00231 void LCD_IO_WriteReg(uint8_t Reg); 00232 uint16_t LCD_IO_ReadData(uint16_t RegValue); 00233 void LCD_Delay (uint32_t delay); 00234 00235 /* Link functions for SD Card peripheral over SPI */ 00236 void SD_IO_Init(void); 00237 void SD_IO_CSState(uint8_t state); 00238 void SD_IO_WriteReadData(const uint8_t *DataIn, uint8_t *DataOut, uint16_t DataLength); 00239 void SD_IO_WriteData(const uint8_t *Data, uint16_t DataLength); 00240 void SD_IO_ReadData(const uint8_t *Data, uint16_t DataLength); 00241 uint8_t SD_IO_WriteByte(uint8_t Data); 00242 #endif /* HAL_SPI_MODULE_ENABLED */ 00243 00244 00245 /** @defgroup STM3210C_EVAL_Exported_Functions STM3210C EVAL Exported Functions 00246 * @{ 00247 */ 00248 00249 /** 00250 * @brief This method returns the STM3210C EVAL BSP Driver revision 00251 * @retval version : 0xXYZR (8bits for each decimal, R for RC) 00252 */ 00253 uint32_t BSP_GetVersion(void) 00254 { 00255 return __STM3210C_EVAL_BSP_VERSION; 00256 } 00257 00258 /** 00259 * @brief Configures LED GPIO. 00260 * @param Led: Specifies the Led to be configured. 00261 * This parameter can be one of following parameters: 00262 * @arg LED1 00263 * @arg LED2 00264 * @arg LED3 00265 * @arg LED4 00266 */ 00267 void BSP_LED_Init(Led_TypeDef Led) 00268 { 00269 GPIO_InitTypeDef gpioinitstruct = {0}; 00270 00271 /* Enable the GPIO_LED clock */ 00272 LEDx_GPIO_CLK_ENABLE(Led); 00273 00274 /* Configure the GPIO_LED pin */ 00275 gpioinitstruct.Pin = LED_PIN[Led]; 00276 gpioinitstruct.Mode = GPIO_MODE_OUTPUT_PP; 00277 gpioinitstruct.Pull = GPIO_NOPULL; 00278 gpioinitstruct.Speed = GPIO_SPEED_FREQ_HIGH; 00279 00280 HAL_GPIO_Init(LED_PORT[Led], &gpioinitstruct); 00281 00282 HAL_GPIO_WritePin(LED_PORT[Led], LED_PIN[Led], GPIO_PIN_RESET); 00283 } 00284 00285 /** 00286 * @brief Turns selected LED On. 00287 * @param Led: Specifies the Led to be set on. 00288 * This parameter can be one of following parameters: 00289 * @arg LED1 00290 * @arg LED2 00291 * @arg LED3 00292 * @arg LED4 00293 */ 00294 void BSP_LED_On(Led_TypeDef Led) 00295 { 00296 HAL_GPIO_WritePin(LED_PORT[Led], LED_PIN[Led], GPIO_PIN_SET); 00297 } 00298 00299 /** 00300 * @brief Turns selected LED Off. 00301 * @param Led: Specifies the Led to be set off. 00302 * This parameter can be one of following parameters: 00303 * @arg LED1 00304 * @arg LED2 00305 * @arg LED3 00306 * @arg LED4 00307 */ 00308 void BSP_LED_Off(Led_TypeDef Led) 00309 { 00310 HAL_GPIO_WritePin(LED_PORT[Led], LED_PIN[Led], GPIO_PIN_RESET); 00311 } 00312 00313 /** 00314 * @brief Toggles the selected LED. 00315 * @param Led: Specifies the Led to be toggled. 00316 * This parameter can be one of following parameters: 00317 * @arg LED1 00318 * @arg LED2 00319 * @arg LED3 00320 * @arg LED4 00321 * @retval None 00322 */ 00323 void BSP_LED_Toggle(Led_TypeDef Led) 00324 { 00325 HAL_GPIO_TogglePin(LED_PORT[Led], LED_PIN[Led]); 00326 } 00327 00328 /** 00329 * @brief Configures push button GPIO and EXTI Line. 00330 * @param Button: Button to be configured. 00331 * This parameter can be one of the following values: 00332 * @arg BUTTON_WAKEUP: Wakeup Push Button 00333 * @arg BUTTON_TAMPER: Tamper Push Button 00334 * @arg BUTTON_KEY: Key Push Button 00335 * @param Button_Mode: Button mode requested. 00336 * This parameter can be one of the following values: 00337 * @arg BUTTON_MODE_GPIO: Button will be used as simple IO 00338 * @arg BUTTON_MODE_EXTI: Button will be connected to EXTI line 00339 * with interrupt generation capability 00340 */ 00341 void BSP_PB_Init(Button_TypeDef Button, ButtonMode_TypeDef Button_Mode) 00342 { 00343 GPIO_InitTypeDef gpioinitstruct = {0}; 00344 00345 /* Enable the corresponding Push Button clock */ 00346 BUTTONx_GPIO_CLK_ENABLE(Button); 00347 00348 /* Configure Push Button pin as input */ 00349 gpioinitstruct.Pin = BUTTON_PIN[Button]; 00350 gpioinitstruct.Pull = GPIO_NOPULL; 00351 gpioinitstruct.Speed = GPIO_SPEED_FREQ_HIGH; 00352 00353 if (Button_Mode == BUTTON_MODE_GPIO) 00354 { 00355 /* Configure Button pin as input */ 00356 gpioinitstruct.Mode = GPIO_MODE_INPUT; 00357 HAL_GPIO_Init(BUTTON_PORT[Button], &gpioinitstruct); 00358 } 00359 else if (Button_Mode == BUTTON_MODE_EXTI) 00360 { 00361 if(Button != BUTTON_WAKEUP) 00362 { 00363 /* Configure Joystick Button pin as input with External interrupt, falling edge */ 00364 gpioinitstruct.Mode = GPIO_MODE_IT_FALLING; 00365 } 00366 else 00367 { 00368 /* Configure Key Push Button pin as input with External interrupt, rising edge */ 00369 gpioinitstruct.Mode = GPIO_MODE_IT_RISING; 00370 } 00371 HAL_GPIO_Init(BUTTON_PORT[Button], &gpioinitstruct); 00372 00373 /* Enable and set Button EXTI Interrupt to the lowest priority */ 00374 HAL_NVIC_SetPriority((IRQn_Type)(BUTTON_IRQn[Button]), 0x0F, 0); 00375 HAL_NVIC_EnableIRQ((IRQn_Type)(BUTTON_IRQn[Button])); 00376 } 00377 } 00378 00379 /** 00380 * @brief Returns the selected button state. 00381 * @param Button: Button to be checked. 00382 * This parameter can be one of the following values: 00383 * @arg BUTTON_TAMPER: Key/Tamper Push Button 00384 * @retval Button state 00385 */ 00386 uint32_t BSP_PB_GetState(Button_TypeDef Button) 00387 { 00388 return HAL_GPIO_ReadPin(BUTTON_PORT[Button], BUTTON_PIN[Button]); 00389 } 00390 00391 #ifdef HAL_I2C_MODULE_ENABLED 00392 /** 00393 * @brief Configures joystick GPIO and EXTI modes. 00394 * @param Joy_Mode: Button mode. 00395 * This parameter can be one of the following values: 00396 * @arg JOY_MODE_GPIO: Joystick pins will be used as simple IOs 00397 * @arg JOY_MODE_EXTI: Joystick pins will be connected to EXTI line 00398 * with interrupt generation capability 00399 * @retval IO_OK: if all initializations are OK. Other value if error. 00400 */ 00401 uint8_t BSP_JOY_Init(JOYMode_TypeDef Joy_Mode) 00402 { 00403 uint8_t ret = 0; 00404 00405 /* Initialize the IO functionalities */ 00406 ret = BSP_IO_Init(); 00407 00408 /* Configure joystick pins in IT mode */ 00409 if((ret == IO_OK) && (Joy_Mode == JOY_MODE_EXTI)) 00410 { 00411 /* Configure joystick pins in IT mode */ 00412 BSP_IO_ConfigPin(JOY_ALL_PINS, IO_MODE_IT_FALLING_EDGE); 00413 } 00414 00415 return ret; 00416 } 00417 00418 /** 00419 * @brief Returns the current joystick status. 00420 * @retval Code of the joystick key pressed 00421 * This code can be one of the following values: 00422 * @arg JOY_NONE 00423 * @arg JOY_SEL 00424 * @arg JOY_DOWN 00425 * @arg JOY_LEFT 00426 * @arg JOY_RIGHT 00427 * @arg JOY_UP 00428 */ 00429 JOYState_TypeDef BSP_JOY_GetState(void) 00430 { 00431 uint32_t tmp = 0; 00432 00433 /* Read the status joystick pins */ 00434 tmp = BSP_IO_ReadPin(JOY_ALL_PINS); 00435 00436 /* Check the pressed keys */ 00437 if((tmp & JOY_NONE_PIN) == JOY_NONE) 00438 { 00439 return(JOYState_TypeDef) JOY_NONE; 00440 } 00441 else if(!(tmp & JOY_SEL_PIN)) 00442 { 00443 return(JOYState_TypeDef) JOY_SEL; 00444 } 00445 else if(!(tmp & JOY_DOWN_PIN)) 00446 { 00447 return(JOYState_TypeDef) JOY_DOWN; 00448 } 00449 else if(!(tmp & JOY_LEFT_PIN)) 00450 { 00451 return(JOYState_TypeDef) JOY_LEFT; 00452 } 00453 else if(!(tmp & JOY_RIGHT_PIN)) 00454 { 00455 return(JOYState_TypeDef) JOY_RIGHT; 00456 } 00457 else if(!(tmp & JOY_UP_PIN)) 00458 { 00459 return(JOYState_TypeDef) JOY_UP; 00460 } 00461 else 00462 { 00463 return(JOYState_TypeDef) JOY_NONE; 00464 } 00465 } 00466 #endif /*HAL_I2C_MODULE_ENABLED*/ 00467 00468 #ifdef HAL_UART_MODULE_ENABLED 00469 /** 00470 * @brief Configures COM port. 00471 * @param COM: Specifies the COM port to be configured. 00472 * This parameter can be one of following parameters: 00473 * @arg COM1 00474 * @param huart: pointer to a UART_HandleTypeDef structure that 00475 * contains the configuration information for the specified UART peripheral. 00476 */ 00477 void BSP_COM_Init(COM_TypeDef COM, UART_HandleTypeDef* huart) 00478 { 00479 GPIO_InitTypeDef gpioinitstruct = {0}; 00480 00481 /* Enable GPIO clock */ 00482 COMx_TX_GPIO_CLK_ENABLE(COM); 00483 COMx_RX_GPIO_CLK_ENABLE(COM); 00484 00485 /* Enable USART clock */ 00486 COMx_CLK_ENABLE(COM); 00487 00488 /* Remap AFIO if needed */ 00489 AFIOCOMx_CLK_ENABLE(COM); 00490 AFIOCOMx_REMAP(COM); 00491 00492 /* Configure USART Tx as alternate function push-pull */ 00493 gpioinitstruct.Pin = COM_TX_PIN[COM]; 00494 gpioinitstruct.Mode = GPIO_MODE_AF_PP; 00495 gpioinitstruct.Speed = GPIO_SPEED_FREQ_HIGH; 00496 gpioinitstruct.Pull = GPIO_PULLUP; 00497 HAL_GPIO_Init(COM_TX_PORT[COM], &gpioinitstruct); 00498 00499 /* Configure USART Rx as alternate function push-pull */ 00500 gpioinitstruct.Mode = GPIO_MODE_INPUT; 00501 gpioinitstruct.Pin = COM_RX_PIN[COM]; 00502 HAL_GPIO_Init(COM_RX_PORT[COM], &gpioinitstruct); 00503 00504 /* USART configuration */ 00505 huart->Instance = COM_USART[COM]; 00506 HAL_UART_Init(huart); 00507 } 00508 #endif /* HAL_UART_MODULE_ENABLED */ 00509 00510 /** 00511 * @} 00512 */ 00513 00514 /** @defgroup STM3210C_EVAL_BusOperations_Functions STM3210C EVAL BusOperations Functions 00515 * @{ 00516 */ 00517 00518 /******************************************************************************* 00519 BUS OPERATIONS 00520 *******************************************************************************/ 00521 00522 #ifdef HAL_I2C_MODULE_ENABLED 00523 /******************************* I2C Routines**********************************/ 00524 00525 /** 00526 * @brief Eval I2Cx MSP Initialization 00527 * @param hi2c: I2C handle 00528 */ 00529 static void I2Cx_MspInit(I2C_HandleTypeDef *hi2c) 00530 { 00531 GPIO_InitTypeDef gpioinitstruct = {0}; 00532 00533 if (hi2c->Instance == EVAL_I2Cx) 00534 { 00535 /*## Configure the GPIOs ################################################*/ 00536 00537 /* Enable GPIO clock */ 00538 EVAL_I2Cx_SDA_GPIO_CLK_ENABLE(); 00539 EVAL_I2Cx_SCL_GPIO_CLK_ENABLE(); 00540 00541 /* Configure I2C Tx as alternate function */ 00542 gpioinitstruct.Pin = EVAL_I2Cx_SCL_PIN; 00543 gpioinitstruct.Mode = GPIO_MODE_AF_OD; 00544 gpioinitstruct.Pull = GPIO_NOPULL; 00545 gpioinitstruct.Speed = GPIO_SPEED_FREQ_HIGH; 00546 HAL_GPIO_Init(EVAL_I2Cx_SCL_GPIO_PORT, &gpioinitstruct); 00547 00548 /* Configure I2C Rx as alternate function */ 00549 gpioinitstruct.Pin = EVAL_I2Cx_SDA_PIN; 00550 HAL_GPIO_Init(EVAL_I2Cx_SDA_GPIO_PORT, &gpioinitstruct); 00551 00552 /*## Configure the Eval I2Cx peripheral #######################################*/ 00553 /* Enable Eval_I2Cx clock */ 00554 EVAL_I2Cx_CLK_ENABLE(); 00555 00556 /* Add delay related to RCC workaround */ 00557 while (READ_BIT(RCC->APB1ENR, RCC_APB1ENR_I2C1EN) != RCC_APB1ENR_I2C1EN) {}; 00558 00559 /* Force the I2C Periheral Clock Reset */ 00560 EVAL_I2Cx_FORCE_RESET(); 00561 00562 /* Release the I2C Periheral Clock Reset */ 00563 EVAL_I2Cx_RELEASE_RESET(); 00564 00565 /* Enable and set Eval I2Cx Interrupt to the highest priority */ 00566 HAL_NVIC_SetPriority(EVAL_I2Cx_EV_IRQn, 0xE, 0); 00567 HAL_NVIC_EnableIRQ(EVAL_I2Cx_EV_IRQn); 00568 00569 /* Enable and set Eval I2Cx Interrupt to the highest priority */ 00570 HAL_NVIC_SetPriority(EVAL_I2Cx_ER_IRQn, 0xE, 0); 00571 HAL_NVIC_EnableIRQ(EVAL_I2Cx_ER_IRQn); 00572 } 00573 } 00574 00575 /** 00576 * @brief Eval I2Cx Bus initialization 00577 */ 00578 static void I2Cx_Init(void) 00579 { 00580 if(HAL_I2C_GetState(&heval_I2c) == HAL_I2C_STATE_RESET) 00581 { 00582 heval_I2c.Instance = EVAL_I2Cx; 00583 heval_I2c.Init.ClockSpeed = BSP_I2C_SPEED; 00584 heval_I2c.Init.DutyCycle = I2C_DUTYCYCLE_2; 00585 heval_I2c.Init.OwnAddress1 = 0; 00586 heval_I2c.Init.AddressingMode = I2C_ADDRESSINGMODE_7BIT; 00587 heval_I2c.Init.DualAddressMode = I2C_DUALADDRESS_DISABLE; 00588 heval_I2c.Init.OwnAddress2 = 0; 00589 heval_I2c.Init.GeneralCallMode = I2C_GENERALCALL_DISABLE; 00590 heval_I2c.Init.NoStretchMode = I2C_NOSTRETCH_DISABLE; 00591 00592 /* Init the I2C */ 00593 I2Cx_MspInit(&heval_I2c); 00594 HAL_I2C_Init(&heval_I2c); 00595 } 00596 } 00597 00598 /** 00599 * @brief Configures I2C Interrupt. 00600 */ 00601 static void I2Cx_ITConfig(void) 00602 { 00603 static uint8_t I2C_IT_Enabled = 0; 00604 GPIO_InitTypeDef gpioinitstruct = {0}; 00605 00606 if(I2C_IT_Enabled == 0) 00607 { 00608 I2C_IT_Enabled = 1; 00609 00610 /* Enable the GPIO EXTI clock */ 00611 IOE_IT_GPIO_CLK_ENABLE(); 00612 00613 gpioinitstruct.Pin = IOE_IT_PIN; 00614 gpioinitstruct.Pull = GPIO_NOPULL; 00615 gpioinitstruct.Speed = GPIO_SPEED_FREQ_HIGH; 00616 gpioinitstruct.Mode = GPIO_MODE_IT_FALLING; 00617 HAL_GPIO_Init(IOE_IT_GPIO_PORT, &gpioinitstruct); 00618 00619 /* Set priority and Enable GPIO EXTI Interrupt */ 00620 HAL_NVIC_SetPriority((IRQn_Type)(IOE_IT_EXTI_IRQn), 0xE, 0); 00621 HAL_NVIC_EnableIRQ((IRQn_Type)(IOE_IT_EXTI_IRQn)); 00622 } 00623 } 00624 00625 /** 00626 * @brief Reads multiple data. 00627 * @param Addr: I2C address 00628 * @param Reg: Reg address 00629 * @param MemAddress: Internal memory address 00630 * @param Buffer: Pointer to data buffer 00631 * @param Length: Length of the data 00632 * @retval Number of read data 00633 */ 00634 static HAL_StatusTypeDef I2Cx_ReadMultiple(uint8_t Addr, uint16_t Reg, uint16_t MemAddress, uint8_t *Buffer, uint16_t Length) 00635 { 00636 HAL_StatusTypeDef status = HAL_OK; 00637 00638 status = HAL_I2C_Mem_Read(&heval_I2c, Addr, (uint16_t)Reg, MemAddress, Buffer, Length, I2cxTimeout); 00639 00640 /* Check the communication status */ 00641 if(status != HAL_OK) 00642 { 00643 /* I2C error occured */ 00644 I2Cx_Error(Addr); 00645 } 00646 return status; 00647 } 00648 00649 /** 00650 * @brief Write a value in a register of the device through BUS. 00651 * @param Addr: Device address on BUS Bus. 00652 * @param Reg: The target register address to write 00653 * @param Value: The target register value to be written 00654 */ 00655 static void I2Cx_WriteData(uint16_t Addr, uint8_t Reg, uint8_t Value) 00656 { 00657 HAL_StatusTypeDef status = HAL_OK; 00658 00659 status = HAL_I2C_Mem_Write(&heval_I2c, Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT, &Value, 1, I2cxTimeout); 00660 00661 /* Check the communication status */ 00662 if(status != HAL_OK) 00663 { 00664 /* Execute user timeout callback */ 00665 I2Cx_Error(Addr); 00666 } 00667 } 00668 00669 /** 00670 * @brief Write a value in a register of the device through BUS. 00671 * @param Addr: Device address on BUS Bus. 00672 * @param Reg: The target register address to write 00673 * @param RegSize: The target register size (can be 8BIT or 16BIT) 00674 * @param pBuffer: The target register value to be written 00675 * @param Length: buffer size to be written 00676 */ 00677 static HAL_StatusTypeDef I2Cx_WriteBuffer(uint16_t Addr, uint8_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length) 00678 { 00679 HAL_StatusTypeDef status = HAL_OK; 00680 00681 status = HAL_I2C_Mem_Write(&heval_I2c, Addr, (uint16_t)Reg, RegSize, pBuffer, Length, I2cxTimeout); 00682 00683 /* Check the communication status */ 00684 if(status != HAL_OK) 00685 { 00686 /* Re-Initiaize the BUS */ 00687 I2Cx_Error(Addr); 00688 } 00689 return status; 00690 } 00691 00692 /** 00693 * @brief Read a value in a register of the device through BUS. 00694 * @param Addr: Device address on BUS Bus. 00695 * @param Reg: The target register address to write 00696 * @retval Data read at register @ 00697 */ 00698 static uint8_t I2Cx_ReadData(uint16_t Addr, uint8_t Reg) 00699 { 00700 HAL_StatusTypeDef status = HAL_OK; 00701 uint8_t value = 0; 00702 00703 status = HAL_I2C_Mem_Read(&heval_I2c, Addr, Reg, I2C_MEMADD_SIZE_8BIT, &value, 1, I2cxTimeout); 00704 00705 /* Check the communication status */ 00706 if(status != HAL_OK) 00707 { 00708 /* Execute user timeout callback */ 00709 I2Cx_Error(Addr); 00710 00711 } 00712 return value; 00713 } 00714 00715 /** 00716 * @brief Reads multiple data on the BUS. 00717 * @param Addr: I2C Address 00718 * @param Reg: Reg Address 00719 * @param RegSize : The target register size (can be 8BIT or 16BIT) 00720 * @param pBuffer: pointer to read data buffer 00721 * @param Length: length of the data 00722 * @retval 0 if no problems to read multiple data 00723 */ 00724 static HAL_StatusTypeDef I2Cx_ReadBuffer(uint16_t Addr, uint8_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length) 00725 { 00726 HAL_StatusTypeDef status = HAL_OK; 00727 00728 status = HAL_I2C_Mem_Read(&heval_I2c, Addr, (uint16_t)Reg, RegSize, pBuffer, Length, I2cxTimeout); 00729 00730 /* Check the communication status */ 00731 if(status != HAL_OK) 00732 { 00733 /* Re-Initiaize the BUS */ 00734 I2Cx_Error(Addr); 00735 } 00736 return status; 00737 } 00738 00739 /** 00740 * @brief Checks if target device is ready for communication. 00741 * @note This function is used with Memory devices 00742 * @param DevAddress: Target device address 00743 * @param Trials: Number of trials 00744 * @retval HAL status 00745 */ 00746 static HAL_StatusTypeDef I2Cx_IsDeviceReady(uint16_t DevAddress, uint32_t Trials) 00747 { 00748 return (HAL_I2C_IsDeviceReady(&heval_I2c, DevAddress, Trials, I2cxTimeout)); 00749 } 00750 00751 /** 00752 * @brief Manages error callback by re-initializing I2C. 00753 * @param Addr: I2C Address 00754 */ 00755 static void I2Cx_Error(uint8_t Addr) 00756 { 00757 /* De-initialize the IOE comunication BUS */ 00758 HAL_I2C_DeInit(&heval_I2c); 00759 00760 /* Re-Initiaize the IOE comunication BUS */ 00761 I2Cx_Init(); 00762 } 00763 00764 #endif /* HAL_I2C_MODULE_ENABLED */ 00765 00766 /******************************* SPI Routines**********************************/ 00767 #ifdef HAL_SPI_MODULE_ENABLED 00768 /** 00769 * @brief Initializes SPI MSP. 00770 */ 00771 static void SPIx_MspInit(void) 00772 { 00773 GPIO_InitTypeDef gpioinitstruct = {0}; 00774 00775 /*** Configure the GPIOs ***/ 00776 /* Enable GPIO clock */ 00777 EVAL_SPIx_SCK_GPIO_CLK_ENABLE(); 00778 EVAL_SPIx_MISO_MOSI_GPIO_CLK_ENABLE(); 00779 __HAL_RCC_AFIO_CLK_ENABLE(); 00780 __HAL_AFIO_REMAP_SPI3_ENABLE(); 00781 00782 /* configure SPI SCK */ 00783 gpioinitstruct.Pin = EVAL_SPIx_SCK_PIN; 00784 gpioinitstruct.Mode = GPIO_MODE_AF_PP; 00785 gpioinitstruct.Pull = GPIO_NOPULL; 00786 gpioinitstruct.Speed = GPIO_SPEED_FREQ_HIGH; 00787 HAL_GPIO_Init(EVAL_SPIx_SCK_GPIO_PORT, &gpioinitstruct); 00788 00789 /* configure SPI MISO and MOSI */ 00790 gpioinitstruct.Pin = (EVAL_SPIx_MISO_PIN | EVAL_SPIx_MOSI_PIN); 00791 gpioinitstruct.Mode = GPIO_MODE_AF_PP; 00792 gpioinitstruct.Pull = GPIO_NOPULL; 00793 gpioinitstruct.Speed = GPIO_SPEED_FREQ_HIGH; 00794 HAL_GPIO_Init(EVAL_SPIx_MISO_MOSI_GPIO_PORT, &gpioinitstruct); 00795 00796 /*** Configure the SPI peripheral ***/ 00797 /* Enable SPI clock */ 00798 EVAL_SPIx_CLK_ENABLE(); 00799 } 00800 00801 /** 00802 * @brief Initializes SPI HAL. 00803 */ 00804 static void SPIx_Init(void) 00805 { 00806 /* DeInitializes the SPI peripheral */ 00807 heval_Spi.Instance = EVAL_SPIx; 00808 HAL_SPI_DeInit(&heval_Spi); 00809 00810 /* SPI Config */ 00811 /* SPI baudrate is set to 9 MHz (PCLK2/SPI_BaudRatePrescaler = 72/8 = 9 MHz) */ 00812 heval_Spi.Init.BaudRatePrescaler = SPI_BAUDRATEPRESCALER_8; 00813 heval_Spi.Init.Direction = SPI_DIRECTION_2LINES; 00814 heval_Spi.Init.CLKPhase = SPI_PHASE_2EDGE; 00815 heval_Spi.Init.CLKPolarity = SPI_POLARITY_HIGH; 00816 heval_Spi.Init.CRCCalculation = SPI_CRCCALCULATION_DISABLE; 00817 heval_Spi.Init.CRCPolynomial = 7; 00818 heval_Spi.Init.DataSize = SPI_DATASIZE_8BIT; 00819 heval_Spi.Init.FirstBit = SPI_FIRSTBIT_MSB; 00820 heval_Spi.Init.NSS = SPI_NSS_SOFT; 00821 heval_Spi.Init.TIMode = SPI_TIMODE_DISABLE; 00822 heval_Spi.Init.Mode = SPI_MODE_MASTER; 00823 00824 SPIx_MspInit(); 00825 if (HAL_SPI_Init(&heval_Spi) != HAL_OK) 00826 { 00827 /* Should not occur */ 00828 while(1) {}; 00829 } 00830 } 00831 00832 /** 00833 * @brief SPI Read 4 bytes from device 00834 * @retval Read data 00835 */ 00836 static uint32_t SPIx_Read(void) 00837 { 00838 HAL_StatusTypeDef status = HAL_OK; 00839 uint32_t readvalue = 0; 00840 uint32_t writevalue = 0xFFFFFFFF; 00841 00842 status = HAL_SPI_TransmitReceive(&heval_Spi, (uint8_t*) &writevalue, (uint8_t*) &readvalue, 1, SpixTimeout); 00843 00844 /* Check the communication status */ 00845 if(status != HAL_OK) 00846 { 00847 /* Execute user timeout callback */ 00848 SPIx_Error(); 00849 } 00850 00851 return readvalue; 00852 } 00853 00854 /** 00855 * @brief SPI Write a byte to device 00856 * @param DataIn: value to be written 00857 * @param DataOut: value to be read 00858 * @param DataLength: length of data 00859 */ 00860 static void SPIx_WriteReadData(const uint8_t *DataIn, uint8_t *DataOut, uint16_t DataLength) 00861 { 00862 HAL_StatusTypeDef status = HAL_OK; 00863 00864 status = HAL_SPI_TransmitReceive(&heval_Spi, (uint8_t*) DataIn, DataOut, DataLength, SpixTimeout); 00865 00866 /* Check the communication status */ 00867 if(status != HAL_OK) 00868 { 00869 /* Execute user timeout callback */ 00870 SPIx_Error(); 00871 } 00872 } 00873 00874 /** 00875 * @brief SPI Write Data to device 00876 * @param Data: value to be written 00877 * @param DataLength: length of data 00878 */ 00879 static void SPIx_WriteData(const uint8_t *Data, uint16_t DataLength) 00880 { 00881 HAL_StatusTypeDef status = HAL_OK; 00882 00883 status = HAL_SPI_Transmit(&heval_Spi, (uint8_t*) Data, DataLength, SpixTimeout); 00884 00885 /* Check the communication status */ 00886 if(status != HAL_OK) 00887 { 00888 /* Execute user timeout callback */ 00889 SPIx_Error(); 00890 } 00891 } 00892 00893 /** 00894 * @brief SPI Read Data from device 00895 * @param Data: value to be read 00896 * @param DataLength: length of data 00897 */ 00898 static void SPIx_ReadData(const uint8_t *Data, uint16_t DataLength) 00899 { 00900 HAL_StatusTypeDef status = HAL_OK; 00901 00902 status = HAL_SPI_Receive(&heval_Spi, (uint8_t*) Data, DataLength, SpixTimeout); 00903 00904 /* Check the communication status */ 00905 if(status != HAL_OK) 00906 { 00907 /* Execute user timeout callback */ 00908 SPIx_Error(); 00909 } 00910 } 00911 00912 /** 00913 * @brief SPI Write a byte to device. 00914 * @param Value: value to be written 00915 */ 00916 static void SPIx_Write(uint8_t Value) 00917 { 00918 HAL_StatusTypeDef status = HAL_OK; 00919 uint8_t data; 00920 00921 status = HAL_SPI_TransmitReceive(&heval_Spi, (uint8_t*) &Value, &data, 1, SpixTimeout); 00922 00923 /* Check the communication status */ 00924 if(status != HAL_OK) 00925 { 00926 /* Execute user timeout callback */ 00927 SPIx_Error(); 00928 } 00929 } 00930 00931 /** 00932 * @brief SPI error treatment function 00933 * @retval None 00934 */ 00935 static void SPIx_Error (void) 00936 { 00937 /* De-initialize the SPI communication BUS */ 00938 HAL_SPI_DeInit(&heval_Spi); 00939 00940 /* Re-Initiaize the SPI communication BUS */ 00941 SPIx_Init(); 00942 } 00943 #endif /* HAL_SPI_MODULE_ENABLED */ 00944 00945 /** 00946 * @} 00947 */ 00948 00949 /** @defgroup STM3210C_EVAL_LinkOperations_Functions STM3210C EVAL LinkOperations Functions 00950 * @{ 00951 */ 00952 00953 /******************************************************************************* 00954 LINK OPERATIONS 00955 *******************************************************************************/ 00956 00957 #ifdef HAL_I2C_MODULE_ENABLED 00958 /***************************** LINK IOE ***************************************/ 00959 00960 /** 00961 * @brief Initializes IOE low level. 00962 */ 00963 void IOE_Init(void) 00964 { 00965 I2Cx_Init(); 00966 } 00967 00968 /** 00969 * @brief Configures IOE low level Interrupt. 00970 */ 00971 void IOE_ITConfig(void) 00972 { 00973 I2Cx_ITConfig(); 00974 } 00975 00976 /** 00977 * @brief IOE writes single data. 00978 * @param Addr: I2C address 00979 * @param Reg: Reg address 00980 * @param Value: Data to be written 00981 */ 00982 void IOE_Write(uint8_t Addr, uint8_t Reg, uint8_t Value) 00983 { 00984 I2Cx_WriteData(Addr, Reg, Value); 00985 } 00986 00987 /** 00988 * @brief IOE reads single data. 00989 * @param Addr: I2C address 00990 * @param Reg: Reg address 00991 * @retval Read data 00992 */ 00993 uint8_t IOE_Read(uint8_t Addr, uint8_t Reg) 00994 { 00995 return I2Cx_ReadData(Addr, Reg); 00996 } 00997 00998 /** 00999 * @brief IOE reads multiple data. 01000 * @param Addr: I2C address 01001 * @param Reg: Reg address 01002 * @param Buffer: Pointer to data buffer 01003 * @param Length: Length of the data 01004 * @retval Number of read data 01005 */ 01006 uint16_t IOE_ReadMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length) 01007 { 01008 return I2Cx_ReadMultiple(Addr, Reg, I2C_MEMADD_SIZE_8BIT, Buffer, Length); 01009 } 01010 01011 /** 01012 * @brief IOE delay. 01013 * @param Delay: Delay in ms 01014 */ 01015 void IOE_Delay(uint32_t Delay) 01016 { 01017 HAL_Delay(Delay); 01018 } 01019 01020 #endif /* HAL_I2C_MODULE_ENABLED */ 01021 01022 #ifdef HAL_SPI_MODULE_ENABLED 01023 /********************************* LINK LCD ***********************************/ 01024 01025 /** 01026 * @brief Configures the LCD_SPI interface. 01027 */ 01028 void LCD_IO_Init(void) 01029 { 01030 GPIO_InitTypeDef gpioinitstruct; 01031 01032 /* Configure the LCD Control pins ------------------------------------------*/ 01033 LCD_NCS_GPIO_CLK_ENABLE(); 01034 01035 /* Configure NCS in Output Push-Pull mode */ 01036 gpioinitstruct.Pin = LCD_NCS_PIN; 01037 gpioinitstruct.Mode = GPIO_MODE_OUTPUT_PP; 01038 gpioinitstruct.Pull = GPIO_NOPULL; 01039 gpioinitstruct.Speed = GPIO_SPEED_FREQ_HIGH; 01040 HAL_GPIO_Init(LCD_NCS_GPIO_PORT, &gpioinitstruct); 01041 01042 /* Set or Reset the control line */ 01043 LCD_CS_LOW(); 01044 LCD_CS_HIGH(); 01045 01046 SPIx_Init(); 01047 } 01048 01049 /** 01050 * @brief Write register value. 01051 * @param pData Pointer on the register value 01052 * @param Size Size of byte to transmit to the register 01053 */ 01054 void LCD_IO_WriteMultipleData(uint8_t *pData, uint32_t Size) 01055 { 01056 uint32_t counter = 0; 01057 01058 /* Reset LCD control line(/CS) and Send data */ 01059 LCD_CS_LOW(); 01060 01061 /* Send Start Byte */ 01062 SPIx_Write(START_BYTE | LCD_WRITE_REG); 01063 01064 for (counter = Size; counter != 0; counter--) 01065 { 01066 while(((heval_Spi.Instance->SR) & SPI_FLAG_TXE) != SPI_FLAG_TXE) 01067 { 01068 } 01069 /* Need to invert bytes for LCD*/ 01070 *((__IO uint8_t*)&heval_Spi.Instance->DR) = *(pData+1); 01071 01072 while(((heval_Spi.Instance->SR) & SPI_FLAG_TXE) != SPI_FLAG_TXE) 01073 { 01074 } 01075 *((__IO uint8_t*)&heval_Spi.Instance->DR) = *pData; 01076 counter--; 01077 pData += 2; 01078 } 01079 01080 /* Wait until the bus is ready before releasing Chip select */ 01081 while(((heval_Spi.Instance->SR) & SPI_FLAG_BSY) != RESET) 01082 { 01083 } 01084 01085 /* Reset LCD control line(/CS) and Send data */ 01086 LCD_CS_HIGH(); 01087 } 01088 01089 /** 01090 * @brief register address. 01091 * @param Reg 01092 */ 01093 void LCD_IO_WriteReg(uint8_t Reg) 01094 { 01095 /* Reset LCD control line(/CS) and Send command */ 01096 LCD_CS_LOW(); 01097 01098 /* Send Start Byte */ 01099 SPIx_Write(START_BYTE | SET_INDEX); 01100 01101 /* Write 16-bit Reg Index (High Byte is 0) */ 01102 SPIx_Write(0x00); 01103 SPIx_Write(Reg); 01104 01105 /* Deselect : Chip Select high */ 01106 LCD_CS_HIGH(); 01107 } 01108 01109 /** 01110 * @brief Read register value. 01111 * @param Reg 01112 */ 01113 uint16_t LCD_IO_ReadData(uint16_t Reg) 01114 { 01115 uint32_t readvalue = 0; 01116 01117 /* Send Reg value to Read */ 01118 LCD_IO_WriteReg(Reg); 01119 01120 /* Reset LCD control line(/CS) and Send command */ 01121 LCD_CS_LOW(); 01122 01123 /* Send Start Byte */ 01124 SPIx_Write(START_BYTE | LCD_READ_REG); 01125 /* Read Upper Byte */ 01126 SPIx_Write(0xFF); 01127 readvalue = SPIx_Read(); 01128 readvalue = readvalue << 8; 01129 readvalue |= SPIx_Read(); 01130 01131 HAL_Delay(10); 01132 01133 /* Deselect : Chip Select high */ 01134 LCD_CS_HIGH(); 01135 return readvalue; 01136 } 01137 01138 /** 01139 * @brief Wait for loop in ms. 01140 * @param Delay in ms. 01141 * @retval None 01142 */ 01143 void LCD_Delay (uint32_t Delay) 01144 { 01145 HAL_Delay(Delay); 01146 } 01147 01148 /******************************** LINK SD Card ********************************/ 01149 01150 /** 01151 * @brief Initializes the SD Card and put it into StandBy State (Ready for 01152 * data transfer). 01153 */ 01154 void SD_IO_Init(void) 01155 { 01156 GPIO_InitTypeDef gpioinitstruct; 01157 uint8_t counter; 01158 01159 /* SD_CS_GPIO and SD_DETECT_GPIO Periph clock enable */ 01160 SD_CS_GPIO_CLK_ENABLE(); 01161 SD_DETECT_GPIO_CLK_ENABLE(); 01162 01163 /* Configure SD_CS_PIN pin: SD Card CS pin */ 01164 gpioinitstruct.Pin = SD_CS_PIN; 01165 gpioinitstruct.Mode = GPIO_MODE_OUTPUT_PP; 01166 gpioinitstruct.Pull = GPIO_PULLUP; 01167 gpioinitstruct.Speed = GPIO_SPEED_FREQ_HIGH; 01168 HAL_GPIO_Init(SD_CS_GPIO_PORT, &gpioinitstruct); 01169 01170 /* Configure SD_DETECT_PIN pin: SD Card detect pin */ 01171 gpioinitstruct.Pin = SD_DETECT_PIN; 01172 gpioinitstruct.Mode = GPIO_MODE_IT_RISING_FALLING; 01173 gpioinitstruct.Pull = GPIO_PULLUP; 01174 HAL_GPIO_Init(SD_DETECT_GPIO_PORT, &gpioinitstruct); 01175 01176 /*------------Put SD in SPI mode--------------*/ 01177 /* SD SPI Config */ 01178 SPIx_Init(); 01179 01180 /* SD chip select high */ 01181 SD_CS_HIGH(); 01182 01183 /* Send dummy byte 0xFF, 10 times with CS high */ 01184 /* Rise CS and MOSI for 80 clocks cycles */ 01185 for (counter = 0; counter <= 9; counter++) 01186 { 01187 /* Send dummy byte 0xFF */ 01188 SD_IO_WriteByte(SD_DUMMY_BYTE); 01189 } 01190 } 01191 01192 /** 01193 * @brief Set the SD_CS pin. 01194 * @param pin value. 01195 * @retval None 01196 */ 01197 void SD_IO_CSState(uint8_t val) 01198 { 01199 if(val == 1) 01200 { 01201 SD_CS_HIGH(); 01202 } 01203 else 01204 { 01205 SD_CS_LOW(); 01206 } 01207 } 01208 01209 /** 01210 * @brief Write a byte on the SD. 01211 * @param DataIn: value to be written 01212 * @param DataOut: value to be read 01213 * @param DataLength: length of data 01214 */ 01215 void SD_IO_WriteReadData(const uint8_t *DataIn, uint8_t *DataOut, uint16_t DataLength) 01216 { 01217 /* Send the byte */ 01218 SPIx_WriteReadData(DataIn, DataOut, DataLength); 01219 } 01220 01221 /** 01222 * @brief Write a byte on the SD. 01223 * @param Data: value to be written 01224 * @param DataLength: length of data 01225 */ 01226 void SD_IO_WriteData(const uint8_t *Data, uint16_t DataLength) 01227 { 01228 /* Send the byte */ 01229 SPIx_WriteData(Data, DataLength); 01230 } 01231 01232 /** 01233 * @brief Read a byte from the SD. 01234 * @param Data: value to be read 01235 * @param DataLength: length of data 01236 */ 01237 void SD_IO_ReadData(const uint8_t *Data, uint16_t DataLength) 01238 { 01239 /* Send the byte */ 01240 SPIx_ReadData(Data, DataLength); 01241 } 01242 01243 /** 01244 * @brief Writes a byte on the SD. 01245 * @param Data: byte to send. 01246 */ 01247 uint8_t SD_IO_WriteByte(uint8_t Data) 01248 { 01249 uint8_t tmp; 01250 /* Send the byte */ 01251 SPIx_WriteReadData(&Data,&tmp,1); 01252 return tmp; 01253 } 01254 01255 #endif /* HAL_SPI_MODULE_ENABLED */ 01256 01257 #ifdef HAL_I2C_MODULE_ENABLED 01258 /********************************* LINK I2C EEPROM *****************************/ 01259 /** 01260 * @brief Initializes peripherals used by the I2C EEPROM driver. 01261 */ 01262 void EEPROM_I2C_IO_Init(void) 01263 { 01264 I2Cx_Init(); 01265 } 01266 01267 /** 01268 * @brief Write data to I2C EEPROM driver 01269 * @param DevAddress: Target device address 01270 * @param MemAddress: Internal memory address 01271 * @param pBuffer: Pointer to data buffer 01272 * @param BufferSize: Amount of data to be sent 01273 * @retval HAL status 01274 */ 01275 HAL_StatusTypeDef EEPROM_I2C_IO_WriteData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize) 01276 { 01277 return (I2Cx_WriteBuffer(DevAddress, MemAddress, I2C_MEMADD_SIZE_16BIT, pBuffer, BufferSize)); 01278 } 01279 01280 /** 01281 * @brief Read data from I2C EEPROM driver 01282 * @param DevAddress: Target device address 01283 * @param MemAddress: Internal memory address 01284 * @param pBuffer: Pointer to data buffer 01285 * @param BufferSize: Amount of data to be read 01286 * @retval HAL status 01287 */ 01288 HAL_StatusTypeDef EEPROM_I2C_IO_ReadData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize) 01289 { 01290 return (I2Cx_ReadBuffer(DevAddress, MemAddress, I2C_MEMADD_SIZE_16BIT, pBuffer, BufferSize)); 01291 } 01292 01293 /** 01294 * @brief Checks if target device is ready for communication. 01295 * @note This function is used with Memory devices 01296 * @param DevAddress: Target device address 01297 * @param Trials: Number of trials 01298 * @retval HAL status 01299 */ 01300 HAL_StatusTypeDef EEPROM_I2C_IO_IsDeviceReady(uint16_t DevAddress, uint32_t Trials) 01301 { 01302 return (I2Cx_IsDeviceReady(DevAddress, Trials)); 01303 } 01304 01305 /********************************* LINK I2C TEMPERATURE SENSOR *****************************/ 01306 /** 01307 * @brief Initializes peripherals used by the I2C Temperature Sensor driver. 01308 * @retval None 01309 */ 01310 void TSENSOR_IO_Init(void) 01311 { 01312 I2Cx_Init(); 01313 } 01314 01315 /** 01316 * @brief Writes one byte to the TSENSOR. 01317 * @param DevAddress: Target device address 01318 * @param pBuffer: Pointer to data buffer 01319 * @param WriteAddr: TSENSOR's internal address to write to. 01320 * @param Length: Number of data to write 01321 */ 01322 void TSENSOR_IO_Write(uint16_t DevAddress, uint8_t* pBuffer, uint8_t WriteAddr, uint16_t Length) 01323 { 01324 I2Cx_WriteBuffer(DevAddress, WriteAddr, I2C_MEMADD_SIZE_8BIT, pBuffer, Length); 01325 } 01326 01327 /** 01328 * @brief Reads one byte from the TSENSOR. 01329 * @param DevAddress: Target device address 01330 * @param pBuffer : pointer to the buffer that receives the data read from the TSENSOR. 01331 * @param ReadAddr : TSENSOR's internal address to read from. 01332 * @param Length: Number of data to read 01333 */ 01334 void TSENSOR_IO_Read(uint16_t DevAddress, uint8_t* pBuffer, uint8_t ReadAddr, uint16_t Length) 01335 { 01336 I2Cx_ReadBuffer(DevAddress, ReadAddr, I2C_MEMADD_SIZE_8BIT, pBuffer, Length); 01337 } 01338 01339 /** 01340 * @brief Checks if Temperature Sensor is ready for communication. 01341 * @param DevAddress: Target device address 01342 * @param Trials: Number of trials 01343 * @retval HAL status 01344 */ 01345 uint16_t TSENSOR_IO_IsDeviceReady(uint16_t DevAddress, uint32_t Trials) 01346 { 01347 return (I2Cx_IsDeviceReady(DevAddress, Trials)); 01348 } 01349 01350 /***************************** LINK ACCELERO *****************************/ 01351 /** 01352 * @brief Configures ACCELEROMETER SPI interface. 01353 */ 01354 void ACCELERO_IO_Init(void) 01355 { 01356 /* Initialize the IO functionalities */ 01357 BSP_IO_Init(); 01358 } 01359 01360 01361 /** 01362 * @brief Configures ACCELERO INT2 config. 01363 EXTI0 is already used by user button so INT1 is configured here 01364 */ 01365 void ACCELERO_IO_ITConfig(void) 01366 { 01367 BSP_IO_ConfigPin(MEMS_ALL_PINS, IO_MODE_IT_FALLING_EDGE); 01368 } 01369 01370 /** 01371 * @brief Writes one byte to the ACCELEROMETER. 01372 * @param pBuffer : pointer to the buffer containing the data to be written to the ACCELEROMETER. 01373 * @param WriteAddr : ACCELEROMETER's internal address to write to. 01374 * @param NumByteToWrite: Number of bytes to write. 01375 */ 01376 void ACCELERO_IO_Write(uint8_t* pBuffer, uint8_t WriteAddr, uint16_t NumByteToWrite) 01377 { 01378 I2Cx_WriteBuffer(L1S302DL_I2C_ADDRESS, WriteAddr, I2C_MEMADD_SIZE_8BIT, pBuffer, NumByteToWrite); 01379 } 01380 01381 /** 01382 * @brief Reads a block of data from the ACCELEROMETER. 01383 * @param pBuffer : pointer to the buffer that receives the data read from the ACCELEROMETER. 01384 * @param ReadAddr : ACCELEROMETER's internal address to read from. 01385 * @param NumByteToRead : number of bytes to read from the ACCELEROMETER. 01386 */ 01387 void ACCELERO_IO_Read(uint8_t* pBuffer, uint8_t ReadAddr, uint16_t NumByteToRead) 01388 { 01389 I2Cx_ReadBuffer(L1S302DL_I2C_ADDRESS, ReadAddr, I2C_MEMADD_SIZE_8BIT, pBuffer, NumByteToRead); 01390 } 01391 01392 /********************************* LINK AUDIO ***********************************/ 01393 01394 /** 01395 * @brief Initializes Audio low level. 01396 */ 01397 void AUDIO_IO_Init(void) 01398 { 01399 /* Initialize the IO functionalities */ 01400 BSP_IO_Init(); 01401 01402 BSP_IO_ConfigPin(AUDIO_RESET_PIN, IO_MODE_OUTPUT); 01403 01404 /* Power Down the codec */ 01405 BSP_IO_WritePin(AUDIO_RESET_PIN, GPIO_PIN_RESET); 01406 01407 /* wait for a delay to insure registers erasing */ 01408 HAL_Delay(5); 01409 01410 /* Power on the codec */ 01411 BSP_IO_WritePin(AUDIO_RESET_PIN, GPIO_PIN_SET); 01412 01413 /* wait for a delay to insure registers erasing */ 01414 HAL_Delay(5); 01415 } 01416 01417 /** 01418 * @brief DeInitializes Audio low level. 01419 * @note This function is intentionally kept empty, user should define it. 01420 */ 01421 void AUDIO_IO_DeInit(void) 01422 { 01423 01424 } 01425 01426 /** 01427 * @brief Writes a single data. 01428 * @param Addr: I2C address 01429 * @param Reg: Reg address 01430 * @param Value: Data to be written 01431 */ 01432 void AUDIO_IO_Write (uint8_t Addr, uint8_t Reg, uint8_t Value) 01433 { 01434 I2Cx_WriteData(Addr, Reg, Value); 01435 } 01436 01437 /** 01438 * @brief Reads a single data. 01439 * @param Addr: I2C address 01440 * @param Reg: Reg address 01441 * @retval Data to be read 01442 */ 01443 uint8_t AUDIO_IO_Read (uint8_t Addr, uint8_t Reg) 01444 { 01445 return I2Cx_ReadData(Addr, Reg); 01446 } 01447 01448 #endif /* HAL_I2C_MODULE_ENABLED */ 01449 01450 /** 01451 * @} 01452 */ 01453 01454 /** 01455 * @} 01456 */ 01457 01458 /** 01459 * @} 01460 */ 01461 01462 /** 01463 * @} 01464 */ 01465 01466 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Fri Apr 14 2017 13:00:47 for STM3210C_EVAL BSP User Manual by
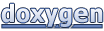