![]() |
Kabylake Intel(R) Firmware Support Package (FSP) Integration Guide
|
GpioConfig.h
Go to the documentation of this file.
64 UINT32 PadMode : 5;
70 UINT32 HostSoftPadOwn : 2;
73 Can choose between In, In with inversion, Out, both In and Out, both In with inversion and out or disabling both.
76 UINT32 Direction : 6;
83 UINT32 OutputState : 2;
90 UINT32 InterruptConfig : 9;
96 UINT32 PowerConfig : 8;
102 UINT32 ElectricalConfig : 9;
108 UINT32 LockConfig : 4;
113 UINT32 OtherSettings : 2;
215 GpioIntEdge = (0x3 << 5), ///< Set interrupt as edge triggered (type of edge depends on input inversion)
220 #define B_GPIO_INT_CONFIG_INT_SOURCE_MASK 0x1F ///< Mask for GPIO_INT_CONFIG for interrupt source
317 #define B_GPIO_ELECTRICAL_CONFIG_TERMINATION_MASK 0x1F ///< Mask for GPIO_ELECTRICAL_CONFIG for termination value
318 #define B_GPIO_ELECTRICAL_CONFIG_1V8_TOLERANCE_MASK 0x60 ///< Mask for GPIO_ELECTRICAL_CONFIG for 1v8 tolerance setting
335 #define B_GPIO_LOCK_CONFIG_PAD_CONF_LOCK_MASK 0x3 ///< Mask for GPIO_LOCK_CONFIG for Pad Configuration Lock
336 #define B_GPIO_LOCK_CONFIG_OUTPUT_LOCK_MASK 0x5 ///< Mask for GPIO_LOCK_CONFIG for Pad Output Lock
GPIO_RESET_CONFIG
GPIO Power Configuration GPIO_RESET_CONFIG allows to set GPIO Reset type (PADCFG_DW0.PadRstCfg) which will be used to reset certain GPIO settings.
Definition: GpioConfig.h:229
GPIO_OUTPUT_STATE
GPIO Output State This field is relevant only if output is enabled.
Definition: GpioConfig.h:181
Deep Sleep Well Reset (DSW_PWROK) GPP: not applicable GPD: PadRstCfg = 00b = "Powergood" Pad settings...
Definition: GpioConfig.h:288
Native function controls pads termination This setting is applicable only to some native modes...
Definition: GpioConfig.h:312
GPIO_OTHER_CONFIG
Other GPIO Configuration GPIO_OTHER_CONFIG is used for less often settings and for future extensions ...
Definition: GpioConfig.h:346
GPIO_ELECTRICAL_CONFIG
GPIO Electrical Configuration Set GPIO termination and Pad Tolerance (applicable only for some pads) ...
Definition: GpioConfig.h:296
GPIO_HOSTSW_OWN
Host Software Pad Ownership modes This setting affects GPIO interrupt status registers.
Definition: GpioConfig.h:146
GPIO_LOCK_CONFIG
GPIO LockConfiguration Set GPIO configuration lock and output state lock.
Definition: GpioConfig.h:329
Set interrupt as edge triggered (type of edge depends on input inversion)
Definition: GpioConfig.h:215
GPP: Reserved; GPD: RSMRST; (PadRstCfg = 11b = "Resume Reset" )
Definition: GpioConfig.h:239
GPIO_INT_CONFIG
GPIO interrupt configuration This setting is applicable only if pad is in GPIO mode and has input ena...
Definition: GpioConfig.h:207
Platform Reset (PLTRST) PadRstCfg = 10b = "GPIO Reset" Pad settings will reset on: ...
Definition: GpioConfig.h:276
Host Deep Reset PadRstCfg = 01b = "Deep GPIO Reset" Pad settings will reset on:
Definition: GpioConfig.h:266
GPIO_PAD_MODE
GPIO Pad Mode Refer to GPIO documentation on native functions available for certain pad...
Definition: GpioConfig.h:132
Generated on Thu Jun 28 2018 21:44:49 for Kabylake Intel(R) Firmware Support Package (FSP) Integration Guide by
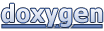