![]() |
W60X_Arduino
|
WiFiUDP Class Reference
Inherits UDP, and SList< WiFiUDP >.
Public Member Functions | |
WiFiUDP () | |
This function is constructor, it's used to creates a named instance of the WiFiUDP class that can send and receive UDP messages. More... | |
WiFiUDP (const WiFiUDP &other) | |
WiFiUDP & | operator= (const WiFiUDP &rhs) |
~WiFiUDP () | |
This function is deconstructor, it's used to release WiFiUDP class. More... | |
operator bool () const | |
virtual uint8_t | begin (uint16_t port) |
This function is used to initializes the WiFiUDP library and network settings, Starts UDP socket, listening at local port. More... | |
virtual void | stop () |
This function is used to disconnect from the server. Release any resource being used during the UDP session. More... | |
uint8_t | beginMulticast (IPAddress interfaceAddr, IPAddress multicast, uint16_t port) |
This function is used to join a multicast group and listen on the given port. More... | |
virtual int | beginPacket (IPAddress ip, uint16_t port) |
This function is used to starts a connection to write UDP data to the remote connection. More... | |
virtual int | beginPacket (const char *host, uint16_t port) |
This function is used to starts a connection to write UDP data to the remote connection. More... | |
virtual int | beginPacketMulticast (IPAddress multicastAddress, uint16_t port, IPAddress interfaceAddress, int ttl=1) |
This function is used to start building up a packet to send to the multicast address. More... | |
virtual int | endPacket () |
This function is used to called after writing UDP data to the remote connection. It finishes off the packet and send it. More... | |
virtual size_t | write (uint8_t) |
This function is used to writes UDP data to the remote connection. More... | |
virtual size_t | write (const uint8_t *buffer, size_t size) |
This function is used to writes UDP data to the remote connection. More... | |
virtual int | parsePacket () |
It starts processing the next available incoming packet, checks for the presence of a UDP packet, and reports the size. More... | |
virtual int | available () |
Get the number of bytes (characters) available for reading from the buffer. This is is data that's already arrived. More... | |
virtual int | read () |
Reads UDP data from the specified buffer. If no arguments are given, it will return the next character in the buffer. More... | |
virtual int | read (unsigned char *buffer, size_t len) |
Reads UDP data from the specified buffer. If no arguments are given, it will return the next character in the buffer. More... | |
virtual int | read (char *buffer, size_t len) |
Reads UDP data from the specified buffer. If no arguments are given, it will return the next character in the buffer. More... | |
virtual int | peek () |
Read a byte from the file without advancing to the next one. That is, successive calls to peek() will return the same value, as will the next call to read(). More... | |
virtual void | flush () |
Discard any bytes that have been written to the client but not yet read. More... | |
virtual IPAddress | remoteIP () |
This function is used to gets the IP address of the remote connection. More... | |
virtual uint16_t | remotePort () |
This function is used to gets the port of the remote UDP connection. More... | |
IPAddress | destinationIP () |
This function is used to distinguish multicast and ordinary packets. More... | |
uint16_t | localPort () |
This function is used to gets the port of the local UDP connection. More... | |
virtual size_t | write (uint8_t)=0 |
This pure virtual function is used to define the operation that writes binary data. More... | |
virtual size_t | write (const uint8_t *buffer, size_t size) |
This function is used to write buffer to the interface defined by the object. More... | |
size_t | write (const char *str) |
This function is used to write buffer to the interface defined by the object. More... | |
size_t | write (const char *buffer, size_t size) |
This function is used to write buffer to the interface defined by the object. More... | |
![]() | |
void | setTimeout (unsigned long timeout) |
setTimeout() sets the maximum milliseconds to wait for stream data, it defaults to 1000 milliseconds. This function is part of the Stream class, and is called by any class that inherits from it (Wire, Serial, etc). See the Stream class main page for more information. More... | |
bool | find (const char *target) |
find() reads data from the stream until the target string of given length is found The function returns true if target string is found, false if timed out. More... | |
bool | find (uint8_t *target) |
find() reads data from the stream until the target string of given length is found The function returns true if target string is found, false if timed out. More... | |
bool | find (const char *target, size_t length) |
find() reads data from the stream until the target string of given length is found The function returns true if target string is found, false if timed out. More... | |
bool | find (const uint8_t *target, size_t length) |
find() reads data from the stream until the target string of given length is found The function returns true if target string is found, false if timed out. More... | |
bool | find (char target) |
find() reads data from the stream until the target string of given length is found The function returns true if target string is found, false if timed out. More... | |
bool | findUntil (const char *target, const char *terminator) |
findUntil() reads data from the stream until the target string of given length or terminator string is found. More... | |
bool | findUntil (const uint8_t *target, const char *terminator) |
findUntil() reads data from the stream until the target string of given length or terminator string is found. More... | |
bool | findUntil (const char *target, size_t targetLen, const char *terminate, size_t termLen) |
findUntil() reads data from the stream until the target string of given length or terminator string is found. More... | |
bool | findUntil (const uint8_t *target, size_t targetLen, const char *terminate, size_t termLen) |
findUntil() reads data from the stream until the target string of given length or terminator string is found. More... | |
long | parseInt () |
parseInt() returns the first valid (long) integer number from the serial buffer. Characters that are not integers (or the minus sign) are skipped. More... | |
float | parseFloat () |
parseFloat() returns the first valid floating point number from the current position. Initial characters that are not digits (or the minus sign) are skipped. parseFloat() is terminated by the first character that is not a floating point number. More... | |
virtual size_t | readBytes (char *buffer, size_t length) |
readBytes() read characters from a stream into a buffer. The function terminates if the determined length has been read, or it times out (see setTimeout()). More... | |
virtual size_t | readBytes (uint8_t *buffer, size_t length) |
readBytes() read characters from a stream into a buffer. The function terminates if the determined length has been read, or it times out (see setTimeout()). More... | |
size_t | readBytesUntil (char terminator, char *buffer, size_t length) |
readBytesUntil() reads characters from a stream into a buffer. The function terminates if the terminator character is detected, the determined length has been read, or it times out (see setTimeout()). More... | |
size_t | readBytesUntil (char terminator, uint8_t *buffer, size_t length) |
readBytesUntil() reads characters from a stream into a buffer. The function terminates if the terminator character is detected, the determined length has been read, or it times out (see setTimeout()). More... | |
String | readString () |
readString() reads characters from a stream into a string. The function terminates if it times out (see setTimeout()). More... | |
String | readStringUntil (char terminator) |
readStringUntil() reads characters from a stream into a string. The function terminates if the terminator character is detected or it times out (see setTimeout()). More... | |
![]() | |
int | getWriteError () |
This function is used to get write error number. More... | |
void | clearWriteError () |
This function is used to clear write error number. More... | |
size_t | write (const char *str) |
This function is used to write buffer to the interface defined by the object. More... | |
size_t | write (const char *buffer, size_t size) |
This function is used to write buffer to the interface defined by the object. More... | |
size_t | print (const String &) |
This function is used to print buffer to the interface defined by the object. More... | |
size_t | print (const char []) |
This function is used to print buffer to the interface defined by the object. More... | |
size_t | print (char) |
This function is used to print buffer to the interface defined by the object. More... | |
size_t | print (unsigned char, int=DEC) |
This function is used to print target to the interface defined by the object. More... | |
size_t | print (int, int=DEC) |
This function is used to print target to the interface defined by the object. More... | |
size_t | print (unsigned int, int=DEC) |
This function is used to print target to the interface defined by the object. More... | |
size_t | print (long, int=DEC) |
This function is used to print target to the interface defined by the object. More... | |
size_t | print (unsigned long, int=DEC) |
This function is used to print target to the interface defined by the object. More... | |
size_t | print (double, int=BIN) |
This function is used to print target to the interface defined by the object. More... | |
size_t | print (const Printable &) |
This function is used to print target to the interface defined by the object. More... | |
size_t | println (void) |
This function is used to print target to the interface defined by the object with carriage ret ('') and new line (' '). More... | |
size_t | println (const String &s) |
This function is used to print target to the interface defined by the object with carriage ret ('') and new line (' '). More... | |
size_t | println (const char []) |
This function is used to print target to the interface defined by the object with carriage ret ('') and new line (' '). More... | |
size_t | println (char) |
This function is used to print target to the interface defined by the object with carriage ret ('') and new line (' '). More... | |
size_t | println (unsigned char, int=DEC) |
This function is used to print target to the interface defined by the object with carriage ret ('') and new line (' '). More... | |
size_t | println (int, int=DEC) |
This function is used to print target to the interface defined by the object with carriage ret ('') and new line (' '). More... | |
size_t | println (unsigned int, int=DEC) |
This function is used to print target to the interface defined by the object with carriage ret ('') and new line (' '). More... | |
size_t | println (long, int=DEC) |
This function is used to print target to the interface defined by the object with carriage ret ('') and new line (' '). More... | |
size_t | println (unsigned long, int=DEC) |
This function is used to print target to the interface defined by the object with carriage ret ('') and new line (' '). More... | |
size_t | println (double, int=BIN) |
This function is used to print target to the interface defined by the object with carriage ret ('') and new line (' '). More... | |
size_t | println (const Printable &) |
This function is used to print target to the interface defined by the object with carriage ret ('') and new line (' '). More... | |
Static Public Member Functions | |
static void | stopAll () |
This function is used to stop all WiFiUDP session. More... | |
static void | stopAllExcept (WiFiUDP *exC) |
This function is used to stop all WiFiUDP session without exC. More... | |
Additional Inherited Members | |
![]() | |
int | timedRead () |
int | timedPeek () |
int | peekNextDigit () |
long | parseInt (char skipChar) |
float | parseFloat (char skipChar) |
![]() | |
void | setWriteError (int err=1) |
![]() | |
static void | _add (WiFiUDP *self) |
static void | _remove (WiFiUDP *self) |
![]() | |
unsigned long | _timeout |
unsigned long | _startMillis |
![]() | |
WiFiUDP * | _next |
![]() | |
static WiFiUDP * | _s_first |
Member Function Documentation
◆ write() [1/4]
|
inline |
This function is used to write buffer to the interface defined by the object.
- Parameters
-
[in] str Specify the buffer of string.
- Returns
- The length of write successfully.
- Note
◆ write() [2/4]
virtual size_t Print::write |
This pure virtual function is used to define the operation that writes binary data.
- Parameters
-
[in] val a value to send as a single byte
- Returns
- The length of write successfully (1 byte).
- Note
◆ write() [3/4]
size_t Print::write |
This function is used to write buffer to the interface defined by the object.
- Parameters
-
[in] buffer Specify the buffer. [in] size Specify the size.
- Returns
- The length of write successfully.
- Note
- Parameters
-
[in] buffer Specify the buffer. [in] size Specify the size.
- Returns
- The length of write successfully (1 byte).
- Note
◆ write() [4/4]
|
inline |
This function is used to write buffer to the interface defined by the object.
- Parameters
-
[in] buffer Specify the buffer of string. [in] size Specify the size.
- Returns
- The length of write successfully.
- Note
Generated by
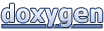