![]() |
Kabylake Intel(R) Firmware Support Package (FSP) Integration Guide
|
FspsUpd.h
Go to the documentation of this file.
74 /// The PCH_DEVICE_INTERRUPT_CONFIG block describes interrupt pin, IRQ and interrupt mode for PCH device.
206 UINT8 SataPortsEnable[8];
212 UINT8 SataPortsDevSlp[8];
218 UINT8 PortUsb20Enable[16];
224 UINT8 PortUsb30Enable[10];
258 UINT8 SerialIoDevMode[11];
265 UINT8 PxRcConfig[8];
318 UINT8 Usb2AfePetxiset[16];
324 UINT8 Usb2AfeTxiset[16];
330 UINT8 Usb2AfePredeemp[16];
336 UINT8 Usb2AfePehalfbit[16];
342 UINT8 Usb3HsioTxDeEmphEnable[10];
348 UINT8 Usb3HsioTxDeEmph[10];
354 UINT8 Usb3HsioTxDownscaleAmpEnable[10];
360 UINT8 Usb3HsioTxDownscaleAmp[10];
377 UINT8 UnusedUpdSpace3[23];
383 UINT8 PcieRpClkReqSupport[24];
389 UINT8 PcieRpClkReqNumber[24];
393 UINT8 UnusedUpdSpace4[5];
404 UINT8 UnusedUpdSpace5[9];
460 UINT8 PcieRpClkSrcNumber[24];
464 UINT8 UnusedUpdSpace6[139];
491 UINT16 PegPhysicalSlotNumber[3];
497 UINT8 PegDeEmphasis[3];
502 UINT8 PegSlotPowerLimitValue[3];
508 UINT8 PegSlotPowerLimitScale[3];
548 UINT8 UnusedUpdSpace7[1];
553 UINT32 VtdBaseAddress[2];
557 UINT8 UnusedUpdSpace8[19];
563 UINT8 SaPostMemProductionRsvd[16];
567 UINT8 UnusedUpdSpace9[7];
573 UINT8 Psi3Enable[5];
579 UINT8 Psi4Enable[5];
585 UINT8 ImonSlope[5];
591 UINT8 ImonOffset[5];
596 UINT8 VrConfigEnable[5];
602 UINT8 TdcEnable[5];
609 UINT8 TdcTimeWindow[5];
615 UINT8 TdcLock[5];
665 UINT8 UnusedUpdSpace10[9];
671 UINT16 TdcPowerLimit[5];
682 UINT8 UnusedUpdSpace11[4];
688 UINT16 AcLoadline[5];
694 UINT16 DcLoadline[5];
700 UINT16 Psi1Threshold[5];
706 UINT16 Psi2Threshold[5];
712 UINT16 Psi3Threshold[5];
717 UINT16 IccMax[5];
722 UINT16 VrVoltageLimit[5];
932 UINT8 PchWriteProtectionEnable[5];
937 UINT8 PchReadProtectionEnable[5];
943 UINT16 PchProtectedRangeLimit[5];
948 UINT16 PchProtectedRangeBase[5];
1212 UINT8 UnusedUpdSpace15[5];
1217 UINT8 PcieRpHotPlug[24];
1222 UINT8 PcieRpPmSci[24];
1227 UINT8 PcieRpExtSync[24];
1232 UINT8 PcieRpTransmitterHalfSwing[24];
1237 UINT8 PcieRpClkReqDetect[24];
1242 UINT8 PcieRpAdvancedErrorReporting[24];
1247 UINT8 PcieRpUnsupportedRequestReport[24];
1252 UINT8 PcieRpFatalErrorReport[24];
1257 UINT8 PcieRpNoFatalErrorReport[24];
1262 UINT8 PcieRpCorrectableErrorReport[24];
1267 UINT8 PcieRpSystemErrorOnFatalError[24];
1272 UINT8 PcieRpSystemErrorOnNonFatalError[24];
1277 UINT8 PcieRpSystemErrorOnCorrectableError[24];
1282 UINT8 PcieRpMaxPayload[24];
1287 UINT8 PcieRpDeviceResetPadActiveHigh[24];
1293 UINT8 PcieRpPcieSpeed[24];
1299 UINT8 PcieRpGen3EqPh3Method[24];
1304 UINT8 PcieRpPhysicalSlotNumber[24];
1307 The root port completion timeout(see: PCH_PCIE_COMPLETION_TIMEOUT). Default is PchPcieCompletionTO_Default.
1309 UINT8 PcieRpCompletionTimeout[24];
1315 UINT32 PcieRpDeviceResetPad[24];
1321 UINT8 PcieRpAspm[24];
1327 UINT8 PcieRpL1Substates[24];
1332 UINT8 PcieRpLtrEnable[24];
1337 UINT8 PcieRpLtrConfigLock[24];
1342 UINT8 PcieEqPh3LaneParamCm[24];
1347 UINT8 PcieEqPh3LaneParamCp[24];
1352 UINT8 PcieSwEqCoeffListCm[5];
1357 UINT8 PcieSwEqCoeffListCp[5];
1412 UINT8 UnusedUpdSpace16[5];
1415 Corresponds to the WOL Enable Override bit in the General PM Configuration B (GEN_PMCON_B) register.
1427 Determine if WLAN wake from Sx, corresponds to the HOST_WLAN_PP_EN bit in the PWRM_CFG3 register.
1473 UINT8 UnusedUpdSpace17[6];
1571 UINT8 SataPortsHotPlug[8];
1576 UINT8 SataPortsInterlockSw[8];
1581 UINT8 SataPortsExternal[8];
1586 UINT8 SataPortsSpinUp[8];
1591 UINT8 SataPortsSolidStateDrive[8];
1596 UINT8 SataPortsEnableDitoConfig[8];
1601 UINT8 SataPortsDmVal[8];
1606 UINT16 SataPortsDitoVal[8];
1611 UINT8 SataPortsZpOdd[8];
1688 UINT8 SataRstPcieEnable[3];
1693 UINT8 SataRstPcieStoragePort[3];
1698 UINT8 SataRstPcieDeviceResetDelay[3];
1736 UINT8 SerialIoI2cVoltage[6];
1741 UINT8 SerialIoSpiCsPolarity[2];
1746 UINT8 SerialIoUartHwFlowCtrl[3];
1942 UINT8 PchMemoryPmsyncEnable[2];
1947 UINT8 PchMemoryC0TransmitEnable[2];
1952 UINT8 PchMemoryPinSelection[2];
1969 UINT8 Usb2OverCurrentPin[16];
1974 UINT8 Usb3OverCurrentPin[10];
1998 UINT8 UnusedUpdSpace19[2];
2028 UINT8 UnusedUpdSpace20[5];
2040 UINT64 BgpdtHash[4];
2059 UINT8 UnusedUpdSpace21[3];
2091 UINT8 ReservedFspsUpd[5];
2139 UINT8 PegMaxPayload[3];
2183 UINT8 SaPostMemTestRsvd[11];
2376 UINT8 UnusedUpdSpace23[2];
2428 UINT8 UnusedUpdSpace24[2];
2655 UINT16 StateRatio[40];
2690 Package Long duration turbo mode power limit. Units are based on POWER_MGMT_CONFIG.CustomPowerUnit.
2696 Package Short duration turbo mode power limit. Units are based on POWER_MGMT_CONFIG.CustomPowerUnit.Valid
2720 Short term Power Limit value for custom cTDP level 1. Units are based on POWER_MGMT_CONFIG.CustomPowerUnit.Valid
2726 Long term Power Limit value for custom cTDP level 1. Units are based on POWER_MGMT_CONFIG.CustomPowerUnit.Valid
2732 Short term Power Limit value for custom cTDP level 2. Units are based on POWER_MGMT_CONFIG.CustomPowerUnit.Valid
2738 Long term Power Limit value for custom cTDP level 2. Units are based on POWER_MGMT_CONFIG.CustomPowerUnit.Valid
2744 Short term Power Limit value for custom cTDP level 3. Units are based on POWER_MGMT_CONFIG.CustomPowerUnit.Valid
2750 Long term Power Limit value for custom cTDP level 3. Units are based on POWER_MGMT_CONFIG.CustomPowerUnit.Valid
2818 UINT8 ReservedCpuPostMemTest[1];
2878 UINT16 PcieRpLtrMaxSnoopLatency[24];
2883 UINT16 PcieRpLtrMaxNoSnoopLatency[24];
2888 UINT8 PcieRpSnoopLatencyOverrideMode[24];
2893 UINT8 PcieRpSnoopLatencyOverrideMultiplier[24];
2898 UINT16 PcieRpSnoopLatencyOverrideValue[24];
2903 UINT8 PcieRpNonSnoopLatencyOverrideMode[24];
2908 UINT8 PcieRpNonSnoopLatencyOverrideMultiplier[24];
2913 UINT16 PcieRpNonSnoopLatencyOverrideValue[24];
2918 UINT8 PcieRpSlotPowerLimitScale[24];
2923 UINT16 PcieRpSlotPowerLimitValue[24];
2928 UINT8 PcieRpUptp[24];
2933 UINT8 PcieRpDptp[24];
2967 UINT8 ReservedFspsTestUpd[15];
2988 UINT8 UnusedUpdSpace26[470];
UINT8 Early8254ClockGatingEnable
Offset 0x071F - Enable 8254 Static Clock Gating in early POST time Set 8254CGE=1 is required for C11 ...
Definition: FspsUpd.h:1982
UINT32 PsysPowerLimit2Power
Offset 0x0878 - Platform PL2 power Platform PL2 power.
Definition: FspsUpd.h:2765
UINT8 ThreeCoreRatioLimit
Offset 0x079E - 3-Core Ratio Limit 3-Core Ratio Limit: LFM to Fused max, For overclocking part: LFM t...
Definition: FspsUpd.h:2203
UINT8 TcoIrqEnable
Offset 0x008A - Enable/Disable Tco IRQ Enable/disable TCO IRQ $EN_DIS.
Definition: FspsUpd.h:286
UINT8 PchHdaIDispLinkFrequency
Offset 0x033A - iDisp-Link Frequency iDisp-Link Freq (PCH_HDAUDIO_LINK_FREQUENCY enum): 4: 96MHz...
Definition: FspsUpd.h:974
UINT8 PchLanClkReqNumber
Offset 0x0362 - CLKREQ# used by GbE Valid if ClkReqSupported is TRUE.
Definition: FspsUpd.h:1173
UINT16 CstateLatencyControl1Irtl
Offset 0x083E - Interrupt Response Time Limit of C-State LatencyContol1 Interrupt Response Time Limit...
Definition: FspsUpd.h:2667
UINT16 PchSkyCamPortBDataTrimValue
Offset 0x0312 - Enable SkyCam Port B Data Trim Value Enable/disable Port B Data Trim Value...
Definition: FspsUpd.h:910
UINT8 PchSkyCamPortACtleCapValue
Offset 0x0306 - Enable SkyCam PortA Ctle Cap Value Enable/disable PortA Ctle Cap Value.
Definition: FspsUpd.h:855
UINT8 PchTTLock
Offset 0x06E5 - Thermal Throttle Lock Thermal Throttle Lock.
Definition: FspsUpd.h:1819
UINT32 Custom3PowerLimit2
Offset 0x0870 - Long term Power Limit value for custom cTDP level 3 Long term Power Limit value for c...
Definition: FspsUpd.h:2753
UINT16 WatchDogTimerBios
Offset 0x015A - BIOS Timer 16 bits Value, Set BIOS watchdog timer.
Definition: FspsUpd.h:447
UINT8 SlowSlewRateForIa
Offset 0x027A - Slew Rate configuration for Deep Package C States for VR IA domain Slew Rate configur...
Definition: FspsUpd.h:647
UINT8 CridEnable
Offset 0x0204 - Enable/Disable SA CRID Enable: SA CRID, Disable (Default): SA CRID $EN_DIS...
Definition: FspsUpd.h:480
UINT8 MeUnconfigOnRtcClear
Offset 0x0778 - Enable/Disable ME Unconfig on RTC clear Enable(Default): Enable ME Unconfig On Rtc Cl...
Definition: FspsUpd.h:2075
UINT8 PchSkyCamPortCDCtleCapValue
Offset 0x0308 - Enable SkyCam PortCD Ctle Cap Value Enable/disable PortCD Ctle Cap Value...
Definition: FspsUpd.h:865
UINT8 PchPmPmcReadDisable
Offset 0x0A2F - PCH Pm Pmc Read Disable Deprecated $EN_DIS.
Definition: FspsUpd.h:2957
UINT16 PchT2Level
Offset 0x06E0 - Thermal Throttling Custimized T2Level Value Custimized T2Level value.
Definition: FspsUpd.h:1794
UINT8 PchPmWoWlanDeepSxEnable
Offset 0x0643 - PCH Pm WoW lan DeepSx Enable Determine if WLAN wake from DeepSx, corresponds to the D...
Definition: FspsUpd.h:1437
UINT32 PchHdaDspPpModuleMask
Offset 0x0344 - Bitmask of supported DSP Pre/Post-Processing Modules Deprecated: Specific pre/post-pr...
Definition: FspsUpd.h:1017
UINT8 SataSalpSupport
Offset 0x0041 - Enable SATA SALP Support Enable/disable SATA Aggressive Link Power Management...
Definition: FspsUpd.h:200
UINT8 PchPmWolEnableOverride
Offset 0x0640 - PCH Pm Wol Enable Override Corresponds to the WOL Enable Override bit in the General ...
Definition: FspsUpd.h:1418
UINT8 PchSkyCamPortDClkTrimValue
Offset 0x030F - Enable SkyCam PortD Clk Trim Value Enable/disable PortD Clk Trim Value.
Definition: FspsUpd.h:900
UINT8 SataP0TDispFinit
Offset 0x06F7 - Port 0 Alternate Fast Init Tdispatch Port 0 Alternate Fast Init Tdispatch.
Definition: FspsUpd.h:1914
UINT8 PsysPowerLimit2
Offset 0x07BB - PL2 Enable Value PL2 Enable activates the PL2 value to limit average platform power...
Definition: FspsUpd.h:2372
UINT8 TTCrossThrottling
Offset 0x06E7 - Enable PCH Cross Throttling Enable/Disable PCH Cross Throttling $EN_DIS.
Definition: FspsUpd.h:1831
UINT8 SataRstCpuAttachedStorage
Offset 0x0721 - PCH SATA RST CPU attached storage RST CPU attached storage $EN_DIS.
Definition: FspsUpd.h:1994
UINT8 NumberOfEntries
Offset 0x07AD - Custom Ratio State Entries The number of custom ratio state entries, ranges from 0 to 40 for a valid custom ratio table.Sets the number of custom P-states.
Definition: FspsUpd.h:2296
UINT8 PchHdaDspEndpointBluetooth
Offset 0x033F - DSP Bluetooth enablement 0: Disable; 1: Enable.
Definition: FspsUpd.h:1003
UINT8 EnergyEfficientTurbo
Offset 0x07CF - Enable or Disable Energy Efficient Turbo Enable or Disable Energy Efficient Turbo...
Definition: FspsUpd.h:2481
UINT16 PchSubSystemVendorId
Offset 0x0366 - PCH Sub system vendor ID Default Subsystem Vendor ID of the PCH devices.
Definition: FspsUpd.h:1197
UINT8 EdramTestMode
Offset 0x0786 - EDRAM Test Mode Enable: PAM register will not be locked by RC, platform code should l...
Definition: FspsUpd.h:2120
UINT16 CstateLatencyControl2Irtl
Offset 0x0840 - Interrupt Response Time Limit of C-State LatencyContol2 Interrupt Response Time Limit...
Definition: FspsUpd.h:2672
UINT8 PchLockDownBiosInterface
Offset 0x0890 - Enable LOCKDOWN BIOS Interface Enable BIOS Interface Lock Down bit to prevent writes ...
Definition: FspsUpd.h:2854
UINT8 PchPmSlpStrchSusUp
Offset 0x0651 - PCH Pm Slp Strch Sus Up Enable SLP_X Stretching After SUS Well Power Up...
Definition: FspsUpd.h:1485
UINT8 PchSkyCamPortBCtleEnable
Offset 0x0304 - Enable SkyCam PortB Ctle Enable/disable PortB Ctle.
Definition: FspsUpd.h:844
UINT32 CpuS3ResumeData
Offset 0x0880 - CpuS3ResumeData Pointer to CPU S3 Resume Data.
Definition: FspsUpd.h:2781
UINT8 C1e
Offset 0x07D9 - Enable or Disable Enhanced C-states Enable or Disable Enhanced C-states.
Definition: FspsUpd.h:2541
UINT8 PpmIrmSetting
Offset 0x07E6 - Interrupt Redirection Mode Select Interrupt Redirection Mode Select.0: Fixed priority; 1: Round robin;2: Hash vector;4: PAIR with fixed priority;5: PAIR with round robin;6: PAIR with hash vector;7: No change.
Definition: FspsUpd.h:2622
UINT8 PchIshGp7GpioAssign
Offset 0x035D - Enable PCH ISH GP_7 GPIO pin assigned 0: Disable; 1: Enable.
Definition: FspsUpd.h:1144
UINT8 IslVrCmd
Offset 0x077A - Activates VR mailbox command for Intersil VR C-state issues.
Definition: FspsUpd.h:2087
UINT64 BiosGuardModulePtr
Offset 0x0758 - BiosGuardModulePtr BiosGuardModulePtr default values.
Definition: FspsUpd.h:2045
UINT32 PowerLimit4
Offset 0x0854 - Package PL4 power limit Package PL4 power limit.
Definition: FspsUpd.h:2711
UINT8 SerialIoEnableDebugUartAfterPost
Offset 0x06D7 - Enable Debug UART Controller Enable debug UART controller after post.
Definition: FspsUpd.h:1756
UINT8 FastPkgCRampDisableSa
Offset 0x02E0 - Disable Fast Slew Rate for Deep Package C States for VR SA domain Disable Fast Slew R...
Definition: FspsUpd.h:740
UINT8 SixCoreRatioLimit
Offset 0x0885 - 6-Core Ratio Limit 6-Core Ratio Limit: LFM to Fused max, For overclocking part: LFM t...
Definition: FspsUpd.h:2793
UINT8 PsysPowerLimit1Time
Offset 0x07BA - PL1 timewindow PL1 timewindow in seconds.Valid values(Unit in seconds) 0 to 8 ...
Definition: FspsUpd.h:2365
UINT8 PchLanEnable
Offset 0x00FB - Enable LAN Enable/disable LAN controller.
Definition: FspsUpd.h:366
UINT32 Custom1PowerLimit2
Offset 0x0860 - Long term Power Limit value for custom cTDP level 1 Long term Power Limit value for c...
Definition: FspsUpd.h:2729
UINT8 PchSkyCamPortCTermOvrEnable
Offset 0x02FD - Enable SkyCam PortC Termination override Enable/disable PortC Termination override...
Definition: FspsUpd.h:802
UINT8 PchIshGp0GpioAssign
Offset 0x0356 - Enable PCH ISH GP_0 GPIO pin assigned 0: Disable; 1: Enable.
Definition: FspsUpd.h:1102
UINT8 CstateLatencyControl3TimeUnit
Offset 0x07E3 - TimeUnit for C-State Latency Control3 TimeUnit for C-State Latency Control3;Valid val...
Definition: FspsUpd.h:2604
UINT8 BiProcHot
Offset 0x07D1 - Enable or Disable Bi-Directional PROCHOT# Enable or Disable Bi-Directional PROCHOT#; ...
Definition: FspsUpd.h:2493
UINT8 PchMemoryThrottlingEnable
Offset 0x06FB - Enable Memory Thermal Throttling Enable Memory Thermal Throttling.
Definition: FspsUpd.h:1937
UINT8 PchLockDownBiosLock
Offset 0x0363 - Enable LOCKDOWN BIOS LOCK Enable the BIOS Lock feature and set EISS bit (D31:F5:RegDC...
Definition: FspsUpd.h:1180
UINT8 PchCrid
Offset 0x0365 - PCH Compatibility Revision ID This member describes whether or not the CRID feature o...
Definition: FspsUpd.h:1192
UINT8 PchCio2Enable
Offset 0x0030 - Enable CIO2 Controller Enable/disable SKYCAM CIO2 Controller.
Definition: FspsUpd.h:137
UINT32 PchHdaVerbTablePtr
Offset 0x008C - PCH HDA Verb Table Pointer Pointer to Array of pointers to Verb Table.
Definition: FspsUpd.h:296
UINT8 OneCoreRatioLimit
Offset 0x079C - 1-Core Ratio Limit 1-Core Ratio Limit: LFM to Fused max, For overclocking part: LFM t...
Definition: FspsUpd.h:2191
UINT8 PkgCStateUnDemotion
Offset 0x07DB - Enable or Disable Package C-State UnDemotion Enable or Disable Package C-State UnDemo...
Definition: FspsUpd.h:2555
UINT16 PchHdaResetWaitTimer
Offset 0x088E - HD Audio Reset Wait Timer The delay timer after Azalia reset, the value is number of ...
Definition: FspsUpd.h:2848
UINT8 EnergyEfficientPState
Offset 0x07CE - Enable or Disable Energy Efficient P-state Enable or Disable Energy Efficient P-state...
Definition: FspsUpd.h:2474
UINT16 PchSkyCamPortADataTrimValue
Offset 0x0310 - Enable SkyCam Port A Data Trim Value Enable/disable Port A Data Trim Value...
Definition: FspsUpd.h:905
UINT16 PchSkyCamPortCDDataTrimValue
Offset 0x0314 - Enable SkyCam C/D Data Trim Value Enable/disable C/D Data Trim Value.
Definition: FspsUpd.h:915
UINT8 PchSkyCamPortBTermOvrEnable
Offset 0x02FC - Enable SkyCam PortB Termination override Enable/disable PortB Termination override...
Definition: FspsUpd.h:796
UINT8 GmmEnable
Offset 0x0219 - Enable or disable GMM device 0=Disable, 1(Default)=Enable $EN_DIS.
Definition: FspsUpd.h:538
UINT8 PchIoApicBusNumber
Offset 0x034A - PCH Io Apic Bus Number Bus/Device/Function used as Requestor / Completer ID...
Definition: FspsUpd.h:1034
UINT8 PchPmPwrCycDur
Offset 0x065A - PCH Pm Reset Power Cycle Duration Could be customized in the unit of second...
Definition: FspsUpd.h:1538
UINT8 CdynmaxClampEnable
Offset 0x078E - Enable/Disable CdynmaxClamp Enable(Default): Enable CdynmaxClamp, Disable: Disable Cd...
Definition: FspsUpd.h:2157
UINT8 Custom1ConfigTdpControl
Offset 0x07B0 - Custom Config Tdp Control Config Tdp Control (0/1/2) value for custom cTDP level 1...
Definition: FspsUpd.h:2311
UINT8 PchScsEmmcHs400RxStrobeDll1
Offset 0x06C7 - Rx Strobe Delay Control Rx Strobe Delay Control - Rx Strobe Delay DLL 1 (HS400 Mode)...
Definition: FspsUpd.h:1715
UINT16 CstateLatencyControl4Irtl
Offset 0x0844 - Interrupt Response Time Limit of C-State LatencyContol4 Interrupt Response Time Limit...
Definition: FspsUpd.h:2682
UINT8 PchPmSlpS3MinAssert
Offset 0x0646 - PCH Pm Slp S3 Min Assert SLP_S3 Minimum Assertion Width Policy.
Definition: FspsUpd.h:1454
UINT8 GtFreqMax
Offset 0x0790 - GT Frequency Limit 0xFF: Auto(Default), 2: 100 Mhz, 3: 150 Mhz, 4: 200 Mhz...
Definition: FspsUpd.h:2177
UINT16 PchSubSystemId
Offset 0x0368 - PCH Sub system ID Default Subsystem ID of the PCH devices.
Definition: FspsUpd.h:1202
UINT8 PchPmSlpS4MinAssert
Offset 0x0647 - PCH Pm Slp S4 Min Assert SLP_S4 Minimum Assertion Width Policy.
Definition: FspsUpd.h:1459
UINT16 DefaultSvid
Offset 0x0200 - Subsystem Vendor ID for SA devices Subsystem ID that will be programmed to SA devices...
Definition: FspsUpd.h:469
UINT16 CstateLatencyControl3Irtl
Offset 0x0842 - Interrupt Response Time Limit of C-State LatencyContol3 Interrupt Response Time Limit...
Definition: FspsUpd.h:2677
UINT32 GraphicsConfigPtr
Offset 0x0028 - Graphics Configuration Ptr Points to VBT.
Definition: FspsUpd.h:103
UINT8 PkgCStateLimit
Offset 0x07DF - Set the Max Pkg Cstate Set the Max Pkg Cstate.
Definition: FspsUpd.h:2580
UINT8 PchTTEnable
Offset 0x06E3 - Enable The Thermal Throttle Enable the thermal throttle function. ...
Definition: FspsUpd.h:1806
UINT8 PchSkyCamPortAClkTrimValue
Offset 0x030C - Enable SkyCam PortA Clk Trim Value Enable/disable PortA Clk Trim Value.
Definition: FspsUpd.h:885
UINT8 PchScsEmmcHs400TuningRequired
Offset 0x06C5 - Enable eMMC HS400 Training Determine if HS400 Training is required.
Definition: FspsUpd.h:1704
UINT8 PchIoApicBdfValid
Offset 0x0349 - Enable PCH Io Apic Set to 1 if BDF value is valid.
Definition: FspsUpd.h:1029
UINT8 ManageabilityMode
Offset 0x0156 - Manageability Mode set by Mebx Enable/Disable.
Definition: FspsUpd.h:428
UINT8 SendVrMbxCmd
Offset 0x02E2 - Enable VR specific mailbox command VR specific mailbox commands.
Definition: FspsUpd.h:752
UINT8 SaImguEnable
Offset 0x0218 - Enable/Disable SA IMGU(SKYCAM) Enable(Default): Enable SA IMGU(SKYCAM), Disable: Disable SA IMGU(SKYCAM) $EN_DIS.
Definition: FspsUpd.h:532
UINT8 CdClock
Offset 0x0216 - CdClock Frequency selection 0=337.5 Mhz, 1=450 Mhz, 2=540 Mhz, 3(Default)= 675 Mhz 0:...
Definition: FspsUpd.h:520
UINT8 PchSkyCamPortBCtleCapValue
Offset 0x0307 - Enable SkyCam PortB Ctle Cap Value Enable/disable PortB Ctle Cap Value.
Definition: FspsUpd.h:860
UINT8 XdciEnable
Offset 0x006C - Enable xDCI controller Enable/disable to xDCI controller.
Definition: FspsUpd.h:230
UINT8 Custom2PowerLimit1Time
Offset 0x07B1 - Custom Short term Power Limit time window Short term Power Limit time window value fo...
Definition: FspsUpd.h:2316
UINT8 DisableD0I3SettingForHeci
Offset 0x088C - D0I3 Setting for HECI Disable Test, 0: disable, 1: enable, Setting this option disabl...
Definition: FspsUpd.h:2837
UINT8 PchIoApicDeviceNumber
Offset 0x034B - PCH Io Apic Device Number Bus/Device/Function used as Requestor / Completer ID...
Definition: FspsUpd.h:1039
UINT8 PchIoApicFunctionNumber
Offset 0x034C - PCH Io Apic Function Number Bus/Device/Function used as Requestor / Completer ID...
Definition: FspsUpd.h:1044
UINT8 DmiIot
Offset 0x0788 - DMI IOT Control Enable: Enable DMI IOT Control, Disable(Default): Disable DMI IOT Con...
Definition: FspsUpd.h:2133
UINT8 ConfigTdpBios
Offset 0x07B8 - Load Configurable TDP SSDT Configure whether to load Configurable TDP SSDT; 0: Disabl...
Definition: FspsUpd.h:2353
UINT8 PchDmiTsawEn
Offset 0x06E8 - DMI Thermal Sensor Autonomous Width Enable DMI Thermal Sensor Autonomous Width Enable...
Definition: FspsUpd.h:1837
UINT32 Custom1PowerLimit1
Offset 0x085C - Short term Power Limit value for custom cTDP level 1 Short term Power Limit value for...
Definition: FspsUpd.h:2723
UINT8 DmiExtSync
Offset 0x0787 - DMI Extended Sync Control Enable: Enable DMI Extended Sync Control, Disable(Default): Disable DMI Extended Sync Control $EN_DIS.
Definition: FspsUpd.h:2127
UINT8 PchLockDownGlobalSmi
Offset 0x088D - Enable LOCKDOWN SMI Enable SMI_LOCK bit to prevent writes to the Global SMI Enable bi...
Definition: FspsUpd.h:2843
UINT8 FourCoreRatioLimit
Offset 0x079F - 4-Core Ratio Limit 4-Core Ratio Limit: LFM to Fused max, For overclocking part: LFM t...
Definition: FspsUpd.h:2209
UINT8 PeiGraphicsPeimInit
Offset 0x0217 - Enable/Disable PeiGraphicsPeimInit Enable: Enable PeiGraphicsPeimInit, Disable(Default): Disable PeiGraphicsPeimInit $EN_DIS.
Definition: FspsUpd.h:526
UINT32 MicrocodeRegionBase
Offset 0x0038 - MicrocodeRegionBase Memory Base of Microcode Updates.
Definition: FspsUpd.h:183
UINT8 PchPmDisableEnergyReport
Offset 0x0A2E - PCH Pm Disable Energy Report Disable/Enable PCH to CPU enery report feature...
Definition: FspsUpd.h:2951
UINT8 Custom1PowerLimit1Time
Offset 0x07AE - Custom Short term Power Limit time window Short term Power Limit time window value fo...
Definition: FspsUpd.h:2301
UINT8 VoltageOptimization
Offset 0x07CC - Enable or Disable Voltage Optimization feature Enable or Disable Voltage Optimization...
Definition: FspsUpd.h:2461
UINT8 PchPmWoWlanEnable
Offset 0x0642 - PCH Pm WoW lan Enable Determine if WLAN wake from Sx, corresponds to the HOST_WLAN_PP...
Definition: FspsUpd.h:1430
UINT8 PchSkyCamPortCDCtleResValue
Offset 0x030B - Enable SkyCam PortCD Ctle Res Value Enable/disable PortCD Ctle Res Value...
Definition: FspsUpd.h:880
UINT8 PchPmDisableDsxAcPresentPulldown
Offset 0x0654 - PCH Pm Disable Dsx Ac Present Pulldown When Disable, PCH will internal pull down AC_P...
Definition: FspsUpd.h:1502
UINT8 PchPmWolOvrWkSts
Offset 0x0659 - PCH Pm WOL_OVR_WK_STS Clear the WOL_OVR_WK_STS bit in the Power and Reset Status (PRS...
Definition: FspsUpd.h:1532
UINT8 DebugInterfaceLockEnable
Offset 0x07C3 - Lock or Unlock debug interface features Lock or Unlock debug interface features; 0: D...
Definition: FspsUpd.h:2412
UINT8 SataPwrOptEnable
Offset 0x065D - PCH Sata Pwr Opt Enable SATA Power Optimizer on PCH side.
Definition: FspsUpd.h:1555
UINT8 PchSirqMode
Offset 0x06D9 - Serial IRQ Mode Select Serial IRQ Mode Select, 0: quiet mode, 1: continuous mode...
Definition: FspsUpd.h:1768
UINT8 PchIshUart0GpioAssign
Offset 0x0351 - Enable PCH ISH UART0 GPIO pins assigned 0: Disable; 1: Enable.
Definition: FspsUpd.h:1072
UINT8 Custom1TurboActivationRatio
Offset 0x07AF - Custom Turbo Activation Ratio Turbo Activation Ratio for custom cTDP level 1...
Definition: FspsUpd.h:2306
UINT8 PowerLimit2
Offset 0x07A4 - Short Duration Turbo Mode Enable or Disable short duration Turbo Mode.
Definition: FspsUpd.h:2238
UINT8 PchSkyCamPortCClkTrimValue
Offset 0x030E - Enable SkyCam PortC Clk Trim Value Enable/disable PortC Clk Trim Value.
Definition: FspsUpd.h:895
UINT8 SataRstHddUnlock
Offset 0x06B8 - PCH Sata Rst Hdd Unlock Indicates that the HDD password unlock in the OS is enabled...
Definition: FspsUpd.h:1664
UINT8 ProcTraceOutputScheme
Offset 0x07C8 - Control on Processor Trace output scheme Control on Processor Trace output scheme; 0:...
Definition: FspsUpd.h:2434
UINT8 SataMode
Offset 0x0092 - SATA Mode Select SATA controller working mode.
Definition: FspsUpd.h:312
UINT8 RaceToHalt
Offset 0x07E9 - Race To Halt Enable/Disable Race To Halt feature.
Definition: FspsUpd.h:2643
UINT8 PchThermalDeviceEnable
Offset 0x06DB - Enable Thermal Device Enable Thermal Device.
Definition: FspsUpd.h:1779
UINT8 TStates
Offset 0x07D0 - Enable or Disable T states Enable or Disable T states; 0: Disable; 1: Enable...
Definition: FspsUpd.h:2487
UINT8 CstateLatencyControl2TimeUnit
Offset 0x07E2 - TimeUnit for C-State Latency Control2 TimeUnit for C-State Latency Control2;Valid val...
Definition: FspsUpd.h:2598
UINT16 CstateLatencyControl5Irtl
Offset 0x0846 - Interrupt Response Time Limit of C-State LatencyContol5 Interrupt Response Time Limit...
Definition: FspsUpd.h:2687
UINT8 SevenCoreRatioLimit
Offset 0x0886 - 7-Core Ratio Limit 7-Core Ratio Limit: LFM to Fused max, For overclocking part: LFM t...
Definition: FspsUpd.h:2799
UINT16 MaxRatio
Offset 0x07EA - Max P-State Ratio Max P-State Ratio , Valid Range 0 to 0x7F.
Definition: FspsUpd.h:2648
UINT8 TwoCoreRatioLimit
Offset 0x079D - 2-Core Ratio Limit 2-Core Ratio Limit: LFM to Fused max, For overclocking part: LFM t...
Definition: FspsUpd.h:2197
UINT8 PowerLimit3DutyCycle
Offset 0x07A7 - Package PL3 Duty Cycle Package PL3 Duty Cycle; Valid Range is 0 to 100...
Definition: FspsUpd.h:2255
UINT8 PchPmLanWakeFromDeepSx
Offset 0x0644 - PCH Pm Lan Wake From DeepSx Determine if enable LAN to wake from deep Sx...
Definition: FspsUpd.h:1443
UINT8 SendVrMbxCmd1
Offset 0x02E3 - Select VR specific mailbox command to send VR specific mailbox commands.
Definition: FspsUpd.h:759
UINT8 ConfigTdpLock
Offset 0x07B7 - ConfigTdp mode settings Lock Lock the ConfigTdp mode settings from runtime changes; 0...
Definition: FspsUpd.h:2347
UINT8 SataThermalSuggestedSetting
Offset 0x06FA - Sata Thermal Throttling Suggested Setting Sata Thermal Throttling Suggested Setting...
Definition: FspsUpd.h:1931
UINT8 SgxSinitNvsData
Offset 0x0764 - SgxSinitNvsData SgxSinitNvsData default values.
Definition: FspsUpd.h:2055
UINT8 ApIdleManner
Offset 0x07C4 - AP Idle Manner of waiting for SIPI AP Idle Manner of waiting for SIPI; 1: HALT loop; ...
Definition: FspsUpd.h:2418
UINT8 DmiTS0TW
Offset 0x06EA - Thermal Sensor 0 Target Width Thermal Sensor 0 Target Width.
Definition: FspsUpd.h:1848
UINT8 PchHdaDspEndpointDmic
Offset 0x033E - DSP DMIC Select (PCH_HDAUDIO_DMIC_TYPE enum) 0: Disable; 1: 2ch array; 2: 4ch array; ...
Definition: FspsUpd.h:997
UINT8 MachineCheckEnable
Offset 0x07C1 - Enable or Disable initialization of machine check registers Enable or Disable initial...
Definition: FspsUpd.h:2400
UINT32 Custom2PowerLimit2
Offset 0x0868 - Long term Power Limit value for custom cTDP level 2 Long term Power Limit value for c...
Definition: FspsUpd.h:2741
UINT8 SataEnable
Offset 0x0091 - Enable SATA Enable/disable SATA controller.
Definition: FspsUpd.h:306
UINT8 PchHdaIDispLinkTmode
Offset 0x033B - iDisp-Link T-mode iDisp-Link T-Mode (PCH_HDAUDIO_IDISP_TMODE enum): 0: 2T...
Definition: FspsUpd.h:979
UINT8 PowerLimit4Lock
Offset 0x07A9 - Package PL4 Lock Package PL4 Lock Enable/Disable; 0: Disable ; 1: Enable $EN_DIS...
Definition: FspsUpd.h:2267
UINT8 PchDisableComplianceMode
Offset 0x0704 - Disable XHCI Compliance Mode This policy will disable XHCI compliance mode on all por...
Definition: FspsUpd.h:1964
UINT8 ProcTraceMemSize
Offset 0x07CA - Memory region allocation for Processor Trace Memory region allocation for Processor T...
Definition: FspsUpd.h:2451
UINT8 PchPmLpcClockRun
Offset 0x0650 - PCH Pm Lpc Clock Run This member describes whether or not the LPC ClockRun feature of...
Definition: FspsUpd.h:1479
UINT8 EightCoreRatioLimit
Offset 0x0887 - 8-Core Ratio Limit 8-Core Ratio Limit: LFM to Fused max, For overclocking part: LFM t...
Definition: FspsUpd.h:2805
UINT8 TccOffsetLock
Offset 0x07AC - Tcc Offset Lock Tcc Offset Lock for Runtime Average Temperature Limit (RATL) to lock ...
Definition: FspsUpd.h:2290
UINT8 PchScsEmmcHs400DriverStrength
Offset 0x06C9 - I/O Driver Strength I/O driver strength: 0 - 33 Ohm, 1 - 40 Ohm, 2 - 50 Ohm...
Definition: FspsUpd.h:1725
UINT8 PchSkyCamPortDTrimEnable
Offset 0x0302 - Enable SkyCam PortD Clk Trim Enable/disable PortD Clk Trim.
Definition: FspsUpd.h:832
UINT8 NumOfDevIntConfig
Offset 0x006F - Number of DevIntConfig Entry Number of Device Interrupt Configuration Entry...
Definition: FspsUpd.h:246
UINT8 Hwp
Offset 0x07A1 - Enable or Disable HWP Enable or Disable HWP(Hardware P states) Support.
Definition: FspsUpd.h:2220
UINT8 SsicPortEnable
Offset 0x006D - Enable XHCI SSIC Enable Enable/disable XHCI SSIC port.
Definition: FspsUpd.h:236
UINT8 PchSkyCamPortDTermOvrEnable
Offset 0x02FE - Enable SkyCam PortD Termination override Enable/disable PortD Termination override...
Definition: FspsUpd.h:808
UINT8 ProcHotLock
Offset 0x07E7 - Lock prochot configuration Lock prochot configuration Enable/Disable; 0: Disable; 1: ...
Definition: FspsUpd.h:2628
UINT8 PchHdaDspEndpointI2s
Offset 0x0348 - DSP I2S enablement 0: Disable; 1: Enable.
Definition: FspsUpd.h:1023
UINT8 PchIshPdtUnlock
Offset 0x035E - PCH ISH PDT Unlock Msg 0: False; 1: True.
Definition: FspsUpd.h:1150
UINT8 PchHdaEnable
Offset 0x002D - Enable Intel HD Audio (Azalia) Enable/disable Azalia controller.
Definition: FspsUpd.h:115
UINT8 SataRstOromUiBanner
Offset 0x06B6 - PCH Sata Rst Orom Ui Banner OROM UI and BANNER.
Definition: FspsUpd.h:1653
UINT8 AutoThermalReporting
Offset 0x07D5 - Enable or Disable Thermal Reporting Enable or Disable Thermal Reporting through ACPI ...
Definition: FspsUpd.h:2517
UINT8 ShowSpiController
Offset 0x0035 - Show SPI controller Enable/disable to show SPI controller.
Definition: FspsUpd.h:167
UINT8 PchIoApicRangeSelect
Offset 0x034F - PCH Io Apic Range Select Define address bits 19:12 for the IOxAPIC range...
Definition: FspsUpd.h:1060
UINT32 DevIntConfigPtr
Offset 0x0070 - Address of PCH_DEVICE_INTERRUPT_CONFIG table.
Definition: FspsUpd.h:251
UINT8 Heci3Enabled
Offset 0x0149 - HECI3 state The HECI3 state from Mbp for reference in S3 path or when MbpHob is not i...
Definition: FspsUpd.h:400
UINT8 TccOffsetClamp
Offset 0x07AB - Tcc Offset Clamp Enable/Disable Tcc Offset Clamp for Runtime Average Temperature Limi...
Definition: FspsUpd.h:2283
UINT8 Custom3TurboActivationRatio
Offset 0x07B5 - Custom Turbo Activation Ratio Turbo Activation Ratio for custom cTDP level 3...
Definition: FspsUpd.h:2336
UINT8 MonitorMwaitEnable
Offset 0x07C0 - Enable or Disable Monitor /MWAIT instructions Enable or Disable Monitor /MWAIT instru...
Definition: FspsUpd.h:2394
UINT16 PchT0Level
Offset 0x06DC - Thermal Throttling Custimized T0Level Value Custimized T0Level value.
Definition: FspsUpd.h:1784
UINT8 SpiFlashCfgLockDown
Offset 0x0036 - Flash Configuration Lock Down Enable/disable flash lock down.
Definition: FspsUpd.h:174
UINT8 PchIshGp2GpioAssign
Offset 0x0358 - Enable PCH ISH GP_2 GPIO pin assigned 0: Disable; 1: Enable.
Definition: FspsUpd.h:1114
UINT8 PchDmiAspm
Offset 0x0316 - Enable DMI ASPM ASPM on PCH side of the DMI Link.
Definition: FspsUpd.h:921
UINT8 CstateLatencyControl5TimeUnit
Offset 0x07E5 - TimeUnit for C-State Latency Control5 TimeUnit for C-State Latency Control5;Valid val...
Definition: FspsUpd.h:2616
UINT64 MicrocodePatchAddress
Offset 0x02F0 - MicrocodePatchAddress Pointer to microcode patch that is suitable for this processor...
Definition: FspsUpd.h:775
UINT8 PsysOffset
Offset 0x0277 - Platform Psys offset correction PCODE MMIO Mailbox: Platform Psys offset correction...
Definition: FspsUpd.h:627
UINT32 PchHdaDspFeatureMask
Offset 0x0340 - Bitmask of supported DSP features [BIT0] - WoV; [BIT1] - BT Sideband; [BIT2] - Codec ...
Definition: FspsUpd.h:1010
UINT8 PowerLimit3Lock
Offset 0x07A8 - Package PL3 Lock Package PL3 Lock Enable/Disable; 0: Disable ; 1: Enable $EN_DIS...
Definition: FspsUpd.h:2261
UINT8 MeUnconfigIsValid
Offset 0x0779 - Check if MeUnconfigOnRtcClear is valid The MeUnconfigOnRtcClear item could be not val...
Definition: FspsUpd.h:2081
UINT8 SataRstOromUiDelay
Offset 0x06B7 - PCH Sata Rst Orom Ui Delay 00b: 2 secs; 01b: 4 secs; 10b: 6 secs; 11: 8 secs (see: PC...
Definition: FspsUpd.h:1658
UINT8 FiveCoreRatioLimit
Offset 0x0884 - 5-Core Ratio Limit 5-Core Ratio Limit: LFM to Fused max, For overclocking part: LFM t...
Definition: FspsUpd.h:2787
UINT8 PchHdaIoBufferOwnership
Offset 0x002F - Select HDAudio IoBuffer Ownership Indicates the ownership of the I/O buffer between I...
Definition: FspsUpd.h:131
UINT8 TTSuggestedSetting
Offset 0x06E6 - Thermal Throttling Suggested Setting Thermal Throttling Suggested Setting...
Definition: FspsUpd.h:1825
UINT8 PsysSlope
Offset 0x0276 - Platform Psys slope correction PCODE MMIO Mailbox: Platform Psys slope correction...
Definition: FspsUpd.h:621
UINT8 PchIoApicEntry24_119
Offset 0x034D - Enable PCH Io Apic Entry 24-119 0: Disable; 1: Enable.
Definition: FspsUpd.h:1050
UINT8 PchSkyCamPortBClkTrimValue
Offset 0x030D - Enable SkyCam PortB Clk Trim Value Enable/disable PortB Clk Trim Value.
Definition: FspsUpd.h:890
UINT8 CStatePreWake
Offset 0x07DC - Enable or Disable CState-Pre wake Enable or Disable CState-Pre wake.
Definition: FspsUpd.h:2561
UINT8 PchLegacyIoLowLatency
Offset 0x036A - PCH Legacy IO Low Latency Enable todo $EN_DIS.
Definition: FspsUpd.h:1208
UINT8 PchPmSlpLanLowDc
Offset 0x0652 - PCH Pm Slp Lan Low Dc Enable/Disable SLP_LAN# Low on DC Power.
Definition: FspsUpd.h:1491
UINT8 CstCfgCtrIoMwaitRedirection
Offset 0x07DE - Enable or Disable IO to MWAIT redirection Enable or Disable IO to MWAIT redirection; ...
Definition: FspsUpd.h:2573
UINT8 PchHdaLinkFrequency
Offset 0x0339 - HD Audio Link Frequency HDA Link Freq (PCH_HDAUDIO_LINK_FREQUENCY enum): 0: 6MHz...
Definition: FspsUpd.h:969
UINT8 PsysPowerLimit1
Offset 0x07B9 - PL1 Enable value PL1 Enable value to limit average platform power.
Definition: FspsUpd.h:2359
UINT8 ThermalMonitor
Offset 0x07D6 - Enable or Disable Thermal Monitor Enable or Disable Thermal Monitor; 0: Disable; 1: E...
Definition: FspsUpd.h:2523
UINT8 DmiSuggestedSetting
Offset 0x06E9 - DMI Thermal Sensor Suggested Setting DMT thermal sensor suggested representative valu...
Definition: FspsUpd.h:1843
UINT8 PchIshGp6GpioAssign
Offset 0x035C - Enable PCH ISH GP_6 GPIO pin assigned 0: Disable; 1: Enable.
Definition: FspsUpd.h:1138
UINT8 PchHdaDspEnable
Offset 0x002E - Enable HD Audio DSP Enable/disable HD Audio DSP feature.
Definition: FspsUpd.h:121
UINT8 PchPmSlpS0VmEnable
Offset 0x063A - PCH Pm Slp S0 Voltage Margining Enable Indicates platform has support for VCCPrim_Cor...
Definition: FspsUpd.h:1408
UINT8 DmiTS1TW
Offset 0x06EB - Thermal Sensor 1 Target Width Thermal Sensor 1 Target Width.
Definition: FspsUpd.h:1853
UINT8 AcousticNoiseMitigation
Offset 0x0278 - Acoustic Noise Mitigation feature Enable or Disable Acoustic Noise Mitigation feature...
Definition: FspsUpd.h:633
UINT8 PchScsEmmcHs400TxDataDll
Offset 0x06C8 - Tx Data Delay Control Tx Data Delay Control 1 - Tx Data Delay (HS400 Mode)...
Definition: FspsUpd.h:1720
UINT8 ConfigTdpLevel
Offset 0x07E8 - Configuration for boot TDP selection Configuration for boot TDP selection; 0: TDP Nom...
Definition: FspsUpd.h:2635
UINT8 EcCmdProvisionEav
Offset 0x0729 - EcCmdProvisionEav Ephemeral Authorization Value default values.
Definition: FspsUpd.h:2019
UINT8 PchSkyCamPortATermOvrEnable
Offset 0x02FB - Enable SkyCam PortA Termination override Enable/disable PortA Termination override...
Definition: FspsUpd.h:790
UINT32 BiosGuardAttr
Offset 0x0760 - BiosGuardAttr BiosGuardAttr default values.
Definition: FspsUpd.h:2050
UINT8 Device4Enable
Offset 0x002C - Enable Device 4 Enable/disable Device 4 $EN_DIS.
Definition: FspsUpd.h:109
UINT8 PchTTState13Enable
Offset 0x06E4 - PMSync State 13 When set to 1 and the programmed GPIO pin is a 1, then PMSync state 1...
Definition: FspsUpd.h:1813
UINT8 SlowSlewRateForGt
Offset 0x027B - Slew Rate configuration for Deep Package C States for VR GT domain Slew Rate configur...
Definition: FspsUpd.h:654
UINT8 Custom2TurboActivationRatio
Offset 0x07B2 - Custom Turbo Activation Ratio Turbo Activation Ratio for custom cTDP level 2...
Definition: FspsUpd.h:2321
UINT8 PchScsEmmcHs400DllDataValid
Offset 0x06C6 - Set HS400 Tuning Data Valid Set if HS400 Tuning Data Valid.
Definition: FspsUpd.h:1710
UINT8 CstateLatencyControl1TimeUnit
Offset 0x07E1 - TimeUnit for C-State Latency Control1 TimeUnit for C-State Latency Control1;Valid val...
Definition: FspsUpd.h:2592
UINT8 ApHandoffManner
Offset 0x07C5 - Settings for AP Handoff to OS Settings for AP Handoff to OS; 1: HALT loop; 2: MWAIT l...
Definition: FspsUpd.h:2424
UINT8 PchHdaVcType
Offset 0x0338 - VC Type Virtual Channel Type Select: 0: VC0, 1: VC1.
Definition: FspsUpd.h:964
UINT8 PchTsmicLock
Offset 0x06E2 - Thermal Device SMI Enable This locks down SMI Enable on Alert Thermal Sensor Trip...
Definition: FspsUpd.h:1800
UINT8 DelayUsbPdoProgramming
Offset 0x00FC - Delay USB PDO Programming Enable/disable delay of PDO programming for USB from PEI ph...
Definition: FspsUpd.h:373
UINT8 PowerLimit3Time
Offset 0x07A6 - Package PL3 time window Package PL3 time window range for this policy in milliseconds...
Definition: FspsUpd.h:2250
UINT8 PchIshGp5GpioAssign
Offset 0x035B - Enable PCH ISH GP_5 GPIO pin assigned 0: Disable; 1: Enable.
Definition: FspsUpd.h:1132
UINT8 PchHdaIDispCodecDisconnect
Offset 0x033D - iDisplay Audio Codec disconnection 0: Not disconnected, enumerable, 1: Disconnected SDI, not enumerable.
Definition: FspsUpd.h:992
UINT16 WatchDogTimerOs
Offset 0x0158 - OS Timer 16 bits Value, Set OS watchdog timer.
Definition: FspsUpd.h:441
UINT8 PmgCstCfgCtrlLock
Offset 0x07D8 - Configure C-State Configuration Lock Configure C-State Configuration Lock; 0: Disable...
Definition: FspsUpd.h:2535
UINT8 PchIshGp1GpioAssign
Offset 0x0357 - Enable PCH ISH GP_1 GPIO pin assigned 0: Disable; 1: Enable.
Definition: FspsUpd.h:1108
UINT8 PchHdaDspUaaCompliance
Offset 0x033C - Universal Audio Architecture compliance for DSP enabled system 0: Not-UAA Compliant (...
Definition: FspsUpd.h:986
UINT8 DmiTS3TW
Offset 0x06ED - Thermal Sensor 3 Target Width Thermal Sensor 3 Target Width.
Definition: FspsUpd.h:1863
UINT8 PowerLimit1Time
Offset 0x07A3 - Package Long duration turbo mode time Package Long duration turbo mode time window in...
Definition: FspsUpd.h:2232
UINT8 PchLockDownRtcLock
Offset 0x0891 - RTC CMOS RAM LOCK Enable RTC lower and upper 128 byte Lock bits to lock Bytes 38h-3Fh...
Definition: FspsUpd.h:2861
UINT8 MlcSpatialPrefetcher
Offset 0x07BF - Enable or Disable MLC Spatial Prefetcher Enable or Disable MLC Spatial Prefetcher; 0:...
Definition: FspsUpd.h:2388
UINT8 Custom2ConfigTdpControl
Offset 0x07B3 - Custom Config Tdp Control Config Tdp Control (0/1/2) value for custom cTDP level 1...
Definition: FspsUpd.h:2326
UINT8 ScsSdCardEnabled
Offset 0x0033 - Enable SdCard Controller Enable/disable SD Card Controller.
Definition: FspsUpd.h:155
UINT8 EsataSpeedLimit
Offset 0x065E - PCH Sata eSATA Speed Limit When enabled, BIOS will configure the PxSCTL.SPD to 2 to limit the eSATA port speed.
Definition: FspsUpd.h:1561
UINT8 PchPwrOptEnable
Offset 0x0317 - Enable Power Optimizer Enable DMI Power Optimizer on PCH side.
Definition: FspsUpd.h:927
UINT32 PchPcieDeviceOverrideTablePtr
Offset 0x0724 - Pch PCIE device override table pointer The PCIe device table is being used to overrid...
Definition: FspsUpd.h:2006
UINT8 HdcControl
Offset 0x07A2 - Hardware Duty Cycle Control Hardware Duty Cycle Control configuration.
Definition: FspsUpd.h:2226
UINT8 Custom3PowerLimit1Time
Offset 0x07B4 - Custom Short term Power Limit time window Short term Power Limit time window value fo...
Definition: FspsUpd.h:2331
UINT8 PchPmPcieWakeFromDeepSx
Offset 0x0641 - PCH Pm Pcie Wake From DeepSx Determine if enable PCIe to wake from deep Sx...
Definition: FspsUpd.h:1424
UINT32 PsysPowerLimit1Power
Offset 0x0874 - Platform PL1 power Platform PL1 power.
Definition: FspsUpd.h:2759
UINT8 SataSpeedLimit
Offset 0x065F - PCH Sata Speed Limit Indicates the maximum speed the SATA controller can support 0h: ...
Definition: FspsUpd.h:1566
UINT32 MicrocodeRegionSize
Offset 0x003C - MicrocodeRegionSize Size of Microcode Updates.
Definition: FspsUpd.h:188
UINT8 PchLanClkReqSupported
Offset 0x0361 - Indicate whether dedicated CLKREQ# is supported 0: Disable; 1: Enable.
Definition: FspsUpd.h:1168
UINT8 PchIshGp3GpioAssign
Offset 0x0359 - Enable PCH ISH GP_3 GPIO pin assigned 0: Disable; 1: Enable.
Definition: FspsUpd.h:1120
UINT8 SataRstSmartStorage
Offset 0x06BB - PCH Sata Rst Smart Storage RST Smart Storage caching Bit.
Definition: FspsUpd.h:1683
UINT8 TurboPowerLimitLock
Offset 0x07A5 - Turbo settings Lock Lock all Turbo settings Enable/Disable; 0: Disable ...
Definition: FspsUpd.h:2244
UINT8 PchIshI2c2GpioAssign
Offset 0x0355 - Enable PCH ISH I2C2 GPIO pins assigned 0: Disable; 1: Enable.
Definition: FspsUpd.h:1096
UINT32 * Data
Pointer to the data buffer. Its length is specified in the header.
Definition: FspsUpd.h:60
UINT8 PchIoApicId
Offset 0x034E - PCH Io Apic ID This member determines IOAPIC ID.
Definition: FspsUpd.h:1055
UINT8 Cx
Offset 0x07D7 - Enable or Disable CPU power states (C-states) Enable or Disable CPU power states (C-s...
Definition: FspsUpd.h:2529
UINT8 SataP1TDispFinit
Offset 0x06F9 - Port 1 Alternate Fast Init Tdispatch Port 1 Alternate Fast Init Tdispatch.
Definition: FspsUpd.h:1925
UINT8 PavpEnable
Offset 0x0215 - Enable/Disable PavpEnable Enable(Default): Enable PavpEnable, Disable: Disable PavpEn...
Definition: FspsUpd.h:514
UINT8 ProcTraceEnable
Offset 0x07C9 - Enable or Disable Processor Trace feature Enable or Disable Processor Trace feature; ...
Definition: FspsUpd.h:2440
UINT8 FastPkgCRampDisableGt
Offset 0x02DF - Disable Fast Slew Rate for Deep Package C States for VR GT domain Disable Fast Slew R...
Definition: FspsUpd.h:733
UINT8 PchLanLtrEnable
Offset 0x035F - Enable PCH Lan LTR capabilty of PCH internal LAN 0: Disable; 1: Enable.
Definition: FspsUpd.h:1156
UINT8 PmSupport
Offset 0x078D - Enable/Disable IGFX PmSupport Enable(Default): Enable IGFX PmSupport, Disable: Disable IGFX PmSupport $EN_DIS.
Definition: FspsUpd.h:2151
UINT8 PchSkyCamPortACtleResValue
Offset 0x0309 - Enable SkyCam PortA Ctle Res Value Enable/disable PortA Ctle Res Value.
Definition: FspsUpd.h:870
UINT8 PchSirqEnable
Offset 0x06D8 - Enable Serial IRQ Determines if enable Serial IRQ.
Definition: FspsUpd.h:1762
FSP CPU Data Config Block.
UINT8 PchIshI2c0GpioAssign
Offset 0x0353 - Enable PCH ISH I2C0 GPIO pins assigned 0: Disable; 1: Enable.
Definition: FspsUpd.h:1084
UINT8 PchPciePort8xhDecodePortIndex
Offset 0x0A2D - PCIE Port8xh Decode Port Index The Index of PCIe Port that is selected for Port8xh De...
Definition: FspsUpd.h:2945
UINT8 SataTestMode
Offset 0x0A30 - PCH Sata Test Mode Allow entrance to the PCH SATA test modes.
Definition: FspsUpd.h:2963
UINT8 CstateLatencyControl4TimeUnit
Offset 0x07E4 - TimeUnit for C-State Latency Control4 TimeUnit for C-State Latency Control4;Valid val...
Definition: FspsUpd.h:2610
UINT32 LogoPtr
Offset 0x0020 - Logo Pointer Points to PEI Display Logo Image.
Definition: FspsUpd.h:93
UINT8 DmiAspm
Offset 0x0205 - DMI ASPM 0=Disable, 2(Default)=L1 0:Disable, 2:L1.
Definition: FspsUpd.h:486
UINT8 SgxSinitDataFromTpm
Offset 0x088A - SgxSinitDataFromTpm SgxSinitDataFromTpm default values.
Definition: FspsUpd.h:2823
UINT8 PchLanK1OffEnable
Offset 0x0360 - Enable PCH Lan use CLKREQ for GbE power management 0: Disable; 1: Enable...
Definition: FspsUpd.h:1162
UINT8 PchHdaVerbTableEntryNum
Offset 0x008B - PCH HDA Verb Table Entry Number Number of Entries in Verb Table.
Definition: FspsUpd.h:291
UINT8 PchSbAccessUnlock
Offset 0x0893 - PCH Psf lock bit The PSF registers will be locked before 3rd party code execution...
Definition: FspsUpd.h:2873
UINT8 PchLockDownSpiEiss
Offset 0x0364 - Enable LOCKDOWN SPI Eiss Enable InSMM.STS (EISS) in SPI.
Definition: FspsUpd.h:1186
UINT8 PchSkyCamPortACtleEnable
Offset 0x0303 - Enable SkyCam PortA Ctle Enable/disable PortA Ctle.
Definition: FspsUpd.h:838
UINT8 Eist
Offset 0x07CD - Enable or Disable Intel SpeedStep Technology Enable or Disable Intel SpeedStep Techno...
Definition: FspsUpd.h:2467
UINT8 PchPmDisableNativePowerButton
Offset 0x0656 - PCH Pm Disable Native Power Button Power button native mode disable.
Definition: FspsUpd.h:1514
UINT8 SataRstIrrtOnly
Offset 0x06BA - PCH Sata Rst Irrt Only Allow only IRRT drives to span internal and external ports...
Definition: FspsUpd.h:1677
UINT32 TccOffsetTimeWindowForRatl
Offset 0x0858 - Tcc Offset Time Window for RATL Package PL4 power limit.
Definition: FspsUpd.h:2717
UINT16 PsysPmax
Offset 0x087C - Platform Power Pmax PCODE MMIO Mailbox: Platform Power Pmax.
Definition: FspsUpd.h:2771
UINT8 PchSkyCamPortBTrimEnable
Offset 0x0300 - Enable SkyCam PortB Clk Trim Enable/disable PortB Clk Trim.
Definition: FspsUpd.h:820
UINT8 ProcHotResponse
Offset 0x07D3 - Enable or Disable PROCHOT# Response Enable or Disable PROCHOT# Response; 0: Disable; ...
Definition: FspsUpd.h:2505
UINT8 PchPmPmeB0S5Dis
Offset 0x0639 - PCH Pm PME_B0_S5_DIS When cleared (default), wake events from PME_B0_STS are allowed ...
Definition: FspsUpd.h:1402
CPU_CONFIG_FSP_DATA CpuConfig
Offset 0x02E8 - Cpu Configuration Cpu Configuration data.
Definition: FspsUpd.h:769
UINT8 SataRstLedLocate
Offset 0x06B9 - PCH Sata Rst Led Locate Indicates that the LED/SGPIO hardware is attached and ping to...
Definition: FspsUpd.h:1671
UINT32 PowerLimit2Power
Offset 0x084C - Package Short duration turbo mode power limit Package Short duration turbo mode power...
Definition: FspsUpd.h:2699
UINT8 PchIshUart1GpioAssign
Offset 0x0352 - Enable PCH ISH UART1 GPIO pins assigned 0: Disable; 1: Enable.
Definition: FspsUpd.h:1078
UINT8 PchPmCapsuleResetType
Offset 0x0655 - PCH Pm Capsule Reset Type Deprecated: Determines type of reset issued during UpdateCa...
Definition: FspsUpd.h:1508
UINT8 PchIshGp4GpioAssign
Offset 0x035A - Enable PCH ISH GP_4 GPIO pin assigned 0: Disable; 1: Enable.
Definition: FspsUpd.h:1126
UINT8 PchIshSpiGpioAssign
Offset 0x0350 - Enable PCH ISH SPI GPIO pins assigned 0: Disable; 1: Enable.
Definition: FspsUpd.h:1066
UINT8 PcieAllowNoLtrIccPllShutdown
Offset 0x0634 - PCIE Allow No Ltr Icc PLL Shutdown Allows BIOS to control ICC PLL Shutdown by determi...
Definition: FspsUpd.h:1377
UINT32 PowerLimit3
Offset 0x0850 - Package PL3 power limit Package PL3 power limit.
Definition: FspsUpd.h:2705
UINT16 PcieDetectTimeoutMs
Offset 0x0636 - PCIE Rp Detect Timeout Ms Will wait for link to exit Detect state for enabled ports b...
Definition: FspsUpd.h:1389
UINT8 DisableVrThermalAlert
Offset 0x07D4 - Enable or Disable VR Thermal Alert Enable or Disable VR Thermal Alert; 0: Disable; 1:...
Definition: FspsUpd.h:2511
UINT8 PchIshEnable
Offset 0x0034 - Enable PCH ISH Controller Enable/disable ISH Controller.
Definition: FspsUpd.h:161
UINT8 DmiTS2TW
Offset 0x06EC - Thermal Sensor 2 Target Width Thermal Sensor 2 Target Width.
Definition: FspsUpd.h:1858
UINT8 PcieEnablePort8xhDecode
Offset 0x0A2C - PCIE RP Enable Port8xh Decode This member describes whether PCIE root port Port 8xh D...
Definition: FspsUpd.h:2940
UINT8 PchPmSlpSusMinAssert
Offset 0x0648 - PCH Pm Slp Sus Min Assert SLP_SUS Minimum Assertion Width Policy. ...
Definition: FspsUpd.h:1464
UINT8 PchSkyCamPortCTrimEnable
Offset 0x0301 - Enable SkyCam PortC Clk Trim Enable/disable PortC Clk Trim.
Definition: FspsUpd.h:826
UINT8 PchSkyCamPortBCtleResValue
Offset 0x030A - Enable SkyCam PortB Ctle Res Value Enable/disable PortB Ctle Res Value.
Definition: FspsUpd.h:875
UINT8 PkgCStateDemotion
Offset 0x07DA - Enable or Disable Package C-State Demotion Enable or Disable Package C-State Demotion...
Definition: FspsUpd.h:2548
UINT32 Custom2PowerLimit1
Offset 0x0864 - Short term Power Limit value for custom cTDP level 2 Short term Power Limit value for...
Definition: FspsUpd.h:2735
UINT8 SerialIoDebugUartNumber
Offset 0x06D6 - UART Number For Debug Purpose UART number for debug purpose.
Definition: FspsUpd.h:1751
UINT8 PchSbiUnlock
Offset 0x0892 - PCH Sbi lock bit This unlock the SBI lock bit to allow SBI after post time...
Definition: FspsUpd.h:2867
UINT8 PchPmPwrBtnOverridePeriod
Offset 0x0653 - PCH Pm Pwr Btn Override Period PCH power button override period.
Definition: FspsUpd.h:1496
UINT32 PowerLimit1
Offset 0x0848 - Package Long duration turbo mode power limit Package Long duration turbo mode power l...
Definition: FspsUpd.h:2693
UINT8 PchPmSlpS0Enable
Offset 0x0657 - PCH Pm Slp S0 Enable Indicates whether SLP_S0# is to be asserted when PCH reaches idl...
Definition: FspsUpd.h:1520
UINT8 PcieComplianceTestMode
Offset 0x0635 - PCIE Compliance Test Mode Compliance Test Mode shall be enabled when using Compliance...
Definition: FspsUpd.h:1383
UINT8 X2ApicOptOut
Offset 0x021A - State of X2APIC_OPT_OUT bit in the DMAR table 0=Disable/Clear, 1=Enable/Set $EN_DIS...
Definition: FspsUpd.h:544
UINT8 PchSkyCamPortATrimEnable
Offset 0x02FF - Enable SkyCam PortA Clk Trim Enable/disable PortA Clk Trim.
Definition: FspsUpd.h:814
UINT8 ChapDeviceEnable
Offset 0x0784 - Enable/Disable Device 7 Enable: Device 7 enabled, Disable (Default): Device 7 disable...
Definition: FspsUpd.h:2106
UINT8 EndOfPostMessage
Offset 0x088B - End of Post message Test, Send End of Post message.
Definition: FspsUpd.h:2830
UINT8 PcieDisableRootPortClockGating
Offset 0x0632 - PCIE Disable RootPort Clock Gating Describes whether the PCI Express Clock Gating for...
Definition: FspsUpd.h:1364
UINT16 PchT1Level
Offset 0x06DE - Thermal Throttling Custimized T1Level Value Custimized T1Level value.
Definition: FspsUpd.h:1789
The PCH_DEVICE_INTERRUPT_CONFIG block describes interrupt pin, IRQ and interrupt mode for PCH device...
Definition: FspsUpd.h:76
UINT8 PchPmSlpAMinAssert
Offset 0x0649 - PCH Pm Slp A Min Assert SLP_A Minimum Assertion Width Policy.
Definition: FspsUpd.h:1469
UINT8 DebugInterfaceEnable
Offset 0x07C2 - Enable or Disable processor debug features Enable or Disable processor debug features...
Definition: FspsUpd.h:2406
UINT32 VrPowerDeliveryDesign
Offset 0x0290 - CPU VR Power Delivery Design Used to communicate the power delivery design capability...
Definition: FspsUpd.h:678
UINT8 FastPkgCRampDisableIa
Offset 0x0279 - Disable Fast Slew Rate for Deep Package C States for VR IA domain Disable Fast Slew R...
Definition: FspsUpd.h:640
UINT8 SkipPamLock
Offset 0x0785 - Skip PAM register lock Enable: PAM register will not be locked by RC...
Definition: FspsUpd.h:2113
UINT8 VtdDisable
Offset 0x078F - Disable VT-d 0=Enable/FALSE(VT-d disabled), 1=Disable/TRUE (VT-d enabled) $EN_DIS...
Definition: FspsUpd.h:2163
UINT8 SataRstOptaneMemory
Offset 0x0720 - PCH Sata Rst Optane Memory Optane Memory $EN_DIS.
Definition: FspsUpd.h:1988
UINT16 CpuS3ResumeDataSize
Offset 0x087E - CpuS3ResumeDataSize Size of CPU S3 Resume Data.
Definition: FspsUpd.h:2776
UINT8 PchStartFramePulse
Offset 0x06DA - Start Frame Pulse Width Start Frame Pulse Width, 0: PchSfpw4Clk, 1: PchSfpw6Clk...
Definition: FspsUpd.h:1773
UINT8 RenderStandby
Offset 0x078C - Enable/Disable IGFX RenderStandby Enable(Default): Enable IGFX RenderStandby, Disable: Disable IGFX RenderStandby $EN_DIS.
Definition: FspsUpd.h:2145
UINT8 PcieRpFunctionSwap
Offset 0x0638 - PCIE Rp Function Swap Allows BIOS to use root port function number swapping when root...
Definition: FspsUpd.h:1396
UINT8 PcieEnablePeerMemoryWrite
Offset 0x0633 - PCIE Enable Peer Memory Write This member describes whether Peer Memory Writes are en...
Definition: FspsUpd.h:1370
UINT8 PchPort61hEnable
Offset 0x065C - PCH Port 61h Config Enable/Disable Used for the emulation feature for Port61h read...
Definition: FspsUpd.h:1549
UINT32 Custom3PowerLimit1
Offset 0x086C - Short term Power Limit value for custom cTDP level 3 Short term Power Limit value for...
Definition: FspsUpd.h:2747
UINT8 SerialIoGpio
Offset 0x06CA - Enable Pch Serial IO GPIO Determines if enable Serial IO GPIO.
Definition: FspsUpd.h:1731
UINT8 SataRstIrrt
Offset 0x06B5 - PCH Sata Rst Irrt Intel Rapid Recovery Technology.
Definition: FspsUpd.h:1647
UINT8 SataRstRaidAlternateId
Offset 0x06B0 - PCH Sata Rst Raid Alternate Id Enable RAID Alternate ID.
Definition: FspsUpd.h:1617
UINT8 PchIshI2c1GpioAssign
Offset 0x0354 - Enable PCH ISH I2C1 GPIO pins assigned 0: Disable; 1: Enable.
Definition: FspsUpd.h:1090
UINT8 TccActivationOffset
Offset 0x07AA - TCC Activation Offset TCC Activation Offset.
Definition: FspsUpd.h:2275
UINT8 Custom3ConfigTdpControl
Offset 0x07B6 - Custom Config Tdp Control Config Tdp Control (0/1/2) value for custom cTDP level 1...
Definition: FspsUpd.h:2341
UINT32 CpuS3ResumeMtrrData
Offset 0x02E4 - CpuS3ResumeMtrrData Pointer to CPU S3 Resume MTRR Data.
Definition: FspsUpd.h:764
UINT16 PchTemperatureHotLevel
Offset 0x0702 - Thermal Device Temperature Decides the temperature.
Definition: FspsUpd.h:1957
UINT8 SlowSlewRateForSa
Offset 0x027C - Slew Rate configuration for Deep Package C States for VR SA domain Slew Rate configur...
Definition: FspsUpd.h:661
UINT8 PchHdaIoBufferVoltage
Offset 0x0337 - IO Buffer Voltage I/O Buffer Voltage Mode Select: 0: 3.3V, 1: 1.8V.
Definition: FspsUpd.h:959
UINT16 CstateLatencyControl0Irtl
Offset 0x083C - Interrupt Response Time Limit of C-State LatencyContol0 Interrupt Response Time Limit...
Definition: FspsUpd.h:2661
UINT8 DisableProcHotOut
Offset 0x07D2 - Enable or Disable PROCHOT# signal being driven externally Enable or Disable PROCHOT# ...
Definition: FspsUpd.h:2499
UINT8 PchPmMeWakeSts
Offset 0x0658 - PCH Pm ME_WAKE_STS Clear the ME_WAKE_STS bit in the Power and Reset Status (PRSTS) re...
Definition: FspsUpd.h:1526
UINT8 MlcStreamerPrefetcher
Offset 0x07BE - Enable or Disable MLC Streamer Prefetcher Enable or Disable MLC Streamer Prefetcher; ...
Definition: FspsUpd.h:2382
UINT16 DefaultSid
Offset 0x0202 - Subsystem Device ID for SA devices Subsystem ID that will be programmed to SA devices...
Definition: FspsUpd.h:474
UINT8 ScsEmmcHs400Enabled
Offset 0x0032 - Enable eMMC HS400 Mode Enable eMMC HS400 Mode.
Definition: FspsUpd.h:149
UINT16 CpuS3ResumeMtrrDataSize
Offset 0x02F8 - CpuS3ResumeMtrrDataSize Size of S3 resume MTRR data.
Definition: FspsUpd.h:780
UINT8 ThreeStrikeCounterDisable
Offset 0x0888 - Set Three Strike Counter Disable False (default): Three Strike counter will be increm...
Definition: FspsUpd.h:2812
UINT8 CstateLatencyControl0TimeUnit
Offset 0x07E0 - TimeUnit for C-State Latency Control0 TimeUnit for C-State Latency Control0; Valid va...
Definition: FspsUpd.h:2586
UINT8 PchSkyCamPortCDCtleEnable
Offset 0x0305 - Enable SkyCam PortCD Ctle Enable/disable PortCD Ctle.
Definition: FspsUpd.h:850
UINT8 ScsEmmcEnabled
Offset 0x0031 - Enable eMMC Controller Enable/disable eMMC Controller.
Definition: FspsUpd.h:143
Generated on Thu Jun 28 2018 21:44:49 for Kabylake Intel(R) Firmware Support Package (FSP) Integration Guide by
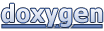