![]() |
W60X_Arduino
|
Functions | |
virtual int | Stream::available ()=0 |
available() gets the number of bytes available in the stream. This is only for bytes that have already arrived. More... | |
virtual int | Stream::read ()=0 |
read() reads characters from an incoming stream to the buffer. More... | |
virtual int | Stream::peek ()=0 |
Read a byte from the file without advancing to the next one. That is, successive calls to peek() will return the same value, as will the next call to read(). More... | |
void | Stream::setTimeout (unsigned long timeout) |
setTimeout() sets the maximum milliseconds to wait for stream data, it defaults to 1000 milliseconds. This function is part of the Stream class, and is called by any class that inherits from it (Wire, Serial, etc). See the Stream class main page for more information. More... | |
bool | Stream::find (const char *target) |
find() reads data from the stream until the target string of given length is found The function returns true if target string is found, false if timed out. More... | |
bool | Stream::find (uint8_t *target) |
find() reads data from the stream until the target string of given length is found The function returns true if target string is found, false if timed out. More... | |
bool | Stream::find (const char *target, size_t length) |
find() reads data from the stream until the target string of given length is found The function returns true if target string is found, false if timed out. More... | |
bool | Stream::find (const uint8_t *target, size_t length) |
find() reads data from the stream until the target string of given length is found The function returns true if target string is found, false if timed out. More... | |
bool | Stream::find (char target) |
find() reads data from the stream until the target string of given length is found The function returns true if target string is found, false if timed out. More... | |
bool | Stream::findUntil (const char *target, const char *terminator) |
findUntil() reads data from the stream until the target string of given length or terminator string is found. More... | |
bool | Stream::findUntil (const uint8_t *target, const char *terminator) |
findUntil() reads data from the stream until the target string of given length or terminator string is found. More... | |
bool | Stream::findUntil (const char *target, size_t targetLen, const char *terminate, size_t termLen) |
findUntil() reads data from the stream until the target string of given length or terminator string is found. More... | |
bool | Stream::findUntil (const uint8_t *target, size_t targetLen, const char *terminate, size_t termLen) |
findUntil() reads data from the stream until the target string of given length or terminator string is found. More... | |
long | Stream::parseInt () |
parseInt() returns the first valid (long) integer number from the serial buffer. Characters that are not integers (or the minus sign) are skipped. More... | |
float | Stream::parseFloat () |
parseFloat() returns the first valid floating point number from the current position. Initial characters that are not digits (or the minus sign) are skipped. parseFloat() is terminated by the first character that is not a floating point number. More... | |
virtual size_t | Stream::readBytes (char *buffer, size_t length) |
readBytes() read characters from a stream into a buffer. The function terminates if the determined length has been read, or it times out (see setTimeout()). More... | |
virtual size_t | Stream::readBytes (uint8_t *buffer, size_t length) |
readBytes() read characters from a stream into a buffer. The function terminates if the determined length has been read, or it times out (see setTimeout()). More... | |
size_t | Stream::readBytesUntil (char terminator, char *buffer, size_t length) |
readBytesUntil() reads characters from a stream into a buffer. The function terminates if the terminator character is detected, the determined length has been read, or it times out (see setTimeout()). More... | |
size_t | Stream::readBytesUntil (char terminator, uint8_t *buffer, size_t length) |
readBytesUntil() reads characters from a stream into a buffer. The function terminates if the terminator character is detected, the determined length has been read, or it times out (see setTimeout()). More... | |
String | Stream::readString () |
readString() reads characters from a stream into a string. The function terminates if it times out (see setTimeout()). More... | |
String | Stream::readStringUntil (char terminator) |
readStringUntil() reads characters from a stream into a string. The function terminates if the terminator character is detected or it times out (see setTimeout()). More... | |
Detailed Description
Function Documentation
◆ available()
|
pure virtual |
available() gets the number of bytes available in the stream. This is only for bytes that have already arrived.
This function is part of the Stream class, and is called by any class that inherits from it (Wire, Serial, etc). See the Stream class main page for more information.
- Parameters
-
[in] None
- Returns
- stream : an instance of a class that inherits from Stream
- Note
Implemented in WiFiUDP, WiFiClient, HardwareSerial, UDP, Client, and TwoWire.
◆ find() [1/5]
bool Stream::find | ( | const char * | target | ) |
find() reads data from the stream until the target string of given length is found The function returns true if target string is found, false if timed out.
This function is part of the Stream class, and is called by any class that inherits from it (Wire, Serial, etc). See the Stream class main page for more information.
- Parameters
-
[in] target : the string to search for (char)
- Returns
- boolean
- Note
◆ find() [2/5]
|
inline |
find() reads data from the stream until the target string of given length is found The function returns true if target string is found, false if timed out.
This function is part of the Stream class, and is called by any class that inherits from it (Wire, Serial, etc). See the Stream class main page for more information.
- Parameters
-
[in] target : the string to search for (char)
- Returns
- boolean
- Note
◆ find() [3/5]
bool Stream::find | ( | const char * | target, |
size_t | length | ||
) |
find() reads data from the stream until the target string of given length is found The function returns true if target string is found, false if timed out.
This function is part of the Stream class, and is called by any class that inherits from it (Wire, Serial, etc). See the Stream class main page for more information.
- Parameters
-
[in] target : the string to search for (char) [in] length : Specify the length.
- Returns
- boolean
- Note
◆ find() [4/5]
|
inline |
find() reads data from the stream until the target string of given length is found The function returns true if target string is found, false if timed out.
This function is part of the Stream class, and is called by any class that inherits from it (Wire, Serial, etc). See the Stream class main page for more information.
- Parameters
-
[in] target : the string to search for (char) [in] length : Specify the length.
- Returns
- boolean
- Note
◆ find() [5/5]
|
inline |
find() reads data from the stream until the target string of given length is found The function returns true if target string is found, false if timed out.
This function is part of the Stream class, and is called by any class that inherits from it (Wire, Serial, etc). See the Stream class main page for more information.
- Parameters
-
[in] target : the string to search for (char)
- Returns
- boolean
- Note
◆ findUntil() [1/4]
bool Stream::findUntil | ( | const char * | target, |
const char * | terminator | ||
) |
findUntil() reads data from the stream until the target string of given length or terminator string is found.
The function returns true if target string is found, false if timed out
This function is part of the Stream class, and is called by any class that inherits from it (Wire, Serial, etc). See the Stream class main page for more information.
- Parameters
-
[in] target : the string to search for (char) [in] terminal : the terminal string in the search (char)
- Returns
- boolean
- Note
◆ findUntil() [2/4]
|
inline |
findUntil() reads data from the stream until the target string of given length or terminator string is found.
The function returns true if target string is found, false if timed out
This function is part of the Stream class, and is called by any class that inherits from it (Wire, Serial, etc). See the Stream class main page for more information.
- Parameters
-
[in] target : the string to search for (char) [in] terminal : the terminal string in the search (char)
- Returns
- boolean
- Note
◆ findUntil() [3/4]
bool Stream::findUntil | ( | const char * | target, |
size_t | targetLen, | ||
const char * | terminate, | ||
size_t | termLen | ||
) |
findUntil() reads data from the stream until the target string of given length or terminator string is found.
The function returns true if target string is found, false if timed out
This function is part of the Stream class, and is called by any class that inherits from it (Wire, Serial, etc). See the Stream class main page for more information.
- Parameters
-
[in] target : the string to search for (char) [in] terminal : the terminal string in the search (char) [in] terminate : Specify the terminate [in] termLen : Specify the termLen
- Returns
- boolean
- Note
◆ findUntil() [4/4]
|
inline |
findUntil() reads data from the stream until the target string of given length or terminator string is found.
The function returns true if target string is found, false if timed out
This function is part of the Stream class, and is called by any class that inherits from it (Wire, Serial, etc). See the Stream class main page for more information.
- Parameters
-
[in] target : the string to search for (char) [in] terminal : the terminal string in the search (char) [in] terminate : Specify the terminate [in] termLen : Specify the termLen
- Returns
- boolean
- Note
◆ parseFloat()
float Stream::parseFloat | ( | ) |
parseFloat() returns the first valid floating point number from the current position. Initial characters that are not digits (or the minus sign) are skipped. parseFloat() is terminated by the first character that is not a floating point number.
This function is part of the Stream class, and is called by any class that inherits from it (Wire, Serial, etc). See the Stream class main page for more information.
- Parameters
-
[in] None
- Returns
- float
- Note
◆ parseInt()
long Stream::parseInt | ( | ) |
parseInt() returns the first valid (long) integer number from the serial buffer. Characters that are not integers (or the minus sign) are skipped.
In particular:
-- Initial characters that are not digits or a minus sign, are skipped; -- Parsing stops when no characters have been read for a configurable time-out value, or a non-digit is read; -- If no valid digits were read when the time-out (see Stream.setTimeout()) occurs, 0 is returned;
This function is part of the Stream class, and is called by any class that inherits from it (Wire, Serial, etc). See the Stream class main page for more information.
- Parameters
-
[in] None
- Returns
- long
- Note
◆ peek()
|
pure virtual |
Read a byte from the file without advancing to the next one. That is, successive calls to peek() will return the same value, as will the next call to read().
This function is part of the Stream class, and is called by any class that inherits from it (Wire, Serial, etc). See the Stream class main page for more information.
- Parameters
-
[in] None
- Returns
- None
- Note
Implemented in WiFiUDP, WiFiClient, UDP, HardwareSerial, Client, and TwoWire.
◆ read()
|
pure virtual |
read() reads characters from an incoming stream to the buffer.
This function is part of the Stream class, and is called by any class that inherits from it (Wire, Serial, etc). See the Stream class main page for more information.
- Parameters
-
[in] None
- Returns
- the first byte of incoming data available (or -1 if no data is available)
- Note
Implemented in WiFiUDP, WiFiClient, UDP, HardwareSerial, Client, and TwoWire.
◆ readBytes() [1/2]
|
virtual |
readBytes() read characters from a stream into a buffer. The function terminates if the determined length has been read, or it times out (see setTimeout()).
readBytes() returns the number of bytes placed in the buffer. A 0 means no valid data was found.
This function is part of the Stream class, and is called by any class that inherits from it (Wire, Serial, etc). See the Stream class main page for more information.
- Parameters
-
[in] buffer the buffer to store the bytes in (char[] or byte[]) [in]
◆ readBytes() [2/2]
|
inlinevirtual |
readBytes() read characters from a stream into a buffer. The function terminates if the determined length has been read, or it times out (see setTimeout()).
readBytes() returns the number of bytes placed in the buffer. A 0 means no valid data was found.
This function is part of the Stream class, and is called by any class that inherits from it (Wire, Serial, etc). See the Stream class main page for more information.
- Parameters
-
[in] buffer the buffer to store the bytes in (char[] or byte[]) [in]
◆ readBytesUntil() [1/2]
size_t Stream::readBytesUntil | ( | char | terminator, |
char * | buffer, | ||
size_t | length | ||
) |
readBytesUntil() reads characters from a stream into a buffer. The function terminates if the terminator character is detected, the determined length has been read, or it times out (see setTimeout()).
readBytesUntil() returns the number of bytes placed in the buffer. A 0 means no valid data was found.
This function is part of the Stream class, and is called by any class that inherits from it (Wire, Serial, etc). See the Stream class main page for more information.
- Parameters
-
[in] character : the character to search for (char) [in] buffer the buffer to store the bytes in (char[] or byte[]) [in] length : the number of bytes to read (int)
- Returns
- The number of bytes placed in the buffer
- Note
◆ readBytesUntil() [2/2]
|
inline |
readBytesUntil() reads characters from a stream into a buffer. The function terminates if the terminator character is detected, the determined length has been read, or it times out (see setTimeout()).
readBytesUntil() returns the number of bytes placed in the buffer. A 0 means no valid data was found.
This function is part of the Stream class, and is called by any class that inherits from it (Wire, Serial, etc). See the Stream class main page for more information.
- Parameters
-
[in] character : the character to search for (char) [in] buffer the buffer to store the bytes in (char[] or byte[]) [in] length : the number of bytes to read (int)
- Returns
- The number of bytes placed in the buffer
- Note
◆ readString()
String Stream::readString | ( | ) |
readString() reads characters from a stream into a string. The function terminates if it times out (see setTimeout()).
This function is part of the Stream class, and is called by any class that inherits from it (Wire, Serial, etc). See the Stream class main page for more information.
- Parameters
-
[in] none
- Returns
- A string read from a stream
- Note
◆ readStringUntil()
String Stream::readStringUntil | ( | char | terminator | ) |
readStringUntil() reads characters from a stream into a string. The function terminates if the terminator character is detected or it times out (see setTimeout()).
This function is part of the Stream class, and is called by any class that inherits from it (Wire, Serial, etc). See the Stream class main page for more information.
- Parameters
-
[in] terminator : the character to search for (char) [out]
◆ setTimeout()
void Stream::setTimeout | ( | unsigned long | timeout | ) |
setTimeout() sets the maximum milliseconds to wait for stream data, it defaults to 1000 milliseconds. This function is part of the Stream class, and is called by any class that inherits from it (Wire, Serial, etc). See the Stream class main page for more information.
- Parameters
-
[in] timeout : timeout duration in milliseconds (long).
- Returns
- None
- Note
Generated by
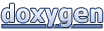