![]() |
W60X_Arduino
|
Functions | |
WiFiClient::WiFiClient () | |
This function is constructor, it's used to creates a client that can connect to to a specified internet IP address and port as defined in client.connect(). More... | |
WiFiClient::WiFiClient (const WiFiClient &) | |
WiFiClient & | WiFiClient::operator= (const WiFiClient &) |
virtual | WiFiClient::~WiFiClient () |
This function is deconstructor, it's used to release WiFiClient class. More... | |
uint8_t | WiFiClient::status () |
return tcp status of WiFiClient. More... | |
virtual int | WiFiClient::connect (IPAddress ip, uint16_t port) |
This function is used to connect to the IP address and port specified in the constructor. More... | |
virtual int | WiFiClient::connect (const char *host, uint16_t port) |
This function is used to connect to the IP address and port specified in the constructor. More... | |
virtual int | WiFiClient::connect (const String host, uint16_t port) |
virtual size_t | WiFiClient::write (uint8_t) |
This function is used to write data to the server the client is connected to. More... | |
virtual size_t | WiFiClient::write (const uint8_t *buf, size_t size) |
This function is used to write data to the server the client is connected to. More... | |
virtual size_t | WiFiClient::write_P (PGM_P buf, size_t size) |
size_t | WiFiClient::write (Stream &stream) |
size_t | WiFiClient::write (Stream &stream, size_t unitSize) __attribute__((deprecated)) |
virtual int | WiFiClient::available () |
Returns the number of bytes available for reading (That is, the amount of data that has been written to the client by the server it is connected to). More... | |
virtual int | WiFiClient::read () |
Read the next byte received from the server the client is connected to (after the last call to read()). More... | |
virtual int | WiFiClient::read (uint8_t *buf, size_t size) |
Read the next byte received from the server the client is connected to (after the last call to read()). More... | |
virtual int | WiFiClient::peek () |
Read a byte from the file without advancing to the next one. That is, successive calls to peek() will return the same value, as will the next call to read(). More... | |
virtual size_t | WiFiClient::peekBytes (uint8_t *buffer, size_t length) |
size_t | WiFiClient::peekBytes (char *buffer, size_t length) |
virtual void | WiFiClient::flush () |
Discard any bytes that have been written to the client but not yet read. More... | |
virtual void | WiFiClient::stop () |
This function is used to disconnect from the server. More... | |
virtual uint8_t | WiFiClient::connected () |
Whether or not the client is connected. More... | |
virtual | WiFiClient::operator bool () |
IPAddress | WiFiClient::remoteIP () |
This function is used to gets the IP address of the remote connection. More... | |
uint16_t | WiFiClient::remotePort () |
This function is used to gets the port of the remote connection. More... | |
IPAddress | WiFiClient::localIP () |
This function is used to gets the IP address of the local tcp connection. More... | |
uint16_t | WiFiClient::localPort () |
This function is used to gets the port of the local tcp connection. More... | |
bool | WiFiClient::getNoDelay () |
This function is used to get whether no delay of the tcp connection. More... | |
void | WiFiClient::setNoDelay (bool nodelay) |
This function is used to set no delay for the tcp connection. More... | |
static void | WiFiClient::setLocalPortStart (uint16_t port) |
This function is used to set local port number. More... | |
size_t | WiFiClient::availableForWrite () |
This function is used to get the length that can be written. More... | |
static void | WiFiClient::stopAll () |
This function is used to stop all WiFiClient session. More... | |
static void | WiFiClient::stopAllExcept (WiFiClient *c) |
This function is used to stop all WiFiClient session without exC. More... | |
void | WiFiClient::keepAlive (uint16_t idle_sec=TCP_DEFAULT_KEEPALIVE_IDLE_SEC, uint16_t intv_sec=TCP_DEFAULT_KEEPALIVE_INTERVAL_SEC, uint8_t count=TCP_DEFAULT_KEEPALIVE_COUNT) |
This function is used to set keep alive. More... | |
bool | WiFiClient::isKeepAliveEnabled () const |
This function is used to get whether enable keep alive. More... | |
uint16_t | WiFiClient::getKeepAliveIdle () const |
This function is used to get idle time interval. More... | |
uint16_t | WiFiClient::getKeepAliveInterval () const |
This function is used to get keep alive time interval. More... | |
uint8_t | WiFiClient::getKeepAliveCount () const |
This function is used to get keep alive count. More... | |
void | WiFiClient::disableKeepAlive () |
This function is used to set disable keep alive. More... | |
Friends | |
class | WiFiServer |
Detailed Description
Function Documentation
◆ available()
|
virtual |
Returns the number of bytes available for reading (That is, the amount of data that has been written to the client by the server it is connected to).
- Parameters
-
[in] None [out] None
- Return values
-
The number of bytes available
- Note
- available() inherits from the Stream utility class.
Implements Client.
◆ availableForWrite()
size_t WiFiClient::availableForWrite | ( | ) |
This function is used to get the length that can be written.
- Parameters
-
[in] None [out] None
- Return values
-
the length that can be written
- Note
◆ connect() [1/2]
|
virtual |
This function is used to connect to the IP address and port specified in the constructor.
- Parameters
-
[in] ip the IP address that the client will connect to (array of 4 bytes) [in] port the port that the client will connect to (int) [out] None
- Return values
-
1 the connection succeeds 0 the connection failed
- Note
Implements Client.
◆ connect() [2/2]
|
virtual |
This function is used to connect to the IP address and port specified in the constructor.
- Parameters
-
[in] host the domain name the client will connect to (string, ex.:"arduino.cc") [in] port the port that the client will connect to (int) [out] None
- Return values
-
1 the connection succeeds 0 the connection failed
- Note
Implements Client.
◆ connected()
|
virtual |
Whether or not the client is connected.
- Parameters
-
[in] None [out] None
- Return values
-
1 the client is connected 0 the client is disconnected
- Note
- that a client is considered connected if the connection has been closed but there is still unread data.
Implements Client.
◆ disableKeepAlive()
|
inline |
This function is used to set disable keep alive.
- Parameters
-
[in] None [out] None
- Returns
- None
- Note
◆ flush()
|
virtual |
◆ getKeepAliveCount()
uint8_t WiFiClient::getKeepAliveCount | ( | ) | const |
This function is used to get keep alive count.
- Parameters
-
[in] None [out] None
- Return values
-
keep alive count
- Note
◆ getKeepAliveIdle()
uint16_t WiFiClient::getKeepAliveIdle | ( | ) | const |
This function is used to get idle time interval.
- Parameters
-
[in] None [out] None
- Return values
-
idle time interval
- Note
◆ getKeepAliveInterval()
uint16_t WiFiClient::getKeepAliveInterval | ( | ) | const |
This function is used to get keep alive time interval.
- Parameters
-
[in] None [out] None
- Return values
-
keep alive time interval
- Note
◆ getNoDelay()
bool WiFiClient::getNoDelay | ( | ) |
This function is used to get whether no delay of the tcp connection.
- Parameters
-
[in] None [out] None
- Return values
-
true no delay false delay
- Note
◆ isKeepAliveEnabled()
bool WiFiClient::isKeepAliveEnabled | ( | ) | const |
This function is used to get whether enable keep alive.
- Parameters
-
[in] None [out] None
- Return values
-
true enable false disable
- Note
◆ keepAlive()
void WiFiClient::keepAlive | ( | uint16_t | idle_sec = TCP_DEFAULT_KEEPALIVE_IDLE_SEC , |
uint16_t | intv_sec = TCP_DEFAULT_KEEPALIVE_INTERVAL_SEC , |
||
uint8_t | count = TCP_DEFAULT_KEEPALIVE_COUNT |
||
) |
This function is used to set keep alive.
- Parameters
-
[in] idle_sec idle time interval [in] intv_sec keep alive time interval [in] count keep alive count [out] None
- Return values
-
None
- Note
◆ localIP()
IPAddress WiFiClient::localIP | ( | ) |
This function is used to gets the IP address of the local tcp connection.
- Parameters
-
[in] None [out] None
- Return values
-
the IP address(4 bytes)
- Note
◆ localPort()
uint16_t WiFiClient::localPort | ( | ) |
This function is used to gets the port of the local tcp connection.
- Parameters
-
[in] None [out] None
- Return values
-
the local port number
- Note
◆ peek()
|
virtual |
Read a byte from the file without advancing to the next one.
That is, successive calls to peek() will return the same value, as will the next call to read().
- Parameters
-
[in] None [out] None
- Return values
-
-1 none is available other the next byte or character
- Note
- This function inherited from the Stream class. See the Stream class main page for more information.
Implements Client.
◆ read() [1/2]
|
virtual |
◆ read() [2/2]
|
virtual |
◆ remoteIP()
IPAddress WiFiClient::remoteIP | ( | ) |
This function is used to gets the IP address of the remote connection.
- Parameters
-
[in] None [out] None
- Return values
-
the IP address(4 bytes)
- Note
◆ remotePort()
uint16_t WiFiClient::remotePort | ( | ) |
This function is used to gets the port of the remote connection.
- Parameters
-
[in] None [out] None
- Return values
-
The port number
- Note
◆ setLocalPortStart()
|
inlinestatic |
This function is used to set local port number.
- Parameters
-
[in] port number [out] None
- Returns
- None
- Note
◆ setNoDelay()
void WiFiClient::setNoDelay | ( | bool | nodelay | ) |
This function is used to set no delay for the tcp connection.
- Parameters
-
[in] None [out] None
- Return values
-
the local port number
- Note
◆ status()
uint8_t WiFiClient::status | ( | ) |
◆ stop()
|
virtual |
This function is used to disconnect from the server.
- Parameters
-
[in] None [out] None
- Returns
- None
- Note
Implements Client.
◆ stopAll()
|
static |
This function is used to stop all WiFiClient session.
- Parameters
-
[in] None [out] None
- Return values
-
None
- Note
◆ stopAllExcept()
|
static |
This function is used to stop all WiFiClient session without exC.
- Parameters
-
[in] None [out] None
- Return values
-
None
- Note
◆ WiFiClient()
WiFiClient::WiFiClient | ( | ) |
This function is constructor, it's used to creates a client that can connect to to a specified internet IP address and port as defined in client.connect().
- Parameters
-
[in] None [out] None
- Returns
- None
- Note
◆ write() [1/2]
|
virtual |
This function is used to write data to the server the client is connected to.
- Parameters
-
[in] the char to write [out] None
- Return values
-
the number of characters written. it is not necessary to read this value.
- Note
Implements Client.
◆ write() [2/2]
|
virtual |
This function is used to write data to the server the client is connected to.
- Parameters
-
[in] buf the byte to write [in] size the size of the buf [out] None
- Return values
-
the number of characters written. it is not necessary to read this value.
- Note
Implements Client.
◆ ~WiFiClient()
|
virtual |
This function is deconstructor, it's used to release WiFiClient class.
- Parameters
-
[in] None [out] None
- Returns
- None
- Note
Generated by
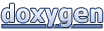