![]() |
W60X_Arduino
|
Functions | |
WiFiUDP::WiFiUDP () | |
This function is constructor, it's used to creates a named instance of the WiFiUDP class that can send and receive UDP messages. More... | |
WiFiUDP::WiFiUDP (const WiFiUDP &other) | |
WiFiUDP & | WiFiUDP::operator= (const WiFiUDP &rhs) |
WiFiUDP::~WiFiUDP () | |
This function is deconstructor, it's used to release WiFiUDP class. More... | |
WiFiUDP::operator bool () const | |
virtual uint8_t | WiFiUDP::begin (uint16_t port) |
This function is used to initializes the WiFiUDP library and network settings, Starts UDP socket, listening at local port. More... | |
virtual void | WiFiUDP::stop () |
This function is used to disconnect from the server. Release any resource being used during the UDP session. More... | |
uint8_t | WiFiUDP::beginMulticast (IPAddress interfaceAddr, IPAddress multicast, uint16_t port) |
This function is used to join a multicast group and listen on the given port. More... | |
virtual int | WiFiUDP::beginPacket (IPAddress ip, uint16_t port) |
This function is used to starts a connection to write UDP data to the remote connection. More... | |
virtual int | WiFiUDP::beginPacket (const char *host, uint16_t port) |
This function is used to starts a connection to write UDP data to the remote connection. More... | |
virtual int | WiFiUDP::beginPacketMulticast (IPAddress multicastAddress, uint16_t port, IPAddress interfaceAddress, int ttl=1) |
This function is used to start building up a packet to send to the multicast address. More... | |
virtual int | WiFiUDP::endPacket () |
This function is used to called after writing UDP data to the remote connection. It finishes off the packet and send it. More... | |
virtual size_t | WiFiUDP::write (uint8_t) |
This function is used to writes UDP data to the remote connection. More... | |
virtual size_t | WiFiUDP::write (const uint8_t *buffer, size_t size) |
This function is used to writes UDP data to the remote connection. More... | |
virtual int | WiFiUDP::parsePacket () |
It starts processing the next available incoming packet, checks for the presence of a UDP packet, and reports the size. More... | |
virtual int | WiFiUDP::available () |
Get the number of bytes (characters) available for reading from the buffer. This is is data that's already arrived. More... | |
virtual int | WiFiUDP::read () |
Reads UDP data from the specified buffer. If no arguments are given, it will return the next character in the buffer. More... | |
virtual int | WiFiUDP::read (unsigned char *buffer, size_t len) |
Reads UDP data from the specified buffer. If no arguments are given, it will return the next character in the buffer. More... | |
virtual int | WiFiUDP::read (char *buffer, size_t len) |
Reads UDP data from the specified buffer. If no arguments are given, it will return the next character in the buffer. More... | |
virtual int | WiFiUDP::peek () |
Read a byte from the file without advancing to the next one. That is, successive calls to peek() will return the same value, as will the next call to read(). More... | |
virtual void | WiFiUDP::flush () |
Discard any bytes that have been written to the client but not yet read. More... | |
virtual IPAddress | WiFiUDP::remoteIP () |
This function is used to gets the IP address of the remote connection. More... | |
virtual uint16_t | WiFiUDP::remotePort () |
This function is used to gets the port of the remote UDP connection. More... | |
IPAddress | WiFiUDP::destinationIP () |
This function is used to distinguish multicast and ordinary packets. More... | |
uint16_t | WiFiUDP::localPort () |
This function is used to gets the port of the local UDP connection. More... | |
static void | WiFiUDP::stopAll () |
This function is used to stop all WiFiUDP session. More... | |
static void | WiFiUDP::stopAllExcept (WiFiUDP *exC) |
This function is used to stop all WiFiUDP session without exC. More... | |
Detailed Description
Function Documentation
◆ available()
|
virtual |
Get the number of bytes (characters) available for reading from the buffer. This is is data that's already arrived.
- Parameters
-
[in] None [out] None
- Return values
-
0 parsePacket hasn't been called yet other the number of bytes available in the current packet
- Note
- This function can only be successfully called after parsePacket(). available() inherits from the Stream utility class.
Implements UDP.
◆ begin()
|
virtual |
◆ beginMulticast()
This function is used to join a multicast group and listen on the given port.
- Parameters
-
[in] interfaceAddress the local IP address of the interface that should be used, use WiFi.localIP() or WiFi.softAPIP() depending on the interface you need [in] multicast multicast group [in] port port number [out] None
- Return values
-
1 successful 0 failed
- Note
◆ beginPacket() [1/2]
|
virtual |
This function is used to starts a connection to write UDP data to the remote connection.
- Parameters
-
[in] ip the IP address of the remote connection (4 bytes) [in] port the port of the remote connection (int) [out] None
- Return values
-
1 successful 0 there was a problem with the supplied IP address or port
- Note
Implements UDP.
◆ beginPacket() [2/2]
|
virtual |
This function is used to starts a connection to write UDP data to the remote connection.
- Parameters
-
[in] host the address of the remote host. It accepts a character string or an IPAddress [in] port the port of the remote connection (int) [out] None
- Return values
-
1 successful 0 there was a problem with the supplied IP address or port
- Note
Implements UDP.
◆ beginPacketMulticast()
|
virtual |
This function is used to start building up a packet to send to the multicast address.
- Parameters
-
[in] multicastAddress muticast address to send to [in] port port number [in] interfaceAddress the local IP address of the interface that should be used, use WiFi.localIP() or WiFi.softAPIP() depending on the interface you need [in] ttl multicast packet TTL (default is 1) [out] None
- Return values
-
1 successful 0 there was a problem with the supplied IP address or port
- Note
◆ destinationIP()
IPAddress WiFiUDP::destinationIP | ( | ) |
This function is used to distinguish multicast and ordinary packets.
- Parameters
-
[in] None [out] None
- Return values
-
the destination address for incoming packets
- Note
◆ endPacket()
|
virtual |
◆ flush()
|
virtual |
◆ localPort()
uint16_t WiFiUDP::localPort | ( | ) |
This function is used to gets the port of the local UDP connection.
- Parameters
-
[in] None [out] None
- Return values
-
the local port for outgoing packets
- Note
◆ parsePacket()
|
virtual |
It starts processing the next available incoming packet, checks for the presence of a UDP packet, and reports the size.
- Parameters
-
[in] None [out] None
- Return values
-
0 no packets are available other the size of the packet in bytes
- Note
- parsePacket() must be called before reading the buffer with read().
Implements UDP.
◆ peek()
|
virtual |
Read a byte from the file without advancing to the next one.
That is, successive calls to peek() will return the same value, as will the next call to read().
- Parameters
-
[in] None [out] None
- Return values
-
-1 none is available other the next byte or character
- Note
- This function inherited from the Stream class. See the Stream class main page for more information.
Implements UDP.
◆ read() [1/3]
|
virtual |
◆ read() [2/3]
|
virtual |
Reads UDP data from the specified buffer. If no arguments are given, it will return the next character in the buffer.
- Parameters
-
[in] buffer buffer to hold incoming packets (unsigned char*) [in] len maximum size of the buffer (int) [out] None
- Return values
-
-1 no buffer is available other the size of the buffer
- Note
Implements UDP.
◆ read() [3/3]
|
inlinevirtual |
Reads UDP data from the specified buffer. If no arguments are given, it will return the next character in the buffer.
- Parameters
-
[in] buffer buffer to hold incoming packets (char*) [in] len maximum size of the buffer (int) [out] None
- Return values
-
-1 no buffer is available other the size of the buffer
- Note
Implements UDP.
◆ remoteIP()
|
virtual |
This function is used to gets the IP address of the remote connection.
- Parameters
-
[in] None [out] None
- Return values
-
the IP address of the host who sent the current incoming packet(4 bytes)
- Note
- This function must be called after parsePacket().
Implements UDP.
◆ remotePort()
|
virtual |
This function is used to gets the port of the remote UDP connection.
- Parameters
-
[in] None [out] None
- Return values
-
The port of the host who sent the current incoming packet
- Note
- This function must be called after parsePacket().
Implements UDP.
◆ stop()
|
virtual |
◆ stopAll()
|
static |
This function is used to stop all WiFiUDP session.
- Parameters
-
[in] None [out] None
- Return values
-
None
- Note
◆ stopAllExcept()
|
static |
This function is used to stop all WiFiUDP session without exC.
- Parameters
-
[in] None [out] None
- Return values
-
None
- Note
◆ WiFiUDP()
WiFiUDP::WiFiUDP | ( | ) |
◆ write() [1/2]
|
virtual |
This function is used to writes UDP data to the remote connection.
- Parameters
-
[in] the outgoing byte [out] None
- Return values
-
single byte into the packet
- Note
- Must be wrapped between beginPacket() and endPacket(). beginPacket() initializes the packet of data, it is not sent until endPacket() is called.
Implements UDP.
◆ write() [2/2]
|
virtual |
This function is used to writes UDP data to the remote connection.
- Parameters
-
[in] buffer the outgoing message [in] size the size of the buffer [out] None
- Return values
-
bytes size from buffer into the packet
- Note
- Must be wrapped between beginPacket() and endPacket(). beginPacket() initializes the packet of data, it is not sent until endPacket() is called.
Implements UDP.
◆ ~WiFiUDP()
WiFiUDP::~WiFiUDP | ( | ) |
This function is deconstructor, it's used to release WiFiUDP class.
- Parameters
-
[in] None [out] None
- Returns
- None
- Note
Generated by
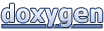