![]() |
W60X_Arduino
|
Inherits Stream.
Inherited by WiFiUDP.
Public Member Functions | |
virtual uint8_t | begin (uint16_t)=0 |
This function is used to initializes the UDP library and network settings, Starts UDP socket, listening at local port. More... | |
virtual void | stop ()=0 |
This function is used to disconnect from the server. Release any resource being used during the UDP session. More... | |
virtual int | beginPacket (IPAddress ip, uint16_t port)=0 |
This function is used to starts a connection to write UDP data to the remote connection. More... | |
virtual int | beginPacket (const char *host, uint16_t port)=0 |
This function is used to starts a connection to write UDP data to the remote connection. More... | |
virtual int | endPacket ()=0 |
This function is used to called after writing UDP data to the remote connection. It finishes off the packet and send it. More... | |
virtual size_t | write (uint8_t)=0 |
This function is used to writes UDP data to the remote connection. More... | |
virtual size_t | write (const uint8_t *buffer, size_t size)=0 |
This function is used to writes UDP data to the remote connection. More... | |
virtual int | parsePacket ()=0 |
It starts processing the next available incoming packet, checks for the presence of a UDP packet, and reports the size. More... | |
virtual int | available ()=0 |
Get the number of bytes (characters) available for reading from the buffer. This is is data that's already arrived. More... | |
virtual int | read ()=0 |
Reads UDP data from the specified buffer. If no arguments are given, it will return the next character in the buffer. More... | |
virtual int | read (unsigned char *buffer, size_t len)=0 |
Reads UDP data from the specified buffer. If no arguments are given, it will return the next character in the buffer. More... | |
virtual int | read (char *buffer, size_t len)=0 |
Reads UDP data from the specified buffer. If no arguments are given, it will return the next character in the buffer. More... | |
virtual int | peek ()=0 |
Read a byte from the file without advancing to the next one. That is, successive calls to peek() will return the same value, as will the next call to read(). More... | |
virtual void | flush ()=0 |
Discard any bytes that have been written to the client but not yet read. More... | |
virtual IPAddress | remoteIP ()=0 |
This function is used to gets the IP address of the remote connection. More... | |
virtual uint16_t | remotePort ()=0 |
This function is used to gets the port of the remote UDP connection. More... | |
![]() | |
void | setTimeout (unsigned long timeout) |
setTimeout() sets the maximum milliseconds to wait for stream data, it defaults to 1000 milliseconds. This function is part of the Stream class, and is called by any class that inherits from it (Wire, Serial, etc). See the Stream class main page for more information. More... | |
bool | find (const char *target) |
find() reads data from the stream until the target string of given length is found The function returns true if target string is found, false if timed out. More... | |
bool | find (uint8_t *target) |
find() reads data from the stream until the target string of given length is found The function returns true if target string is found, false if timed out. More... | |
bool | find (const char *target, size_t length) |
find() reads data from the stream until the target string of given length is found The function returns true if target string is found, false if timed out. More... | |
bool | find (const uint8_t *target, size_t length) |
find() reads data from the stream until the target string of given length is found The function returns true if target string is found, false if timed out. More... | |
bool | find (char target) |
find() reads data from the stream until the target string of given length is found The function returns true if target string is found, false if timed out. More... | |
bool | findUntil (const char *target, const char *terminator) |
findUntil() reads data from the stream until the target string of given length or terminator string is found. More... | |
bool | findUntil (const uint8_t *target, const char *terminator) |
findUntil() reads data from the stream until the target string of given length or terminator string is found. More... | |
bool | findUntil (const char *target, size_t targetLen, const char *terminate, size_t termLen) |
findUntil() reads data from the stream until the target string of given length or terminator string is found. More... | |
bool | findUntil (const uint8_t *target, size_t targetLen, const char *terminate, size_t termLen) |
findUntil() reads data from the stream until the target string of given length or terminator string is found. More... | |
long | parseInt () |
parseInt() returns the first valid (long) integer number from the serial buffer. Characters that are not integers (or the minus sign) are skipped. More... | |
float | parseFloat () |
parseFloat() returns the first valid floating point number from the current position. Initial characters that are not digits (or the minus sign) are skipped. parseFloat() is terminated by the first character that is not a floating point number. More... | |
virtual size_t | readBytes (char *buffer, size_t length) |
readBytes() read characters from a stream into a buffer. The function terminates if the determined length has been read, or it times out (see setTimeout()). More... | |
virtual size_t | readBytes (uint8_t *buffer, size_t length) |
readBytes() read characters from a stream into a buffer. The function terminates if the determined length has been read, or it times out (see setTimeout()). More... | |
size_t | readBytesUntil (char terminator, char *buffer, size_t length) |
readBytesUntil() reads characters from a stream into a buffer. The function terminates if the terminator character is detected, the determined length has been read, or it times out (see setTimeout()). More... | |
size_t | readBytesUntil (char terminator, uint8_t *buffer, size_t length) |
readBytesUntil() reads characters from a stream into a buffer. The function terminates if the terminator character is detected, the determined length has been read, or it times out (see setTimeout()). More... | |
String | readString () |
readString() reads characters from a stream into a string. The function terminates if it times out (see setTimeout()). More... | |
String | readStringUntil (char terminator) |
readStringUntil() reads characters from a stream into a string. The function terminates if the terminator character is detected or it times out (see setTimeout()). More... | |
![]() | |
int | getWriteError () |
This function is used to get write error number. More... | |
void | clearWriteError () |
This function is used to clear write error number. More... | |
size_t | write (const char *str) |
This function is used to write buffer to the interface defined by the object. More... | |
size_t | write (const char *buffer, size_t size) |
This function is used to write buffer to the interface defined by the object. More... | |
size_t | print (const String &) |
This function is used to print buffer to the interface defined by the object. More... | |
size_t | print (const char []) |
This function is used to print buffer to the interface defined by the object. More... | |
size_t | print (char) |
This function is used to print buffer to the interface defined by the object. More... | |
size_t | print (unsigned char, int=DEC) |
This function is used to print target to the interface defined by the object. More... | |
size_t | print (int, int=DEC) |
This function is used to print target to the interface defined by the object. More... | |
size_t | print (unsigned int, int=DEC) |
This function is used to print target to the interface defined by the object. More... | |
size_t | print (long, int=DEC) |
This function is used to print target to the interface defined by the object. More... | |
size_t | print (unsigned long, int=DEC) |
This function is used to print target to the interface defined by the object. More... | |
size_t | print (double, int=BIN) |
This function is used to print target to the interface defined by the object. More... | |
size_t | print (const Printable &) |
This function is used to print target to the interface defined by the object. More... | |
size_t | println (void) |
This function is used to print target to the interface defined by the object with carriage ret ('') and new line (' '). More... | |
size_t | println (const String &s) |
This function is used to print target to the interface defined by the object with carriage ret ('') and new line (' '). More... | |
size_t | println (const char []) |
This function is used to print target to the interface defined by the object with carriage ret ('') and new line (' '). More... | |
size_t | println (char) |
This function is used to print target to the interface defined by the object with carriage ret ('') and new line (' '). More... | |
size_t | println (unsigned char, int=DEC) |
This function is used to print target to the interface defined by the object with carriage ret ('') and new line (' '). More... | |
size_t | println (int, int=DEC) |
This function is used to print target to the interface defined by the object with carriage ret ('') and new line (' '). More... | |
size_t | println (unsigned int, int=DEC) |
This function is used to print target to the interface defined by the object with carriage ret ('') and new line (' '). More... | |
size_t | println (long, int=DEC) |
This function is used to print target to the interface defined by the object with carriage ret ('') and new line (' '). More... | |
size_t | println (unsigned long, int=DEC) |
This function is used to print target to the interface defined by the object with carriage ret ('') and new line (' '). More... | |
size_t | println (double, int=BIN) |
This function is used to print target to the interface defined by the object with carriage ret ('') and new line (' '). More... | |
size_t | println (const Printable &) |
This function is used to print target to the interface defined by the object with carriage ret ('') and new line (' '). More... | |
Additional Inherited Members | |
![]() | |
int | timedRead () |
int | timedPeek () |
int | peekNextDigit () |
long | parseInt (char skipChar) |
float | parseFloat (char skipChar) |
![]() | |
void | setWriteError (int err=1) |
![]() | |
unsigned long | _timeout |
unsigned long | _startMillis |
Member Function Documentation
◆ available()
|
pure virtual |
Get the number of bytes (characters) available for reading from the buffer. This is is data that's already arrived.
- Parameters
-
[in] None [out] None
- Return values
-
0 parsePacket hasn't been called yet other the number of bytes available in the current packet
- Note
- This function can only be successfully called after UDP.parsePacket(). available() inherits from the Stream utility class.
Implements Stream.
Implemented in WiFiUDP.
◆ begin()
|
pure virtual |
◆ beginPacket() [1/2]
|
pure virtual |
This function is used to starts a connection to write UDP data to the remote connection.
- Parameters
-
[in] ip the IP address of the remote connection (4 bytes) [in] port the port of the remote connection (int) [out] None
- Return values
-
1 successful 0 there was a problem with the supplied IP address or port
- Note
Implemented in WiFiUDP.
◆ beginPacket() [2/2]
|
pure virtual |
This function is used to starts a connection to write UDP data to the remote connection.
- Parameters
-
[in] host the address of the remote host. It accepts a character string or an IPAddress [in] port the port of the remote connection (int) [out] None
- Return values
-
1 successful 0 there was a problem with the supplied IP address or port
- Note
Implemented in WiFiUDP.
◆ endPacket()
|
pure virtual |
◆ flush()
|
pure virtual |
◆ parsePacket()
|
pure virtual |
It starts processing the next available incoming packet, checks for the presence of a UDP packet, and reports the size.
- Parameters
-
[in] None [out] None
- Return values
-
0 no packets are available other the size of the packet in bytes
- Note
- parsePacket() must be called before reading the buffer with UDP.read().
Implemented in WiFiUDP.
◆ peek()
|
pure virtual |
Read a byte from the file without advancing to the next one.
That is, successive calls to peek() will return the same value, as will the next call to read().
- Parameters
-
[in] None [out] None
- Return values
-
-1 none is available other the next byte or character
- Note
- This function inherited from the Stream class. See the Stream class main page for more information.
Implements Stream.
Implemented in WiFiUDP.
◆ read() [1/3]
|
pure virtual |
◆ read() [2/3]
|
pure virtual |
Reads UDP data from the specified buffer. If no arguments are given, it will return the next character in the buffer.
- Parameters
-
[in] buffer buffer to hold incoming packets (unsigned char*) [in] len maximum size of the buffer (int) [out] None
- Return values
-
-1 no buffer is available other the size of the buffer
- Note
Implemented in WiFiUDP.
◆ read() [3/3]
|
pure virtual |
Reads UDP data from the specified buffer. If no arguments are given, it will return the next character in the buffer.
- Parameters
-
[in] buffer buffer to hold incoming packets (char*) [in] len maximum size of the buffer (int) [out] None
- Return values
-
-1 no buffer is available other the size of the buffer
- Note
Implemented in WiFiUDP.
◆ remoteIP()
|
pure virtual |
This function is used to gets the IP address of the remote connection.
- Parameters
-
[in] None [out] None
- Return values
-
the IP address of the host who sent the current incoming packet(4 bytes)
- Note
- This function must be called after UDP.parsePacket().
Implemented in WiFiUDP.
◆ remotePort()
|
pure virtual |
This function is used to gets the port of the remote UDP connection.
- Parameters
-
[in] None [out] None
- Return values
-
The port of the host who sent the current incoming packet
- Note
- This function must be called after UDP.parsePacket().
Implemented in WiFiUDP.
◆ stop()
|
pure virtual |
◆ write() [1/2]
|
pure virtual |
This function is used to writes UDP data to the remote connection.
- Parameters
-
[in] the outgoing byte [out] None
- Return values
-
single byte into the packet
- Note
- Must be wrapped between beginPacket() and endPacket(). beginPacket() initializes the packet of data, it is not sent until endPacket() is called.
Implements Print.
Implemented in WiFiUDP.
◆ write() [2/2]
|
pure virtual |
This function is used to writes UDP data to the remote connection.
- Parameters
-
[in] buffer the outgoing message [in] size the size of the buffer [out] None
- Return values
-
bytes size from buffer into the packet
- Note
- Must be wrapped between beginPacket() and endPacket(). beginPacket() initializes the packet of data, it is not sent until endPacket() is called.
Reimplemented from Print.
Implemented in WiFiUDP.
Generated by
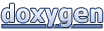