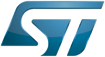

VL53L0X API Specification
1.0.2.4823vl53l0x_platform.h
Go to the documentation of this file.
128 VL53L0X_Error VL53L0X_WriteMulti(VL53L0X_DEV Dev, uint8_t index, uint8_t *pdata, uint32_t count);
139 VL53L0X_Error VL53L0X_ReadMulti(VL53L0X_DEV Dev, uint8_t index, uint8_t *pdata, uint32_t count);
213 VL53L0X_Error VL53L0X_UpdateByte(VL53L0X_DEV Dev, uint8_t index, uint8_t AndData, uint8_t OrData);
230 VL53L0X_Error VL53L0X_PollingDelay(VL53L0X_DEV Dev); /* usually best implemented as a real function */
VL53L0X_Error VL53L0X_UnlockSequenceAccess(VL53L0X_DEV Dev)
Unlock comms interface to serialize all commands to a shared I2C interface for a specific device...
VL53L0X_Error VL53L0X_RdByte(VL53L0X_DEV Dev, uint8_t index, uint8_t *data)
Read single byte register.
VL53L0X_Error VL53L0X_PollingDelay(VL53L0X_DEV Dev)
execute delay in all polling API call
VL53L0X_Error VL53L0X_RdWord(VL53L0X_DEV Dev, uint8_t index, uint16_t *data)
Read word (2byte) register.
VL53L0X_Dev_t * VL53L0X_DEV
Declare the device Handle as a pointer of the structure VL53L0X_Dev_t.
Definition: vl53l0x_platform.h:73
VL53L0X_Error VL53L0X_ReadMulti(VL53L0X_DEV Dev, uint8_t index, uint8_t *pdata, uint32_t count)
Reads the requested number of bytes from the device.
VL53L0X PAL device ST private data structure End user should never access any of these field directl...
Definition: vl53l0x_def.h:433
VL53L0X_Error VL53L0X_WrDWord(VL53L0X_DEV Dev, uint8_t index, uint32_t data)
Write double word (4 byte) register.
Generic PAL device type that does link between API and platform abstraction layer.
Definition: vl53l0x_platform.h:58
VL53L0X_Error VL53L0X_WrByte(VL53L0X_DEV Dev, uint8_t index, uint8_t data)
Write single byte register.
VL53L0X_Error VL53L0X_WrWord(VL53L0X_DEV Dev, uint8_t index, uint16_t data)
Write word register.
VL53L0X_Error VL53L0X_UpdateByte(VL53L0X_DEV Dev, uint8_t index, uint8_t AndData, uint8_t OrData)
Threat safe Update (read/modify/write) single byte register.
VL53L0X_Error VL53L0X_WriteMulti(VL53L0X_DEV Dev, uint8_t index, uint8_t *pdata, uint32_t count)
Writes the supplied byte buffer to the device.
Type definitions for VL53L0X API.
VL53L0X_Error VL53L0X_LockSequenceAccess(VL53L0X_DEV Dev)
Lock comms interface to serialize all commands to a shared I2C interface for a specific device...
platform log function definition
VL53L0X_Error VL53L0X_RdDWord(VL53L0X_DEV Dev, uint8_t index, uint32_t *data)
Read dword (4byte) register.