Main Page | Class Hierarchy | Alphabetical List | Class List | Directories | File List | Class Members | File Members
CMatrix.h
Go to the documentation of this file.00001 #ifndef __CMatrix_h__ 00002 #define __CMatrix_h__ 00003 00005 // MarSTD version 2004 - (c)2004 - Marcel Smit // 00006 // // 00007 // Marcel_Smit_101@hotmail.com // 00008 // marcel.athlon@hccnet.nl // 00009 // // 00010 // This code may not be used in a commercial product without my // 00011 // permission. If you redistribute it, this message must remain // 00012 // intact. If you use this code, some acknowledgement would be // 00013 // appreciated. ;-) // 00015 00017 00018 00019 00020 // FIXME: Use custom matrix functions, not Allegro's ones. 00021 // FIXME: Use degress instead of radians? (OpenGL like). 00022 // FIXME: Use true 4x4 matrix, not allegro hack. Same ordering as OpenGL. 00023 00024 #include "CMath.h" 00025 #include "CVector.h" 00026 00027 //--------------------------------------------------------------------------- 00028 00029 #define MATRIX_STACK_SIZE 10 00030 00031 //--------------------------------------------------------------------------- 00032 00034 class CMatrix3 00035 { 00036 00037 public: 00038 00039 CMatrix3(); 00040 ~CMatrix3(); 00041 00042 public: 00043 00044 float v[9]; 00045 00046 public: 00047 00048 void makeIdentity(); 00049 void transpose(); 00050 void makeXRotation(float r); 00051 void makeYRotation(float r); 00052 void makeZRotation(float r); 00053 void makeRotation(float x, float y, float z); 00054 void makeScaling(float x, float y, float z); 00055 void mul(float x, float y, float z, float* xout, float* yout, float* zout); 00056 CVector mul(CVector& vector, CVector& out); 00057 void mul(CMatrix3& matrix); 00058 00059 public: 00060 00061 float determinant(); 00062 void invert(); 00063 00064 public: 00065 00066 float& operator()(int x, int y); 00067 CVector operator*(CVector& vector); 00068 CMatrix3& operator*=(CMatrix3& matrix); 00069 00070 }; 00071 00072 #include "CMatrix4.h" 00073 00074 //--------------------------------------------------------------------------- 00075 00077 00111 class CMatrix 00112 { 00113 00114 public: 00115 00116 CMatrix() 00117 { 00118 depth = 0; 00119 identity(); 00120 } 00121 ~CMatrix() 00122 { 00123 00124 } 00125 00126 public: 00127 00128 CMatrix4 m[MATRIX_STACK_SIZE]; 00129 int depth; 00130 CMatrix4 tmp; 00131 00132 public: 00133 00139 int push() 00140 { 00141 m[depth+1] = m[depth]; 00142 depth++; 00143 return depth; 00144 } 00150 int pop() 00151 { 00152 depth--; 00153 return depth; 00154 } 00159 void mul() 00160 { 00161 m[depth] *= tmp; 00162 } 00163 00164 public: 00165 00169 void identity() 00170 { 00171 m[depth].makeIdentity(); 00172 } 00179 void scale(float x, float y, float z) 00180 { 00181 tmp.makeScaling(x, y, z); 00182 mul(); 00183 } 00189 void scale(CVector vector) 00190 { 00191 scale(vector[0], vector[1], vector[2]); 00192 } 00199 void translate(float x, float y, float z) 00200 { 00201 tmp.makeTranslation(x, y, z); 00202 mul(); 00203 } 00209 void translate(CVector vector) 00210 { 00211 translate(vector[0], vector[1], vector[2]); 00212 } 00219 void rotate(float x, float y, float z) 00220 { 00221 tmp.makeRotation(x, y, z); 00222 mul(); 00223 } 00228 void rotate(CVector vector) 00229 { 00230 rotate(vector[0], vector[1], vector[2]); 00231 } 00239 void st(float sx, float sy, float sz, float tx, float ty, float tz) 00240 { 00241 translate(tx, ty, tz); 00242 scale(sx, sy, sz); 00243 } 00249 void clearRotation() 00250 { 00251 00252 } 00258 void clearTranslation() 00259 { 00260 00261 } 00271 void apply(float x, float y, float z, float& xout, float& yout, float& zout) 00272 { 00273 CVector tmp = m[depth] * CVector(x, y, z); 00274 xout = tmp[0]; 00275 yout = tmp[1]; 00276 zout = tmp[2]; 00277 } 00283 void apply(CVector& v, CVector& vout) 00284 { 00285 vout = m[depth] * v; 00286 } 00287 00288 }; 00289 00290 //--------------------------------------------------------------------------- 00291 00292 #endif
Generated on Tue Feb 8 21:59:02 2005 for MarSTDv2004 by
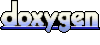