Main Page | Class Hierarchy | Alphabetical List | Class List | Directories | File List | Class Members | File Members
CBrush Class Reference
Collaboration diagram for CBrush: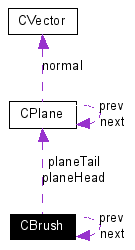
Detailed Description
The brush class is used to define a convex volume enclosed by planes. The brush is defined by planes and can be converted to a mesh. Note the planes' normals must point outwards for a valid volume to be enclosed. Brushes can be added, subtracted and or-ed together using CSG.// Example: void make_random_shape(CMesh& mesh, float radius) { // Probably not the most optimized way to do this. ;) CBrush brush; CVector origin; // Origin = (0.0, 0.0, 0.0). for (int i = 0; i < 3; i++) { CPlane* plane = brush.add(new CPlane); // Make a random normal. for (int j = 0; j < 3; j++) plane->normal[j] = (rand() & 1023) / 1023.0 - 0.5; plane.normal.normalize(); // Offset plane from origin. plane->distance = radius; // Make sure every plane's normal points away from origin. if (plane * origin > 0.0) plane = - plane; } // Convert brush to mesh, and move polygons to output mesh. CMesh* tmp = brush.mesh(); tmp->move(mesh); delete tmp; }
Definition at line 72 of file CBrush.h.
Public Member Functions | |
CPlane * | add (CPlane *plane) |
CPlane * | addHead (CPlane *plane) |
CPlane * | addTail (CPlane *plane) |
void | remove (CPlane *plane) |
CPlane * | unlink (CPlane *plane) |
void | clear () |
bool | convex () |
Returns true if this is a convex brush. | |
CPoly * | initial (CPlane *plane) |
CPoly * | poly (CPlane *plane) |
CMesh * | mesh () |
CEdge * | points () |
CBrush * | copy () |
Returns a copy of the brush. | |
CBrush * | clip (CPlane *plane) |
Clips the brush, returning the resulting brush. | |
CBrush * | unlink () |
Public Attributes | |
CPlane * | planeHead |
First plane in DLL. | |
CPlane * | planeTail |
Last plane in DLL. | |
int | planeCount |
Number of planes in DLL. | |
void * | data |
Custom data field. | |
CBrush * | prev |
CBrush * | next |
Member Function Documentation
|
Creates an "initial" polygon. An initial polygon is a polygon that has a very large size and lies on the specified plane. This "initial" polygon will be used further to clip against the brush's other planes to create one of the brush's side faces.
|
|
Creates a polygon (brush side) for the given plane.
|
|
Convert the brush to a mesh. |
|
Return the corner points of the brush converted to wireframe. This will NOT remove duplicate points. |
The documentation for this class was generated from the following file:
Generated on Tue Feb 8 21:59:30 2005 for MarSTDv2004 by
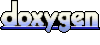