Main Page | Class Hierarchy | Alphabetical List | Class List | Directories | File List | Class Members | File Members
CMatrix4.h
Go to the documentation of this file.00001 #ifndef __CMatrix4_h__ 00002 #define __CMatrix4_h__ 00003 00005 // MarSTD version 2004 - (c)2004 - Marcel Smit // 00006 // // 00007 // [email protected] // 00008 // [email protected] // 00009 // // 00010 // This code may not be used in a commercial product without my // 00011 // permission. If you redistribute it, this message must remain // 00012 // intact. If you use this code, some acknowledgement would be // 00013 // appreciated. ;-) // 00015 00017 00018 00019 00021 00024 class CMatrix4 00025 { 00026 00027 public: 00028 00029 CMatrix4(); 00030 ~CMatrix4(); 00031 00032 public: 00033 00034 float v[16]; 00035 00036 public: 00037 00038 void makeIdentity(); 00039 void transpose(); 00040 void makeXRotation(float r); 00041 void makeYRotation(float r); 00042 void makeZRotation(float r); 00043 void makeRotation(float x, float y, float z); 00044 void makeScaling(float x, float y, float z); 00045 void makeTranslation(float x, float y, float z); 00046 void mul(float x, float y, float z, float w, float* xout, float* yout, float* zout, float* wout); 00047 void mul4x3(float x, float y, float z, float* xout, float* yout, float* zout); 00048 void mul3x3(float x, float y, float z, float* xout, float* yout, float* zout); 00049 void mul(CVector& vector, CVector& out); 00050 void mul4x3(CVector& vector, CVector& out); 00051 void mul3x3(CVector& vector, CVector& out); 00052 void mul(CMatrix4& matrix); 00053 void mul3x3(CMatrix3& matrix); 00054 00078 void mul4x3(CMatrix3& matrix); 00079 void mul(CMatrix4& matrix, CMatrix4& out); 00080 void mul3x3(CMatrix3& matrix, CMatrix4& out); 00081 void mul4x3(CMatrix3& matrix, CMatrix4& out); 00082 00083 public: 00084 00085 float determinant(); 00086 void invert(); 00087 00088 public: 00089 00090 float operator()(int x, int y); 00091 CVector operator*(CVector vector); 00092 CMatrix4 operator*(CMatrix4& matrix); 00093 CMatrix4 operator*(CMatrix3& matrix); 00094 CMatrix4& operator*=(CMatrix4& matrix); 00095 CMatrix4& operator*=(CMatrix3& matrix); 00096 00097 }; 00098 00099 // TODO: look for optimizations in Allegro source code. 00100 00101 class CMatrix4Stack 00102 { 00103 00104 public: 00105 00106 CMatrix4Stack(); 00107 ~CMatrix4Stack(); 00108 00109 public: 00110 00111 std::vector<CMatrix4> stack; 00112 00113 CMatrix4 matrix; 00114 00115 public: 00116 00117 void push(); 00118 void pop(); 00119 00120 }; 00121 00122 //--------------------------------------------------------------------------- 00123 00124 #endif 00125
Generated on Tue Feb 8 21:59:02 2005 for MarSTDv2004 by
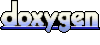