PxVehicleNoDrive Class Reference
[Vehicle]
Data structure with instanced dynamics data and configuration data of a vehicle with no drive model.
More...
#include <PxVehicleNoDrive.h>
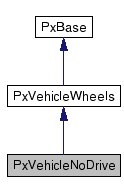

Public Member Functions | |
void | free () |
Deallocate a PxVehicleNoDrive instance. | |
void | setup (PxPhysics *physics, PxRigidDynamic *vehActor, const PxVehicleWheelsSimData &wheelsData) |
Set up a vehicle using simulation data for the wheels. | |
void | setToRestState () |
Set a vehicle to its rest state. Aside from the rigid body transform, this will set the vehicle and rigid body to the state they were in immediately after setup or create. | |
void | setBrakeTorque (const PxU32 id, const PxReal brakeTorque) |
Set the brake torque to be applied to a specific wheel. | |
void | setDriveTorque (const PxU32 id, const PxReal driveTorque) |
Set the drive torque to be applied to a specific wheel. | |
void | setSteerAngle (const PxU32 id, const PxReal steerAngle) |
Set the steer angle to be applied to a specific wheel. | |
PxReal | getBrakeTorque (const PxU32 id) const |
Get the brake torque that has been applied to a specific wheel. | |
PxReal | getDriveTorque (const PxU32 id) const |
Get the drive torque that has been applied to a specific wheel. | |
PxReal | getSteerAngle (const PxU32 id) const |
Get the steer angle that has been applied to a specific wheel. | |
PxVehicleNoDrive (PxBaseFlags baseFlags) | |
virtual void | exportExtraData (PxSerializationContext &) |
void | importExtraData (PxDeserializationContext &) |
virtual const char * | getConcreteTypeName () const |
Returns string name of dynamic type. | |
virtual bool | isKindOf (const char *name) const |
Returns whether a given type name matches with the type of this instance. | |
PxU32 | getNbSteerAngle () const |
PxU32 | getNbDriveTorque () const |
PxU32 | getNbBrakeTorque () const |
Static Public Member Functions | |
static PxVehicleNoDrive * | allocate (const PxU32 nbWheels) |
Allocate a PxVehicleNoDrive instance for a vehicle without drive model and with nbWheels. | |
static PxVehicleNoDrive * | create (PxPhysics *physics, PxRigidDynamic *vehActor, const PxVehicleWheelsSimData &wheelsData) |
Allocate and set up a vehicle using simulation data for the wheels. | |
static PxVehicleNoDrive * | createObject (PxU8 *&address, PxDeserializationContext &context) |
static void | getBinaryMetaData (PxOutputStream &stream) |
Protected Member Functions | |
PxVehicleNoDrive () | |
~PxVehicleNoDrive () | |
Private Member Functions | |
bool | isValid () const |
Test if the instanced dynamics and configuration data has legal values. | |
Private Attributes | |
PxReal * | mSteerAngles |
PxReal * | mDriveTorques |
PxReal * | mBrakeTorques |
PxU32 | mPad [1] |
Friends | |
class | PxVehicleUpdate |
Detailed Description
Data structure with instanced dynamics data and configuration data of a vehicle with no drive model.Constructor & Destructor Documentation
PxVehicleNoDrive::PxVehicleNoDrive | ( | PxBaseFlags | baseFlags | ) | [inline] |
PxVehicleNoDrive::PxVehicleNoDrive | ( | ) | [protected] |
PxVehicleNoDrive::~PxVehicleNoDrive | ( | ) | [inline, protected] |
Member Function Documentation
static PxVehicleNoDrive* PxVehicleNoDrive::allocate | ( | const PxU32 | nbWheels | ) | [static] |
Allocate a PxVehicleNoDrive instance for a vehicle without drive model and with nbWheels.
- Parameters:
-
[in] nbWheels is the number of wheels on the vehicle.
- Returns:
- The instantiated vehicle.
static PxVehicleNoDrive* PxVehicleNoDrive::create | ( | PxPhysics * | physics, | |
PxRigidDynamic * | vehActor, | |||
const PxVehicleWheelsSimData & | wheelsData | |||
) | [static] |
Allocate and set up a vehicle using simulation data for the wheels.
- Parameters:
-
[in] physics is a PxPhysics instance that is needed to create special vehicle constraints that are maintained by the vehicle. [in] vehActor is a PxRigidDynamic instance that is used to represent the vehicle in the PhysX SDK. [in] wheelsData describes the configuration of all suspension/tires/wheels of the vehicle. The vehicle instance takes a copy of this data.
- Note:
- It is assumed that the first shapes of the actor are the wheel shapes, followed by the chassis shapes. To break this assumption use PxVehicleWheels::setWheelShapeMapping.
- Returns:
- The instantiated vehicle.
- See also:
- allocate, free, setToRestState, PxVehicleWheels::setWheelShapeMapping
static PxVehicleNoDrive* PxVehicleNoDrive::createObject | ( | PxU8 *& | address, | |
PxDeserializationContext & | context | |||
) | [static] |
virtual void PxVehicleNoDrive::exportExtraData | ( | PxSerializationContext & | ) | [virtual] |
Reimplemented from PxVehicleWheels.
void PxVehicleNoDrive::free | ( | ) |
static void PxVehicleNoDrive::getBinaryMetaData | ( | PxOutputStream & | stream | ) | [static] |
Reimplemented from PxVehicleWheels.
PxReal PxVehicleNoDrive::getBrakeTorque | ( | const PxU32 | id | ) | const |
Get the brake torque that has been applied to a specific wheel.
- Parameters:
-
[in] id is the wheel being queried for its brake torque
- Returns:
- The brake torque applied to the queried wheel.
virtual const char* PxVehicleNoDrive::getConcreteTypeName | ( | ) | const [inline, virtual] |
Returns string name of dynamic type.
- Returns:
- Class name of most derived type of this object.
Reimplemented from PxVehicleWheels.
PxReal PxVehicleNoDrive::getDriveTorque | ( | const PxU32 | id | ) | const |
Get the drive torque that has been applied to a specific wheel.
- Parameters:
-
[in] id is the wheel being queried for its drive torque
- Returns:
- The drive torque applied to the queried wheel.
PxU32 PxVehicleNoDrive::getNbBrakeTorque | ( | ) | const [inline] |
PxU32 PxVehicleNoDrive::getNbDriveTorque | ( | ) | const [inline] |
PxU32 PxVehicleNoDrive::getNbSteerAngle | ( | ) | const [inline] |
PxReal PxVehicleNoDrive::getSteerAngle | ( | const PxU32 | id | ) | const |
Get the steer angle that has been applied to a specific wheel.
- Parameters:
-
[in] id is the wheel being queried for its steer angle
- Returns:
- The steer angle (in radians) applied to the queried wheel.
void PxVehicleNoDrive::importExtraData | ( | PxDeserializationContext & | ) |
Reimplemented from PxVehicleWheels.
virtual bool PxVehicleNoDrive::isKindOf | ( | const char * | superClass | ) | const [inline, virtual] |
Returns whether a given type name matches with the type of this instance.
Reimplemented from PxVehicleWheels.
References PxBase::isKindOf().
bool PxVehicleNoDrive::isValid | ( | ) | const [private] |
Test if the instanced dynamics and configuration data has legal values.
Reimplemented from PxVehicleWheels.
void PxVehicleNoDrive::setBrakeTorque | ( | const PxU32 | id, | |
const PxReal | brakeTorque | |||
) |
Set the brake torque to be applied to a specific wheel.
- Note:
- The applied brakeTorque persists until the next call to setBrakeTorque
The brake torque is specified in Newton metres.
- Parameters:
-
[in] id is the wheel being given the brake torque [in] brakeTorque is the value of the brake torque
void PxVehicleNoDrive::setDriveTorque | ( | const PxU32 | id, | |
const PxReal | driveTorque | |||
) |
Set the drive torque to be applied to a specific wheel.
- Note:
- The applied driveTorque persists until the next call to setDriveTorque
The brake torque is specified in Newton metres.
- Parameters:
-
[in] id is the wheel being given the brake torque [in] driveTorque is the value of the brake torque
void PxVehicleNoDrive::setSteerAngle | ( | const PxU32 | id, | |
const PxReal | steerAngle | |||
) |
Set the steer angle to be applied to a specific wheel.
- Note:
- The applied steerAngle persists until the next call to setSteerAngle
The steer angle is specified in radians.
- Parameters:
-
[in] id is the wheel being given the steer angle [in] steerAngle is the value of the steer angle in radians.
void PxVehicleNoDrive::setToRestState | ( | ) |
Set a vehicle to its rest state. Aside from the rigid body transform, this will set the vehicle and rigid body to the state they were in immediately after setup or create.
- Note:
- Calling setToRestState invalidates the cached raycast hit planes under each wheel meaning that suspension line raycasts need to be performed at least once with PxVehicleSuspensionRaycasts before calling PxVehicleUpdates.
- See also:
- setup, create, PxVehicleSuspensionRaycasts, PxVehicleUpdates
Reimplemented from PxVehicleWheels.
void PxVehicleNoDrive::setup | ( | PxPhysics * | physics, | |
PxRigidDynamic * | vehActor, | |||
const PxVehicleWheelsSimData & | wheelsData | |||
) |
Set up a vehicle using simulation data for the wheels.
- Parameters:
-
[in] physics is a PxPhysics instance that is needed to create special vehicle constraints that are maintained by the vehicle. [in] vehActor is a PxRigidDynamic instance that is used to represent the vehicle in the PhysX SDK. [in] wheelsData describes the configuration of all suspension/tires/wheels of the vehicle. The vehicle instance takes a copy of this data.
- Note:
- It is assumed that the first shapes of the actor are the wheel shapes, followed by the chassis shapes. To break this assumption use PxVehicleWheels::setWheelShapeMapping.
- See also:
- allocate, free, setToRestState, PxVehicleWheels::setWheelShapeMapping
Friends And Related Function Documentation
friend class PxVehicleUpdate [friend] |
Reimplemented from PxVehicleWheels.
Member Data Documentation
PxReal* PxVehicleNoDrive::mBrakeTorques [private] |
PxReal* PxVehicleNoDrive::mDriveTorques [private] |
PxU32 PxVehicleNoDrive::mPad[1] [private] |
Reimplemented from PxVehicleWheels.
PxReal* PxVehicleNoDrive::mSteerAngles [private] |
The documentation for this class was generated from the following file:
Copyright © 2008-2017 NVIDIA Corporation, 2701 San Tomas Expressway, Santa Clara, CA 95050 U.S.A. All rights reserved. www.nvidia.com