![]() |
W60X_Arduino
|
SPI library. More...
Functions | |
void | SPIClass::begin (void) |
Initialize the SPI instance. More... | |
void | SPIClass::end (void) |
Deinitialize the SPI instance and stop it. More... | |
void | SPIClass::beginTransaction (SPISettings settings) |
This function should be used to configure the SPI instance in case you don't use the default parameters set by the begin() function. More... | |
void | SPIClass::endTransaction (void) |
settings associated to the SPI instance. More... | |
uint8_t | SPIClass::transfer (uint8_t _data) |
Transfer one byte on the SPI bus. More... | |
uint16_t | SPIClass::transfer16 (uint16_t _data) |
Transfer two bytes on the SPI bus in 16 bits format. More... | |
void | SPIClass::transferWrite (void *_buf, size_t _count) |
send several bytes. More... | |
void | SPIClass::transferRead (void *_buf, size_t _count) |
receive several bytes. More... | |
void | SPIClass::transfer (void *_buf, size_t _count) |
Transfer several bytes. Only one buffer used to send and receive data. More... | |
void | SPIClass::transfer (void *_bufout, void *_bufin, size_t _count) |
Transfer several bytes. One buffer contains the data to send and another one will contains the data received. More... | |
void | SPIClass::setBitOrder (BitOrder) |
Deprecated function. Configure the bit order: MSB first or LSB first. More... | |
void | SPIClass::setDataMode (uint8_t _mode) |
Deprecated function. Configure the data mode (clock polarity and clock phase) More... | |
void | SPIClass::setFrequency (uint32_t freq) |
Configure the spi frequency. More... | |
Detailed Description
SPI library.
Function Documentation
◆ begin()
void SPIClass::begin | ( | void | ) |
Initialize the SPI instance.
- Parameters
-
[in] none
- Returns
- none
- Note
- none
◆ beginTransaction()
void SPIClass::beginTransaction | ( | SPISettings | settings | ) |
This function should be used to configure the SPI instance in case you don't use the default parameters set by the begin() function.
- Parameters
-
[in] settings SPI settings(clock speed, bit order, data mode).
- Returns
- none
- Note
- none
◆ end()
void SPIClass::end | ( | void | ) |
Deinitialize the SPI instance and stop it.
- Parameters
-
[in] none
- Returns
- none
- Note
- none
◆ endTransaction()
void SPIClass::endTransaction | ( | void | ) |
settings associated to the SPI instance.
- Parameters
-
[in] none
- Returns
- none
- Note
- none
◆ setBitOrder()
void SPIClass::setBitOrder | ( | BitOrder | _bitOrder | ) |
Deprecated function. Configure the bit order: MSB first or LSB first.
- Parameters
-
[in] _bitOrder MSBFIRST or LSBFIRST
- Returns
- none
- Note
- none
◆ setDataMode()
void SPIClass::setDataMode | ( | uint8_t | _mode | ) |
Deprecated function. Configure the data mode (clock polarity and clock phase)
- Parameters
-
[in] _mode SPI_MODE0, SPI_MODE1, SPI_MODE2 or SPI_MODE3
- Returns
- none
- Note
- none
◆ setFrequency()
void SPIClass::setFrequency | ( | uint32_t | freq | ) |
Configure the spi frequency.
- Parameters
-
[in] freq max 20MHz
- Returns
- none
- Note
- none
◆ transfer() [1/3]
uint8_t SPIClass::transfer | ( | uint8_t | data | ) |
Transfer one byte on the SPI bus.
- Parameters
-
[in] data byte to send.
- Returns
- byte received from the slave
- Note
- none
◆ transfer() [2/3]
void SPIClass::transfer | ( | void * | _buf, |
size_t | _count | ||
) |
Transfer several bytes. Only one buffer used to send and receive data.
- Parameters
-
[in] _buf pointer to the bytes to send. The bytes received are copy in this buffer. [in] _count number of bytes to send/receive
- Returns
- none
- Note
- none
◆ transfer() [3/3]
void SPIClass::transfer | ( | void * | _bufout, |
void * | _bufin, | ||
size_t | _count | ||
) |
Transfer several bytes. One buffer contains the data to send and another one will contains the data received.
- Parameters
-
[in] _bufout pointer to the bytes to send. [in] _bufin pointer to the bytes received. [in] _count number of bytes to send/receive
- Returns
- none
- Note
- none
◆ transfer16()
uint16_t SPIClass::transfer16 | ( | uint16_t | data | ) |
Transfer two bytes on the SPI bus in 16 bits format.
- Parameters
-
[in] data bytes to send.
- Returns
- bytes received from the slave in 16 bits format.
- Note
- none
◆ transferRead()
void SPIClass::transferRead | ( | void * | _buf, |
size_t | _count | ||
) |
receive several bytes.
- Parameters
-
[in] _buf pointer to the bytes to received. [in] _count number of bytes to received
- Returns
- none
- Note
- none
◆ transferWrite()
void SPIClass::transferWrite | ( | void * | _buf, |
size_t | _count | ||
) |
send several bytes.
- Parameters
-
[in] _buf pointer to the bytes to send. [in] _count number of bytes to send
- Returns
- none
- Note
- none
Generated by
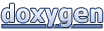