CFile Class Reference
List of all members.Detailed Description
FILE* wrapper. Closes file automatically when leaving scope. Also adds cleaner reading and writing functions as well as formatted text outputting.// Example: bool print_file_info(char* filename) { CFile file; if (!file.open(filename, "rb")) return false; printf("size: %d", file.size()); char c; if (file.read(&c, 1)) printf("%c", c); printf("position: %d", file.position()); return true; }
Definition at line 52 of file CFile.h.
Public Member Functions | |
bool | open (char *fn, char *mode) |
void | close () |
bool | opened () |
bool | read (void *p, unsigned int size) |
bool | write (void *p, unsigned int size) |
bool | printf (char *format,...) |
unsigned long | position () |
bool | seek (unsigned long position) |
int | size () |
Protected Attributes | |
FILE * | f |
File handle. |
Member Function Documentation
|
Same as fopen(fn, mode).
|
Here is the call graph for this function:
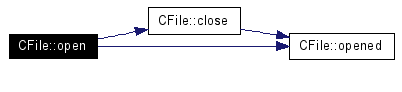
|
Same as fclose(f). |
Here is the call graph for this function:

|
Returns true if the file is opened.
|
|
Reads size bytes from file to p.
|
Here is the call graph for this function:

|
Writes size bytes to file from p.
|
Here is the call graph for this function:

|
Writes formatted text to file. Same as regular printf(...).
|
Here is the call graph for this function:

|
Returns the file position in bytes.
|
Here is the call graph for this function:

|
Seeks position bytes from the beginning of the file.
|
Here is the call graph for this function:

|
Returns the size of the file in bytes.
|
Here is the call graph for this function:

The documentation for this class was generated from the following file:
Generated on Tue Feb 8 21:59:37 2005 for MarSTDv2004 by
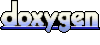