PxHeightField Class Reference
[Geomutils]
A height field class.
More...
#include <PxHeightField.h>
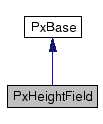
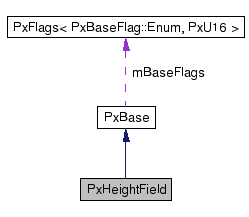
Public Member Functions | |
virtual PX_PHYSX_COMMON_API void | release ()=0 |
Decrements the reference count of a height field and releases it if the new reference count is zero. | |
virtual PX_PHYSX_COMMON_API PxU32 | saveCells (void *destBuffer, PxU32 destBufferSize) const =0 |
Writes out the sample data array. | |
virtual PX_PHYSX_COMMON_API bool | modifySamples (PxI32 startCol, PxI32 startRow, const PxHeightFieldDesc &subfieldDesc, bool shrinkBounds=false)=0 |
Replaces a rectangular subfield in the sample data array. | |
virtual PX_PHYSX_COMMON_API PxU32 | getNbRows () const =0 |
Retrieves the number of sample rows in the samples array. | |
virtual PX_PHYSX_COMMON_API PxU32 | getNbColumns () const =0 |
Retrieves the number of sample columns in the samples array. | |
virtual PX_PHYSX_COMMON_API PxHeightFieldFormat::Enum | getFormat () const =0 |
Retrieves the format of the sample data. | |
virtual PX_PHYSX_COMMON_API PxU32 | getSampleStride () const =0 |
Retrieves the offset in bytes between consecutive samples in the array. | |
virtual PX_PHYSX_COMMON_API PxReal | getThickness () const =0 |
Retrieves the thickness of the height volume in the vertical direction. | |
virtual PX_PHYSX_COMMON_API PxReal | getConvexEdgeThreshold () const =0 |
Retrieves the convex edge threshold. | |
virtual PX_PHYSX_COMMON_API PxHeightFieldFlags | getFlags () const =0 |
Retrieves the flags bits, combined from values of the enum PxHeightFieldFlag. | |
virtual PX_PHYSX_COMMON_API PxReal | getHeight (PxReal x, PxReal z) const =0 |
Retrieves the height at the given coordinates in grid space. | |
virtual PX_PHYSX_COMMON_API PxU32 | getReferenceCount () const =0 |
Returns the reference count for shared heightfields. | |
virtual PX_PHYSX_COMMON_API void | acquireReference ()=0 |
Acquires a counted reference to a heightfield. | |
virtual PX_PHYSX_COMMON_API PxMaterialTableIndex | getTriangleMaterialIndex (PxTriangleID triangleIndex) const =0 |
Returns material table index of given triangle. | |
virtual PX_PHYSX_COMMON_API PxVec3 | getTriangleNormal (PxTriangleID triangleIndex) const =0 |
Returns a triangle face normal for a given triangle index. | |
virtual PX_PHYSX_COMMON_API PxU32 | getTimestamp () const =0 |
Returns the number of times the heightfield data has been modified. | |
virtual PX_PHYSX_COMMON_API const char * | getConcreteTypeName () const |
Returns string name of dynamic type. | |
Protected Member Functions | |
PX_INLINE | PxHeightField (PxType concreteType, PxBaseFlags baseFlags) |
PX_INLINE | PxHeightField (PxBaseFlags baseFlags) |
virtual PX_PHYSX_COMMON_API | ~PxHeightField () |
virtual PX_PHYSX_COMMON_API bool | isKindOf (const char *name) const |
Returns whether a given type name matches with the type of this instance. |
Detailed Description
A height field class.Height fields work in a similar way as triangle meshes specified to act as height fields, with some important differences:
Triangle meshes can be made of nonuniform geometry, while height fields are regular, rectangular grids. This means that with PxHeightField, you sacrifice flexibility in return for improved performance and decreased memory consumption.
In local space rows extend in X direction, columns in Z direction and height in Y direction.
Like Convexes and TriangleMeshes, HeightFields are referenced by shape instances (see PxHeightFieldGeometry, PxShape).
To avoid duplicating data when you have several instances of a particular height field differently, you do not use this class to represent a height field object directly. Instead, you create an instance of this height field via the PxHeightFieldGeometry and PxShape classes.
Creation
To create an instance of this class call PxPhysics::createHeightField() or PxCooking::createHeightField(const PxHeightFieldDesc&, PxPhysicsInsertionCallback&). To delete it call release(). This is only possible once you have released all of its PxHeightFiedShape instances.
Visualizations:
- PxVisualizationParameter::eCOLLISION_AABBS
- PxVisualizationParameter::eCOLLISION_SHAPES
- PxVisualizationParameter::eCOLLISION_AXES
- PxVisualizationParameter::eCOLLISION_FNORMALS
- PxVisualizationParameter::eCOLLISION_EDGES
- See also:
- PxHeightFieldDesc PxHeightFieldGeometry PxShape PxPhysics.createHeightField() PxCooking.createHeightField()
Constructor & Destructor Documentation
PX_INLINE PxHeightField::PxHeightField | ( | PxType | concreteType, | |
PxBaseFlags | baseFlags | |||
) | [inline, protected] |
PX_INLINE PxHeightField::PxHeightField | ( | PxBaseFlags | baseFlags | ) | [inline, protected] |
virtual PX_PHYSX_COMMON_API PxHeightField::~PxHeightField | ( | ) | [inline, protected, virtual] |
Member Function Documentation
virtual PX_PHYSX_COMMON_API void PxHeightField::acquireReference | ( | ) | [pure virtual] |
Acquires a counted reference to a heightfield.
This method increases the reference count of the heightfield by 1. Decrement the reference count by calling release()
virtual PX_PHYSX_COMMON_API const char* PxHeightField::getConcreteTypeName | ( | ) | const [inline, virtual] |
Returns string name of dynamic type.
- Returns:
- Class name of most derived type of this object.
Implements PxBase.
virtual PX_PHYSX_COMMON_API PxReal PxHeightField::getConvexEdgeThreshold | ( | ) | const [pure virtual] |
Retrieves the convex edge threshold.
- Returns:
- The convex edge threshold.
virtual PX_PHYSX_COMMON_API PxHeightFieldFlags PxHeightField::getFlags | ( | ) | const [pure virtual] |
Retrieves the flags bits, combined from values of the enum PxHeightFieldFlag.
- Returns:
- The flags bits, combined from values of the enum PxHeightFieldFlag.
- See also:
- PxHeightFieldDesc.flags PxHeightFieldFlag
virtual PX_PHYSX_COMMON_API PxHeightFieldFormat::Enum PxHeightField::getFormat | ( | ) | const [pure virtual] |
Retrieves the format of the sample data.
- Returns:
- The format of the sample data.
virtual PX_PHYSX_COMMON_API PxReal PxHeightField::getHeight | ( | PxReal | x, | |
PxReal | z | |||
) | const [pure virtual] |
Retrieves the height at the given coordinates in grid space.
- Returns:
- The height at the given coordinates or 0 if the coordinates are out of range.
virtual PX_PHYSX_COMMON_API PxU32 PxHeightField::getNbColumns | ( | ) | const [pure virtual] |
Retrieves the number of sample columns in the samples array.
- Returns:
- The number of sample columns in the samples array.
- See also:
- PxHeightFieldDesc.nbColumns
virtual PX_PHYSX_COMMON_API PxU32 PxHeightField::getNbRows | ( | ) | const [pure virtual] |
Retrieves the number of sample rows in the samples array.
- Returns:
- The number of sample rows in the samples array.
- See also:
- PxHeightFieldDesc.nbRows
virtual PX_PHYSX_COMMON_API PxU32 PxHeightField::getReferenceCount | ( | ) | const [pure virtual] |
Returns the reference count for shared heightfields.
At creation, the reference count of the heightfield is 1. Every shape referencing this heightfield increments the count by 1. When the reference count reaches 0, and only then, the heightfield gets destroyed automatically.
- Returns:
- the current reference count.
virtual PX_PHYSX_COMMON_API PxU32 PxHeightField::getSampleStride | ( | ) | const [pure virtual] |
Retrieves the offset in bytes between consecutive samples in the array.
- Returns:
- The offset in bytes between consecutive samples in the array.
- See also:
- PxHeightFieldDesc.sampleStride
virtual PX_PHYSX_COMMON_API PxReal PxHeightField::getThickness | ( | ) | const [pure virtual] |
Retrieves the thickness of the height volume in the vertical direction.
- Returns:
- The thickness of the height volume in the vertical direction.
- See also:
- PxHeightFieldDesc.thickness
virtual PX_PHYSX_COMMON_API PxU32 PxHeightField::getTimestamp | ( | ) | const [pure virtual] |
Returns the number of times the heightfield data has been modified.
This method returns the number of times modifySamples has been called on this heightfield, so that code that has retained state that depends on the heightfield can efficiently determine whether it has been modified.
- Returns:
- the number of times the heightfield sample data has been modified.
virtual PX_PHYSX_COMMON_API PxMaterialTableIndex PxHeightField::getTriangleMaterialIndex | ( | PxTriangleID | triangleIndex | ) | const [pure virtual] |
Returns material table index of given triangle.
- Note:
- This function takes a post cooking triangle index.
- Parameters:
-
[in] triangleIndex (internal) index of desired triangle
- Returns:
- Material table index, or 0xffff if no per-triangle materials are used
virtual PX_PHYSX_COMMON_API PxVec3 PxHeightField::getTriangleNormal | ( | PxTriangleID | triangleIndex | ) | const [pure virtual] |
Returns a triangle face normal for a given triangle index.
- Note:
- This function takes a post cooking triangle index.
- Parameters:
-
[in] triangleIndex (internal) index of desired triangle
- Returns:
- Triangle normal for a given triangle index
virtual PX_PHYSX_COMMON_API bool PxHeightField::isKindOf | ( | const char * | superClass | ) | const [inline, protected, virtual] |
Returns whether a given type name matches with the type of this instance.
Reimplemented from PxBase.
References PxBase::isKindOf().
virtual PX_PHYSX_COMMON_API bool PxHeightField::modifySamples | ( | PxI32 | startCol, | |
PxI32 | startRow, | |||
const PxHeightFieldDesc & | subfieldDesc, | |||
bool | shrinkBounds = false | |||
) | [pure virtual] |
Replaces a rectangular subfield in the sample data array.
The user provides the description of a rectangular subfield in subfieldDesc. The data is formatted and arranged as PxHeightFieldDesc.samples.
- Parameters:
-
[in] startCol First cell in the destination heightfield to be modified. Can be negative. [in] startRow First row in the destination heightfield to be modified. Can be negative. [in] subfieldDesc Description of the source subfield to read the samples from. [in] shrinkBounds If left as false, the bounds will never shrink but only grow. If set to true the bounds will be recomputed from all HF samples at O(nbColums*nbRows) perf cost.
- Returns:
- True on success, false on failure. Failure can occur due to format mismatch.
- Note:
- Modified samples are constrained to the same height quantization range as the original heightfield. Source samples that are out of range of target heightfield will be clipped with no error. PhysX does not keep a mapping from the heightfield to heightfield shapes that reference it. Call PxShape::setGeometry on each shape which references the height field, to ensure that internal data structures are updated to reflect the new geometry. Please note that PxShape::setGeometry does not guarantee correct/continuous behavior when objects are resting on top of old or new geometry.
virtual PX_PHYSX_COMMON_API void PxHeightField::release | ( | ) | [pure virtual] |
Decrements the reference count of a height field and releases it if the new reference count is zero.
Implements PxBase.
virtual PX_PHYSX_COMMON_API PxU32 PxHeightField::saveCells | ( | void * | destBuffer, | |
PxU32 | destBufferSize | |||
) | const [pure virtual] |
Writes out the sample data array.
The user provides destBufferSize bytes storage at destBuffer. The data is formatted and arranged as PxHeightFieldDesc.samples.
- Parameters:
-
[out] destBuffer The destination buffer for the sample data. [in] destBufferSize The size of the destination buffer.
- Returns:
- The number of bytes written.
- See also:
- PxHeightFieldDesc.samples
The documentation for this class was generated from the following file:
Copyright © 2008-2017 NVIDIA Corporation, 2701 San Tomas Expressway, Santa Clara, CA 95050 U.S.A. All rights reserved. www.nvidia.com