Main Page | Class Hierarchy | Alphabetical List | Class List | Directories | File List | Class Members | File Members
CFile.h
Go to the documentation of this file.00001 #ifndef __Cfile_h__ 00002 #define __Cfile_h__ 00003 00005 // MarSTD version 2004 - (c)2004 - Marcel Smit // 00006 // // 00007 // [email protected] // 00008 // [email protected] // 00009 // // 00010 // This code may not be used in a commercial product without my // 00011 // permission. If you redistribute it, this message must remain // 00012 // intact. If you use this code, some acknowledgement would be // 00013 // appreciated. ;-) // 00015 00017 00018 00019 00020 // NOTE: File closes automatically when leaving scope. 00021 00022 #include <io.h> 00023 #include <stdarg.h> 00024 #include <stdio.h> 00025 00027 00052 class CFile 00053 { 00054 00055 public: 00056 00057 CFile() 00058 { 00059 f = 0; 00060 } 00061 ~CFile() 00062 { 00063 close(); 00064 } 00065 00066 protected: 00067 00068 FILE* f; 00069 00070 public: 00071 00078 bool open(char* fn, char* mode) 00079 { 00080 MASSERT(fn); 00081 close(); 00082 f = fopen(fn, mode); 00083 return opened(); 00084 } 00088 void close() 00089 { 00090 if (opened()) 00091 return; 00092 fclose(f); 00093 f = 0; 00094 } 00099 bool opened() 00100 { 00101 return f == 0 ? false : true; 00102 } 00103 00104 public: 00105 00112 bool read(void* p, unsigned int size) 00113 { 00114 MASSERT(p); 00115 if (!opened()) 00116 return false; 00117 return fread(p, 1, size, f) == size; 00118 } 00125 bool write(void* p, unsigned int size) 00126 { 00127 MASSERT(p); 00128 if (!opened()) 00129 return false; 00130 return fwrite(p, 1, size, f) == size; 00131 } 00138 bool printf(char* format, ...) 00139 { 00140 MASSERT(format); 00141 if (!opened()) 00142 return false; 00143 char text[1024]; 00144 va_list ap; 00145 va_start(ap, format); 00146 vsprintf(text, format, ap); 00147 va_end(ap); 00148 if (fprintf(f, text) <= 0) 00149 return false; 00150 return true; 00151 } 00156 unsigned long position() 00157 { 00158 if (!opened()) 00159 return (unsigned long)(-1); 00160 return ftell(f); 00161 } 00167 bool seek(unsigned long position) 00168 { 00169 if (!opened()) 00170 return false; 00171 if (fseek(f, position, SEEK_SET)) 00172 return false; 00173 return true; 00174 } 00179 int size() 00180 { 00181 if (!opened()) 00182 return -1; 00183 return filelength(fileno(f)); 00184 } 00185 00186 }; 00187 00188 //--------------------------------------------------------------------------- 00189 00190 #endif
Generated on Tue Feb 8 21:59:02 2005 for MarSTDv2004 by
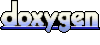