Main Page | Class Hierarchy | Alphabetical List | Class List | Directories | File List | Class Members | File Members
CCsg2D Class Reference
List of all members.Detailed Description
The 2D CSG routines are used to add, subtract and intersect 2D geometry, as in polygons. The algoritm makes no assumptions about in which planes the polygons lie. They could even be in different planes, however this would yield incorrect results. One should make sure geometry lies in the same plane - any plane - and everything will work well. Also, one should note CSG operations are only available for convex polygons. Should you wish to use concave object, please deconstruct your geometry first into convex parts and perform the CSG operation on each part seperately.// Example: void make_shape(CMesh& mesh) { Poly poly1; // poly1 and poly2 will be added together. Poly poly2; Poly poly3; // After poly1 and poly2 are added, poly3 will be subtracted. // my_make_square(CPoly& poly, CVector origin, float size) is a custom function used to create a square with sides length size and centered at origin. my_make_square(poly1, CVector(-1.0, -1.0, 0.0), 2.0); my_make_square(poly2, CVector(+1.0, +1.0, 0.0), 2.0); my_make_square(poly3, CVector( 0.0, 0.0, 0.0), 1.0); // Make sure the mesh is clear. mesh.clear(); // Addition may yield more than one polygon. So we need a mesh to temporarily store the intermediate result, before we subtract poly3. CMesh temp; CCsg2D::I().addition(&poly1, &poly2, &temp); // Subtract poly3 from the intermediate results stored in temp. This is done by for every polygon in temp, calculating the subtraction of poly3. for (CPoly* poly = temp.polyHead; poly; poly = poly->next) CCsg2D::I().subtraction(poly, &poly3, &mesh); }
Definition at line 66 of file CCsg2D.h.
Public Member Functions | |
void | addition (CPoly *poly1, CPoly *poly2, CMesh *out) |
Add two polygons together and store the result in out. | |
void | subtraction (CPoly *poly1, CPoly *poly2, CMesh *out) |
Subtract poly2 from poly1, and store the result in out. | |
void | intersection (CPoly *poly1, CPoly *poly2, CPoly *out) |
Calculate the intersection (overlapping area) of poly1 and poly2, and store the result in out. Note this function stored it's result in a polygon rather than a mesh, because the intersection of 2 convex polygon can never yield more than 1 (convex) polygon. | |
void | exclusiveor (CPoly *poly1, CPoly *poly2, CMesh *out) |
Calculate the inverse of the intersection, and store the result in out. | |
Static Public Member Functions | |
static CCsg2D & | I () |
Member Function Documentation
|
Use this function to get a reference to the 2d csg singleton object. |
The documentation for this class was generated from the following file:
Generated on Tue Feb 8 21:59:34 2005 for MarSTDv2004 by
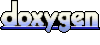